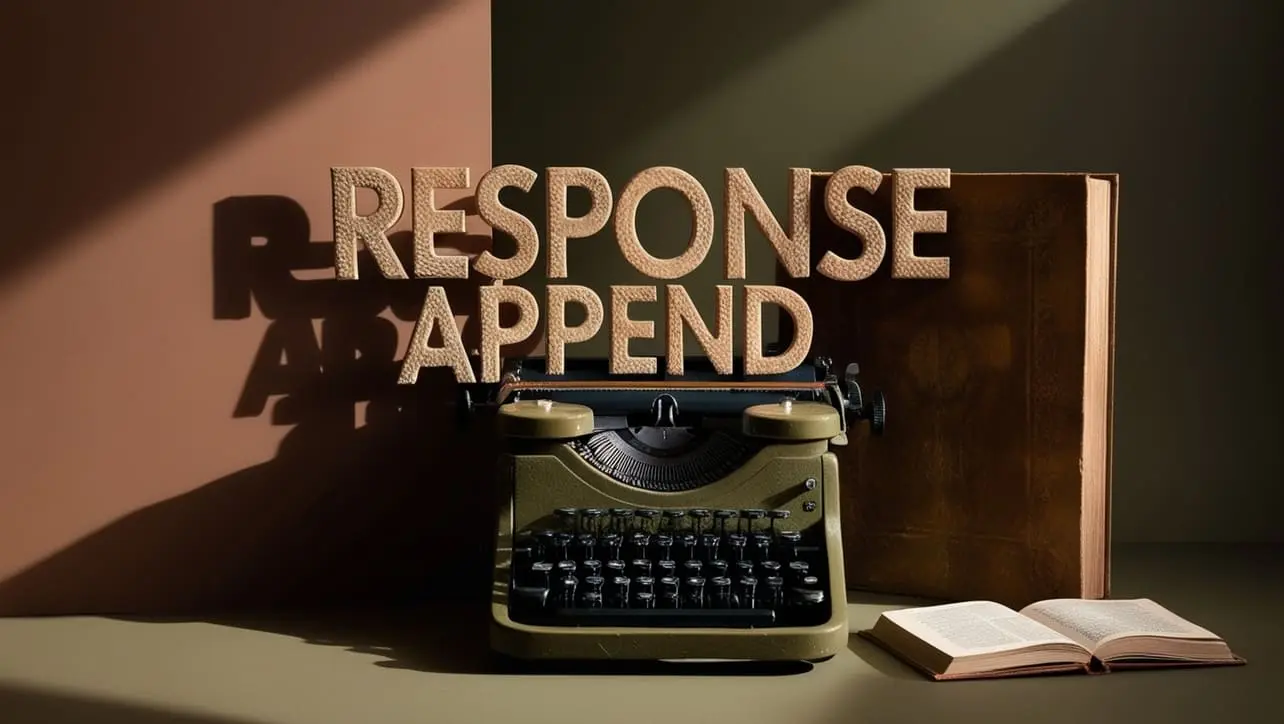
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.redirect() Method
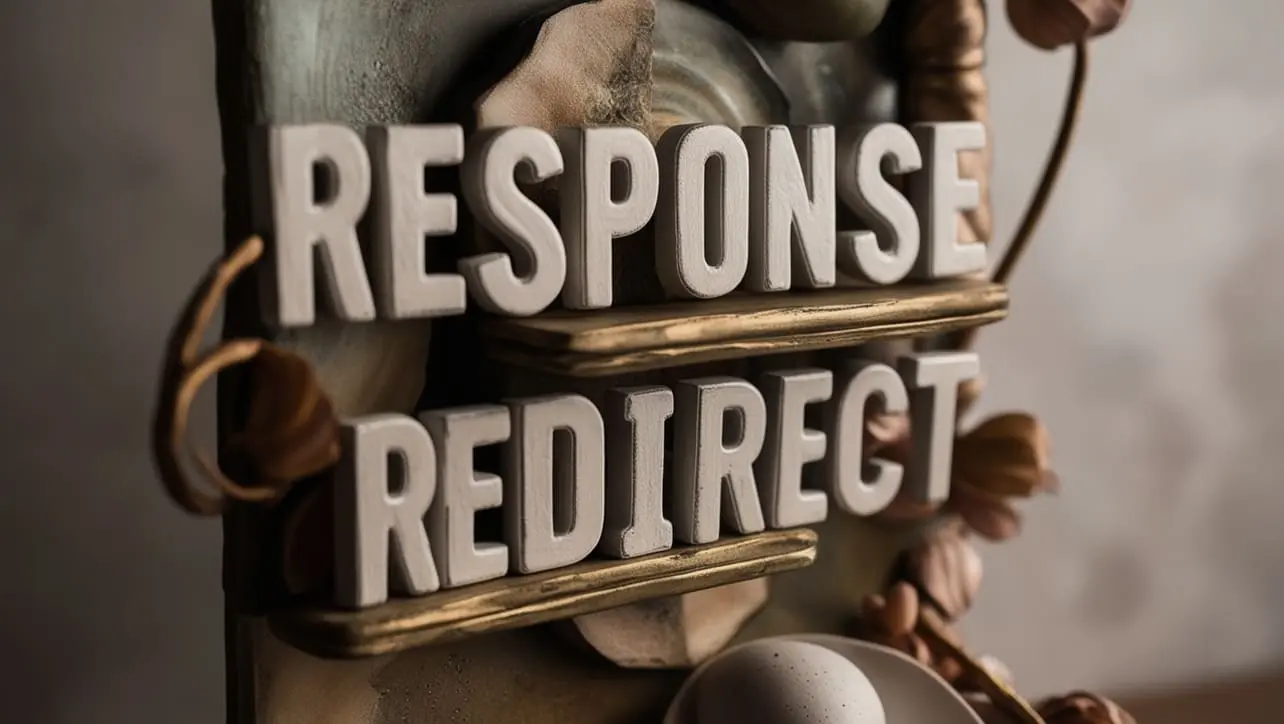
Photo Credit to CodeToFun
🙋 Introduction
In web development, redirecting users from one route to another is a common task. Express.js simplifies this process with the res.redirect()
method.
In this guide, we'll explore the syntax, use cases, and best practices of using res.redirect()
to enhance navigation in your Express.js applications.
💡 Syntax
The syntax for the res.redirect()
method is straightforward:
res.redirect([status,] path)
- status (Optional): The HTTP status code to use for the redirect (default is 302).
- path: The URL to redirect the user to.
❓ How res.redirect() Works
The res.redirect()
method sends a redirect response to the client, instructing the browser to navigate to a different URL. It's commonly used for scenarios like handling form submissions, authentication, or guiding users after a successful action.
app.post('/login', (req, res) => {
// Logic to validate login
if (userAuthenticated) {
res.redirect('/dashboard');
} else {
res.redirect('/login?error=1');
}
});
In this example, after validating the user's login credentials, the server uses res.redirect()
to send them to the dashboard or back to the login page with an error query parameter.
📚 Use Cases
After Form Submissions:
After successfully processing a form submission, redirect the user to a success page to provide feedback.
example.jsCopiedapp.post('/submit-form', (req, res) => { // Process form data // ... res.redirect('/success'); });
Authentication:
Use
res.redirect()
to guide users based on their authentication status. In this example, authenticated users are redirected to the admin dashboard, while unauthenticated users are sent to the login page.example.jsCopiedapp.get('/admin', (req, res) => { if (req.isAuthenticated()) { res.redirect('/admin/dashboard'); } else { res.redirect('/login'); } });
🏆 Best Practices
Specify HTTP Status Code:
When using
res.redirect()
, consider specifying the HTTP status code to indicate the type of redirect. Use 301 for permanent redirects and 302 for temporary redirects.example.jsCopied// Permanent redirect res.redirect(301, '/new-url'); // Temporary redirect (default) res.redirect('/temporary-url');
Include Query Parameters:
Include relevant query parameters in the redirect URL to provide additional context or information to the redirected route.
example.jsCopied// Redirect with query parameter res.redirect('/error-page?status=404');
Use Absolute Paths:
Consider using absolute paths for redirects to avoid issues with relative paths, especially in complex applications.
example.jsCopied// Redirect using an absolute path res.redirect('https://example.com/new-page');
🎉 Conclusion
The res.redirect()
method in Express.js is a powerful tool for guiding users through your web application. Whether handling form submissions, managing authentication, or providing feedback, understanding how to use res.redirect()
effectively will enhance user experience in your Express.js projects.
Now, armed with knowledge about the res.redirect()
method, go ahead and streamline navigation in your Express.js applications!
👨💻 Join our Community:
Author
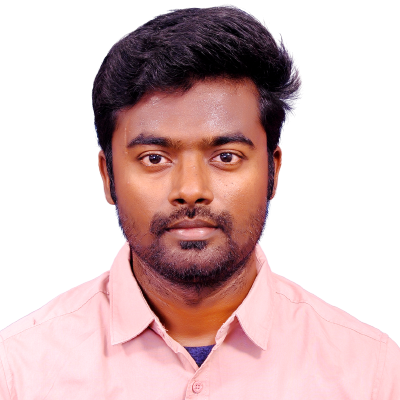
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.redirect() Method), please comment here. I will help you immediately.