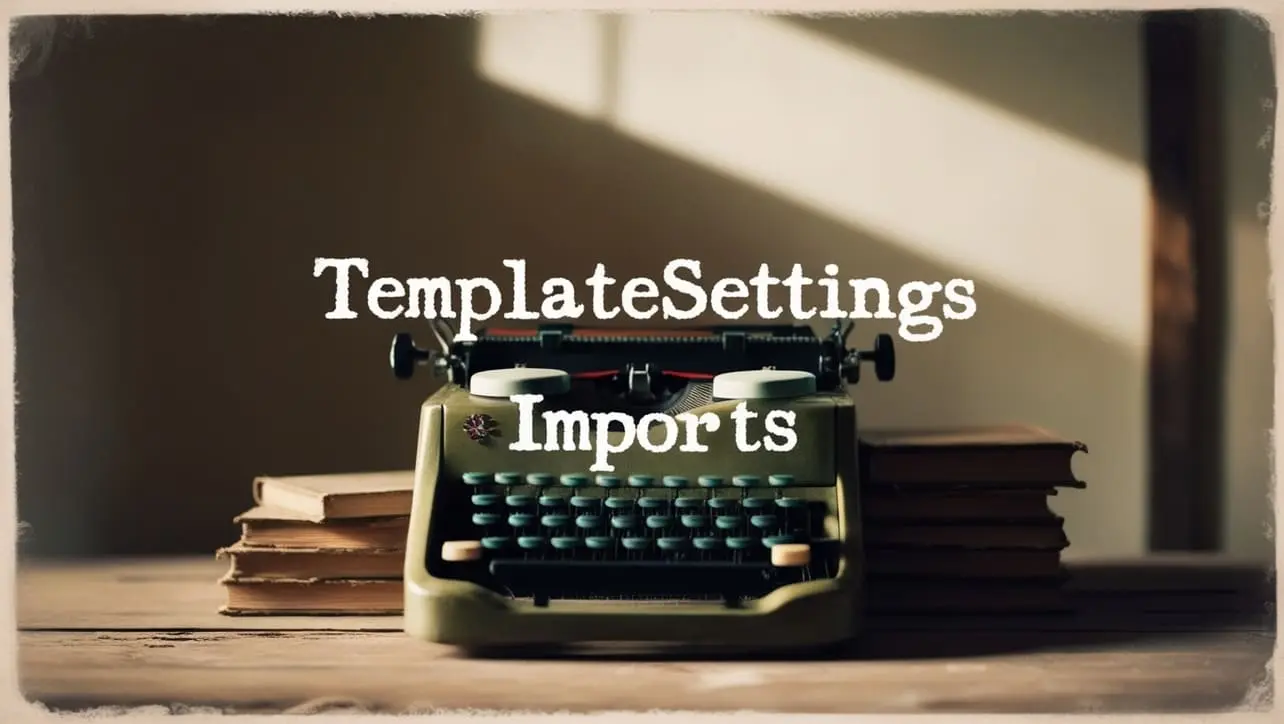
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.uniqBy() Array Method
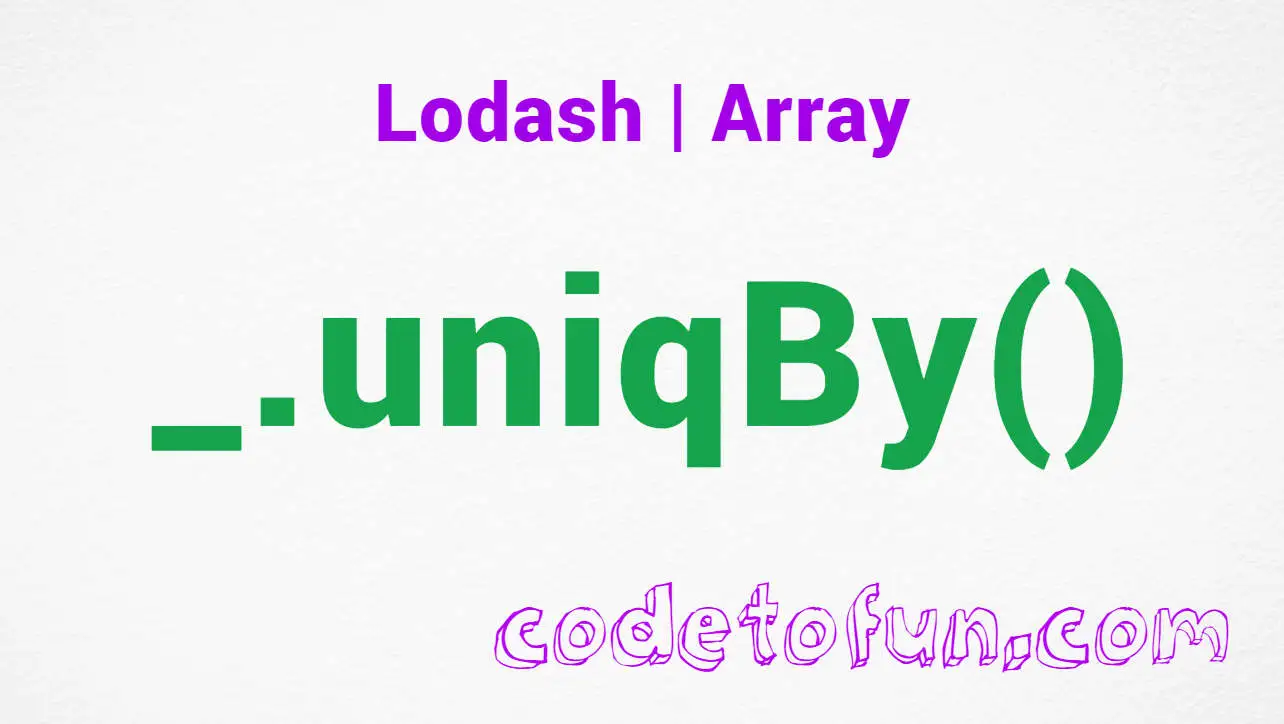
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, effective array manipulation is a fundamental aspect of creating robust applications. Lodash, a comprehensive utility library, provides a range of functions to simplify array operations. Among these functions is the _.uniqBy()
method, a powerful tool for creating an array with unique values based on a specific criterion.
This method enhances code clarity and flexibility, making it essential for developers dealing with diverse datasets.
🧠 Understanding _.uniqBy()
The _.uniqBy()
method in Lodash is designed to create a new array with unique values based on the result of applying an iteratee function to each element. This allows developers to define a custom criterion for uniqueness, offering precision in handling arrays with complex data structures.
💡 Syntax
_.uniqBy(array, [iteratee])
- array: The array to process.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a practical example to understand the functionality of _.uniqBy()
:
const _ = require('lodash');
const arrayWithDuplicates = [
{ id: 1, name: 'apple' },
{ id: 2, name: 'banana' },
{ id: 3, name: 'apple' },
{ id: 4, name: 'orange' },
];
const uniqueArray = _.uniqBy(arrayWithDuplicates, obj => obj.name);
console.log(uniqueArray);
// Output: [{ id: 1, name: 'apple' }, { id: 2, name: 'banana' }, { id: 4, name: 'orange' }]
In this example, the arrayWithDuplicates is processed by _.uniqBy()
, resulting in a new array containing only unique elements based on the 'name' property.
🏆 Best Practices
Define Custom Criteria:
Leverage the iteratee parameter to define a custom criterion for uniqueness. This allows you to tailor the behavior of
_.uniqBy()
according to your specific requirements.example.jsCopiedconst arrayOfObjects = [ { id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' }, { id: 3, category: 'fruit' }, ]; const uniqueCategories = _.uniqBy(arrayOfObjects, obj => obj.category); console.log(uniqueCategories); // Output: [{ id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' }]
Handling Complex Objects:
When dealing with complex objects, ensure that the iteratee function provides a unique identifier to accurately identify duplicate entries.
example.jsCopiedconst complexObjects = [ { id: 1, details: { type: 'A', value: 10 } }, { id: 2, details: { type: 'B', value: 20 } }, { id: 3, details: { type: 'A', value: 10 } }, ]; const uniqueObjects = _.uniqBy(complexObjects, obj => `${obj.details.type}-${obj.details.value}`); console.log(uniqueObjects); // Output: [ { id: 1, details: { type: 'A', value: 10 } }, { id: 2, details: { type: 'B', value: 20 } } ]
Performance Considerations:
Be mindful of performance when working with large datasets. While
_.uniqBy()
is efficient, handling massive arrays may benefit from optimization strategies.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; console.time('uniqBy'); const uniqueValues = _.uniqBy(largeDataset, 'property'); console.timeEnd('uniqBy'); console.log(uniqueValues);
📚 Use Cases
Removing Duplicate Entries:
_.uniqBy()
is particularly useful when dealing with datasets containing duplicate entries. By removing duplicates, you can ensure data integrity and streamline subsequent operations.example.jsCopiedconst arrayWithDuplicates = [1, 2, 2, 3, 3, 4, 5, 5, 5]; const uniqueValues = _.uniqBy(arrayWithDuplicates); console.log(uniqueValues); // Output: [1, 2, 3, 4, 5]
Data Normalization:
In scenarios where data normalization is necessary,
_.uniqBy()
can be employed to condense redundant information, facilitating cleaner and more concise data representation.example.jsCopiedconst dataWithDuplicates = [ { id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' }, { id: 3, category: 'fruit' }, { id: 4, category: 'vegetable' }, ]; const uniqueCategories = _.uniqBy(dataWithDuplicates, 'category'); console.log(uniqueCategories); // Output: [ { id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' } ]
Filtering Unique Values:
When you need to filter an array and retain only unique values based on a specific criterion,
_.uniqBy()
becomes a valuable ally.example.jsCopiedconst products = [ { id: 1, name: 'apple', category: 'fruit' }, { id: 2, name: 'banana', category: 'fruit' }, { id: 3, name: 'carrot', category: 'vegetable' }, { id: 4, name: 'apple', category: 'fruit' }, ]; const uniqueFruits = _.uniqBy(products, 'name'); console.log(uniqueFruits); // Output: [ { id: 1, name: 'apple', category: 'fruit' }, { id: 2, name: 'banana', category: 'fruit' }, { id: 3, name: 'carrot', category: 'vegetable' } ]
🎉 Conclusion
The _.uniqBy()
method in Lodash provides an efficient solution for creating arrays with unique values based on a custom criterion. Whether you're working with simple arrays or complex data structures, this method offers versatility and clarity in array manipulation.
Harness the power of _.uniqBy()
to streamline your JavaScript development and ensure data uniqueness in your applications!
👨💻 Join our Community:
Author
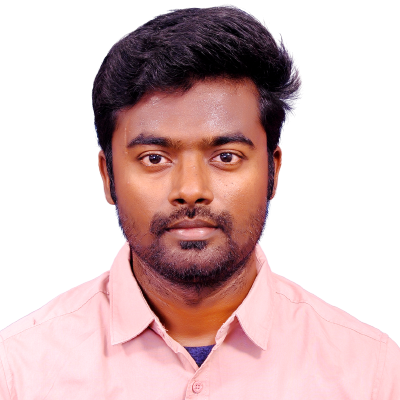
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
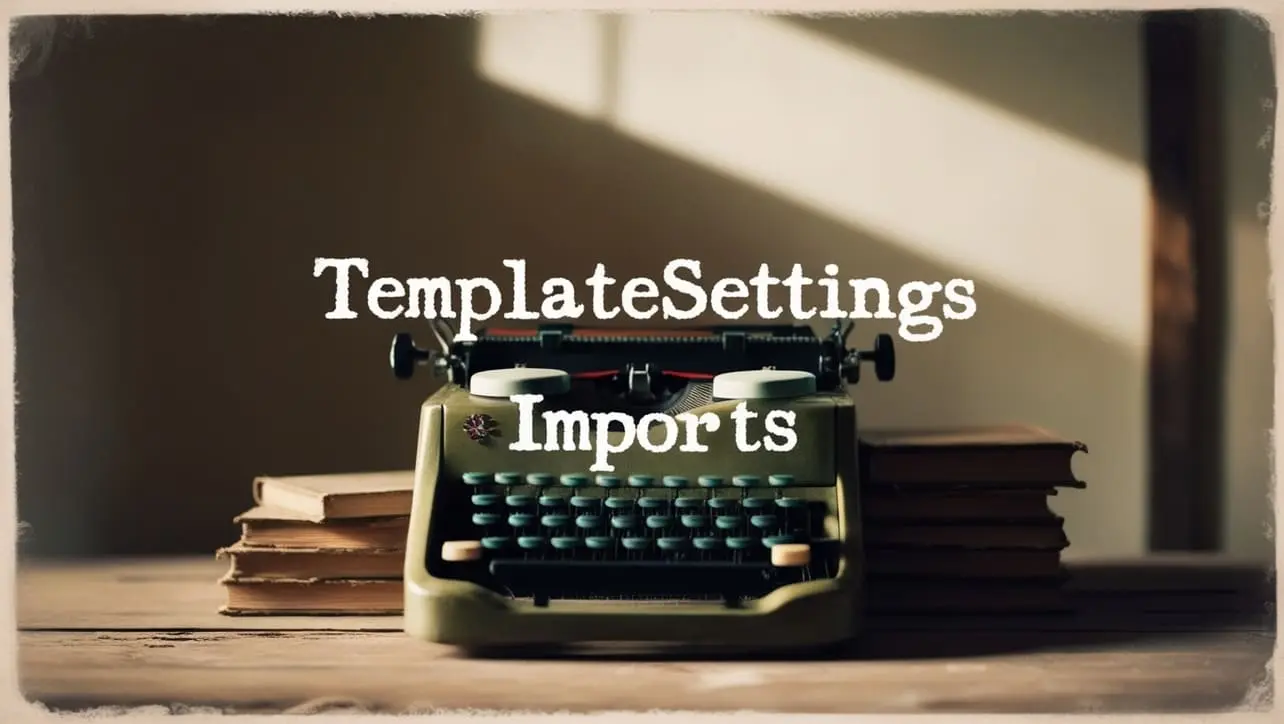
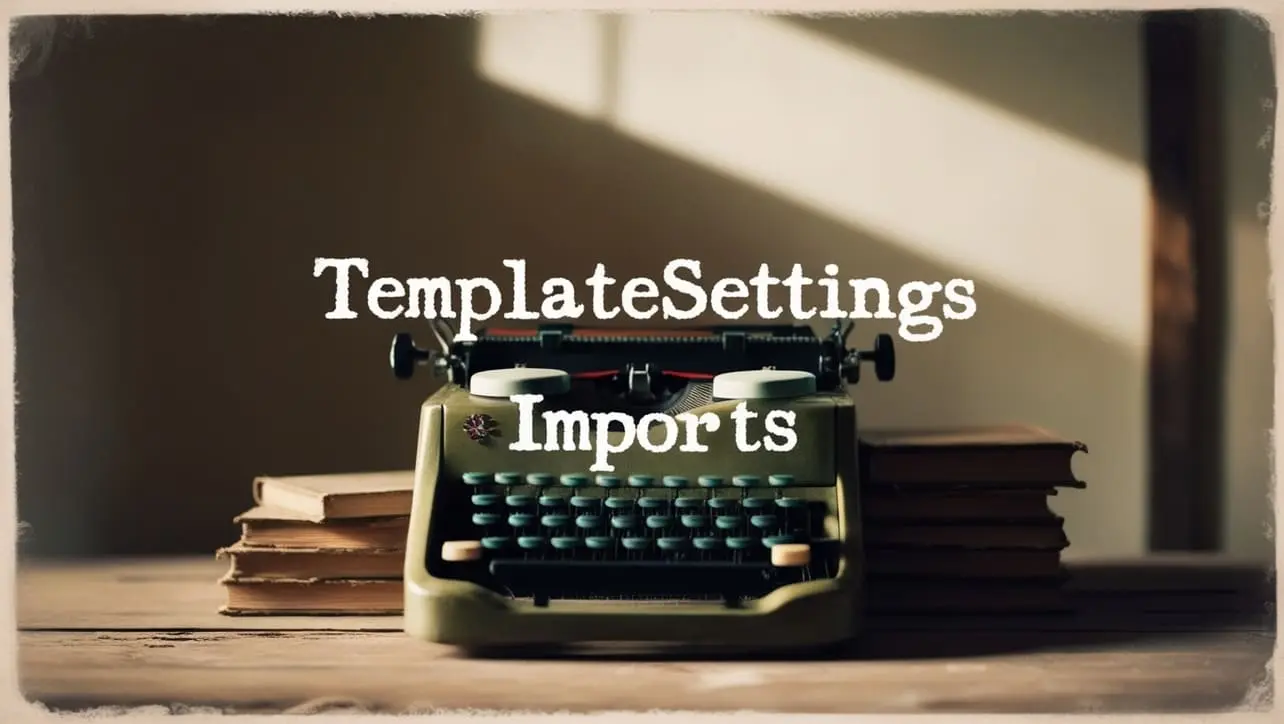
Lodash _.templateSettings.imports Property
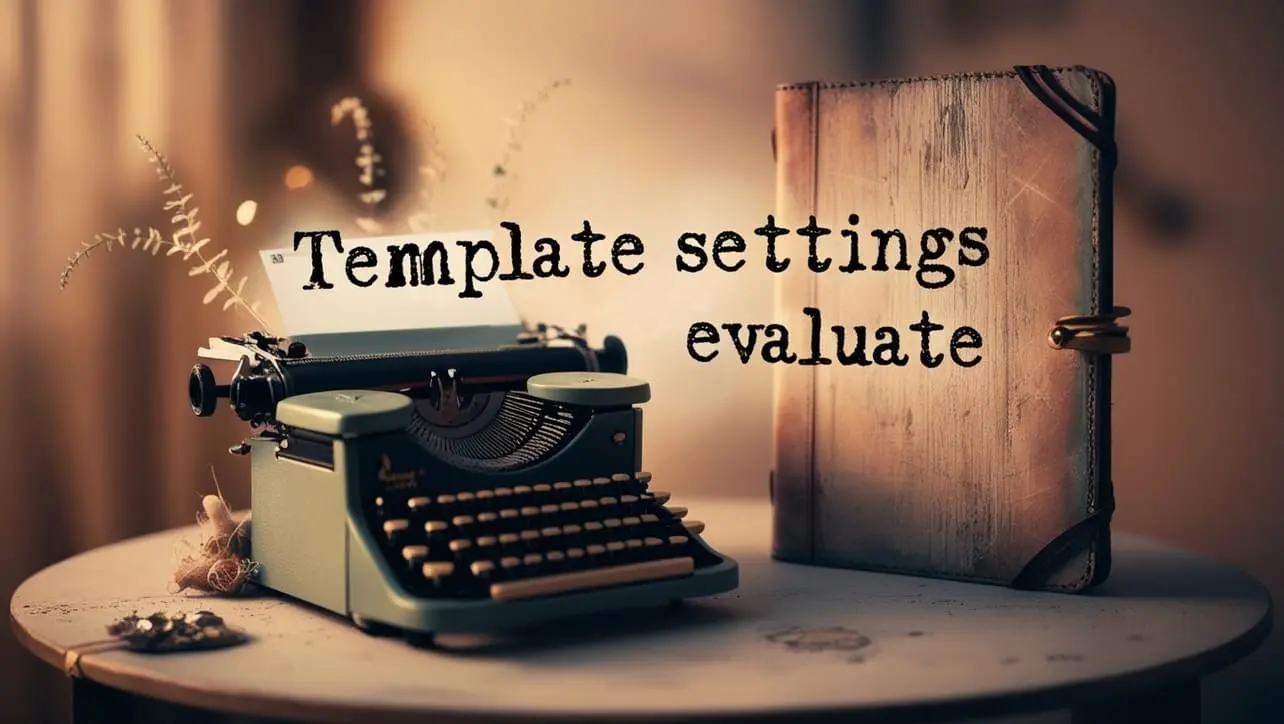
Lodash _.templateSettings.evaluate Property
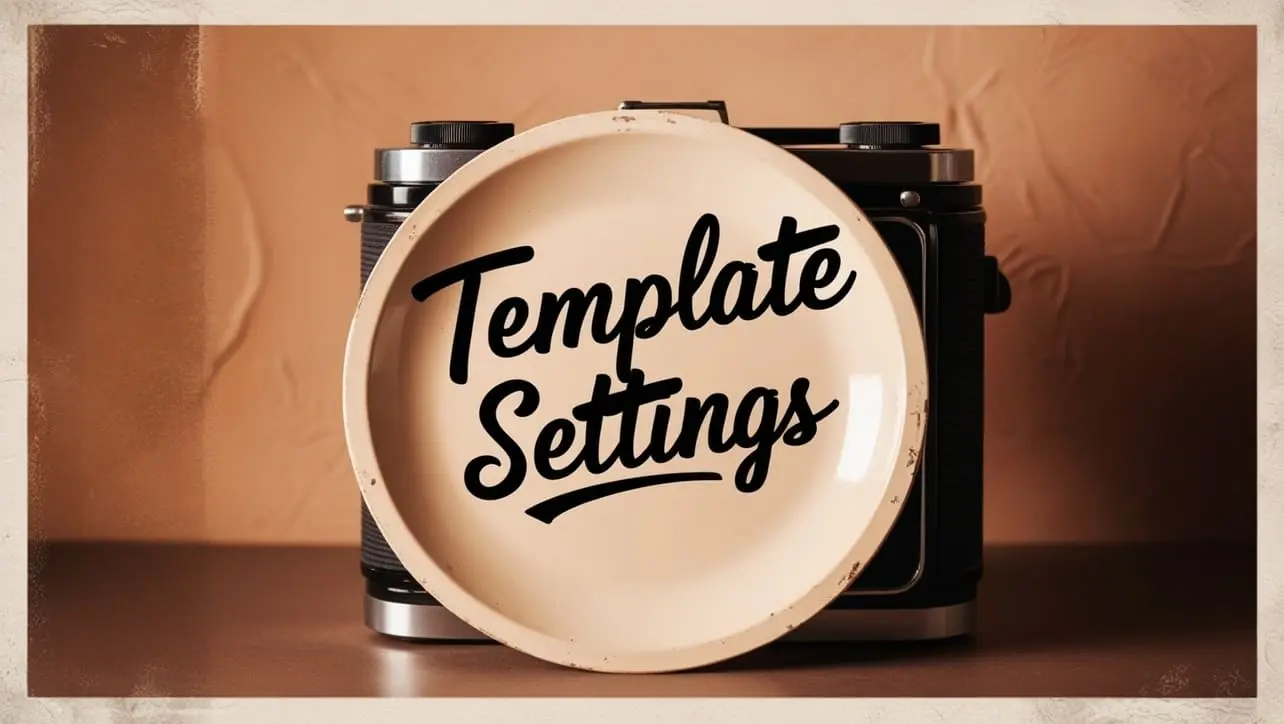
Lodash _.templateSettings Property
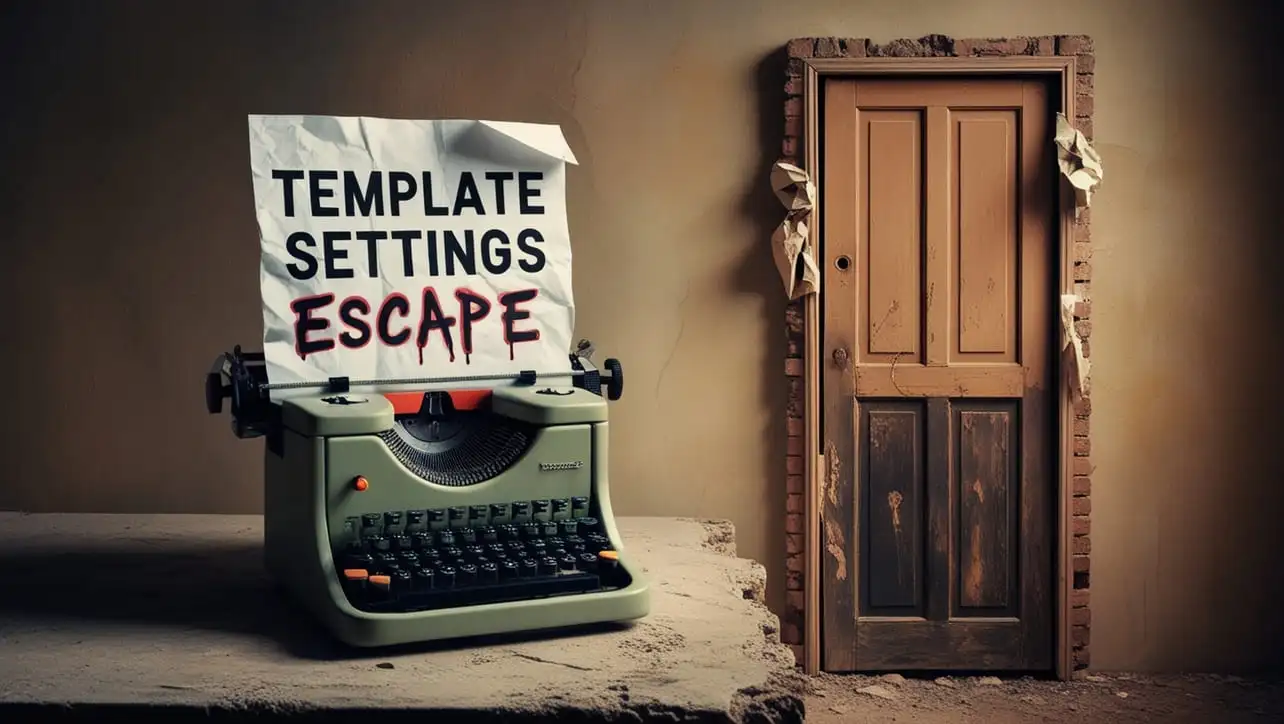
Lodash _.templateSettings.escape Property
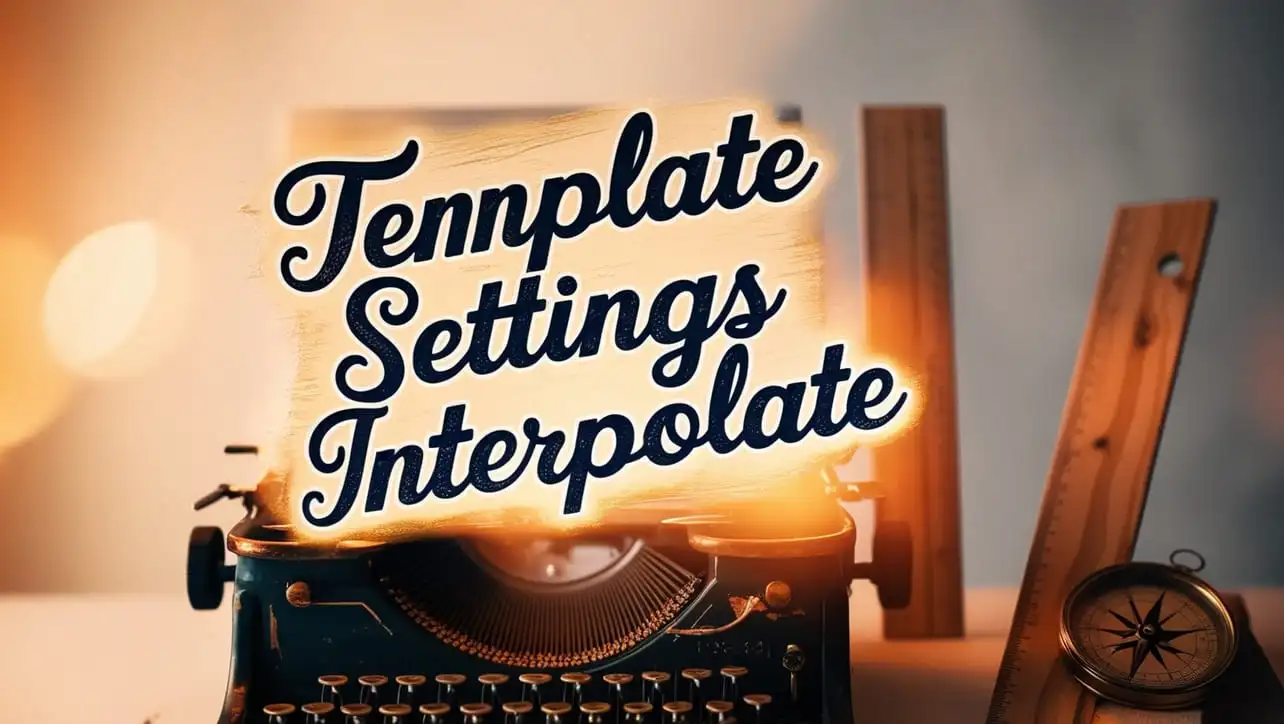
Lodash _.templateSettings.interpolate Property
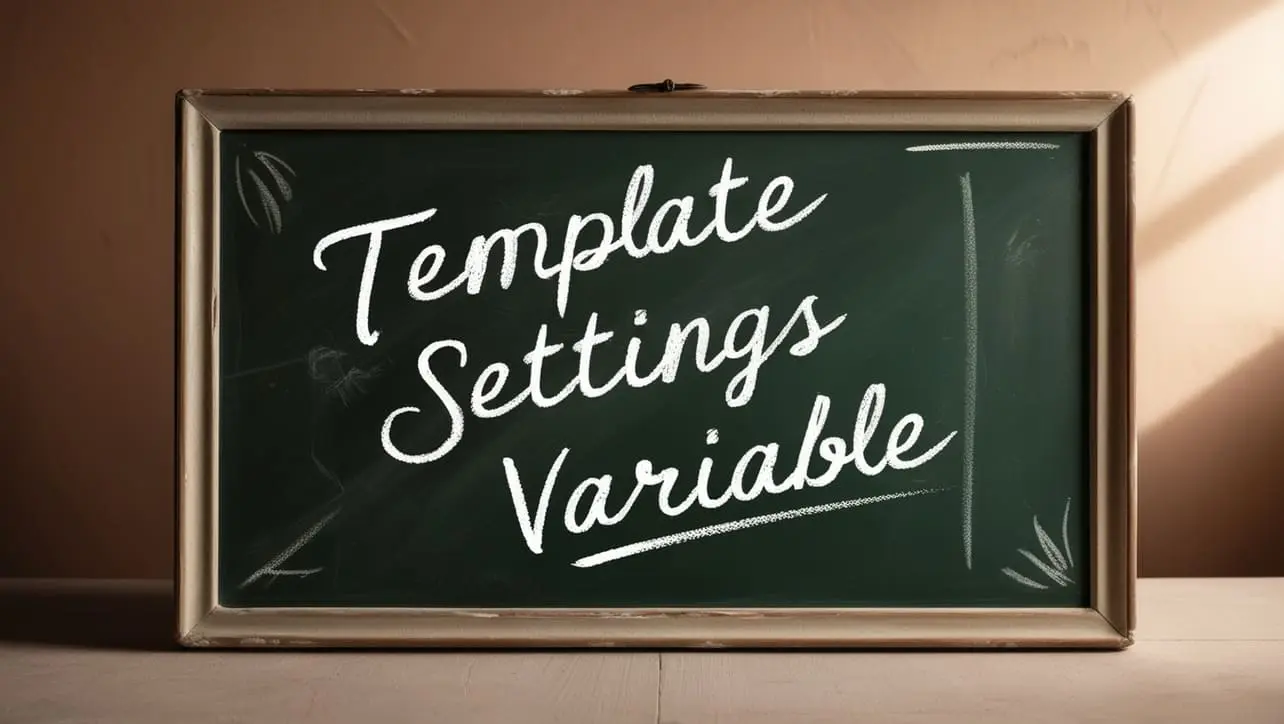
If you have any doubts regarding this article (Lodash _.uniqBy() Array Method), please comment here. I will help you immediately.