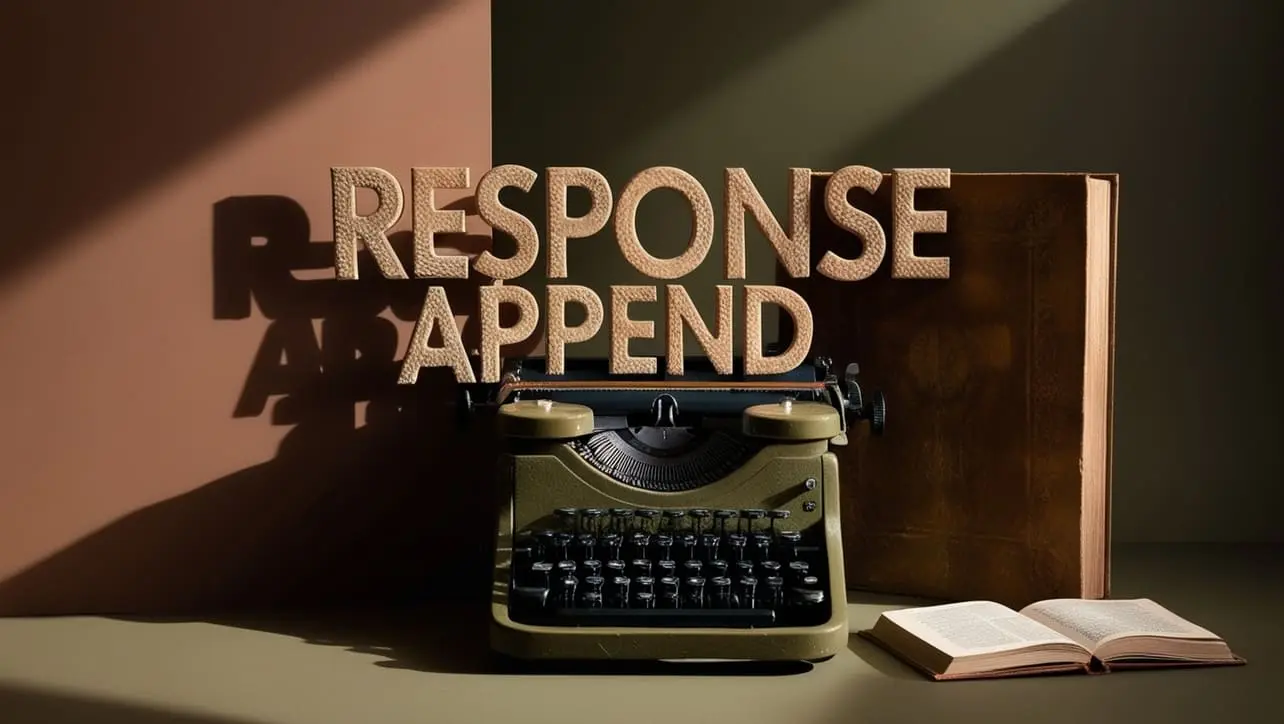
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.render() Method
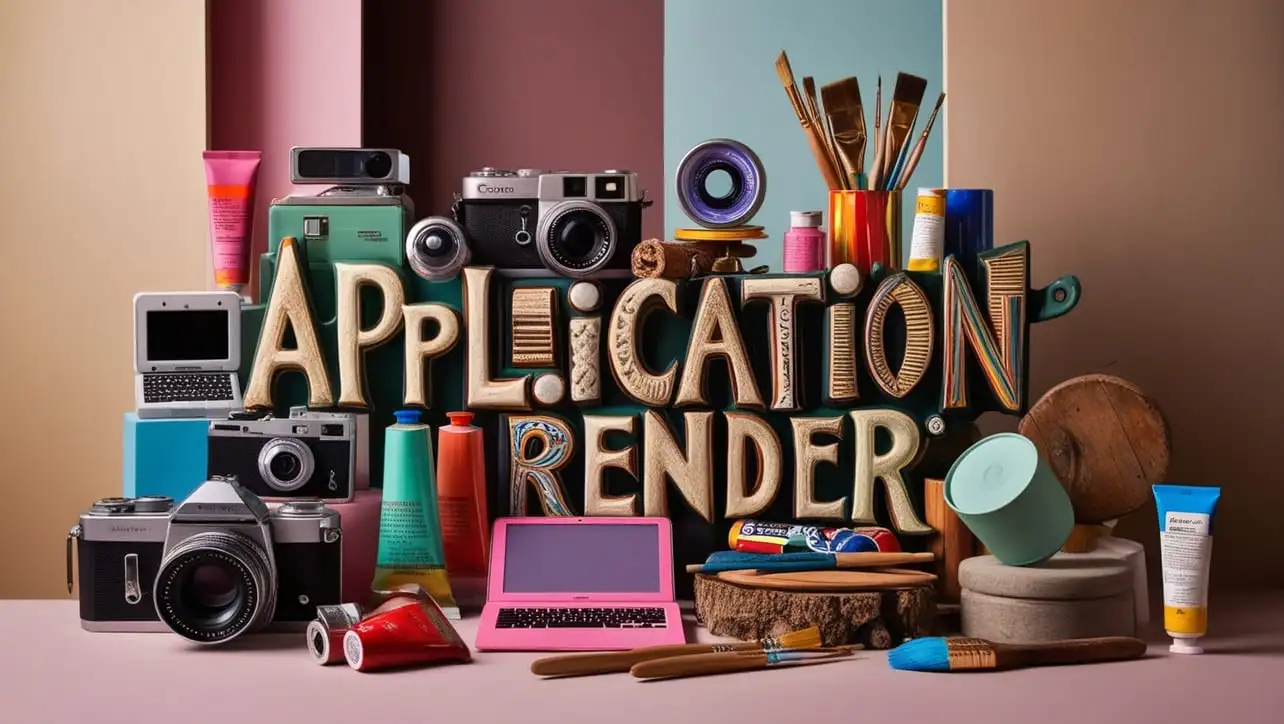
Photo Credit to CodeToFun
๐ Introduction
Server-side rendering (SSR) plays a crucial role in delivering performant and SEO-friendly web applications. Express.js, a popular web application framework for Node.js, provides the app.render()
method, facilitating server-side rendering.
In this guide, we'll explore the syntax, usage, and examples of using app.render()
to enhance your Express.js applications.
๐ก Syntax
The app.render()
method in Express.js has the following syntax:
app.render(view, [locals], callback)
- view: A string representing the name of the view template to render.
- locals (optional): An object containing local variables that will be passed to the view.
- callback (optional): A callback function that receives the rendered HTML as its argument.
โ How app.render() Works
The app.render()
method is used to render a view template specified by the view parameter. It's particularly useful for server-side rendering, where you generate HTML on the server and send it as the response.
app.get('/home', (req, res) => {
// Rendering the 'home' view template
app.render('home', { title: 'Express SSR' }, (err, html) => {
if (err) {
res.status(500).send('Internal Server Error');
} else {
res.send(html);
}
});
});
In this example, when a GET request is made to '/home', the app.render()
method renders the 'home' view template with the provided locals ({ title: 'Express SSR' }) and sends the resulting HTML as the response.
๐ Use Cases
Dynamic Content Generation:
Use
app.render()
to dynamically generate HTML content based on data retrieved from a database or another source.example.jsCopiedapp.get('/article/:id', (req, res) => { const articleId = req.params.id; // Fetch article data from a database or other source const articleData = // ...; // Render the 'article' view template with dynamic content app.render('article', { articleId, articleData }, (err, html) => { if (err) { res.status(500).send('Internal Server Error'); } else { res.send(html); } }); });
Consistent Layouts:
Ensure consistent layouts by using
app.render()
to render views with shared components, headers, or footers.example.jsCopiedapp.get('/dashboard', (req, res) => { // Render the 'dashboard' view template with a consistent layout app.render('dashboard', { user: req.user }, (err, html) => { if (err) { res.status(500).send('Internal Server Error'); } else { res.send(html); } }); });
๐ Best Practices
Error Handling:
Implement robust error handling within the
app.render()
callback to handle potential rendering errors gracefully.example.jsCopiedapp.get('/page', (req, res) => { // Rendering the 'page' view template app.render('page', { data: /* ... */ }, (err, html) => { if (err) { console.error('Error rendering page:', err); res.status(500).send('Internal Server Error'); } else { res.send(html); } }); });
Asynchronous Rendering:
Leverage the asynchronous nature of
app.render()
when rendering views that require asynchronous data fetching.example.jsCopiedapp.get('/async', async (req, res) => { const asyncData = await fetchDataAsync(); // Render the 'async' view template with asynchronously fetched data app.render('async', { asyncData }, (err, html) => { if (err) { res.status(500).send('Internal Server Error'); } else { res.send(html); } }); });
๐ Conclusion
The app.render()
method in Express.js facilitates server-side rendering, allowing you to generate HTML dynamically and provide a better user experience. Whether you're rendering dynamic content or ensuring consistent layouts, understanding and utilizing app.render()
will enhance your Express.js applications.
Now, equipped with knowledge about the app.render()
method, explore the world of server-side rendering in Express.js and elevate your web development projects!
๐จโ๐ป Join our Community:
Author
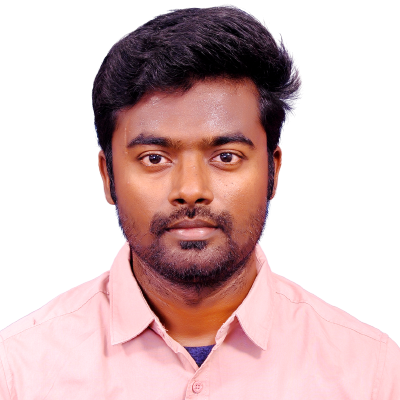
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.render() Method), please comment here. I will help you immediately.