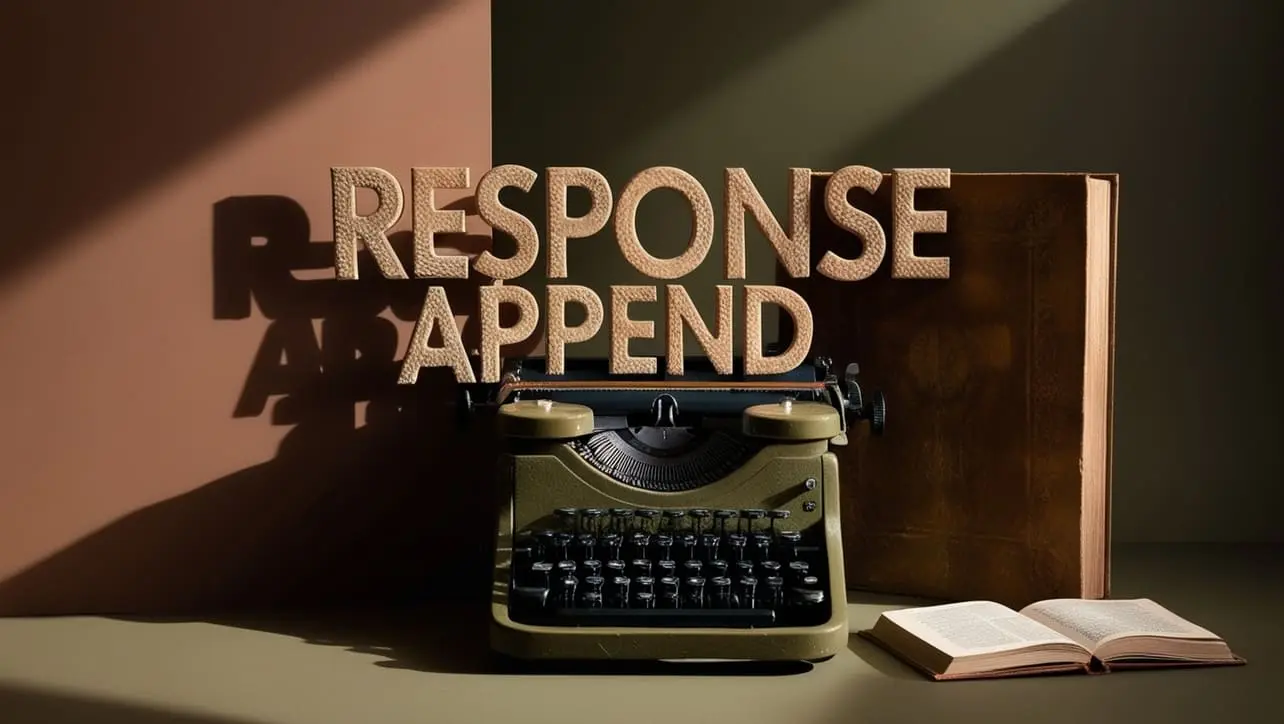
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.locals Property
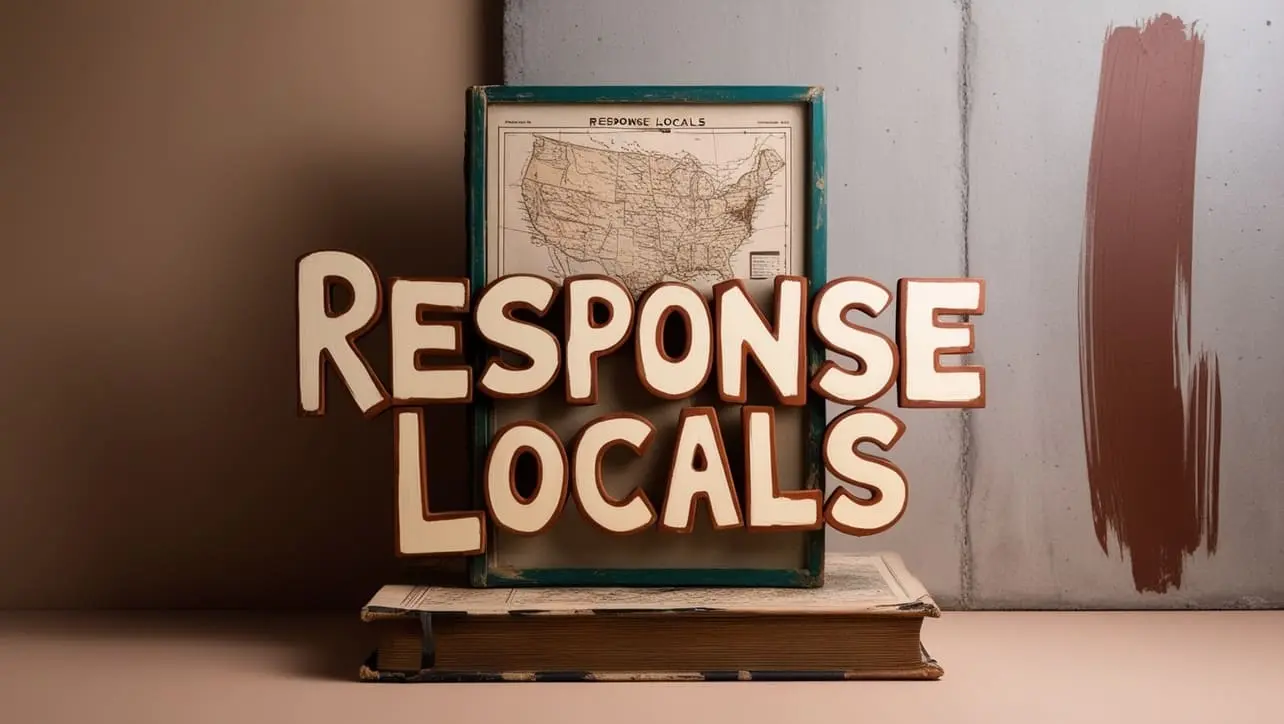
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a widely-used Node.js web application framework, provides developers with powerful features for building flexible and maintainable web applications. Among these features is the res.locals
property, allowing you to define response-scoped variables that are accessible within a specific request-response cycle.
In this guide, we'll explore the syntax, usage, and best practices of res.locals
to enhance your Express.js applications.
💡 Syntax
The syntax for using res.locals
is straightforward:
res.locals.name = value;
- name: The name of the variable you want to define.
- value: The value assigned to the variable.
❓ How res.locals Works
The res.locals
property in Express.js allows you to define variables that are specific to the current request-response cycle. These variables are available to middleware functions and route handlers within the same cycle, providing a convenient way to pass data between different parts of your application during the processing of a single request.
// Middleware setting a response-local variable
app.use((req, res, next) => {
res.locals.currentUser = req.user;
next();
});
// Route handler accessing the response-local variable
app.get('/profile', (req, res) => {
res.send(`Welcome, ${res.locals.currentUser.username}!`);
});
In this example, the currentUser variable is set in middleware and accessed in a subsequent route handler within the same request-response cycle.
📚 Use Cases
User Information:
Store user information in
res.locals
during authentication middleware for easy access in subsequent route handlers.user-information.jsCopied// Middleware setting user information in res.locals app.use((req, res, next) => { if (req.isAuthenticated()) { res.locals.currentUser = req.user; } next(); }); // Route handler using the user information app.get('/profile', (req, res) => { res.send(`Welcome, ${res.locals.currentUser.username}!`); });
Template Data:
Set template data in
res.locals
to dynamically provide information to your views.template-data.jsCopied// Middleware setting template data app.use((req, res, next) => { res.locals.siteTitle = 'My Express Site'; next(); }); // Route handler using the template data app.get('/', (req, res) => { res.render('index', { title: res.locals.siteTitle }); });
🏆 Best Practices
Limited Scope:
Use
res.locals
for data that is specific to the current request-response cycle. Avoid storing data that needs to persist across multiple requests.limited-scope.jsCopied// Good practice: Using res.locals for response-specific data app.use((req, res, next) => { res.locals.message = 'Hello from res.locals!'; next(); });
Avoid Global Variables:
Resist the temptation to use
res.locals
as a replacement for global variables. Keep the scope limited to the current response.avoid-global-variables.jsCopied// Bad practice: Using res.locals as a global variable res.locals.globalData = 'This is accessible in all routes';
🎉 Conclusion
The res.locals
property in Express.js is a powerful tool for managing response-scoped variables, allowing you to pass data seamlessly between middleware functions and route handlers within the same request-response cycle. By understanding its usage and following best practices, you can enhance the flexibility and maintainability of your Express.js applications.
Now equipped with knowledge about res.locals
, go ahead and optimize your Express.js projects with efficient handling of response-specific variables!
👨💻 Join our Community:
Author
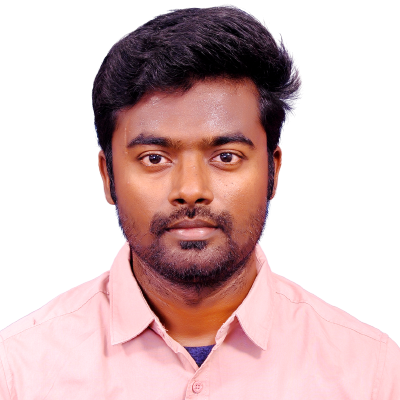
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.locals Property), please comment here. I will help you immediately.