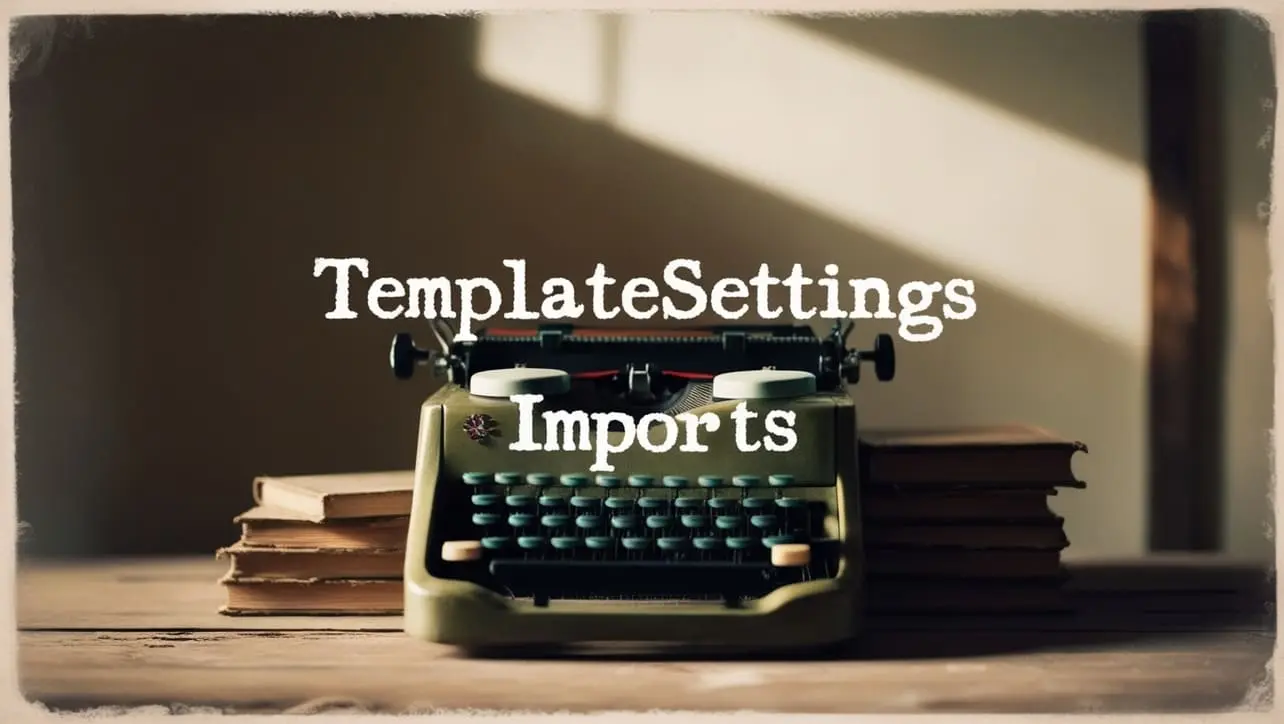
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.intersectionBy() Array Method
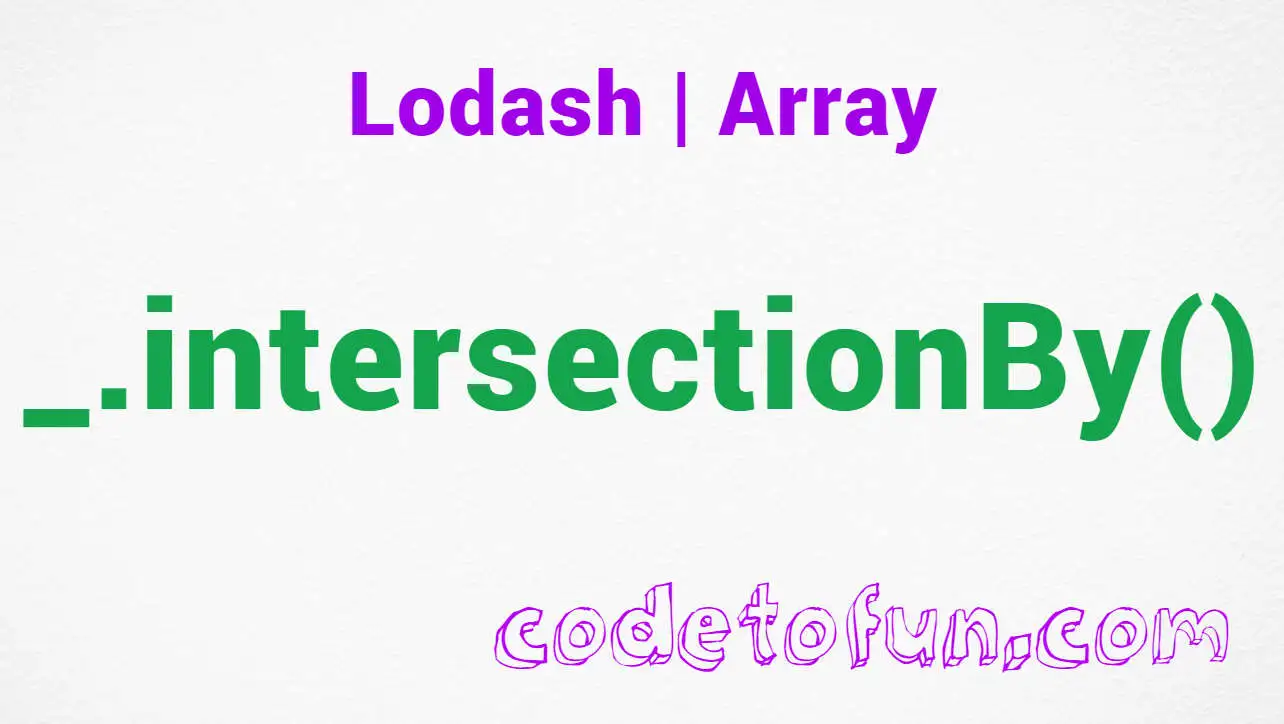
Photo Credit to CodeToFun
🙋 Introduction
Efficiently comparing and finding the common elements between arrays is a common task in JavaScript programming.
Lodash provides a powerful utility function, _.intersectionBy()
, which simplifies the process of finding the intersection of arrays based on a specific property or criteria. This method is particularly useful when working with complex data structures or objects.
🧠 Understanding _.intersectionBy()
The _.intersectionBy()
method in Lodash allows you to find the common elements between two or more arrays based on a specified property or iteratee function. This goes beyond the standard intersection by allowing a custom rule to determine equality.
💡 Syntax
_.intersectionBy([arrays], [iteratee=_.identity])
- arrays: The arrays to inspect.
- iteratee: The iteratee invoked per element (default is _.identity).
📝 Example
Let's delve into an example to illustrate the power of _.intersectionBy()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [{ 'x': 1 }, { 'x': 2 }, { 'x': 3 }];
const array2 = [{ 'x': 1 }, { 'x': 2 }, { 'x': 4 }];
const commonElements = _.intersectionBy(array1, array2, 'x');
console.log(commonElements);
// Output: [{ 'x': 1 }, { 'x': 2 }]
In this example, _.intersectionBy()
is used to find the common elements between array1 and array2 based on the x property.
🏆 Best Practices
Choose an Appropriate Iteratee:
Select a meaningful property or provide a custom iteratee function to accurately compare elements. This is essential for finding intersections based on specific criteria.
appropriate-iteratee.jsCopiedconst array1 = [{ 'x': 1 }, { 'x': 2 }, { 'x': 3 }]; const array2 = [{ 'x': 1 }, { 'x': 2 }, { 'x': 4 }]; // Using a custom iteratee function to compare objects based on the sum of their 'x' and 'y' properties const customIteratee = (obj) => obj.x + obj.y; const commonElements = _.intersectionBy(array1, array2, customIteratee); console.log(commonElements);
Handle Edge Cases:
Account for edge cases, such as empty arrays or undefined iteratee functions. Ensure your code gracefully handles these scenarios to avoid unexpected behavior.
handle-edge-cases.jsCopiedconst emptyArray = []; const undefinedIteratee = undefined; const result1 = _.intersectionBy(emptyArray, [], 'x'); // Returns: [] const result2 = _.intersectionBy([1, 2, 3], [4, 5, 6], undefinedIteratee); // Returns: [] console.log(result1); console.log(result2);
📚 Use Cases
Filtering Based on Property:
_.intersectionBy()
can be used to filter objects based on a specific property, providing a concise way to find common elements.filtering-based-on-property.jsCopiedconst usersArray = [{ 'id': 1, 'name': 'Alice' }, { 'id': 2, 'name': 'Bob' }, { 'id': 3, 'name': 'Charlie' }]; const activeUsersArray = [{ 'id': 1, 'active': true }, { 'id': 2, 'active': false }]; const commonUsers = _.intersectionBy(usersArray, activeUsersArray, 'id'); console.log(commonUsers); // Output: [{ 'id': 1, 'name': 'Alice' }]
Complex Object Intersection:
When dealing with arrays of complex objects,
_.intersectionBy()
allows you to find common elements based on a nested property or a custom comparison function.complex-object-intersection.jsCopiedconst array1 = [{ 'user': { 'id': 1, 'name': 'Alice' } }, { 'user': { 'id': 2, 'name': 'Bob' } }]; const array2 = [{ 'user': { 'id': 1, 'name': 'Alice' } }, { 'user': { 'id': 3, 'name': 'Charlie' } }]; const commonUsers = _.intersectionBy(array1, array2, 'user.id'); console.log(commonUsers); // Output: [{ 'user': { 'id': 1, 'name': 'Alice' } }]
🎉 Conclusion
The _.intersectionBy()
method in Lodash is a valuable tool for JavaScript developers needing a flexible and efficient way to find the intersection of arrays based on specific properties or criteria. By incorporating this method into your code, you can streamline your array comparison tasks and improve the maintainability of your projects.
Explore the capabilities of _.intersectionBy()
and elevate your array manipulation skills with Lodash!
👨💻 Join our Community:
Author
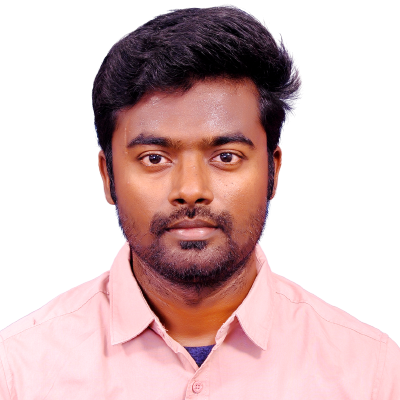
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
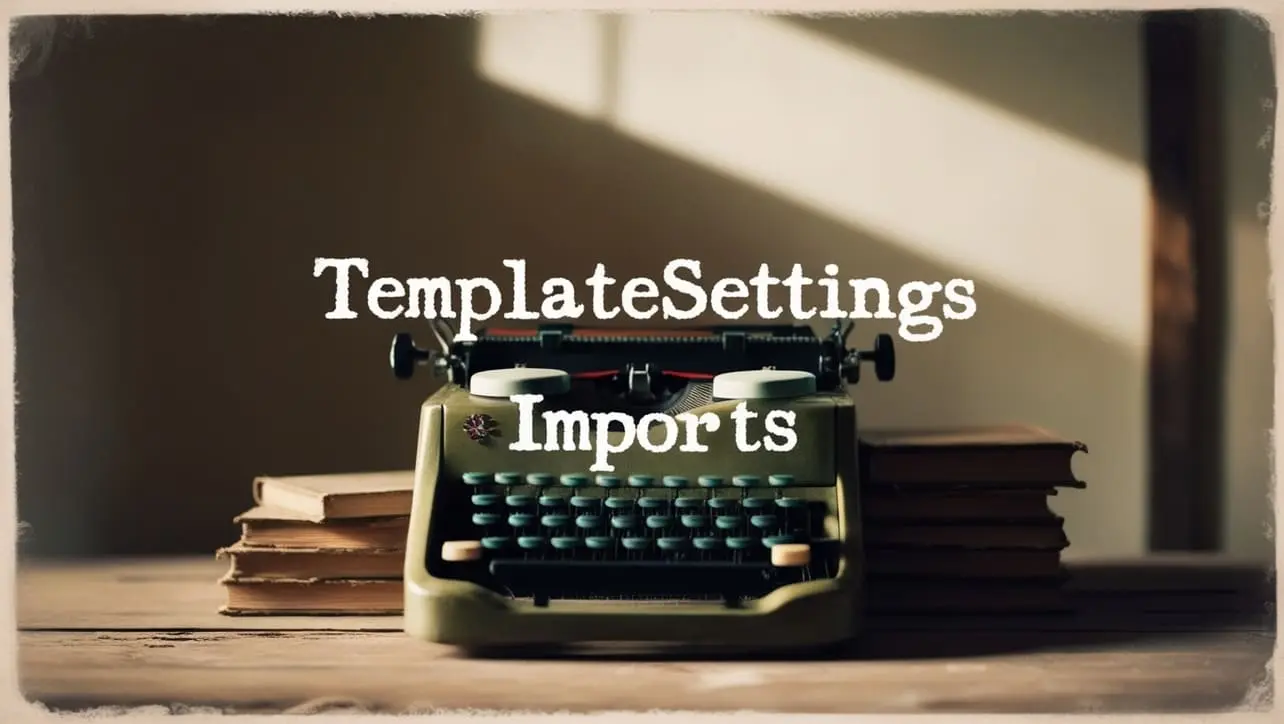
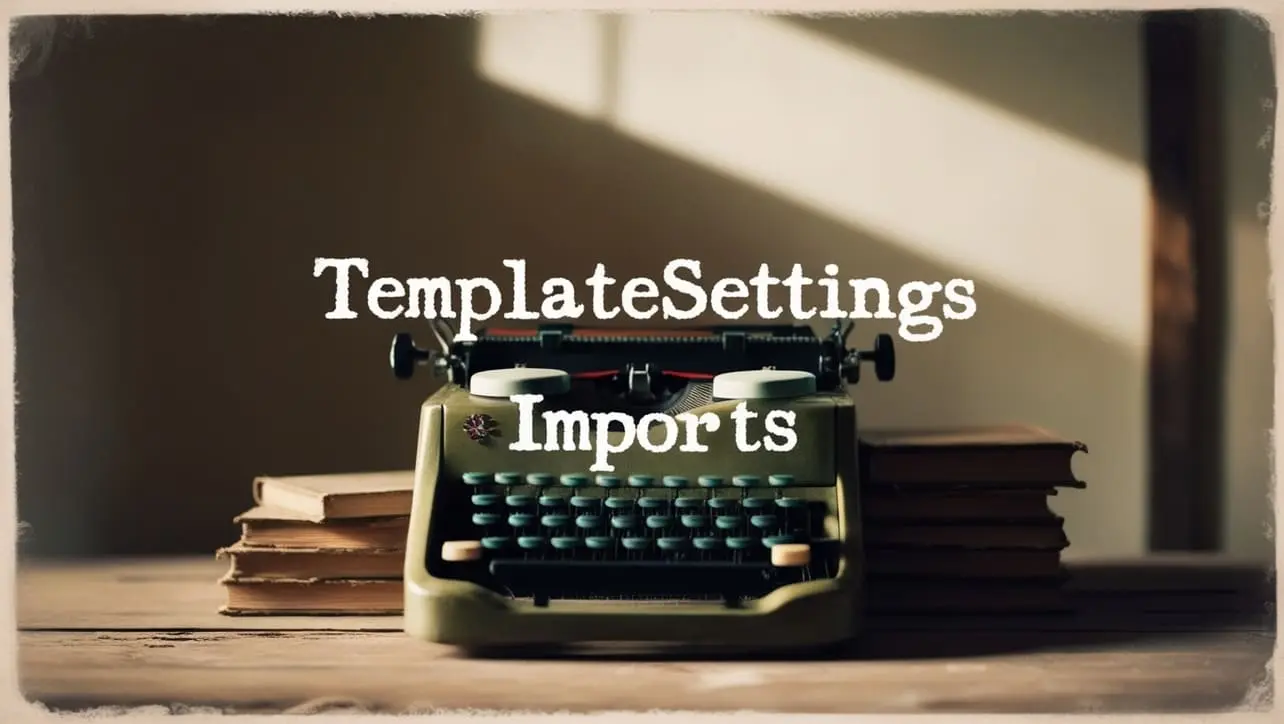
Lodash _.templateSettings.imports Property
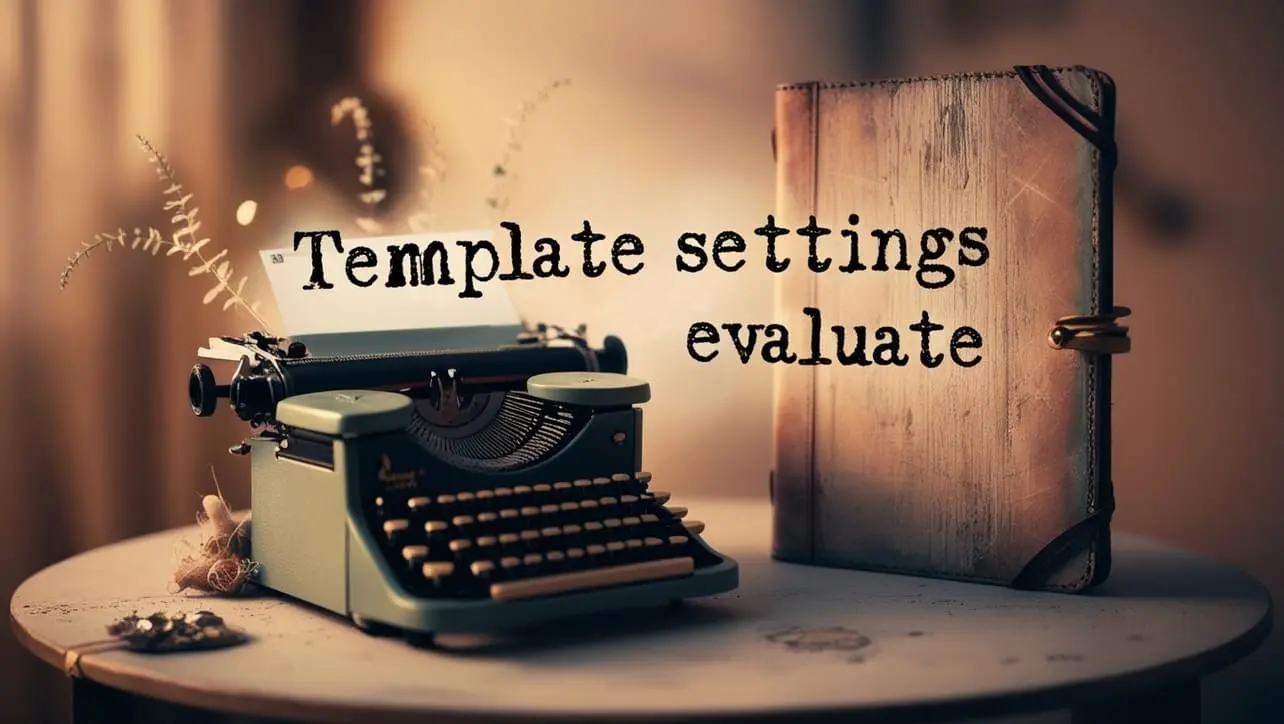
Lodash _.templateSettings.evaluate Property
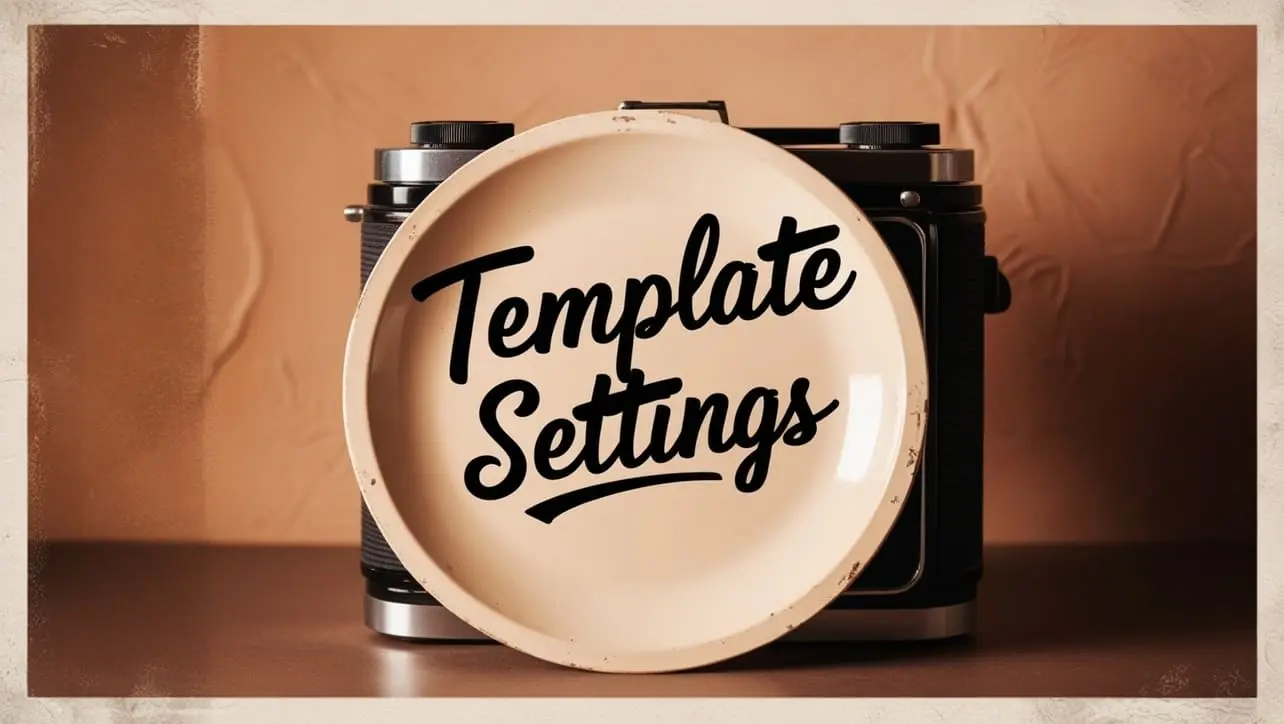
Lodash _.templateSettings Property
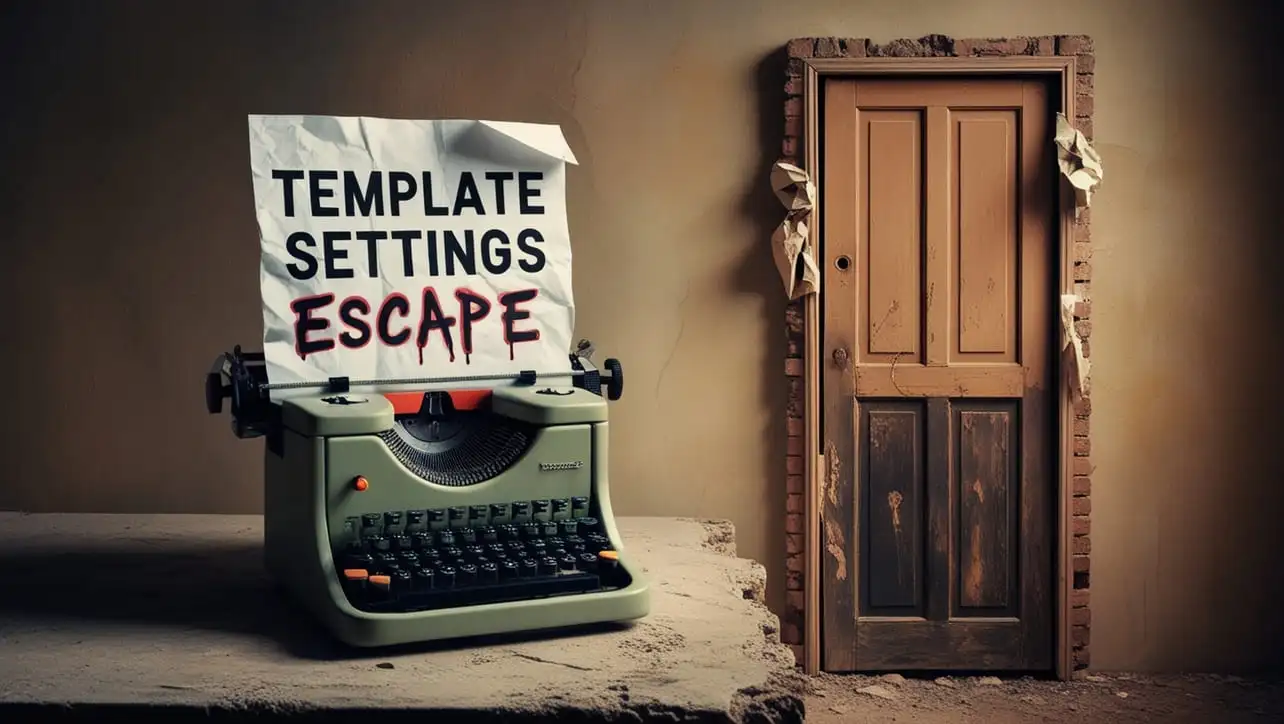
Lodash _.templateSettings.escape Property
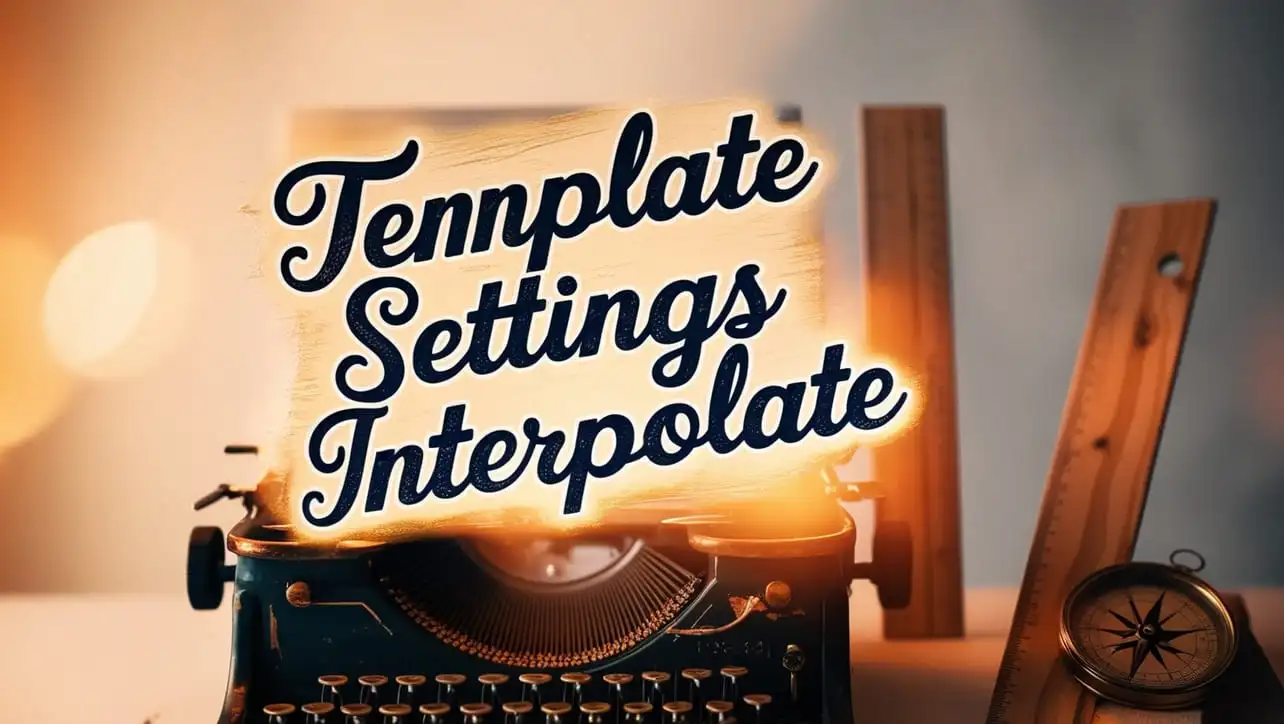
Lodash _.templateSettings.interpolate Property
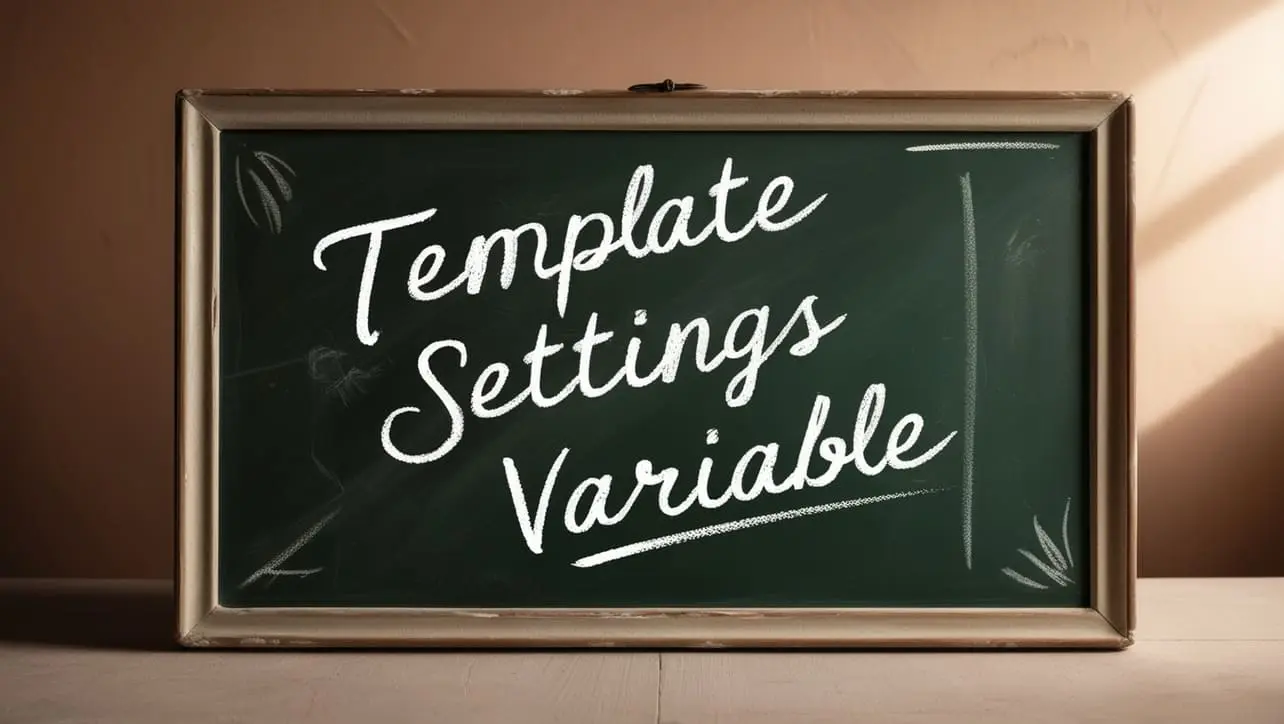
If you have any doubts regarding this article (Lodash _.intersectionBy() Array Method), please comment here. I will help you immediately.