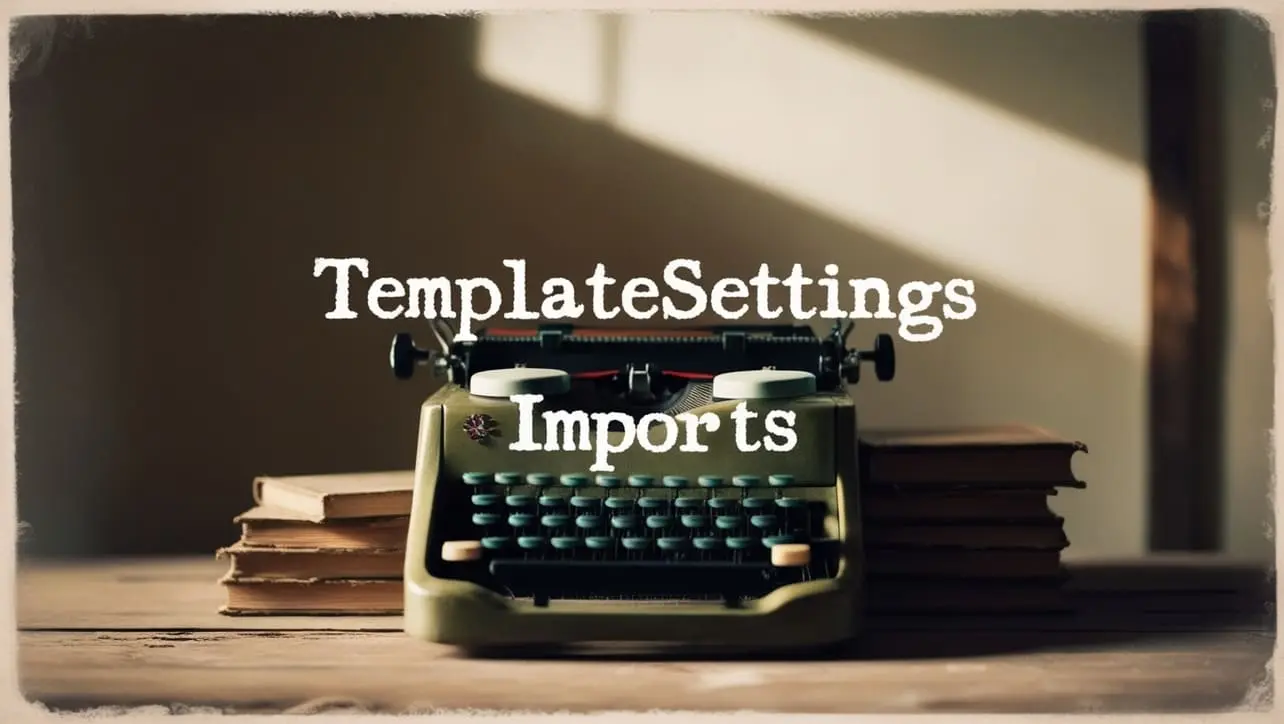
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.slice() Array Method
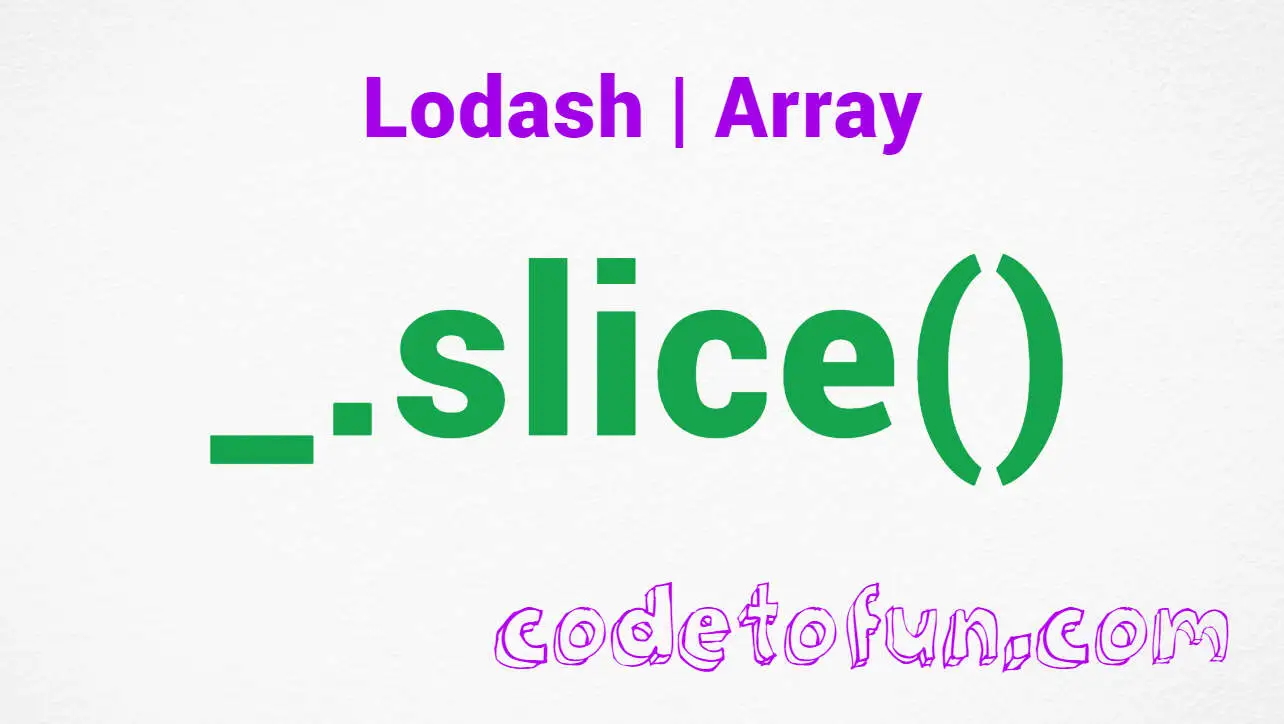
Photo Credit to CodeToFun
🙋 Introduction
Efficiently extracting portions of an array is a common task in JavaScript development. Lodash, a powerful utility library, offers a solution with its _.slice()
method.
This method allows developers to create a new array containing elements from the original array, providing flexibility and ease of use in array manipulation.
🧠 Understanding _.slice()
The _.slice()
method in Lodash is designed to extract a portion of an array, creating a new array with the selected elements. This method is particularly useful when you need to work with a subset of data or create shallow copies for various purposes.
💡 Syntax
_.slice(array, [start=0], [end=array.length])
- array: The array to slice.
- start: The start index (default is 0).
- end: The end index (default is the length of the array).
📝 Example
Let's explore a practical example to illustrate the usage of _.slice()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const slicedArray = _.slice(originalArray, 2, 6);
console.log(slicedArray);
// Output: [3, 4, 5, 6]
In this example, _.slice()
is used to extract elements from index 2 to 5 (end index is exclusive), creating a new array with the specified range.
🏆 Best Practices
Validate Inputs:
Before using
_.slice()
, ensure that the input array is valid. Validate start and end indices to prevent unexpected behavior.example.jsCopiedif (!Array.isArray(originalArray) || originalArray.length === 0) { console.error('Invalid input array'); return; } const startIndex = 2; const endIndex = 6; if (startIndex < 0 || endIndex > originalArray.length || startIndex >= endIndex) { console.error('Invalid start or end indices'); return; } const validatedSlicedArray = _.slice(originalArray, startIndex, endIndex); console.log(validatedSlicedArray);
Handle Edge Cases:
Consider edge cases, such as negative indices or an end index greater than the array length. Implement error handling or default behaviors accordingly.
example.jsCopiedconst negativeStartIndex = -2; const endBeyondArrayLength = 15; const edgeCaseSlicedArray = _.slice(originalArray, negativeStartIndex, endBeyondArrayLength); console.log(edgeCaseSlicedArray);
Shallow Copy:
Be aware that
_.slice()
creates a shallow copy of the selected elements. If dealing with nested arrays or objects, additional steps may be needed for a deep copy.example.jsCopiedconst nestedArray = [[1, 2], [3, 4]]; const shallowCopy = _.slice(nestedArray); console.log(shallowCopy); // Output: [[1, 2], [3, 4]] // Modifying the shallow copy affects the original array shallowCopy[0][0] = 99; console.log(nestedArray); // Output: [[99, 2], [3, 4]]
📚 Use Cases
Extracting Subsets:
_.slice()
is perfect for extracting specific subsets of an array based on start and end indices.example.jsCopiedconst dataForAnalysis = /* ...fetch data from API or elsewhere... */; const relevantSubset = _.slice(dataForAnalysis, 10, 20); console.log(relevantSubset);
Pagination:
In pagination scenarios,
_.slice()
can help fetch and display a specific page of data.example.jsCopiedconst allData = /* ...fetch all data from API or elsewhere... */; const itemsPerPage = 5; const currentPage = 3; const paginatedData = _.slice(allData, (currentPage - 1) * itemsPerPage, currentPage * itemsPerPage); console.log(paginatedData);
Creating Snapshots:
When working with dynamic data, creating snapshots using
_.slice()
can be beneficial for tracking changes over time.example.jsCopiedconst dynamicData = /* ...fetch dynamic data from API or elsewhere... */; const snapshot1 = _.slice(dynamicData); // Perform operations on dynamicData... const snapshot2 = _.slice(dynamicData); console.log(snapshot1); console.log(snapshot2);
🎉 Conclusion
The _.slice()
method in Lodash provides a versatile way to extract elements from arrays, offering flexibility and control over the data you work with. Whether it's creating subsets, handling pagination, or creating snapshots, _.slice()
is a valuable addition to your array manipulation toolkit.
Explore the capabilities of Lodash and unlock the potential of array slicing with _.slice()
!
👨💻 Join our Community:
Author
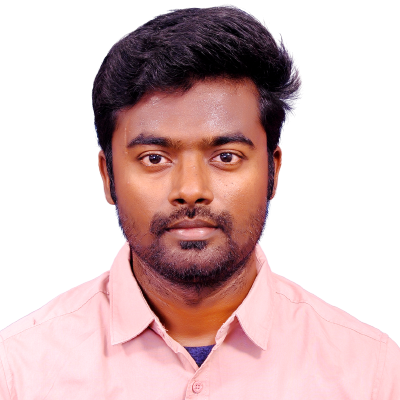
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
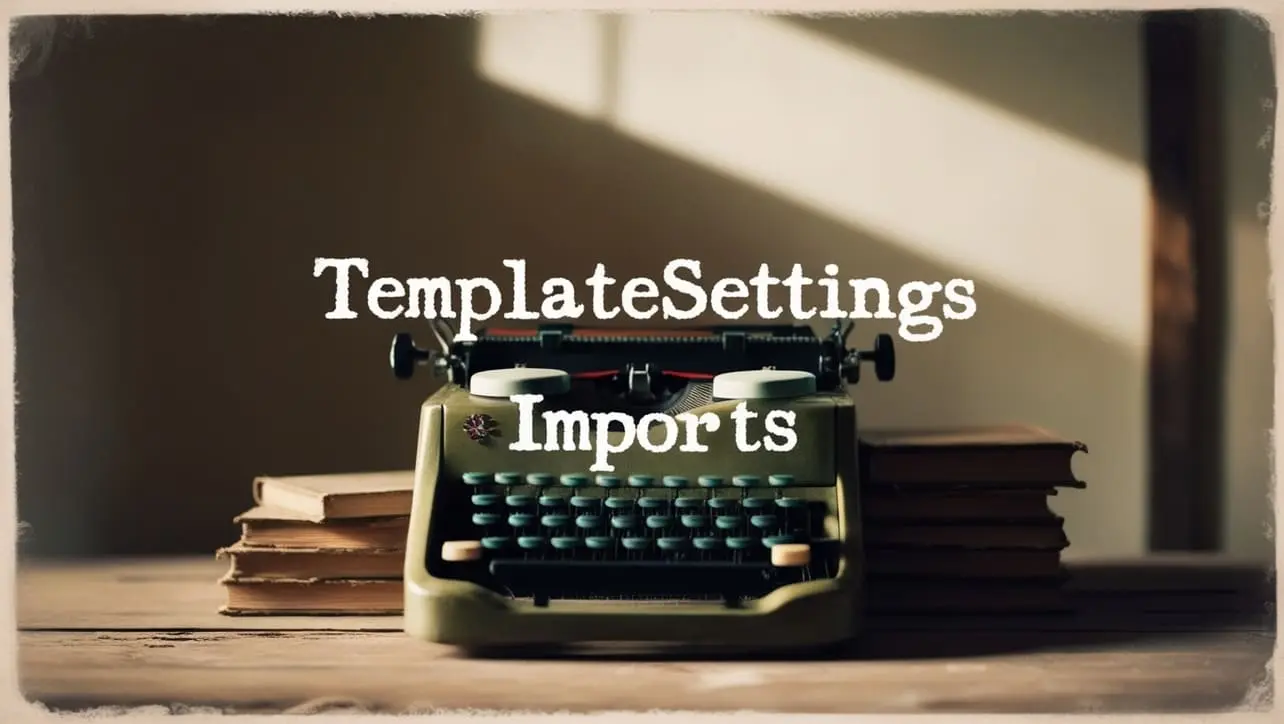
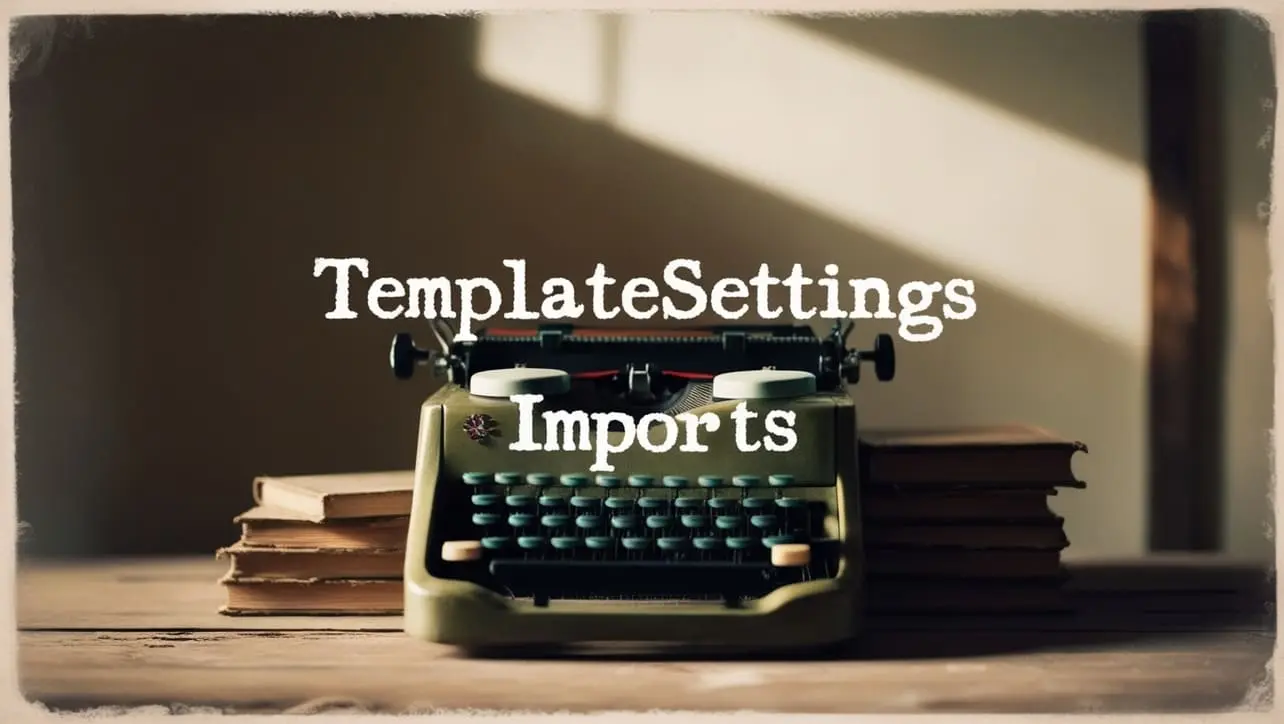
Lodash _.templateSettings.imports Property
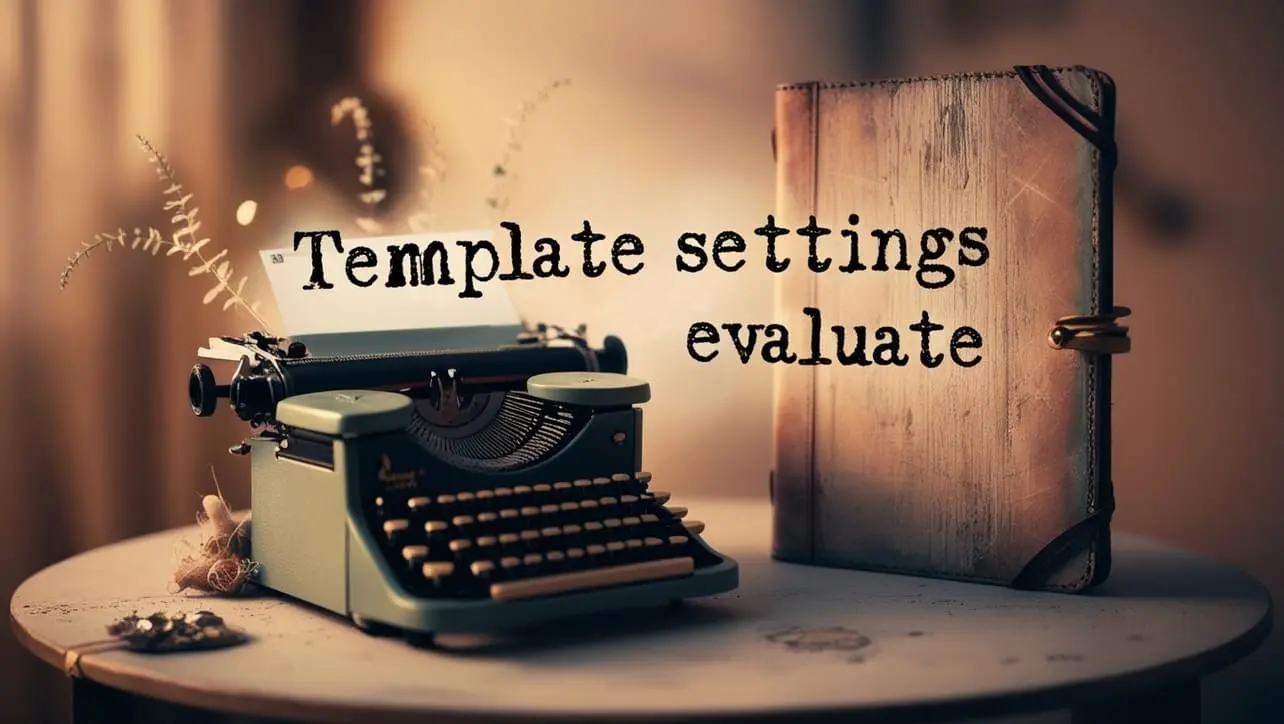
Lodash _.templateSettings.evaluate Property
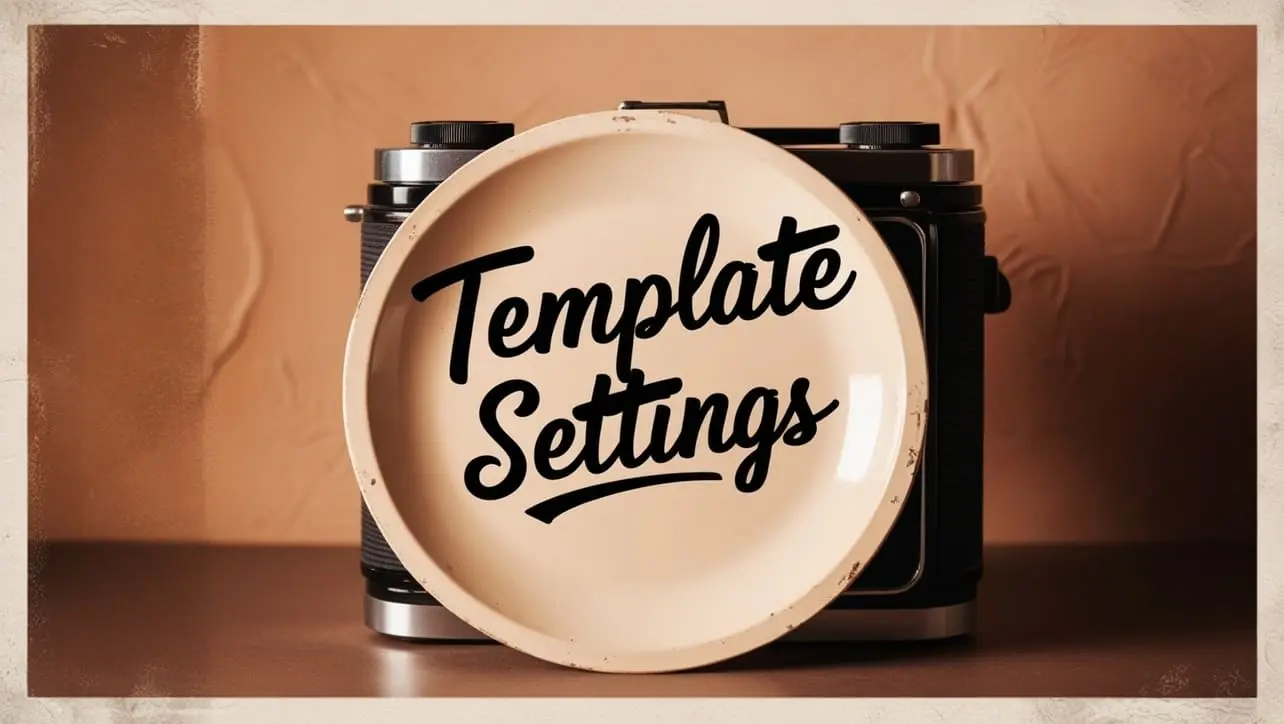
Lodash _.templateSettings Property
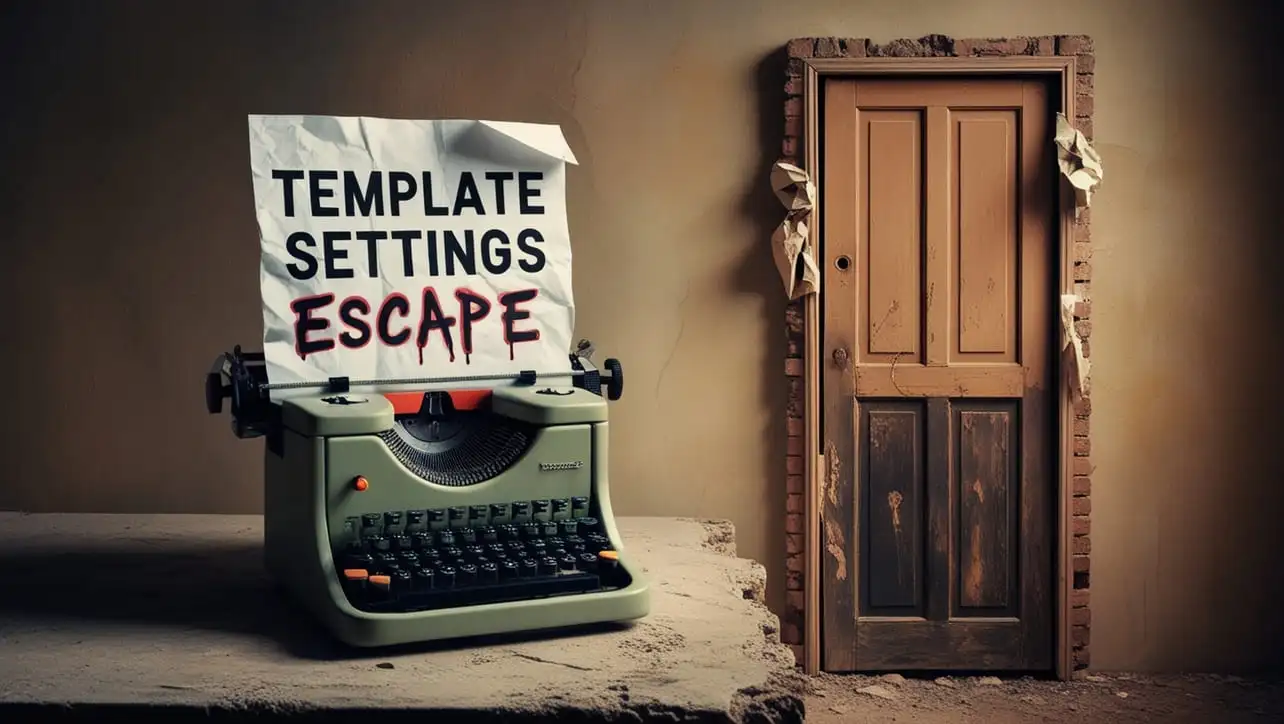
Lodash _.templateSettings.escape Property
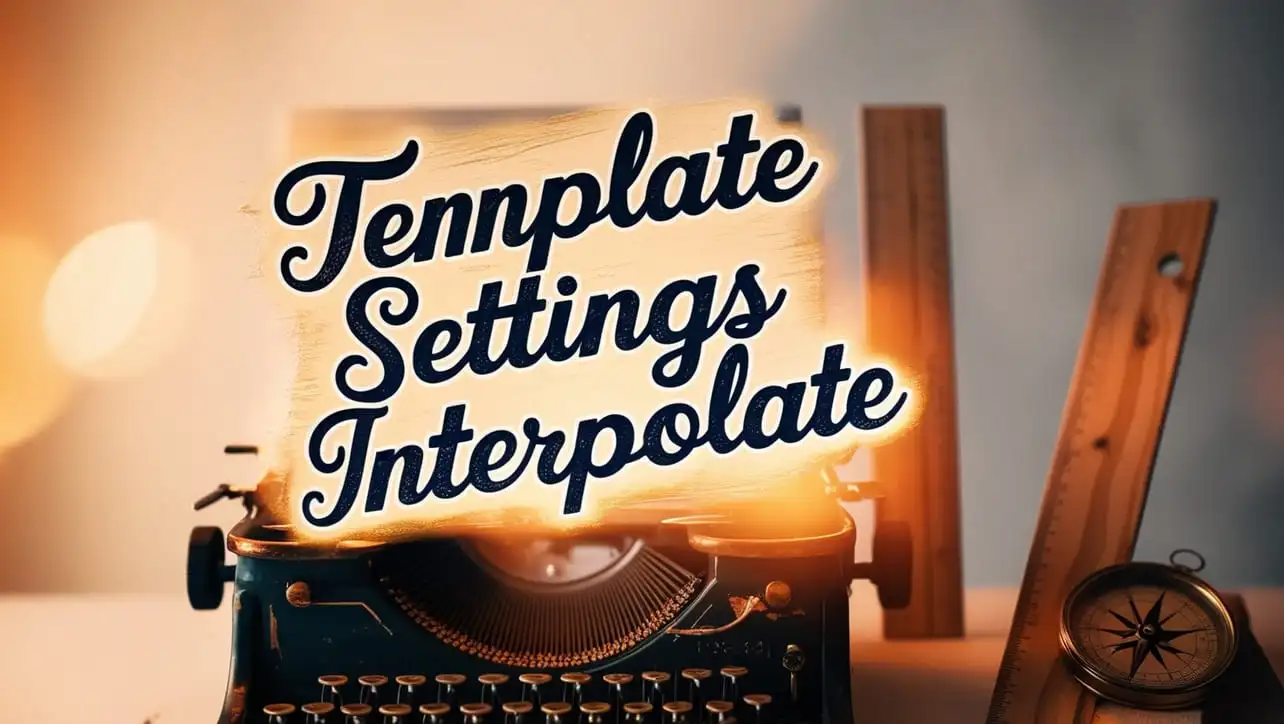
Lodash _.templateSettings.interpolate Property
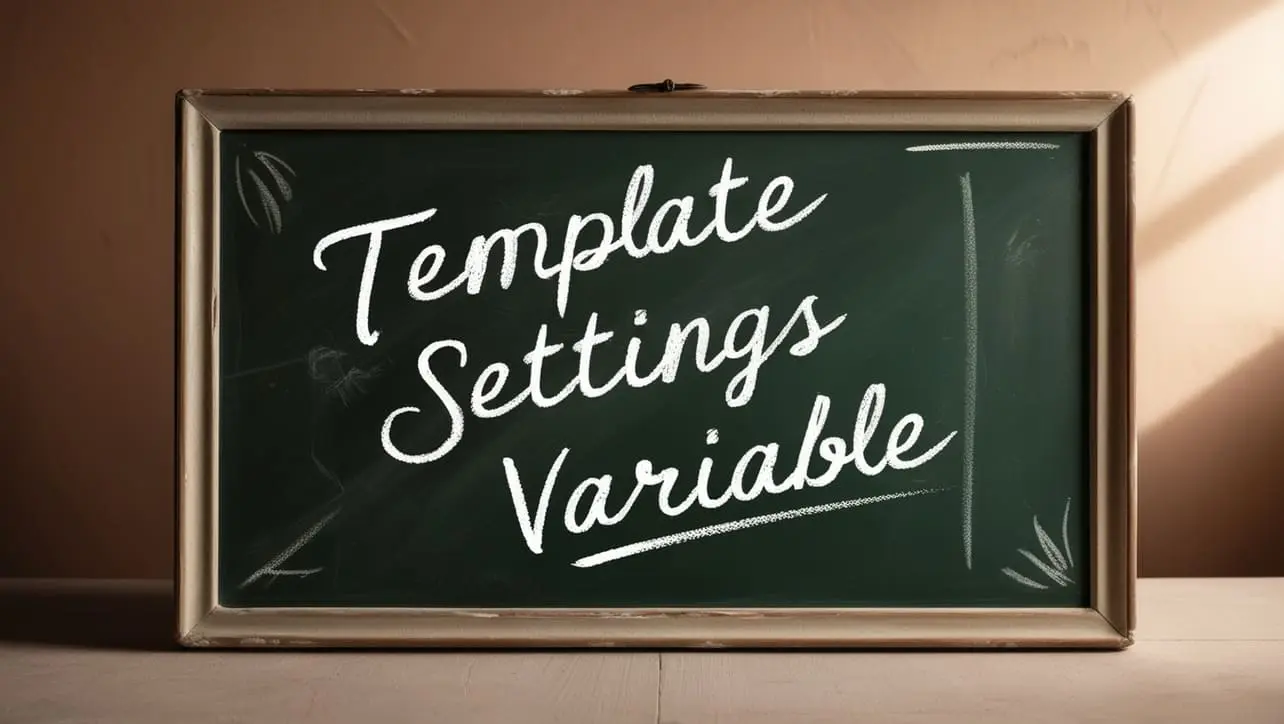
If you have any doubts regarding this article (Lodash _.slice() Array Method), please comment here. I will help you immediately.