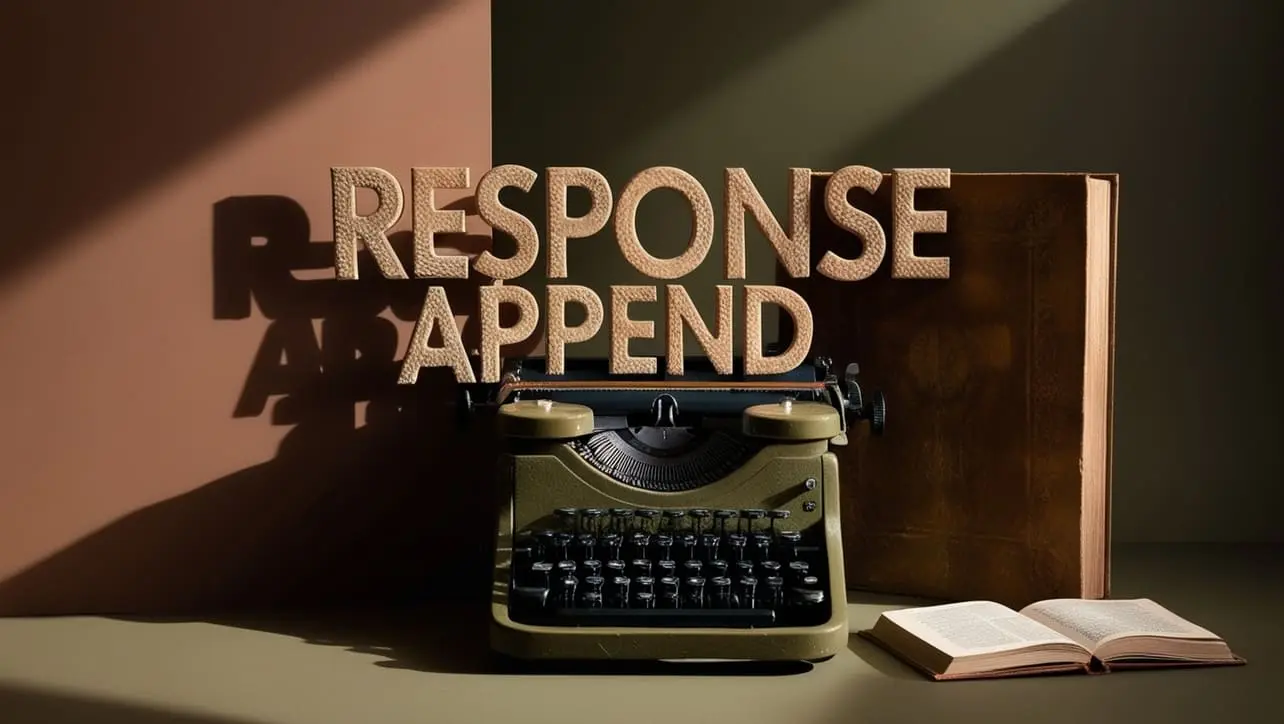
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.end() Method
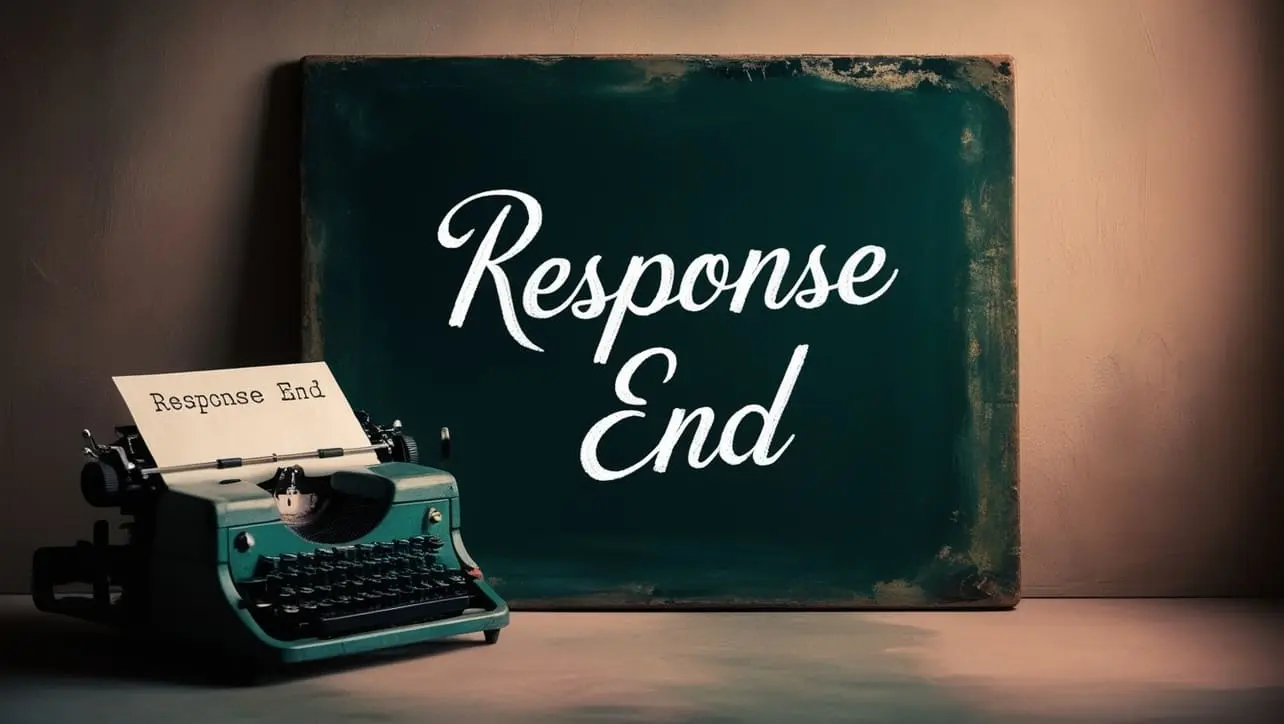
Photo Credit to CodeToFun
🙋 Introduction
In the realm of web development using Node.js and Express.js, managing HTTP responses is a fundamental aspect.
The res.end()
method plays a crucial role in finalizing and sending a response to the client.
In this guide, we'll explore the syntax, use cases, and best practices associated with the res.end()
method in Express.js.
💡 Syntax
The syntax for the res.end()
method is straightforward:
res.end([data] [, encoding] [, callback])
- data (Optional): A data parameter that can be a string or Buffer, representing the response body.
- encoding (Optional): A string specifying the encoding for the data.
- callback (Optional): A callback function that will be executed once the response has been sent.
❓ How res.end() Works
The res.end()
method is used to quickly end the response process by sending data to the client. It can be called with or without data, and it signifies the end of the response stream.
app.get('/hello', (req, res) => {
res.end('Hello, World!');
});
In this example, the server responds to a GET request to '/hello' with the string 'Hello, World!' using res.end()
.
📚 Use Cases
Sending Plain Text:
The
res.end()
method is often used to send simple plain text responses to clients.example.jsCopiedapp.get('/plaintext', (req, res) => { res.end('This is plain text response.'); });
Handling Empty Responses:
In this example, if a specific condition is met, the server responds with a 204 No Content status using res.status(204).end().
example.jsCopiedapp.get('/nocontent', (req, res) => { // Logic to handle a specific condition if (/* some condition */) { res.status(204).end(); } else { // Other logic res.end('Data to be sent'); } });
🏆 Best Practices
Set Appropriate HTTP Status Codes:
Ensure that you set the appropriate HTTP status codes before calling
res.end()
. This communicates the outcome of the request to the client.example.jsCopiedapp.get('/notfound', (req, res) => { res.status(404).end('Resource not found.'); });
Use res.send() for JSON Responses:
For JSON responses, consider using res.send() instead of
res.end()
as it automatically sets the 'Content-Type' header to 'application/json'.example.jsCopiedapp.get('/json', (req, res) => { const jsonData = { message: 'This is a JSON response.' }; res.send(jsonData); });
Leverage res.end() for Binary Data:
When dealing with binary data, such as images or files,
res.end()
can be more appropriate.example.jsCopiedapp.get('/image', (req, res) => { // Logic to read and send image binary data res.end(imageBinaryData, 'binary'); });
🎉 Conclusion
Understanding and effectively using the res.end()
method in Express.js is key to crafting efficient and tailored responses in your web applications. Whether you're sending plain text or handling specific conditions, res.end()
provides a flexible way to conclude the response cycle.
Now equipped with knowledge about the res.end()
method, go ahead and optimize your Express.js applications for better response management!
👨💻 Join our Community:
Author
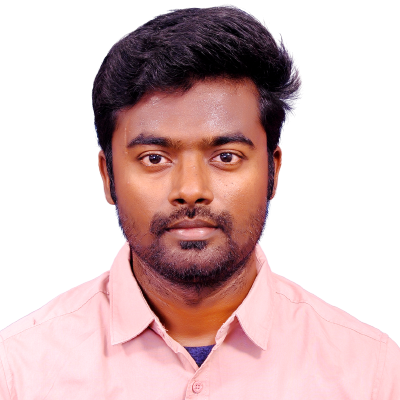
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.end() Method), please comment here. I will help you immediately.