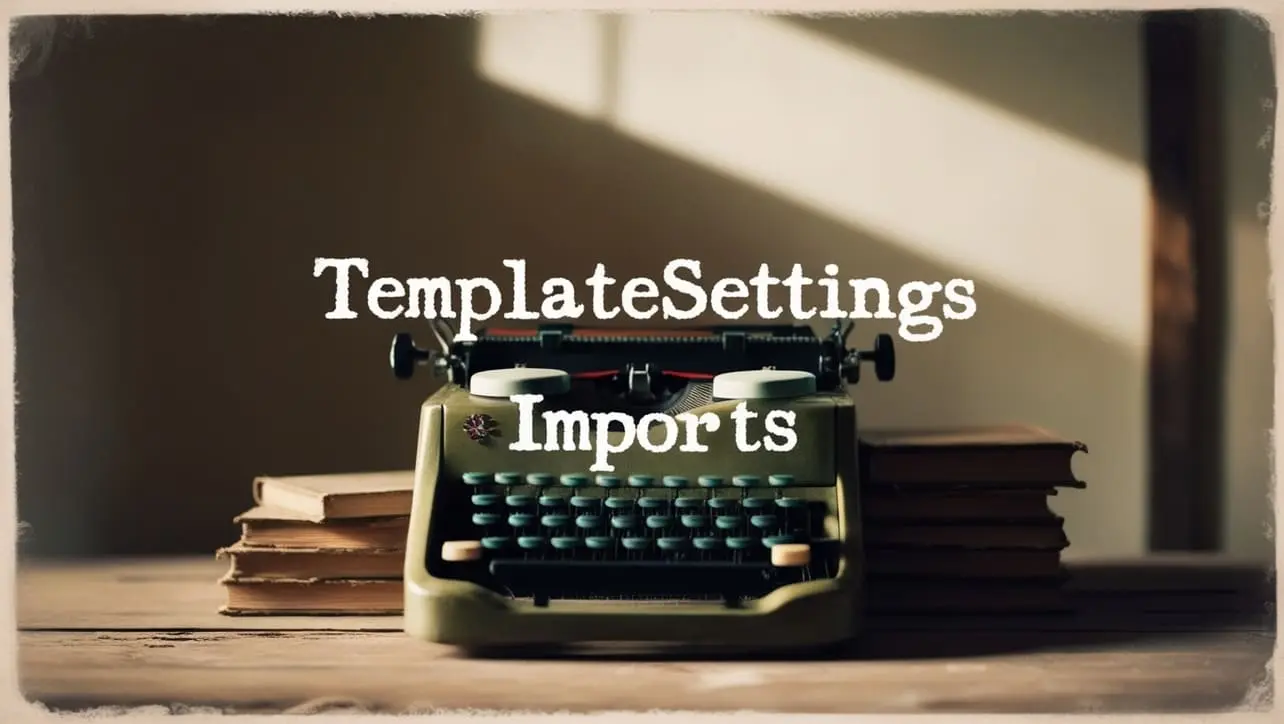
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.intersection() Array Method
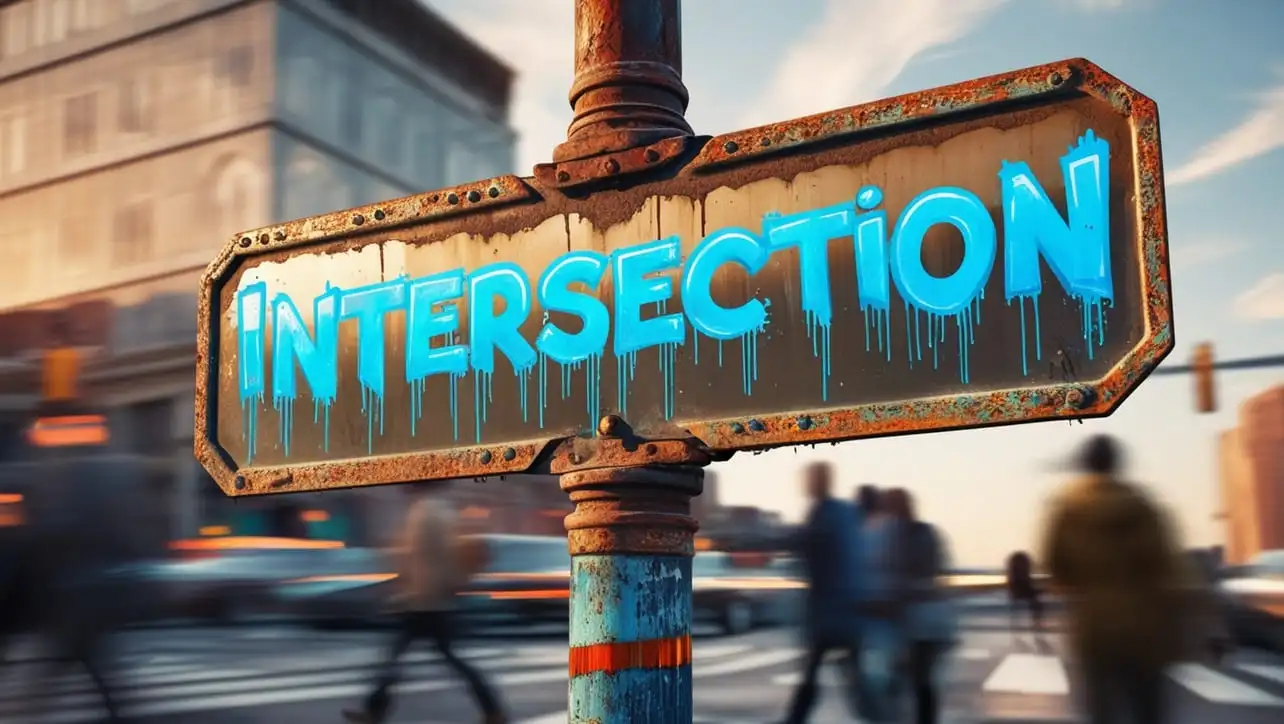
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, working with arrays often involves finding common elements among multiple arrays. The _.intersection()
method in the Lodash library is a powerful tool designed for this specific purpose.
This method allows developers to efficiently identify the intersection of values shared between two or more arrays, simplifying complex data manipulations.
🧠 Understanding _.intersection()
The _.intersection()
method in Lodash compares arrays and returns a new array containing the common elements found across all provided arrays. This can be particularly useful in scenarios where you need to filter out shared values or perform set operations.
💡 Syntax
_.intersection([arrays])
- arrays: Arrays to inspect.
📝 Example
Let's explore a practical example to demonstrate the functionality of _.intersection()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [1, 2, 3, 4];
const array2 = [2, 3, 4, 5];
const array3 = [3, 4, 5, 6];
const commonElements = _.intersection(array1, array2, array3);
console.log(commonElements);
// Output: [3, 4]
In this example, commonElements contains the values that are present in all three arrays.
🏆 Best Practices
Validate Inputs:
Before using
_.intersection()
, ensure that the input arrays are valid and contain elements.validate-inputs.jsCopiedconst array1 = [1, 2, 3, 4]; const array2 = [2, 3, 4, 5]; const array3 = []; if (!Array.isArray(array1) || !Array.isArray(array2) || !Array.isArray(array3)) { console.error('Invalid input arrays'); return; } const commonElements = _.intersection(array1, array2, array3); console.log(commonElements); // Output: []
Empty Array Handling:
Consider cases where one or more arrays may be empty. Ensure your code accounts for such scenarios.
empty-array-handling.jsCopiedconst array1 = [1, 2, 3, 4]; const array2 = []; const array3 = [3, 4, 5, 6]; const commonElements = _.intersection(array1, array2, array3); console.log(commonElements); // Output: []
Array Length:
Be mindful of the length of the arrays involved. If one array is significantly larger than others, it may impact performance.
array-length.jsCopiedconst array1 = [/* ...large array... */]; const array2 = [/* ...small array... */]; const commonElements = _.intersection(array1, array2); console.log(commonElements);
📚 Use Cases
Filtering Common Values:
_.intersection()
is ideal for scenarios where you need to filter out values common to multiple arrays.filtering-common-values.jsCopiedconst userPreferences = ['darkMode', 'largeText', 'highContrast']; const systemPreferences = ['largeText', 'autoSave', 'darkMode']; const commonPreferences = _.intersection(userPreferences, systemPreferences); console.log(commonPreferences); // Output: ['darkMode', 'largeText']
Set Operations:
Performing set operations, such as finding the common elements between arrays, is a common use case.
set-operations.jsCopiedconst setA = [1, 2, 3, 4, 5]; const setB = [4, 5, 6, 7, 8]; const commonElements = _.intersection(setA, setB); console.log(commonElements); // Output: [4, 5]
Data Validation:
Use
_.intersection()
for data validation, ensuring that only common and expected values are present.data-validation.jsCopiedconst allowedColors = ['red', 'green', 'blue']; const userSelectedColors = ['green', 'yellow', 'blue']; const validColors = _.intersection(allowedColors, userSelectedColors); console.log(validColors); // Output: ['green', 'blue']
🎉 Conclusion
The _.intersection()
method in Lodash is a valuable asset when it comes to efficiently identifying common elements among arrays. Whether you're filtering data, performing set operations, or validating inputs, this method provides a clean and concise solution to complex array comparisons. Integrate _.intersection()
into your projects to enhance the robustness and efficiency of your array-related operations.
Explore the diverse capabilities of Lodash and unlock the potential of array manipulation with _.intersection()
!
👨💻 Join our Community:
Author
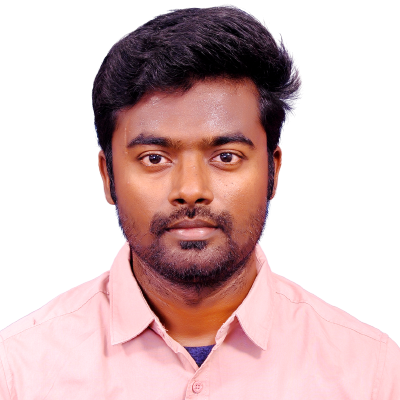
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
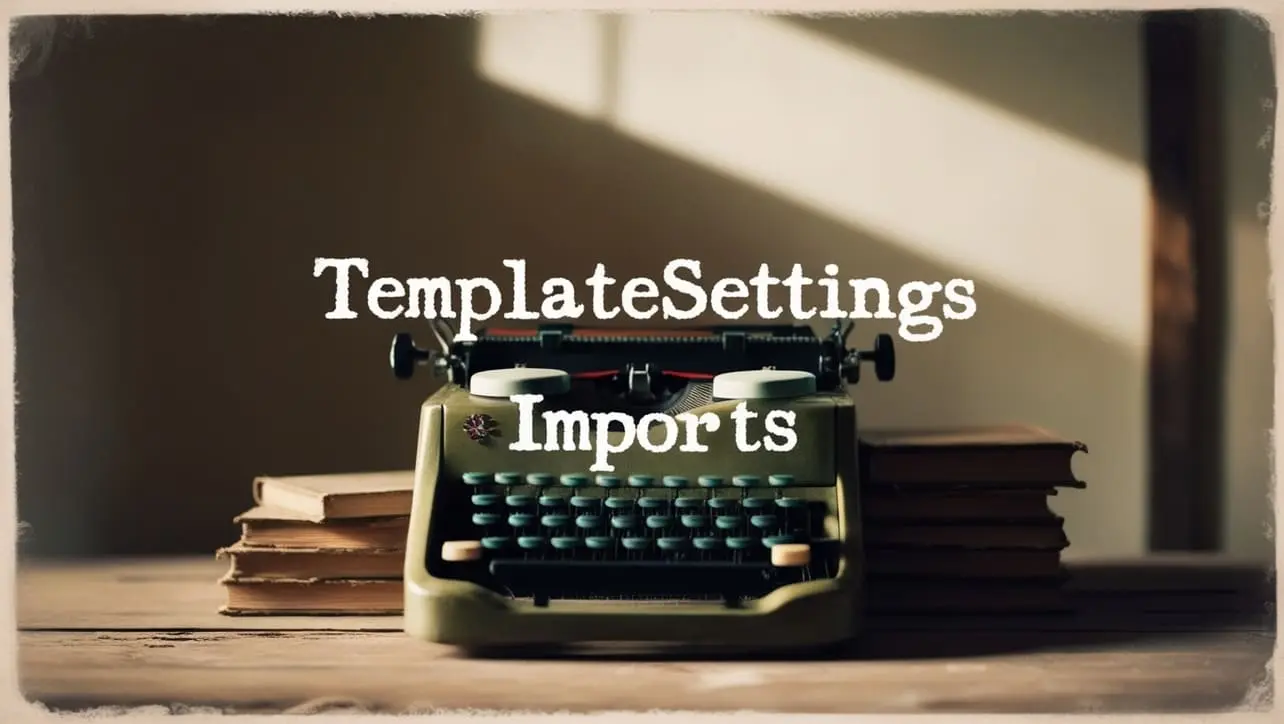
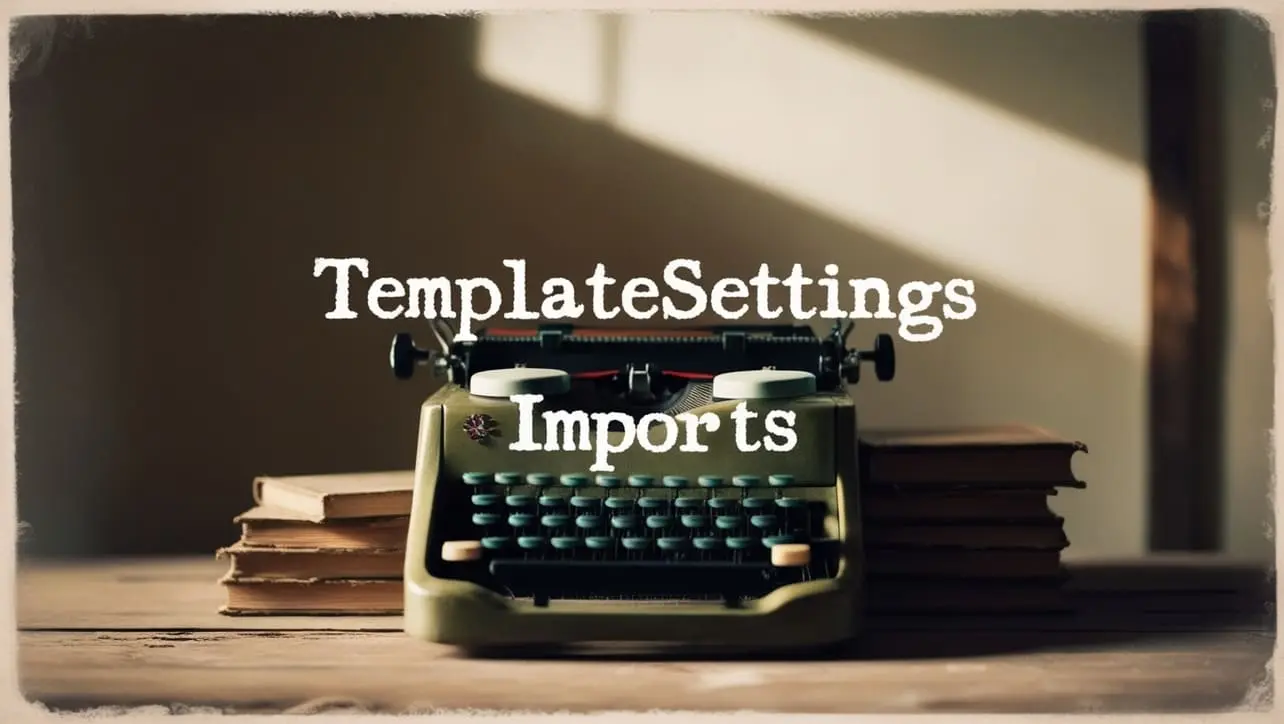
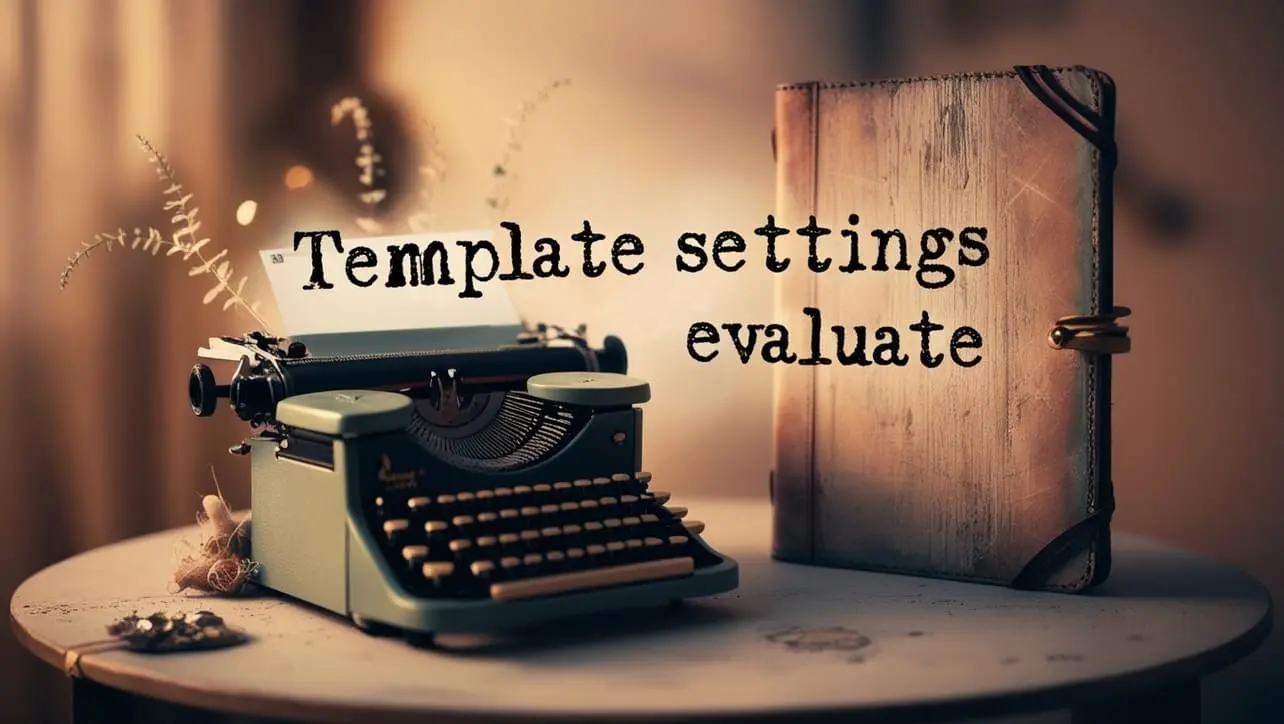
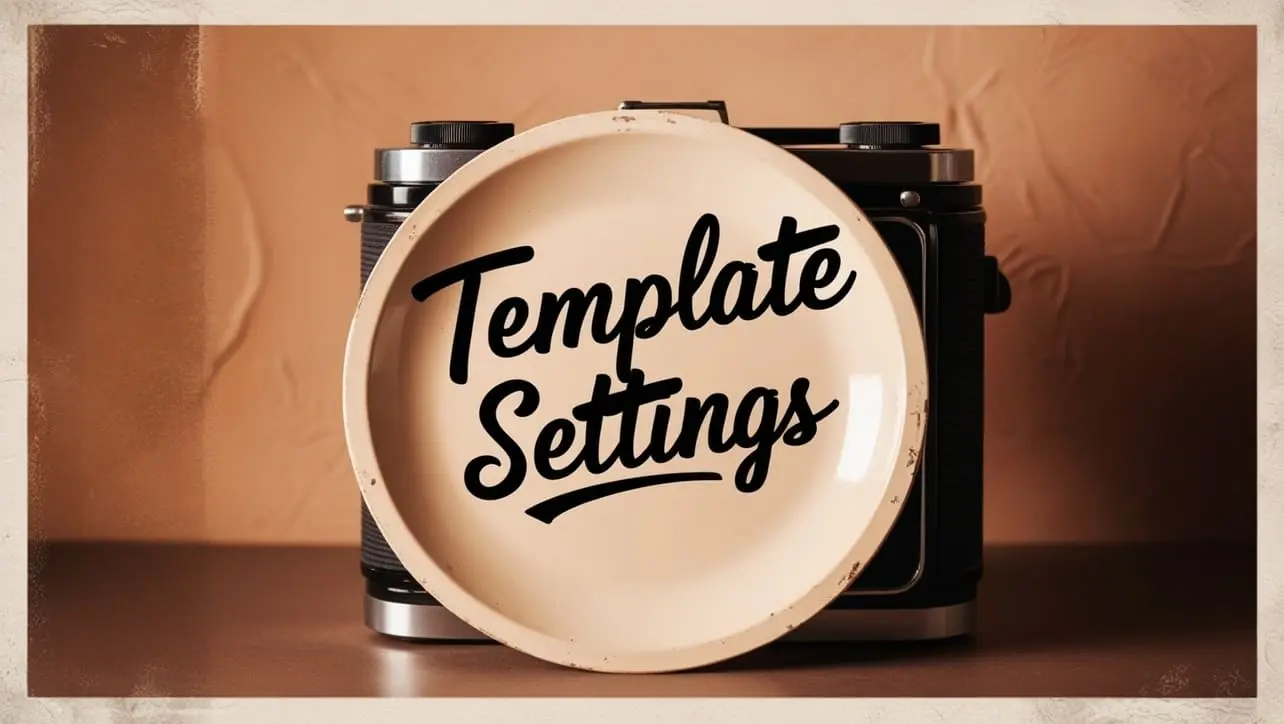
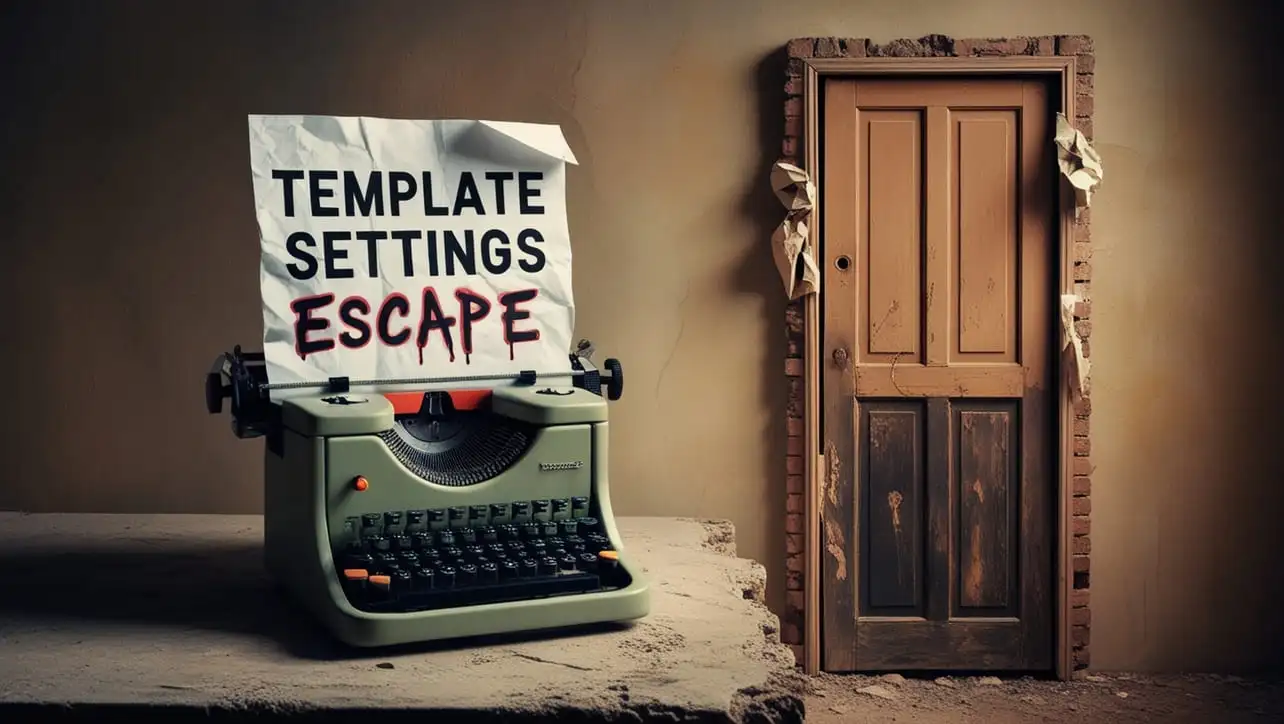
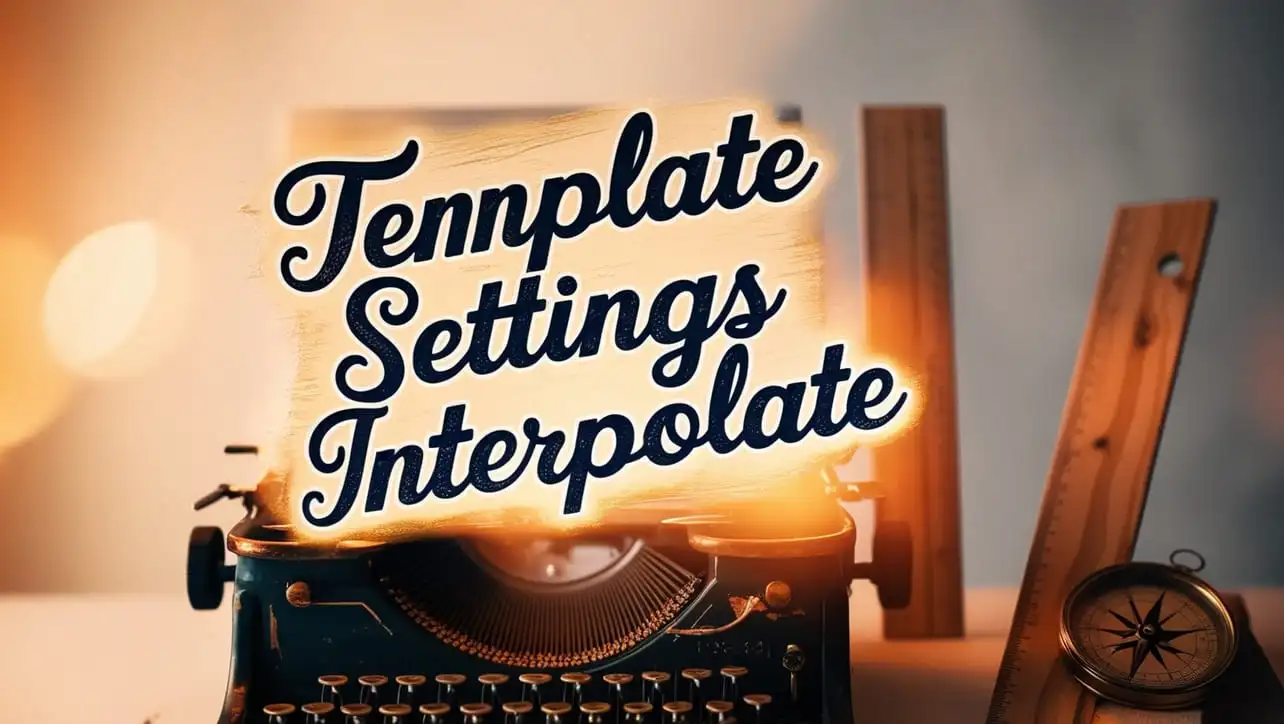
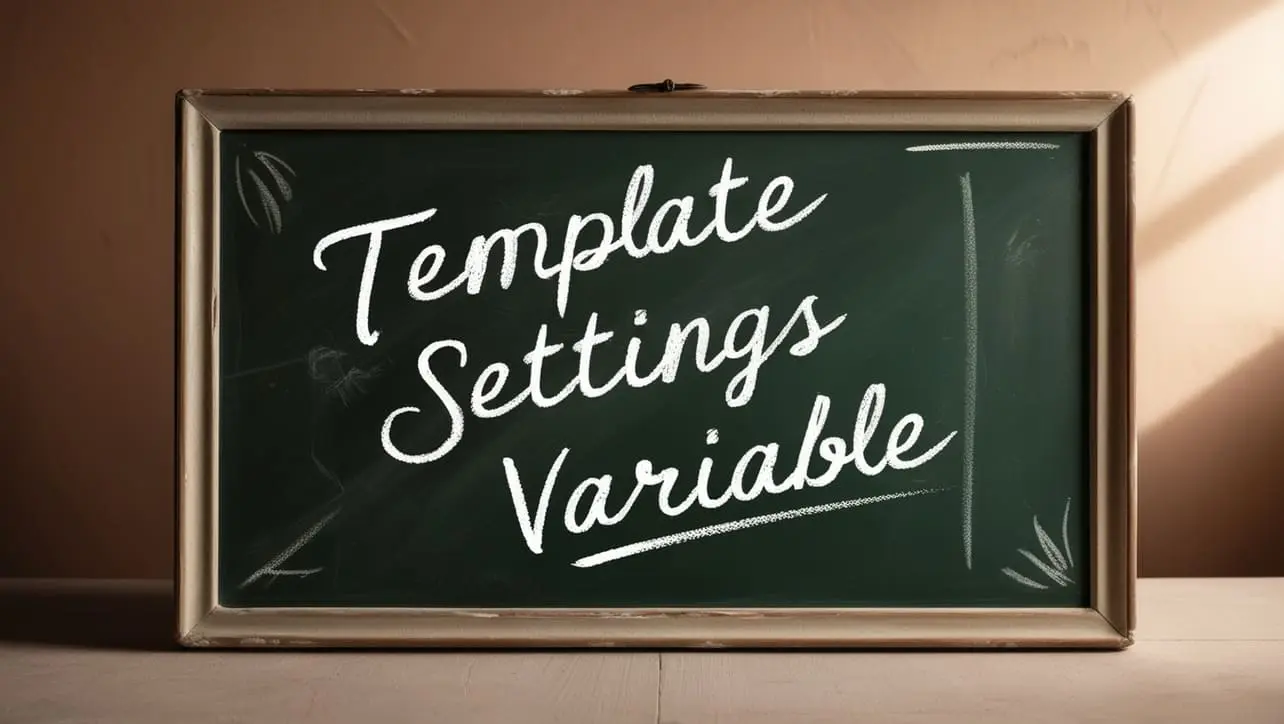
If you have any doubts regarding this article (Lodash _.intersection() Array Method), please comment here. I will help you immediately.