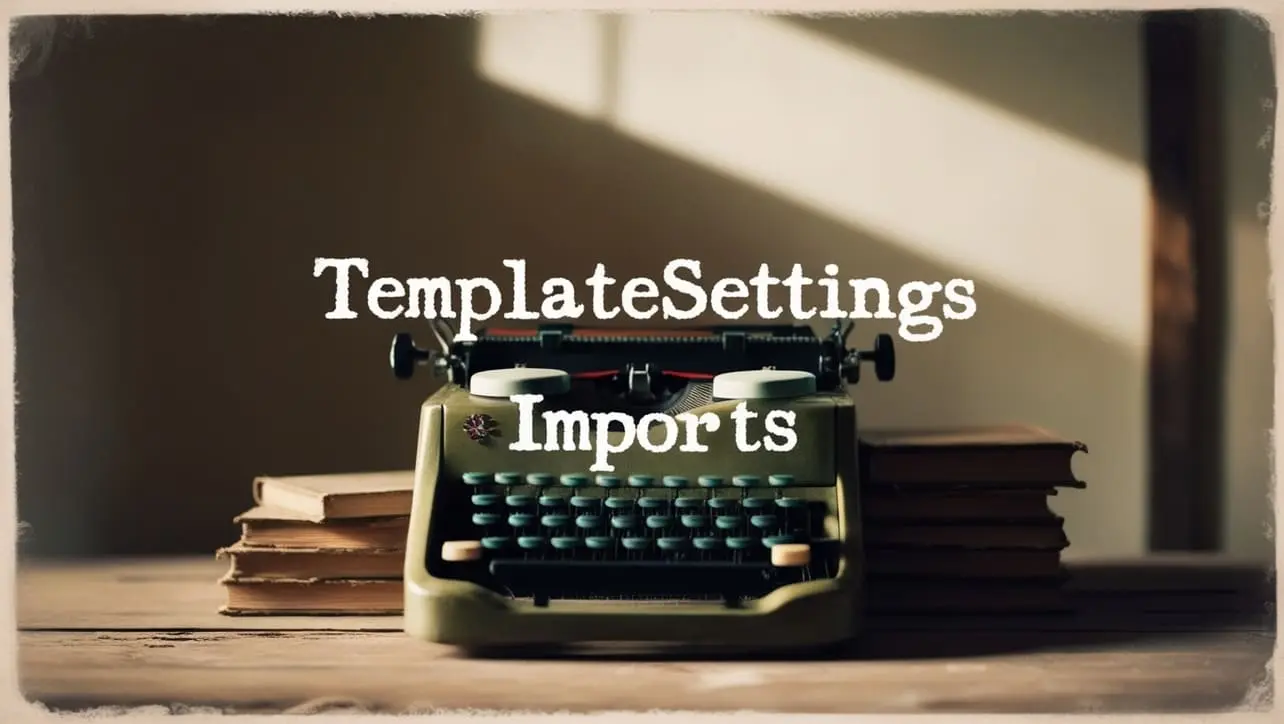
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedIndexOf() Array Method
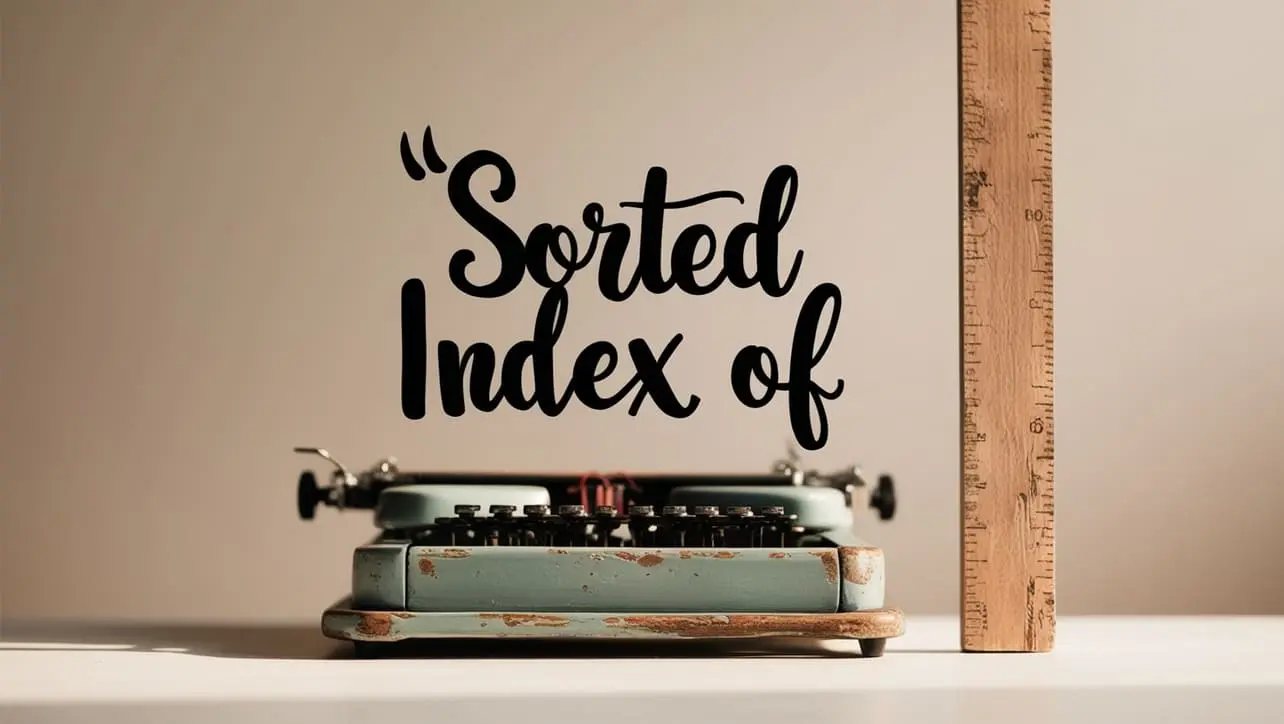
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, efficient searching and indexing within arrays are common tasks.
Lodash, a popular utility library, provides a range of helpful functions, and one such gem is the _.sortedIndexOf()
method. This method is designed for arrays that are already sorted, offering an optimized way to find the index of a specified value.
🧠 Understanding _.sortedIndexOf()
The _.sortedIndexOf()
method in Lodash is specifically crafted for sorted arrays. It performs a binary search to find the index of the first occurrence of a given value. This can be advantageous in scenarios where you're working with ordered datasets, as binary searches are more efficient than linear searches.
💡 Syntax
_.sortedIndexOf(array, value)
- array: The sorted array to search.
- value: The value to find in the array.
📝 Example
Let's explore a practical example to showcase the functionality of _.sortedIndexOf()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [1, 3, 5, 7, 9, 11, 13];
const targetValue = 7;
const indexOfValue = _.sortedIndexOf(sortedArray, targetValue);
console.log(indexOfValue);
// Output: 3
In this example, the sortedArray is searched for the value 7, and the method returns the index 3, which is the first occurrence of 7 in the sorted array.
🏆 Best Practices
Array Sorting:
Ensure that the input array is sorted before using
_.sortedIndexOf()
. If the array is not sorted, consider using _.indexOf() or sort the array first using _.sortBy() or other sorting methods.example.jsCopiedconst unsortedArray = [13, 5, 1, 7, 9, 3, 11]; const targetValue = 7; // Sort the array first const sortedArray = _.sortBy(unsortedArray); const indexOfValue = _.sortedIndexOf(sortedArray, targetValue); console.log(indexOfValue);
Value Existence Check:
Before utilizing the method, check if the target value exists in the array. If the value is not present, the method will still return a valid index, potentially leading to unexpected results.
example.jsCopiedconst nonExistentValue = 8; const indexOfNonExistentValue = _.sortedIndexOf(sortedArray, nonExistentValue); if (indexOfNonExistentValue === -1) { console.log('Value not found in the array'); } else { console.log('Index of the value:', indexOfNonExistentValue); }
📚 Use Cases
Efficient Searching in Sorted Data:
_.sortedIndexOf()
is ideal for scenarios where you have a sorted array, and you need to efficiently find the index of a specific value. This is common in applications dealing with time-series data, numerical datasets, or any sorted collection.example.jsCopiedconst timestampArray = /* ...fetch sorted timestamps from database or elsewhere... */; const targetTimestamp = /* ...get target timestamp... */; const indexOfTimestamp = _.sortedIndexOf(timestampArray, targetTimestamp); console.log(indexOfTimestamp);
Optimizing Range Queries:
When working with sorted arrays,
_.sortedIndexOf()
can be employed to optimize range queries, providing the starting index for the range.example.jsCopiedconst rangeStart = 5; const rangeEnd = 10; const startIndex = _.sortedIndexOf(sortedArray, rangeStart); const endIndex = _.sortedIndexOf(sortedArray, rangeEnd); const range = sortedArray.slice(startIndex, endIndex + 1); console.log(range);
🎉 Conclusion
The _.sortedIndexOf()
method in Lodash is a valuable addition to your toolkit when dealing with sorted arrays. Its efficiency in finding the index of a value in a sorted context makes it a powerful tool for tasks requiring fast and precise searching.
Explore the capabilities of _.sortedIndexOf()
and leverage its benefits when working with ordered datasets. Enhance the performance of your array operations with Lodash!
👨💻 Join our Community:
Author
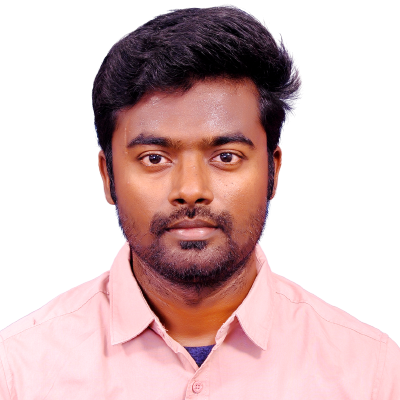
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
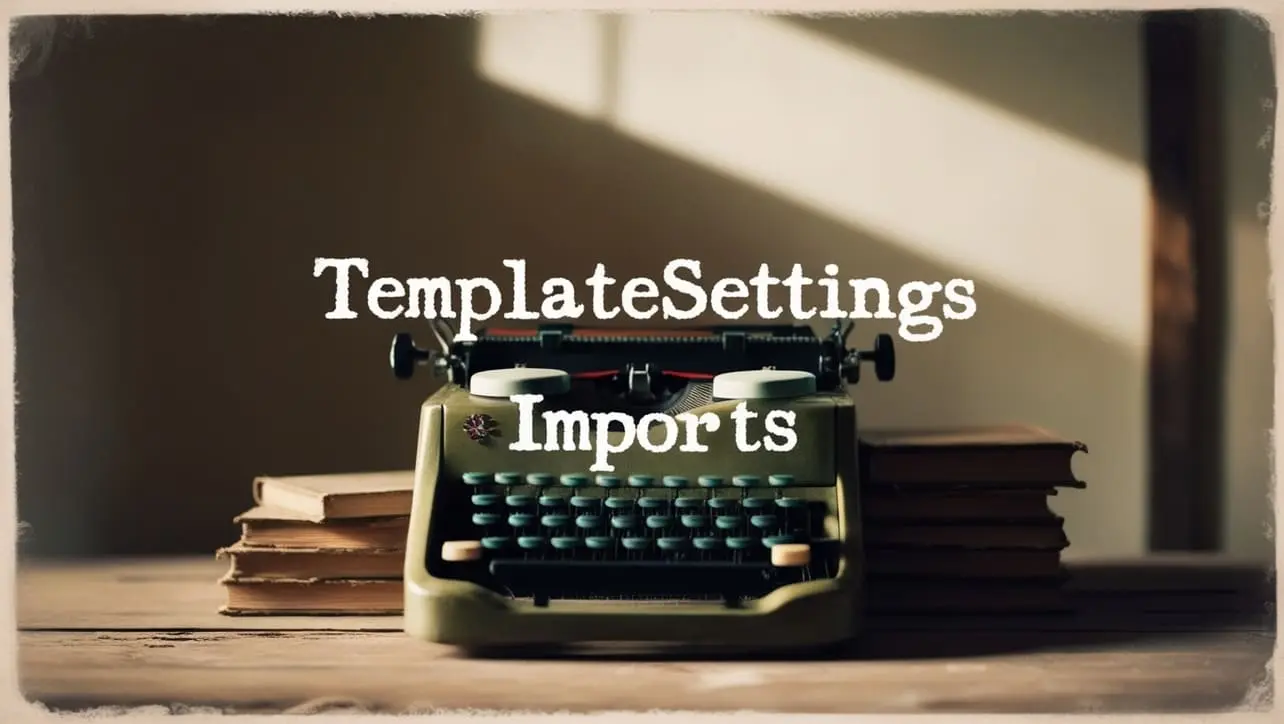
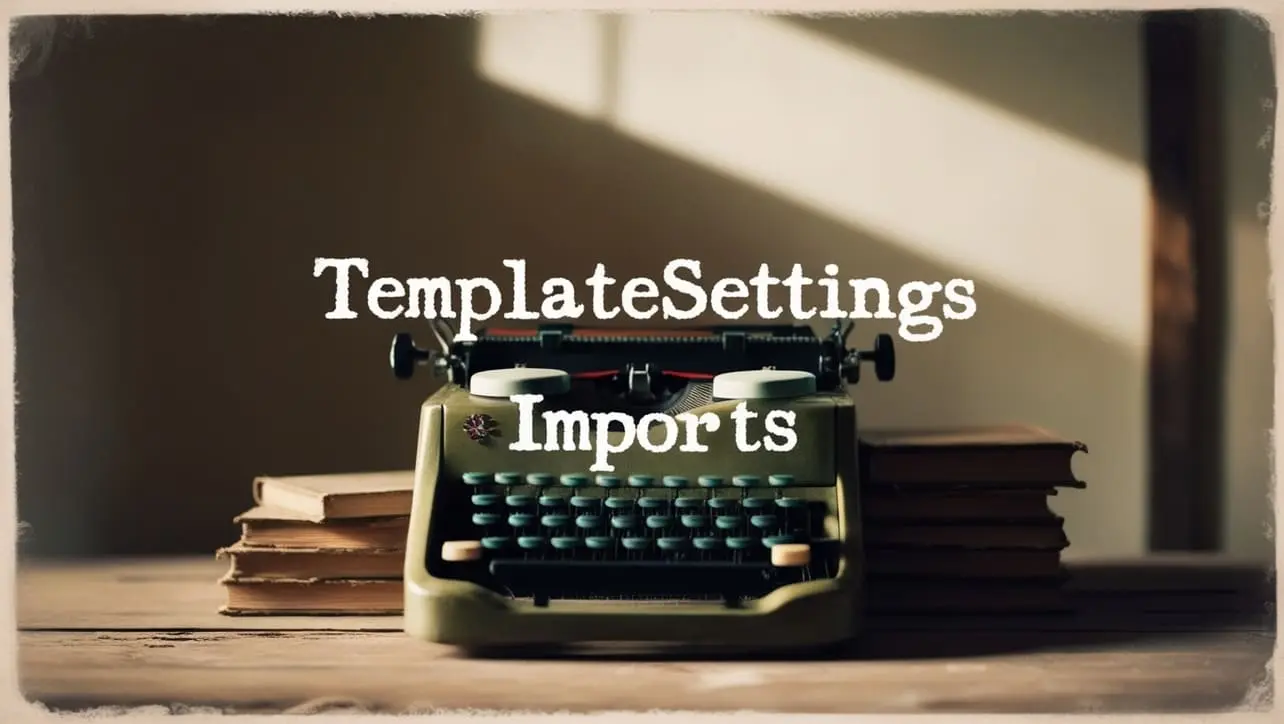
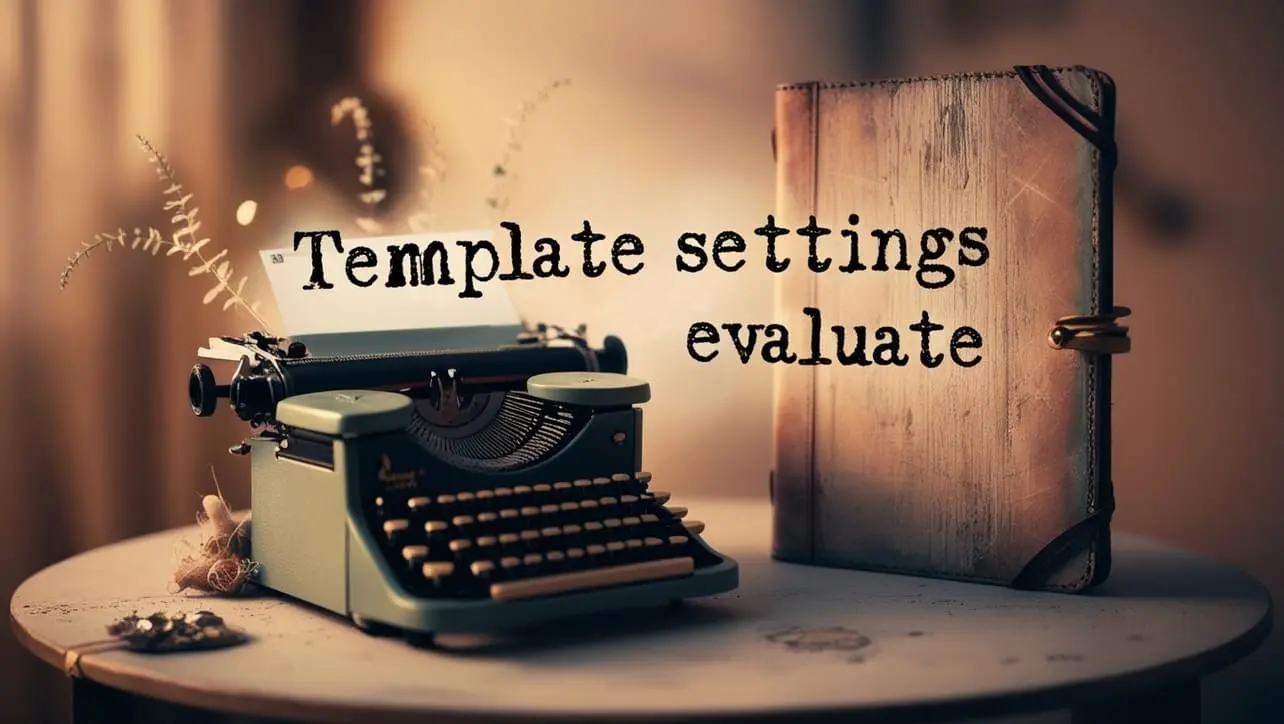
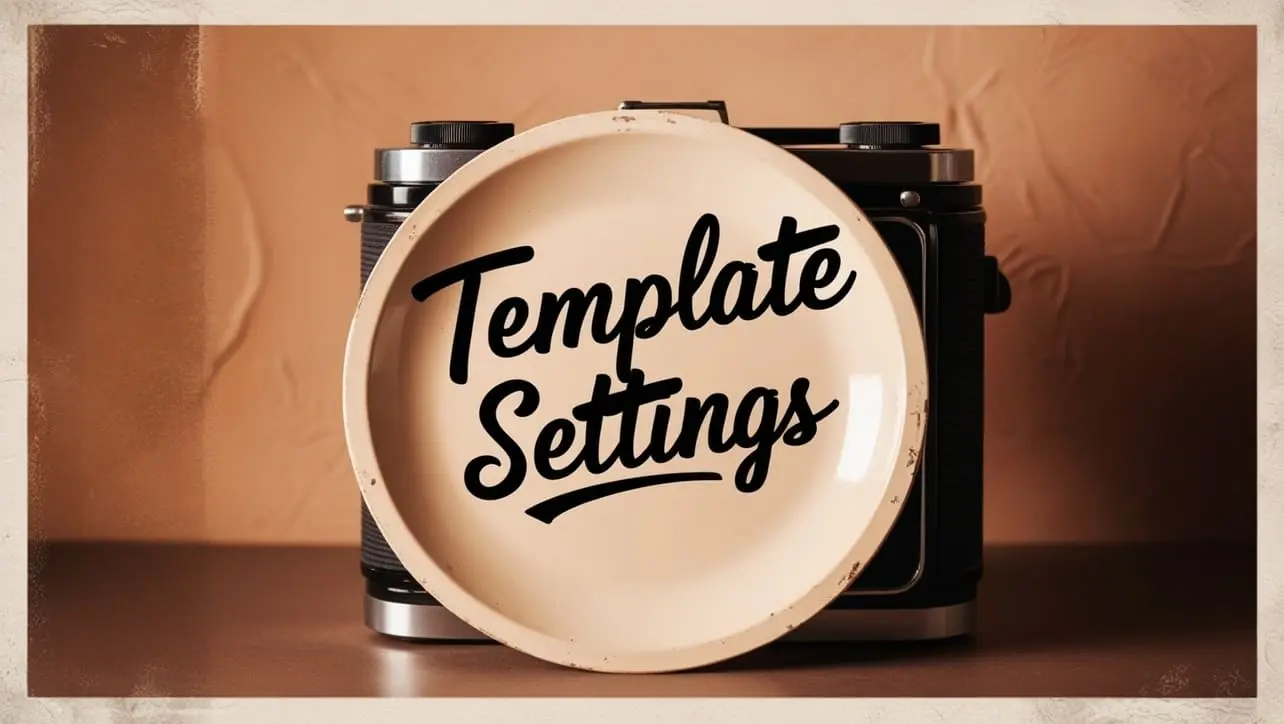
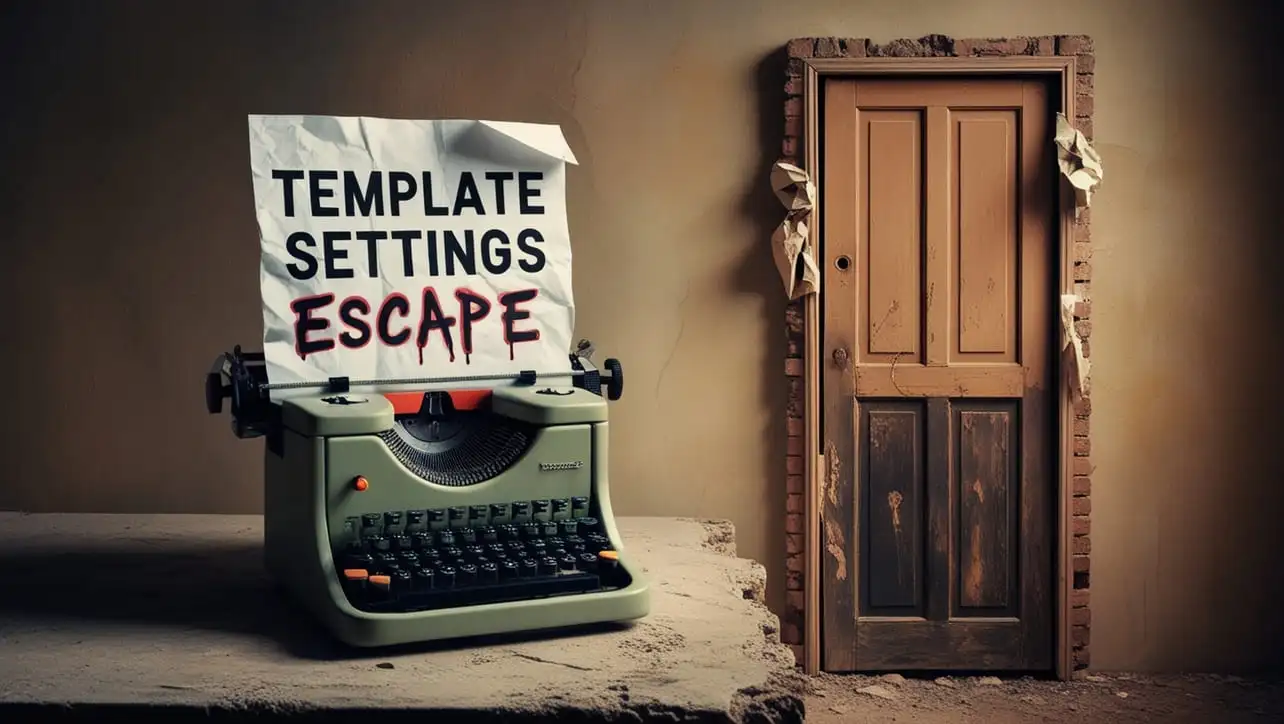
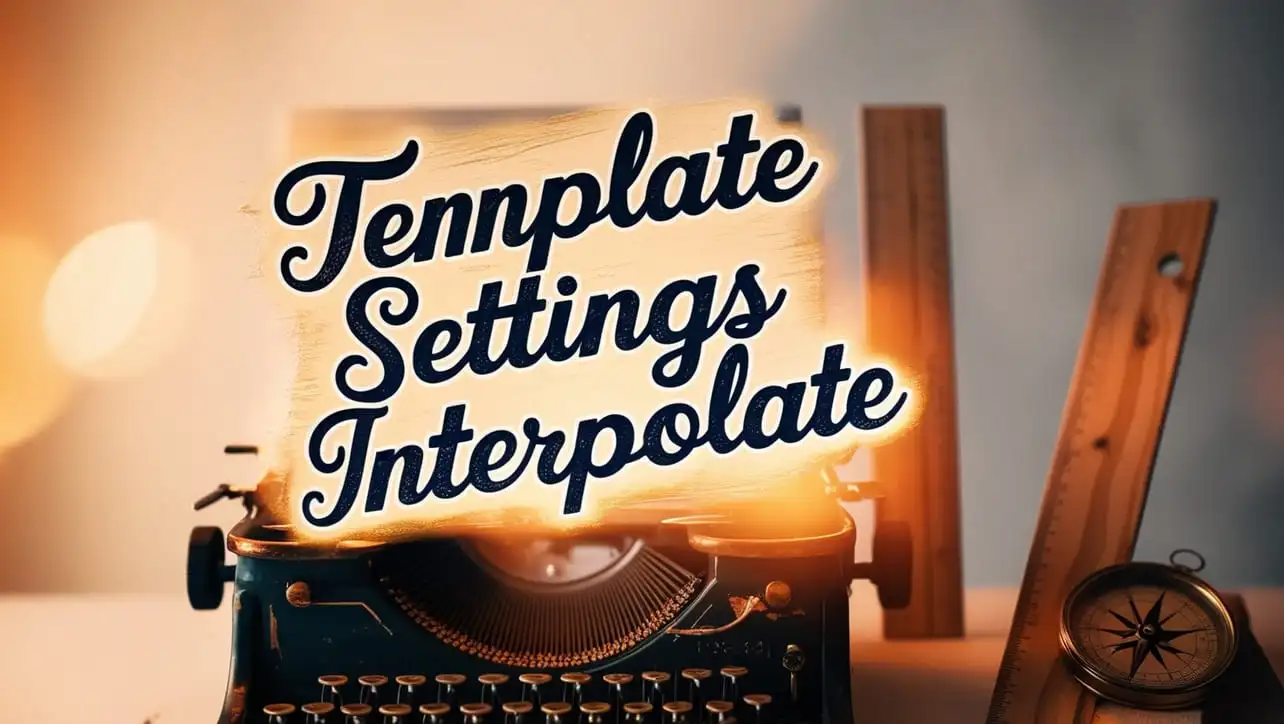
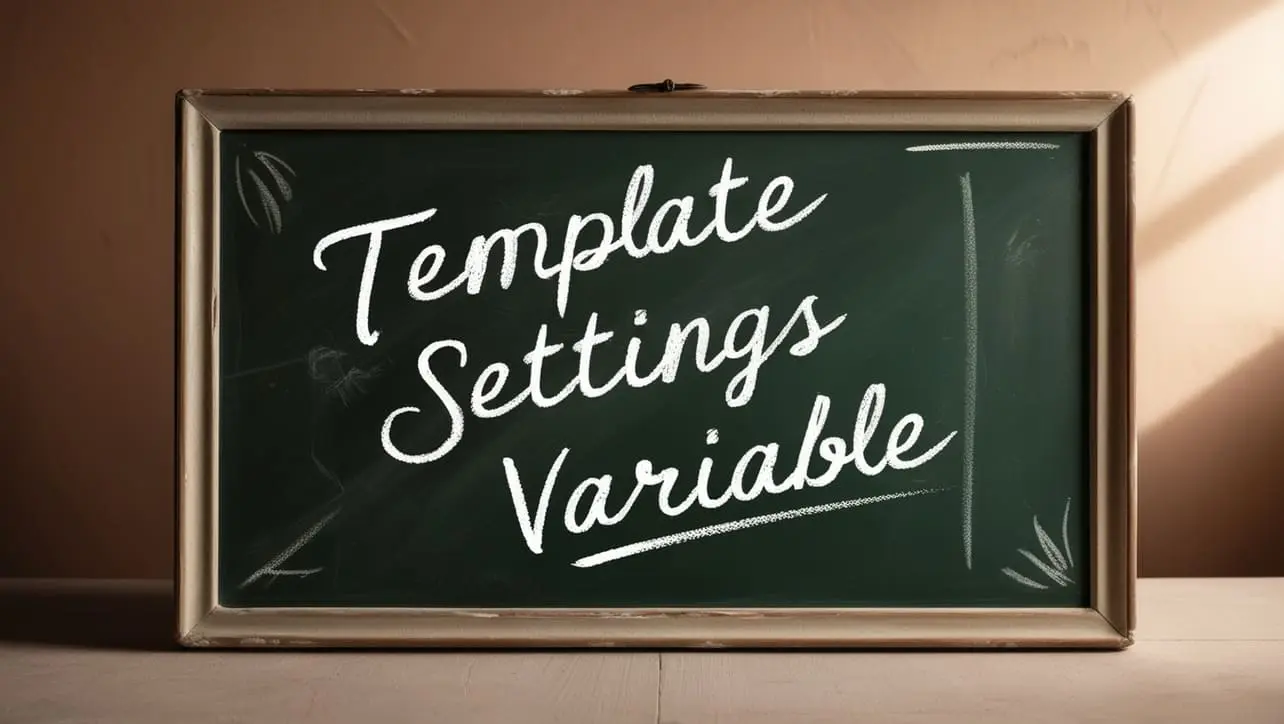
If you have any doubts regarding this article (Lodash _.sortedIndexOf() Array Method), please comment here. I will help you immediately.