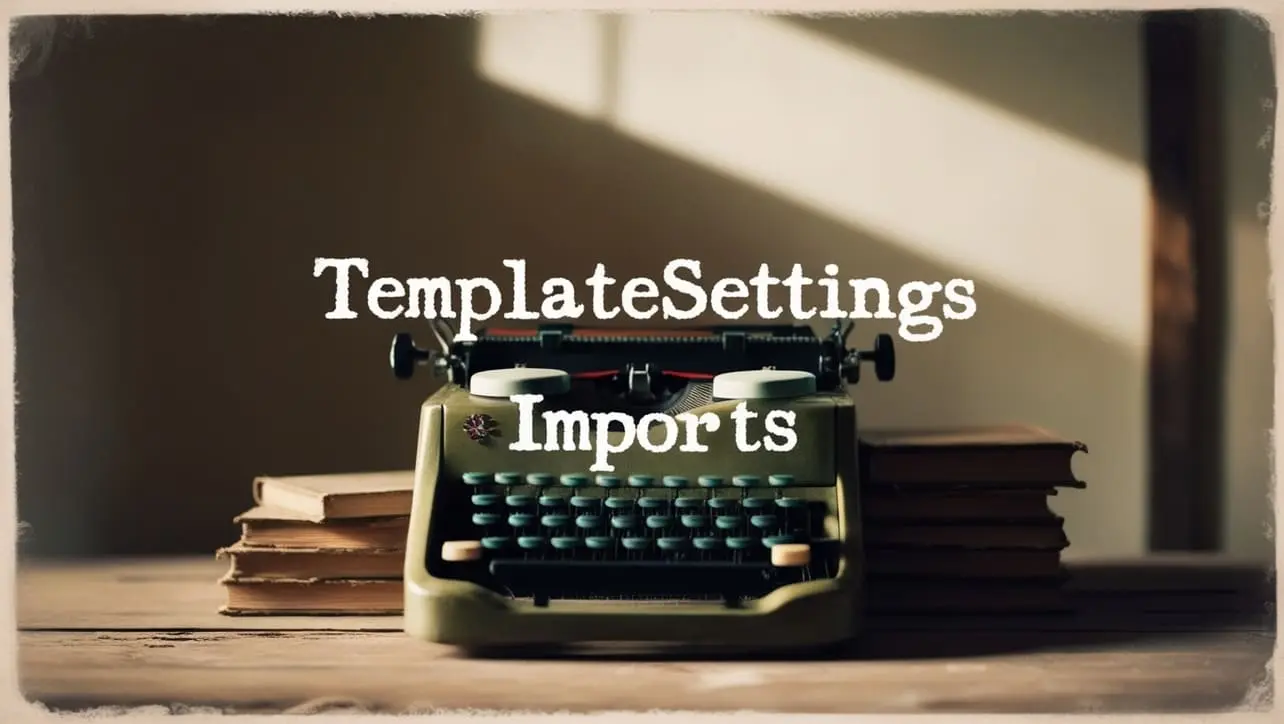
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.tail() Array Method
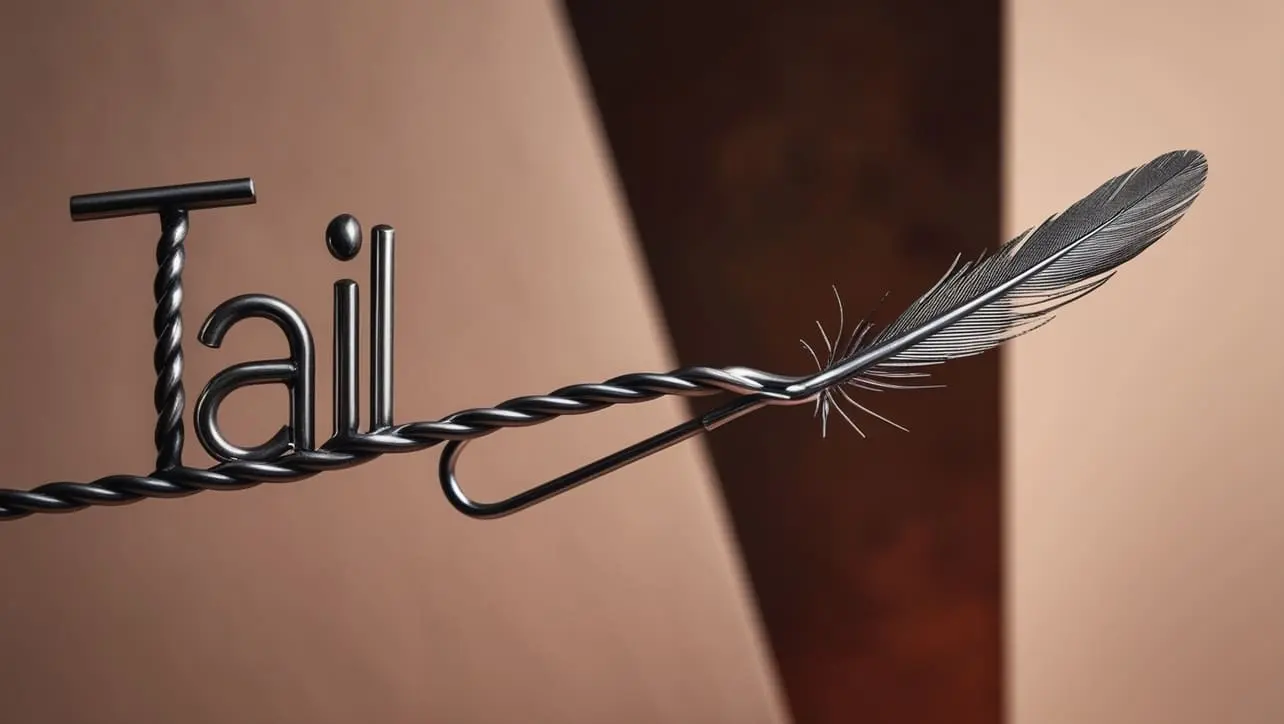
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, working with arrays is a fundamental aspect of coding. Lodash, a feature-rich utility library, provides developers with a multitude of tools for array manipulation, and one such tool is the _.tail()
method.
This method simplifies the process of extracting all elements of an array except for the first one, offering a concise and efficient solution for various programming scenarios.
🧠 Understanding _.tail()
The _.tail()
method in Lodash allows you to retrieve all elements from an array except the first one. This is particularly useful when you need a subset of an array starting from a specific position, enhancing code readability and reducing the need for manual slicing and splicing.
💡 Syntax
_.tail(array)
- array: The array to process.
📝 Example
Let's dive into a practical example to illustrate the simplicity and utility of _.tail()
:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const tailElements = _.tail(originalArray);
console.log(tailElements);
// Output: [2, 3, 4, 5]
In this example, the originalArray is processed by _.tail()
, resulting in a new array containing all elements except the first one.
🏆 Best Practices
Array Validation:
Before applying
_.tail()
, ensure that the input array is valid and contains elements. This helps prevent unexpected errors when attempting to extract elements.example.jsCopiedconst emptyArray = []; const nonEmptyArray = [1, 2, 3]; const tailOfEmptyArray = _.tail(emptyArray); // Returns: [] const tailOfNonEmptyArray = _.tail(nonEmptyArray); // Returns: [2, 3] console.log(tailOfEmptyArray); console.log(tailOfNonEmptyArray);
Immutability:
Keep in mind that
_.tail()
does not modify the original array; it returns a new array with the desired elements. Embrace this immutability to maintain the integrity of your data.example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const tailElements = _.tail(originalArray); console.log(originalArray); // Output: [1, 2, 3, 4, 5] console.log(tailElements); // Output: [2, 3, 4, 5]
Positional Flexibility:
Experiment with using
_.tail()
in different positions within your code. Its flexibility allows you to tailor its usage based on the specific needs of your algorithms or data transformations.example.jsCopiedconst dataProcessingFunction = (array) => { // Perform some operations on the tail of the array const processedData = processData(_.tail(array)); // ...rest of the function return processedData; };
📚 Use Cases
Skip First Element:
The primary use case for
_.tail()
is skipping the first element of an array, which is beneficial when the first element holds distinct information or is not relevant to the current operation.example.jsCopiedconst temperatureReadings = [25, 26, 27, 28, 29]; const relevantReadings = _.tail(temperatureReadings); console.log(relevantReadings); // Output: [26, 27, 28, 29]
Subsetting Arrays:
When you need a subset of an array excluding the initial elements,
_.tail()
provides a concise solution for extracting the desired portion.example.jsCopiedconst sensorReadings = [100, 102, 105, 104, 101]; const subset = _.tail(sensorReadings); console.log(subset); // Output: [102, 105, 104, 101]
Chaining Operations:
Combine
_.tail()
with other Lodash methods to create expressive and efficient chains of array operations.example.jsCopiedconst data = [1, 2, 3, 4, 5]; const processedData = _.chain(data) .tail() .map(value => value * 2) .filter(value => value > 5) .value(); console.log(processedData); // Output: [6, 8, 10]
🎉 Conclusion
The _.tail()
method in Lodash provides a clean and efficient solution for extracting all elements of an array except the first one. Whether you're skipping irrelevant data, creating subsets, or building complex data processing pipelines, _.tail()
is a valuable addition to your array manipulation toolkit.
Explore the versatility of _.tail()
and streamline your array operations with this simple yet powerful Lodash method!
👨💻 Join our Community:
Author
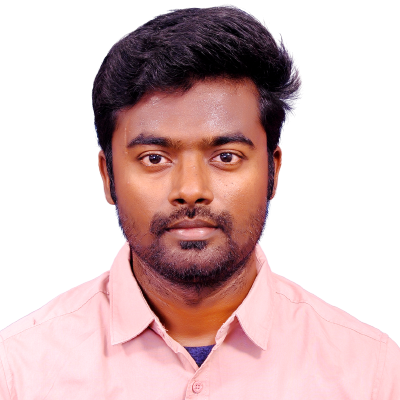
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
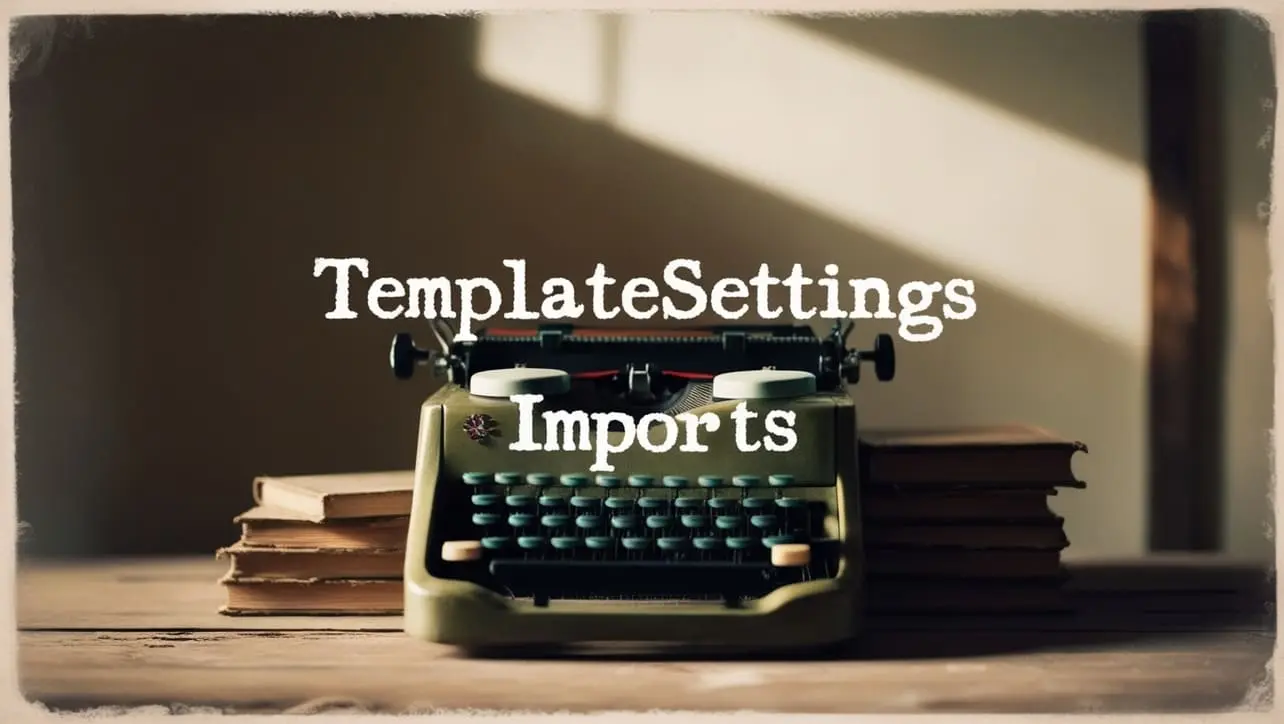
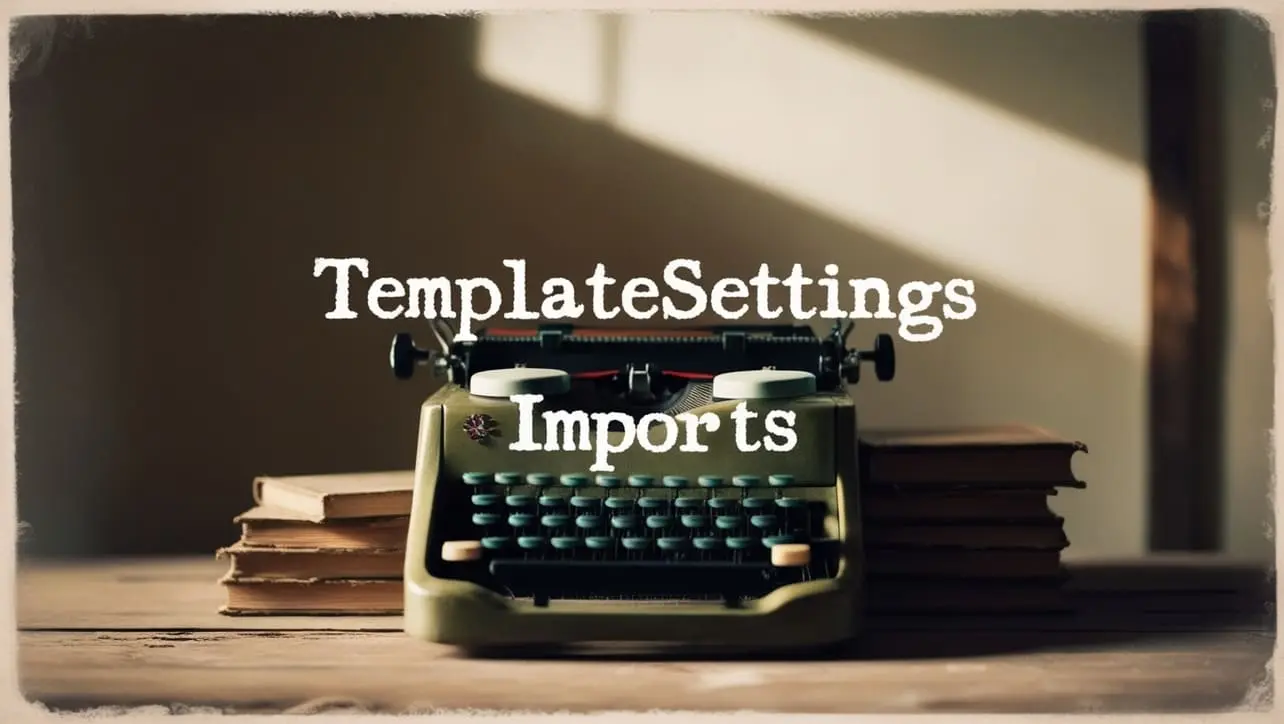
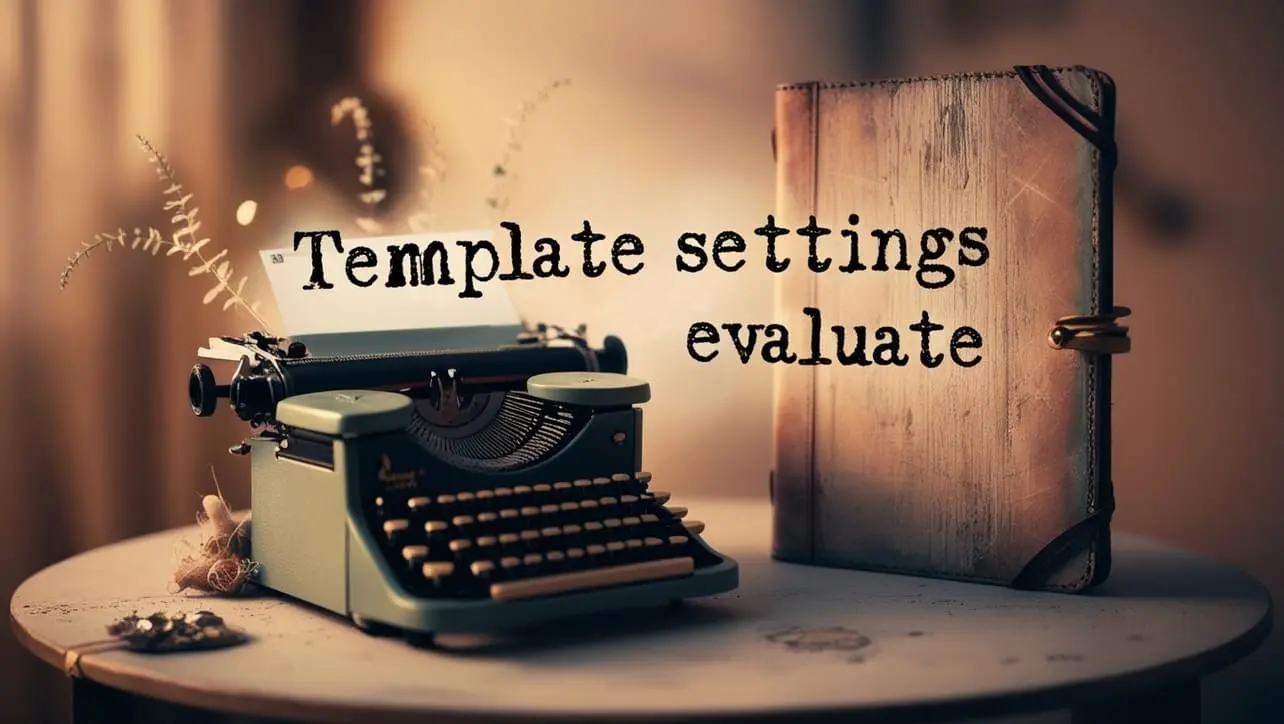
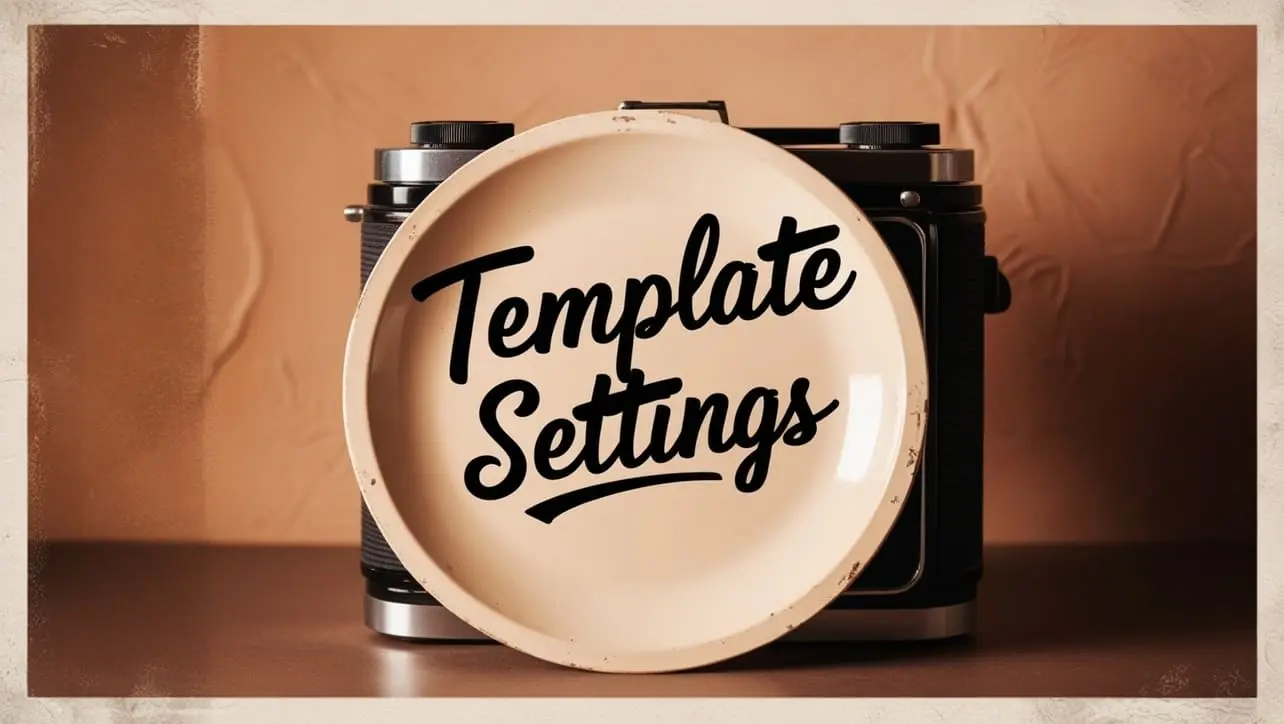
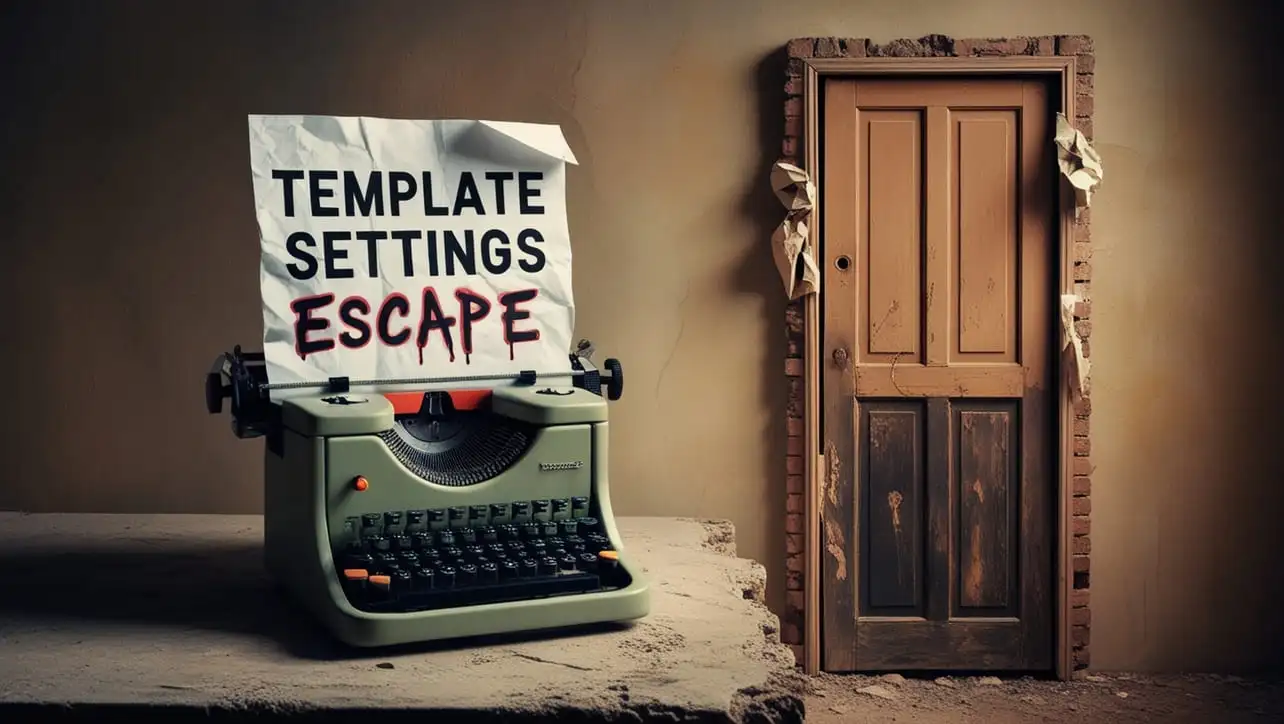
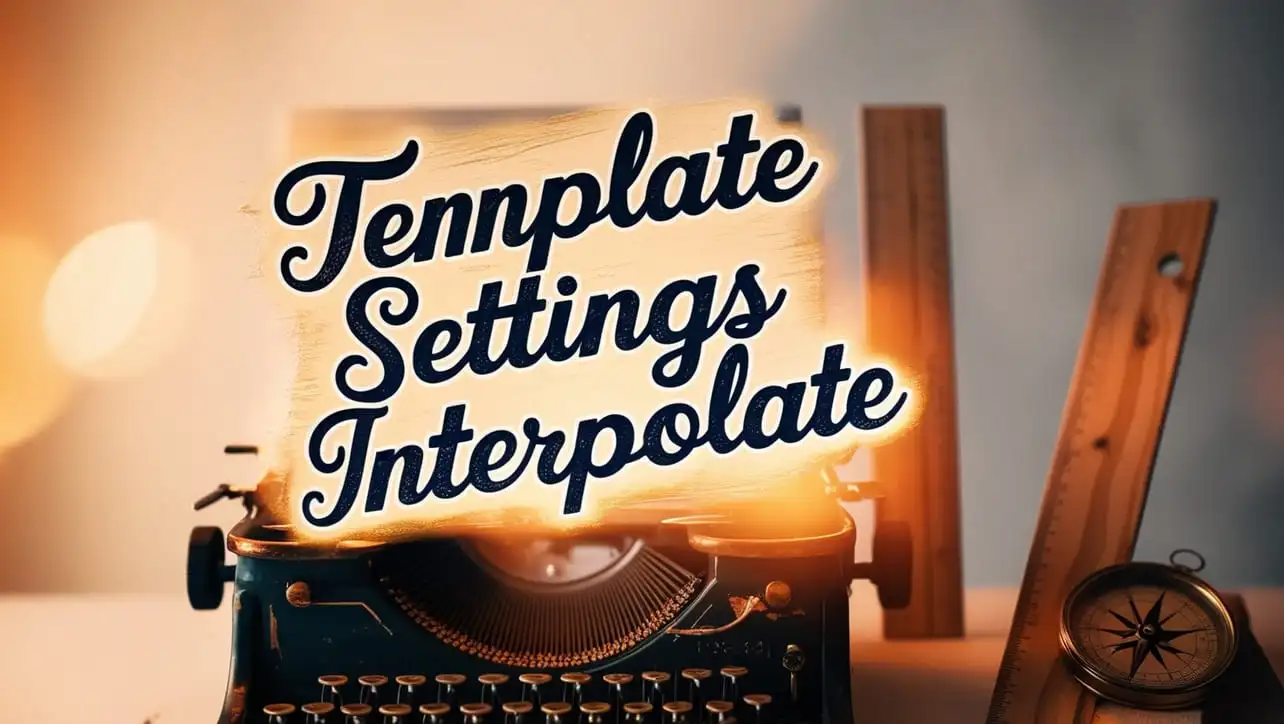
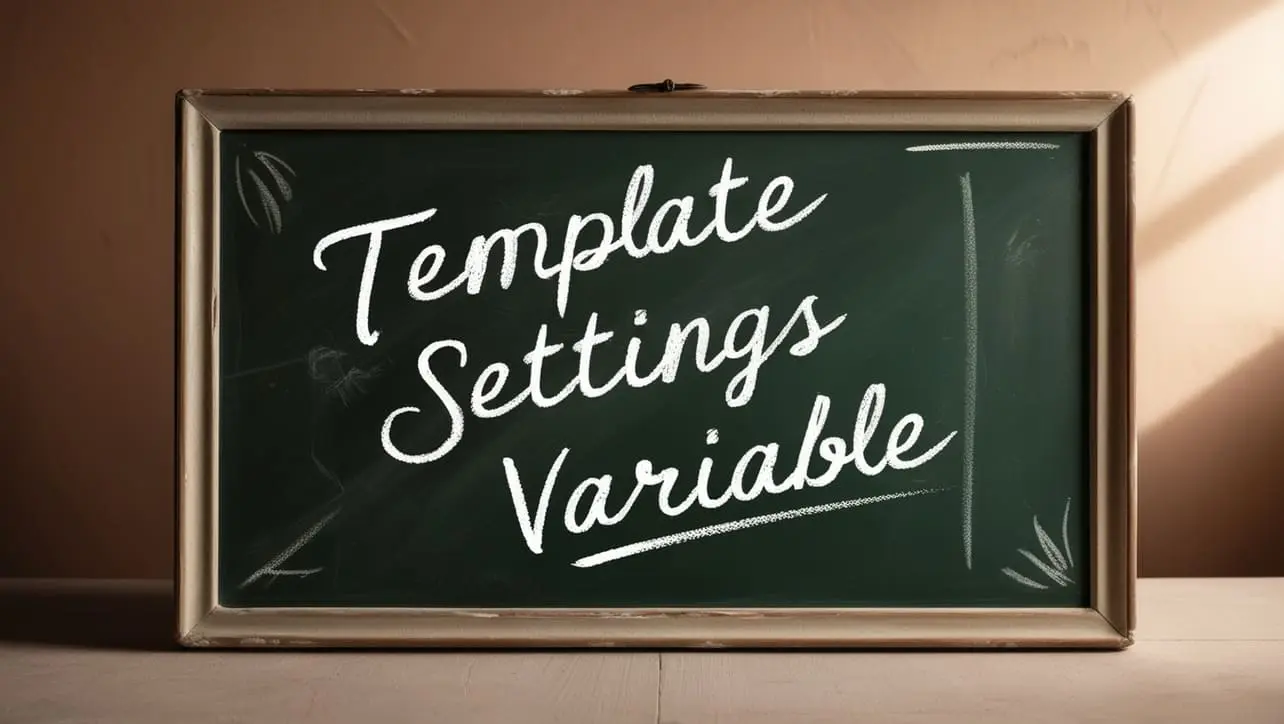
If you have any doubts regarding this article (Lodash _.tail() Array Method), please comment here. I will help you immediately.