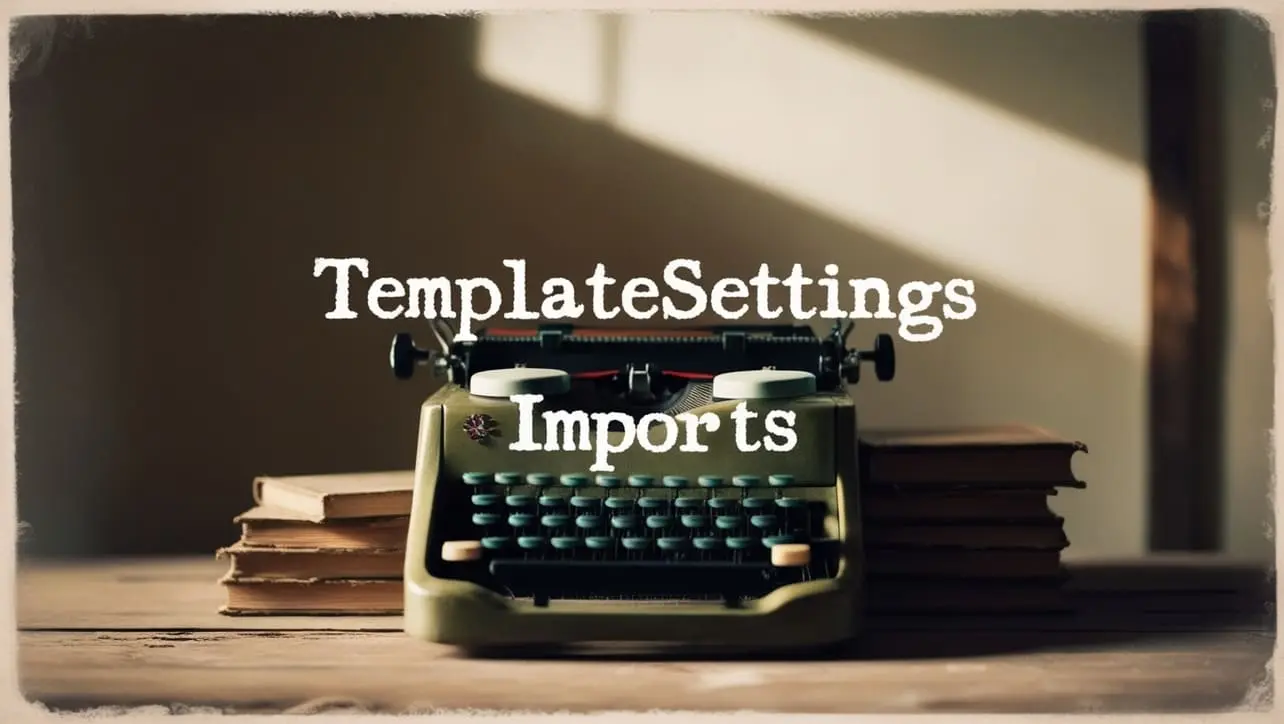
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.intersectionWith() Array Method
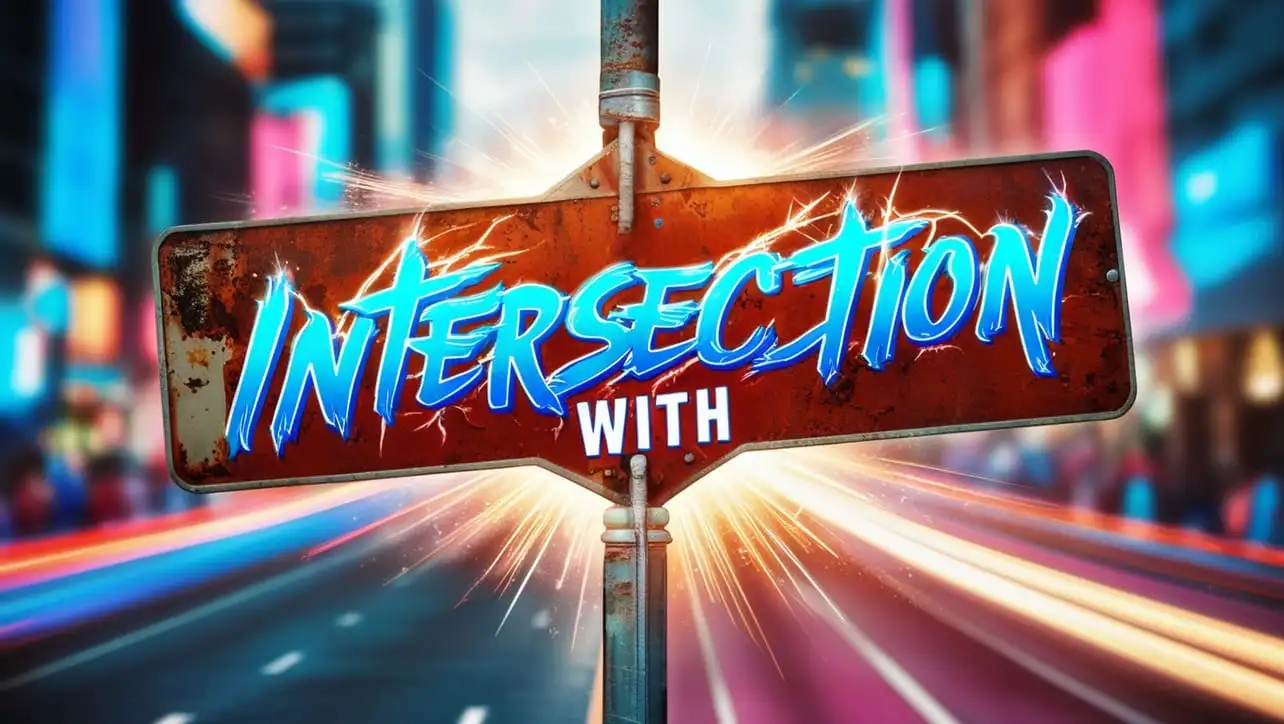
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, managing arrays efficiently is a common challenge.
Lodash, a powerful utility library, provides a variety of functions to address such challenges. One notable method is _.intersectionWith()
, which facilitates the intersection of arrays based on custom equality comparisons.
This method proves invaluable when dealing with complex datasets and the need to find common elements using custom comparison logic.
🧠 Understanding _.intersectionWith()
The _.intersectionWith()
method in Lodash allows you to find the intersection of arrays using a custom comparator function. This means you can specify your own rules for determining equality between elements in arrays, providing flexibility beyond strict equality checks.
💡 Syntax
_.intersectionWith(array1, array2, [comparator])
- array1, array2: The arrays to inspect.
- comparator: The comparator function invoked per element.
📝 Example
Let's delve into a practical example to illustrate the power of _.intersectionWith()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [{ x: 1, y: 2 }, { x: 2, y: 3 }];
const array2 = [{ x: 1, y: 2 }, { x: 3, y: 4 }];
const intersectionResult = _.intersectionWith(array1, array2, _.isEqual);
console.log(intersectionResult);
// Output: [{ x: 1, y: 2 }]
In this example, _.isEqual is used as the comparator function to find the intersection based on deep equality.
🏆 Best Practices
Understand Your Data Structure:
Before using
_.intersectionWith()
, thoroughly understand the structure of your data. The custom comparator function should be tailored to the specific attributes or properties you want to compare.data-structure.jsCopiedconst array1 = [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]; const array2 = [{ id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }]; const commonUsers = _.intersectionWith(array1, array2, (user1, user2) => user1.id === user2.id); console.log(commonUsers); // Output: [{ id: 2, name: 'Bob' }]
Choose the Right Comparator:
Select or create a comparator function that suits your comparison needs. Lodash provides utilities like _.isEqual for deep equality checks, but you can create custom comparators for more specific scenarios.
right-comparator.jsCopiedconst datesArray1 = [new Date('2022-01-01'), new Date('2022-02-01')]; const datesArray2 = [new Date('2022-01-01'), new Date('2022-03-01')]; const commonDates = _.intersectionWith(datesArray1, datesArray2, (date1, date2) => date1.getMonth() === date2.getMonth()); console.log(commonDates); // Output: [Sun Jan 01 2023 00:00:00 GMT+0000 (Coordinated Universal Time)]
Array Order Matters:
Keep in mind that
_.intersectionWith()
considers the order of arrays. If the order is essential, ensure it aligns with your requirements.array-order-matters.jsCopiedconst array1 = [1, 2, 3, 4]; const array2 = [4, 3, 2, 1]; const intersectionResult = _.intersectionWith(array1, array2, (value1, value2) => value1 === value2); console.log(intersectionResult); // Output: [] (order matters, elements at corresponding positions are different)
📚 Use Cases
Object Intersection:
When dealing with arrays of objects and needing to find common elements based on specific properties,
_.intersectionWith()
becomes a handy tool.object-intersection.jsCopiedconst usersInGroup1 = [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]; const usersInGroup2 = [{ id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }]; const commonUsers = _.intersectionWith(usersInGroup1, usersInGroup2, (user1, user2) => user1.id === user2.id); console.log(commonUsers); // Output: [{ id: 2, name: 'Bob' }]
Custom Equality Logic:
For scenarios where standard equality checks are insufficient,
_.intersectionWith()
allows you to define custom logic for comparing elements.custom-equality-logic.jsCopiedconst datesArray1 = [new Date('2022-01-01'), new Date('2022-02-01')]; const datesArray2 = [new Date('2022-01-01'), new Date('2022-03-01')]; const commonDates = _.intersectionWith(datesArray1, datesArray2, (date1, date2) => date1.getMonth() === date2.getMonth()); console.log(commonDates); // Output: [Sun Jan 01 2023 00:00:00 GMT+0000 (Coordinated Universal Time)]
🎉 Conclusion
The _.intersectionWith()
method in Lodash provides a robust solution for finding the intersection of arrays with custom equality comparisons. Its flexibility allows developers to tailor the comparison logic to their specific needs, making it an essential tool for scenarios where standard equality checks fall short.
Explore the capabilities of _.intersectionWith()
and elevate your array manipulation skills within the realm of JavaScript development. Enhance your projects by efficiently finding common elements using custom comparison logic!
👨💻 Join our Community:
Author
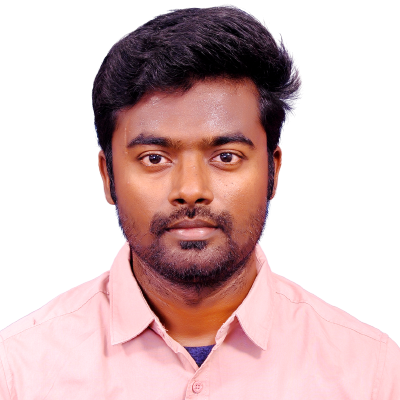
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
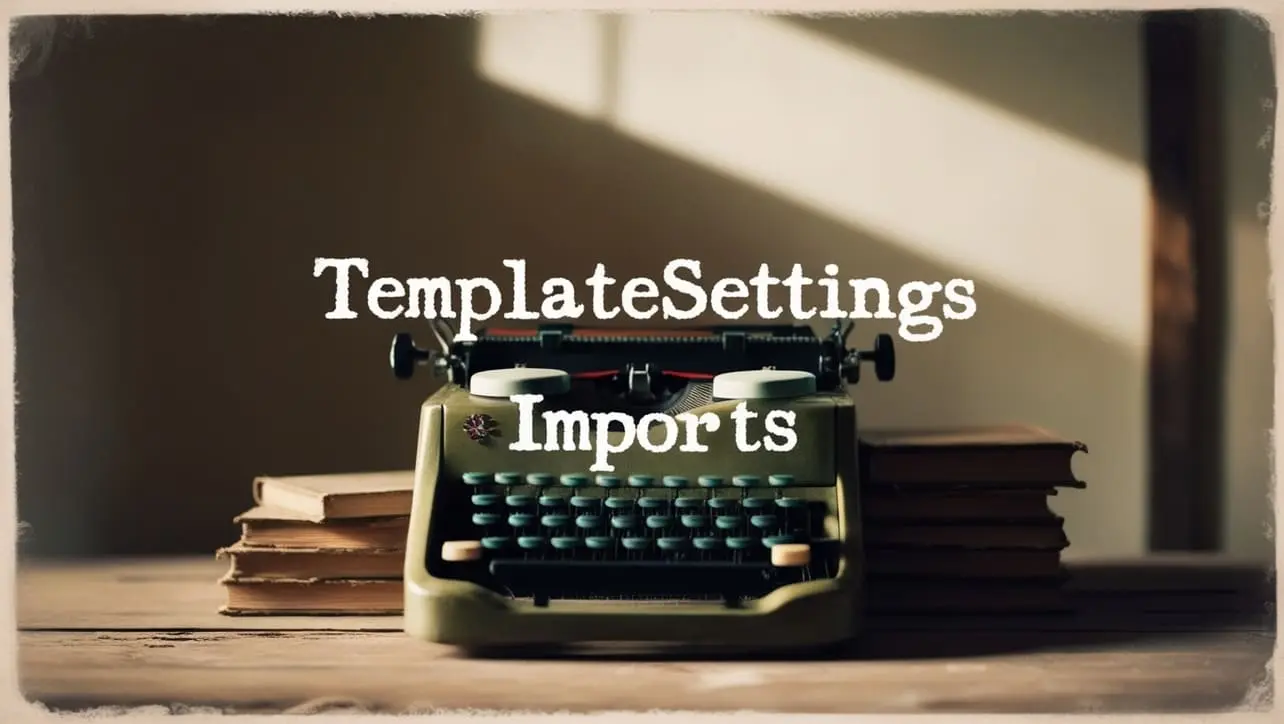
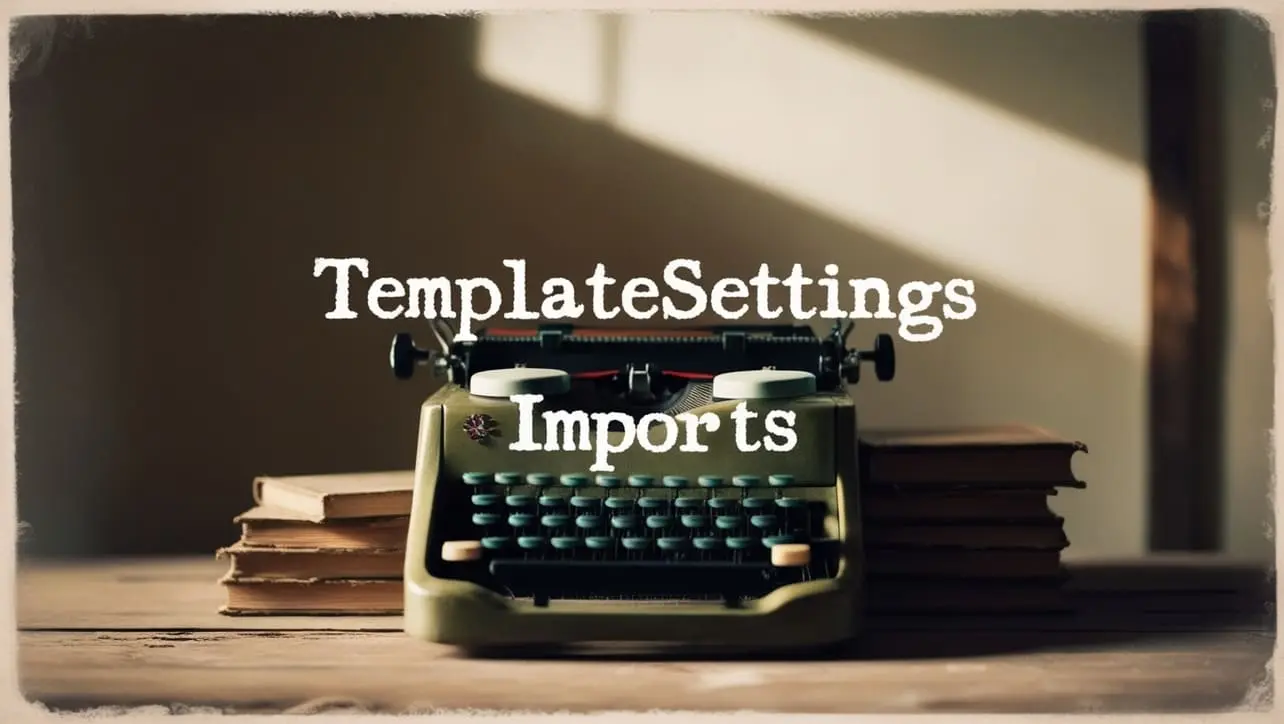
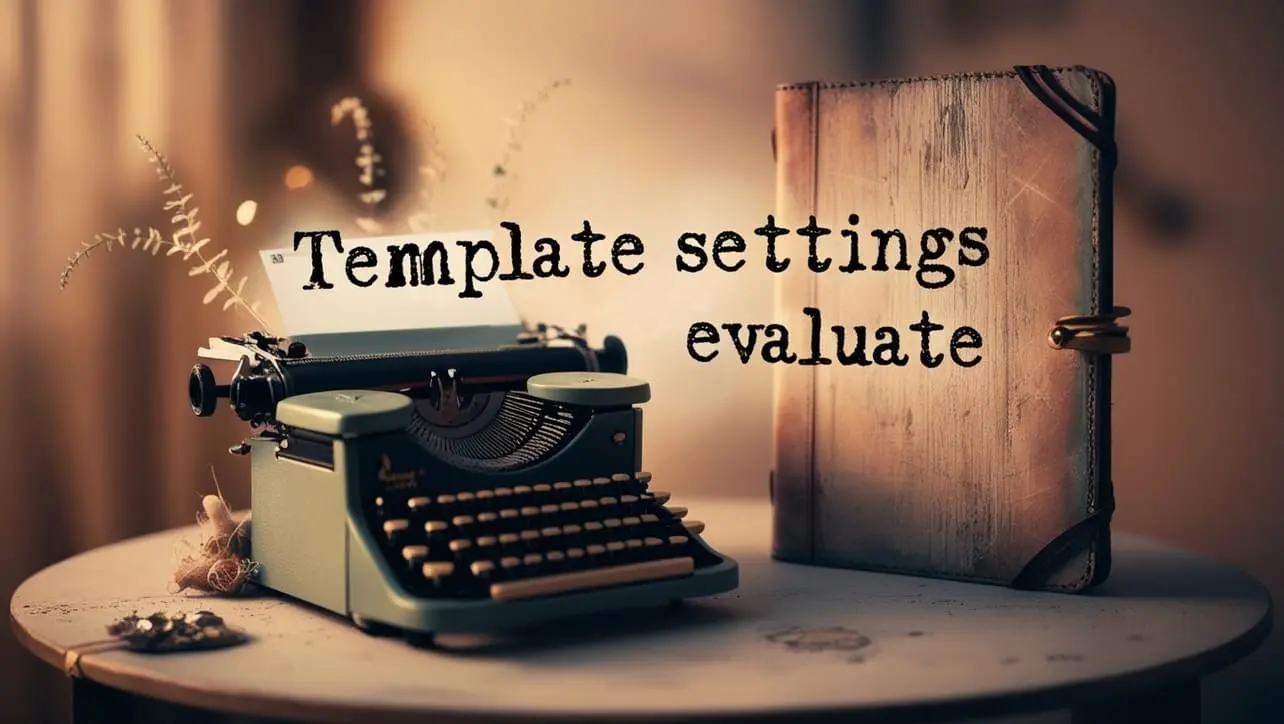
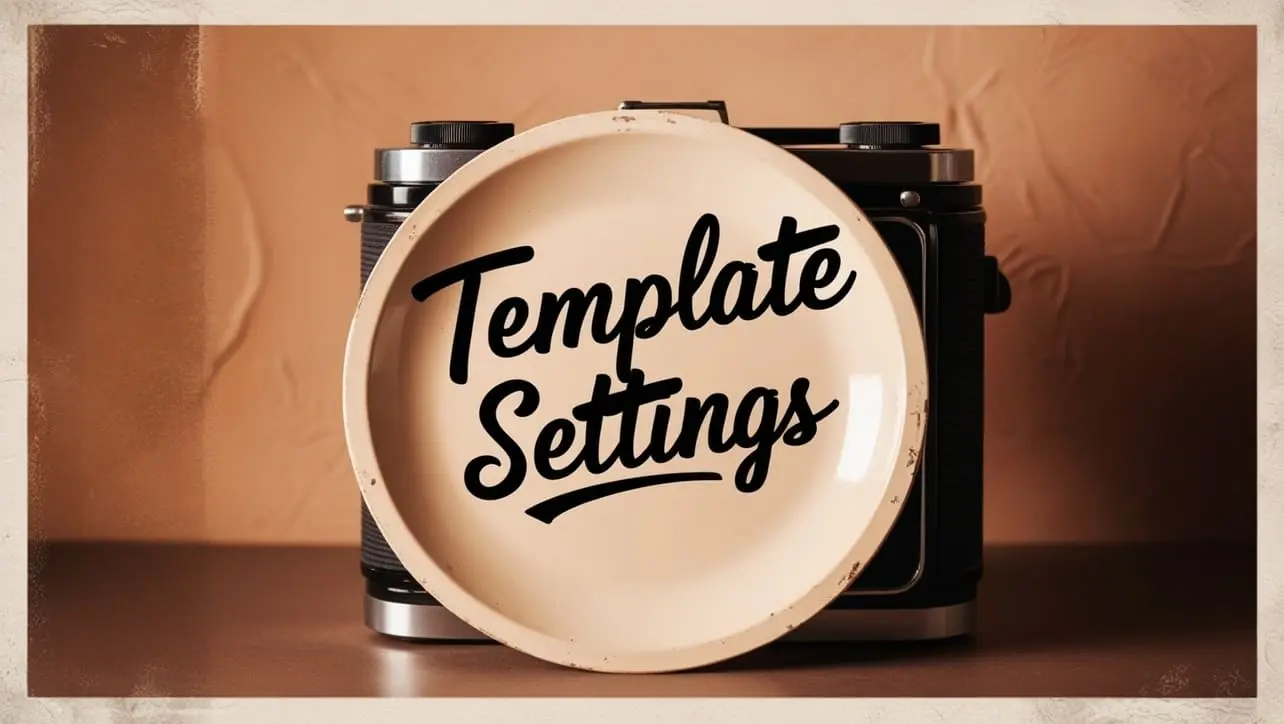
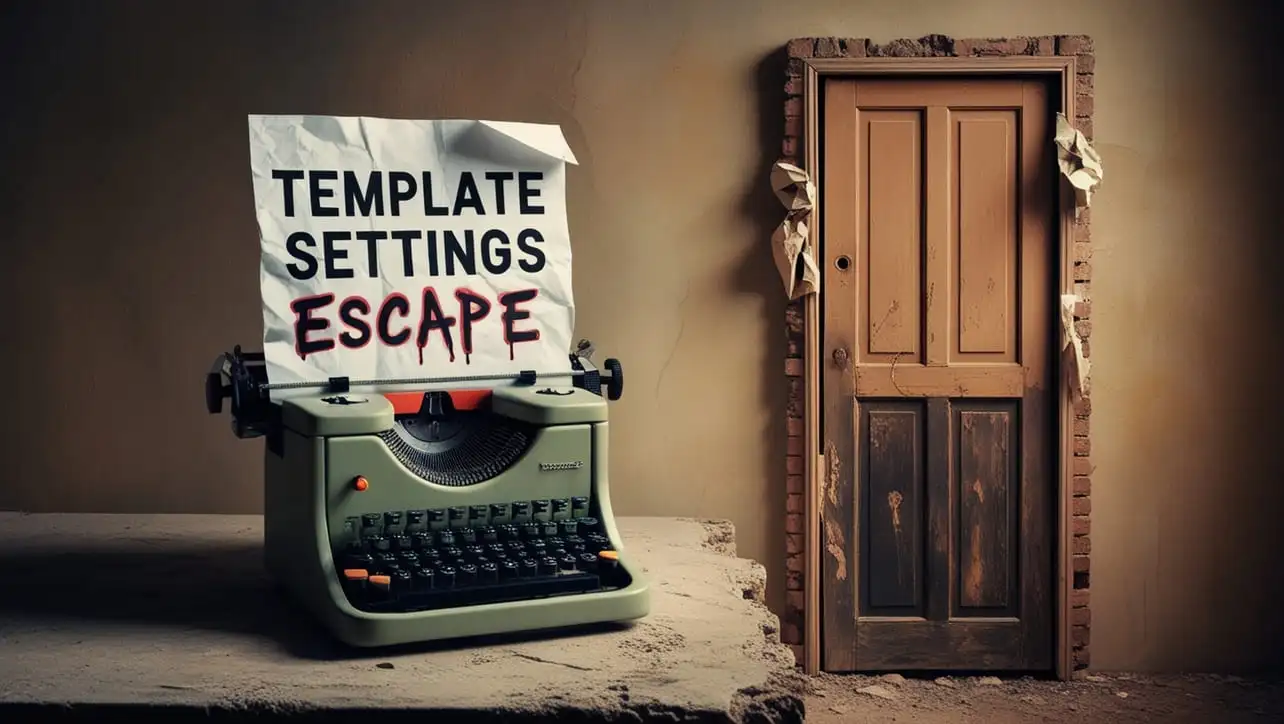
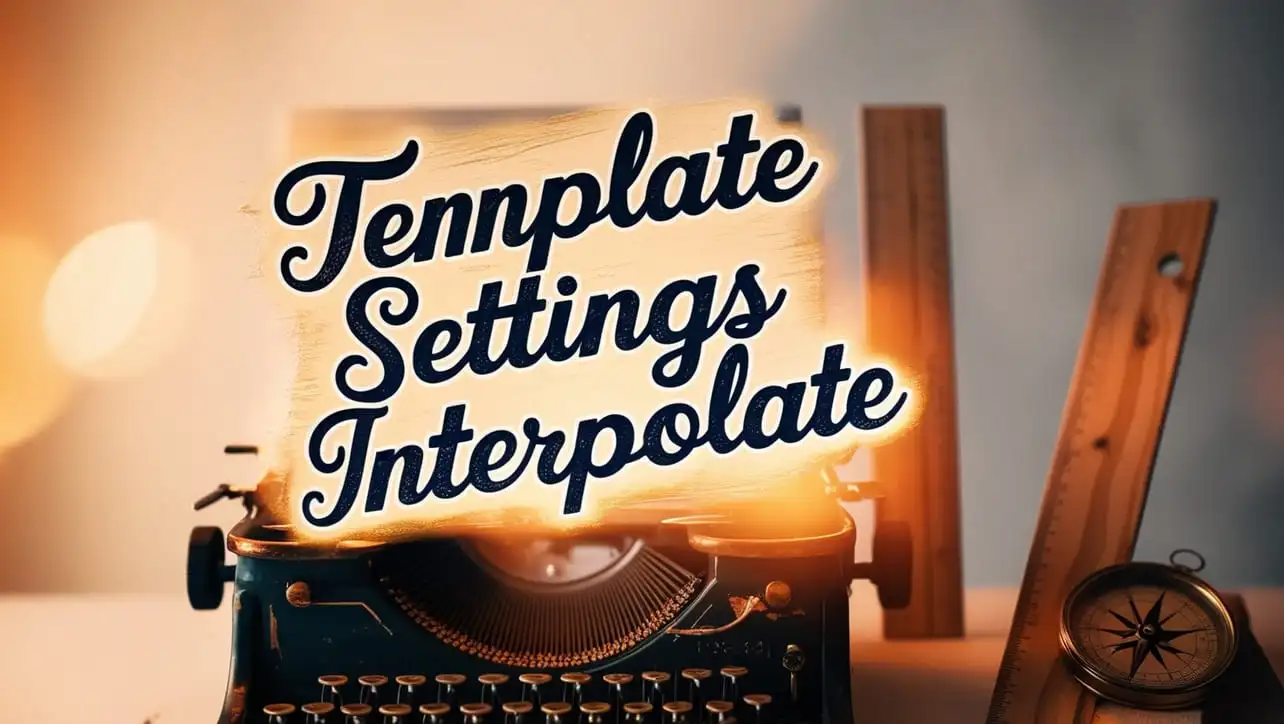
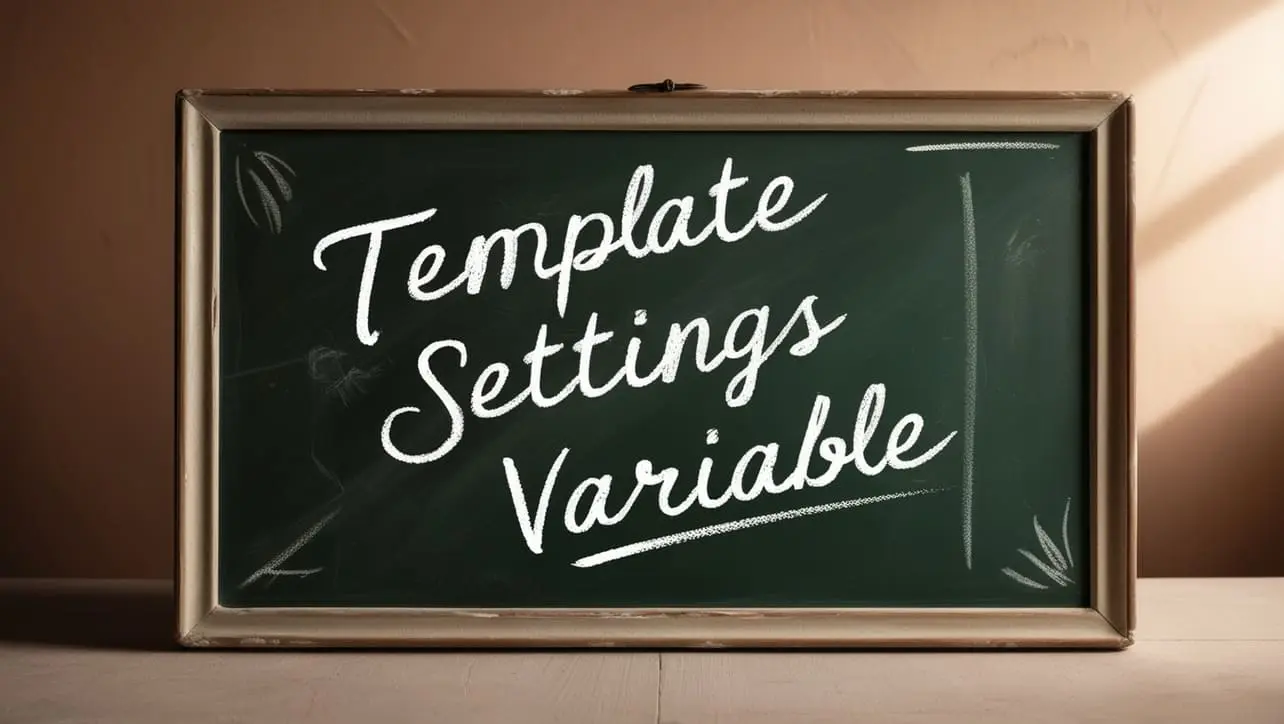
If you have any doubts regarding this article (Lodash _.intersectionWith() Array Method), please comment here. I will help you immediately.