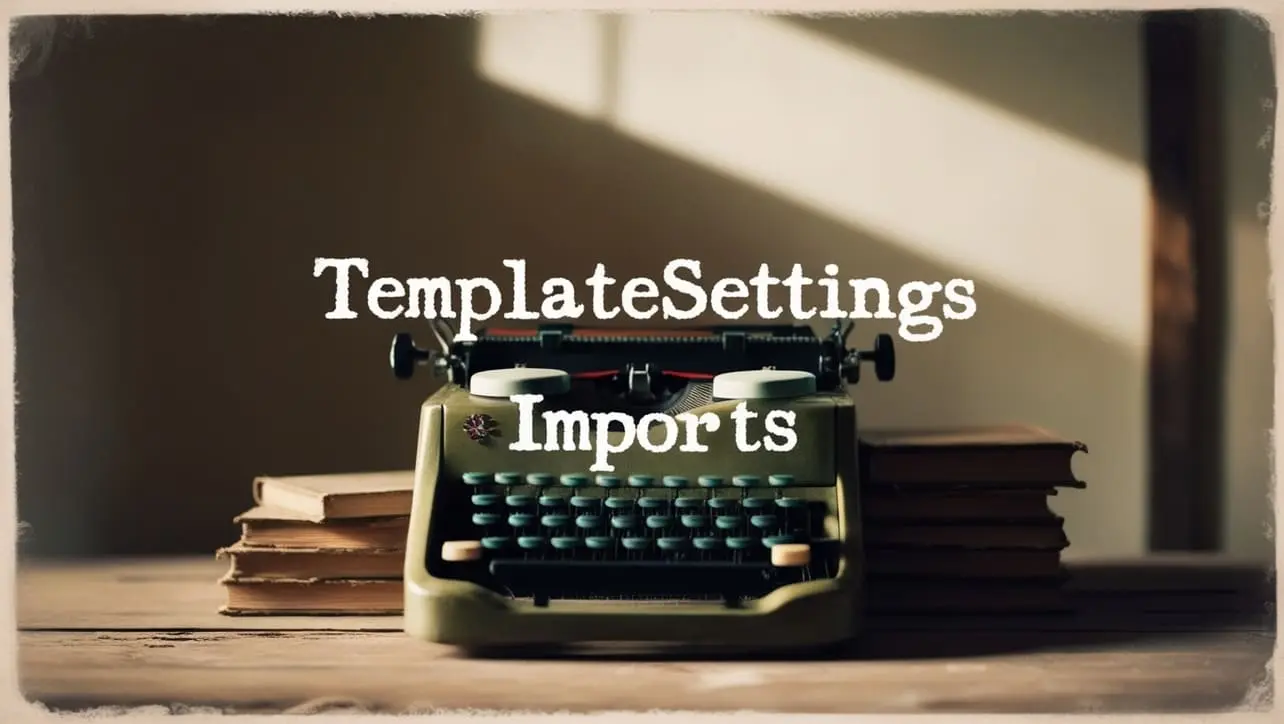
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.zipObject() Array Method
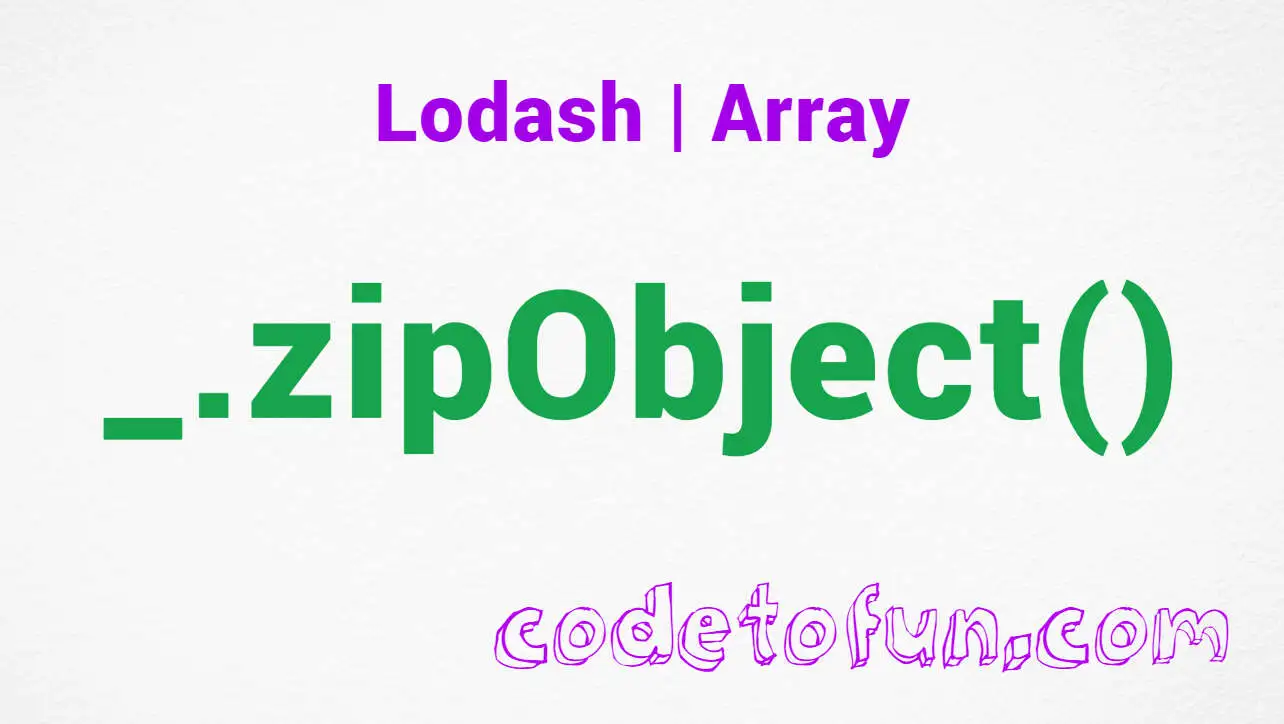
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript development, manipulating arrays is a common task. Lodash, a feature-rich utility library, provides developers with a suite of functions to simplify array operations. One such versatile function is _.zipObject()
, designed to create an object from arrays, where one array represents keys and the other values.
This method is invaluable for streamlining data structures and enhancing code readability.
🧠 Understanding _.zipObject()
The _.zipObject()
method in Lodash allows you to create an object by combining two arrays, one containing keys and the other values. This is particularly useful when dealing with parallel arrays or when you need to transform data from one format to another.
💡 Syntax
_.zipObject([keys], [values])
- keys: An array representing the keys of the resulting object.
- values: An array representing the values associated with the keys.
📝 Example
Let's explore a practical example to illustrate the utility of _.zipObject()
:
const _ = require('lodash');
const keys = ['name', 'age', 'city'];
const values = ['John Doe', 30, 'New York'];
const personObject = _.zipObject(keys, values);
console.log(personObject);
// Output: { name: 'John Doe', age: 30, city: 'New York' }
In this example, the keys array and values array are combined using _.zipObject()
, resulting in an object with corresponding key-value pairs.
🏆 Best Practices
Arrays of Equal Length:
Ensure that the keys and values arrays are of equal length. Mismatched lengths may lead to unexpected behavior, with excess keys or values being ignored.
example.jsCopiedconst keys = ['name', 'age', 'city']; const values = ['John Doe', 30]; const personObject = _.zipObject(keys, values); console.log(personObject); // Output: { name: 'John Doe', age: 30 } // 'city' is ignored due to mismatched lengths
Handling Duplicates:
Be cautious with arrays containing duplicate keys.
_.zipObject()
does not handle duplicate keys gracefully; the last occurrence takes precedence.example.jsCopiedconst keys = ['name', 'age', 'name']; const values = ['John Doe', 30, 'Jane Doe']; const personObject = _.zipObject(keys, values); console.log(personObject); // Output: { name: 'Jane Doe', age: 30 } // 'name' is overridden by the last occurrence
Object Property Shorthand:
Leverage object property shorthand when the keys match variable names. This enhances code readability and conciseness.
example.jsCopiedconst name = 'John Doe'; const age = 30; const city = 'New York'; const personObject = _.zipObject(['name', 'age', 'city'], [name, age, city]); console.log(personObject); // Output: { name: 'John Doe', age: 30, city: 'New York' }
📚 Use Cases
Data Transformation:
_.zipObject()
is ideal for transforming data from parallel arrays into a structured object, providing a clean and readable representation.example.jsCopiedconst properties = ['color', 'size', 'type']; const values = ['red', 'medium', 'shirt']; const productDetails = _.zipObject(properties, values); console.log(productDetails); // Output: { color: 'red', size: 'medium', type: 'shirt' }
Dynamic Object Creation:
When dealing with dynamic data where keys and values may change,
_.zipObject()
can dynamically create objects based on provided arrays.example.jsCopiedconst dynamicKeys = ['firstName', 'lastName', 'email']; const dynamicValues = ['John', 'Doe', 'john.doe@example.com']; const dynamicObject = _.zipObject(dynamicKeys, dynamicValues); console.log(dynamicObject); // Output: { firstName: 'John', lastName: 'Doe', email: 'john.doe@example.com' }
Form Data Processing:
In scenarios involving form data,
_.zipObject()
can be employed to structure the input, making it easier to handle and manipulate.example.jsCopiedconst formDataKeys = ['username', 'password', 'email']; const formDataValues = /* ...retrieve form data values... */; const formDataObject = _.zipObject(formDataKeys, formDataValues); console.log(formDataObject); // Output: { username: '...', password: '...', email: '...' }
🎉 Conclusion
The _.zipObject()
method in Lodash is a versatile tool for creating objects from parallel arrays, providing an elegant solution for data transformation and dynamic object creation. By incorporating this method into your code, you can enhance the structure and readability of your JavaScript projects.
Explore the capabilities of _.zipObject()
and unlock a streamlined approach to array-to-object conversion in your applications!
👨💻 Join our Community:
Author
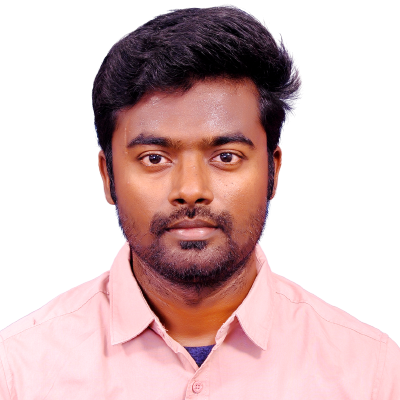
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
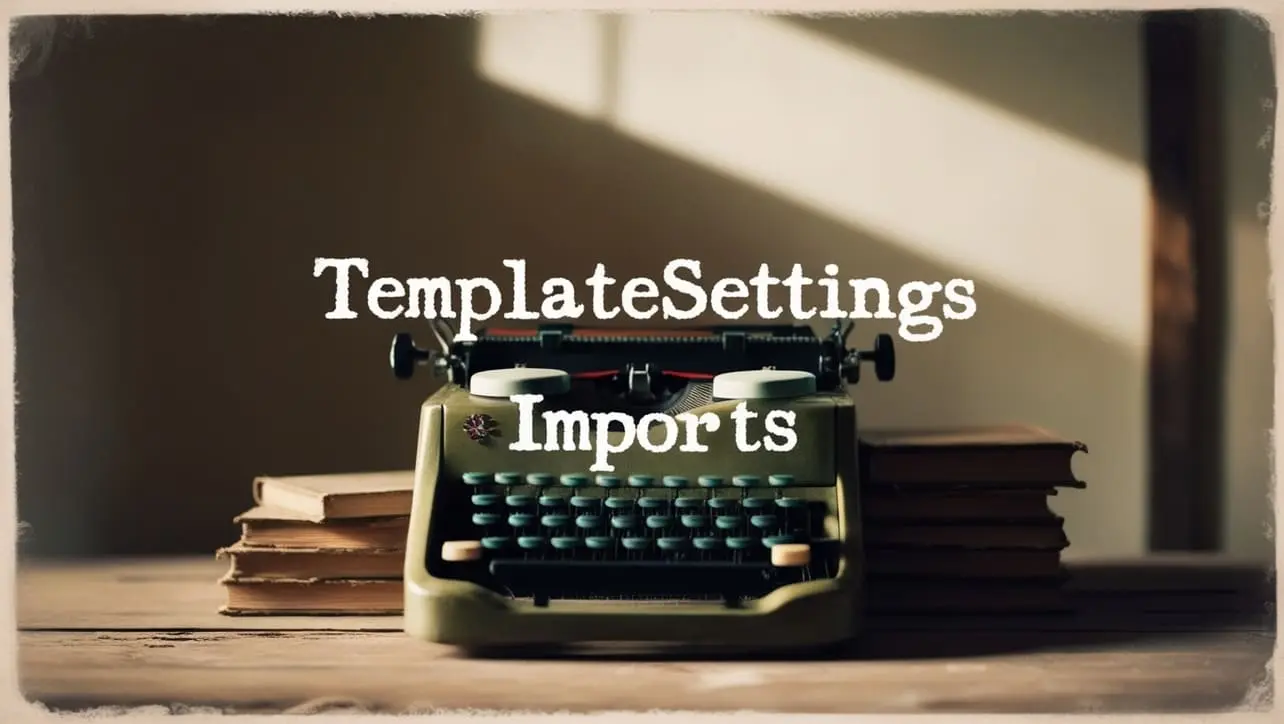
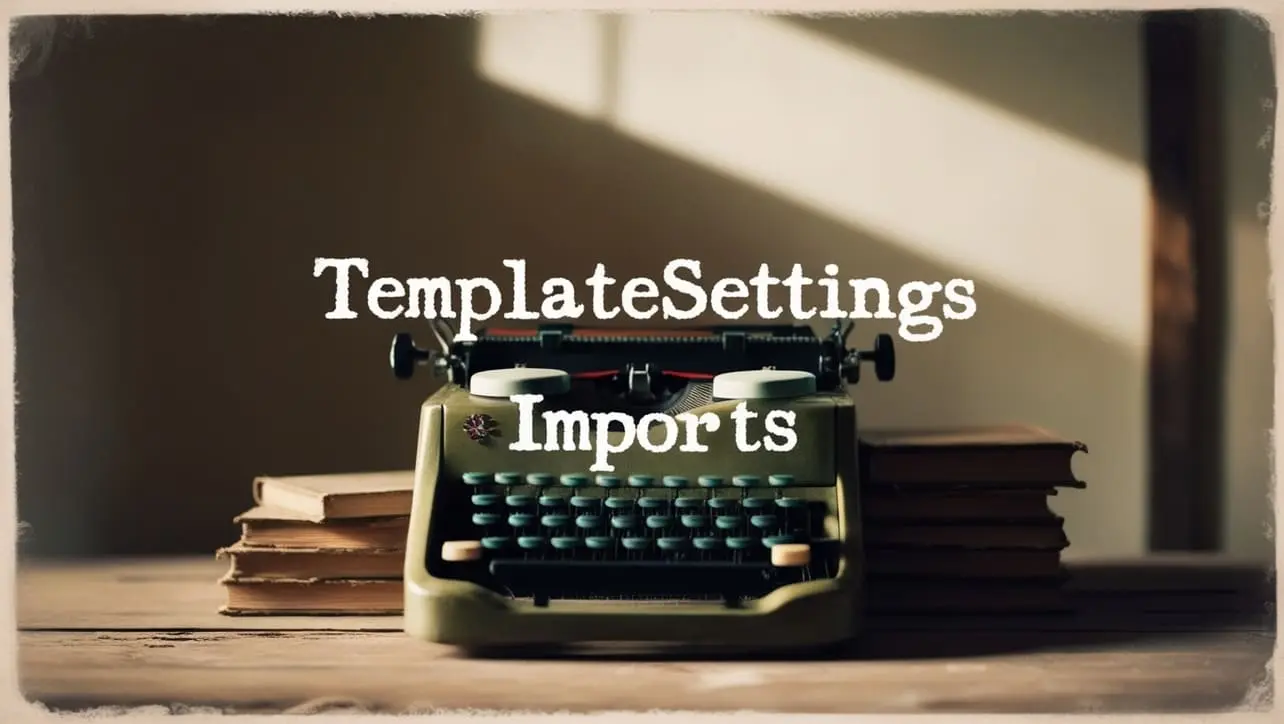
Lodash _.templateSettings.imports Property
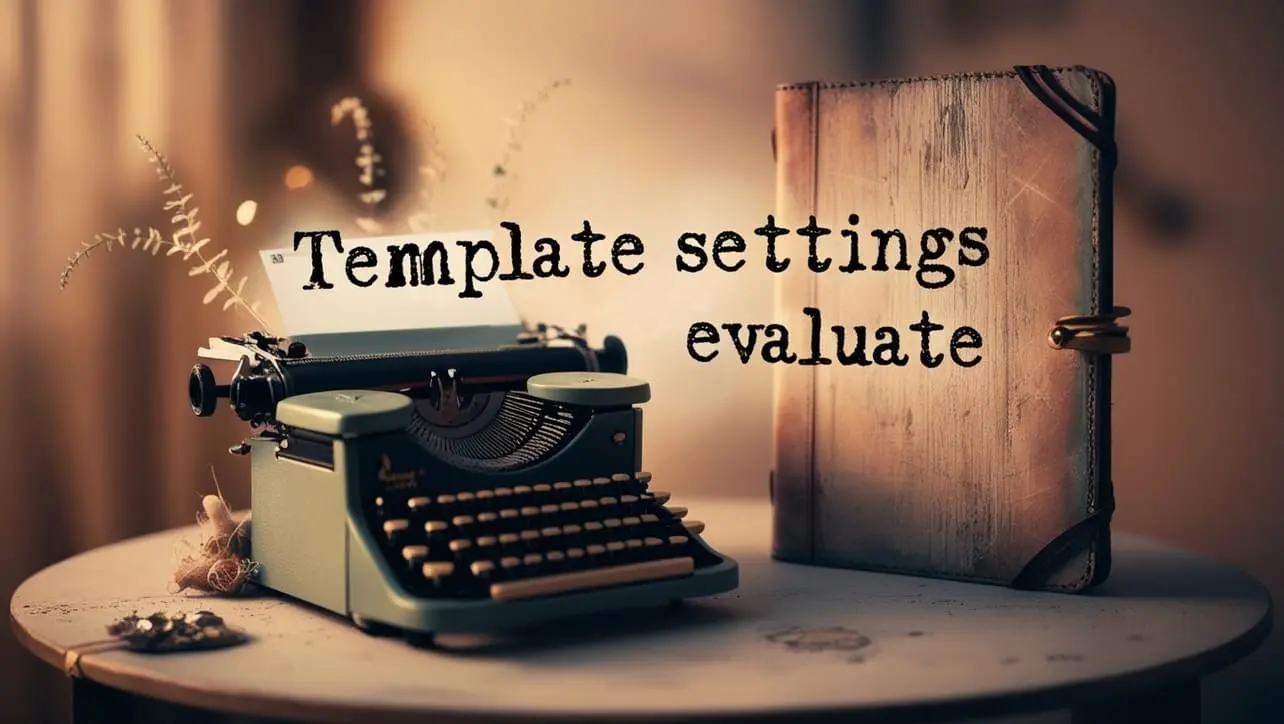
Lodash _.templateSettings.evaluate Property
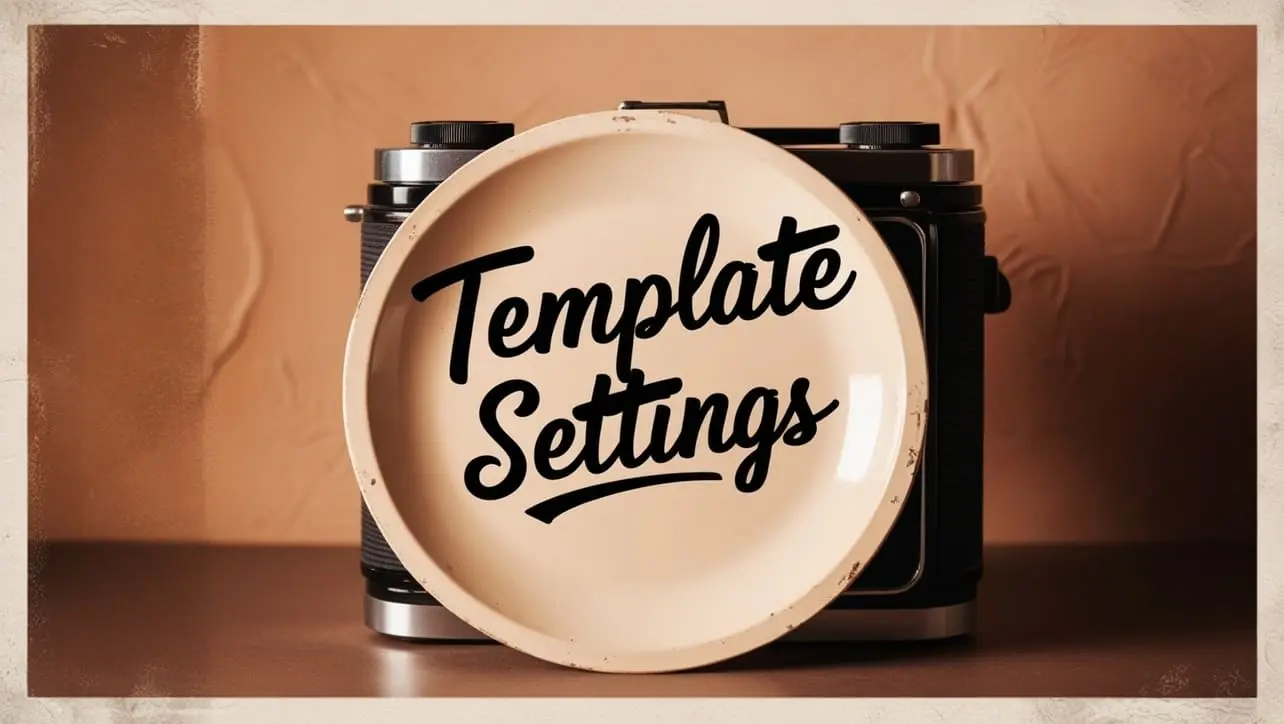
Lodash _.templateSettings Property
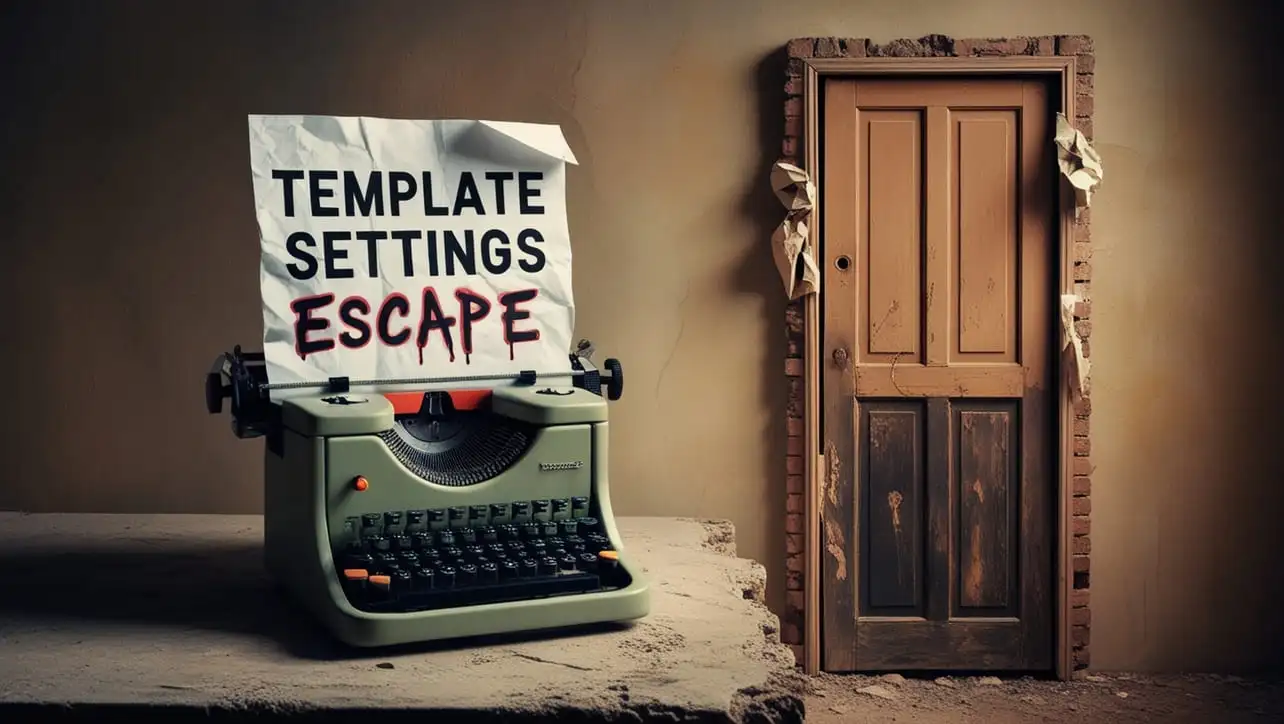
Lodash _.templateSettings.escape Property
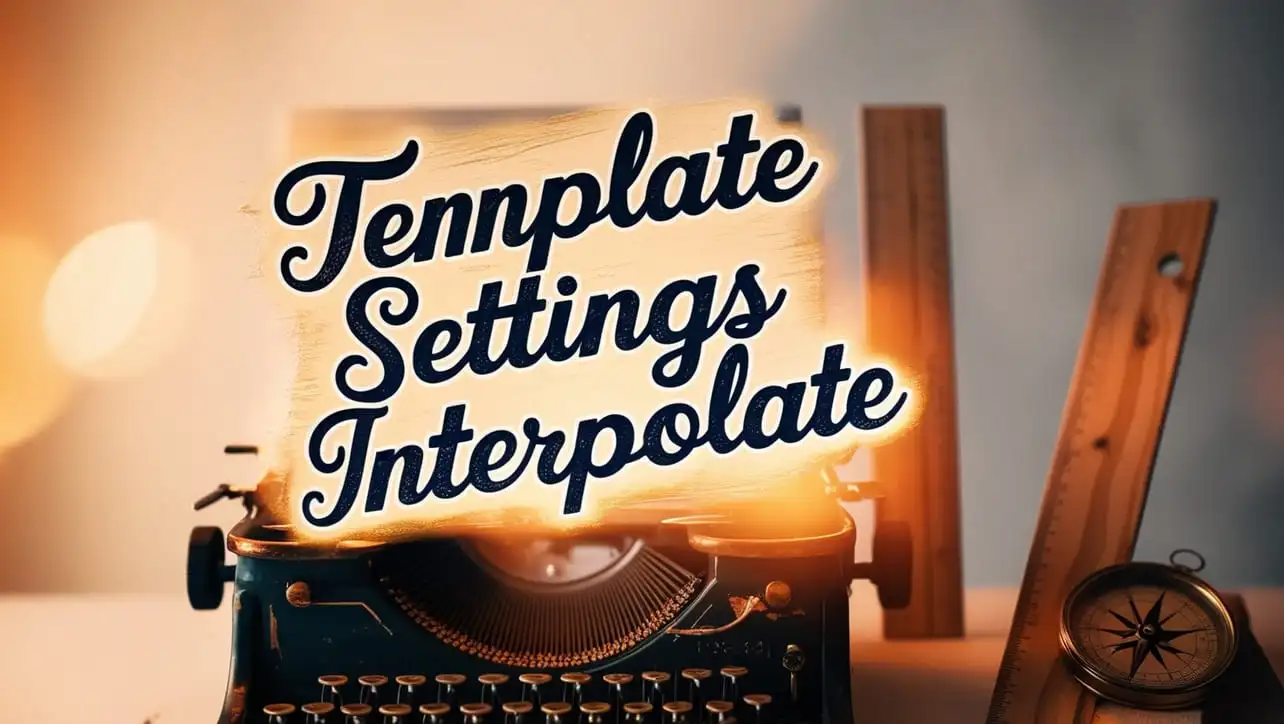
Lodash _.templateSettings.interpolate Property
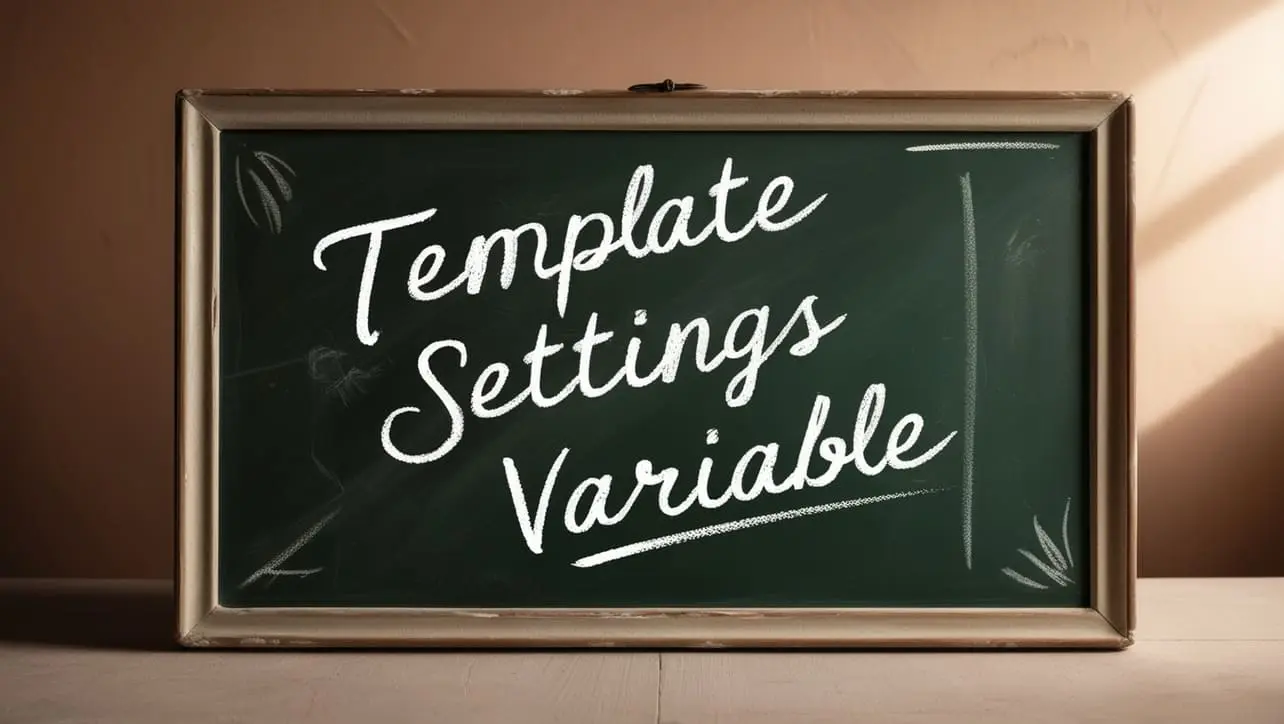
If you have any doubts regarding this article (Lodash _.zipObject() Array Method), please comment here. I will help you immediately.