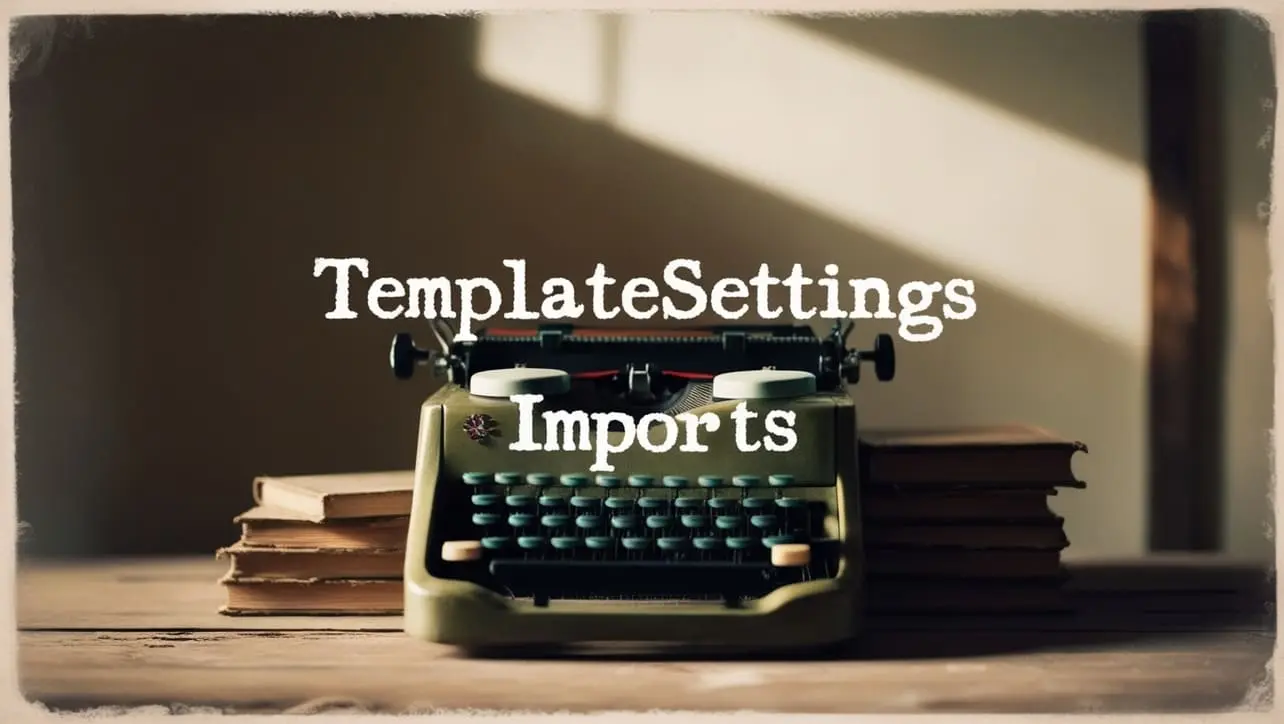
Lodash _.flatMapDepth() Collection Method
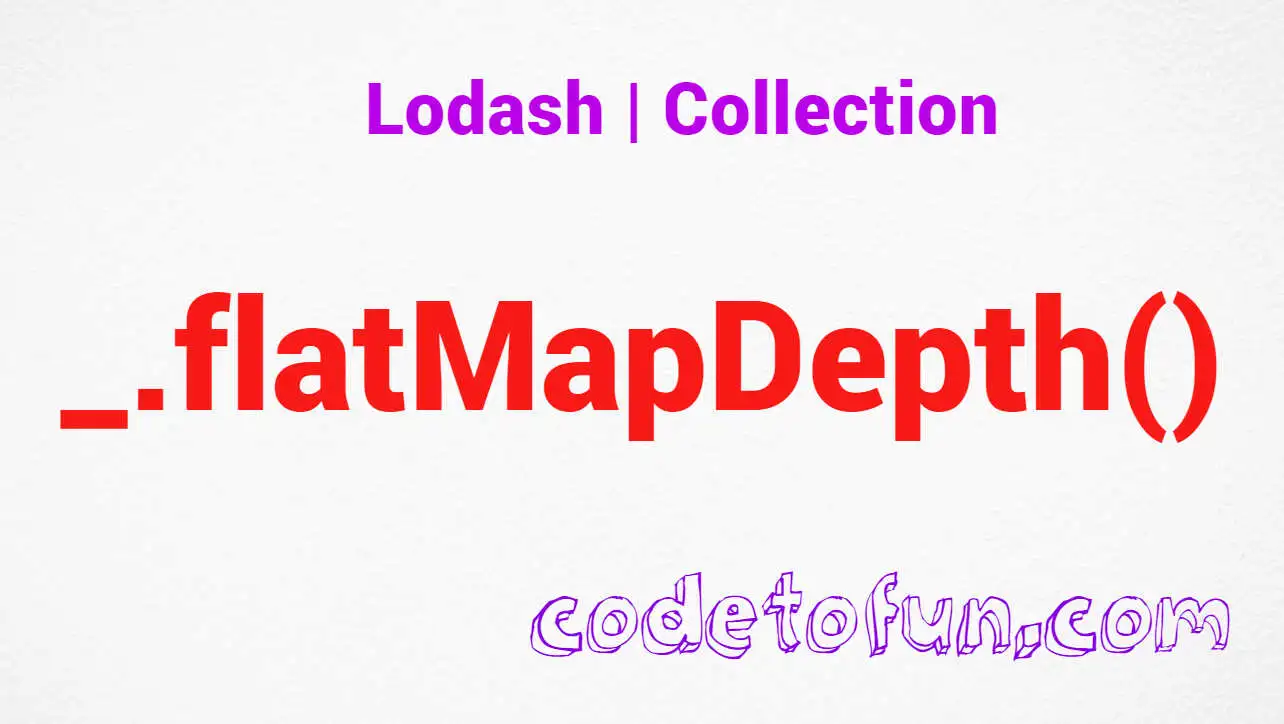
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, efficient manipulation and transformation of data within collections are essential tasks. Lodash, a comprehensive utility library, provides a wealth of functions, and among them is the _.flatMapDepth()
method.
This powerful tool simplifies the process of flattening and mapping nested arrays within a collection, offering flexibility and ease for developers dealing with complex data structures.
🧠 Understanding _.flatMapDepth()
The _.flatMapDepth()
method in Lodash combines the functionalities of flatMap and flattenDepth. It allows you to apply a mapping function to each element of a collection and then flatten the result to a specified depth. This is particularly useful when dealing with nested arrays or deeply nested objects.
💡 Syntax
_.flatMapDepth(collection, iteratee, [depth=1])
- collection: The collection to iterate over.
- iteratee: The function invoked per iteration.
- depth (Optional): The maximum recursion depth (default is 1).
📝 Example
Let's dive into a practical example to illustrate the power of _.flatMapDepth()
:
const _ = require('lodash');
const nestedArray = [1, [2, [3, [4]], 5]];
const flattenedArray = _.flatMapDepth(nestedArray, value => {
if (Array.isArray(value)) {
return value.map(innerValue => innerValue * 2);
}
return value * 2;
}, 2);
console.log(flattenedArray);
// Output: [2, 4, 6, 8, 10]
In this example, the nestedArray is processed by _.flatMapDepth()
, applying a mapping function that doubles each element and flattening the result to a depth of 2.
🏆 Best Practices
Understand the Structure of the Collection:
Before applying
_.flatMapDepth()
, have a clear understanding of the structure of your collection. Knowing the depth of nesting will help you determine the appropriate value for the depth parameter.example.jsCopiedconst deeplyNestedArray = [1, [2, [3, [4, [5]]]]]; const result = _.flatMapDepth(deeplyNestedArray, value => value, 3); console.log(result); // Output: [1, 2, 3, [4, [5]]]
Define a Concise Iteratee Function:
Keep the iteratee function concise and focused on the transformation logic. This enhances code readability and makes your intentions clear to other developers.
example.jsCopiedconst dataToTransform = /* ...some collection... */; const transformedData = _.flatMapDepth(dataToTransform, item => { // Transformation logic return /* ...transformed value... */; }, 1); console.log(transformedData);
Consider Performance Implications:
For large collections or deeply nested structures, be mindful of performance implications. Adjust the depth parameter as needed to balance between transformation accuracy and execution speed.
example.jsCopiedconst largeNestedData = /* ...large nested collection... */; console.time('flatMapDepth'); const transformedResult = _.flatMapDepth(largeNestedData, value => /* ...transformation logic... */, 2); console.timeEnd('flatMapDepth'); console.log(transformedResult);
📚 Use Cases
Flattening and Mapping Nested Arrays:
_.flatMapDepth()
is particularly useful when dealing with collections containing nested arrays. It allows you to both flatten and apply a mapping function to elements at a specified depth.example.jsCopiedconst dataWithNestedArrays = [1, [2, [3, [4]]]]; const transformedData = _.flatMapDepth(dataWithNestedArrays, value => value * 2, 2); console.log(transformedData); // Output: [2, 4, 6, 8]
Handling Nested Objects:
When working with collections containing nested objects,
_.flatMapDepth()
can simplify the process of transforming and flattening the structure.example.jsCopiedconst dataWithNestedObjects = [ { id: 1, values: [2, 3] }, { id: 2, values: [4, 5] }, ]; const transformedData = _.flatMapDepth(dataWithNestedObjects, 'values', 1); console.log(transformedData); // Output: [2, 3, 4, 5]
Recursive Data Processing:
For collections with recursive patterns, such as trees or hierarchical structures,
_.flatMapDepth()
enables recursive data processing up to a specified depth.example.jsCopiedconst recursiveData = { id: 1, children: [{ id: 2, children: [{ id: 3 }] }] }; const flattenedData = _.flatMapDepth(recursiveData, node => node.id, 2); console.log(flattenedData); // Output: [1, 2, 3]
🎉 Conclusion
The _.flatMapDepth()
method in Lodash is a versatile tool for transforming and flattening nested arrays within a collection. Whether you're dealing with deeply nested structures or recursive data, this method provides a concise and efficient solution. Incorporate _.flatMapDepth()
into your JavaScript projects to streamline collection manipulation and enhance the readability of your code.
Explore the capabilities of _.flatMapDepth()
and elevate your data transformation workflows in JavaScript!
👨💻 Join our Community:
Author
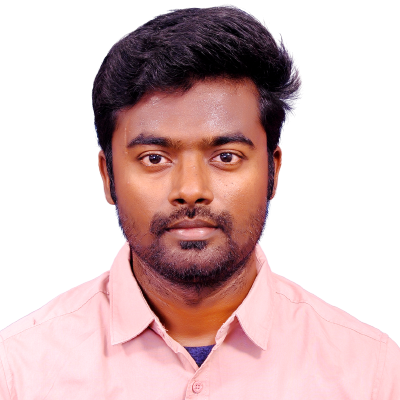
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
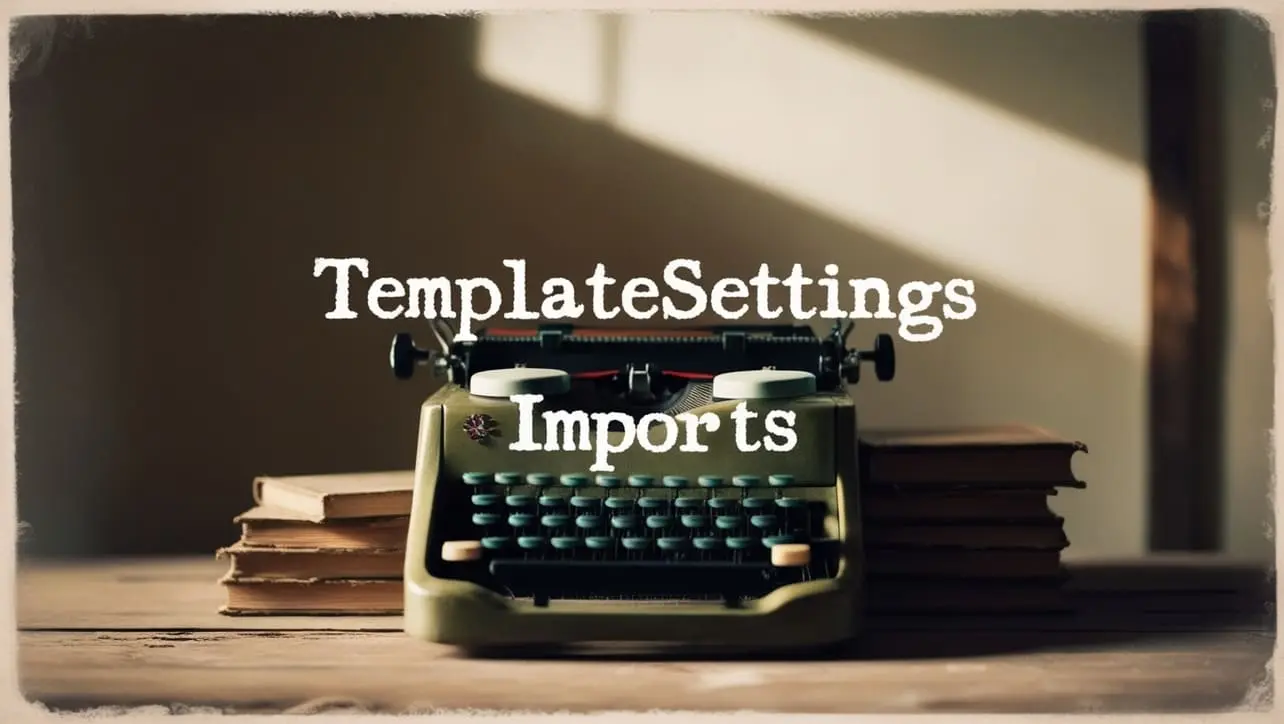
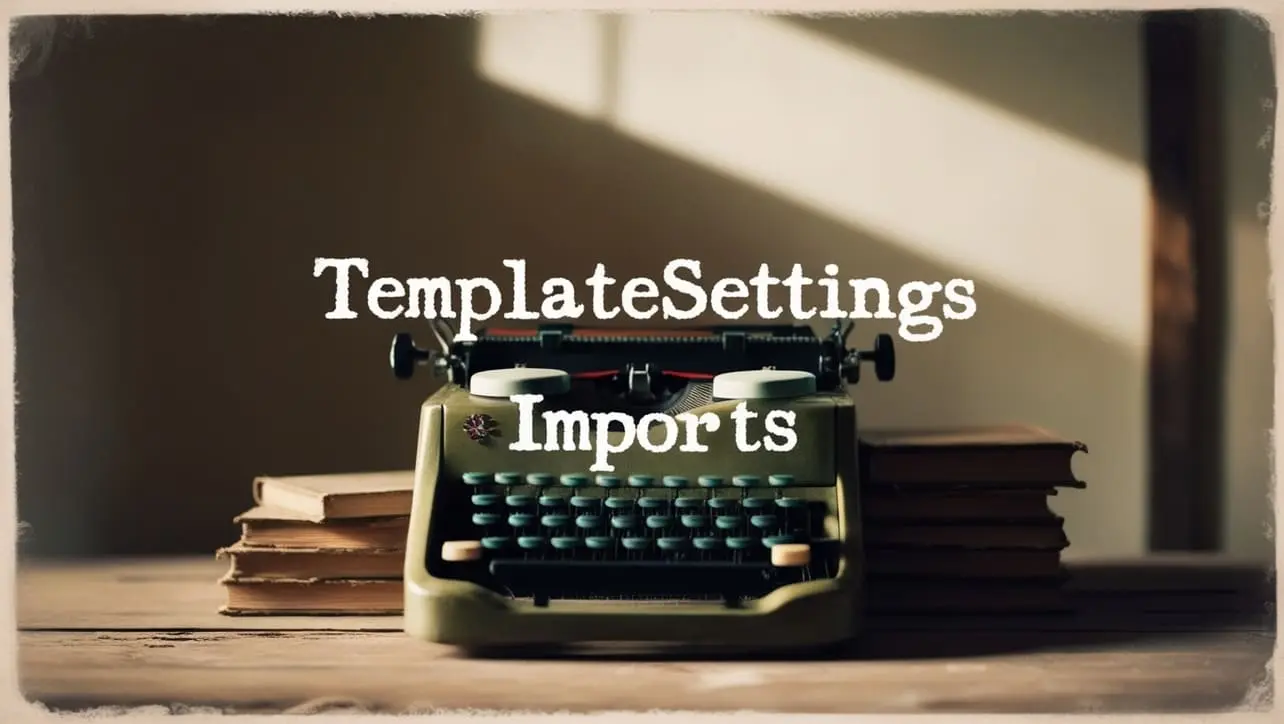
Lodash _.templateSettings.imports Property
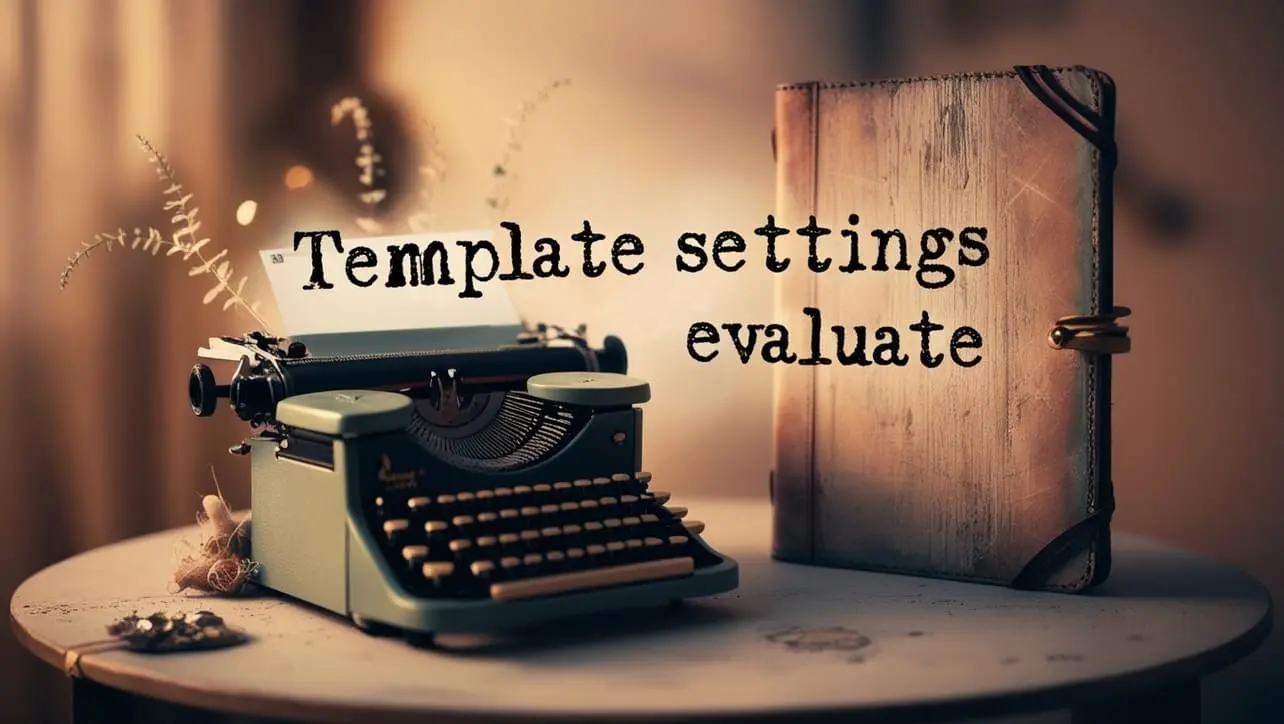
Lodash _.templateSettings.evaluate Property
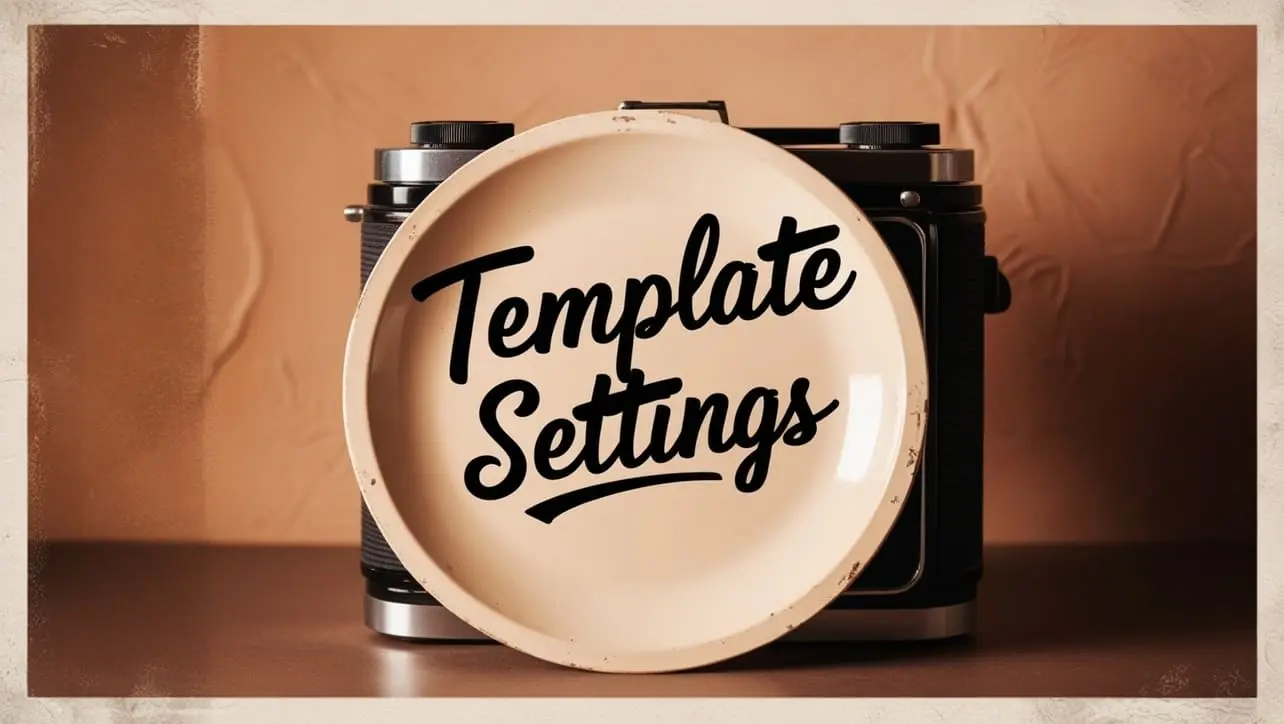
Lodash _.templateSettings Property
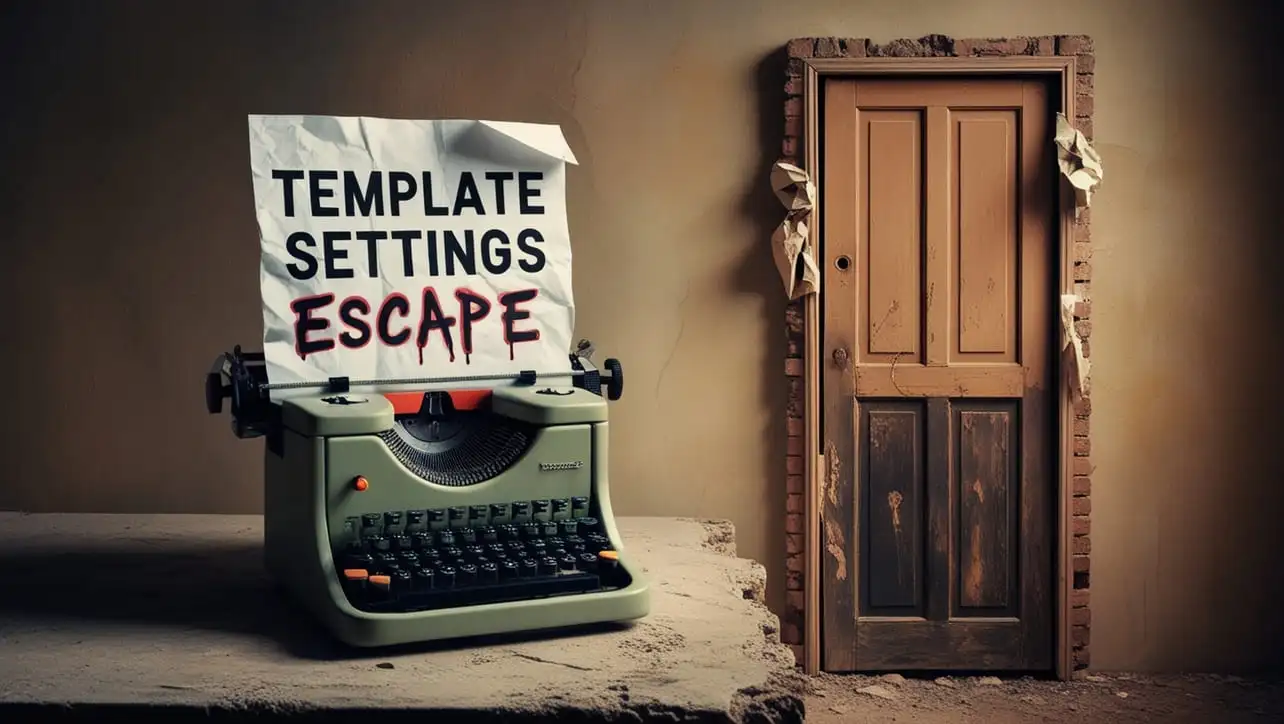
Lodash _.templateSettings.escape Property
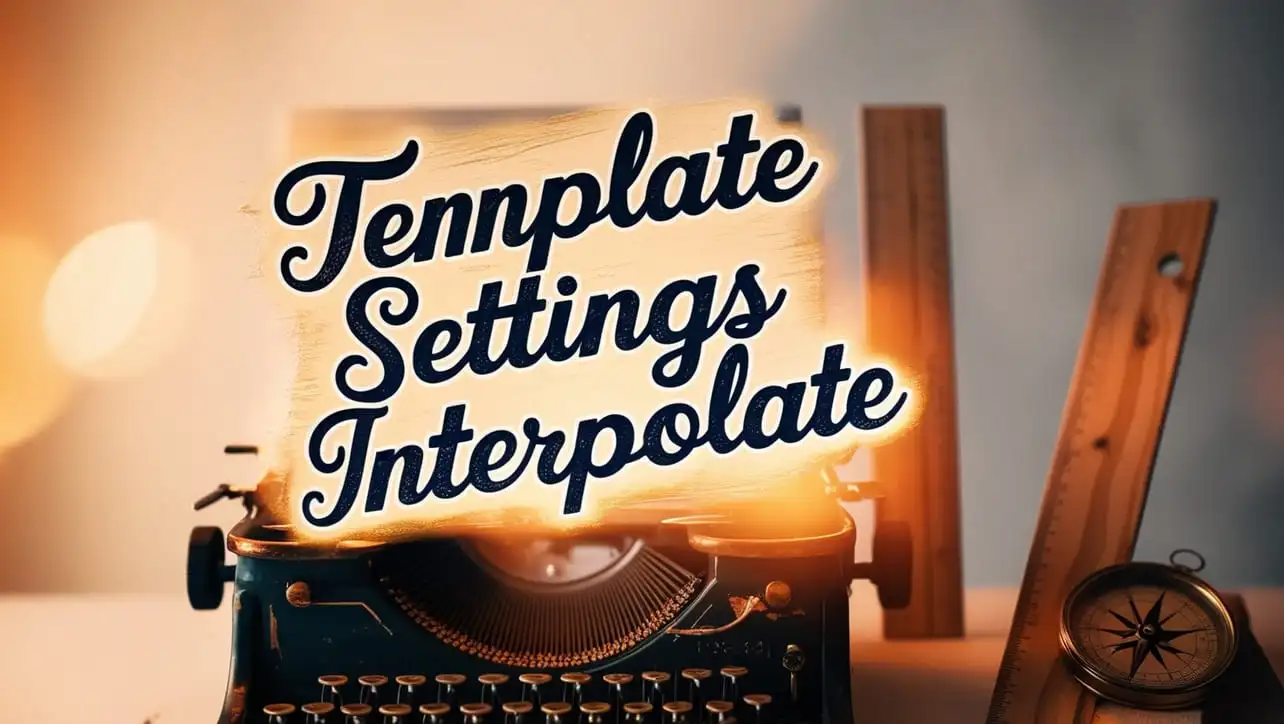
Lodash _.templateSettings.interpolate Property
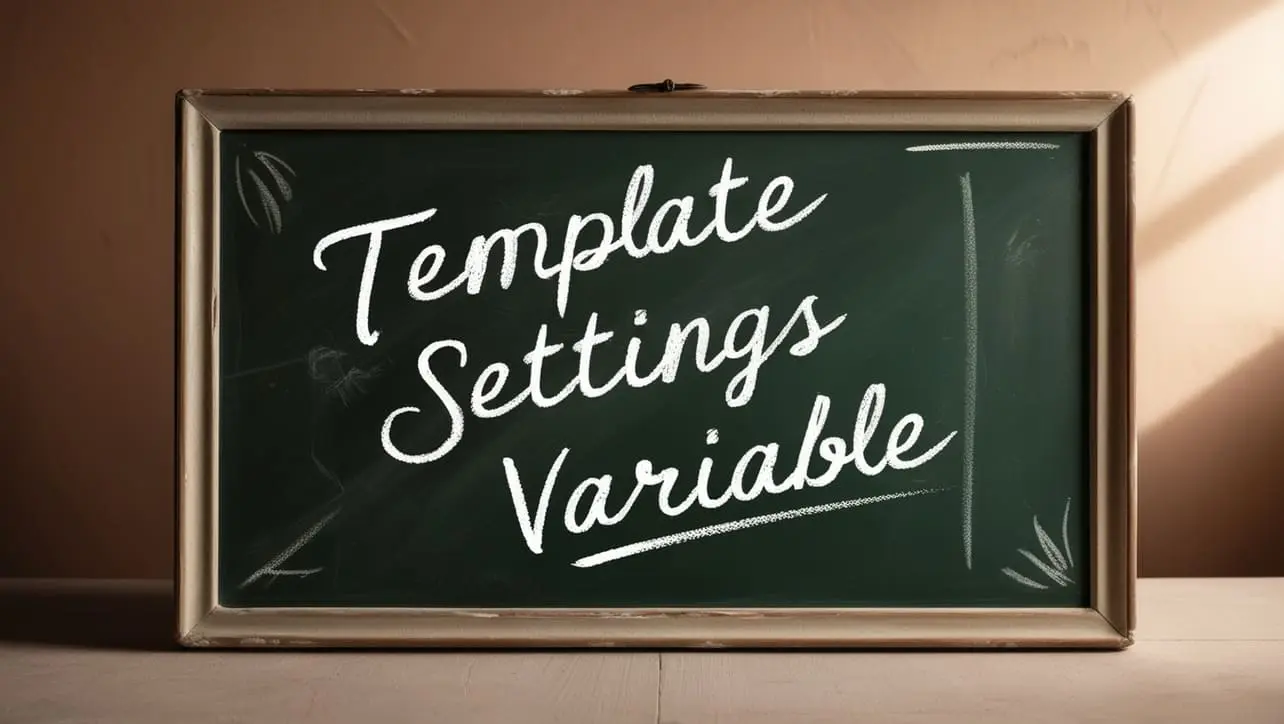
If you have any doubts regarding this article (Lodash _.flatMapDepth() Collection Method), please comment here. I will help you immediately.