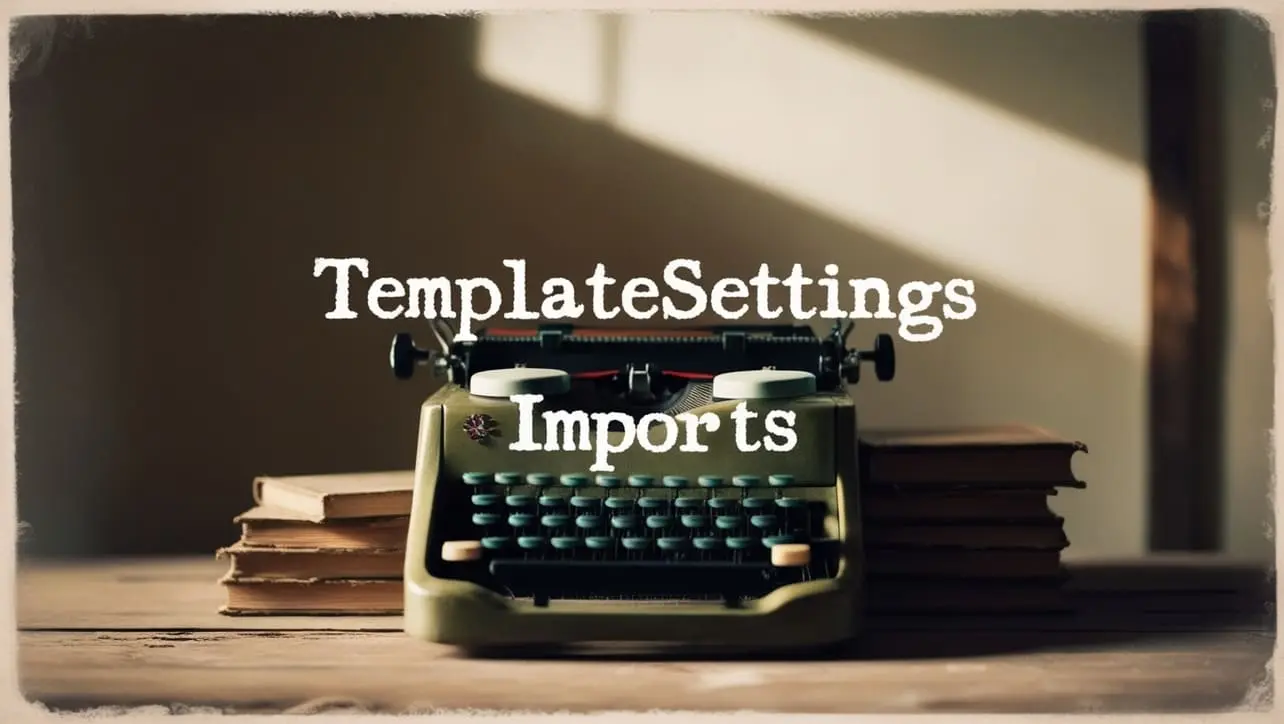
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedLastIndexOf() Array Method
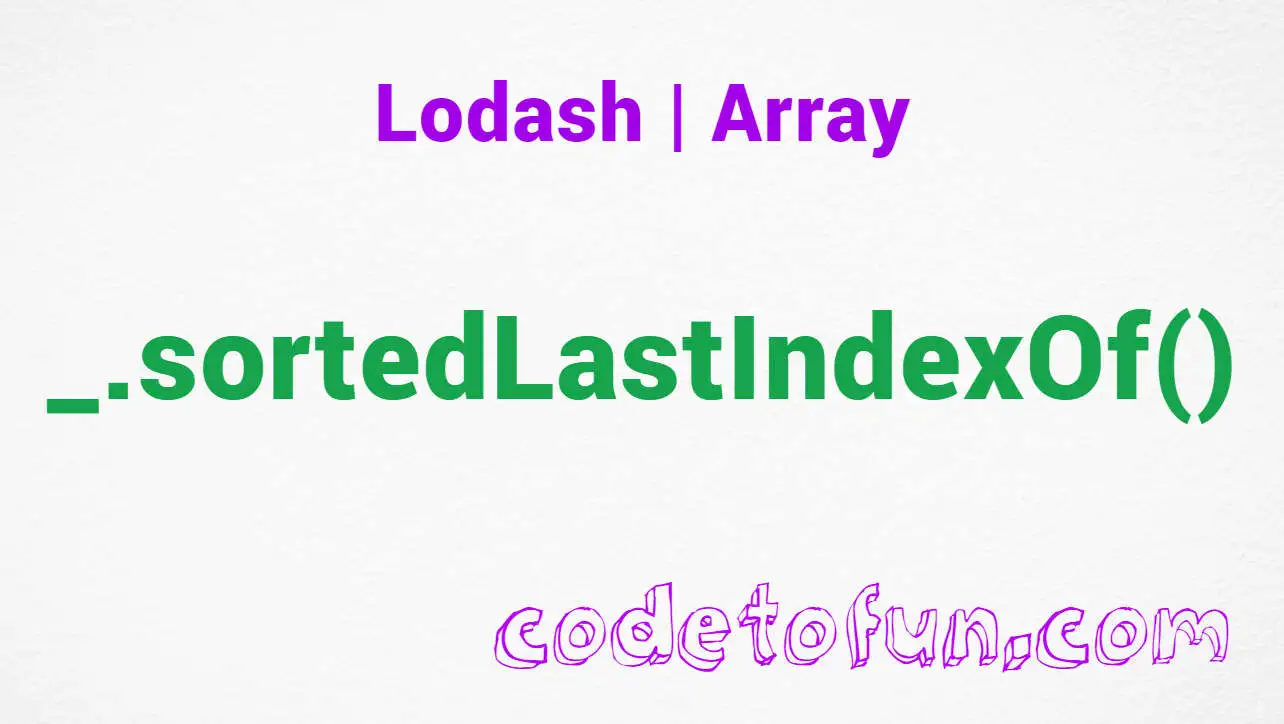
Photo Credit to CodeToFun
🙋 Introduction
Sorting arrays is a common task in JavaScript, and Lodash provides a powerful tool for this with the _.sortedLastIndexOf()
method.
This method allows developers to efficiently find the index of the last occurrence of a value in a sorted array. This can be invaluable when working with ordered data sets and searching for specific elements.
🧠 Understanding _.sortedLastIndexOf()
The _.sortedLastIndexOf()
method in Lodash performs a binary search on a sorted array to find the index of the last occurrence of a specified value.
💡 Syntax
_.sortedLastIndexOf(array, value)
- array: The sorted array to search.
- value: The value to search for.
📝 Example
Let's dive into a practical example to illustrate the usage of _.sortedLastIndexOf()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [1, 2, 2, 2, 3, 4, 5];
const targetValue = 2;
const lastIndex = _.sortedLastIndexOf(sortedArray, targetValue);
console.log(lastIndex);
// Output: 3
In this example, the sortedArray is searched for the last occurrence of the value 2, and the index 3 is returned.
🏆 Best Practices
Use with Sorted Arrays:
Ensure that the input array is sorted before using
_.sortedLastIndexOf()
. The method's efficiency relies on the array being in ascending order.example.jsCopiedconst unsortedArray = [3, 1, 4, 2, 5]; const unsortedIndex = _.sortedLastIndexOf(unsortedArray, 2); console.log(unsortedIndex); // Output: -1 (Incorrect result on an unsorted array)
Handle Non-Existent Values:
Handle cases where the target value does not exist in the array. The method returns -1 when the value is not found.
example.jsCopiedconst nonExistentValue = 6; const nonExistentIndex = _.sortedLastIndexOf(sortedArray, nonExistentValue); console.log(nonExistentIndex); // Output: -1
Leverage Binary Search Efficiency:
Take advantage of the binary search algorithm employed by
_.sortedLastIndexOf()
for efficient searches in large sorted arrays.example.jsCopiedconst largeSortedArray = [...Array(100000).keys()]; const targetValue = 50000; const efficientIndex = _.sortedLastIndexOf(largeSortedArray, targetValue); console.log(efficientIndex); // Output: 50000
📚 Use Cases
Finding Last Occurrence:
The primary use case for
_.sortedLastIndexOf()
is finding the index of the last occurrence of a value in a sorted array.example.jsCopiedconst lastOccurrenceIndex = _.sortedLastIndexOf(sortedArray, 2); console.log(lastOccurrenceIndex); // Output: 3
Search in Ranges:
Use this method to find the last index within a specific range of a sorted array.
example.jsCopiedconst rangeStart = 1; const rangeEnd = 4; const lastIndexInRange = _.sortedLastIndexOf(sortedArray, 2, rangeStart, rangeEnd); console.log(lastIndexInRange); // Output: 3
🎉 Conclusion
The _.sortedLastIndexOf()
method in Lodash is a valuable addition to the toolkit of any JavaScript developer working with sorted arrays. Its efficiency and ease of use make it an excellent choice for tasks involving the identification of the last occurrence of a value. By incorporating this method into your code, you can enhance both the performance and reliability of your array-related operations.
Explore the capabilities of _.sortedLastIndexOf()
in Lodash, and unlock the potential for streamlined searches in your sorted arrays!
👨💻 Join our Community:
Author
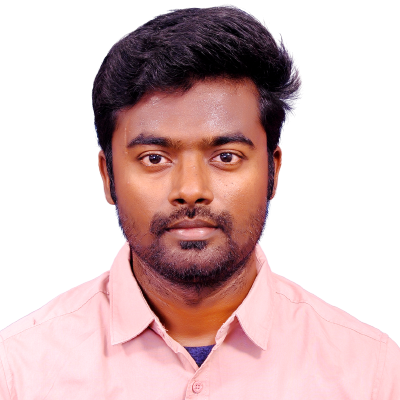
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
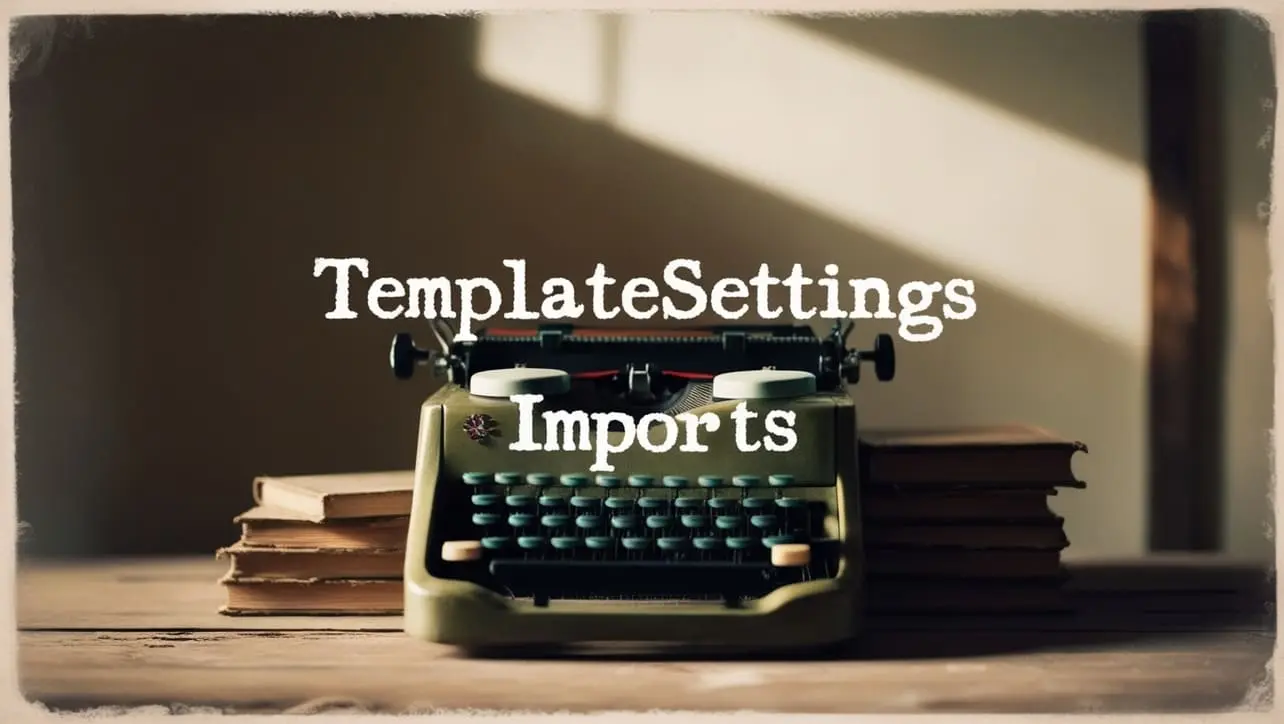
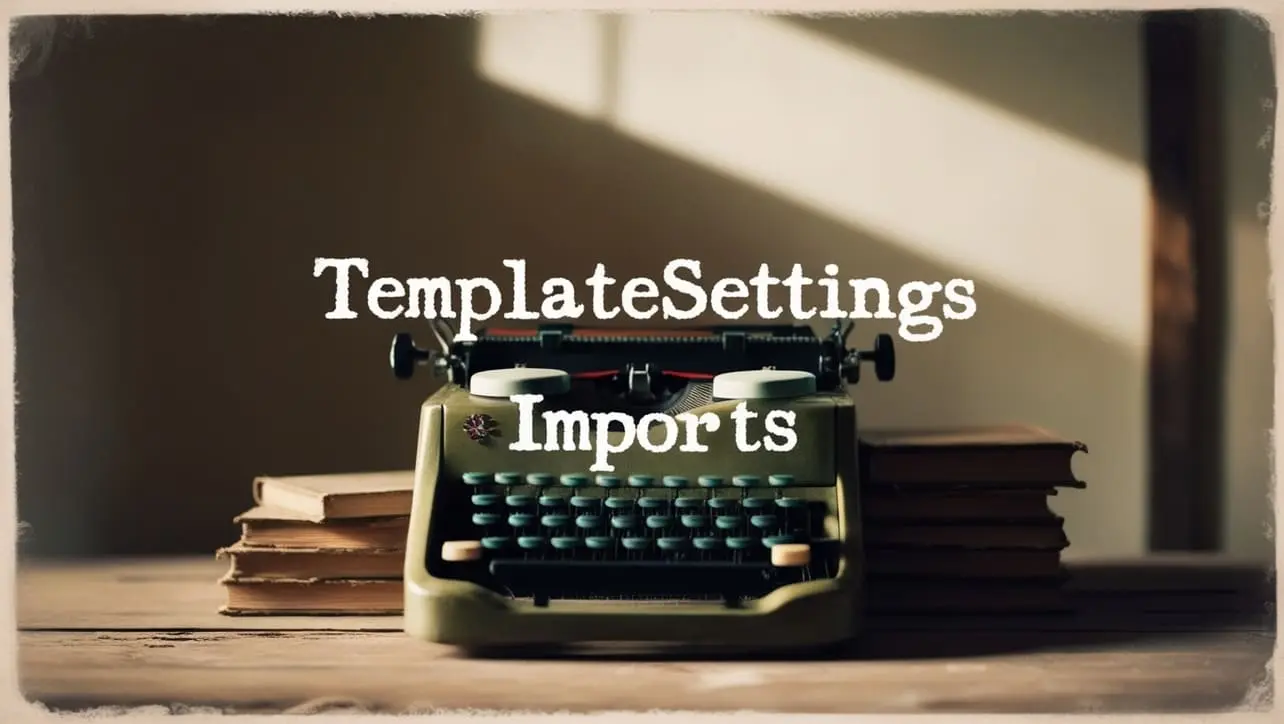
Lodash _.templateSettings.imports Property
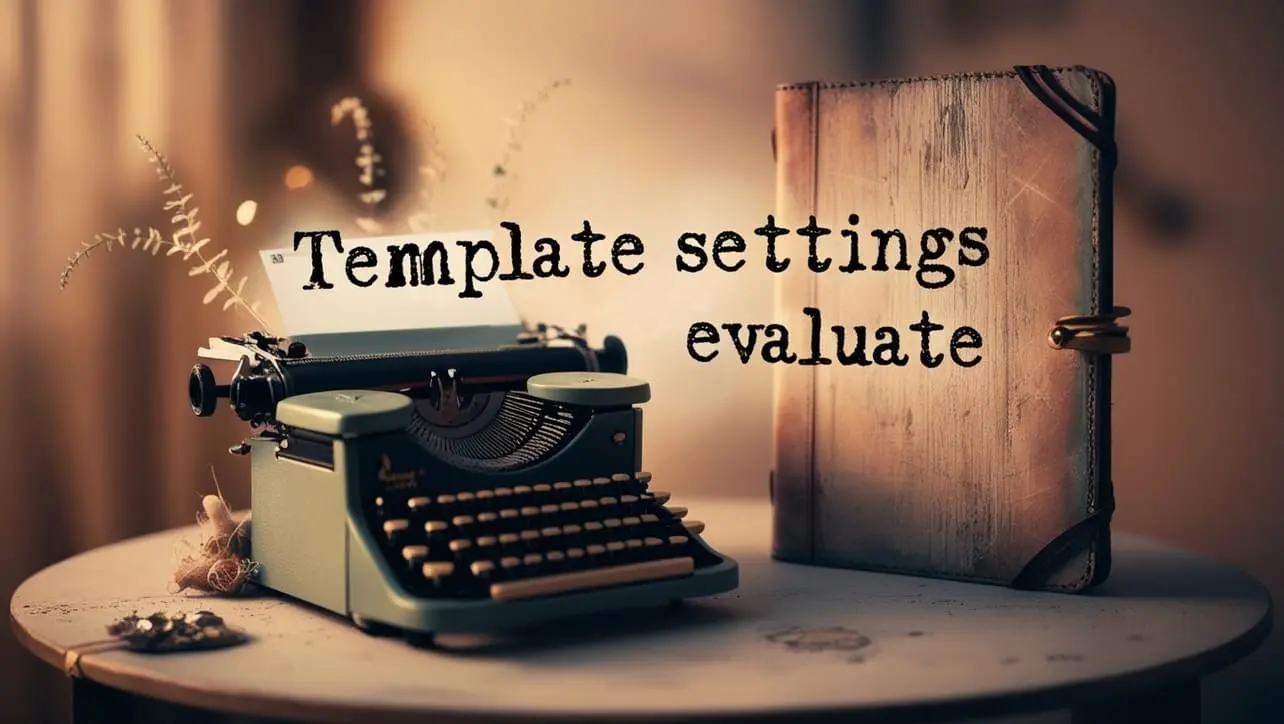
Lodash _.templateSettings.evaluate Property
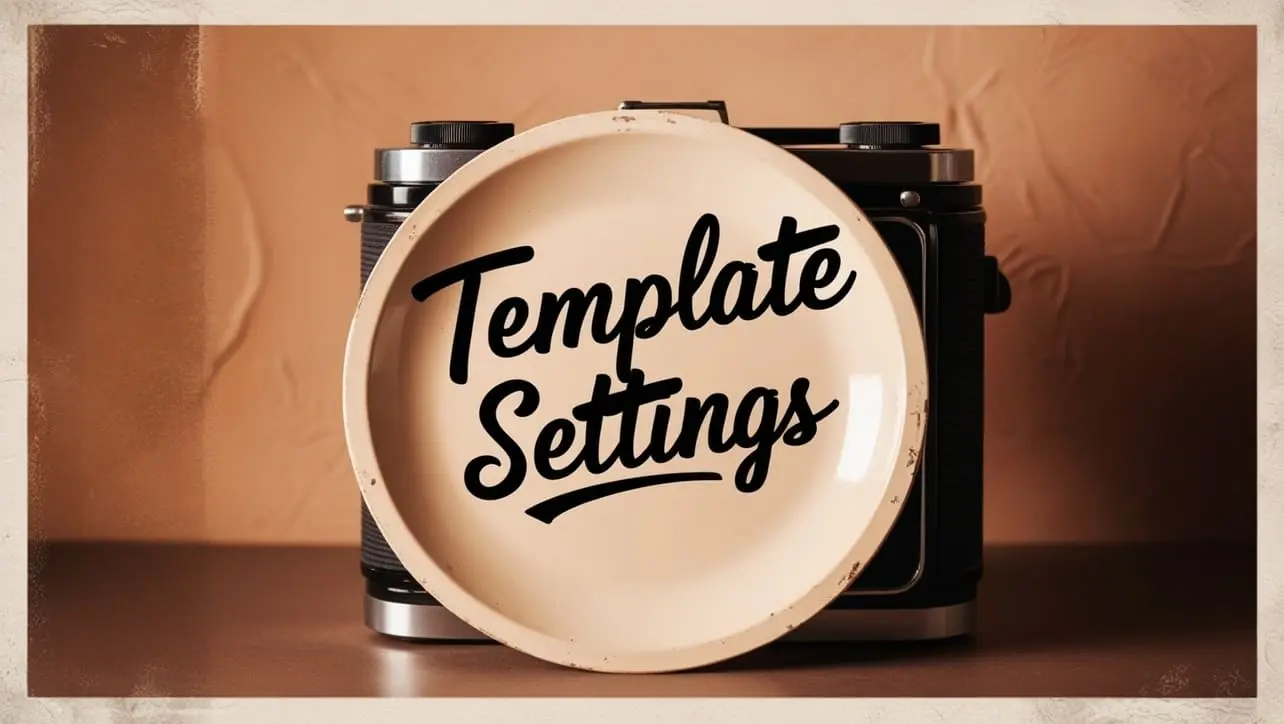
Lodash _.templateSettings Property
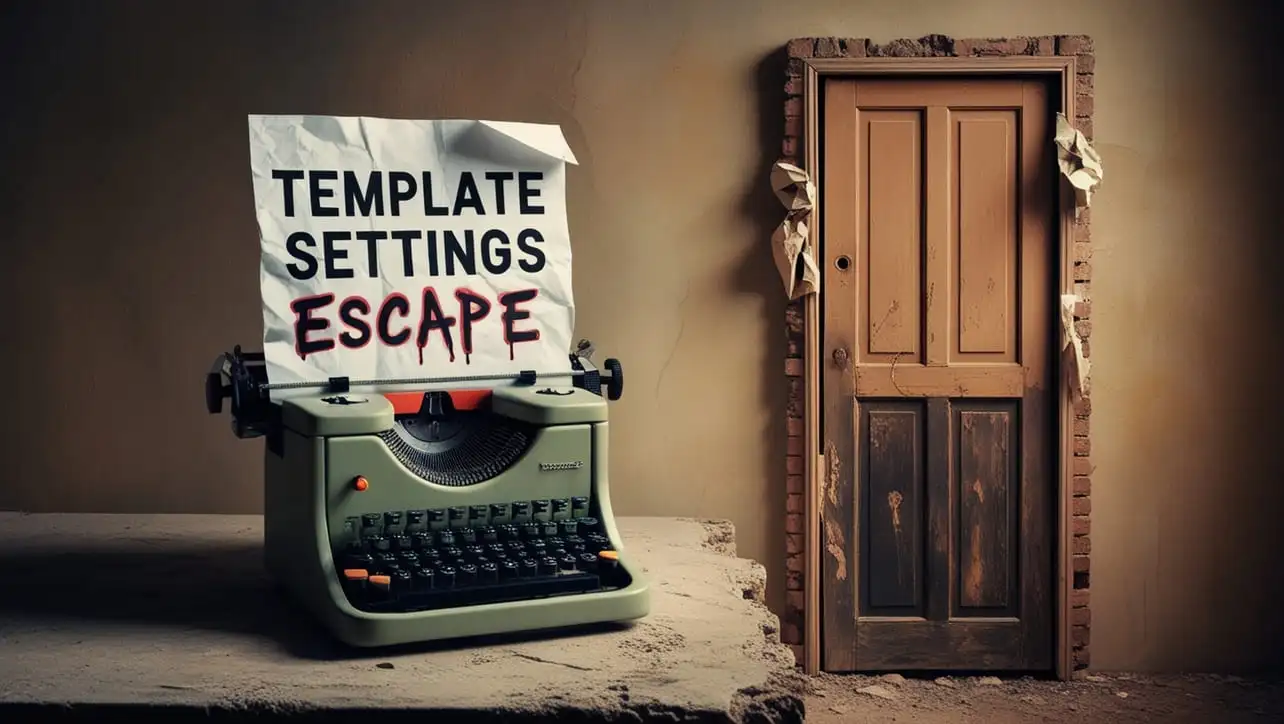
Lodash _.templateSettings.escape Property
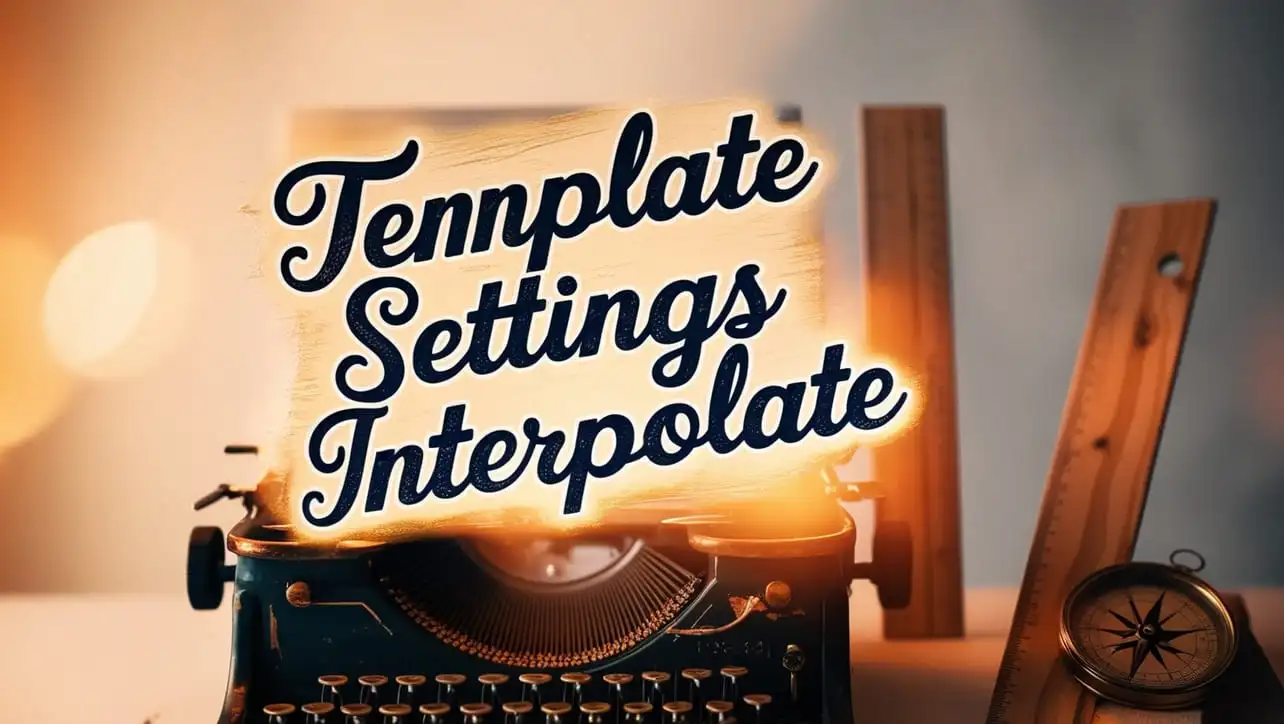
Lodash _.templateSettings.interpolate Property
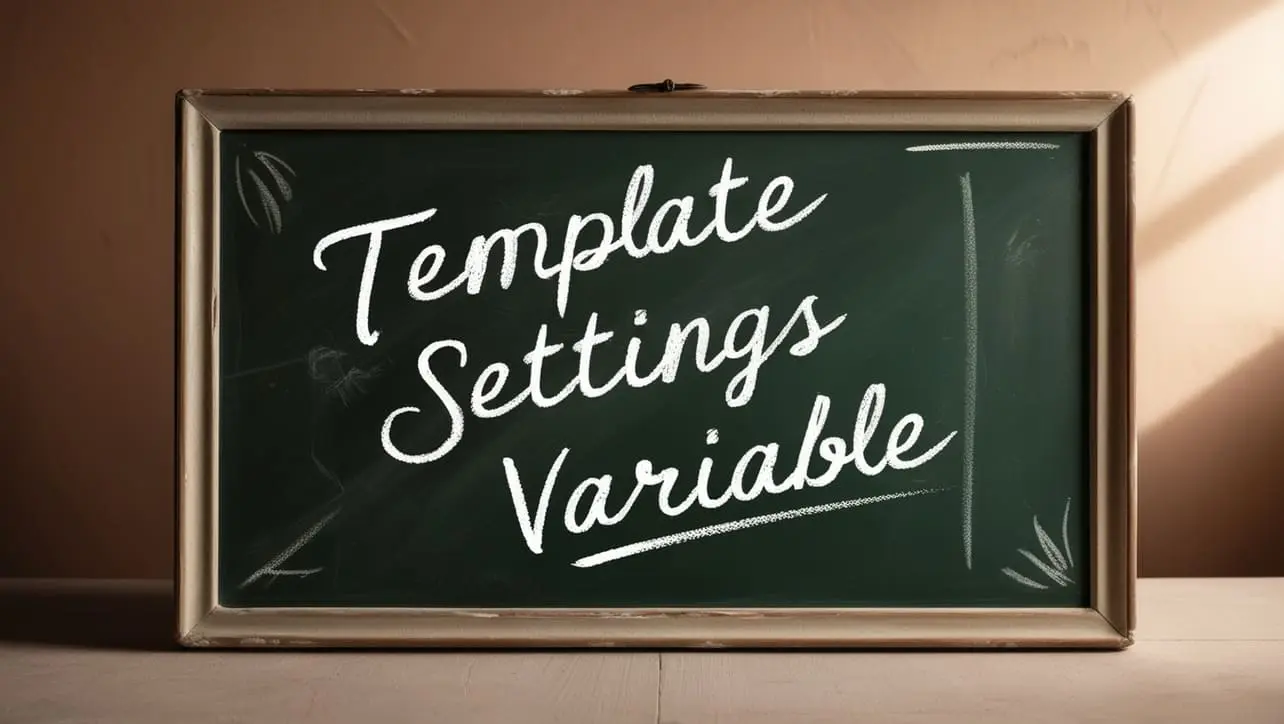
If you have any doubts regarding this article (Lodash _.sortedLastIndexOf() Array Method), please comment here. I will help you immediately.