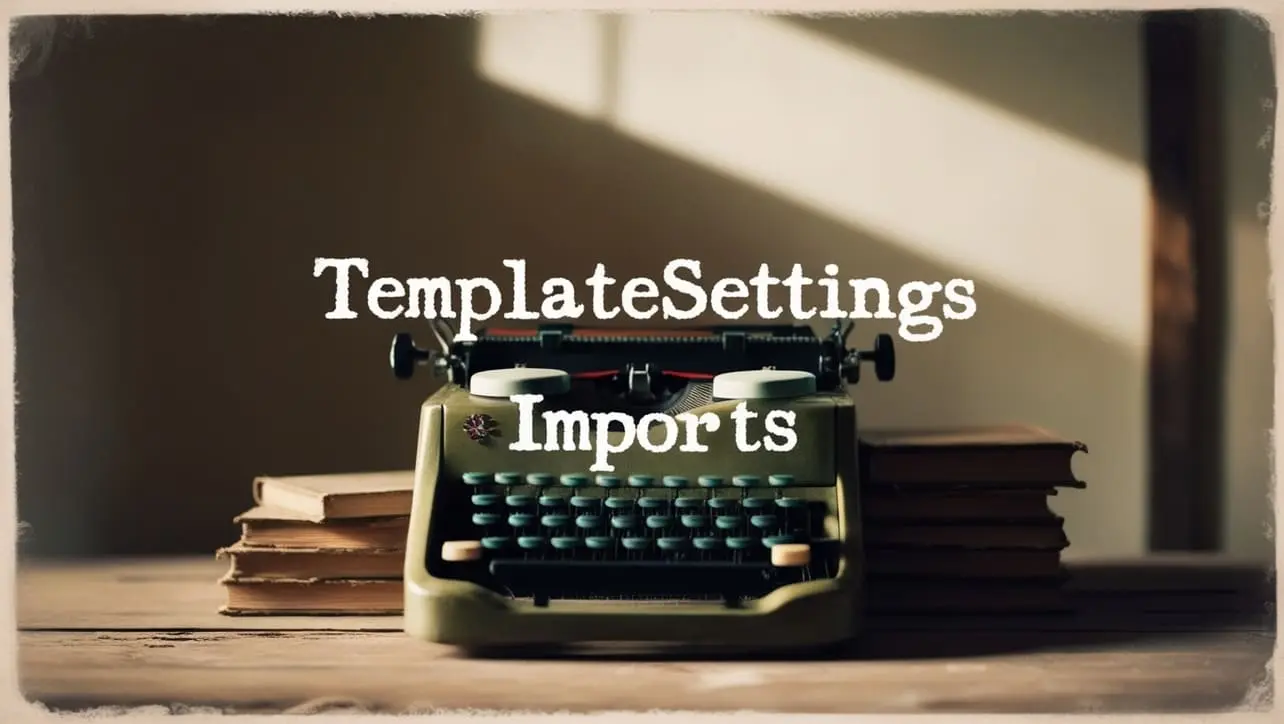
Lodash _.filter() Collection Method
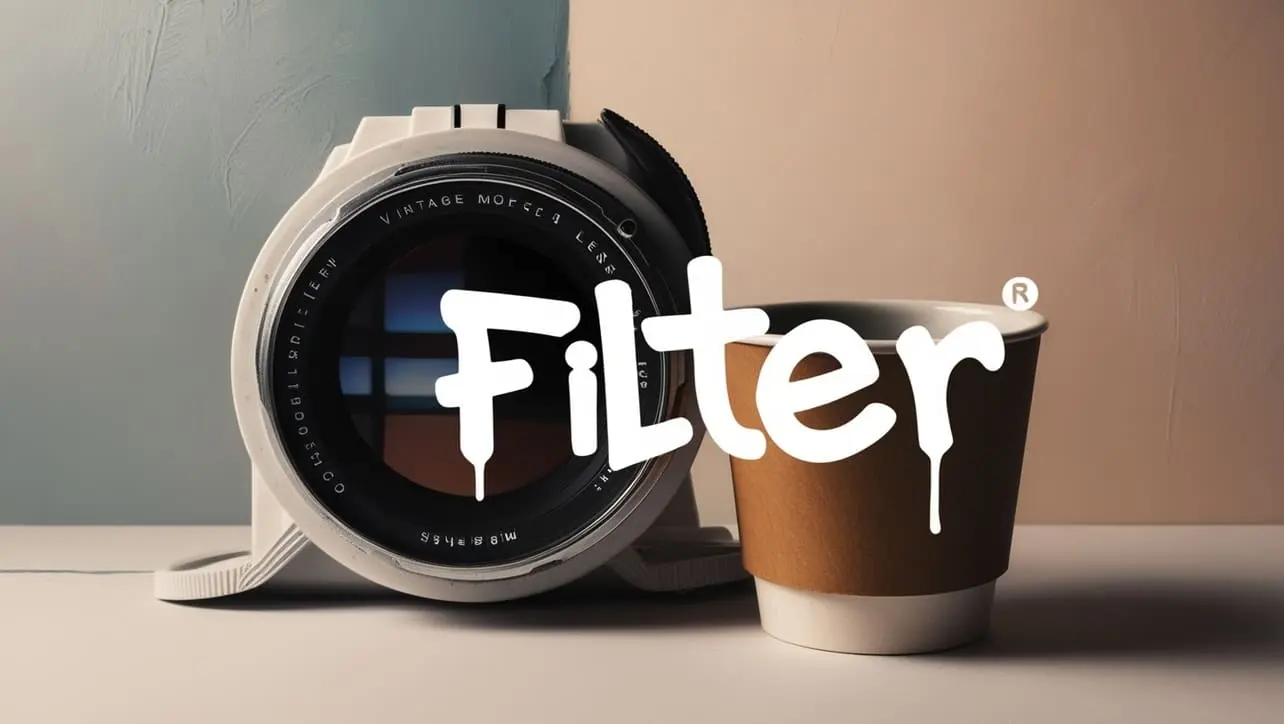
Photo Credit to CodeToFun
🙋 Introduction
Efficiently working with collections in JavaScript is a common requirement for developers, and Lodash provides a powerful set of tools to streamline this process. Among these tools, the _.filter()
method stands out as a versatile and handy function for selectively extracting elements from a collection based on a given predicate.
Whether you're dealing with arrays, objects, or other iterable data structures, _.filter()
proves to be a valuable asset in your JavaScript toolkit.
🧠 Understanding _.filter()
The _.filter()
method in Lodash allows you to sift through a collection and retrieve elements that satisfy a specified condition. This condition is defined by a predicate function, giving you the flexibility to tailor the filtering process according to your specific requirements.
💡 Syntax
_.filter(collection, predicate)
- collection: The collection to iterate over.
- predicate: The function invoked per iteration.
📝 Example
Let's dive into a practical example to illustrate the functionality of _.filter()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const evenNumbers = _.filter(numbers, num => num % 2 === 0);
console.log(evenNumbers);
// Output: [2, 4, 6, 8]
In this example, the numbers array is filtered to extract only the even numbers, creating a new array named evenNumbers.
🏆 Best Practices
Clear Predicate Function:
Ensure that the predicate function used with
_.filter()
returns a clear and consistent boolean result. This clarity is essential for understanding the filtering criteria.example.jsCopiedconst words = ['apple', 'banana', 'grape', 'orange']; const filteredWords = _.filter(words, word => word.length > 5); console.log(filteredWords); // Output: ['banana', 'orange']
Leverage Inline Functions:
For simple filtering criteria, consider using inline functions directly within the
_.filter()
call. This can enhance code readability, especially when the predicate logic is concise.example.jsCopiedconst scores = [90, 85, 75, 95, 80]; const passingScores = _.filter(scores, score => score >= 80); console.log(passingScores); // Output: [90, 85, 95, 80]
Combine with Other Lodash Methods:
Explore the synergy of Lodash methods by combining
_.filter()
with others like _.map() or _.sortBy() to perform complex transformations on your collections.example.jsCopiedconst students = [ { name: 'Alice', grade: 'A' }, { name: 'Bob', grade: 'B' }, { name: 'Charlie', grade: 'A' }, ]; const topPerformers = _.filter(students, student => student.grade === 'A'); const topPerformersNames = _.map(topPerformers, 'name'); console.log(topPerformersNames); // Output: ['Alice', 'Charlie']
📚 Use Cases
Filtering Objects in an Array:
_.filter()
is ideal for extracting specific objects from an array based on a given condition, making it valuable in scenarios where you need to narrow down your data.example.jsCopiedconst users = [ { id: 1, role: 'admin' }, { id: 2, role: 'user' }, { id: 3, role: 'admin' }, ]; const admins = _.filter(users, user => user.role === 'admin'); console.log(admins); // Output: [{ id: 1, role: 'admin' }, { id: 3, role: 'admin' }]
Dynamic Filtering with External Input:
When dealing with dynamic filtering criteria based on external input,
_.filter()
allows you to adapt your filtering logic dynamically.example.jsCopiedconst products = [ { id: 1, category: 'electronics' }, { id: 2, category: 'clothing' }, { id: 3, category: 'electronics' }, ]; const selectedCategory = 'electronics'; const filteredProducts = _.filter(products, { category: selectedCategory }); console.log(filteredProducts); // Output: [{ id: 1, category: 'electronics' }, { id: 3, category: 'electronics' }]
Filtering Based on Complex Conditions:
For intricate filtering conditions involving multiple criteria,
_.filter()
shines by providing a clean and maintainable way to express complex logic.example.jsCopiedconst inventory = [ { id: 1, type: 'laptop', brand: 'Dell', price: 1200 }, { id: 2, type: 'tablet', brand: 'Apple', price: 800 }, { id: 3, type: 'laptop', brand: 'HP', price: 1000 }, ]; const affordableLaptops = _.filter(inventory, item => item.type === 'laptop' && item.price < 1100); console.log(affordableLaptops); // Output: [{ id: 3, type: 'laptop', brand: 'HP', price: 1000 }]
🎉 Conclusion
The _.filter()
method in Lodash empowers you to selectively extract elements from collections, bringing clarity and flexibility to your JavaScript code. Whether you're dealing with simple filtering criteria or complex conditions, this method proves to be an invaluable asset in enhancing the manipulation of your data.
Explore the capabilities of _.filter()
and elevate your collection handling in JavaScript!
👨💻 Join our Community:
Author
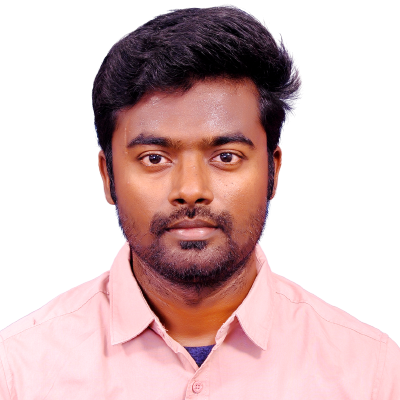
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
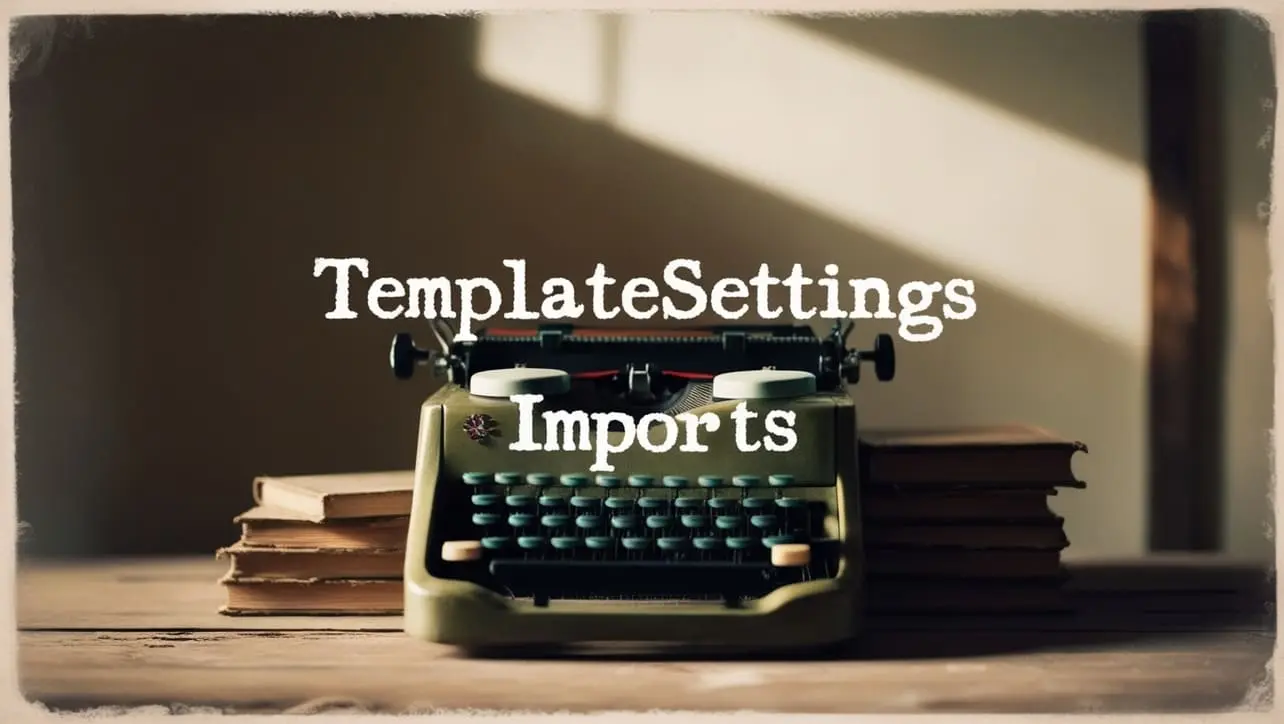
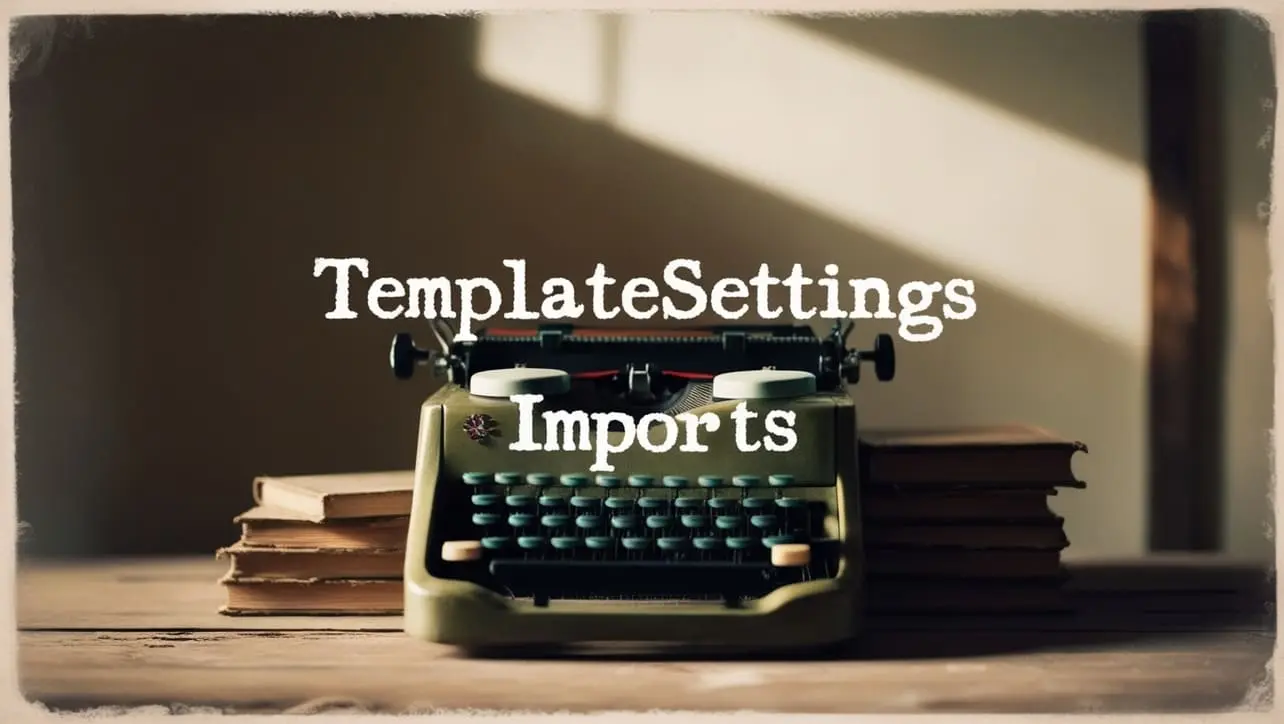
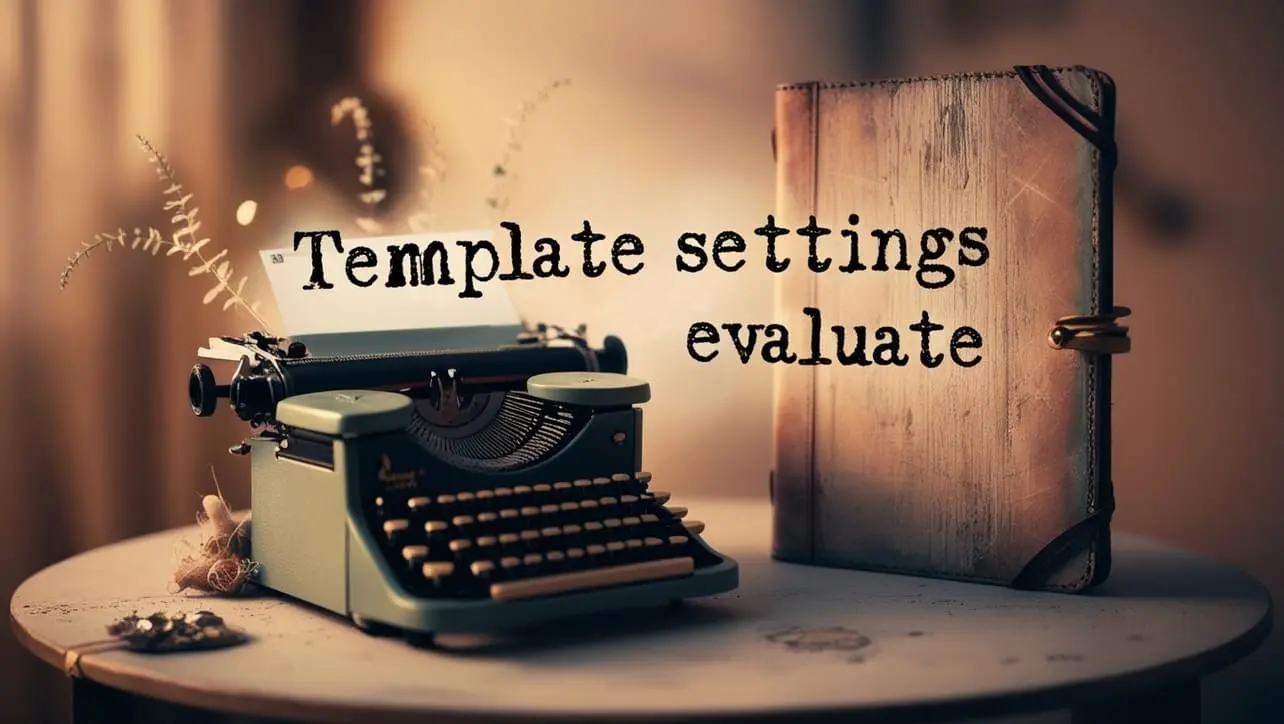
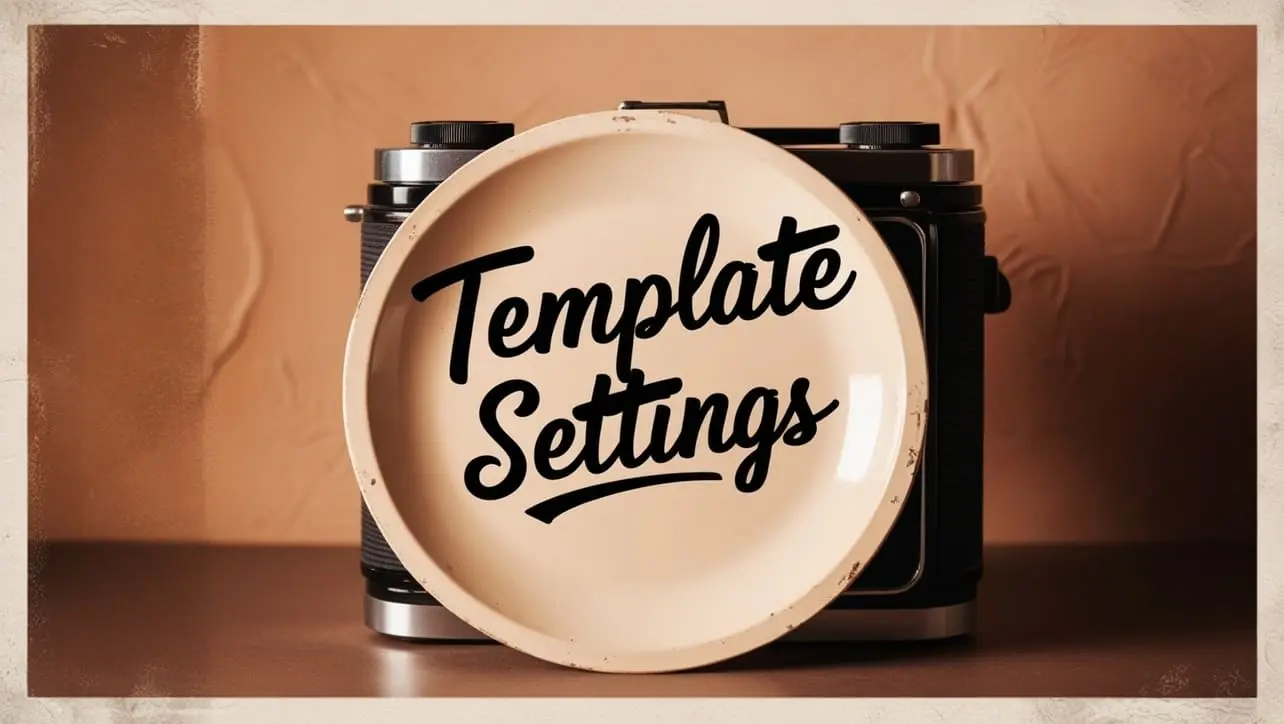
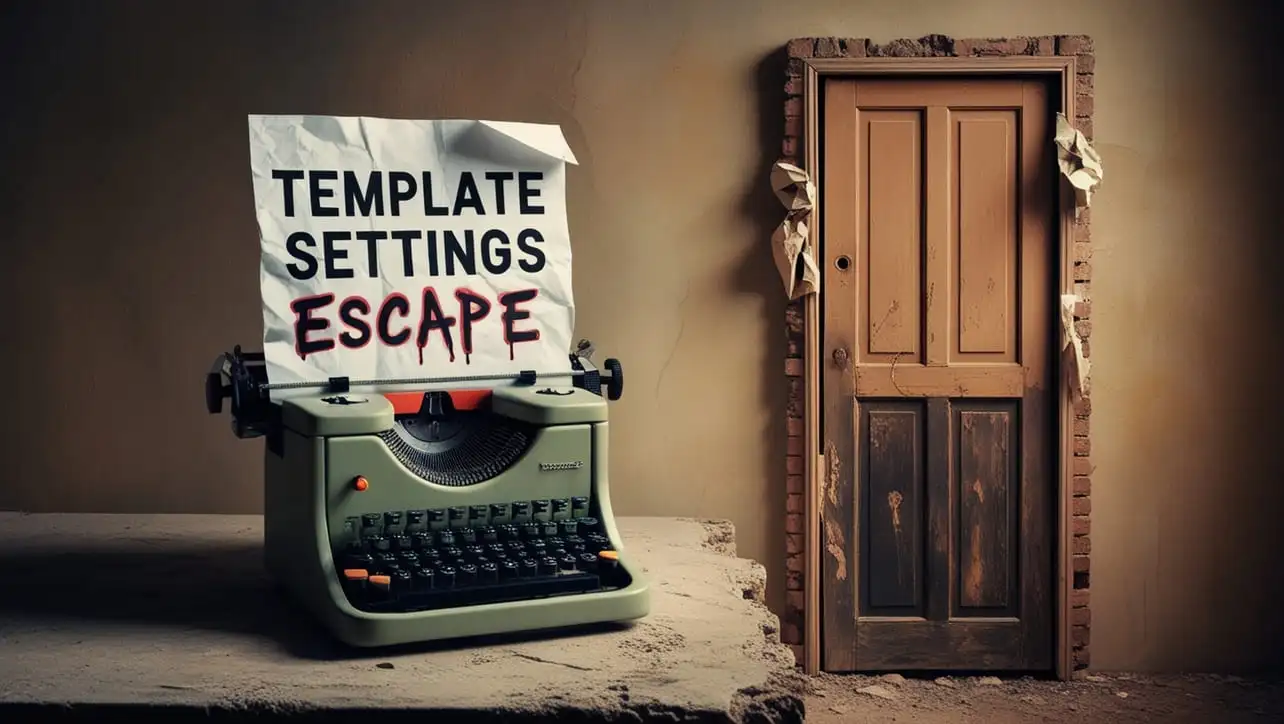
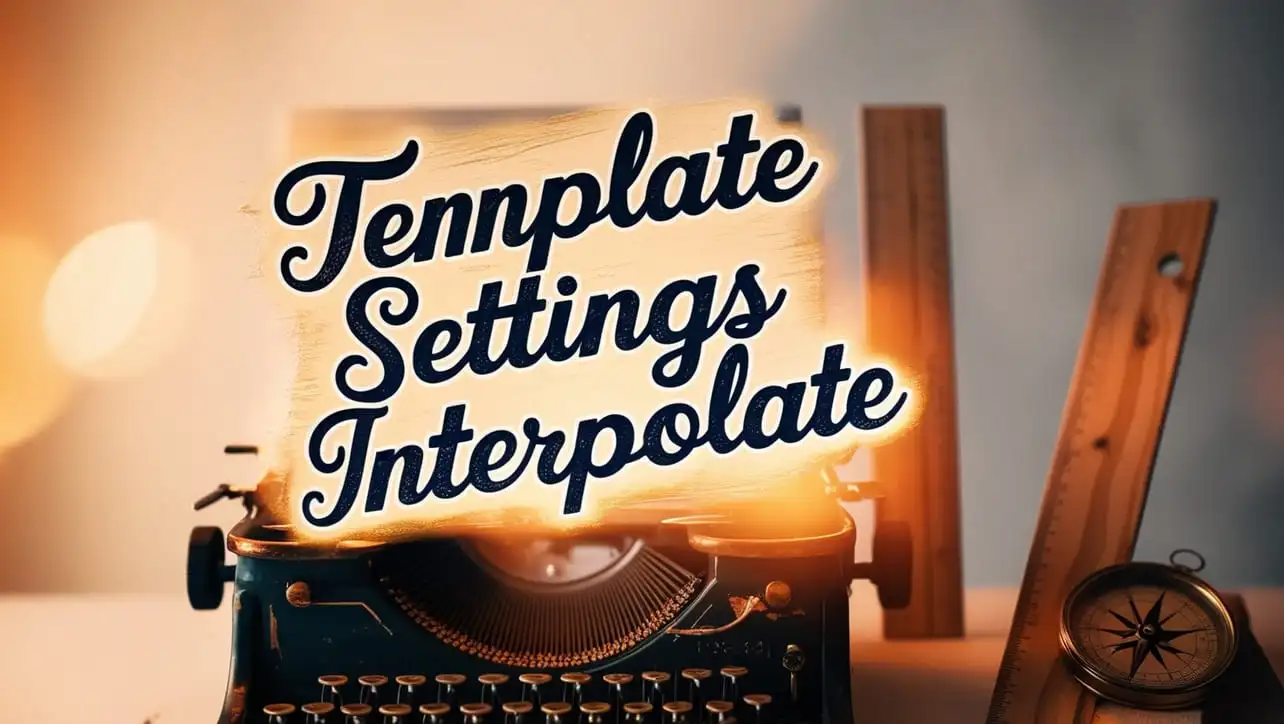
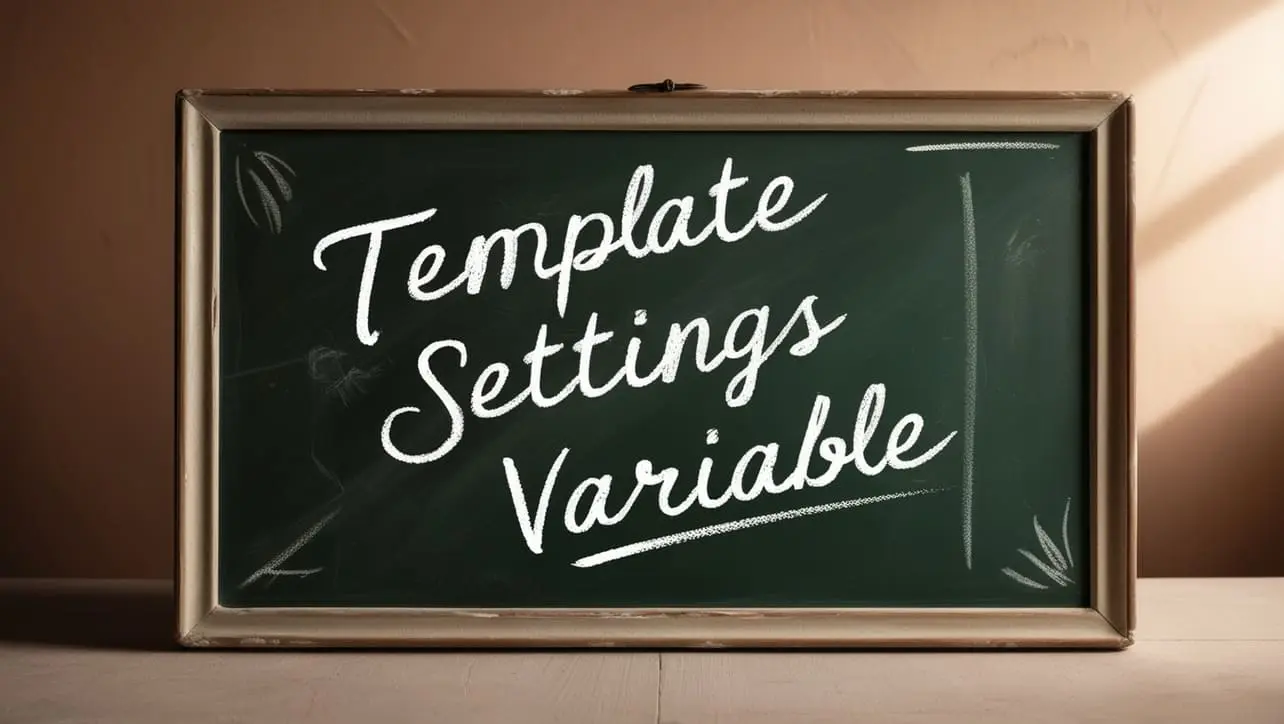
If you have any doubts regarding this article (Lodash _.filter() Collection Method), please comment here. I will help you immediately.