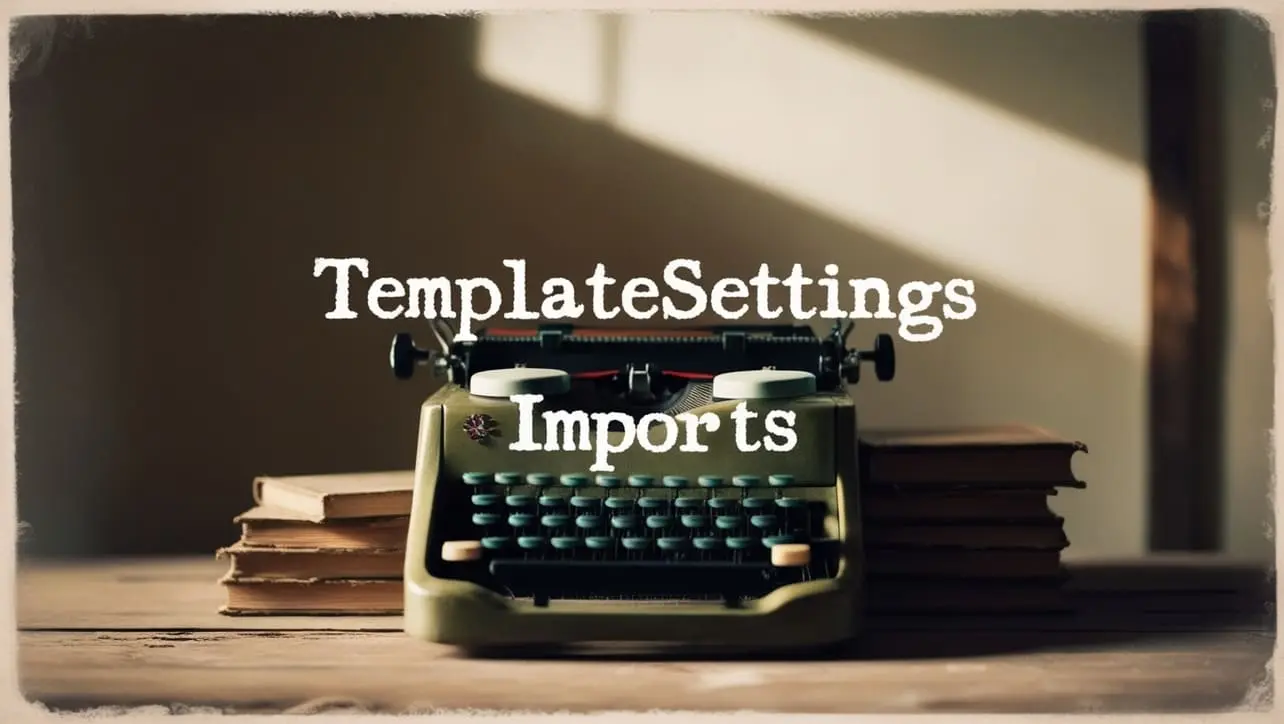
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.without() Array Method
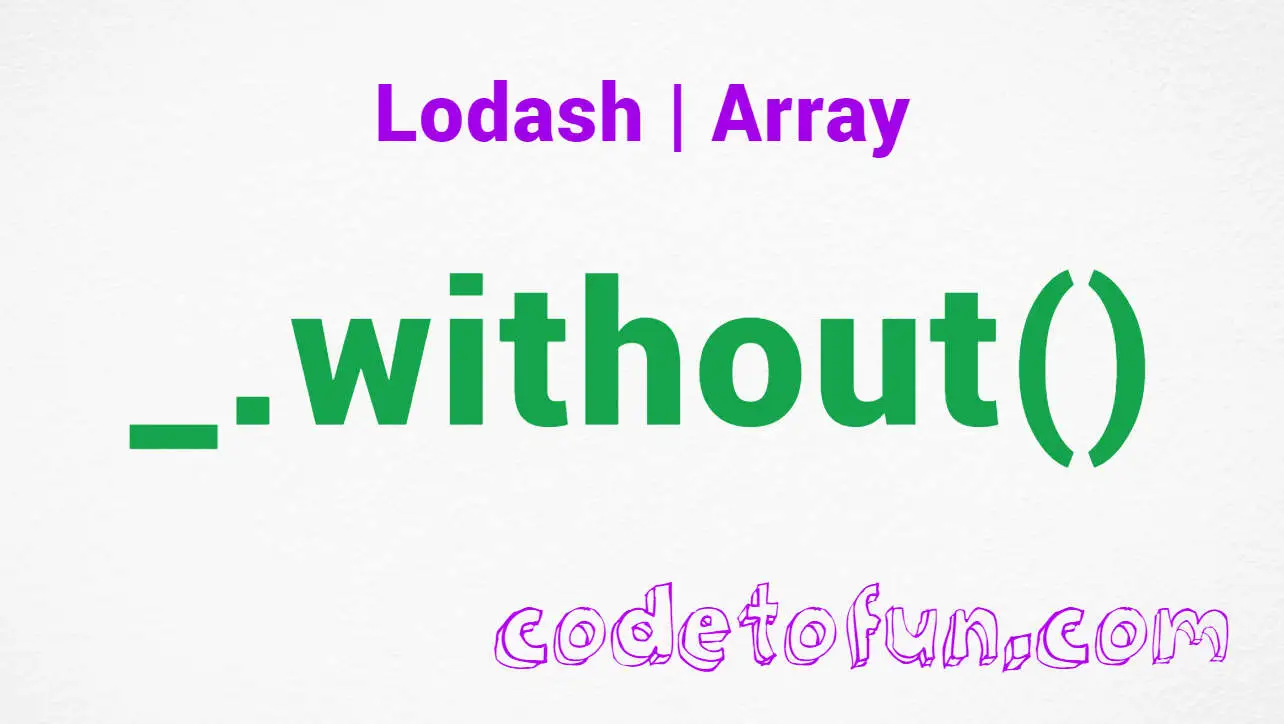
Photo Credit to CodeToFun
🙋 Introduction
Efficiently manipulating arrays is a fundamental aspect of JavaScript programming. In the expansive toolkit of array functions provided by Lodash, the _.without()
method stands out. This method empowers developers to effortlessly create new arrays by excluding specified values.
Whether you're filtering out undesired elements or simplifying your data, _.without()
proves to be a valuable asset in your array manipulation arsenal.
🧠 Understanding _.without()
The _.without()
method in Lodash facilitates the creation of a new array that excludes specified values. This can be immensely useful when you need to filter out certain elements from an array, providing a clean and concise way to manage your data.
💡 Syntax
_.without(array, [values])
- array: The array to process.
- values: The values to exclude from the new array.
📝 Example
Let's explore a practical example to grasp the functionality of _.without()
:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const newArray = _.without(originalArray, 3, 5);
console.log(newArray);
// Output: [1, 2, 4]
In this example, the originalArray is processed by _.without()
, resulting in a new array that excludes the specified values (3 and 5).
🏆 Best Practices
Use Case-Specific Exclusion:
Tailor the
_.without()
method to your use case by excluding values that are specific to your application requirements. This ensures that the resulting array aligns with your intended data structure.example.jsCopiedconst data = ['apple', 'banana', 'orange', 'kiwi']; const fruitsToExclude = ['orange', 'kiwi']; const filteredFruits = _.without(data, ...fruitsToExclude); console.log(filteredFruits); // Output: ['apple', 'banana']
Consider Immutability:
To maintain immutability in your code and avoid unintended side effects, assign the result of
_.without()
to a new variable rather than modifying the original array in place.example.jsCopiedconst originalArray = [10, 20, 30]; const valueToRemove = 20; const newArray = _.without(originalArray, valueToRemove); console.log(originalArray); // Output: [10, 20, 30] (original array remains unchanged) console.log(newArray); // Output: [10, 30] (new array with specified value excluded)
Array of Values to Exclude:
Take advantage of the ability to pass an array of values to exclude from the original array. This provides flexibility and conciseness in your code.
example.jsCopiedconst data = [1, 2, 3, 4, 5]; const valuesToExclude = [2, 4]; const filteredData = _.without(data, ...valuesToExclude); console.log(filteredData); // Output: [1, 3, 5]
📚 Use Cases
Filtering Unwanted Elements:
_.without()
is ideal for scenarios where you need to filter out specific elements from an array, creating a refined dataset.example.jsCopiedconst userRoles = ['admin', 'user', 'guest']; const rolesToRemove = ['guest']; const filteredRoles = _.without(userRoles, ...rolesToRemove); console.log(filteredRoles); // Output: ['admin', 'user']
Data Cleanup:
When dealing with datasets that may contain undesired values,
_.without()
simplifies the process of cleaning up your data.example.jsCopiedconst messyData = ['apple', null, 'banana', undefined, 'orange']; const valuesToExclude = [null, undefined]; const cleanedData = _.without(messyData, ...valuesToExclude); console.log(cleanedData); // Output: ['apple', 'banana', 'orange']
Dynamic Exclusion:
Utilize the dynamic nature of
_.without()
to exclude values based on runtime conditions or user input.example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const valueToExclude = /* ...get value dynamically... */; const filteredNumbers = _.without(numbers, valueToExclude); console.log(filteredNumbers);
🎉 Conclusion
The _.without()
method in Lodash provides a convenient way to create new arrays by excluding specified values. Whether you're filtering out elements based on specific criteria or cleaning up your data, _.without()
proves to be a versatile and valuable tool in array manipulation.
Explore the capabilities of _.without()
and enhance your JavaScript development experience by streamlining array filtering and data management!
👨💻 Join our Community:
Author
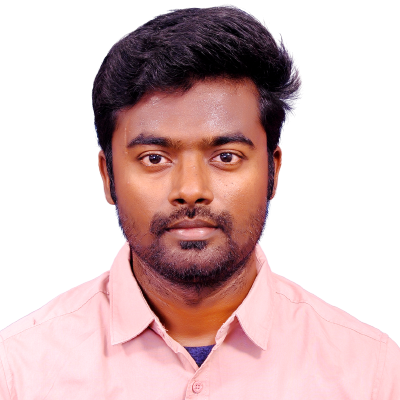
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
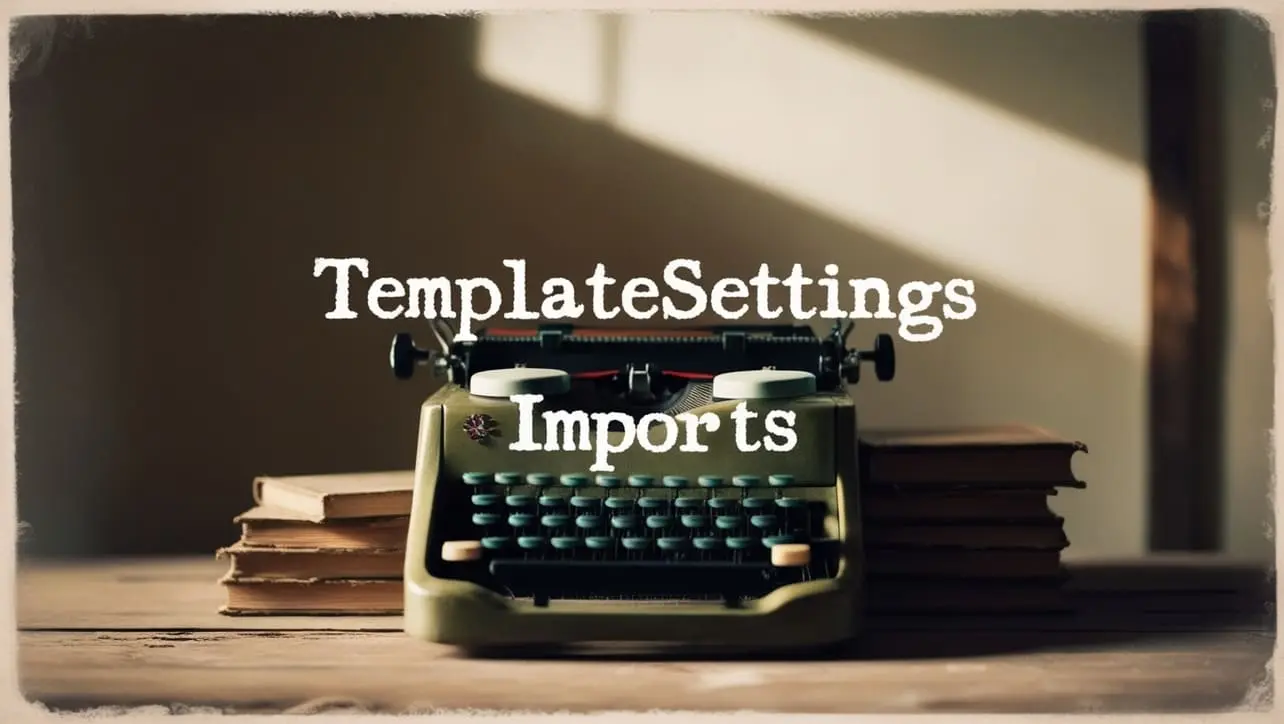
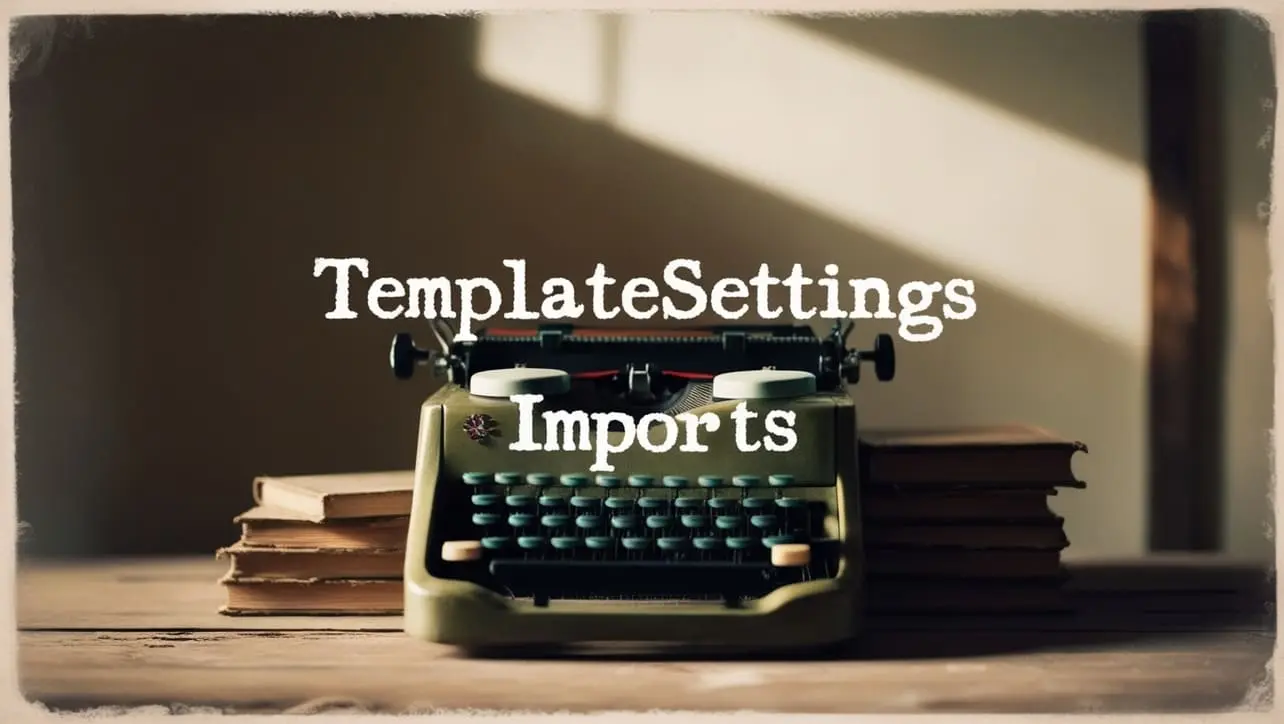
Lodash _.templateSettings.imports Property
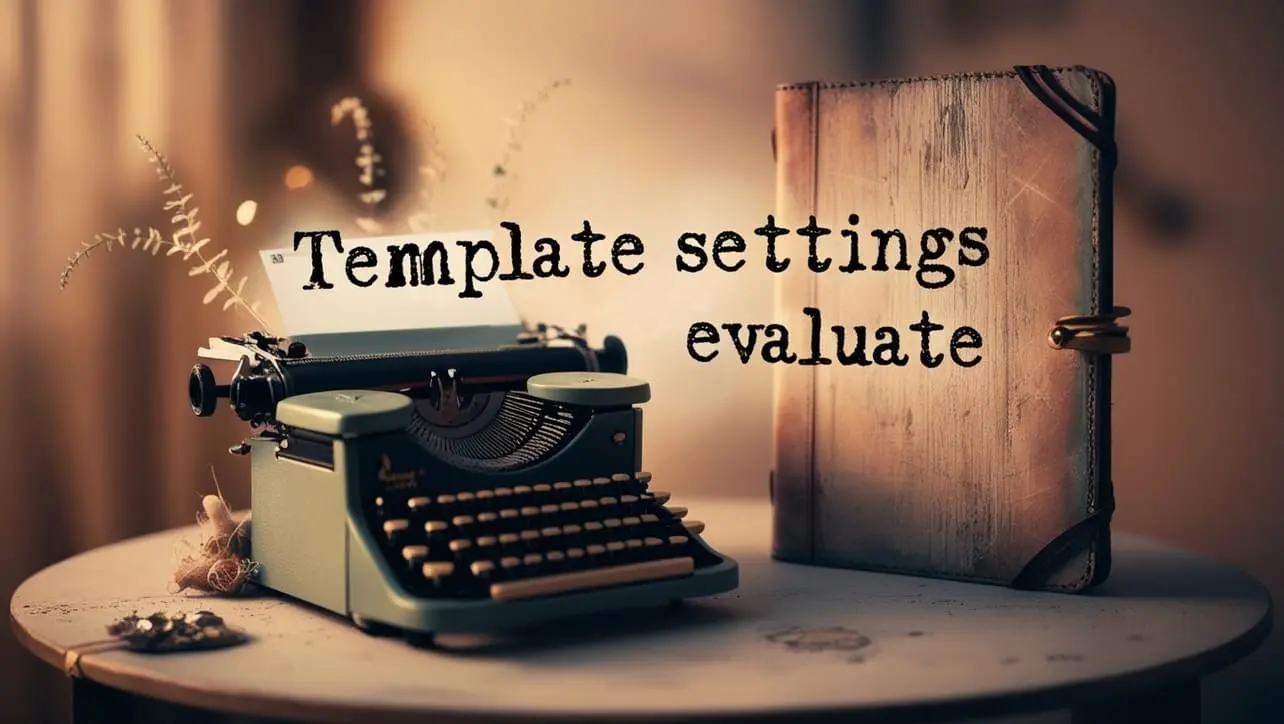
Lodash _.templateSettings.evaluate Property
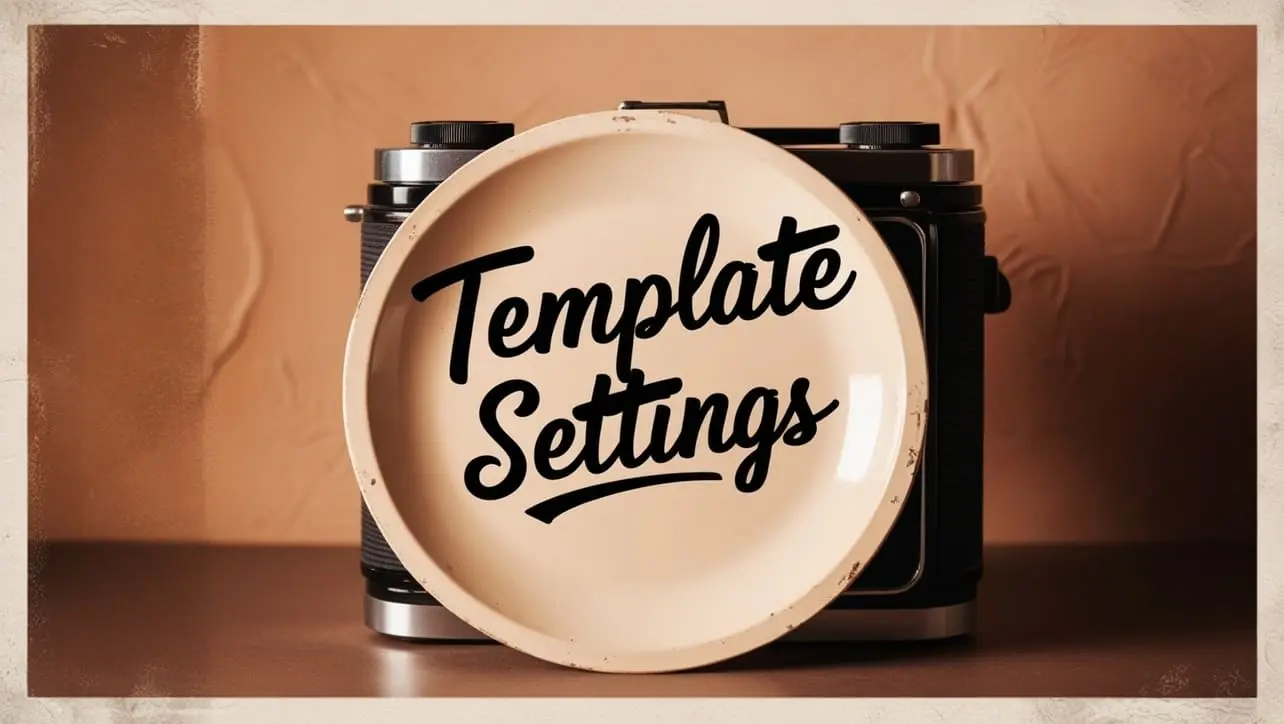
Lodash _.templateSettings Property
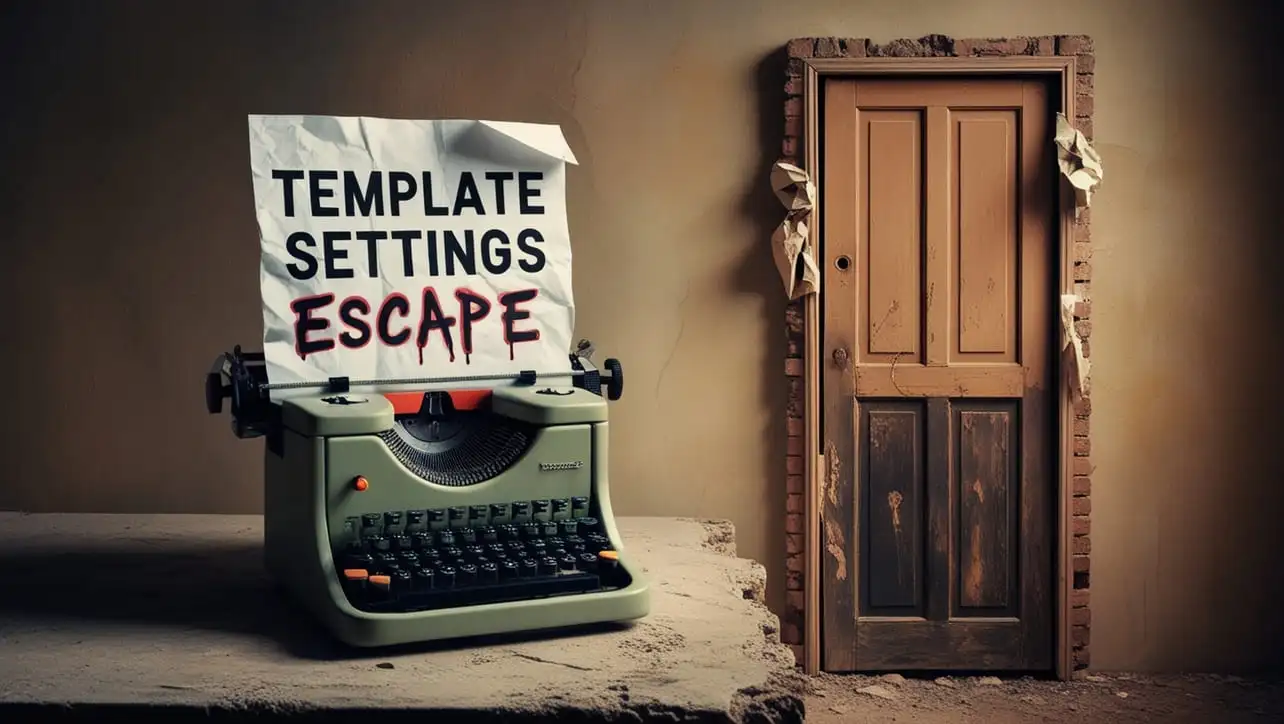
Lodash _.templateSettings.escape Property
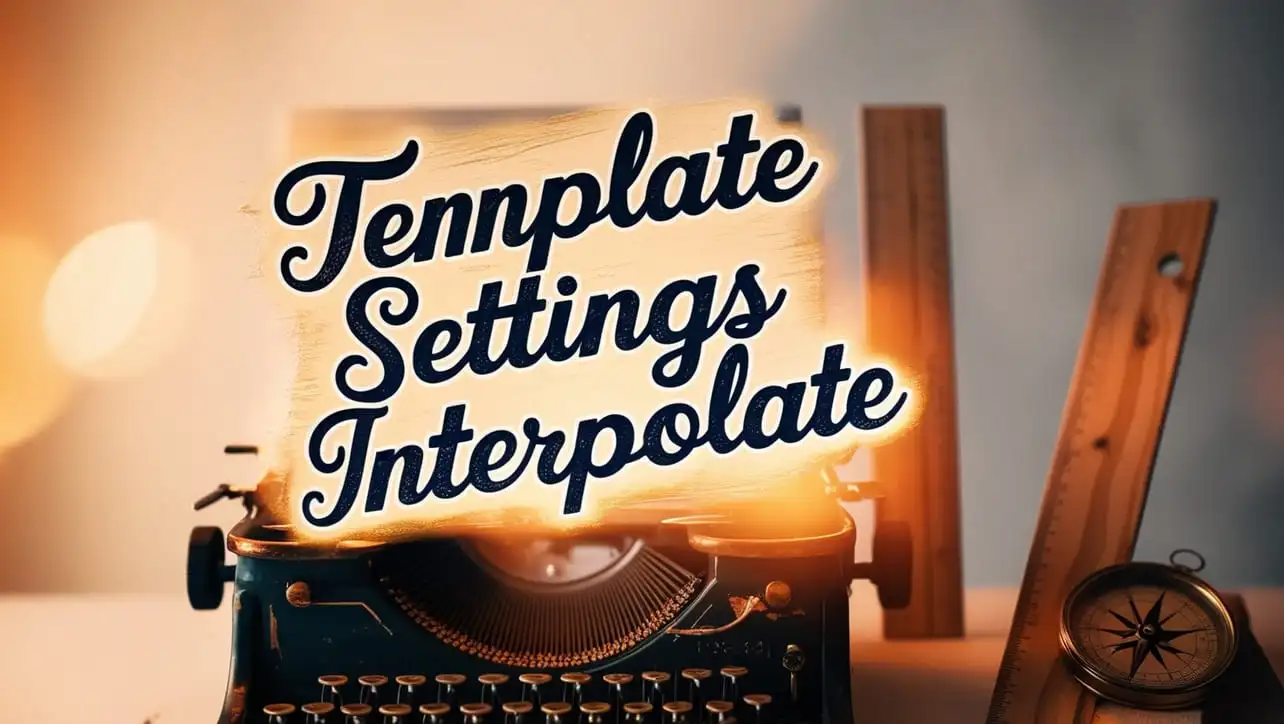
Lodash _.templateSettings.interpolate Property
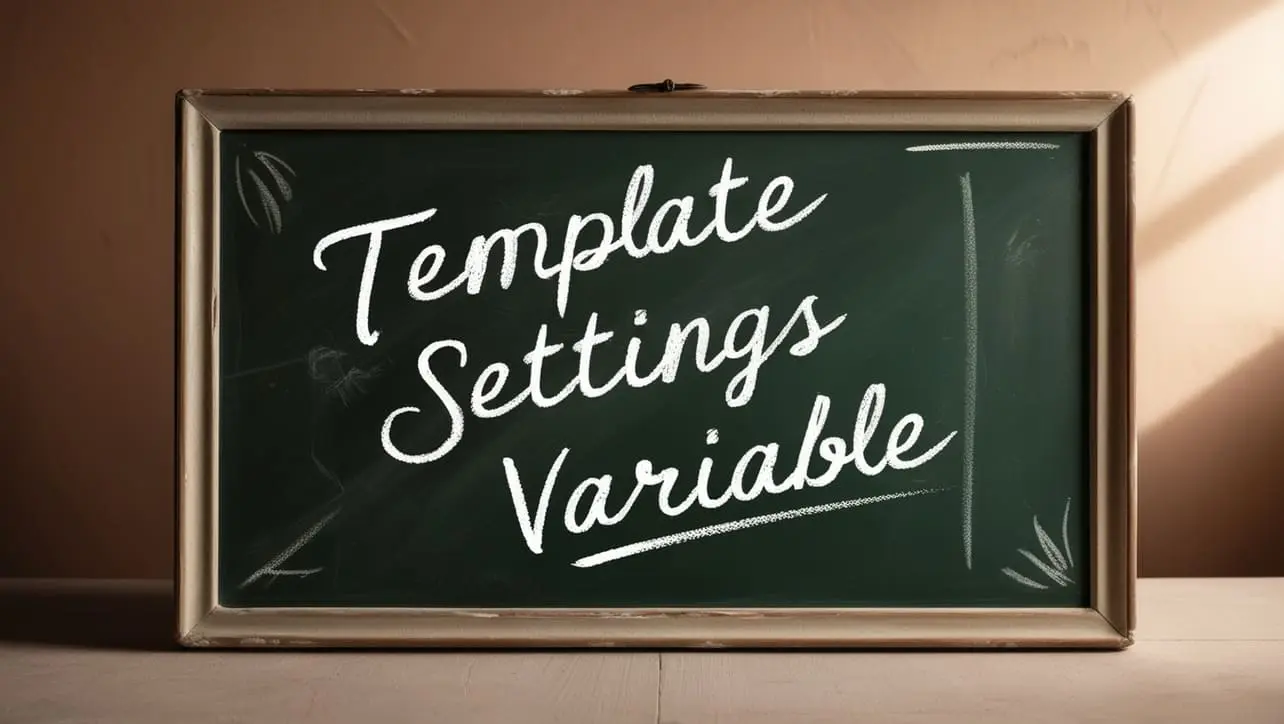
If you have any doubts regarding this article (Lodash _.without() Array Method), please comment here. I will help you immediately.