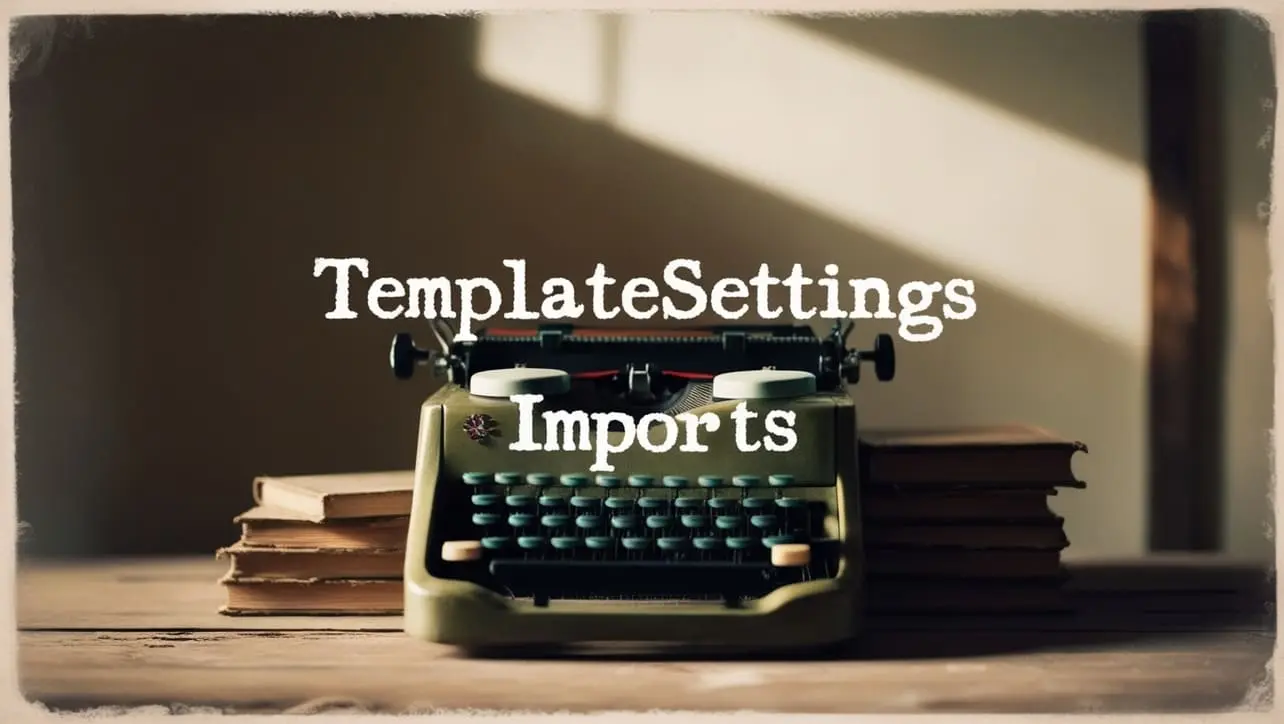
Lodash _.groupBy() Collection Method
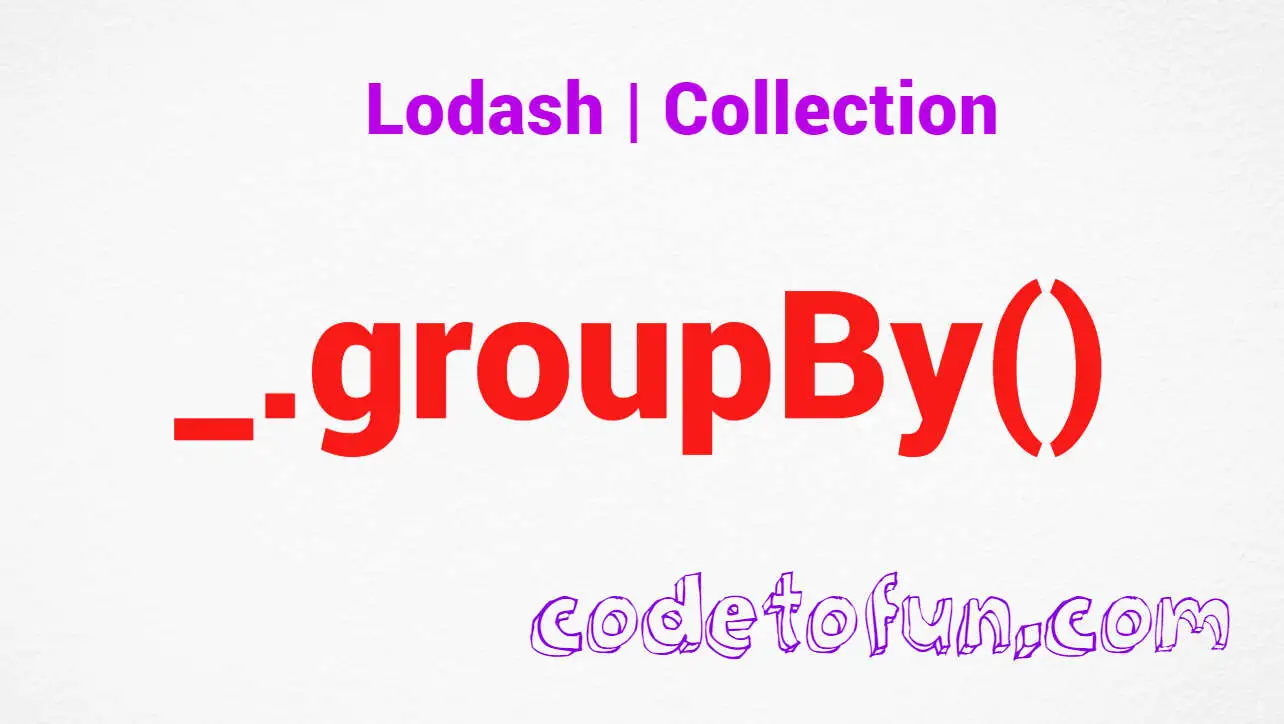
Photo Credit to CodeToFun
🙋 Introduction
Array manipulation in JavaScript often involves grouping elements based on specific criteria, and this is where the Lodash library shines. Among its arsenal of utility functions, the _.groupBy()
method stands out as a powerful tool for grouping elements of an array based on a provided iteratee function.
Whether you're working with complex data structures or aiming to streamline data analysis, _.groupBy()
proves to be an invaluable asset.
🧠 Understanding _.groupBy()
The _.groupBy()
method in Lodash facilitates the grouping of elements in a collection based on a specified criterion. This criterion is defined by an iteratee function, allowing developers to tailor the grouping logic according to their unique requirements. The result is a new object where keys represent the groups and values are arrays containing elements that share the same group.
💡 Syntax
_.groupBy(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a practical example to understand the functionality of _.groupBy()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const groupedByOddEven = _.groupBy(numbers, num => (num % 2 === 0 ? 'even' : 'odd'));
console.log(groupedByOddEven);
/*
Output:
{
odd: [1, 3, 5, 7, 9],
even: [2, 4, 6, 8]
}
*/
In this example, the numbers array is grouped into two categories, 'odd' and 'even', based on the provided iteratee function.
🏆 Best Practices
Define a Clear Iteratee Function:
When using
_.groupBy()
, ensure that the iteratee function clearly defines the grouping logic. A well-defined iteratee function enhances code readability and makes the grouping criteria explicit.example.jsCopiedconst people = [ { name: 'Alice', age: 28 }, { name: 'Bob', age: 32 }, { name: 'Charlie', age: 28 }, ]; const groupedByAge = _.groupBy(people, person => (person.age >= 30 ? 'above30' : 'below30')); console.log(groupedByAge); /* Output: { above30: [ { name: 'Bob', age: 32 }, ], below30: [ { name: 'Alice', age: 28 }, { name: 'Charlie', age: 28 }, ] } */
Handle Edge Cases:
Consider scenarios where the iteratee function might return undefined or null. Implement appropriate handling to ensure accurate grouping and avoid unexpected behavior.
example.jsCopiedconst items = [null, undefined, 1, 2, 3, 4, 5]; const groupedItems = _.groupBy(items, item => (item ? 'valid' : 'invalid')); console.log(groupedItems); /* Output: { invalid: [null, undefined], valid: [1, 2, 3, 4, 5] } */
Utilize Object Keys:
Take advantage of the resulting object's keys for easy access to the groups. This can simplify subsequent operations or data retrieval.
example.jsCopiedconst fruits = ['apple', 'banana', 'orange', 'grape', 'apple']; const groupedFruits = _.groupBy(fruits); console.log(groupedFruits.apple); // Output: ['apple', 'apple']
📚 Use Cases
Categorizing Data:
_.groupBy()
is ideal for categorizing data based on specific attributes. Whether it's grouping products by category or users by region, this method streamlines the categorization process.example.jsCopiedconst products = [ { name: 'Laptop', category: 'Electronics' }, { name: 'Coffee Maker', category: 'Appliances' }, { name: 'Headphones', category: 'Electronics' }, ]; const groupedProducts = _.groupBy(products, 'category'); console.log(groupedProducts); /* Output: { Electronics: [ { name: 'Laptop', category: 'Electronics' }, { name: 'Headphones', category: 'Electronics' }, ], Appliances: [ { name: 'Coffee Maker', category: 'Appliances' }, ] } */
Data Analysis:
For data analysis tasks,
_.groupBy()
simplifies the process of aggregating and organizing data. It is particularly useful for generating summary reports or extracting meaningful insights.example.jsCopiedconst salesData = [ { product: 'Widget', revenue: 1200 }, { product: 'Gadget', revenue: 800 }, { product: 'Widget', revenue: 1500 }, ]; const groupedByProduct = _.groupBy(salesData, 'product'); console.log(groupedByProduct); /* Output: { Widget: [ { product: 'Widget', revenue: 1200 }, { product: 'Widget', revenue: 1500 }, ], Gadget: [ { product: 'Gadget', revenue: 800 }, ] } */
Streamlining UI Rendering:
When rendering dynamic content in a user interface,
_.groupBy()
can simplify the process by organizing data into groups, making it easier to iterate and display information efficiently.example.jsCopiedconst tasks = [ { id: 1, status: 'pending', description: 'Task 1' }, { id: 2, status: 'completed', description: 'Task 2' }, { id: 3, status: 'pending', description: 'Task 3' }, ]; const groupedByStatus = _.groupBy(tasks, 'status'); console.log(groupedByStatus); /* Output: { pending: [ { id: 1, status: 'pending', description: 'Task 1' }, { id: 3, status: 'pending', description: 'Task 3' }, ], completed: [ { id: 2, status: 'completed', description: 'Task 2' }, ] } */
🎉 Conclusion
The _.groupBy()
method in Lodash empowers JavaScript developers to efficiently group elements in a collection based on a specified criterion. Whether you're categorizing data, performing data analysis, or streamlining UI rendering, _.groupBy()
proves to be a versatile and essential tool in your array manipulation toolkit.
Explore the possibilities, enhance code readability, and simplify complex data manipulations with _.groupBy()
!
👨💻 Join our Community:
Author
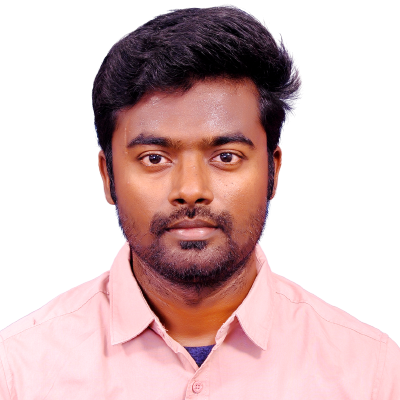
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
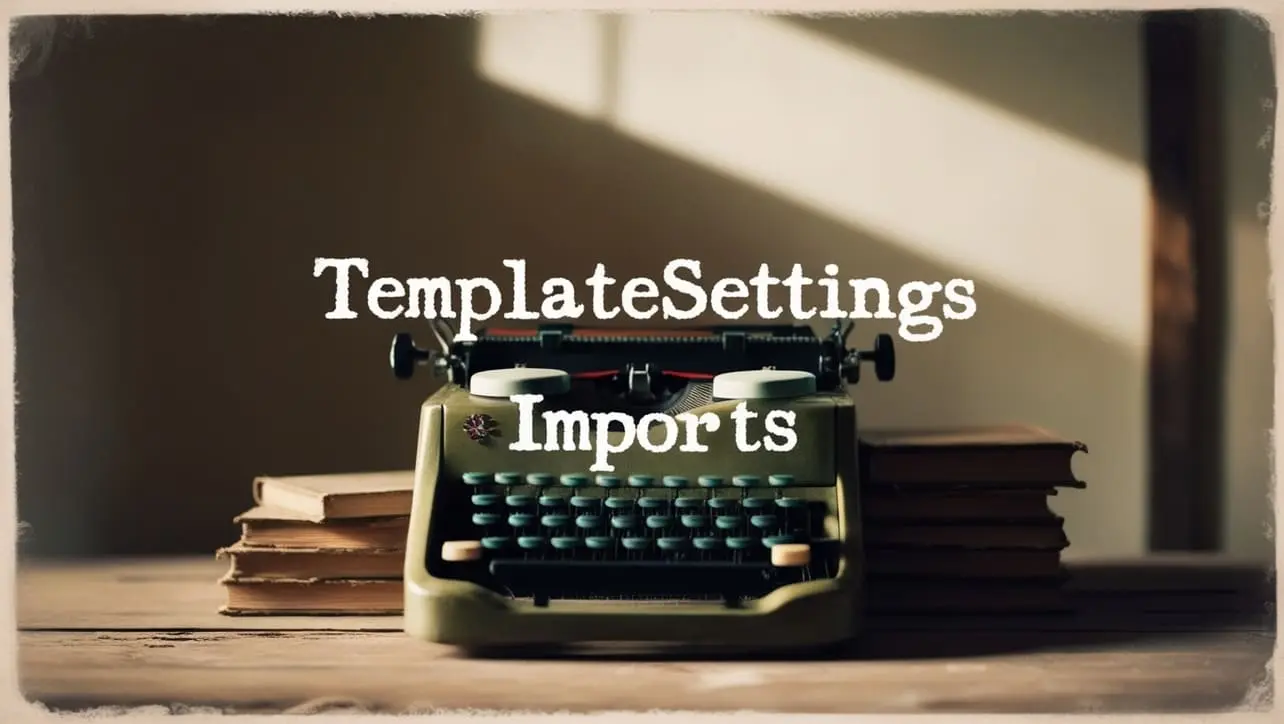
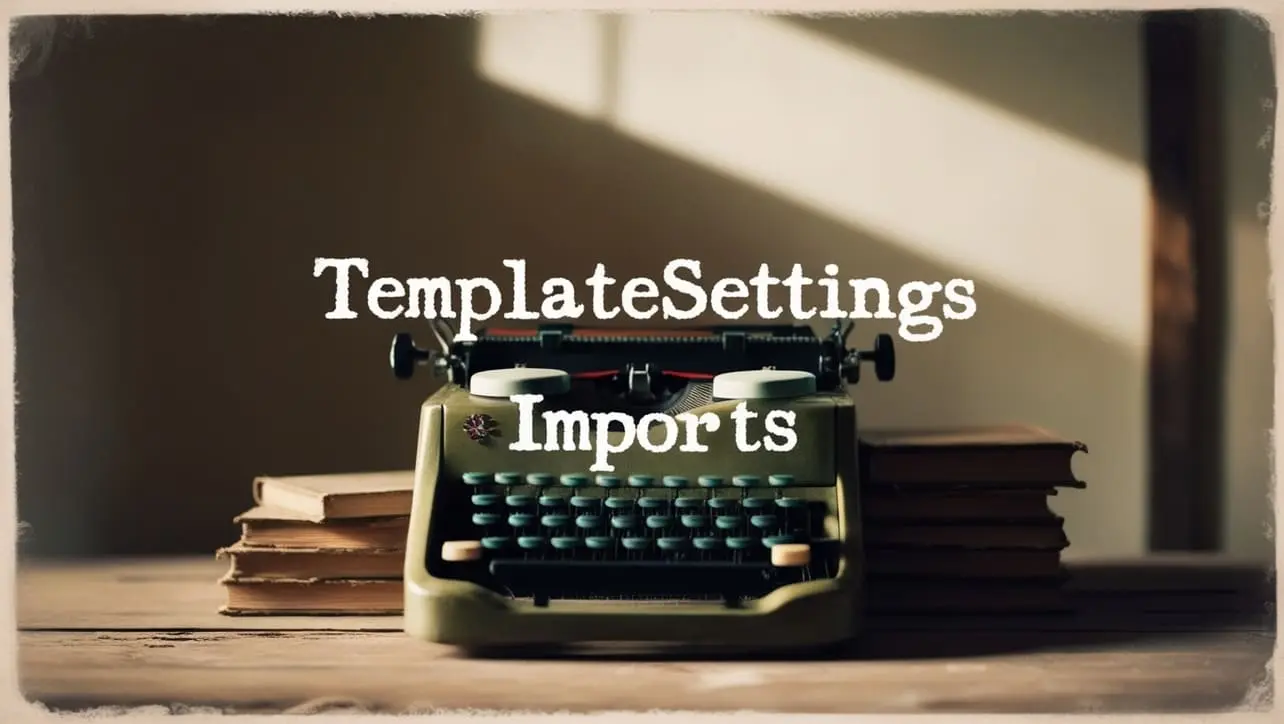
Lodash _.templateSettings.imports Property
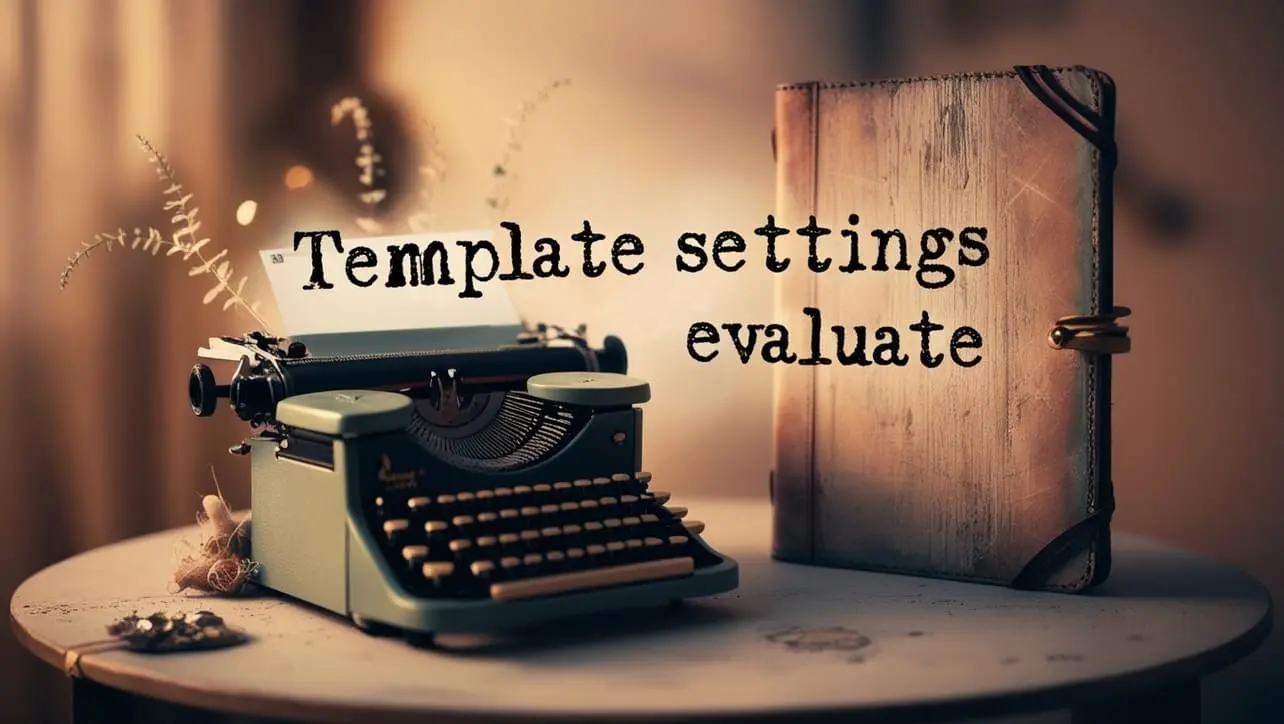
Lodash _.templateSettings.evaluate Property
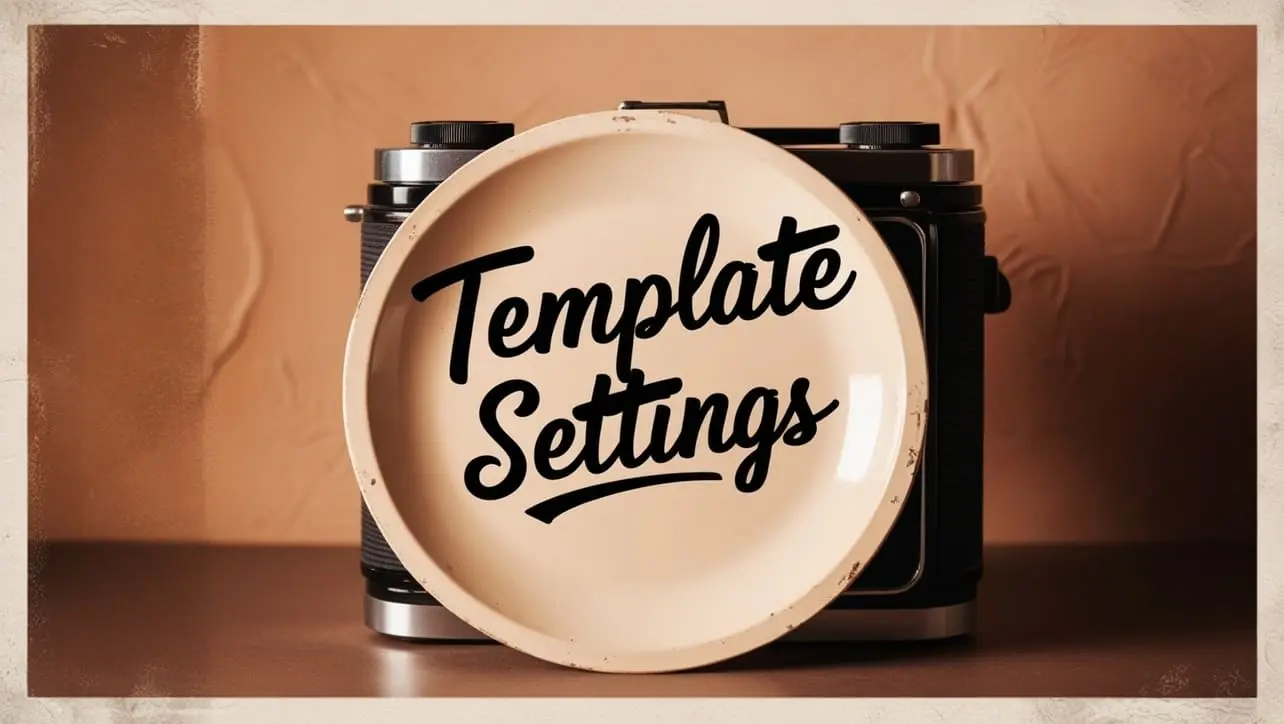
Lodash _.templateSettings Property
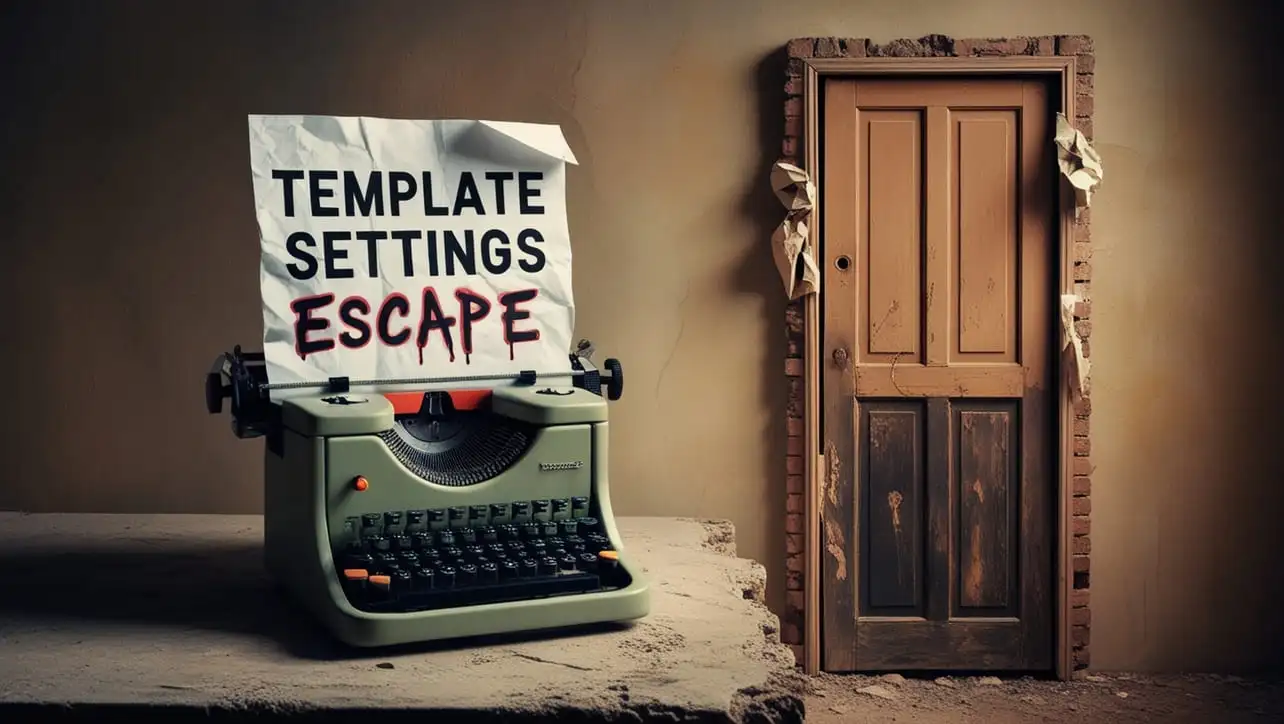
Lodash _.templateSettings.escape Property
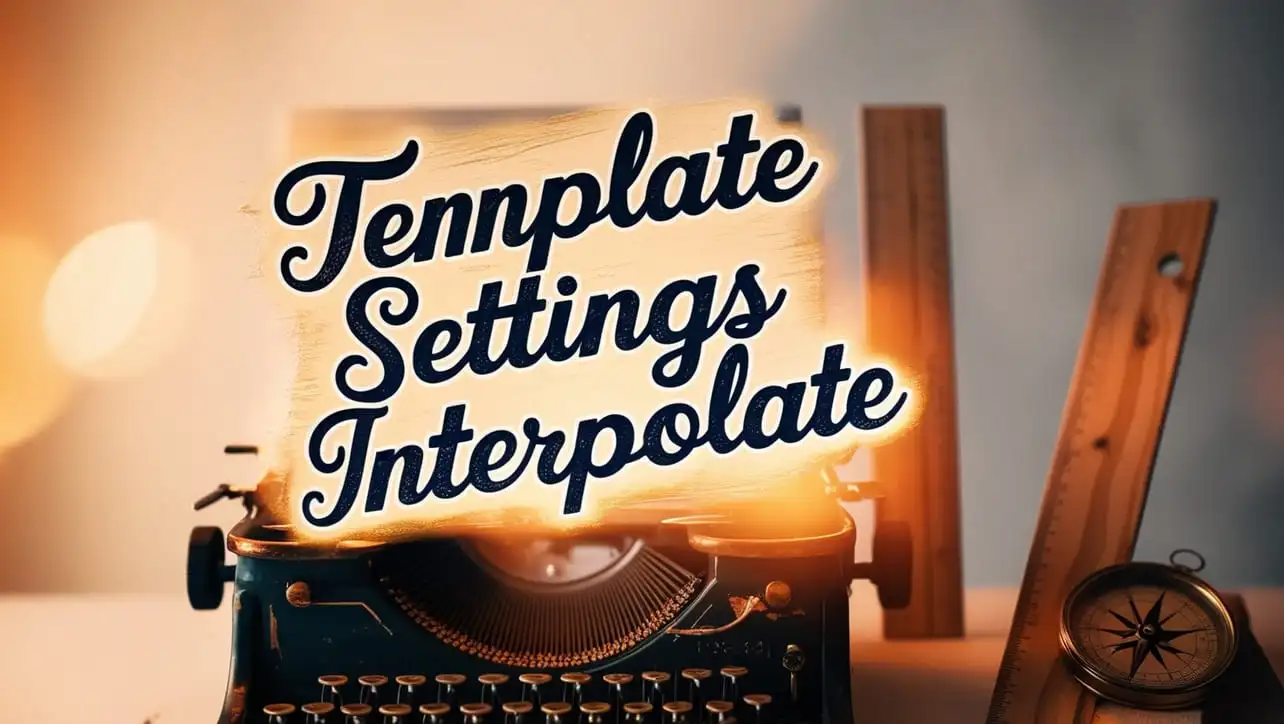
Lodash _.templateSettings.interpolate Property
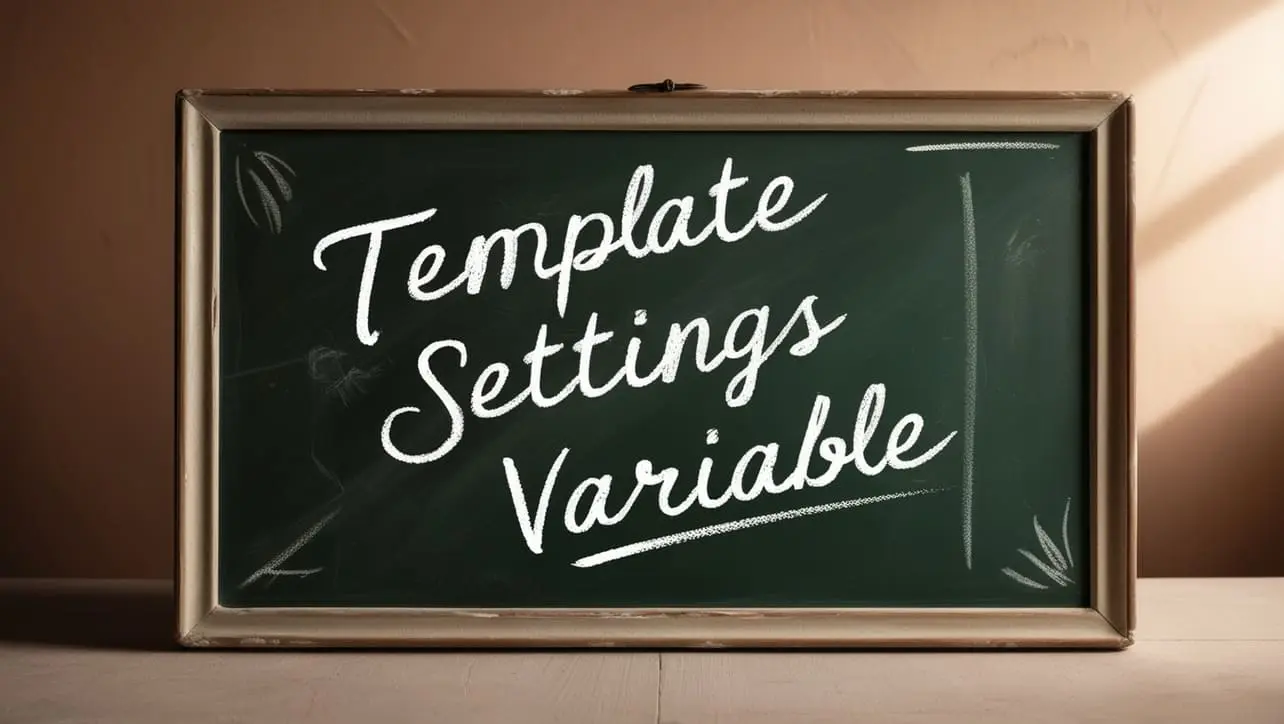
If you have any doubts regarding this article (Lodash _.groupBy() Collection Method), please comment here. I will help you immediately.