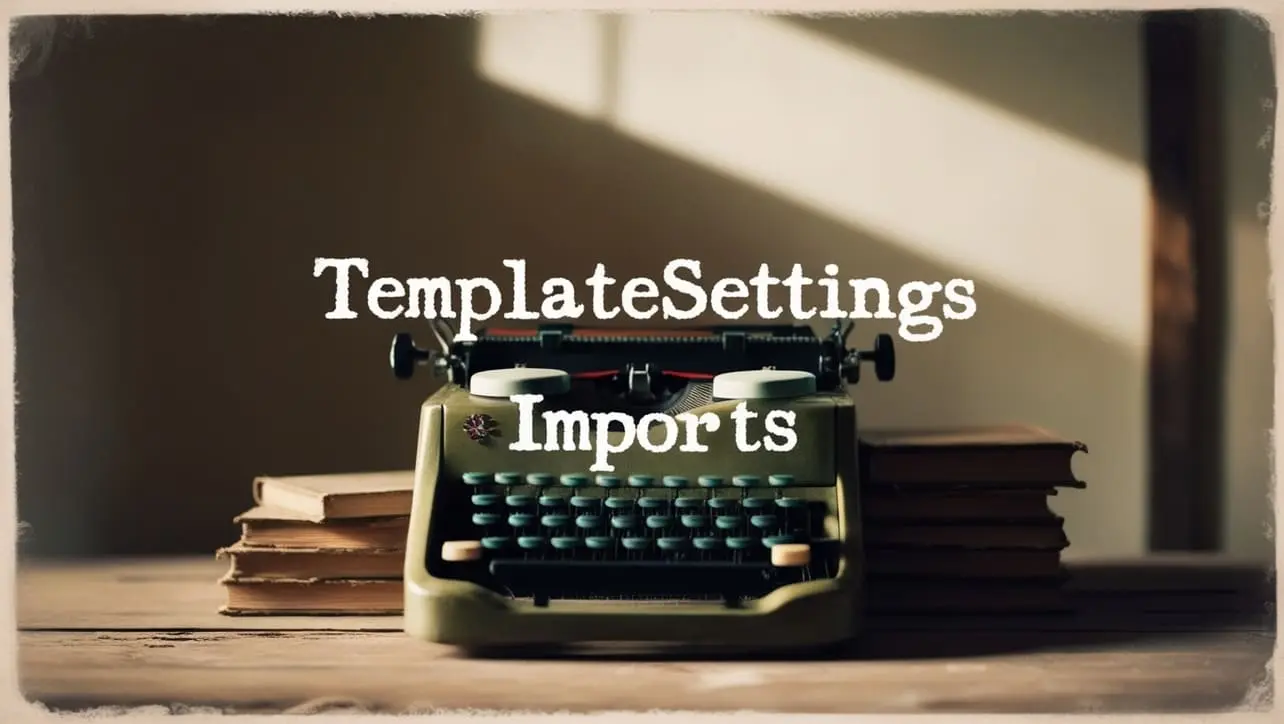
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.xorBy() Array Method
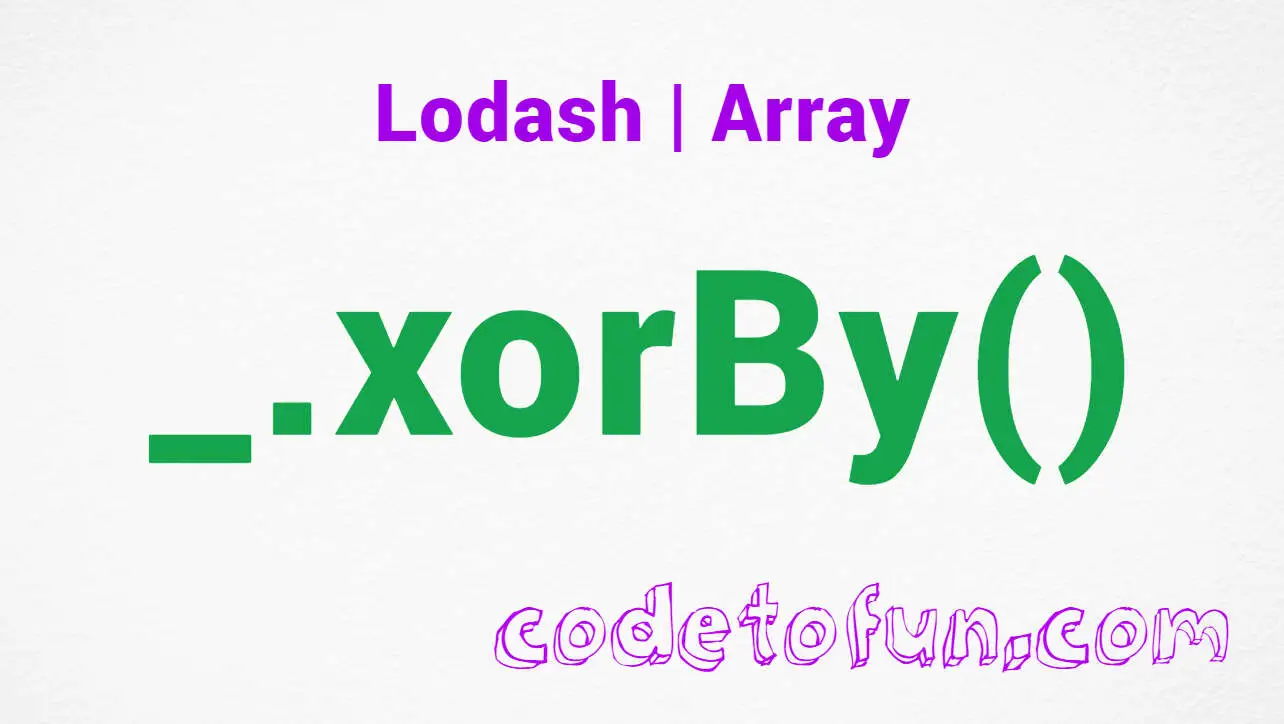
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, manipulating arrays efficiently is a common challenge. Lodash, a comprehensive utility library, provides an arsenal of functions to simplify these tasks. One such function is _.xorBy()
, a method designed to compute the symmetric difference of arrays based on a custom iteratee function.
This method enhances the process of array comparison, making it a valuable tool for developers dealing with complex data structures.
🧠 Understanding _.xorBy()
The _.xorBy()
method in Lodash is employed to obtain the symmetric difference of arrays based on a provided iteratee function. This iteratee function allows developers to define custom criteria for comparing elements, providing flexibility in array manipulation.
💡 Syntax
_.xorBy([arrays], iteratee)
- arrays: The arrays to inspect.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a practical example to understand the functionality of _.xorBy()
:
const _ = require('lodash');
const array1 = [1, 2, 3];
const array2 = [2, 3, 4];
const customIteratee = value => value % 2;
const symmetricDifference = _.xorBy(array1, array2, customIteratee);
console.log(symmetricDifference);
// Output: [1, 4]
In this example, the symmetric difference of array1 and array2 is computed based on the custom criteria defined by the customIteratee function.
🏆 Best Practices
Understanding Symmetric Difference:
Grasp the concept of symmetric difference in set theory to fully utilize
_.xorBy()
. Symmetric difference represents elements that are present in either of the arrays, but not in both.example.jsCopiedconst setA = [1, 2, 3]; const setB = [2, 3, 4]; const symmetricDifferenceAB = _.xorBy(setA, setB); console.log(symmetricDifferenceAB); // Output: [1, 4]
Define Custom Criteria:
Leverage the power of custom criteria by utilizing the iteratee function. This allows you to tailor the comparison logic to specific attributes or conditions.
example.jsCopiedconst arrayA = [{ id: 1, name: 'apple' }, { id: 2, name: 'banana' }]; const arrayB = [{ id: 2, name: 'banana' }, { id: 3, name: 'orange' }]; const customCriteria = obj => obj.name; const symmetricDifferenceObjects = _.xorBy(arrayA, arrayB, customCriteria); console.log(symmetricDifferenceObjects); // Output: [{ id: 1, name: 'apple' }, { id: 3, name: 'orange' }]
Input Validation:
Ensure the input arrays are valid before applying
_.xorBy()
. This includes checking for the presence of arrays and confirming that the iteratee function is specified.example.jsCopiedconst invalidArrays = _.xorBy(undefined, null, value => value); console.log(invalidArrays); // Output: []
📚 Use Cases
Finding Unique Elements:
Identify unique elements across multiple arrays using
_.xorBy()
. This is particularly useful when dealing with datasets containing duplicate values.example.jsCopiedconst dataset1 = [1, 2, 3, 4]; const dataset2 = [3, 4, 5, 6]; const uniqueValues = _.xorBy(dataset1, dataset2); console.log(uniqueValues); // Output: [1, 2, 5, 6]
Handling Array Differences in Objects:
When working with arrays of objects,
_.xorBy()
can be applied to find objects with distinct attributes based on a custom criteria.example.jsCopiedconst arraySet1 = [{ id: 1, name: 'apple' }, { id: 2, name: 'banana' }]; const arraySet2 = [{ id: 2, name: 'banana' }, { id: 3, name: 'orange' }]; const uniqueObjects = _.xorBy(arraySet1, arraySet2, obj => obj.name); console.log(uniqueObjects); // Output: [{ id: 1, name: 'apple' }, { id: 3, name: 'orange' }]
Symmetric Difference with Custom Logic:
Employ
_.xorBy()
to find the symmetric difference based on a custom logic or attribute of the elements.example.jsCopiedconst setX = [1, 2, 3, 4, 5]; const setY = [4, 5, 6, 7, 8]; const symmetricDifferenceCustomLogic = _.xorBy(setX, setY, value => value % 2); console.log(symmetricDifferenceCustomLogic); // Output: [1, 2, 6, 7, 8]
🎉 Conclusion
The _.xorBy()
method in Lodash provides a powerful solution for computing the symmetric difference of arrays based on a custom iteratee function. Whether you're working with simple datasets or complex data structures, this method offers a versatile tool for array manipulation in JavaScript.
Unlock the potential of array comparison with _.xorBy()
and enhance your JavaScript development experience!
👨💻 Join our Community:
Author
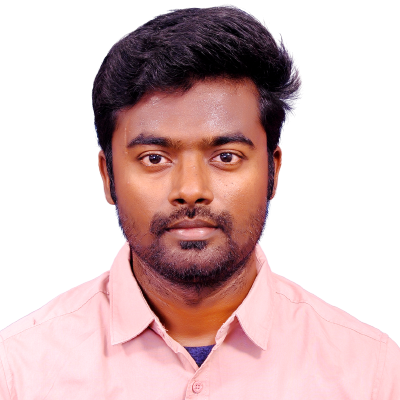
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
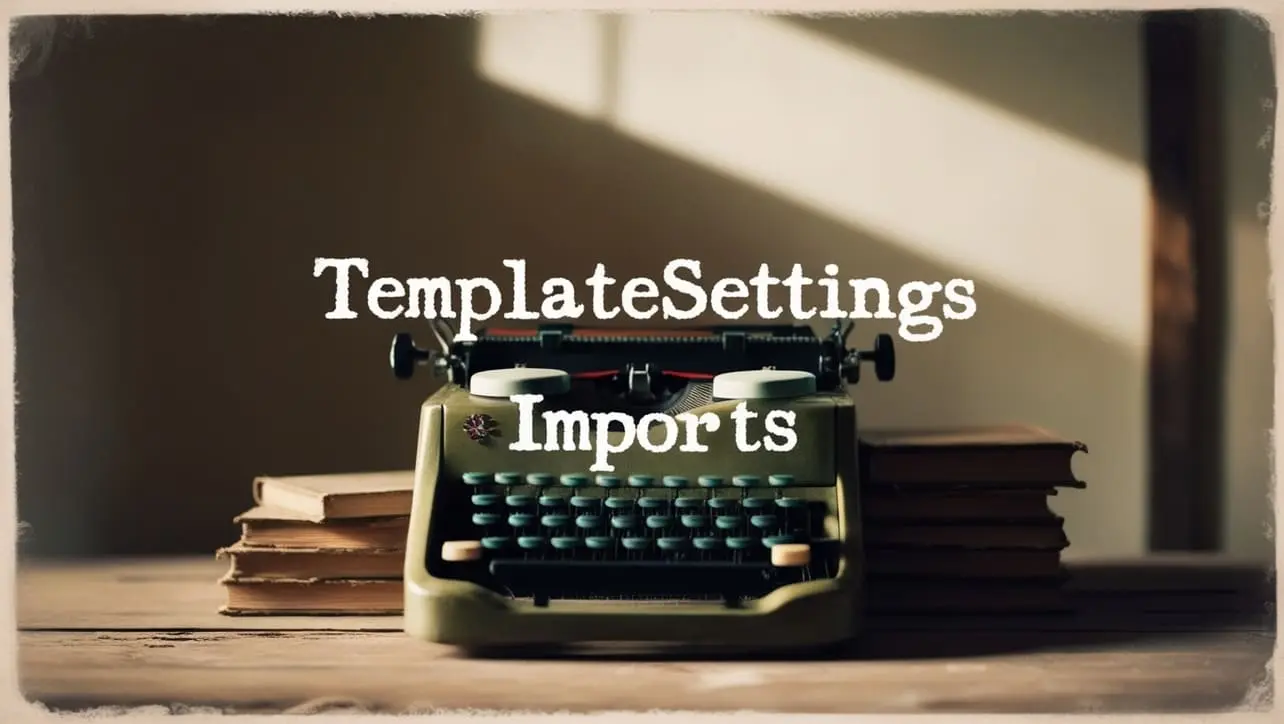
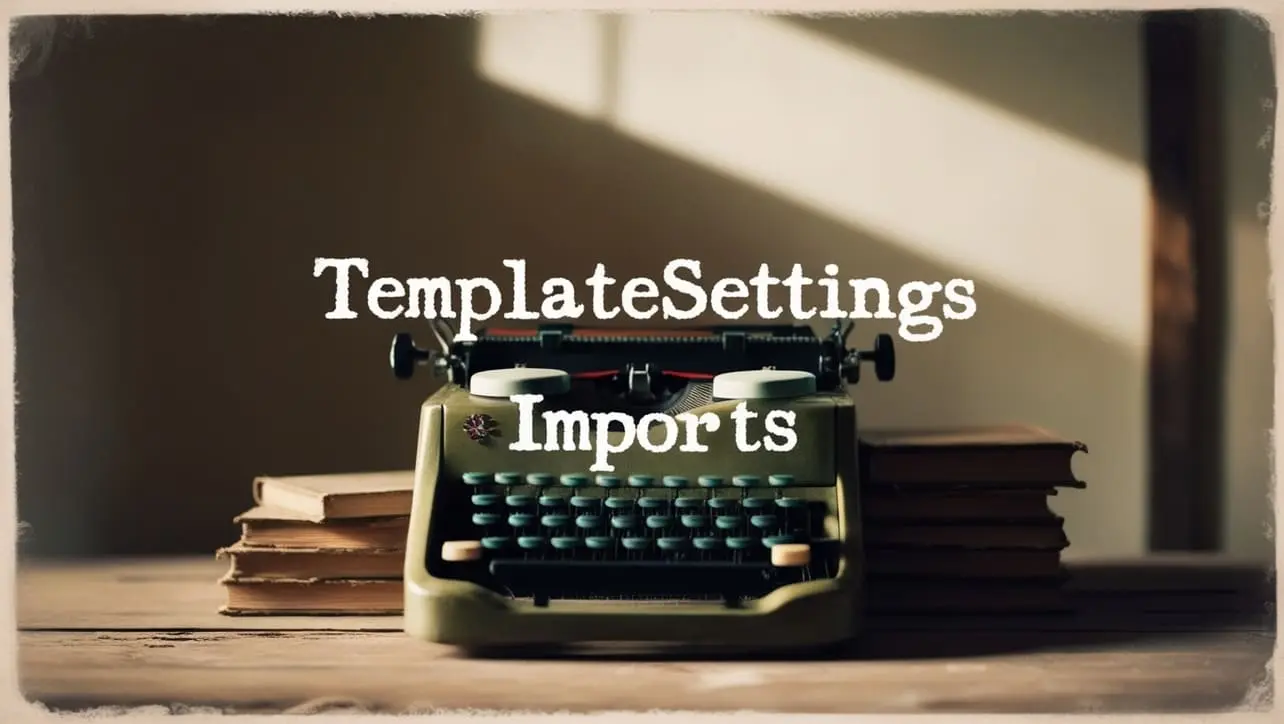
Lodash _.templateSettings.imports Property
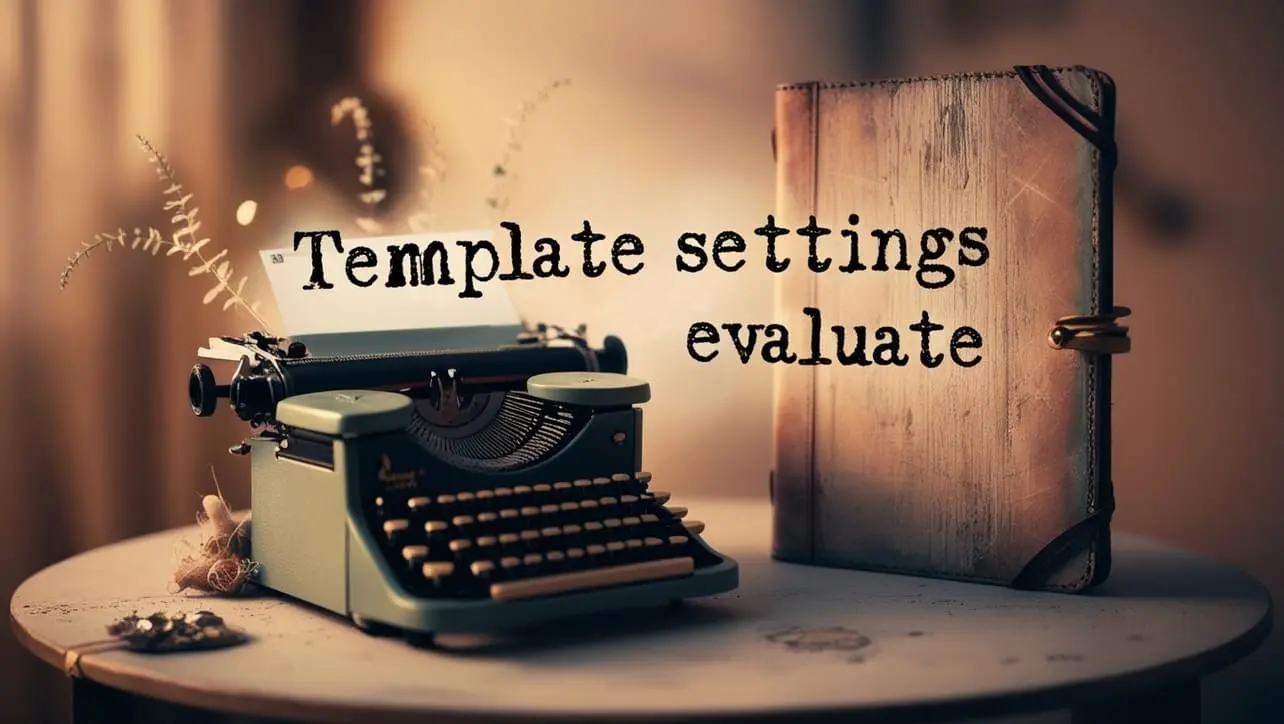
Lodash _.templateSettings.evaluate Property
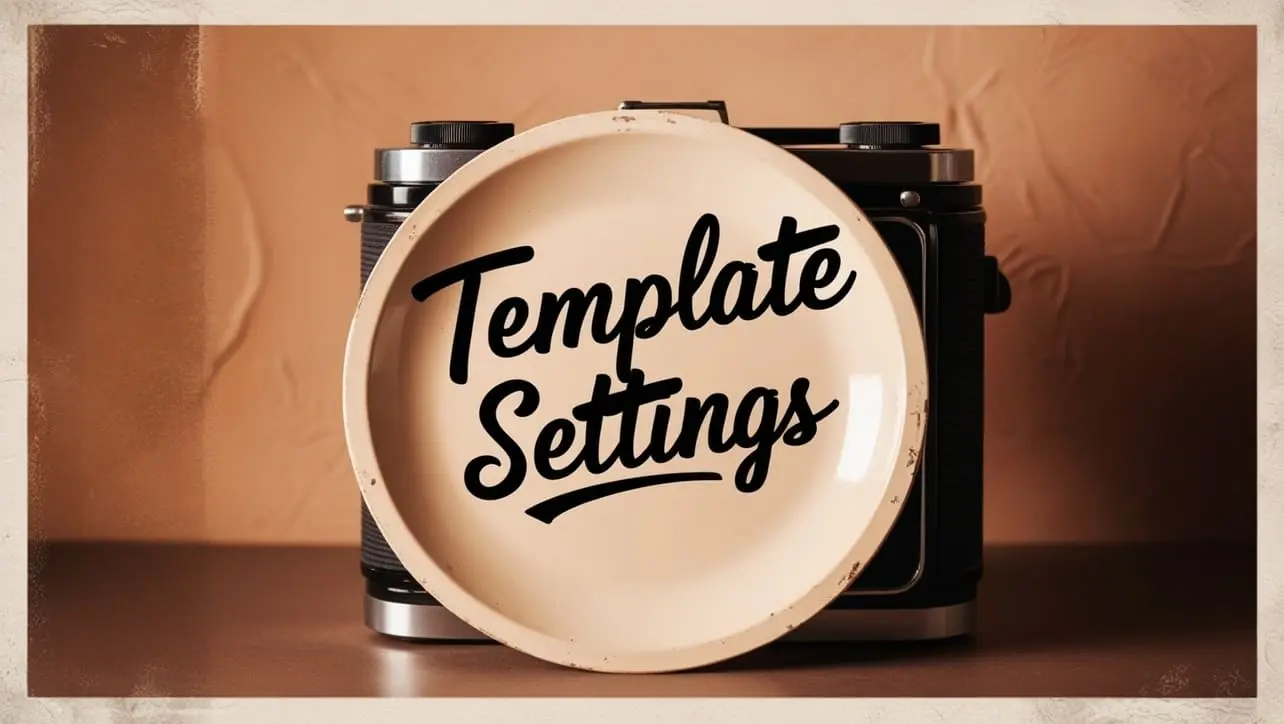
Lodash _.templateSettings Property
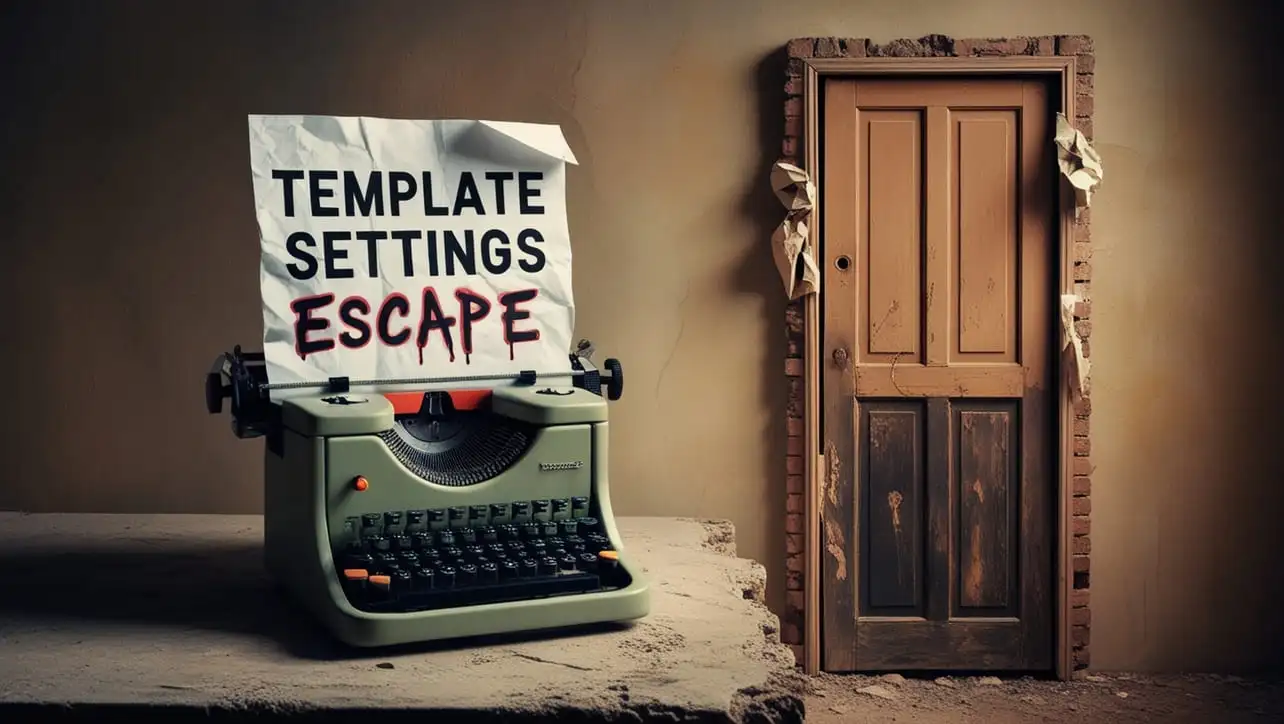
Lodash _.templateSettings.escape Property
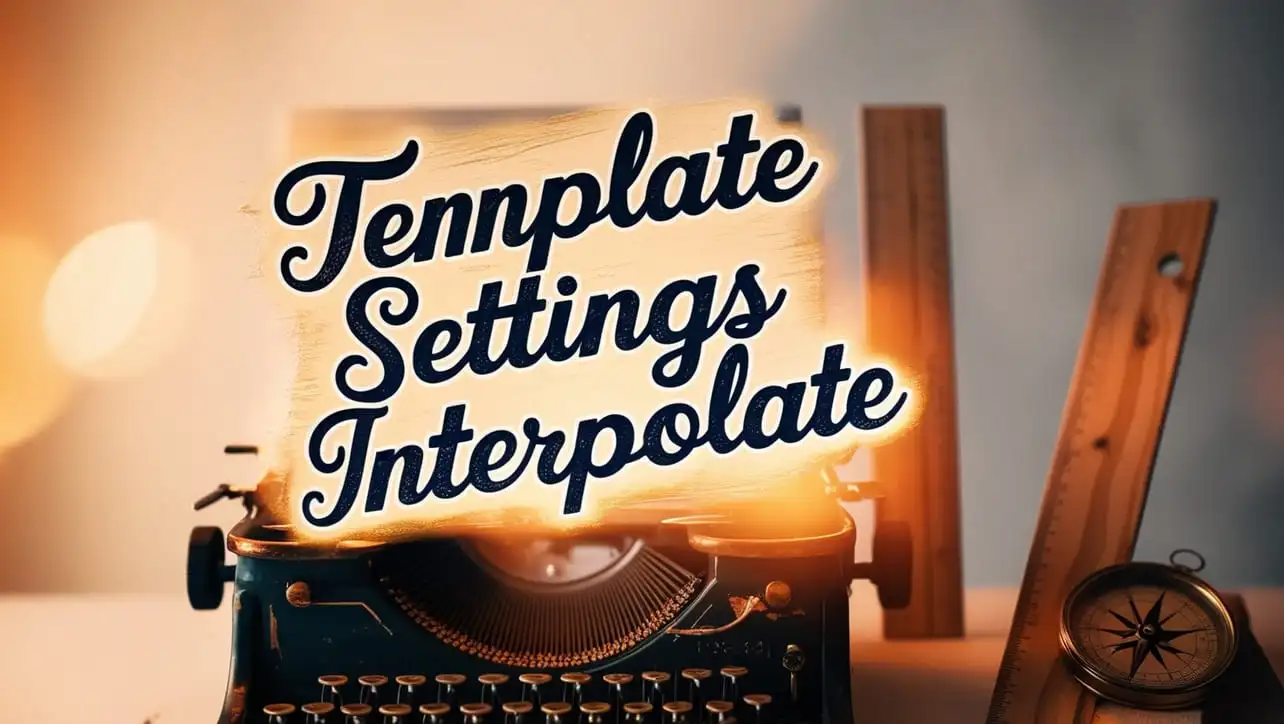
Lodash _.templateSettings.interpolate Property
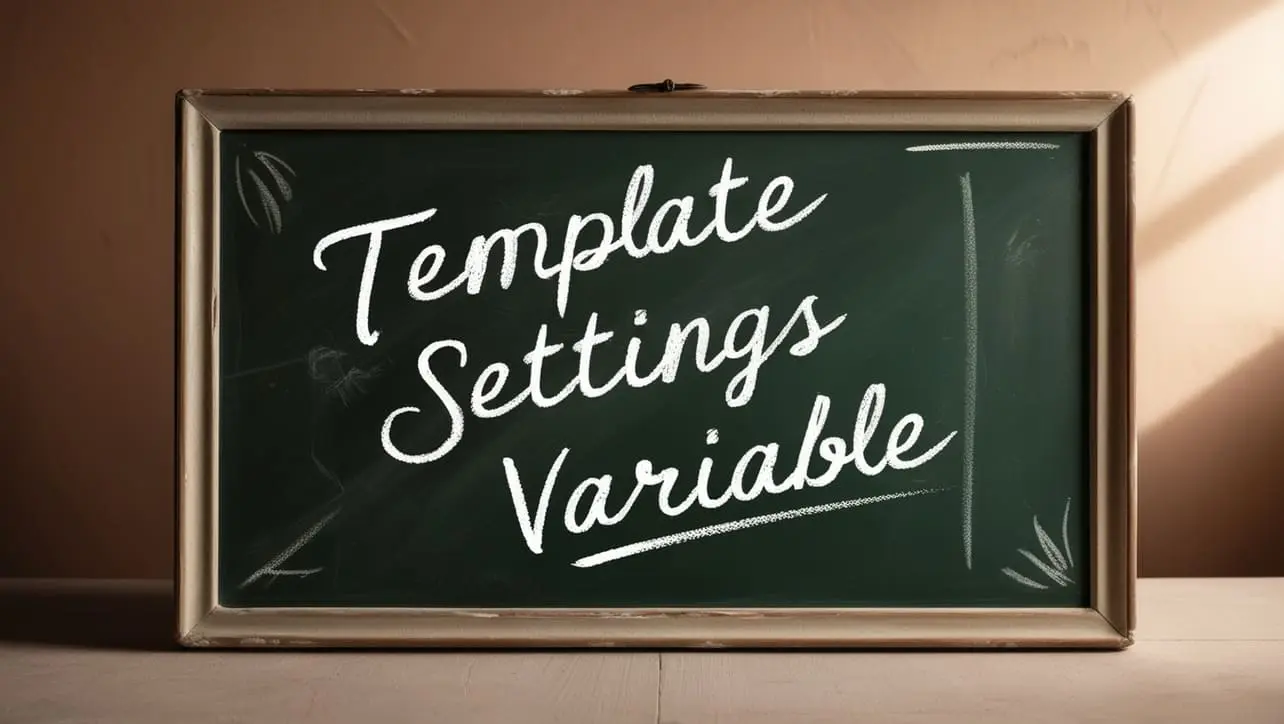
If you have any doubts regarding this article (Lodash _.xorBy() Array Method), please comment here. I will help you immediately.