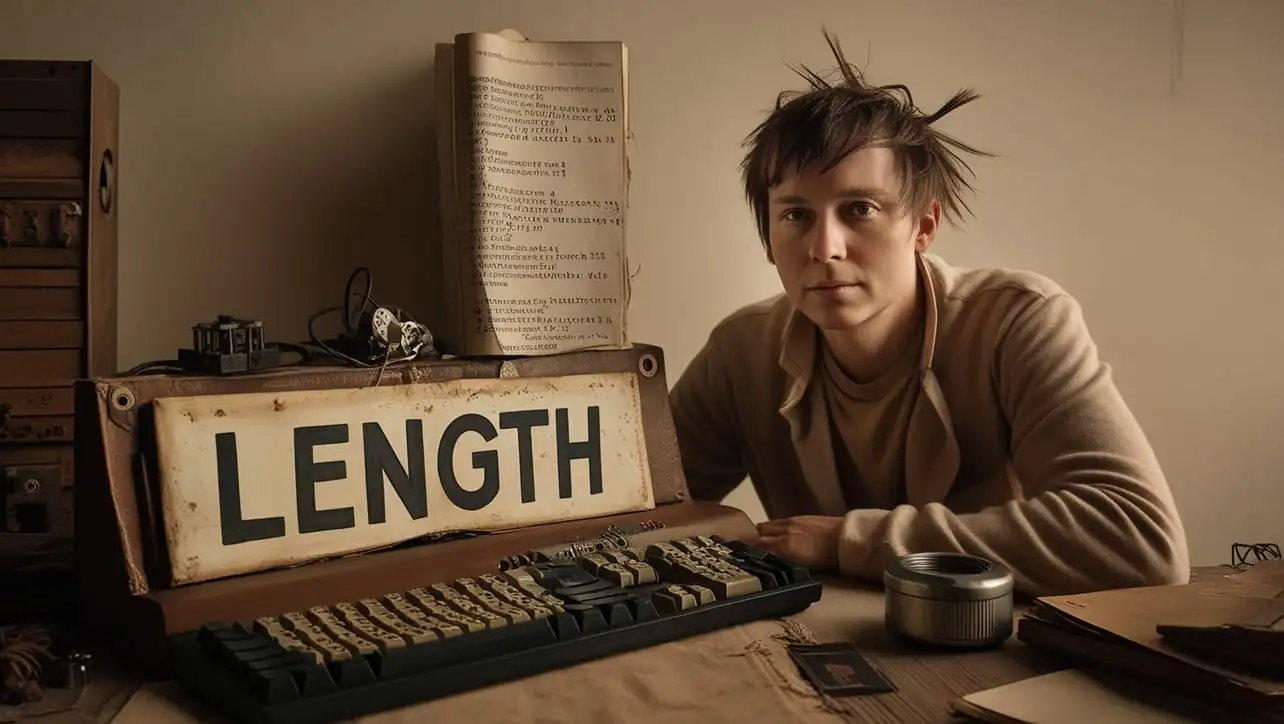
JS Window Methods
JavaScript Window blur() Method

Photo Credit to CodeToFun
🙋 Introduction
The blur()
method in JavaScript is a powerful tool when it comes to managing the focus of browser windows. This method allows you to programmatically remove focus from the current window, providing enhanced control over user interactions.
In this comprehensive guide, we'll explore the blur()
method, its syntax, usage, best practices, and practical examples to help you integrate this functionality seamlessly into your web applications.
🧠 Understanding blur() Method
The blur()
method is part of the Window interface in JavaScript and is used to remove focus from the current window. When applied, it triggers the blur event, which can be useful in various scenarios, such as improving user experience or controlling the focus flow within your web application.
💡 Syntax
The syntax for the blur()
method is straightforward:
window.blur();
- window: The global Window object representing the browser window.
📝 Example
Let's jump into a simple example to demonstrate the application of the blur()
method:
// Applying blur() to remove focus from the window
function removeFocus() {
window.blur();
}
// Execute the function after a certain delay
setTimeout(removeFocus, 2000);
In this example, the removeFocus function is defined to apply blur()
to the window after a delay of 2000 milliseconds (2 seconds).
🏆 Best Practices
When working with the blur()
method, consider the following best practices:
User Interaction:
Ensure that the use of
blur()
aligns with a positive user experience. Abruptly removing focus might not be suitable for all scenarios.example.jsCopied// Confirm with the user before applying blur() const confirmBlur = window.confirm("Remove focus from the window?"); if (confirmBlur) { window.blur(); }
Event Handling:
Leverage the blur event to perform additional actions when the window loses focus.
example.jsCopiedwindow.addEventListener('blur', () => { console.log('Window lost focus!'); // Additional actions can be performed here });
Compatibility Checking:
Verify the compatibility of the
blur()
method in your target browsers.example.jsCopied// Check if blur() is supported if (typeof window.blur === 'function') { // Safe to use blur() window.blur(); } else { console.error('blur() method not supported in this environment.'); }
📚 Use Cases
Creating Smooth Transitions:
The
blur()
method can be employed to create smooth transitions between different views or components within a single-page application.example.jsCopiedfunction transitionToNextPage() { // Execute actions before transitioning // ... // Remove focus from the window to create a seamless transition window.blur(); }
Enhancing Accessibility:
In scenarios where your application requires specific user interactions or attention, using
blur()
can guide users to the desired elements or prompts.example.jsCopied// Display an important message and remove focus from other elements window.alert('Attention: Complete the form below.'); document.getElementById('importantInput').focus();
🎉 Conclusion
The blur()
method provides a valuable tool for managing focus within the browser window.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the blur()
method in your JavaScript projects.
👨💻 Join our Community:
Author
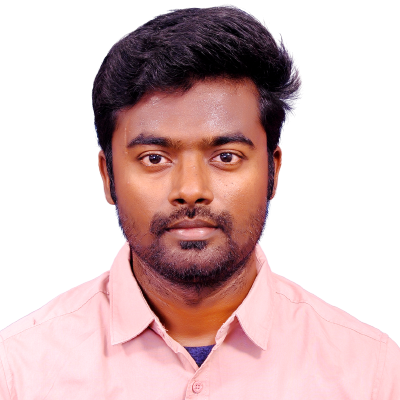
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window blur() Method), please comment here. I will help you immediately.