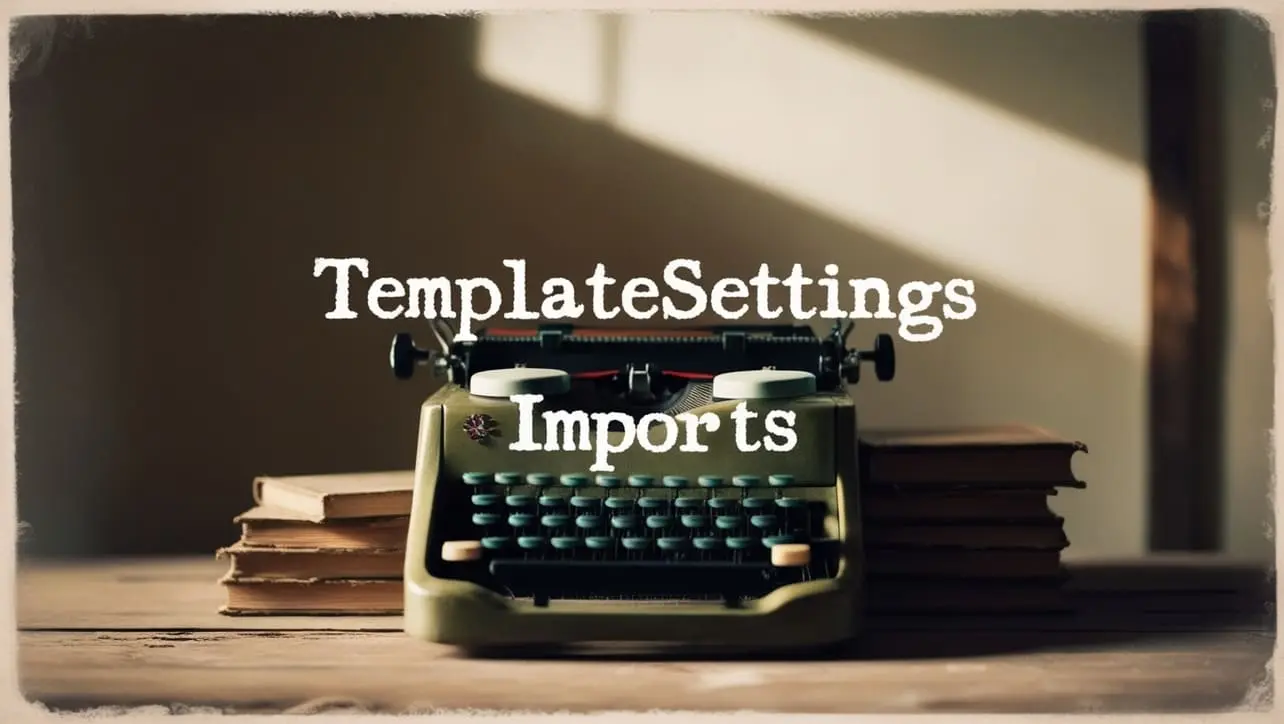
Lodash _.keyBy() Collection Method
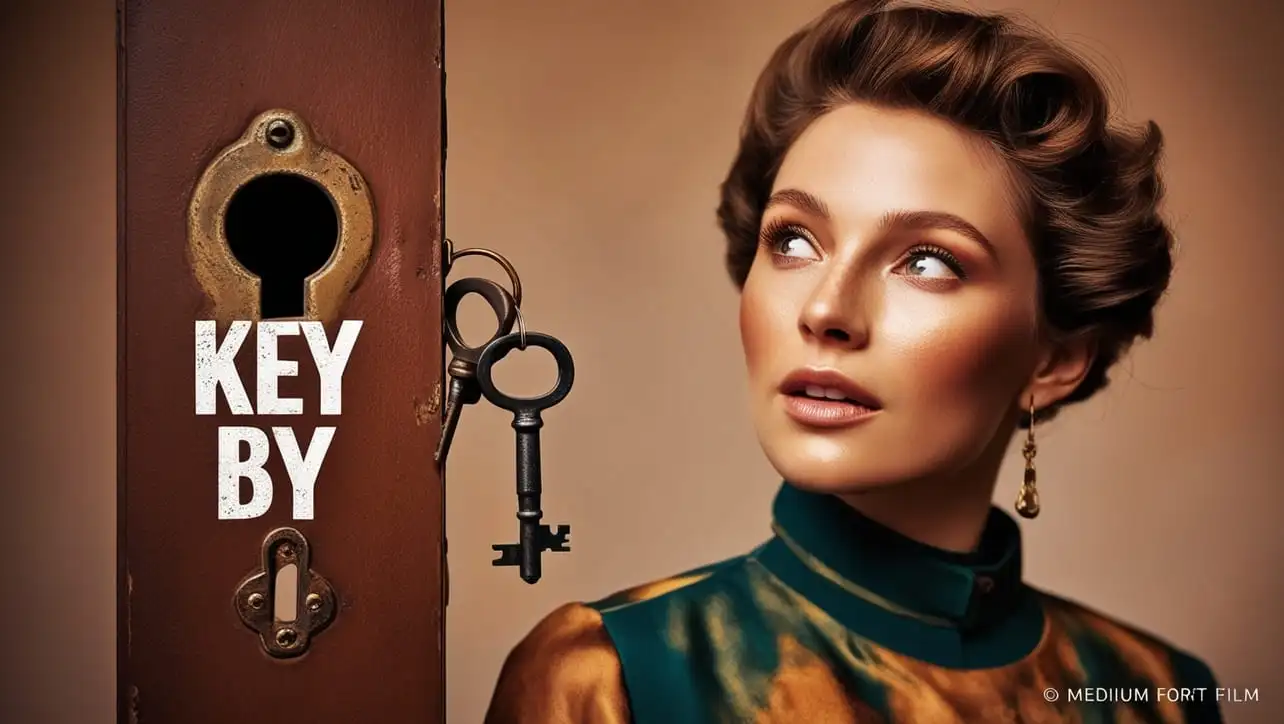
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, efficient data organization is a key aspect of writing clean and maintainable code. Lodash, a widely-used utility library, provides developers with a diverse set of functions, and among them is the _.keyBy()
method. This method proves invaluable when it comes to transforming an array or collection into an object, where keys are determined by a specified property.
Let's explore how _.keyBy()
can enhance your code by simplifying the process of creating key-value pairs.
🧠 Understanding _.keyBy()
The _.keyBy()
method in Lodash is designed for transforming arrays or collections into objects, where each key is determined by a specified property of the elements. This can lead to more efficient data access and manipulation, providing a convenient way to organize information.
💡 Syntax
_.keyBy(collection, [iteratee])
- collection: The array or collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a practical example to illustrate the power of _.keyBy()
:
const _ = require('lodash');
const users = [
{ id: 'user1', name: 'Alice' },
{ id: 'user2', name: 'Bob' },
{ id: 'user3', name: 'Charlie' }
];
const usersById = _.keyBy(users, 'id');
console.log(usersById);
// Output: { 'user1': { id: 'user1', name: 'Alice' }, 'user2': { id: 'user2', name: 'Bob' }, 'user3': { id: 'user3', name: 'Charlie' } }
In this example, the users array is transformed into an object usersById where each user is accessed by their id.
🏆 Best Practices
Choose a Unique Identifier:
Ensure that the property used as the key (iteratee) is unique within the collection. Using non-unique keys may overwrite values, leading to unexpected behavior.
example.jsCopiedconst nonUniqueData = [ { code: 'A', value: 'Apple' }, { code: 'B', value: 'Banana' }, { code: 'A', value: 'Apricot' } ]; const keyedData = _.keyBy(nonUniqueData, 'code'); console.log(keyedData); // Output: { 'A': { code: 'A', value: 'Apricot' }, 'B': { code: 'B', value: 'Banana' } }
Use a Function for Complex Keys:
When dealing with complex keys or when the key needs to be derived from multiple properties, use a function as the iteratee parameter.
example.jsCopiedconst complexData = [ { id: 1, category: 'fruit', name: 'Apple' }, { id: 2, category: 'fruit', name: 'Banana' }, { id: 3, category: 'vegetable', name: 'Carrot' } ]; const keyedData = _.keyBy(complexData, item => `${item.category}-${item.id}`); console.log(keyedData); // Output: { 'fruit-1': { id: 1, category: 'fruit', name: 'Apple' }, 'fruit-2': { id: 2, category: 'fruit', name: 'Banana' }, 'vegetable-3': { id: 3, category: 'vegetable', name: 'Carrot' } }
Handle Empty Collections:
Consider scenarios where the input collection might be empty. Implement appropriate checks to handle such cases.
example.jsCopiedconst emptyCollection = []; const keyedData = _.keyBy(emptyCollection, 'id'); console.log(keyedData); // Output: {}
📚 Use Cases
Quick Data Lookup:
_.keyBy()
is particularly useful when you need to quickly look up and access elements based on a specific property.example.jsCopiedconst books = [ { isbn: '978-0-7475-3269-9', title: 'Harry Potter and the Philosopher\'s Stone' }, { isbn: '978-0-7475-3849-3', title: 'Harry Potter and the Chamber of Secrets' }, { isbn: '978-0-7475-4215-5', title: 'Harry Potter and the Prisoner of Azkaban' } ]; const booksByISBN = _.keyBy(books, 'isbn'); console.log(booksByISBN['978-0-7475-3269-9']); // Output: { isbn: '978-0-7475-3269-9', title: 'Harry Potter and the Philosopher\'s Stone' }
Grouping Data:
Use
_.keyBy()
to efficiently group data based on a specific property.example.jsCopiedconst transactions = [ { transactionId: 'txn1', amount: 50 }, { transactionId: 'txn2', amount: 75 }, { transactionId: 'txn3', amount: 50 } ]; const transactionsByAmount = _.keyBy(transactions, 'amount'); console.log(transactionsByAmount); // Output: { '50': { transactionId: 'txn3', amount: 50 }, '75': { transactionId: 'txn2', amount: 75 } }
Simplifying Data Processing:
When working with datasets where quick access to specific elements is crucial,
_.keyBy()
simplifies the process of transforming arrays into objects.example.jsCopiedconst users = /* ...fetch users from a data source... */; const usersById = _.keyBy(users, 'id'); // Now, quick access to a user by ID is straightforward const userIdToLookup = 'user2'; const user = usersById[userIdToLookup]; console.log(user);
🎉 Conclusion
The _.keyBy()
method in Lodash serves as a versatile tool for transforming arrays or collections into objects, facilitating efficient data organization and access. Whether you're creating key-value pairs for quick lookups or grouping data based on specific properties, _.keyBy()
proves to be a valuable asset in your JavaScript development toolkit.
Explore the capabilities of _.keyBy()
and elevate your code by enhancing data organization and access in a clean and concise manner!
👨💻 Join our Community:
Author
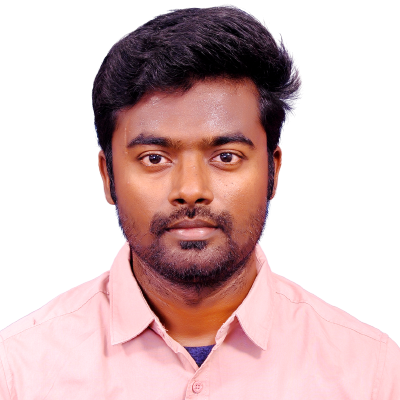
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
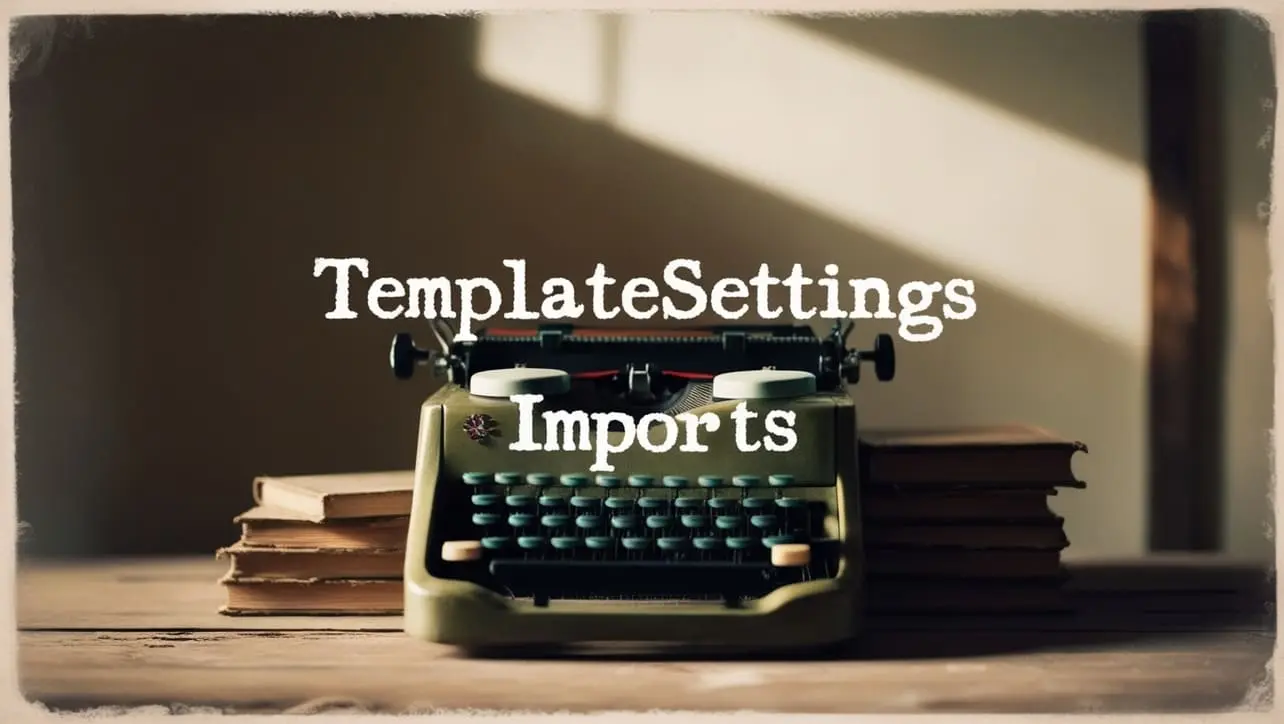
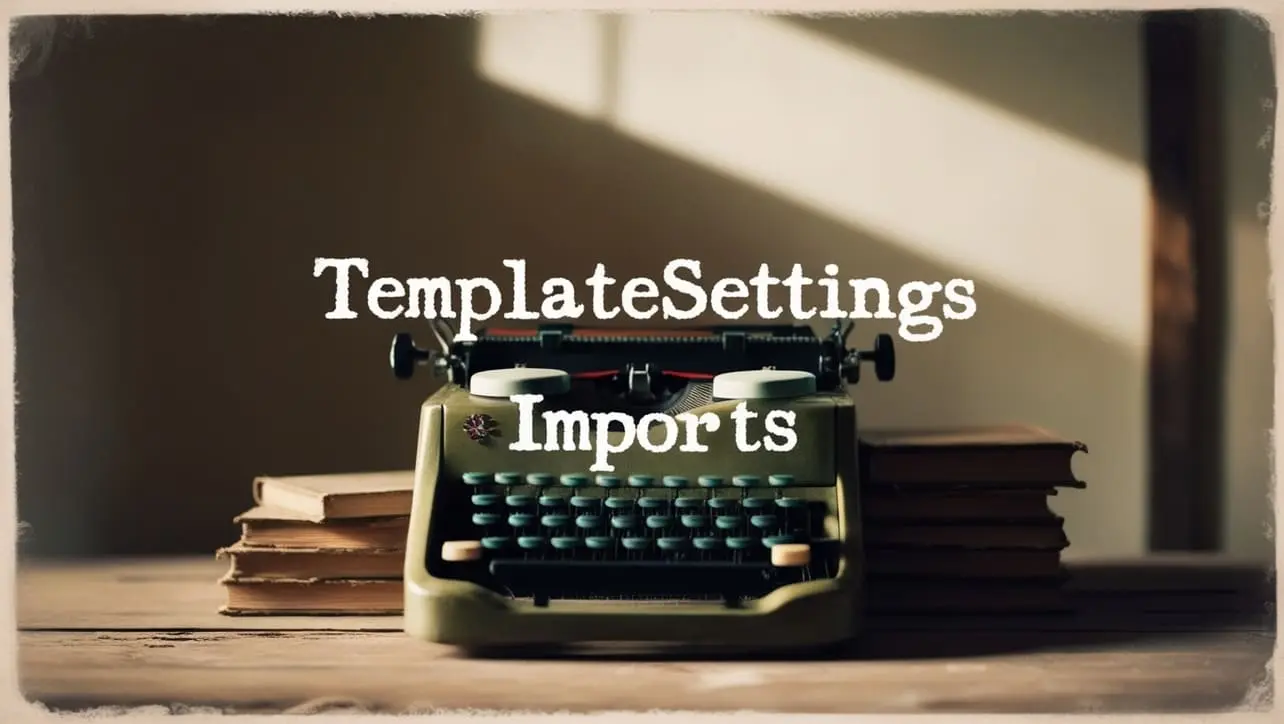
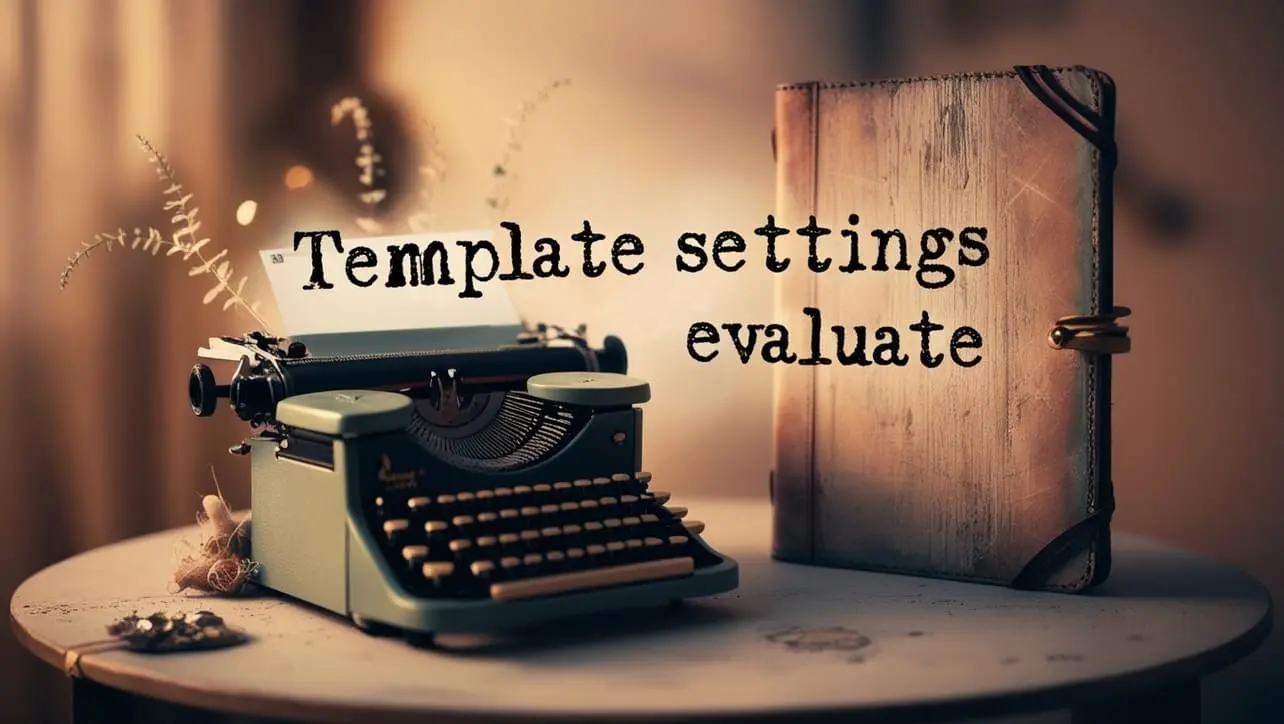
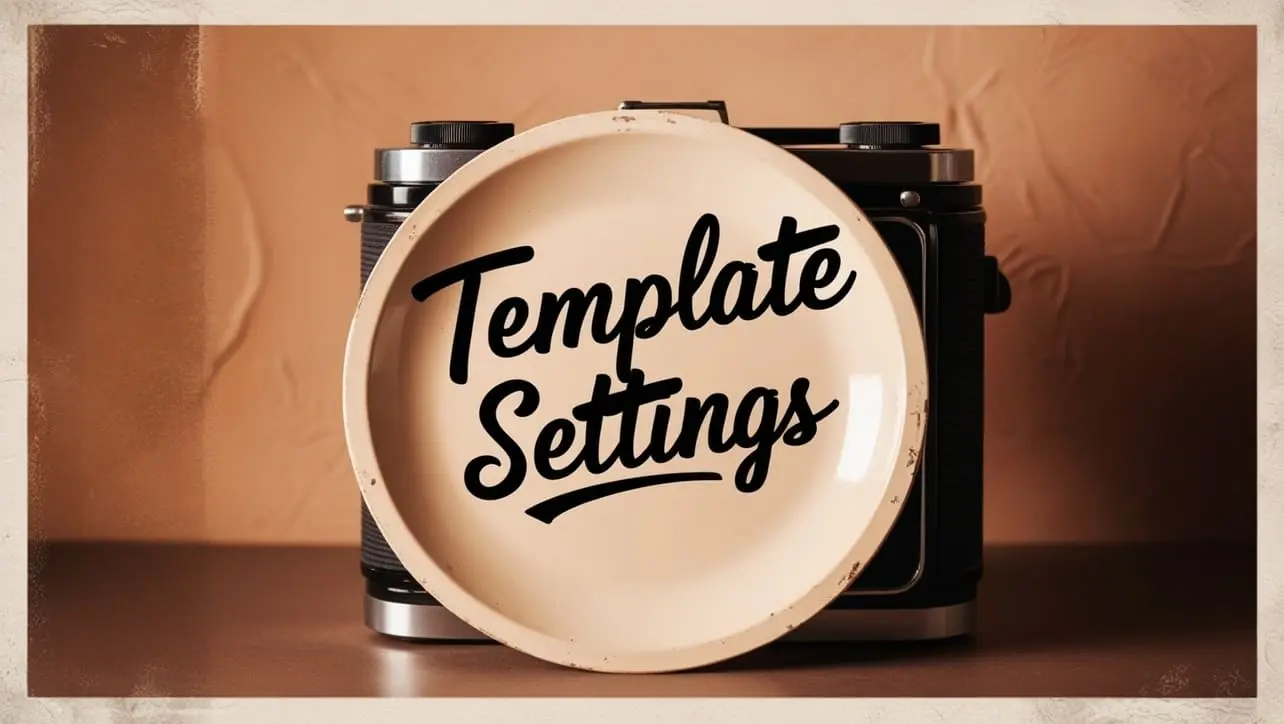
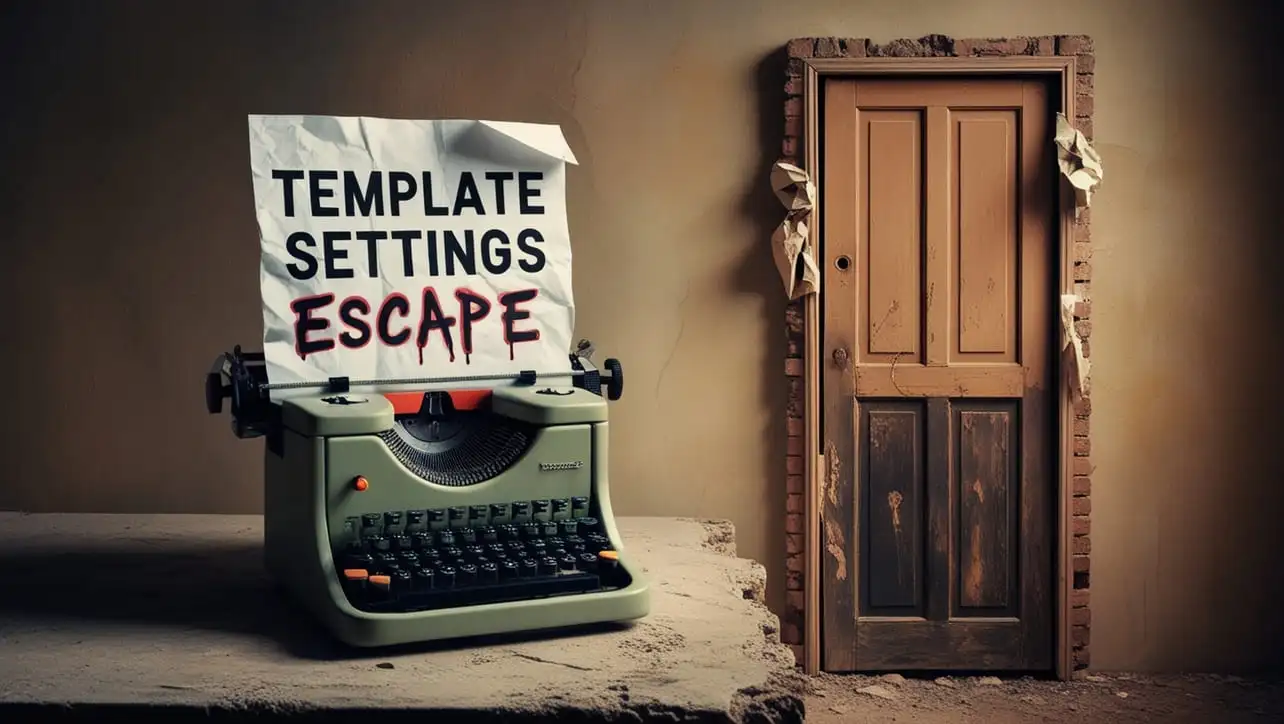
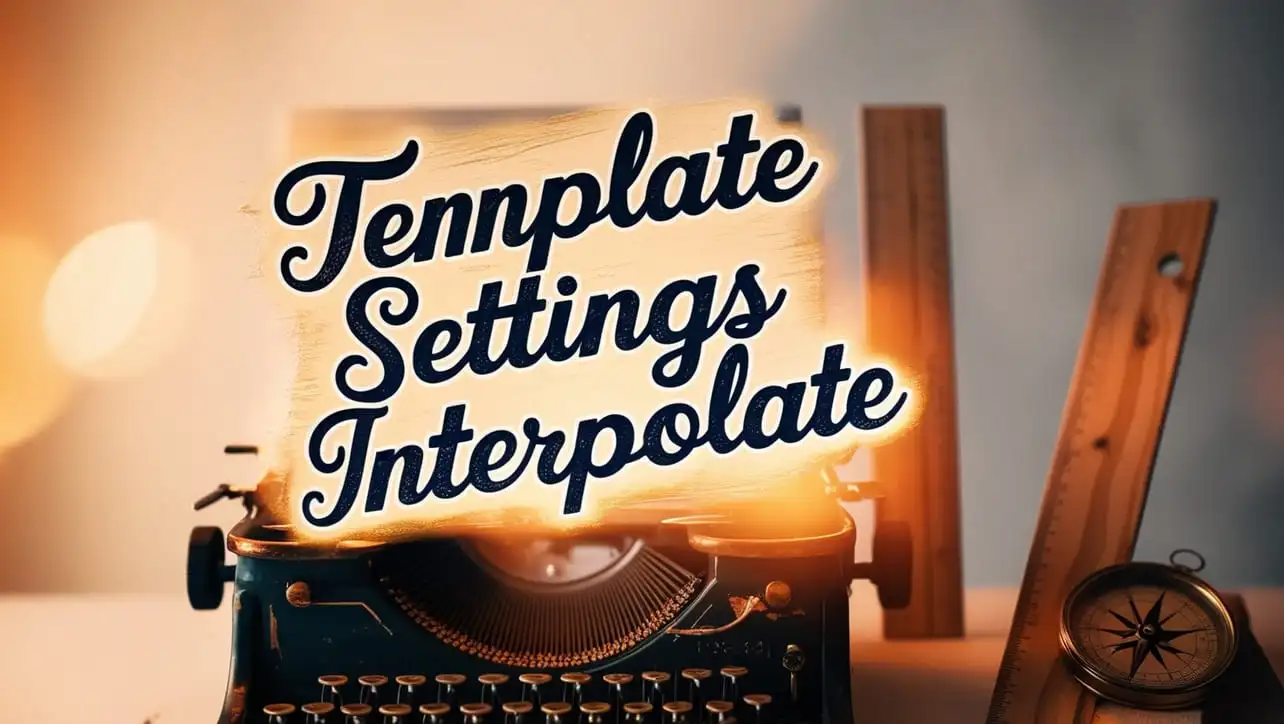
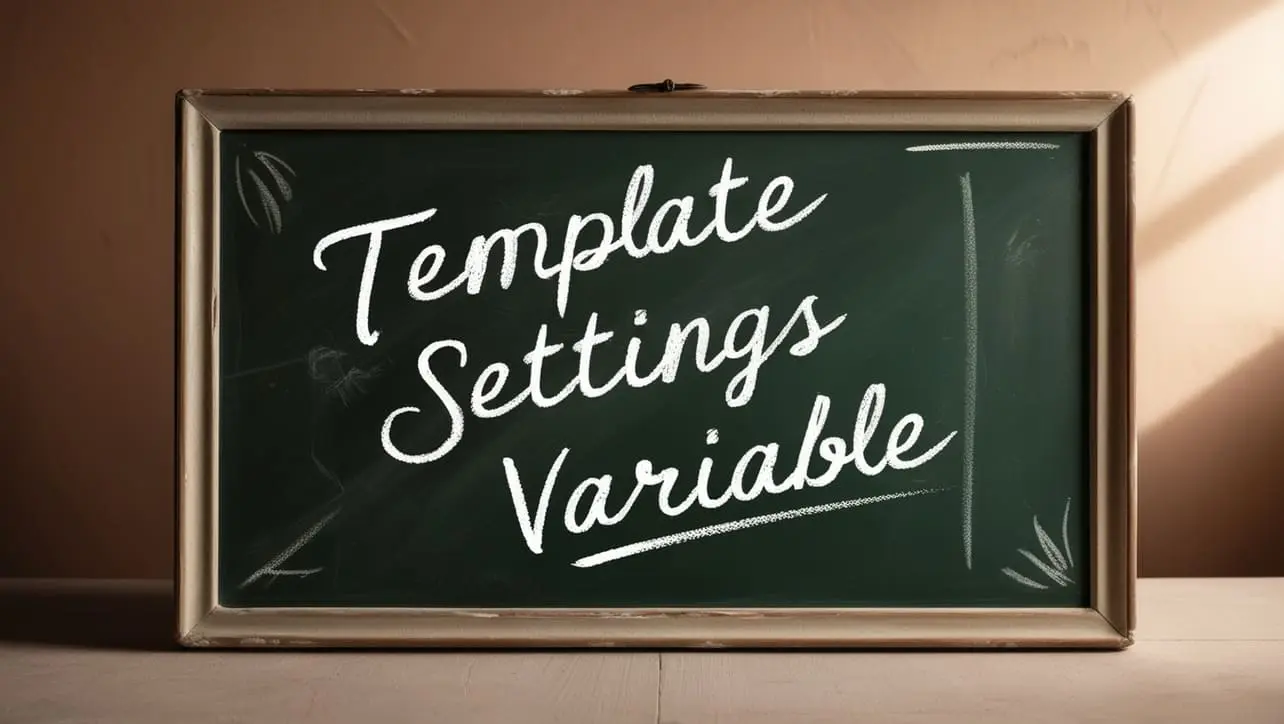
If you have any doubts regarding this article (Lodash _.keyBy() Collection Method), please comment here. I will help you immediately.