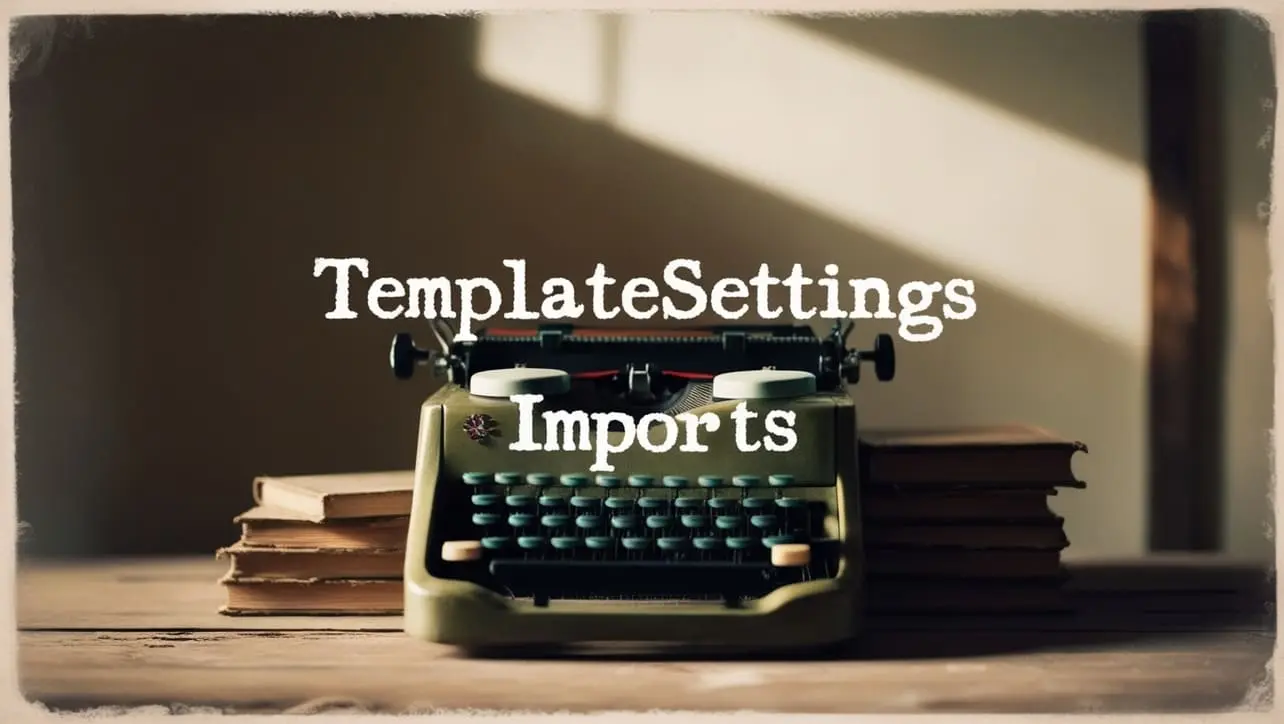
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedUniq() Array Method
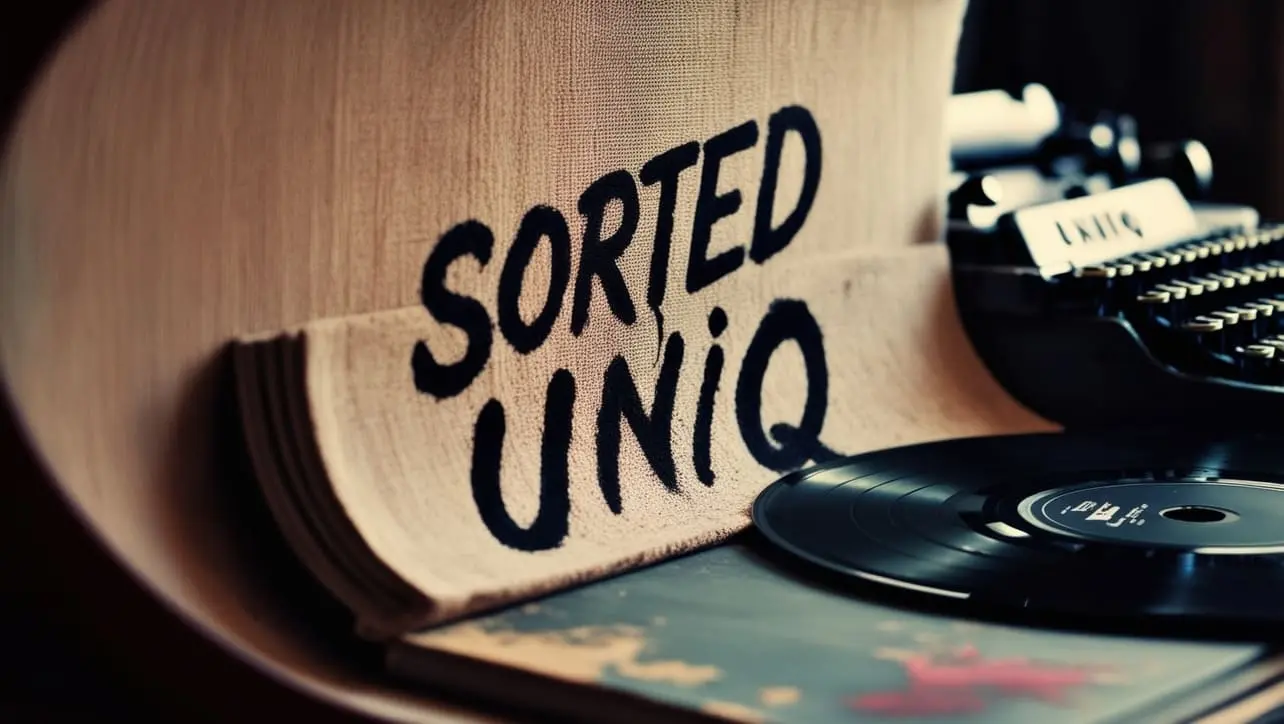
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient handling of arrays is fundamental.
Lodash, a robust utility library, offers a wealth of functions to streamline such operations. Among them, the _.sortedUniq()
method stands out, providing a convenient way to work with sorted arrays. This method proves invaluable when dealing with datasets requiring unique elements while maintaining a sorted order.
🧠 Understanding _.sortedUniq()
The _.sortedUniq()
method in Lodash is designed for arrays with sorted elements. It efficiently removes duplicate values, leaving a unique and sorted array. This functionality proves beneficial when working with data that requires consistency and order.
💡 Syntax
_.sortedUniq(array)
- array: The sorted array from which to remove duplicates.
📝 Example
Let's delve into a practical example to illustrate the utility of _.sortedUniq()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArrayWithDuplicates = [1, 2, 2, 3, 4, 4, 4, 5, 6, 6, 7];
const uniqueSortedArray = _.sortedUniq(sortedArrayWithDuplicates);
console.log(uniqueSortedArray);
// Output: [1, 2, 3, 4, 5, 6, 7]
In this example, the sortedArrayWithDuplicates is processed by _.sortedUniq()
, resulting in a new array without duplicate values and maintaining the original order.
🏆 Best Practices
Ensure Sorted Input:
Before applying
_.sortedUniq()
, ensure that the input array is sorted. This method is optimized for sorted arrays, and using it on an unsorted array may not yield the expected results.example.jsCopiedconst unsortedArray = [3, 1, 2, 4, 3, 5]; const sortedUniqResult = _.sortedUniq(unsortedArray); console.log(sortedUniqResult); // Output: [3, 1, 2, 4, 5] (result may not be as expected)
Optimize for Sorted Data:
If you are frequently working with sorted datasets that require unique elements, consider maintaining the sorted order throughout your data pipeline. This can optimize performance when utilizing
_.sortedUniq()
.example.jsCopied// Include Lodash library (ensure it's installed via npm) const _ = require('lodash'); // Assume this function fetches and returns sorted data from an API const fetchSortedDataFromAPI = () => { return /* ...fetch and return sorted data... */; }; // Fetch sorted data const sortedData = fetchSortedDataFromAPI(); // Optimize for sorted data by directly using _.sortedUniq() const uniqueSortedData = _.sortedUniq(sortedData); console.log(uniqueSortedData);
📚 Use Cases
Maintaining Unique Values in Sorted Data:
When dealing with datasets that must remain sorted,
_.sortedUniq()
ensures the removal of duplicate elements while preserving the order.example.jsCopiedconst sortedDataWithDuplicates = /* ...fetch data from API or elsewhere... */; const uniqueSortedData = _.sortedUniq(sortedDataWithDuplicates); console.log(uniqueSortedData);
Data Normalization:
In scenarios where you need to normalize sorted data by removing duplicate entries,
_.sortedUniq()
provides a clean and efficient solution.example.jsCopiedconst normalizedData = _.sortedUniq(/* ...your sorted data... */); console.log(normalizedData);
🎉 Conclusion
The _.sortedUniq()
method in Lodash is a powerful tool for managing arrays with sorted elements. Its simplicity and efficiency make it an excellent choice for scenarios where maintaining order and uniqueness is crucial. By incorporating this method into your code, you can enhance both the performance and reliability of your array manipulations.
Explore the capabilities of Lodash and streamline your array operations with _.sortedUniq()
!
👨💻 Join our Community:
Author
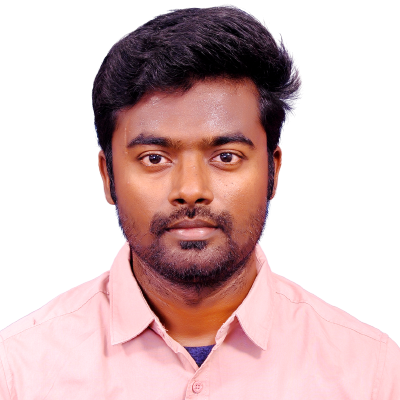
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
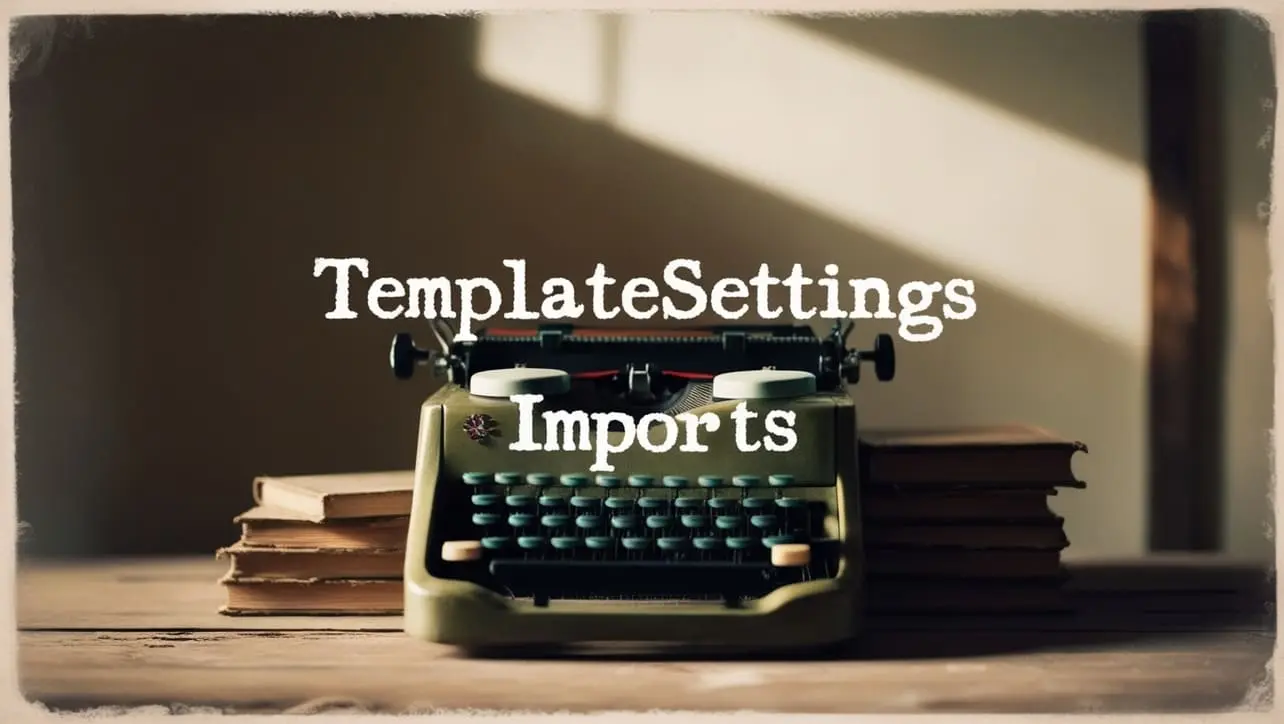
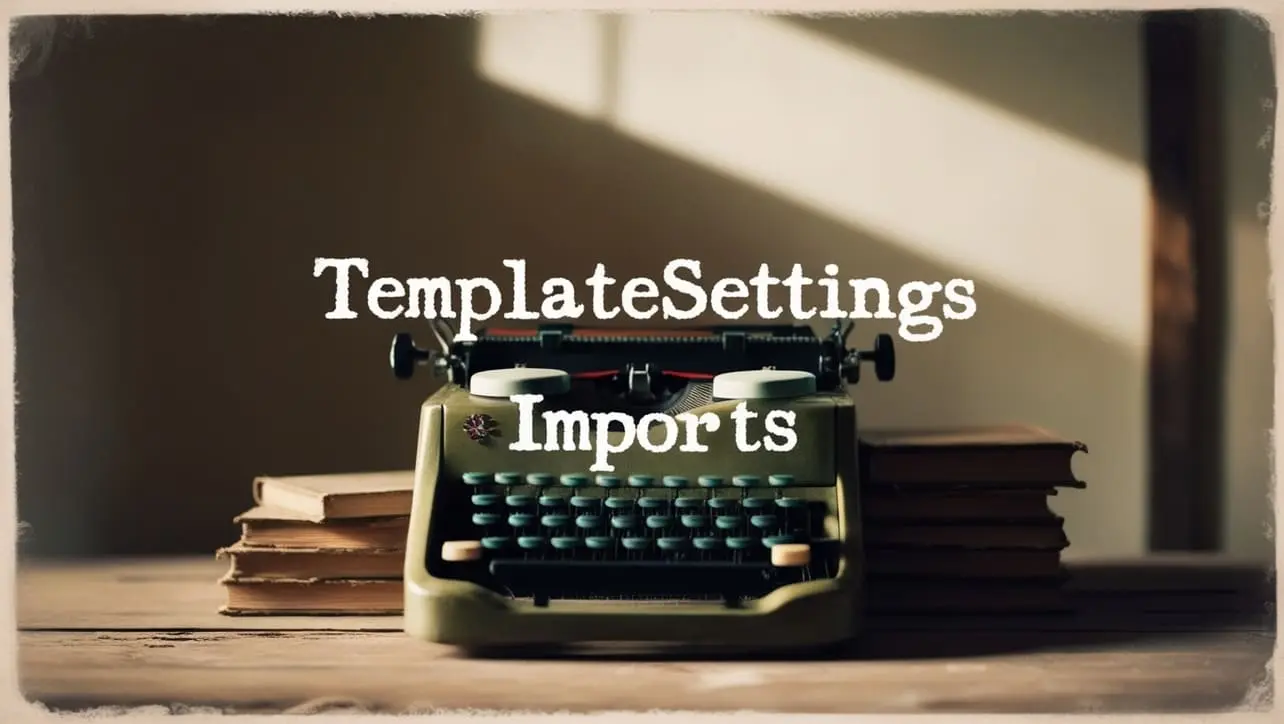
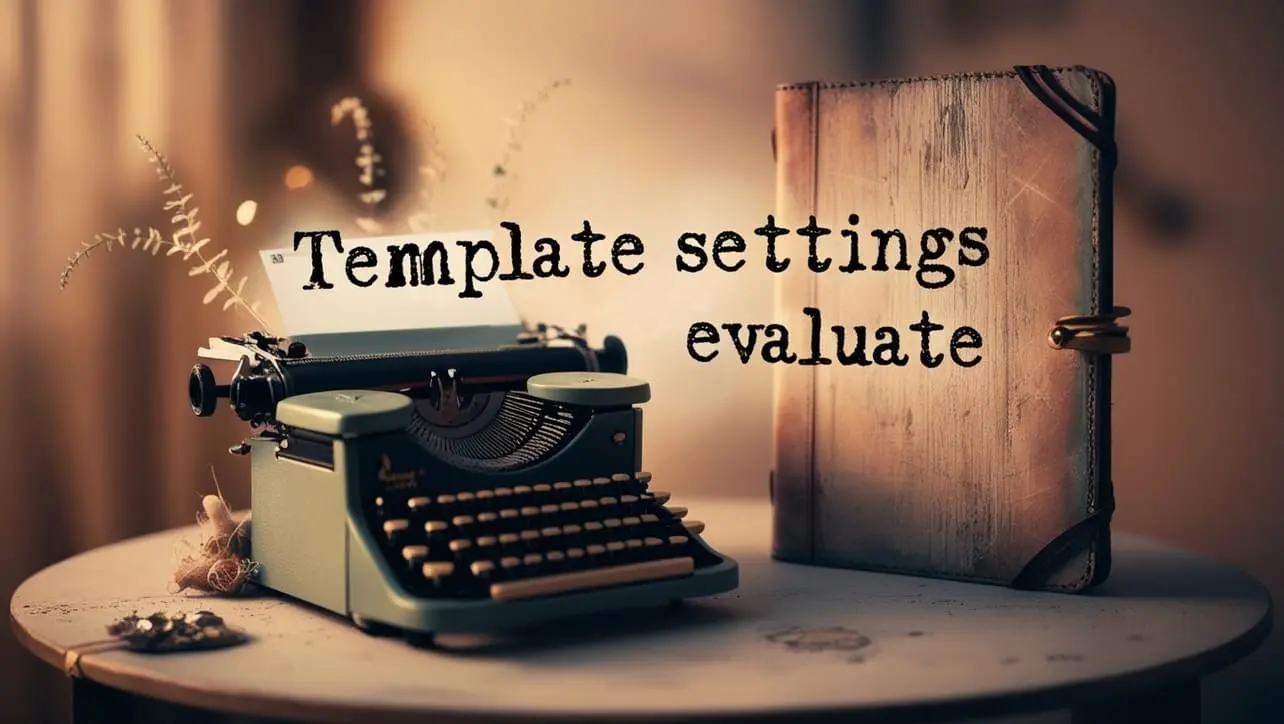
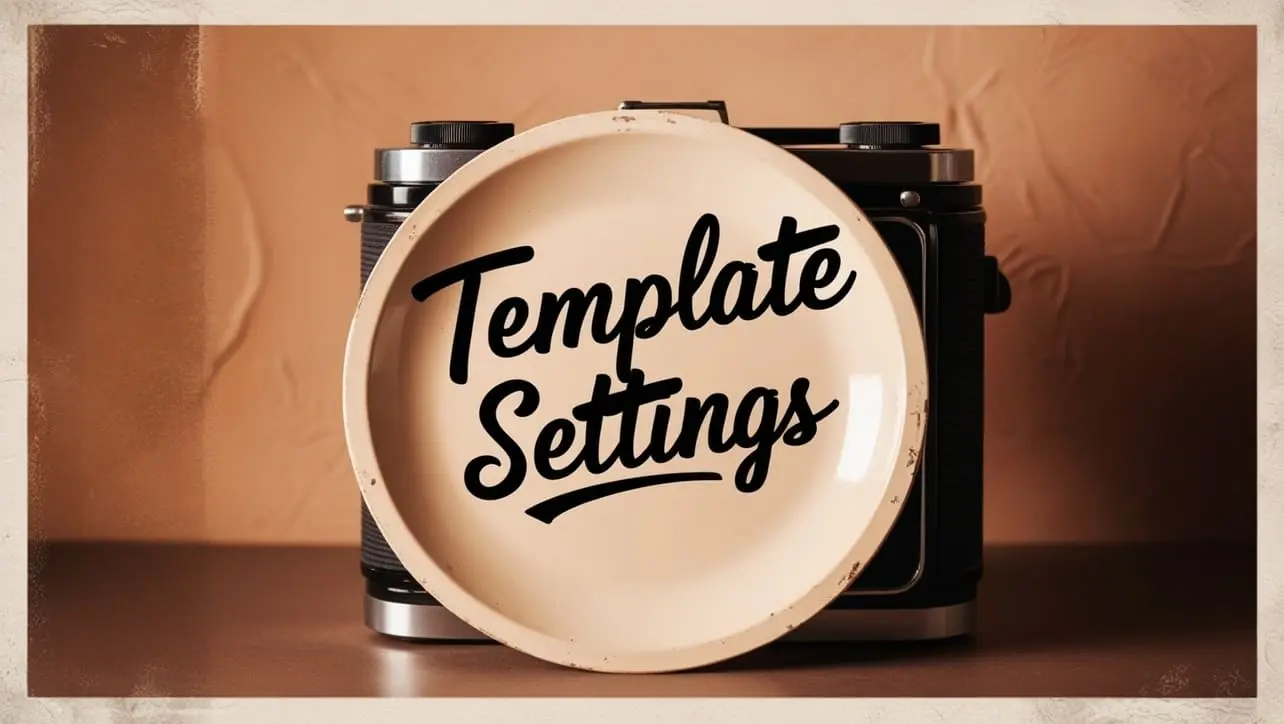
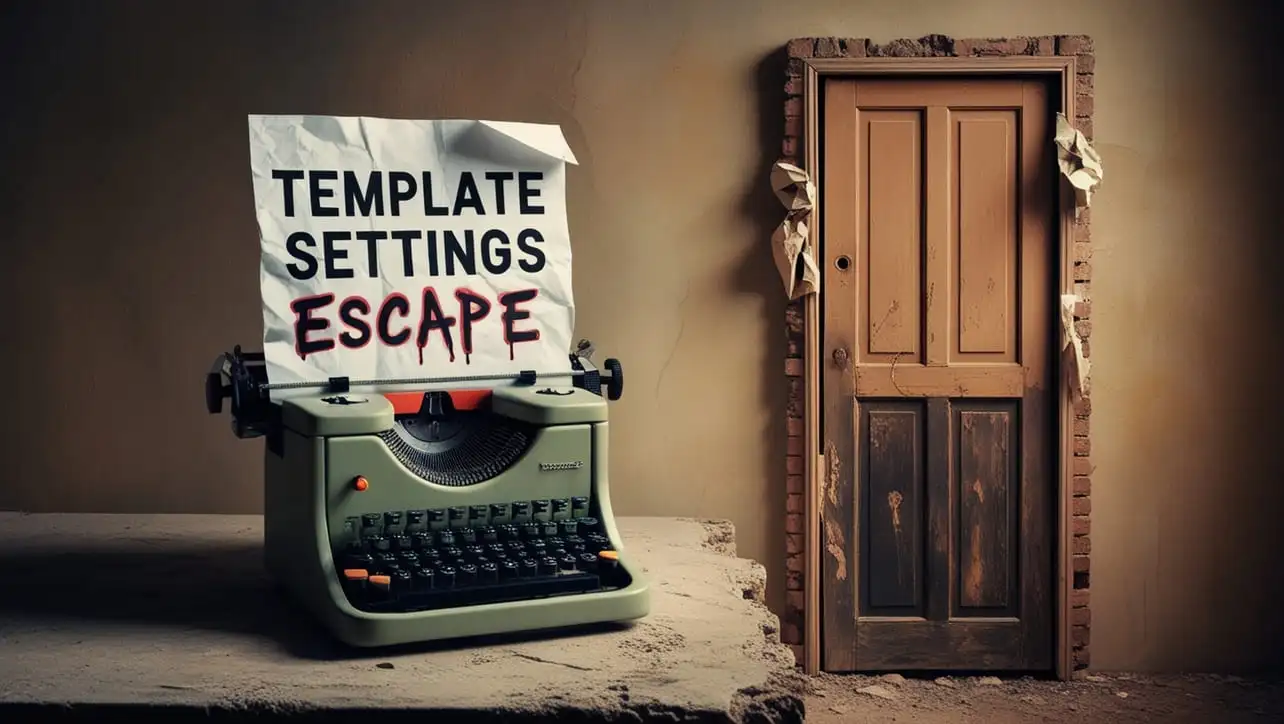
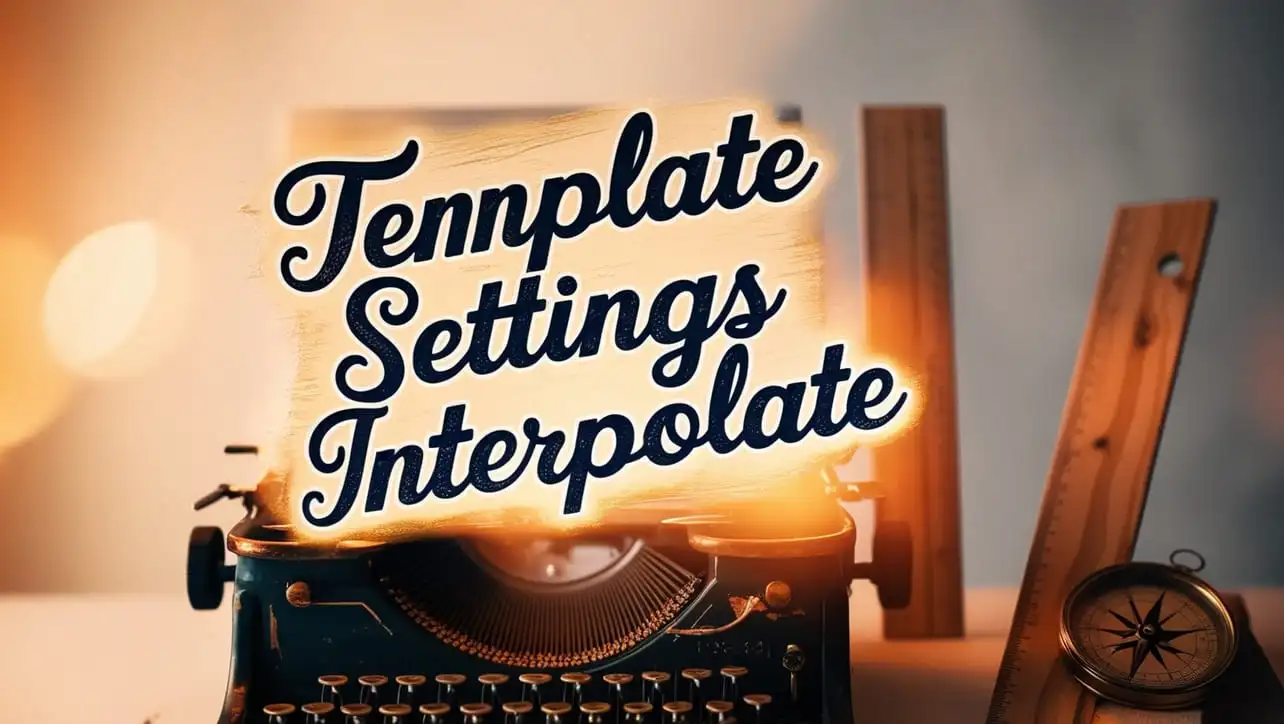
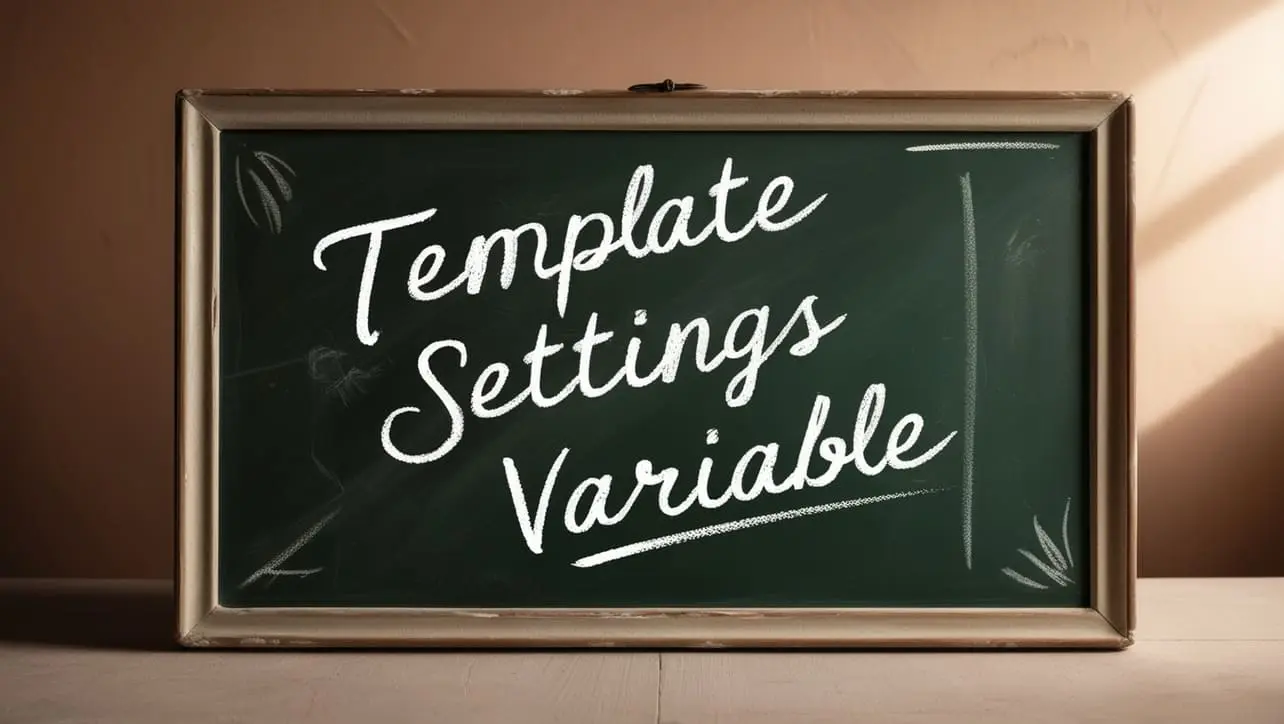
If you have any doubts regarding this article (Lodash _.sortedUniq() Array Method), please comment here. I will help you immediately.