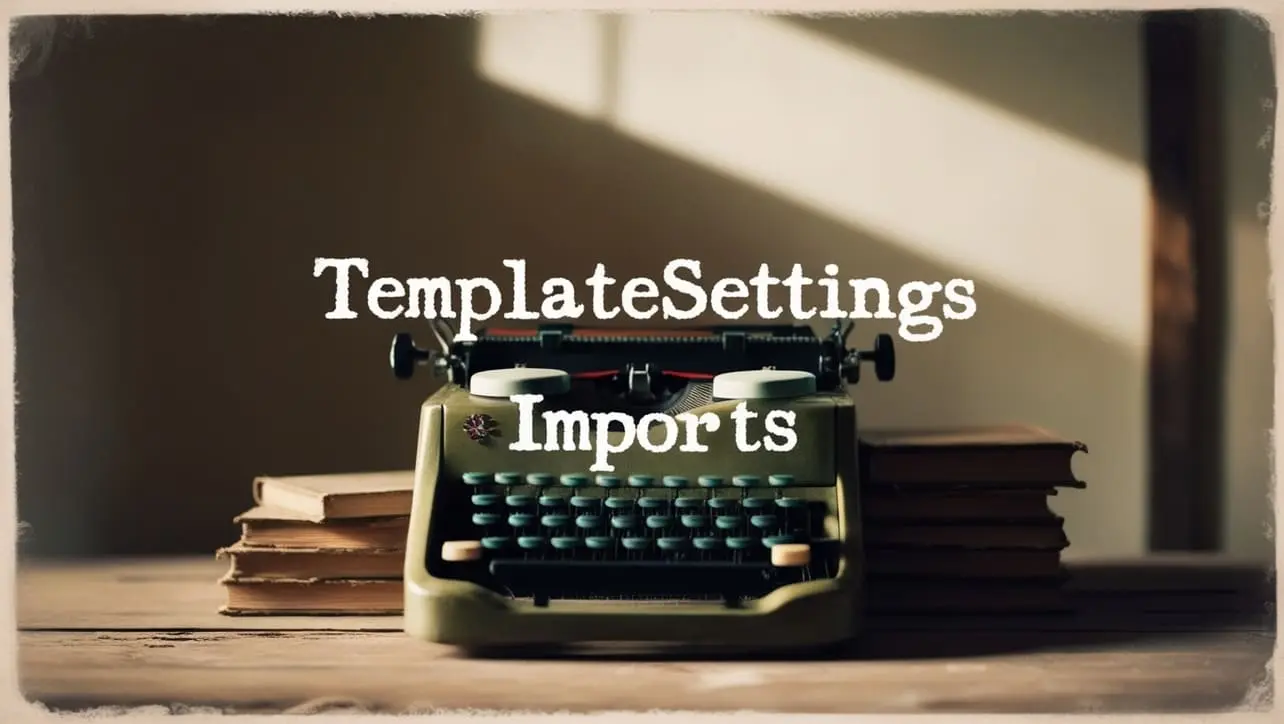
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedLastIndex() Array Method
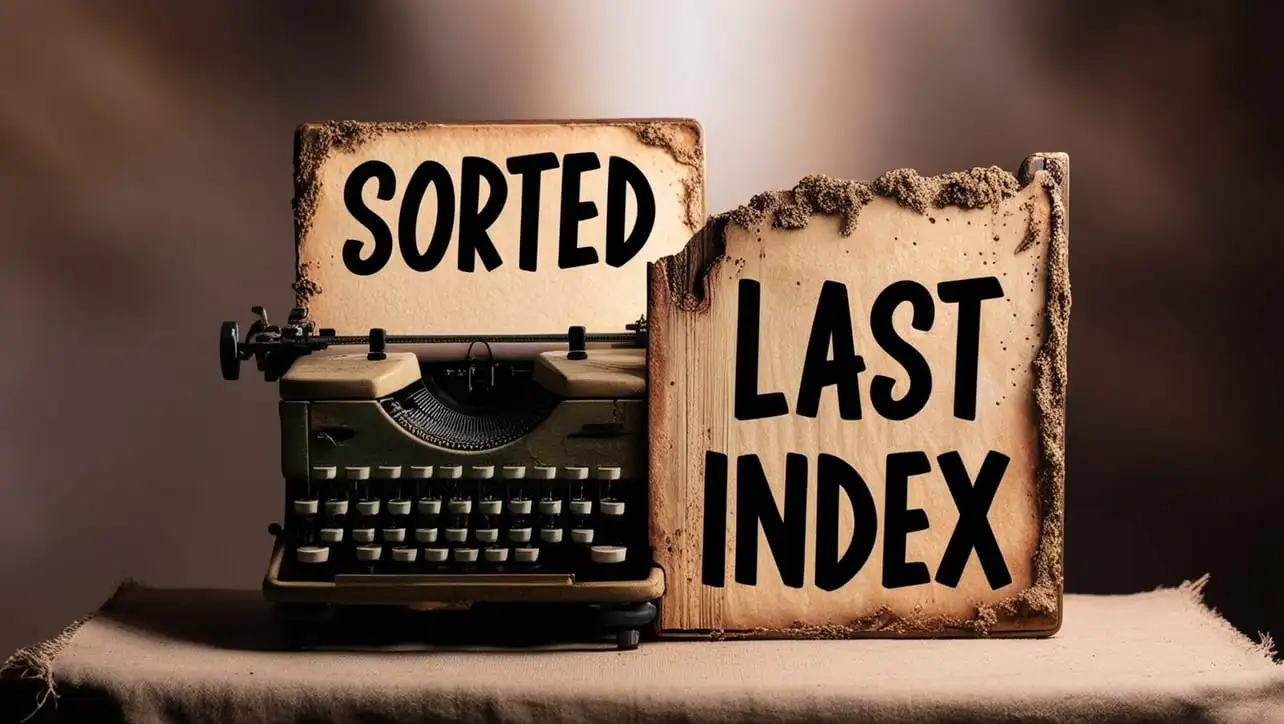
Photo Credit to CodeToFun
🙋 Introduction
When it comes to efficiently working with sorted arrays in JavaScript, the Lodash library provides a powerful utility function known as _.sortedLastIndex()
. This method simplifies the process of finding the index at which a given value should be inserted to maintain the array's sort order.
In this guide, we'll explore the intricacies of _.sortedLastIndex()
and how it can be leveraged to enhance your array manipulation tasks.
🧠 Understanding _.sortedLastIndex()
The _.sortedLastIndex()
method in Lodash is designed for sorted arrays and enables you to find the highest index at which a value should be inserted without disrupting the array's order.
💡 Syntax
_.sortedLastIndex(array, value)
- array: The sorted array to inspect.
- value: The value to evaluate.
📝 Example
Let's delve into a practical example to illustrate the functionality of _.sortedLastIndex()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [10, 20, 30, 40, 50];
const insertValue = 25;
const lastIndex = _.sortedLastIndex(sortedArray, insertValue);
console.log(lastIndex);
// Output: 3
In this example, _.sortedLastIndex()
determines that the value 25 should be inserted at index 3 to maintain the sorted order of sortedArray.
🏆 Best Practices
Ensure Array is Sorted:
Before using
_.sortedLastIndex()
, ensure that your array is sorted. If the array is unsorted, the results may not be as expected.example.jsCopiedconst unsortedArray = [30, 10, 40, 20, 50]; const unsortedIndex = _.sortedLastIndex(unsortedArray, 25); // Results may not be accurate console.log(unsortedIndex);
Handle Edge Cases:
Consider edge cases, such as an empty array or scenarios where the value is greater than all elements in the array. Implement appropriate error handling or default behaviors.
example.jsCopiedconst emptyArray = []; const emptyArrayIndex = _.sortedLastIndex(emptyArray, 10); // Returns: 0 const greaterValue = 60; const greaterValueIndex = _.sortedLastIndex(sortedArray, greaterValue); // Returns: 5 console.log(emptyArrayIndex); console.log(greaterValueIndex);
Leverage Result for Insertion:
Understand that the index returned by
_.sortedLastIndex()
represents the position at which the value should be inserted to maintain the array's sorted order.example.jsCopiedconst newIndex = _.sortedLastIndex(sortedArray, 35); sortedArray.splice(newIndex, 0, 35); console.log(sortedArray); // Output: [10, 20, 30, 35, 40, 50]
📚 Use Cases
Binary Search Augmentation:
_.sortedLastIndex()
is particularly useful in binary search algorithms, enhancing their efficiency when working with sorted arrays.example.jsCopied// Binary search using _.sortedLastIndex() const binarySearch = (arr, target) => { const index = _.sortedLastIndex(arr, target); return arr[index - 1] === target ? index - 1 : -1; }; const targetValue = 30; const searchResult = binarySearch(sortedArray, targetValue); console.log(searchResult); // Output: 2
Insertion in Sorted Lists:
When dealing with sorted lists or maintaining sorted data structures,
_.sortedLastIndex()
facilitates easy insertion.example.jsCopiedconst sortedList = [100, 200, 400, 500]; const insertNewValue = 300; const insertionIndex = _.sortedLastIndex(sortedList, insertNewValue); sortedList.splice(insertionIndex, 0, insertNewValue); console.log(sortedList); // Output: [100, 200, 300, 400, 500]
🎉 Conclusion
The _.sortedLastIndex()
method in Lodash is a valuable tool for JavaScript developers working with sorted arrays. Its ability to efficiently determine the insertion point for maintaining order makes it an essential component of various algorithms and data manipulation tasks.
Incorporate _.sortedLastIndex()
into your projects to optimize binary searches, facilitate sorted list maintenance, and streamline your array manipulation operations. Explore the vast capabilities of Lodash and unlock the potential of array manipulation with _.sortedLastIndex()
!
👨💻 Join our Community:
Author
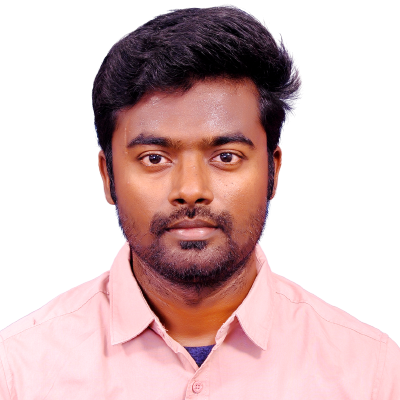
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
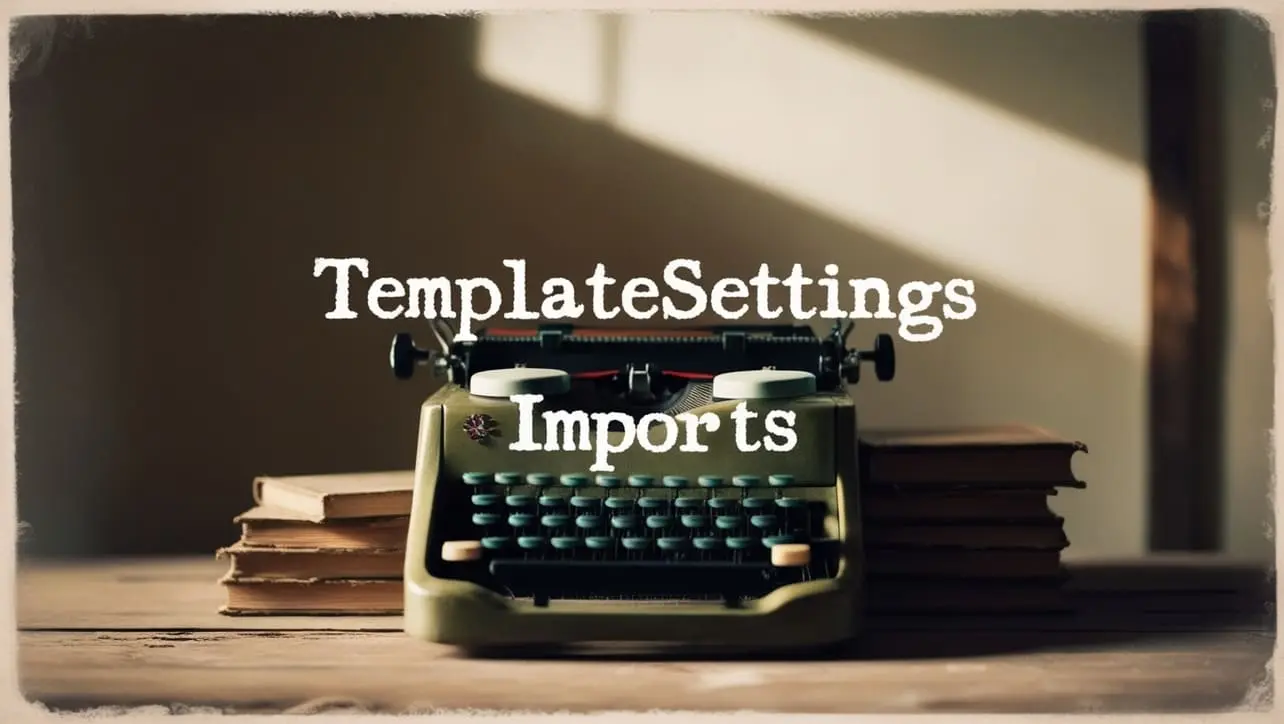
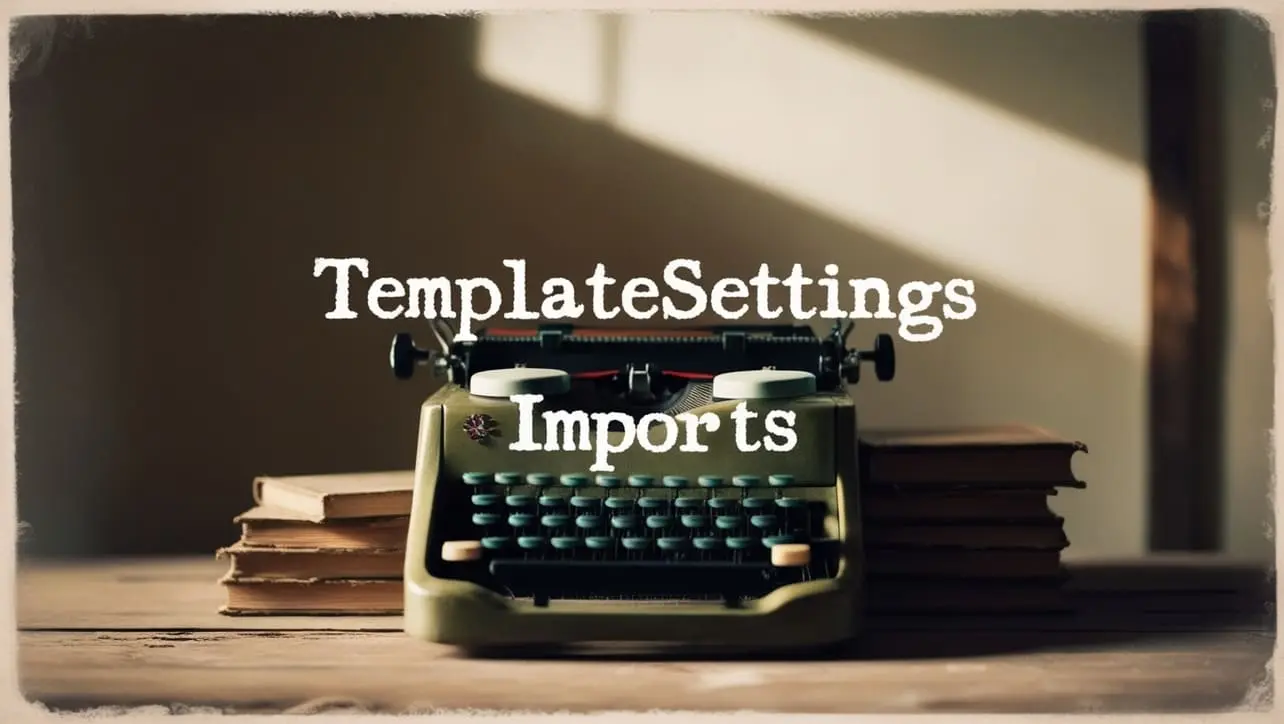
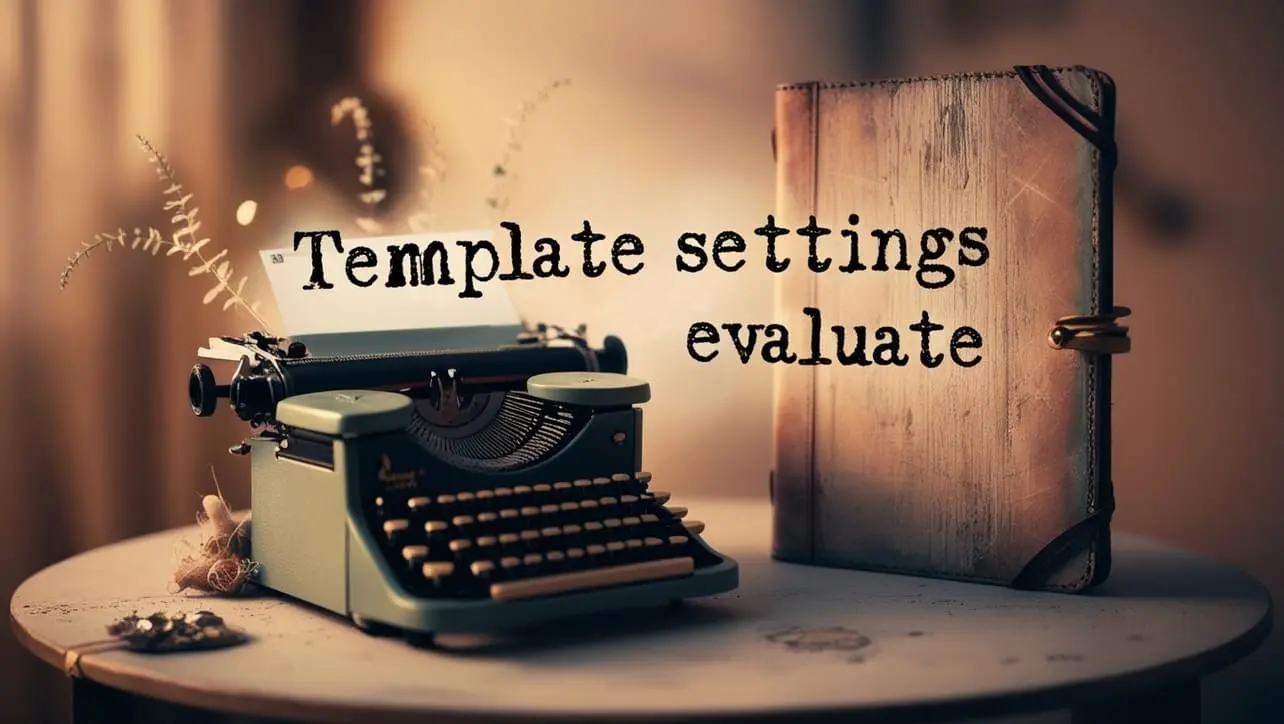
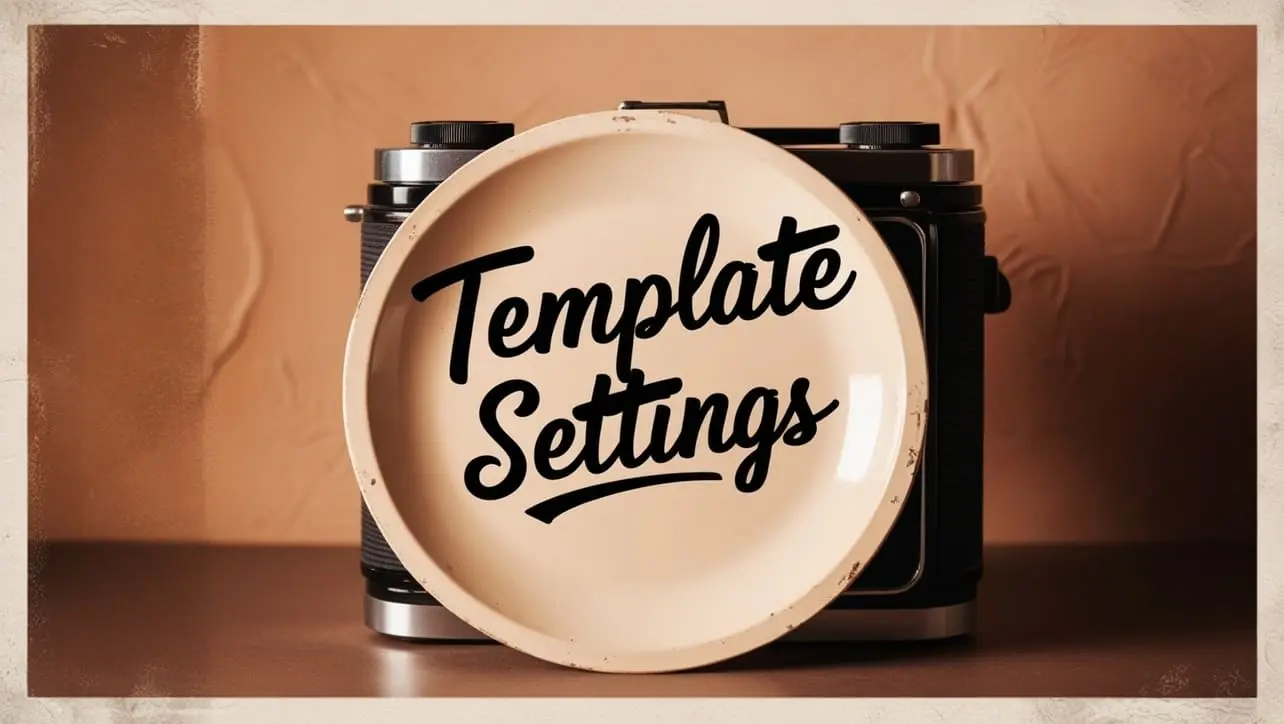
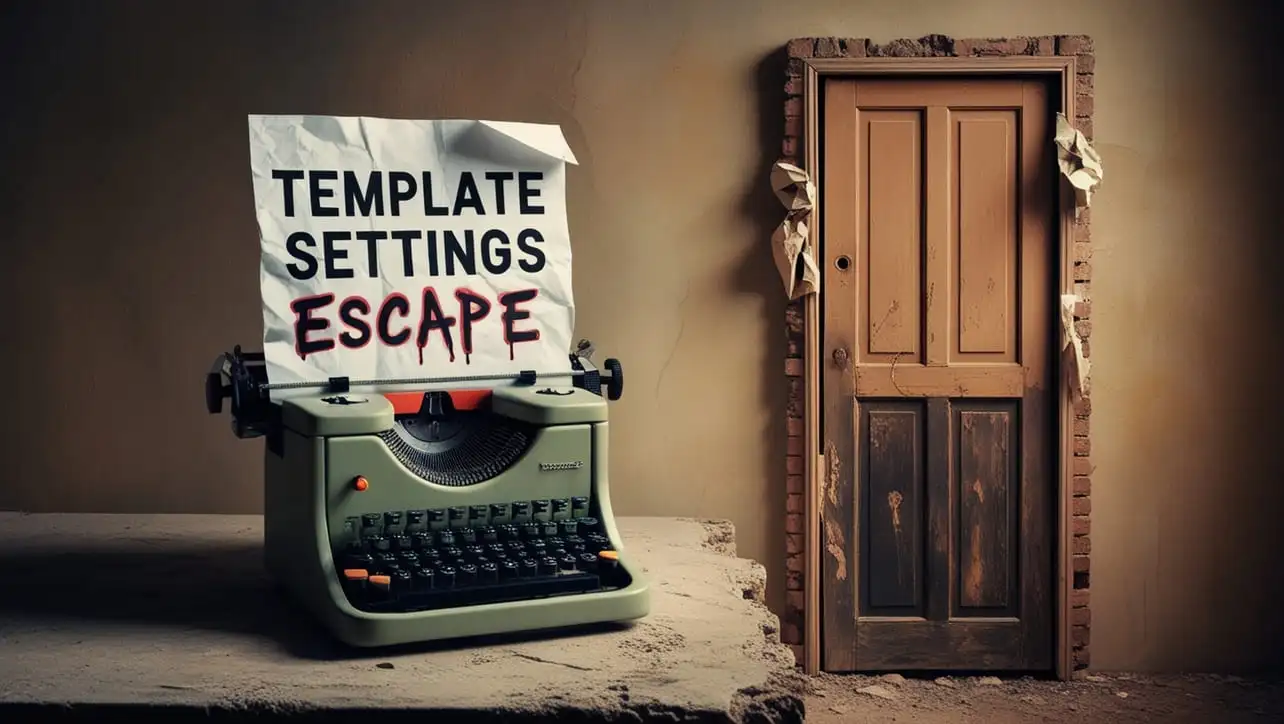
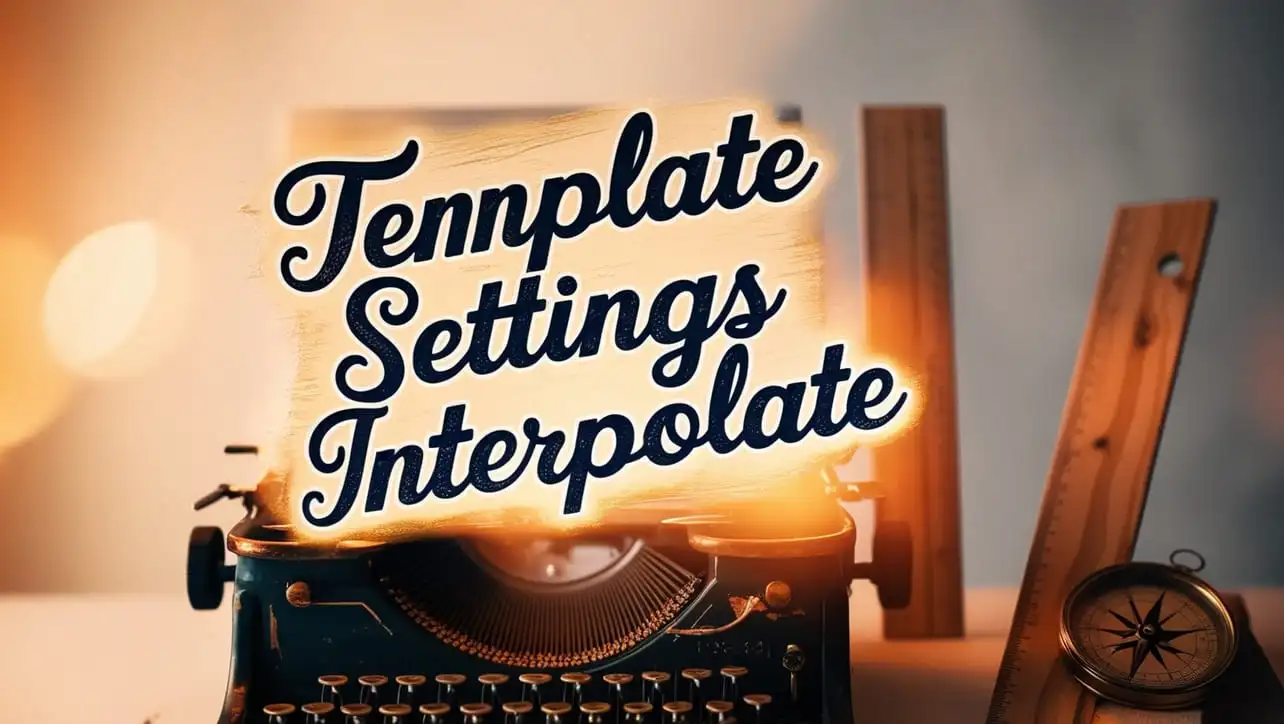
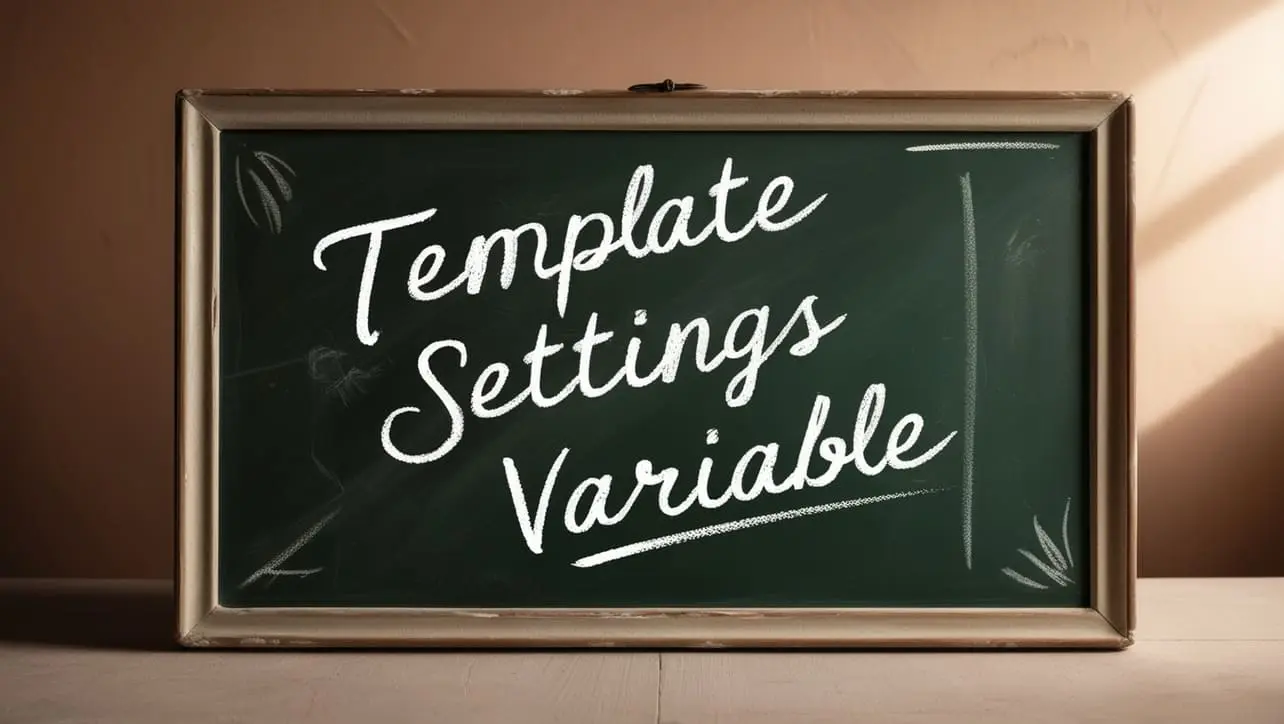
If you have any doubts regarding this article (Lodash _.sortedLastIndex() Array Method), please comment here. I will help you immediately.