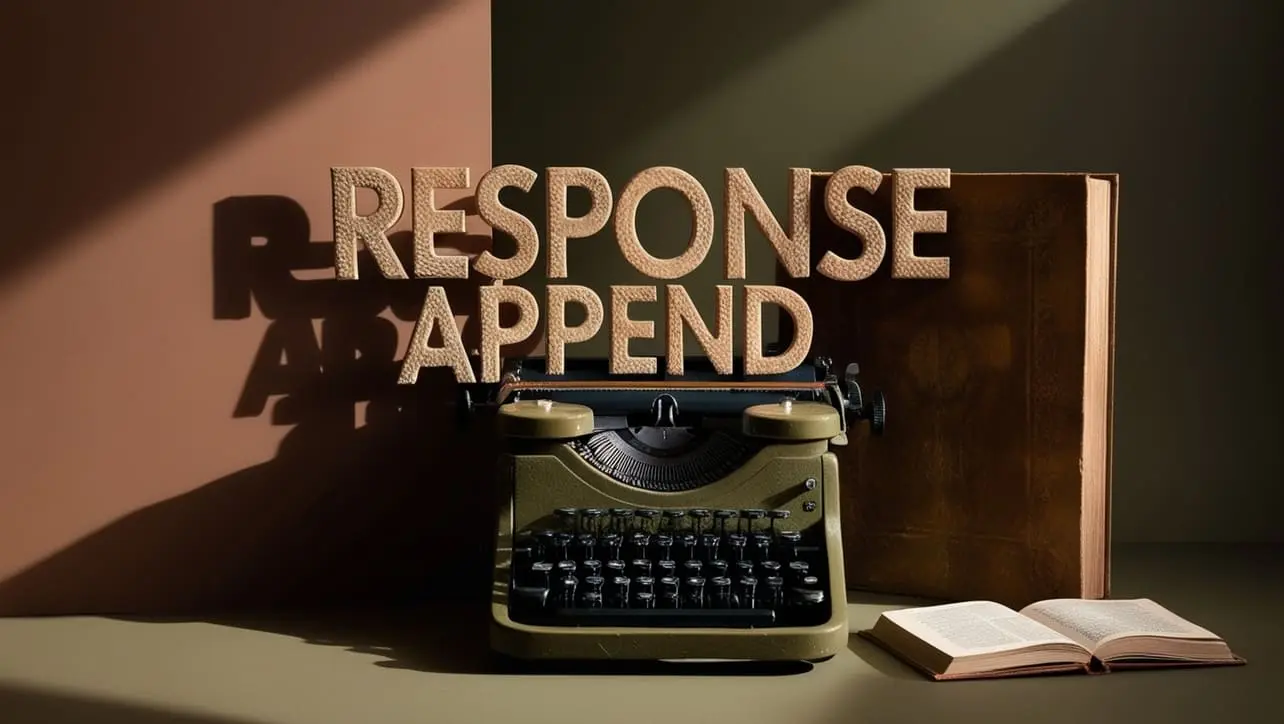
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.cookies Property
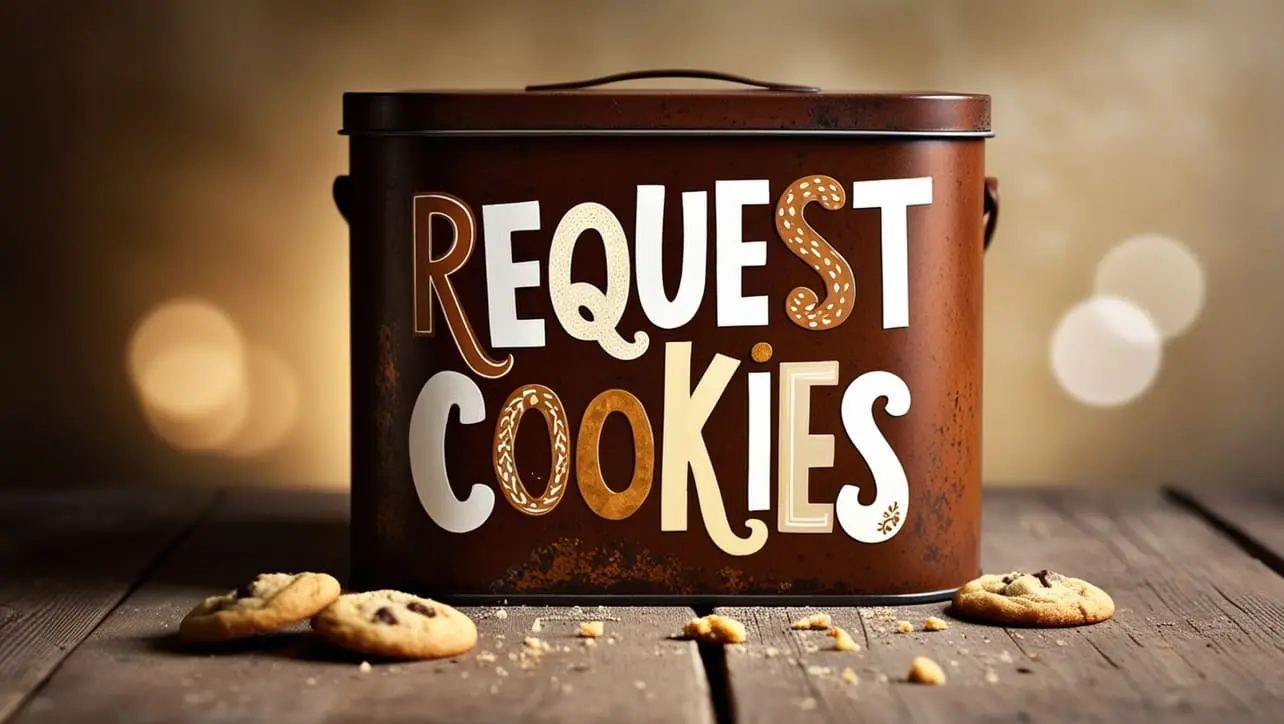
Photo Credit to CodeToFun
🙋 Introduction
Cookies are a fundamental aspect of web development, enabling developers to store small pieces of information on the client-side. In the context of Express.js, handling cookies is made easy through the req.cookies
property.
This guide will walk you through the syntax, usage, and best practices for utilizing req.cookies
to manage cookies effectively in your Express.js applications.
💡 Syntax
Accessing cookies using req.cookies
in Express.js is straightforward:
const cookieValue = req.cookies.cookieName;
- cookieName: The name of the cookie you want to retrieve.
❓ How req.cookies Works
req.cookies
in Express.js provides a convenient way to access cookies sent by the client in the HTTP request. It automatically parses the cookie header and provides a simple interface to read the values associated with each cookie.
app.get('/read-cookie', (req, res) => {
const username = req.cookies.username;
res.send(`Hello, ${username}!`);
});
In this example, the route handler uses req.cookies
to read the value of the "username" cookie and sends a personalized greeting.
📚 Use Cases
User Authentication:
Leverage
req.cookies
to retrieve user authentication information stored in a cookie.user-authentication.jsCopiedapp.get('/dashboard', (req, res) => { const userId = req.cookies.userId; // Validate user authentication based on userId // Render the user dashboard });
Remember User Preferences:
Use
req.cookies
to read user preferences stored in cookies and provide a personalized experience.user-preferences.jsCopiedapp.post('/update-preferences', (req, res) => { // Update user preferences in the database // Set a cookie to remember the user's preferences res.cookie('theme', req.body.theme, { maxAge: 86400000 }); // Cookie expires in 24 hours res.send('Preferences updated successfully!'); });
🏆 Best Practices
Secure Cookies:
When setting cookies, consider using the secure option to ensure that the cookie is only sent over HTTPS connections.
secure-cookies.jsCopiedres.cookie('sessionId', '123456', { secure: true });
Cookie Expiry:
Set an expiry time for cookies using the maxAge option to control how long the client should keep the cookie.
cookie-expiry.jsCopiedres.cookie('token', 'abcdef123456', { maxAge: 604800000 }); // Cookie expires in 7 days
🎉 Conclusion
The req.cookies
property in Express.js simplifies the process of working with cookies in your web applications. Whether it's for user authentication, storing preferences, or other use cases, understanding how to effectively use req.cookies
is crucial for building feature-rich and secure Express.js applications.
Now armed with knowledge about req.cookies
, go ahead and enhance your Express.js projects with effective cookie management!
👨💻 Join our Community:
Author
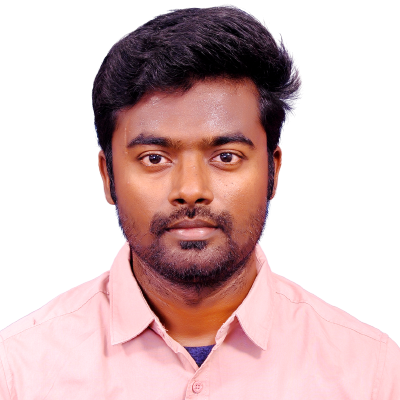
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.cookies Property), please comment here. I will help you immediately.