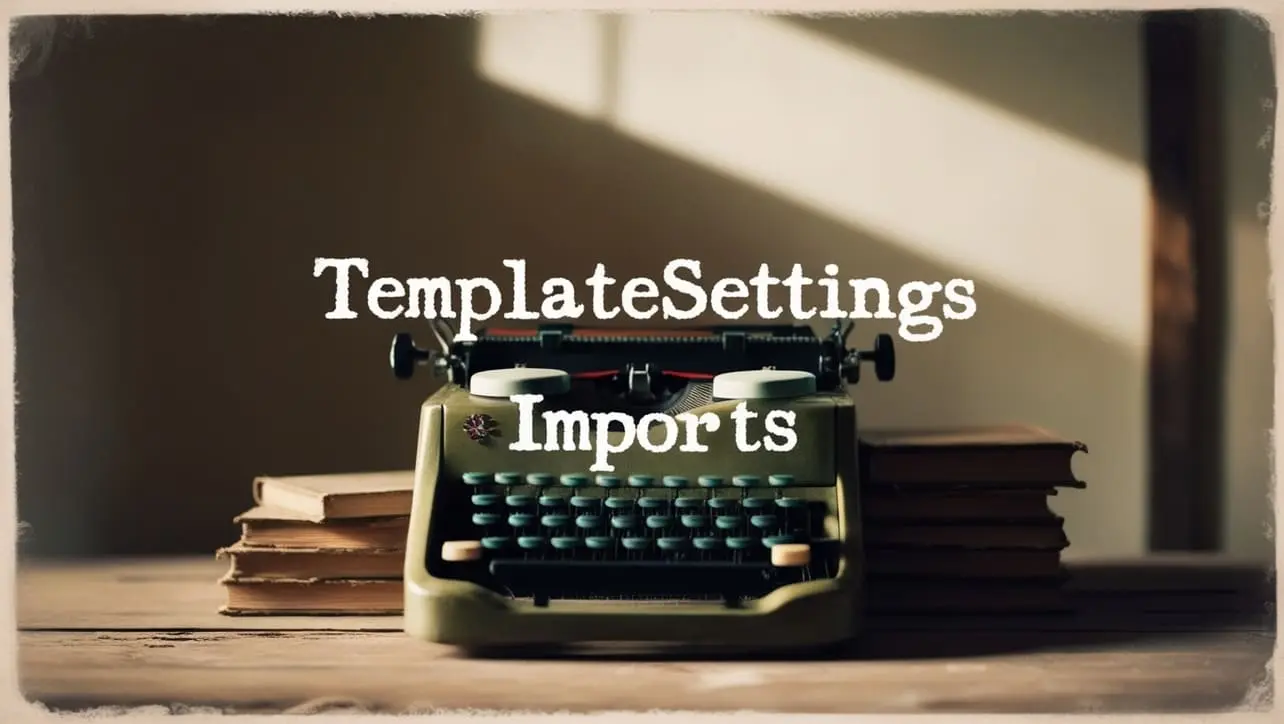
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.zipObjectDeep() Array Method
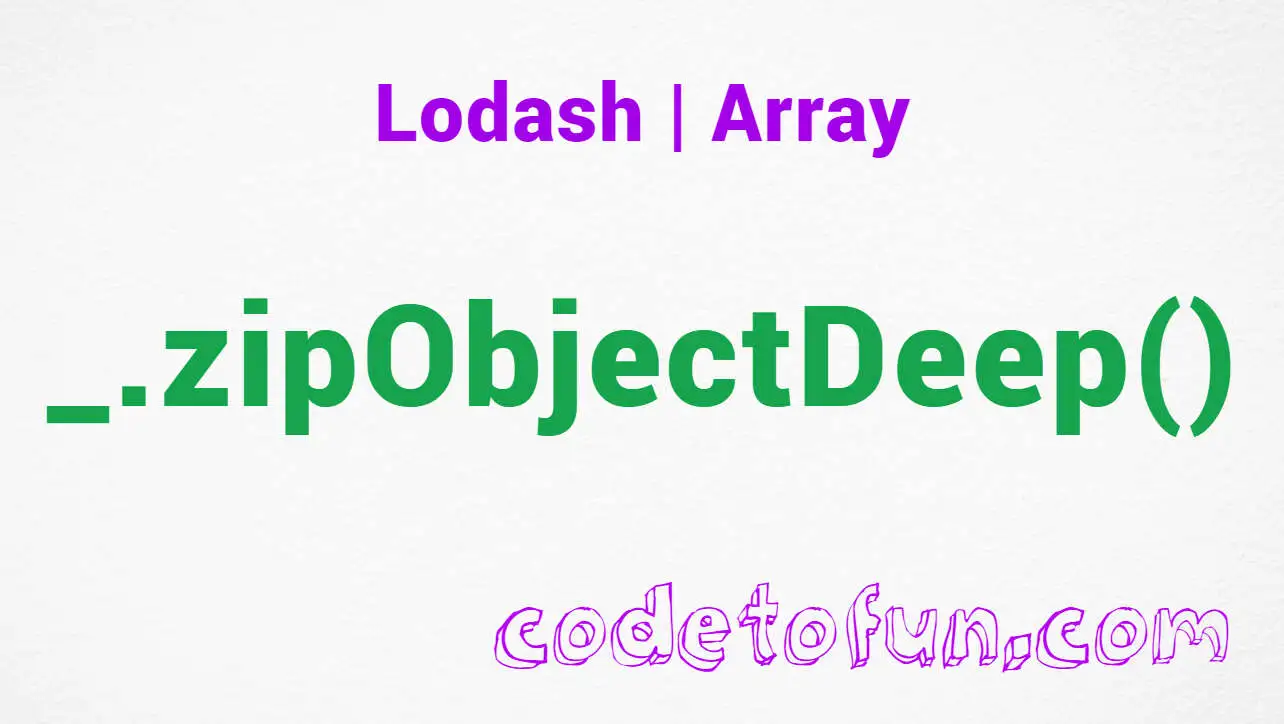
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, efficient handling and transformation of data structures are essential tasks. Lodash, a versatile utility library, offers a plethora of functions, and among them is the _.zipObjectDeep()
method. This powerful method enables developers to create nested objects from parallel arrays, providing a clean and concise way to structure data.
In this exploration, we'll delve into the intricacies of _.zipObjectDeep()
and discover how it can elevate your JavaScript coding experience.
🧠 Understanding _.zipObjectDeep()
The _.zipObjectDeep()
method in Lodash is designed to create nested objects by combining parallel arrays. This method is particularly useful when you have data distributed across multiple arrays and want to structure it hierarchically.
💡 Syntax
_.zipObjectDeep([props], [values])
- props: An array of property identifiers.
- values: An array of values corresponding to the properties.
📝 Example
Let's dive into a practical example to illustrate the functionality of _.zipObjectDeep()
:
const _ = require('lodash');
const propertyNames = ['user', 'details', 'address', 'city'];
const propertyValues = ['John Doe', { age: 30, gender: 'male' }, { street: '123 Main St', zip: '12345' }, 'New York'];
const nestedObject = _.zipObjectDeep(propertyNames, propertyValues);
console.log(nestedObject);
/* Output:
{
user: 'John Doe',
details: { age: 30, gender: 'male' },
address: { street: '123 Main St', zip: '12345' },
city: 'New York'
}
*/
In this example, the propertyNames array is used as identifiers for nested properties, and the propertyValues array contains corresponding values. The result is a nested object created by zipping these arrays together.
🏆 Best Practices
Consistent Array Lengths:
Ensure that the arrays provided to
_.zipObjectDeep()
have consistent lengths. Mismatched lengths may result in unexpected behavior, with excess values or missing properties in the generated object.example.jsCopiedconst inconsistentProps = ['name', 'age']; const inconsistentValues = ['John Doe']; const inconsistentObject = _.zipObjectDeep(inconsistentProps, inconsistentValues); console.log(inconsistentObject); // Output: { name: 'John Doe', age: undefined }
Utilize Nested Structures:
Take advantage of the ability to create nested structures with
_.zipObjectDeep()
. This is especially powerful when dealing with complex data representations.example.jsCopiedconst nestedProps = ['user', 'details', 'address', 'city']; const nestedValues = ['John Doe', { age: 30, gender: 'male' }, { street: '123 Main St', zip: '12345' }, 'New York']; const nestedObject = _.zipObjectDeep(nestedProps, nestedValues); console.log(nestedObject); /* Output: { user: 'John Doe', details: { age: 30, gender: 'male' }, address: { street: '123 Main St', zip: '12345' }, city: 'New York' } */
Dynamic Property Generation:
Experiment with dynamically generating property names based on your data. This can be achieved by using arrays of strings or numbers as property identifiers.
example.jsCopiedconst dynamicProps = ['user', 'details', 0, 1]; const dynamicValues = ['John Doe', { age: 30, gender: 'male' }, 'extraValue', 'anotherValue']; const dynamicObject = _.zipObjectDeep(dynamicProps, dynamicValues); console.log(dynamicObject); /* Output: { user: 'John Doe', details: { age: 30, gender: 'male' }, 0: 'extraValue', 1: 'anotherValue' } */
📚 Use Cases
Structuring Form Data:
_.zipObjectDeep()
is handy when dealing with form data where input names or identifiers are distributed across different arrays.example.jsCopiedconst formDataFields = ['user', 'details', 'address', 'city']; const formDataValues = ['John Doe', { age: 30, gender: 'male' }, '123 Main St', 'New York']; const formDataObject = _.zipObjectDeep(formDataFields, formDataValues); console.log(formDataObject); /* Output: { user: 'John Doe', details: { age: 30, gender: 'male' }, address: '123 Main St', city: 'New York' } */
JSON Object Creation:
When dealing with dynamic JSON object creation,
_.zipObjectDeep()
simplifies the process of combining identifiers and values into a structured object.example.jsCopiedconst jsonProps = ['header', 'content', 'footer']; const jsonValues = ['Welcome', { text: 'Lorem Ipsum' }, 'Contact us']; const jsonObject = _.zipObjectDeep(jsonProps, jsonValues); console.log(jsonObject); /* Output: { header: 'Welcome', content: { text: 'Lorem Ipsum' }, footer: 'Contact us' } */
Database Record Transformation:
When transforming database records, use
_.zipObjectDeep()
to map property names and values seamlessly.example.jsCopiedconst dbRecordFields = ['id', 'name', 'age']; const dbRecordValues = [1, 'Alice', 28]; const dbRecordObject = _.zipObjectDeep(dbRecordFields, dbRecordValues); console.log(dbRecordObject); /* Output: { id: 1, name: 'Alice', age: 28 } */
🎉 Conclusion
The _.zipObjectDeep()
method in Lodash is a versatile tool for creating nested objects from parallel arrays. Whether you're structuring form data, dynamically generating JSON objects, or transforming database records, this method simplifies the process with clean and concise syntax.
Explore the capabilities of _.zipObjectDeep()
and enhance your JavaScript coding experience by efficiently structuring and transforming data!
👨💻 Join our Community:
Author
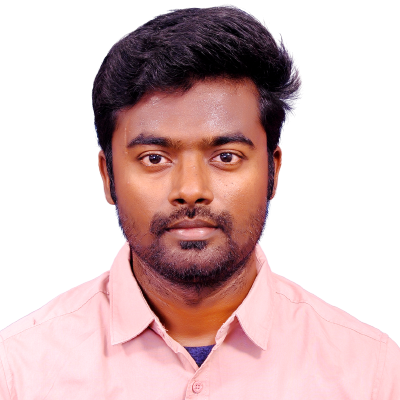
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
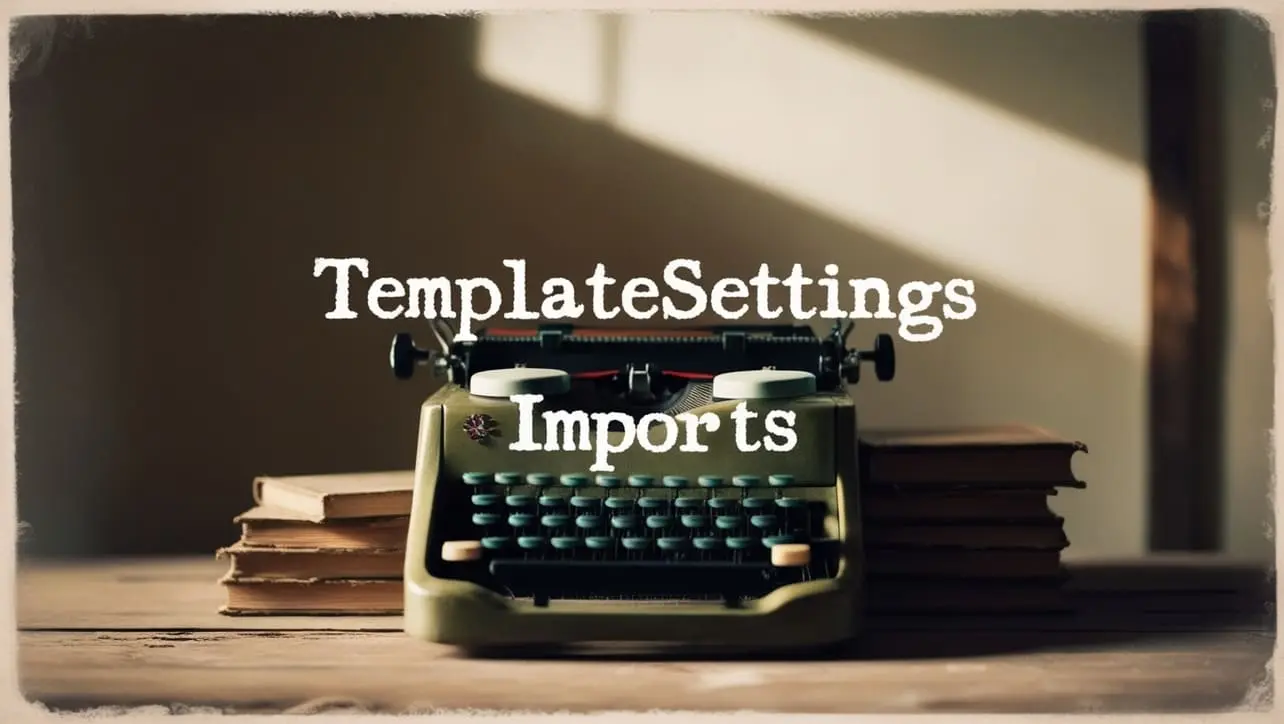
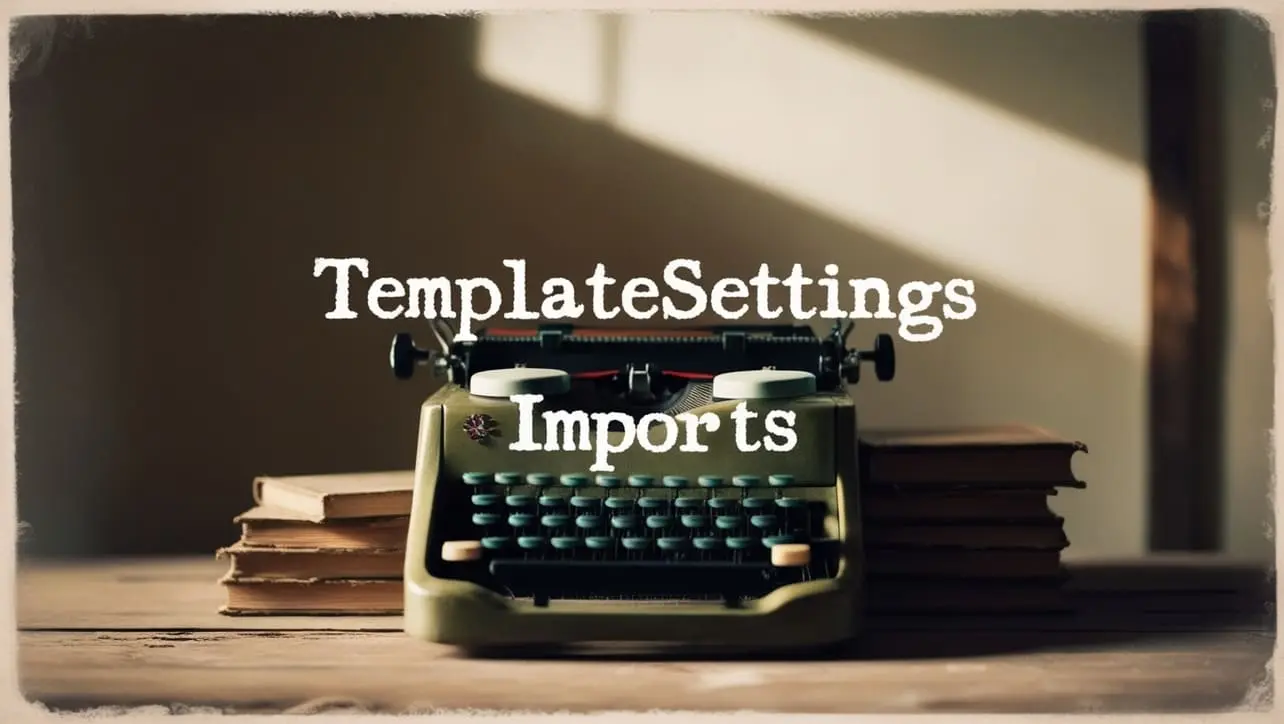
Lodash _.templateSettings.imports Property
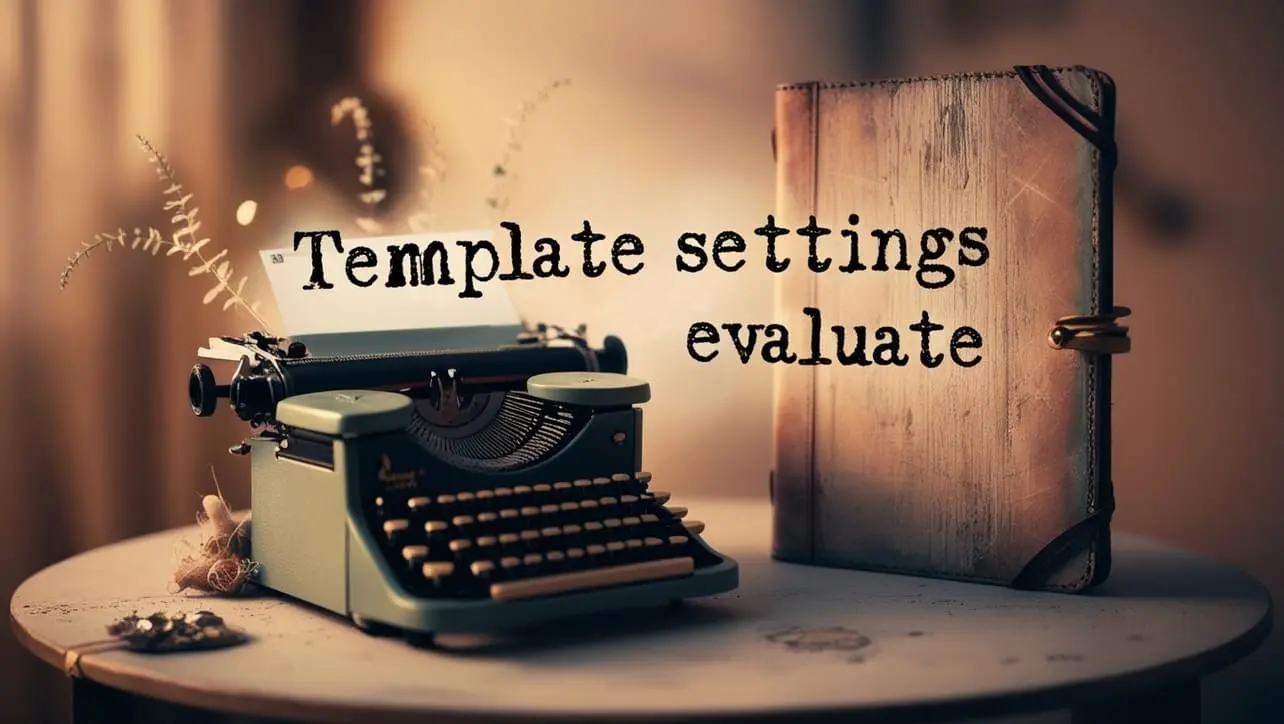
Lodash _.templateSettings.evaluate Property
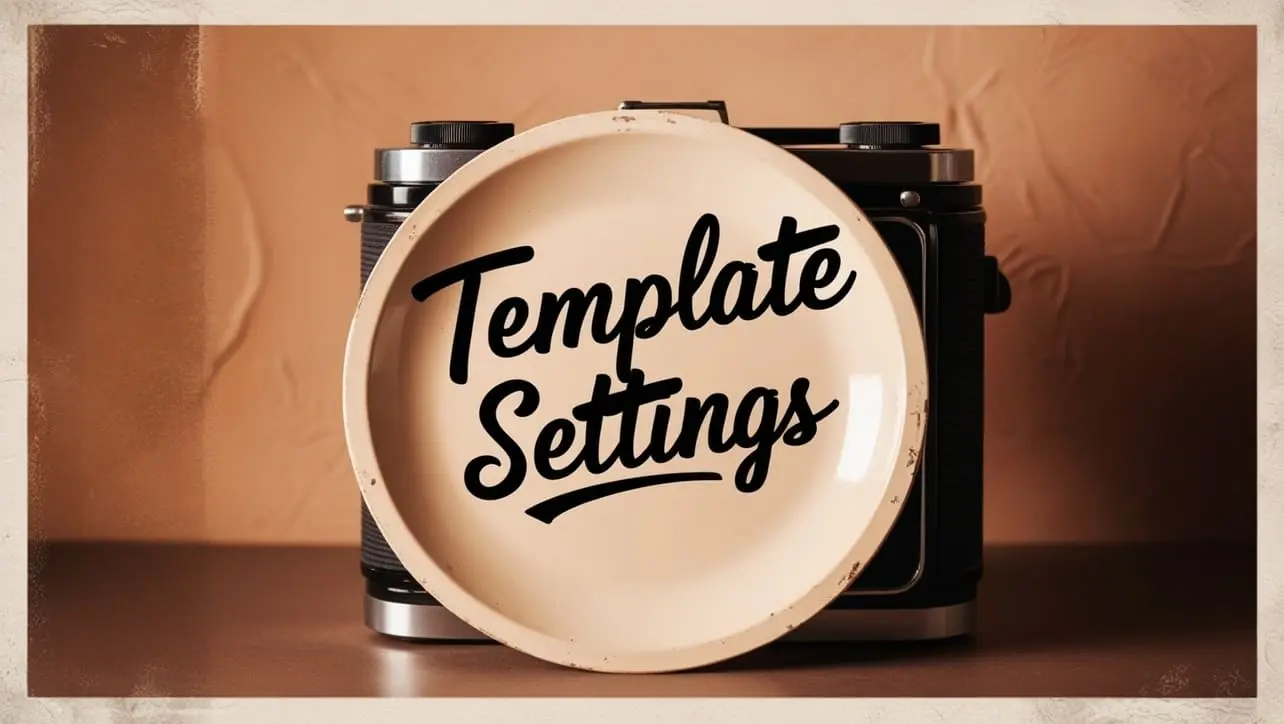
Lodash _.templateSettings Property
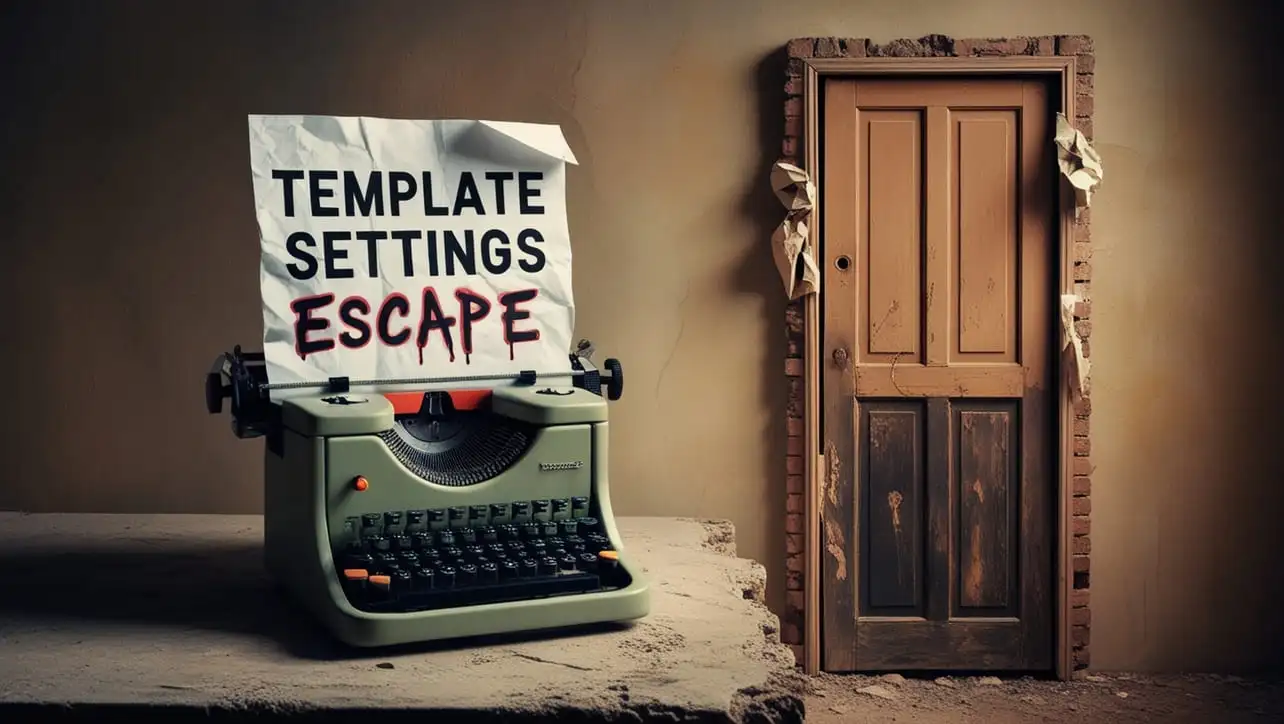
Lodash _.templateSettings.escape Property
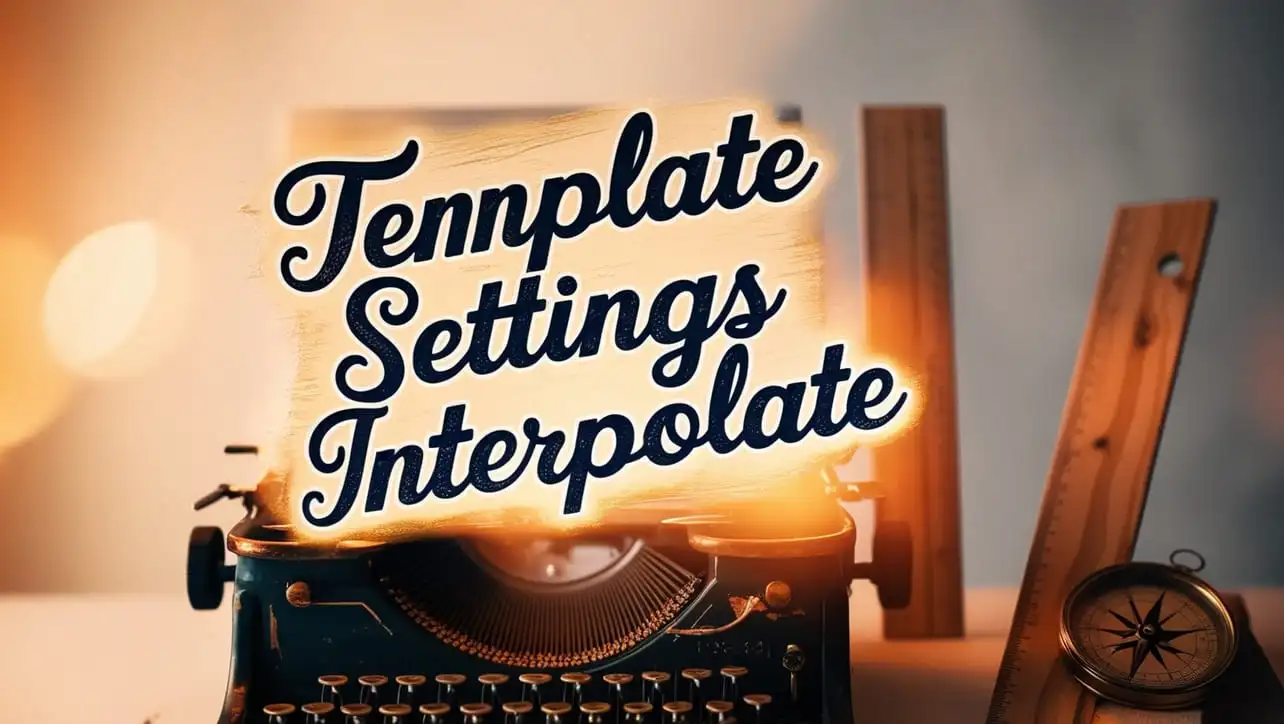
Lodash _.templateSettings.interpolate Property
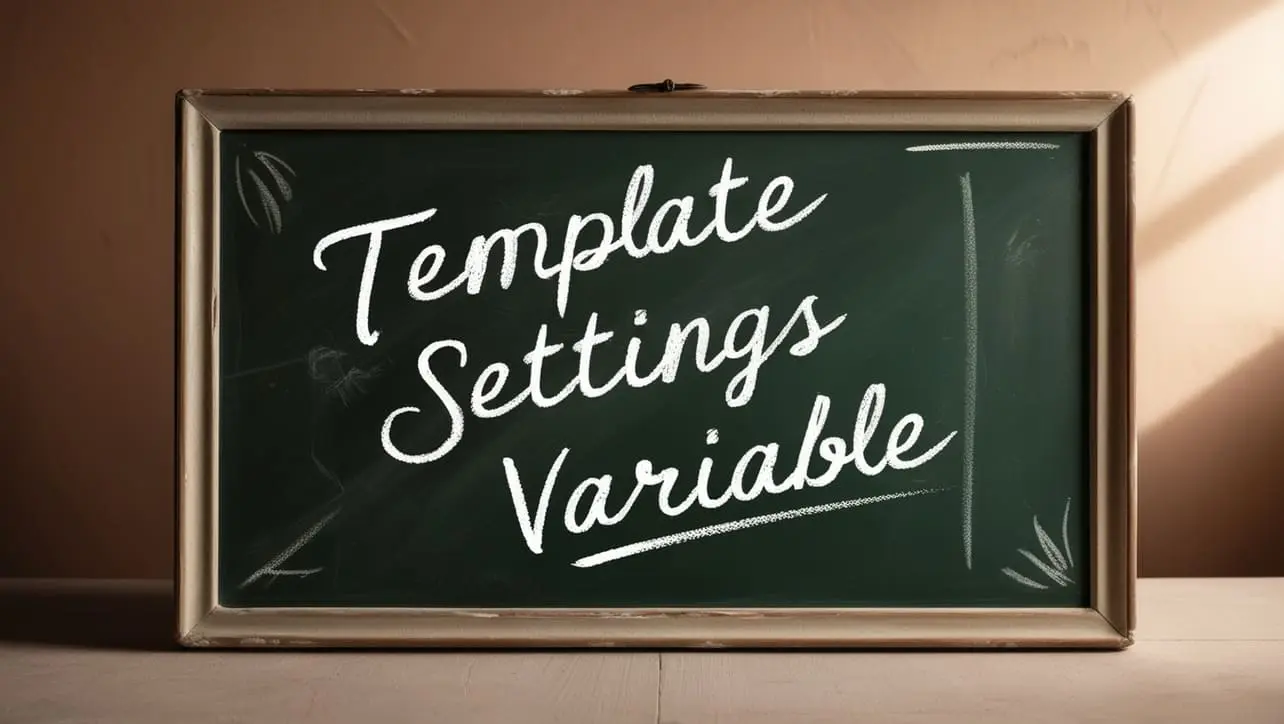
If you have any doubts regarding this article (Lodash _.zipObjectDeep() Array Method), please comment here. I will help you immediately.