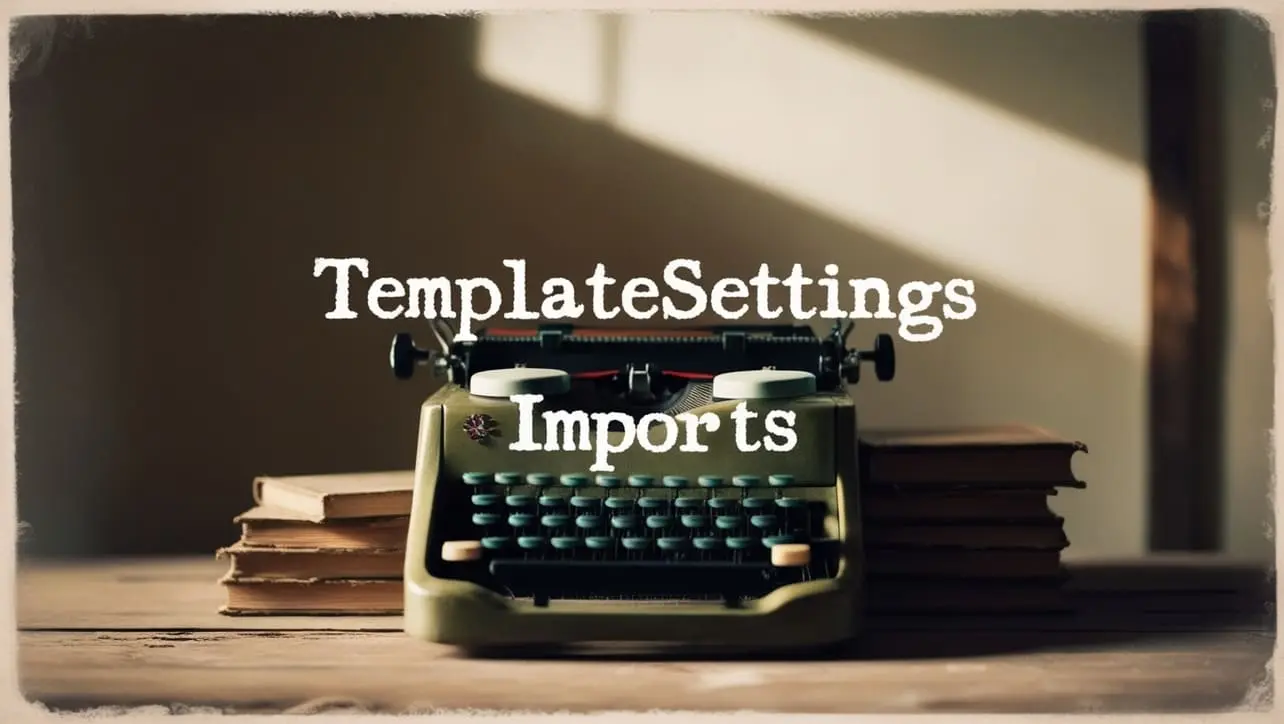
Lodash _.flatMap() Collection Method
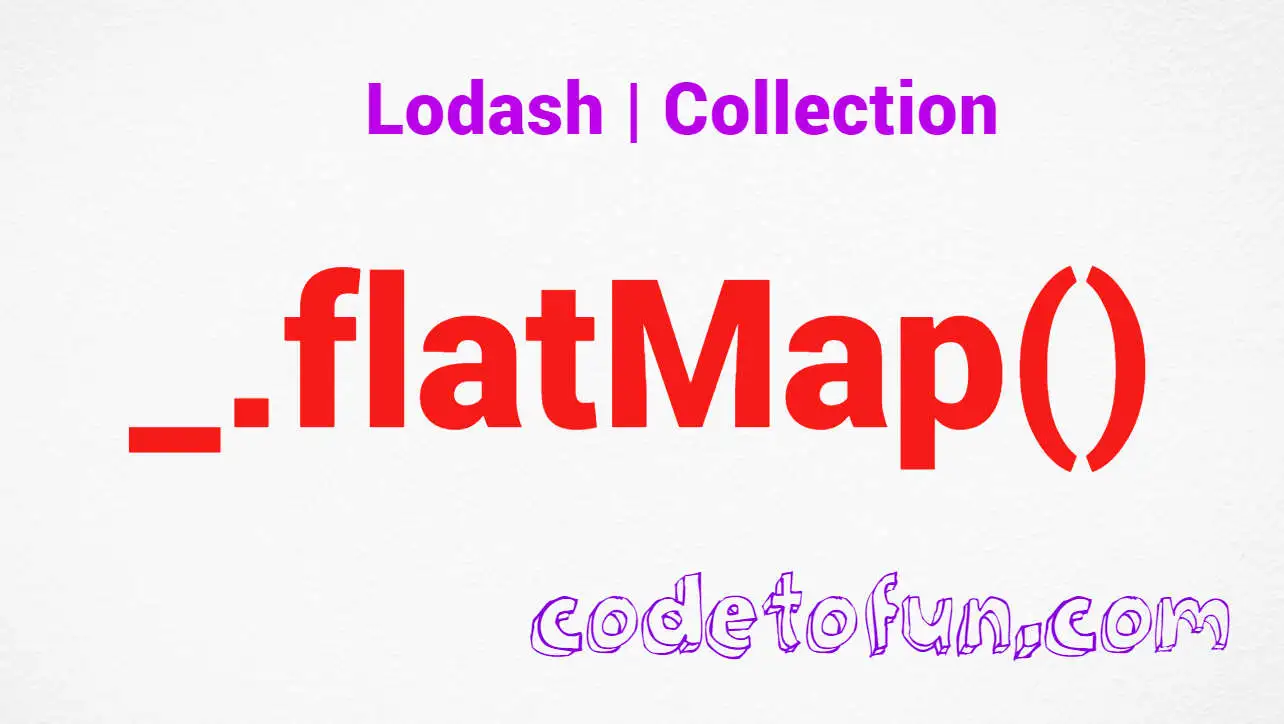
Photo Credit to CodeToFun
🙋 Introduction
Efficiently transforming and manipulating collections is a common task in JavaScript development. Lodash, a powerful utility library, provides a range of functions to streamline these operations. One such versatile function is _.flatMap()
, designed to flatten and map elements of a collection simultaneously.
This method proves invaluable for developers seeking concise and readable code when working with complex nested structures.
🧠 Understanding _.flatMap()
The _.flatMap()
method in Lodash is a combination of flattening and mapping. It first maps each element using a mapping function and then flattens the result into a single array. This dual functionality simplifies the process of handling collections with nested arrays or objects.
💡 Syntax
_.flatMap(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a practical example to illustrate the power of _.flatMap()
:
const _ = require('lodash');
const nestedArray = [[1, 2], [3, 4], [5, 6]];
const flattenedAndMapped = _.flatMap(nestedArray, array => array.map(num => num * 2));
console.log(flattenedAndMapped);
// Output: [2, 4, 6, 8, 10, 12]
In this example, the nestedArray is flattened and mapped using a function that doubles each element.
🏆 Best Practices
Understanding Flattening:
Be aware that
_.flatMap()
not only maps elements but also flattens the result. Ensure this behavior aligns with your intentions, especially when working with deeply nested structures.example.jsCopiedconst deeplyNestedArray = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]; const flattenedAndMappedDeep = _.flatMap(deeplyNestedArray, array => array.map(num => num * 2)); console.log(flattenedAndMappedDeep); // Output: [2, 4, 6, 8, 10, 12, 14, 16]
Custom Mapping Logic:
Take advantage of the iteratee parameter to provide custom mapping logic. This allows you to tailor the transformation according to the specific requirements of your project.
example.jsCopiedconst arrayOfObjects = [{ numbers: [1, 2] }, { numbers: [3, 4] }]; const flattenedAndMappedObjects = _.flatMap(arrayOfObjects, obj => obj.numbers.map(num => num * 2)); console.log(flattenedAndMappedObjects); // Output: [2, 4, 6, 8]
Maintaining Context:
When using
_.flatMap()
with methods that rely on the this context, such as class methods, consider using arrow functions to maintain the correct context.example.jsCopiedclass Transformer { constructor(factor) { this.factor = factor; } transformArray(arr) { return _.flatMap(arr, num => num * this.factor); } } const transformerInstance = new Transformer(3); const resultWithContext = transformerInstance.transformArray([[1, 2], [3, 4]]); console.log(resultWithContext); // Output: [3, 6, 9, 12]
📚 Use Cases
Flattening and Mapping Arrays:
The primary use case for
_.flatMap()
is flattening and mapping arrays simultaneously. This is especially handy when working with arrays of arrays or arrays of objects.example.jsCopiedconst arrayOfArrays = [[1, 2], [3, 4], [5, 6]]; const result = _.flatMap(arrayOfArrays, array => array.map(num => num * 2)); console.log(result); // Output: [2, 4, 6, 8, 10, 12]
Transforming Object Properties:
When dealing with an array of objects,
_.flatMap()
can be used to transform specific properties of each object.example.jsCopiedconst arrayOfObjects = [{ numbers: [1, 2] }, { numbers: [3, 4] }]; const transformedNumbers = _.flatMap(arrayOfObjects, obj => obj.numbers.map(num => num * 2)); console.log(transformedNumbers); // Output: [2, 4, 6, 8]
Handling Data with Irregular Structures:
When working with data that has irregular structures, such as nested arrays of varying depths,
_.flatMap()
can simplify the transformation process.example.jsCopiedconst irregularData = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]; const flattenedAndMapped = _.flatMap(irregularData, array => array.map(num => num * 2)); console.log(flattenedAndMapped); // Output: [2, 4, 6, 8, 10, 12, 14, 16]
🎉 Conclusion
The _.flatMap()
method in Lodash is a powerful tool for efficiently handling collections with nested structures. Whether you're flattening arrays, transforming object properties, or dealing with irregular data, _.flatMap()
provides a concise and expressive solution to simplify your JavaScript code.
Explore the capabilities of _.flatMap()
and enhance your collection manipulation strategies in JavaScript!
👨💻 Join our Community:
Author
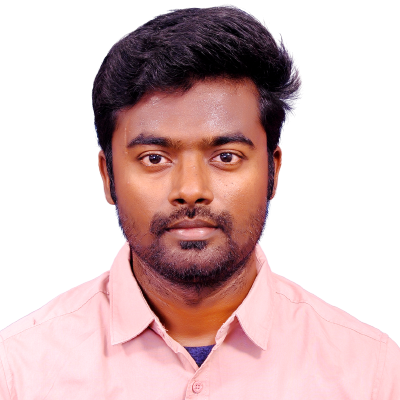
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
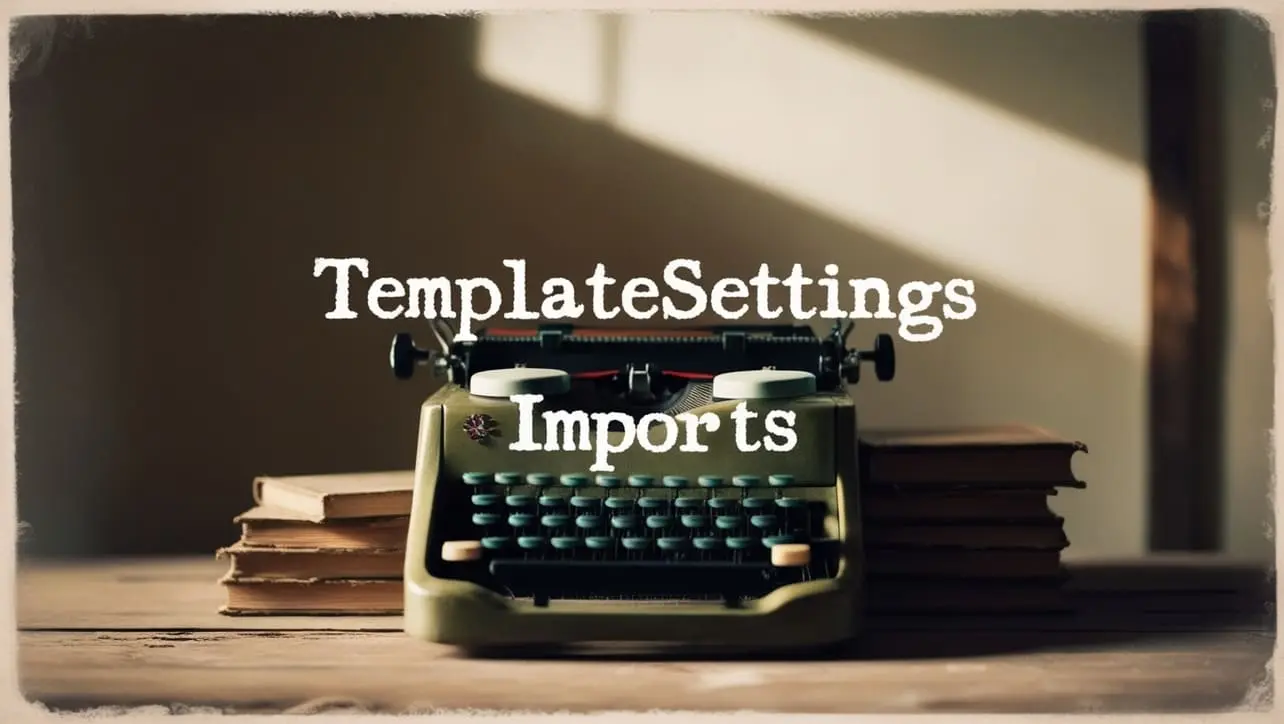
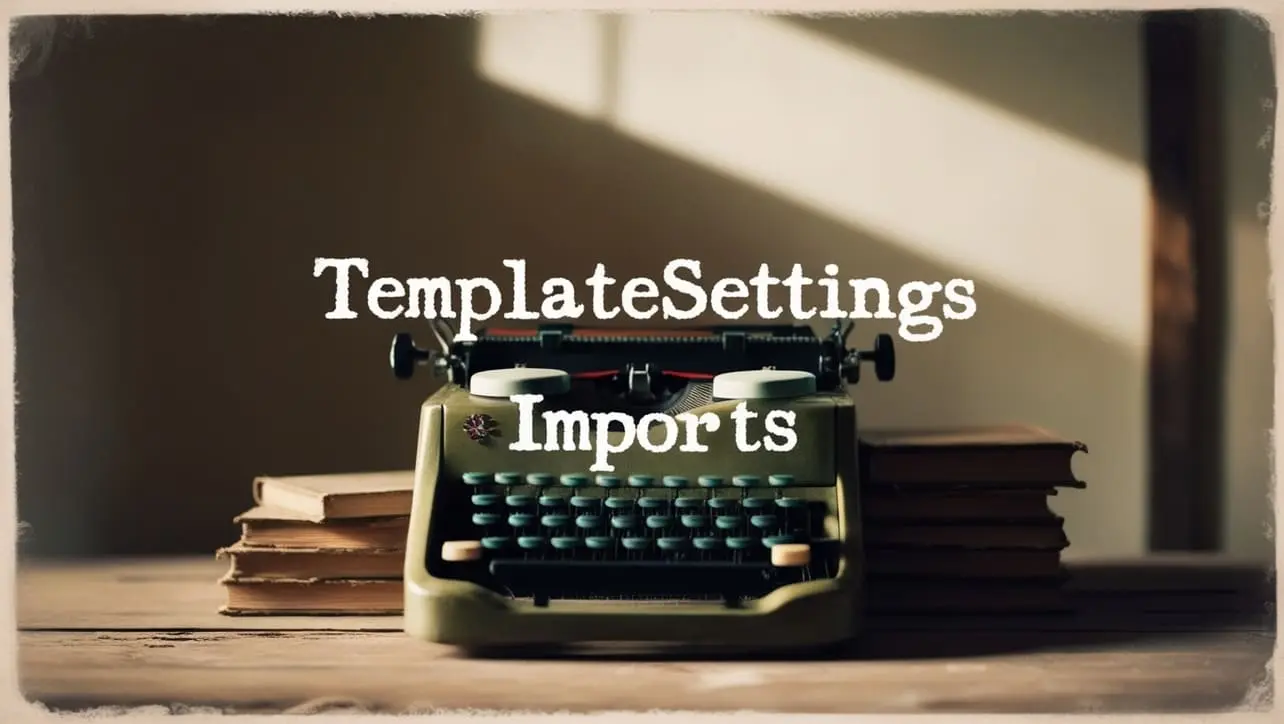
Lodash _.templateSettings.imports Property
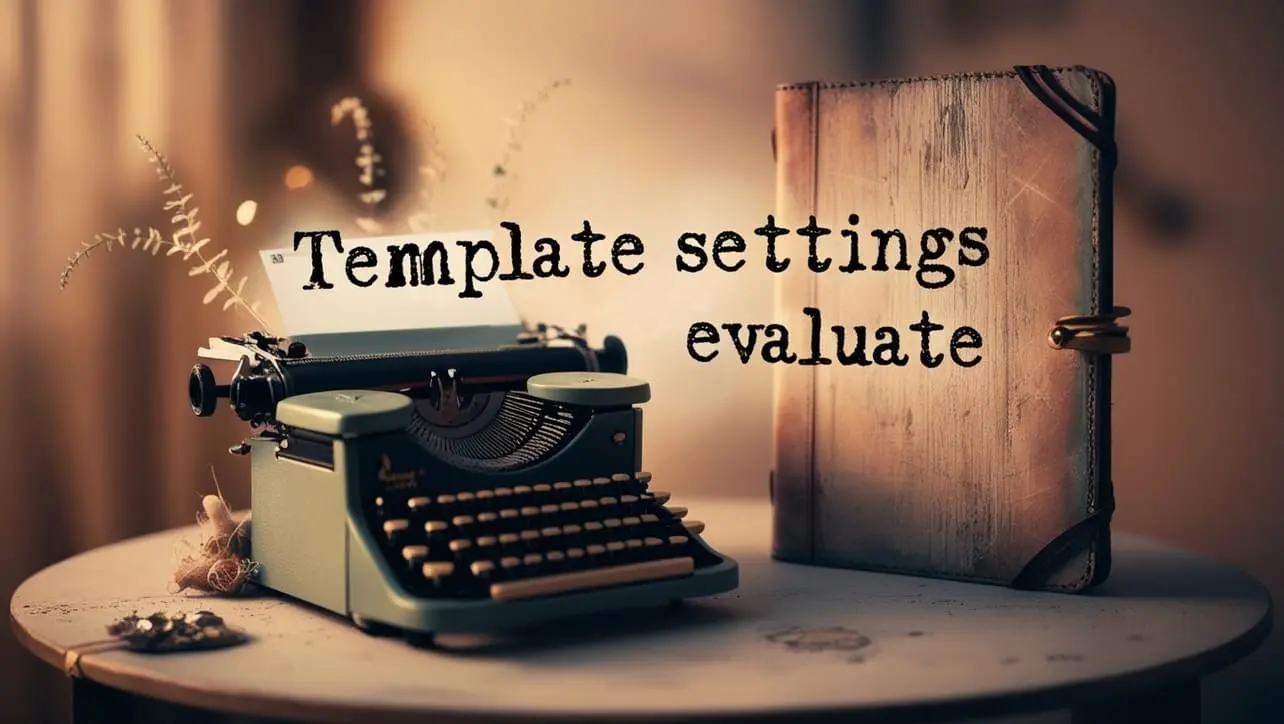
Lodash _.templateSettings.evaluate Property
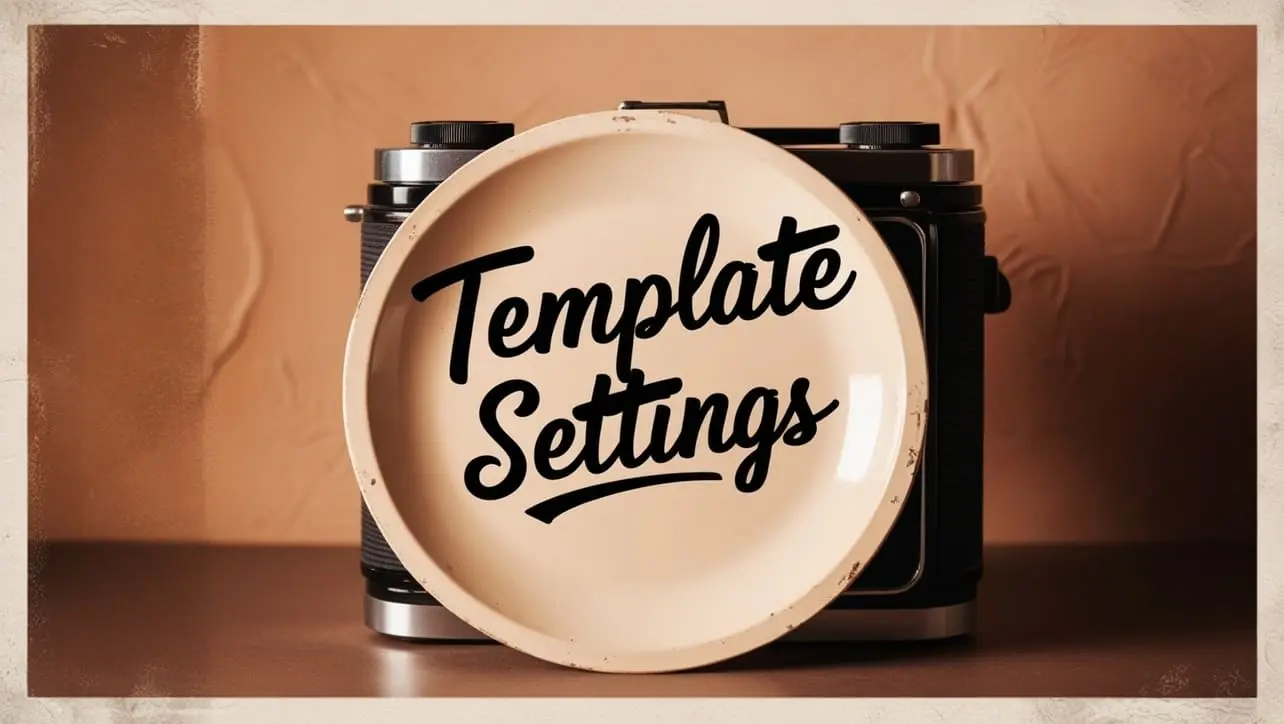
Lodash _.templateSettings Property
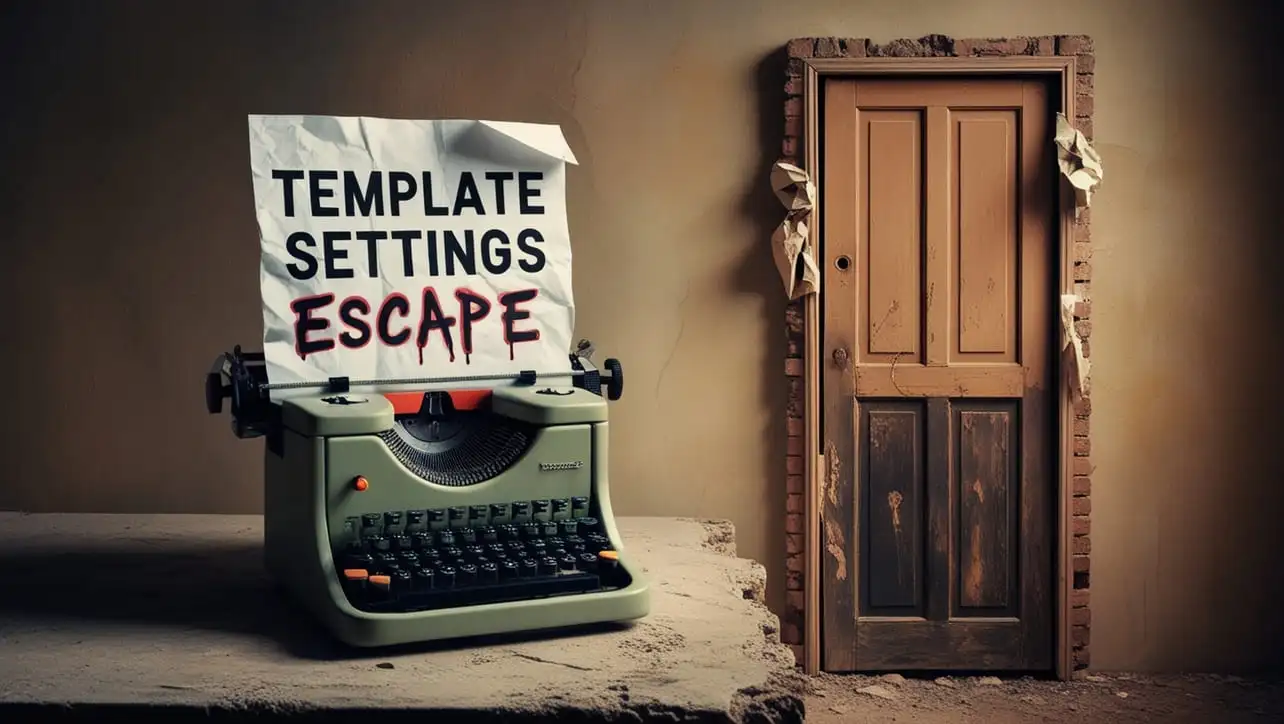
Lodash _.templateSettings.escape Property
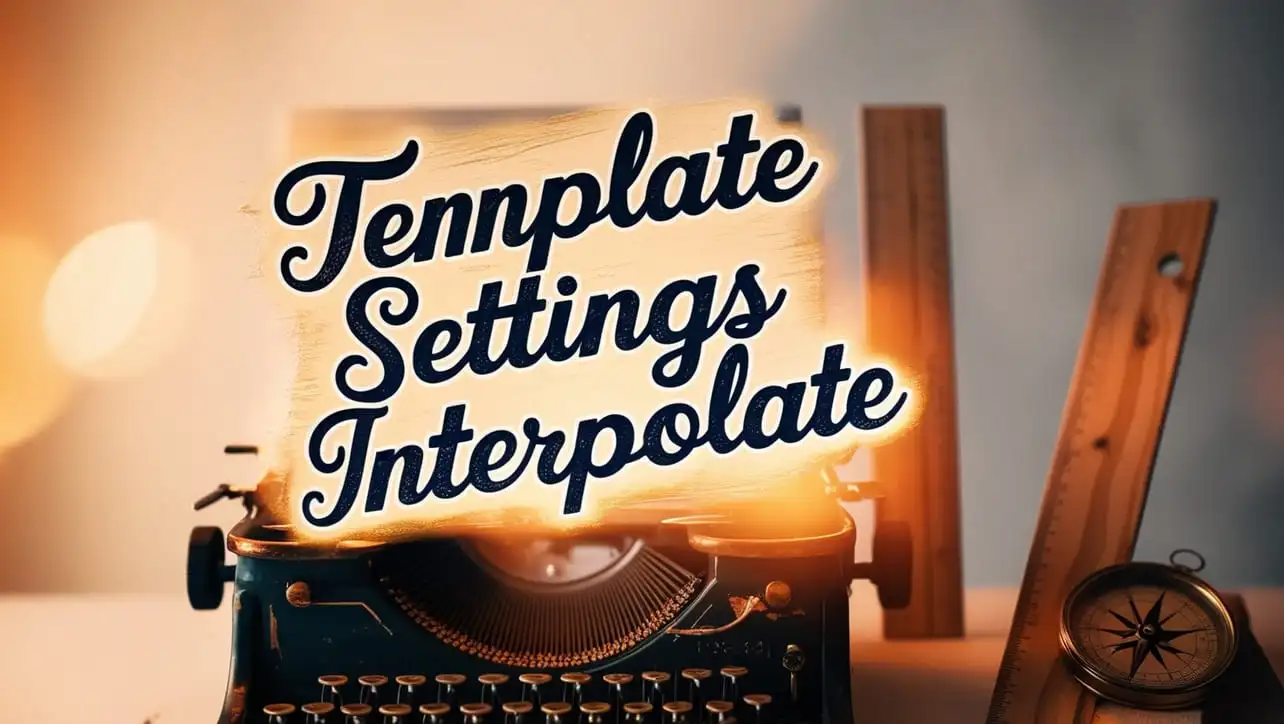
Lodash _.templateSettings.interpolate Property
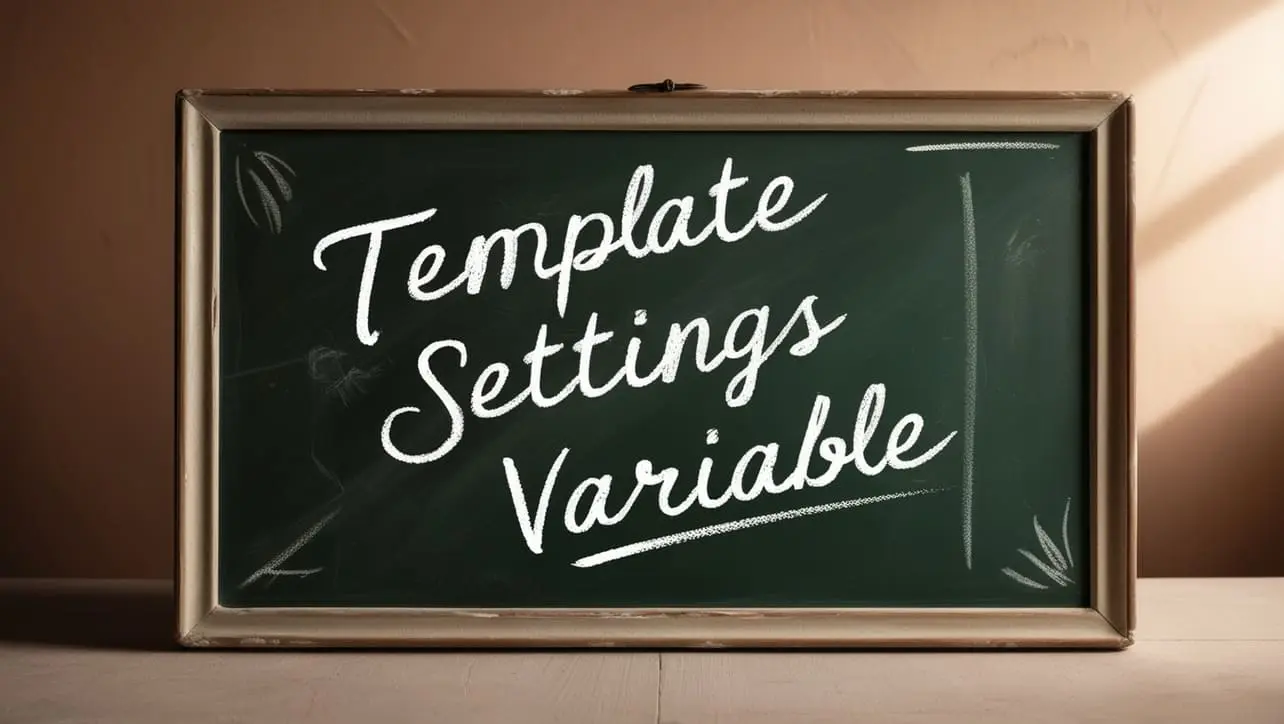
If you have any doubts regarding this article (Lodash _.flatMap() Collection Method), please comment here. I will help you immediately.