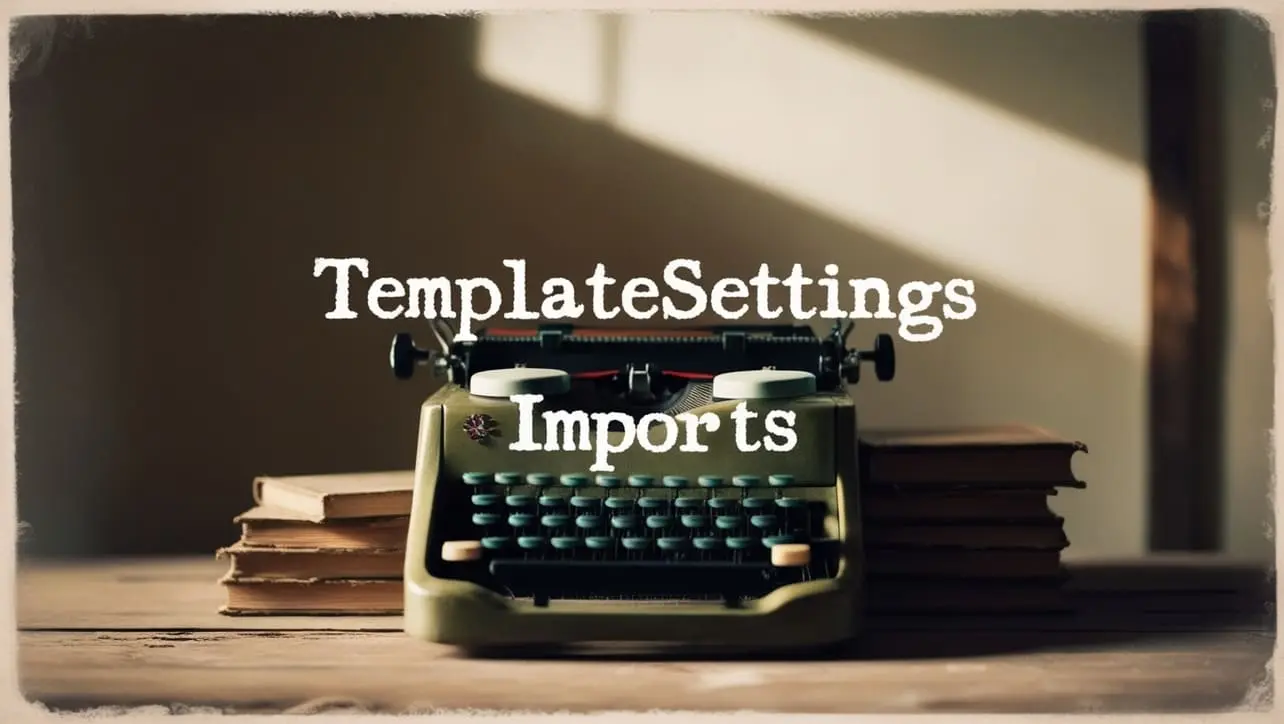
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.takeWhile() Array Method
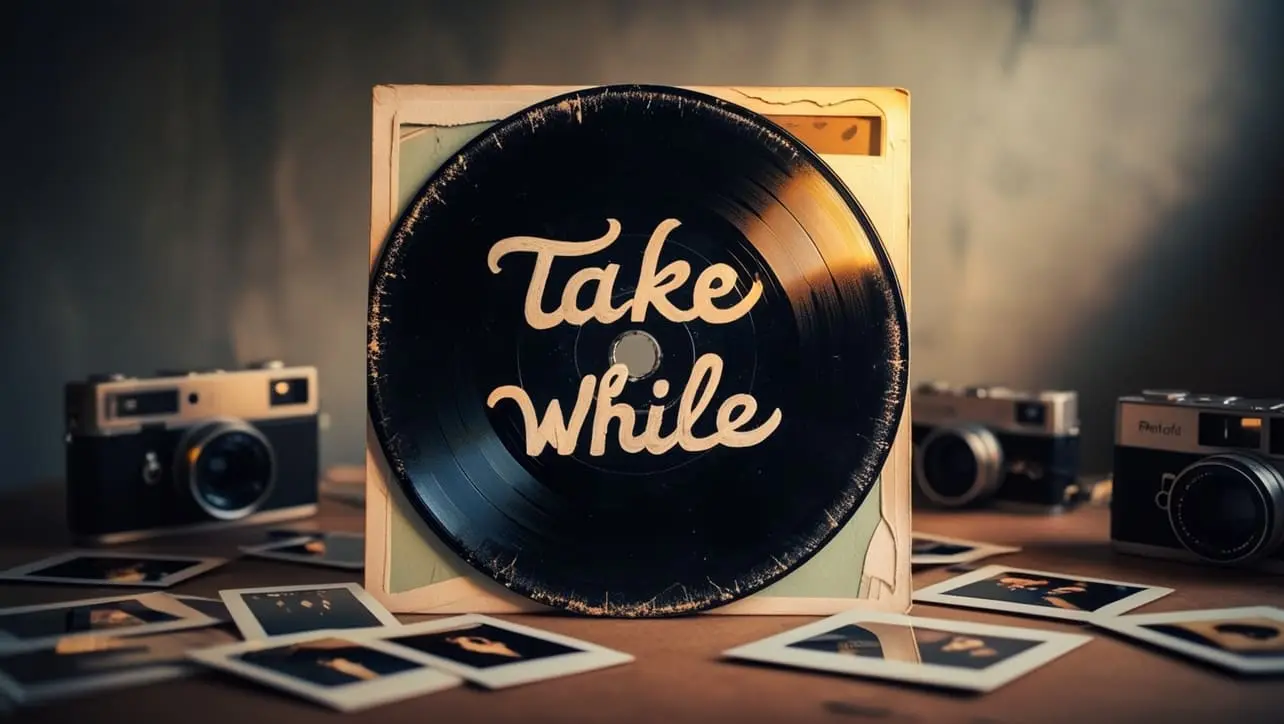
Photo Credit to CodeToFun
🙋 Introduction
Effective array manipulation is a cornerstone of JavaScript development, and Lodash provides a comprehensive set of utility functions to streamline this process. Among these functions, the _.takeWhile()
method stands out as a powerful tool for extracting elements from the beginning of an array based on a given condition.
This method enhances the flexibility and readability of code, making it invaluable for developers dealing with complex datasets.
🧠 Understanding _.takeWhile()
The _.takeWhile()
method in Lodash is designed to create a new array with elements taken from the beginning of the provided array. Elements are taken as long as the given predicate function returns truthy. This empowers developers to extract a subset of data that meets specific criteria, facilitating efficient data processing.
💡 Syntax
_.takeWhile(array, [predicate])
- array: The array to process.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's explore a practical example to understand the functionality of _.takeWhile()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const takenNumbers = _.takeWhile(numbers, num => num < 5);
console.log(takenNumbers);
// Output: [1, 2, 3, 4]
In this example, the numbers array is processed by _.takeWhile()
, creating a new array containing elements taken from the beginning as long as the condition num < 5 holds true.
🏆 Best Practices
Define Clear Predicates:
Ensure that the predicate function used with
_.takeWhile()
clearly defines the condition for taking elements. This enhances code readability and reduces the risk of unintended behavior.example.jsCopiedconst products = [ { name: 'Laptop', price: 1200 }, { name: 'Smartphone', price: 800 }, { name: 'Tablet', price: 500 }, { name: 'Smartwatch', price: 200 }, ]; const affordableProducts = _.takeWhile(products, product => product.price < 1000); console.log(affordableProducts); // Output: [{ name: 'Smartphone', price: 800 }, { name: 'Tablet', price: 500 }]
Combine with Sorted Data:
For optimal performance, consider using
_.takeWhile()
with sorted data. This allows for early termination of the iteration once the predicate becomes false, improving efficiency.example.jsCopiedconst sortedNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const takenSortedNumbers = _.takeWhile(sortedNumbers, num => num < 5); console.log(takenSortedNumbers); // Output: [1, 2, 3, 4]
Use with Objects:
_.takeWhile()
is not limited to arrays of primitives; it can be used with arrays of objects as well. Define predicate functions that operate on object properties for flexible data extraction.example.jsCopiedconst students = [ { name: 'Alice', grade: 85 }, { name: 'Bob', grade: 92 }, { name: 'Charlie', grade: 78 }, { name: 'David', grade: 95 }, ]; const topPerformers = _.takeWhile(students, student => student.grade >= 90); console.log(topPerformers); // Output: [{ name: 'Alice', grade: 85 }, { name: 'Bob', grade: 92 }]
📚 Use Cases
Filtering Based on Conditions:
_.takeWhile()
is ideal for scenarios where you need to filter the beginning of an array based on specific conditions, such as extracting elements until a certain value is reached.example.jsCopiedconst temperatures = [30, 25, 22, 18, 15, 12, 10, 8, 5]; const warmDays = _.takeWhile(temperatures, temp => temp >= 20); console.log(warmDays); // Output: [30, 25, 22]
Early Termination in Sorted Data:
When working with sorted data,
_.takeWhile()
can provide early termination, optimizing performance in situations where you want to extract elements until a particular condition is met.example.jsCopiedconst sortedTransactionAmounts = [10, 20, 30, 40, 50, 60, 70]; const takenTransactions = _.takeWhile(sortedTransactionAmounts, amount => amount <= 40); console.log(takenTransactions); // Output: [10, 20, 30, 40]
Dynamic Data Extraction:
Combine
_.takeWhile()
with dynamically changing conditions to create flexible data extraction mechanisms based on runtime criteria.example.jsCopiedconst data = [100, 150, 120, 90, 80, 60, 55]; let condition = amount => amount > 80; const extractedData = _.takeWhile(data, condition); console.log(extractedData); // Output: [100, 150, 120, 90]
🎉 Conclusion
The _.takeWhile()
method in Lodash offers a versatile solution for extracting elements from the beginning of an array based on dynamic conditions. Whether you're working with numeric data, objects, or sorted arrays, _.takeWhile()
provides a powerful tool for efficient and flexible data extraction in JavaScript.
Enhance your array manipulation capabilities with _.takeWhile()
and discover a new level of control over your data processing!
👨💻 Join our Community:
Author
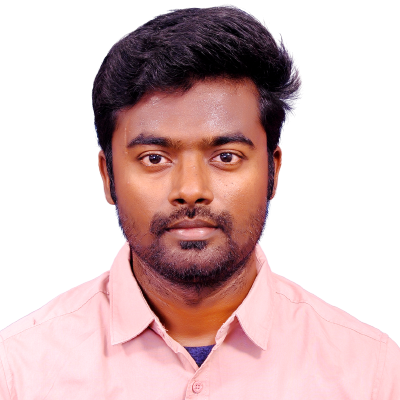
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
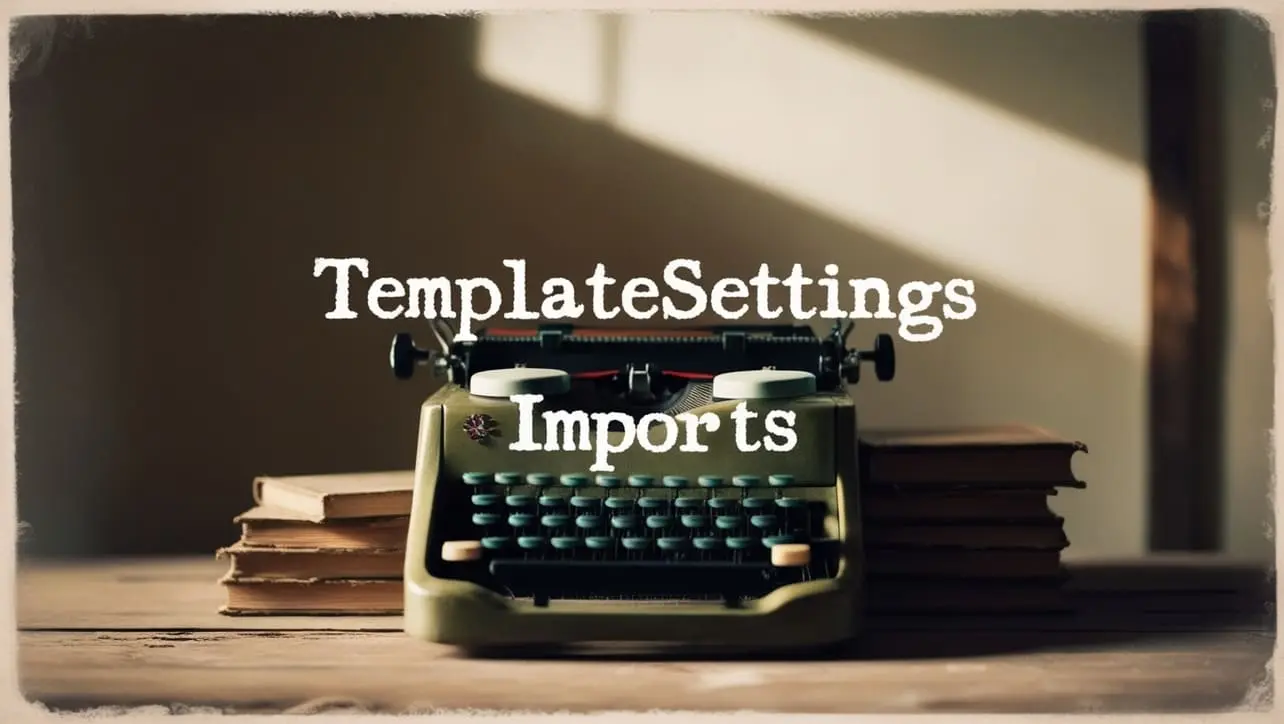
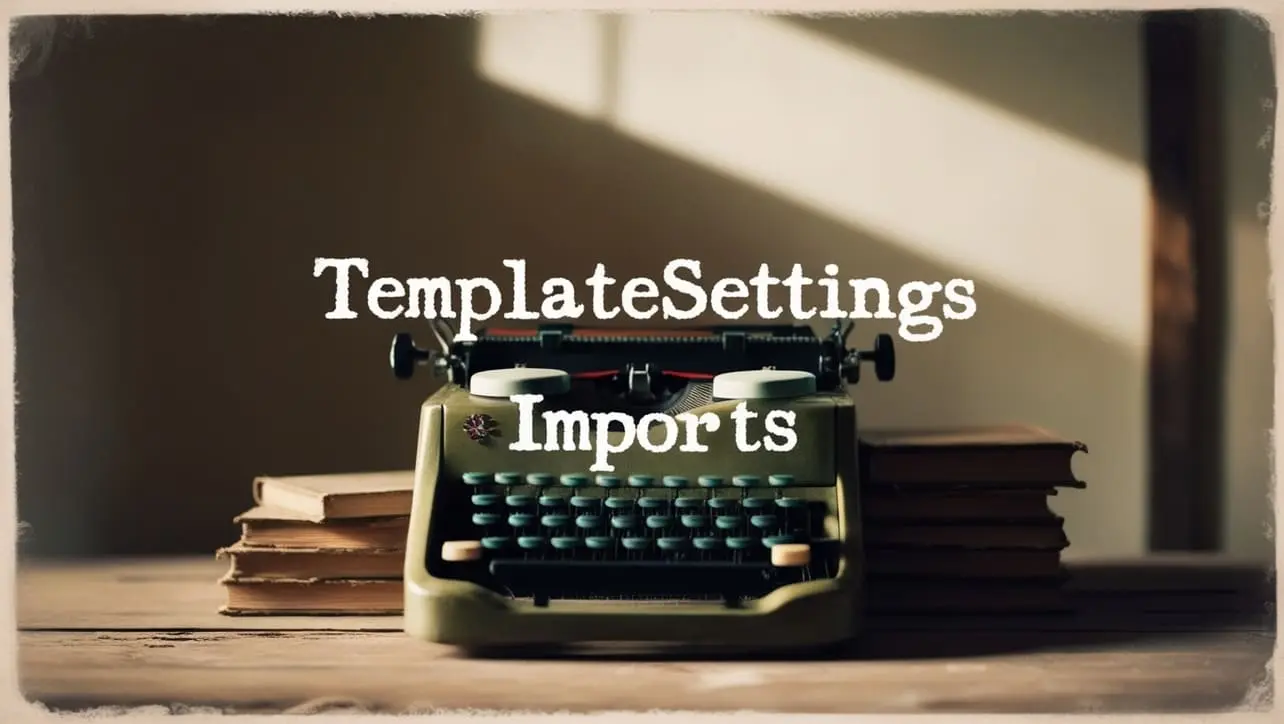
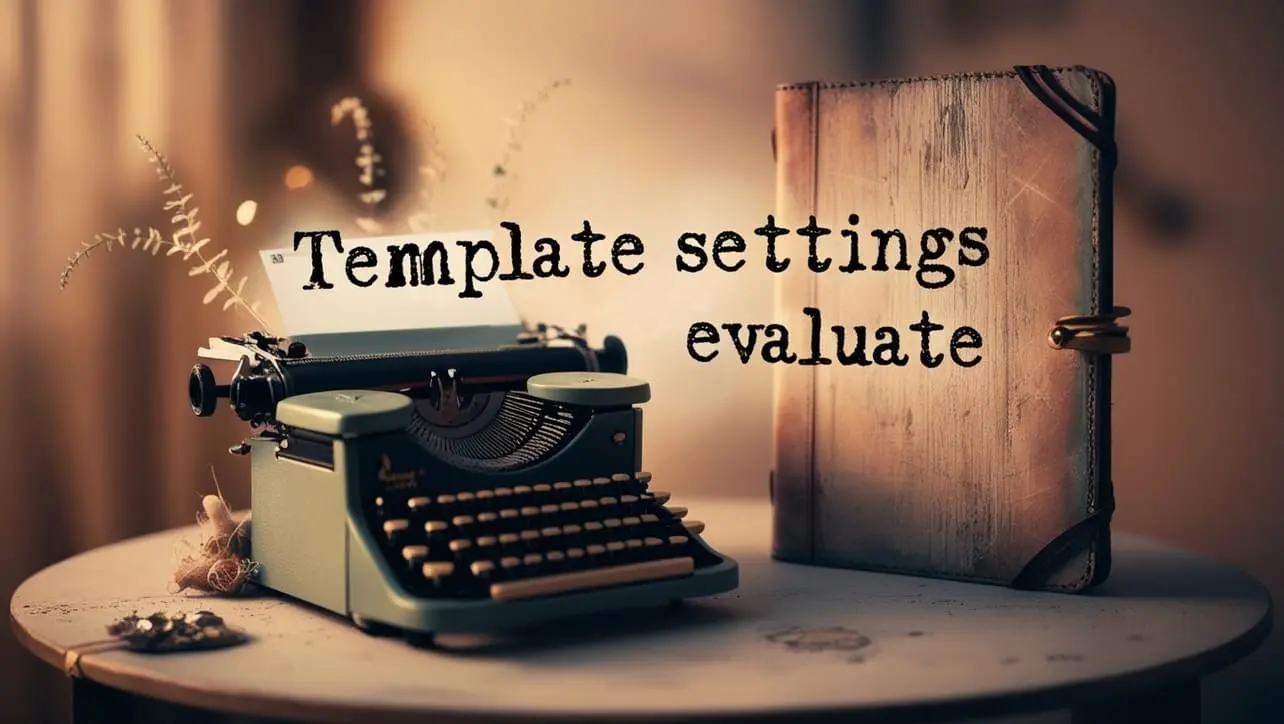
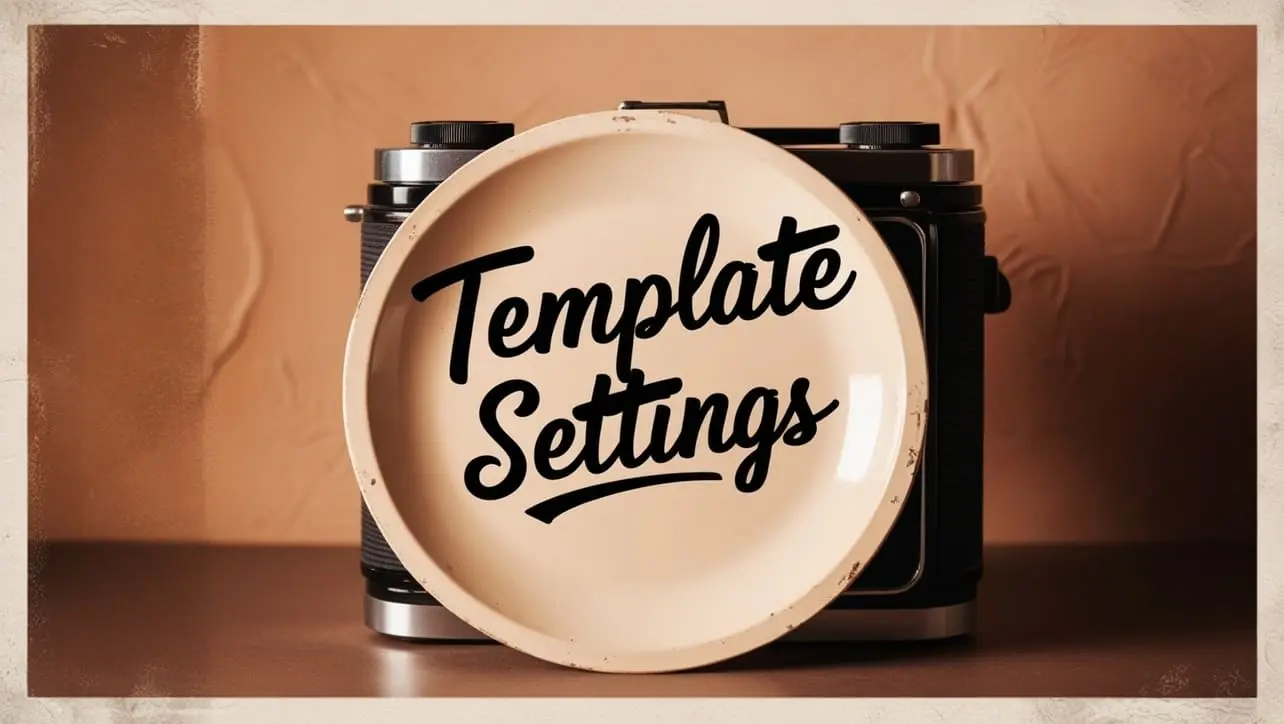
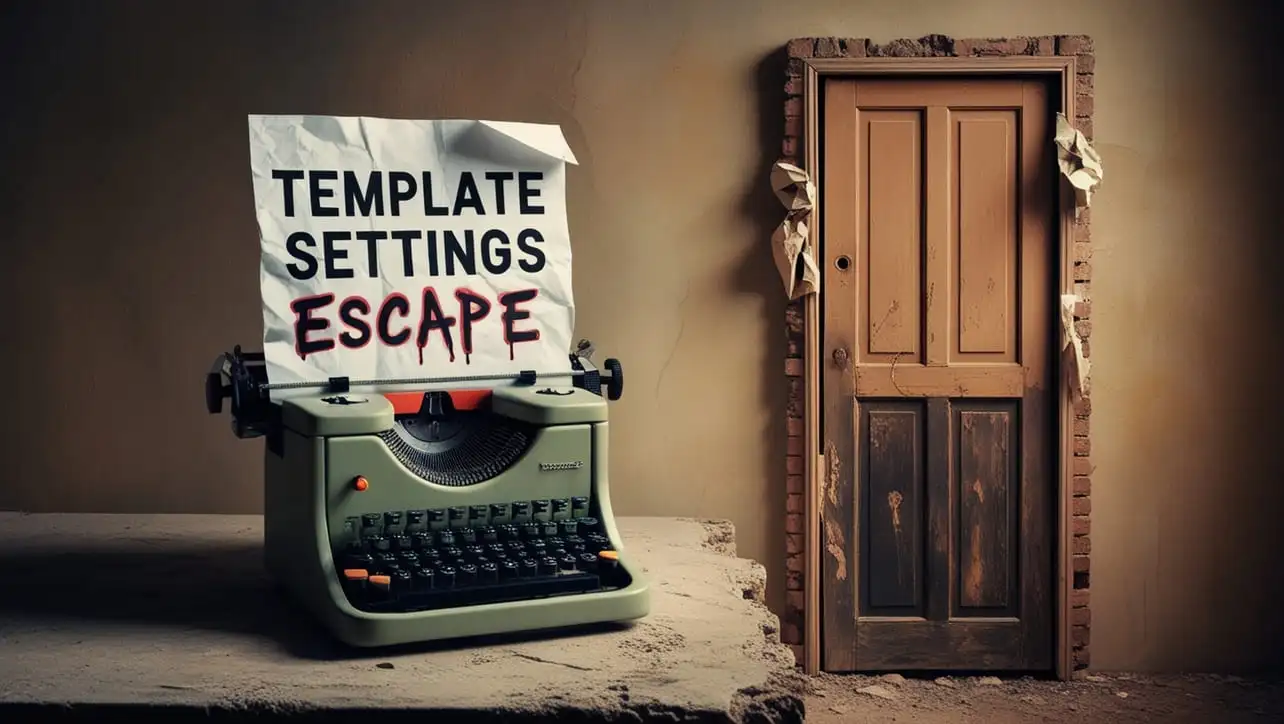
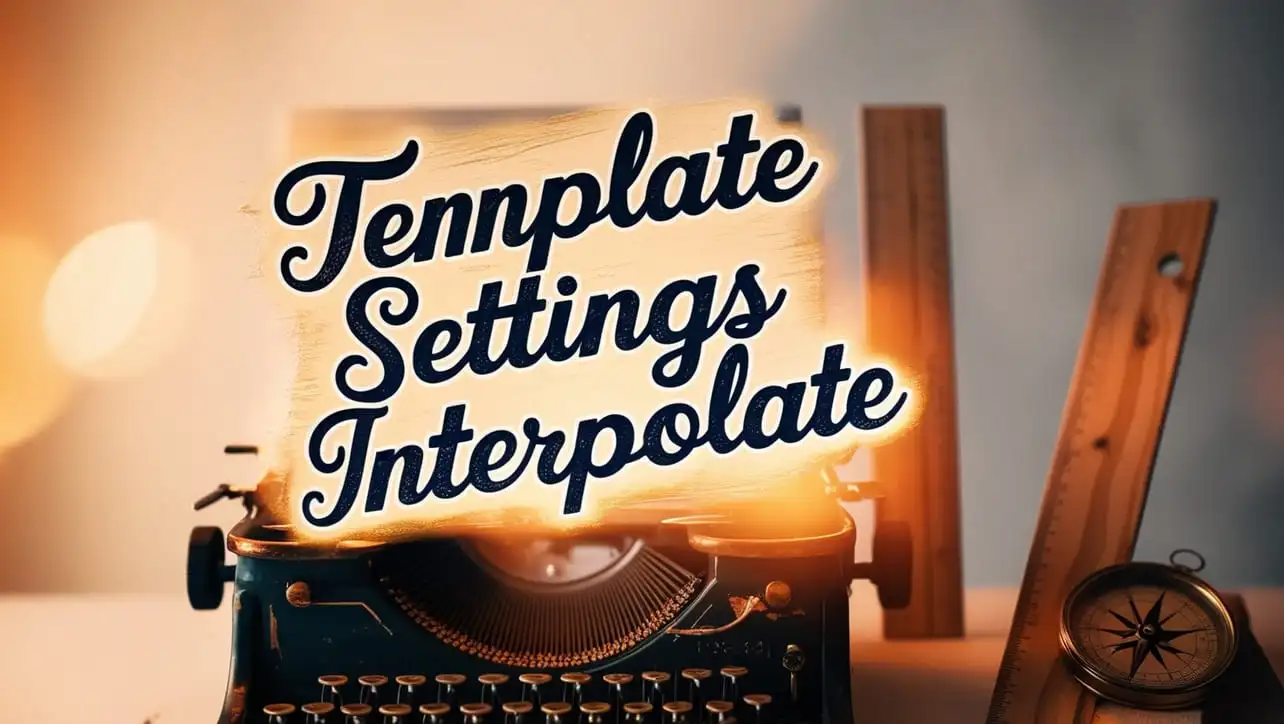
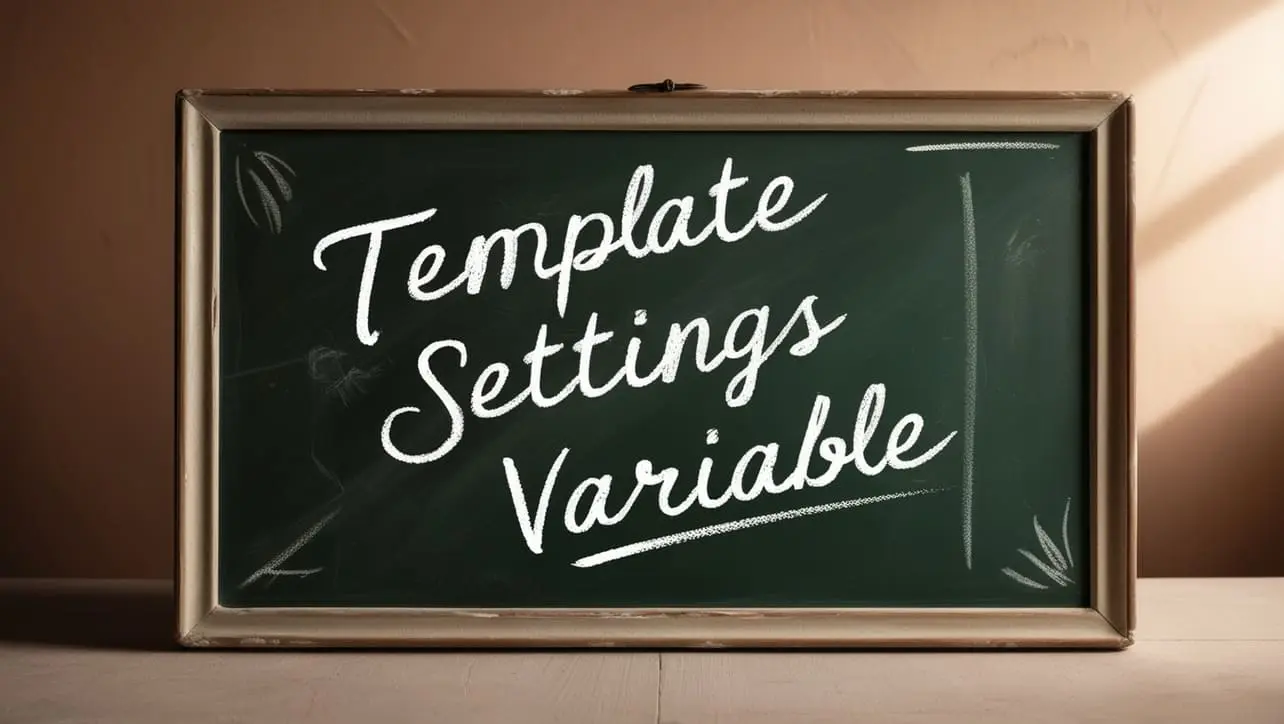
If you have any doubts regarding this article (Lodash _.takeWhile() Array Method), please comment here. I will help you immediately.