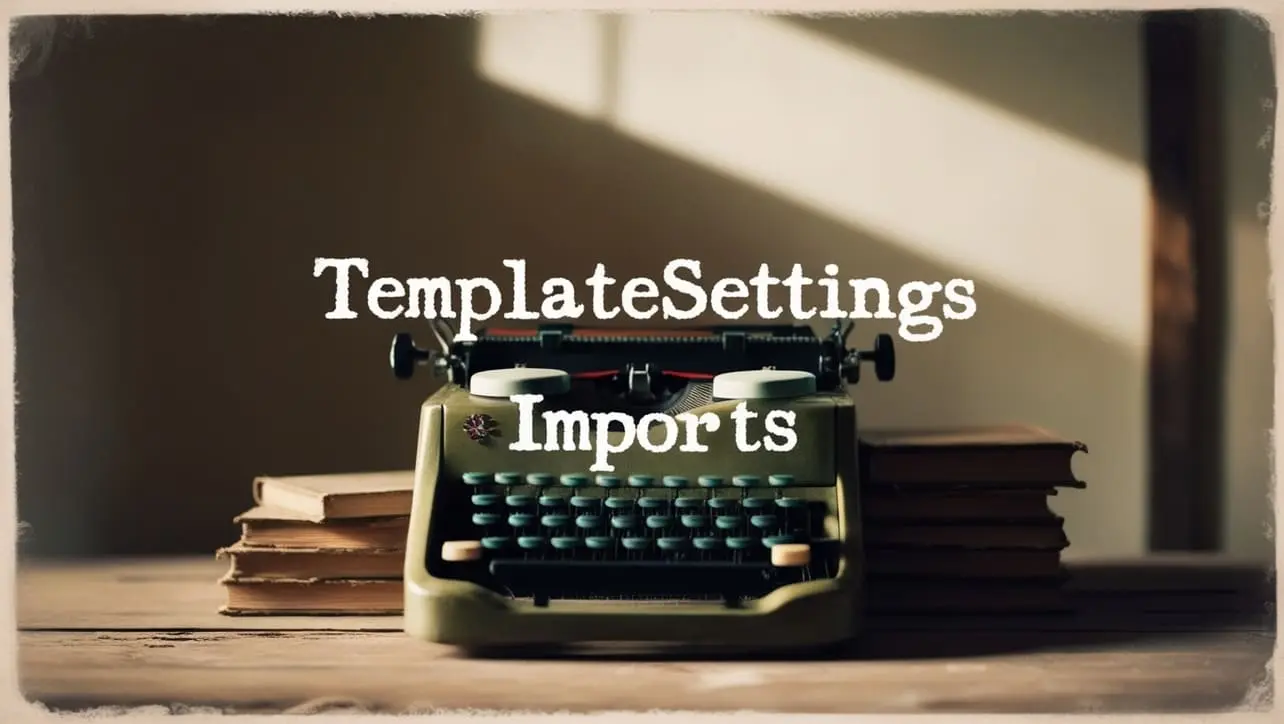
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.remove() Array Method
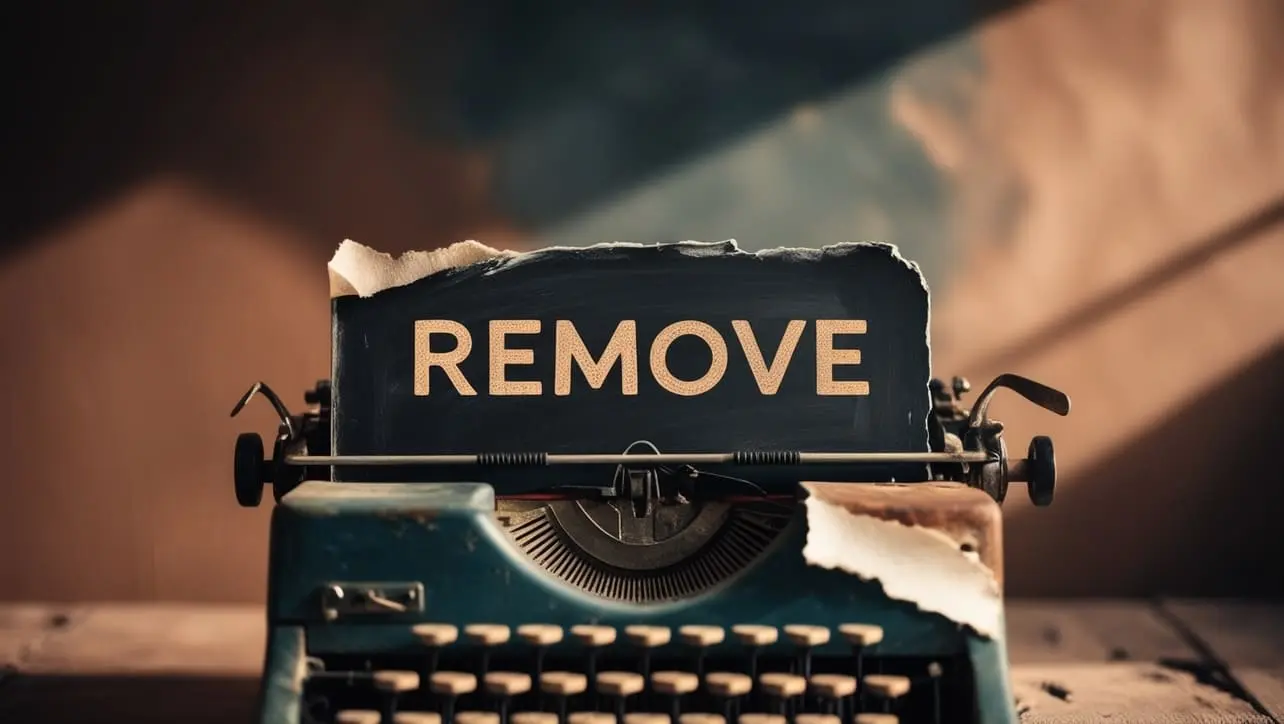
Photo Credit to CodeToFun
🙋 Introduction
Efficiently managing and modifying arrays is a common task in JavaScript programming.
Lodash, a powerful utility library, provides the _.remove()
method to simplify the process of removing elements from an array based on a specified condition.
This method proves to be a valuable asset for developers looking to clean, filter, or transform arrays with ease.
🧠 Understanding _.remove()
The _.remove()
method in Lodash is designed to remove elements from an array that satisfy a given predicate function. This flexible and intuitive approach allows developers to tailor the removal logic to their specific needs, making array manipulation a breeze.
💡 Syntax
_.remove(array, [predicate])
- array: The array to modify.
- predicate: The function invoked per iteration to determine removal.
📝 Example
Let's explore a practical example to illustrate the power of _.remove()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const condition = (num) => num % 2 === 0;
_.remove(originalArray, condition);
console.log(originalArray);
// Output: [1, 3, 5, 7, 9]
In this example, the originalArray is modified to remove all even numbers based on the provided condition.
🏆 Best Practices
Ensure Valid Inputs:
Before utilizing
_.remove()
, ensure that the input array is valid and contains elements. Additionally, validate the predicate function to avoid unexpected behavior.example.jsCopiedif (!Array.isArray(originalArray) || originalArray.length === 0) { console.error('Invalid input array'); return; } const validCondition = (num) => typeof num === 'number'; _.remove(originalArray, validCondition); console.log(originalArray);
Use Carefully with Large Arrays:
When working with large arrays, be mindful of the potential performance impact of using
_.remove()
in a loop. Consider alternatives or optimizations if dealing with substantial datasets.example.jsCopied// Example of using
_.remove()
in a loop (not recommended for large arrays) const largeArray = /* ...fetch data from API or elsewhere... */; const conditionToRemove = (item) => /* ...some condition... */; largeArray.forEach((item, index) => { _.remove(largeArray, conditionToRemove); }); console.log(largeArray);Make Predicate Functions Explicit:
For better code readability and maintainability, consider declaring predicate functions explicitly rather than inline.
example.jsCopiedconst isEven = (num) => num % 2 === 0; _.remove(originalArray, isEven); console.log(originalArray);
📚 Use Cases
Data Filtering:
_.remove()
is ideal for filtering arrays based on custom conditions, providing a clean and concise way to transform datasets.example.jsCopiedconst dataToFilter = /* ...fetch data from API or elsewhere... */; const conditionToFilter = (item) => /* ...some filtering condition... */; _.remove(dataToFilter, conditionToFilter); console.log(dataToFilter);
Cleanup Operations:
Performing cleanup operations on arrays, such as removing invalid or unwanted elements, is simplified with
_.remove()
.example.jsCopiedconst dataToClean = /* ...fetch data from API or elsewhere... */; const conditionToRemoveInvalid = (item) => /* ...some condition to identify invalid elements... */; _.remove(dataToClean, conditionToRemoveInvalid); console.log(dataToClean);
Dynamic Modifications:
When array modifications need to be dynamic and based on runtime conditions,
_.remove()
excels in providing a flexible solution.example.jsCopiedconst dynamicCondition = /* ...calculate condition dynamically... */; _.remove(originalArray, dynamicCondition); console.log(originalArray);
🎉 Conclusion
The _.remove()
method in Lodash empowers JavaScript developers with a versatile tool for modifying arrays. Whether you're cleaning up data, filtering elements, or performing dynamic modifications, _.remove()
offers an efficient and expressive solution. Integrate this method into your projects to enhance the clarity and effectiveness of your array manipulation tasks.
Explore the capabilities of Lodash and revolutionize your array manipulation with _.remove()
!
👨💻 Join our Community:
Author
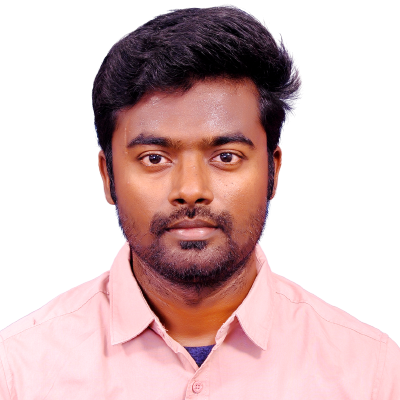
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
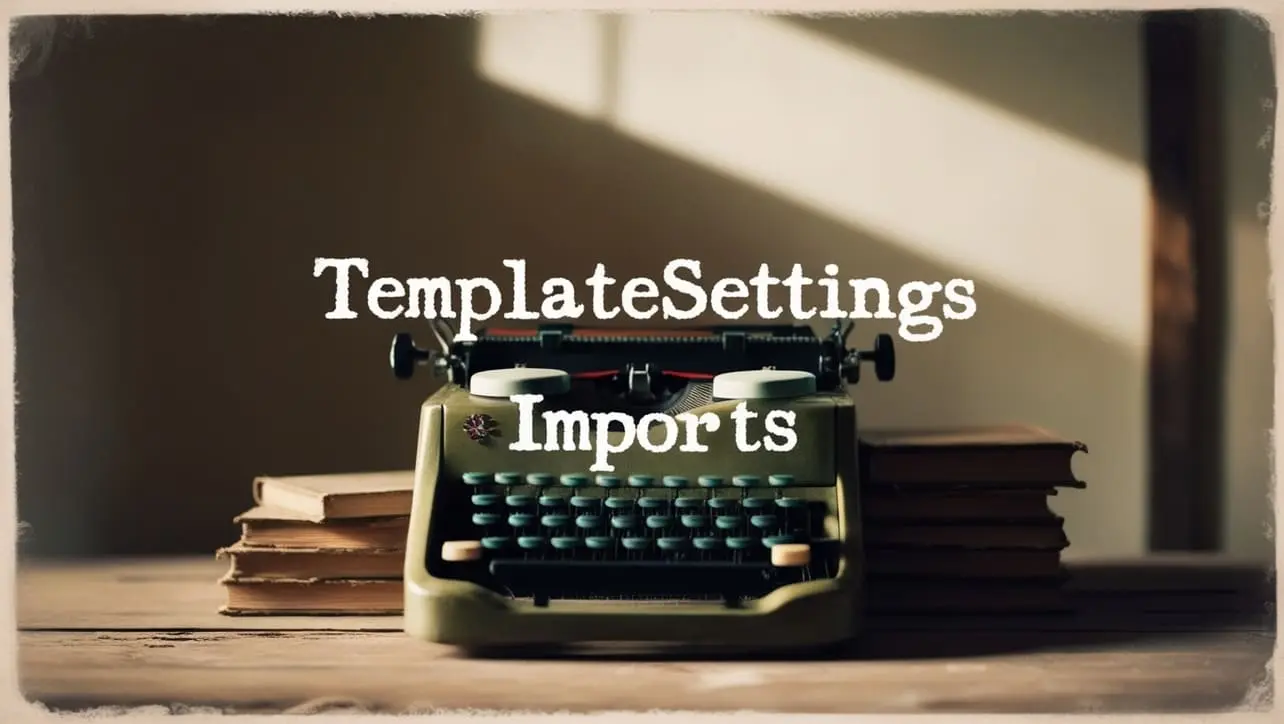
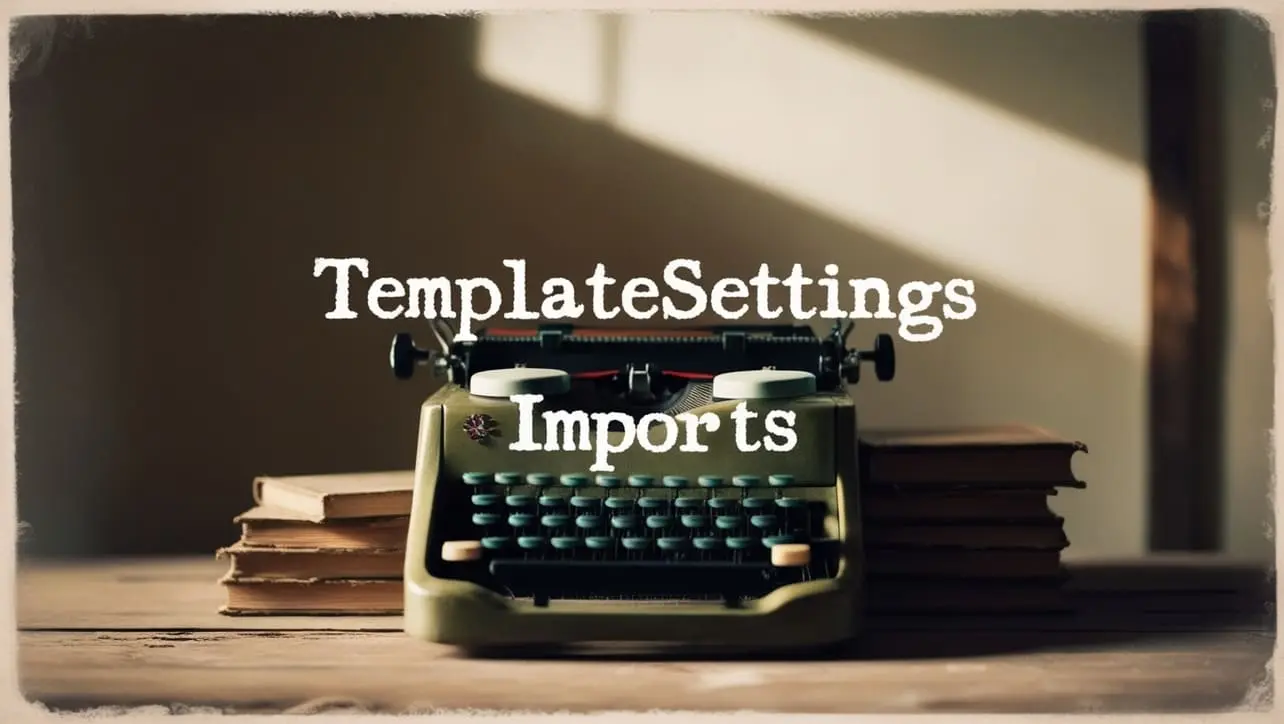
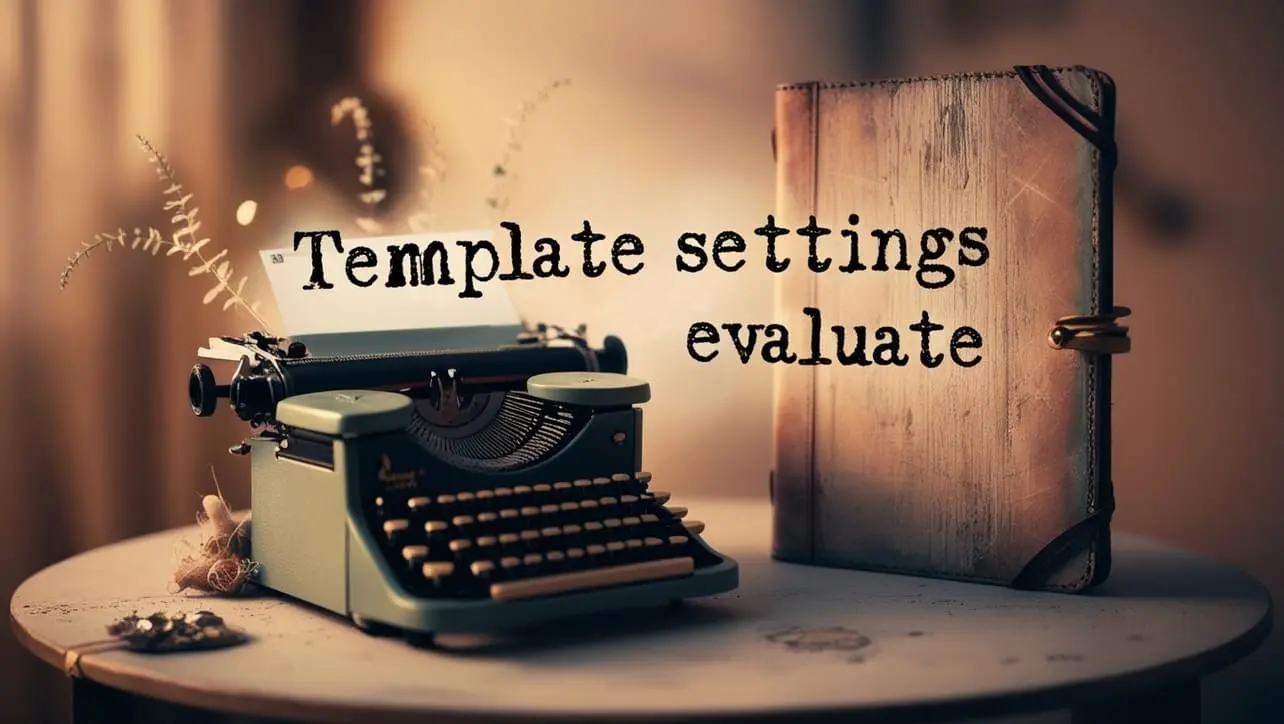
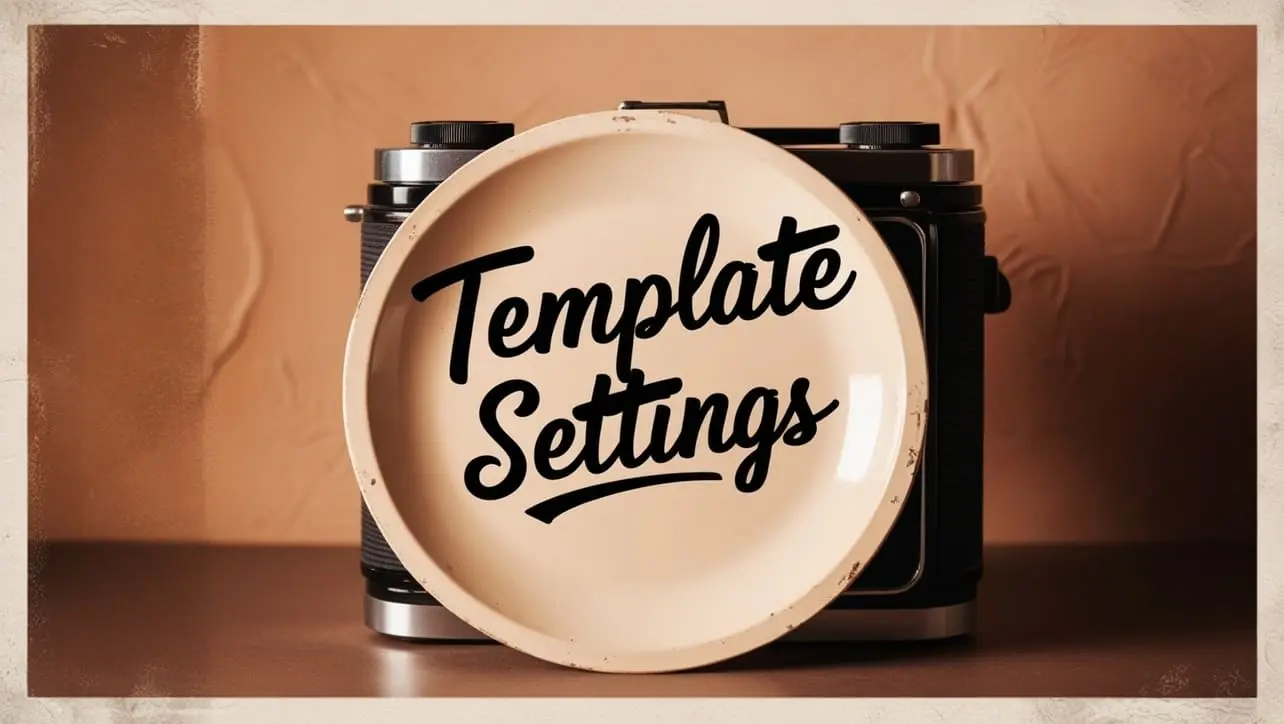
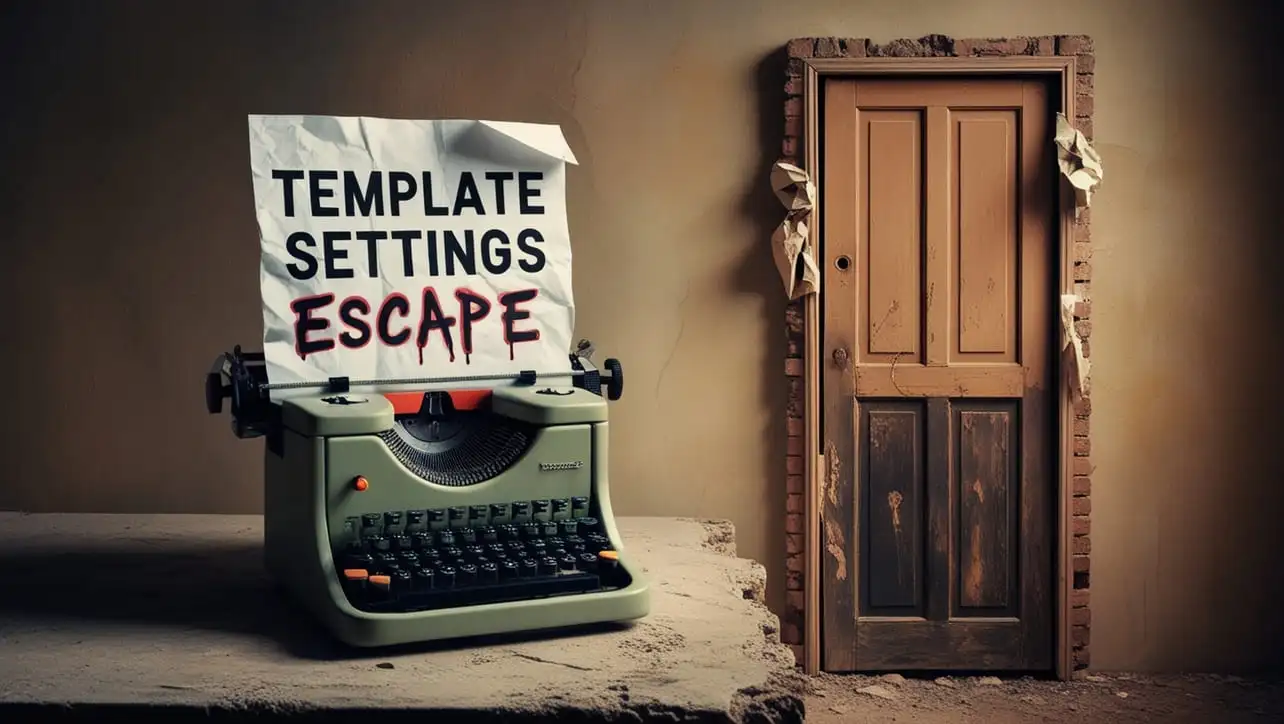
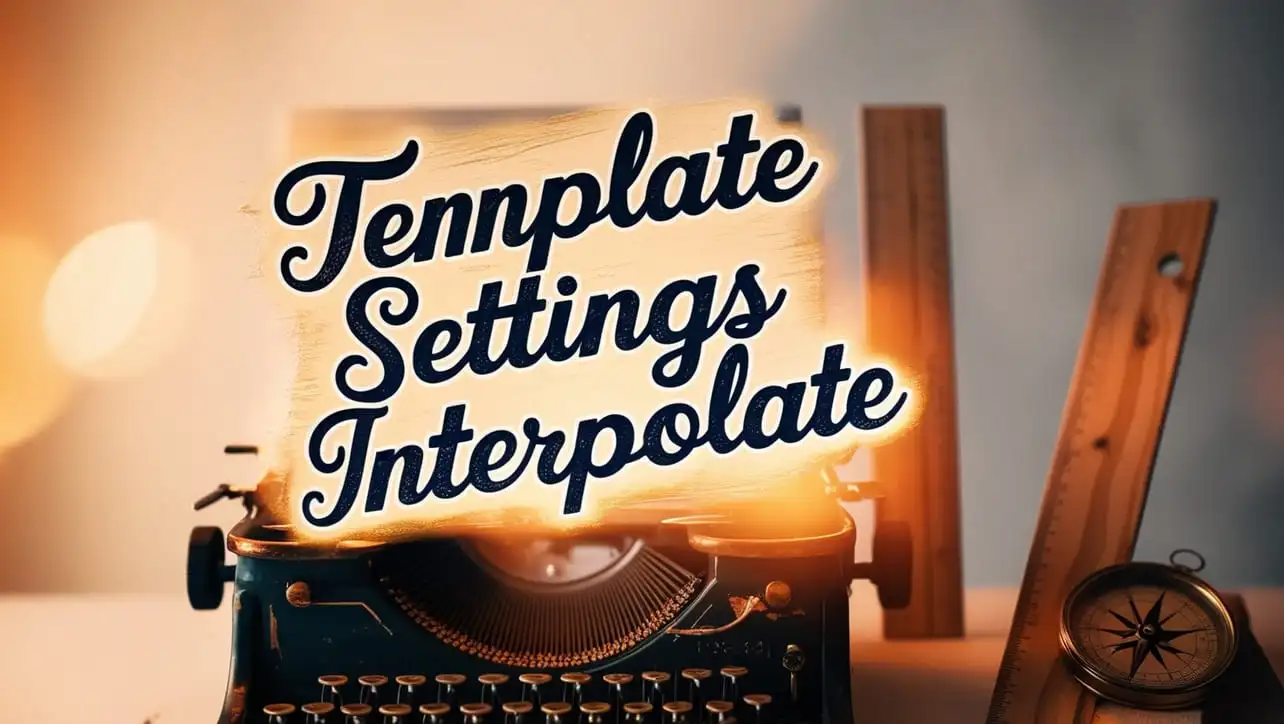
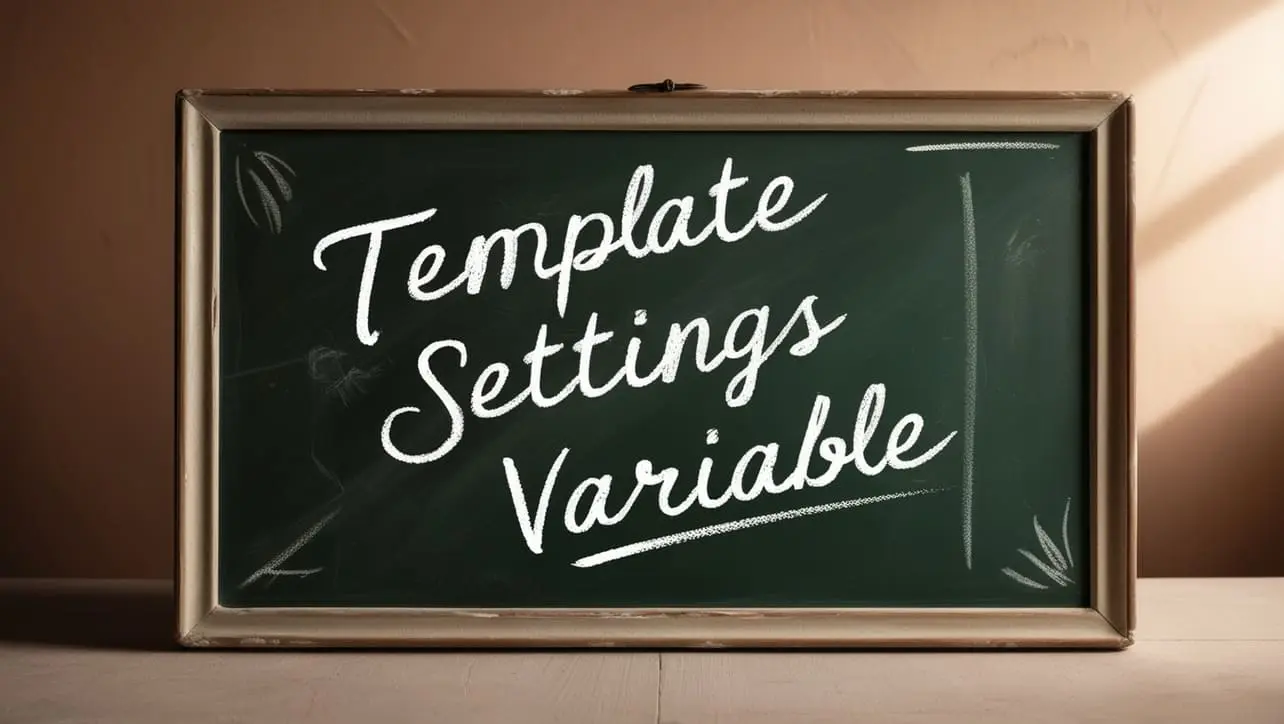
If you have any doubts regarding this article (Lodash _.remove() Array Method), please comment here. I will help you immediately.