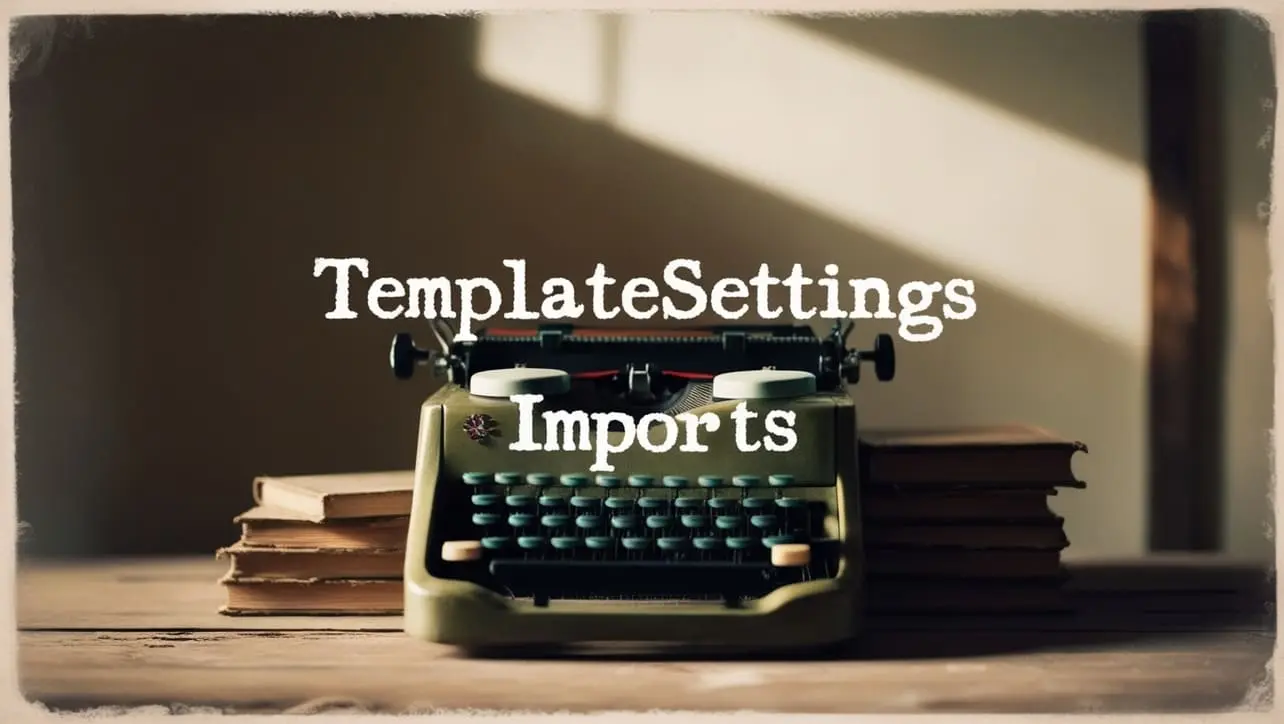
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.indexOf() Array Method
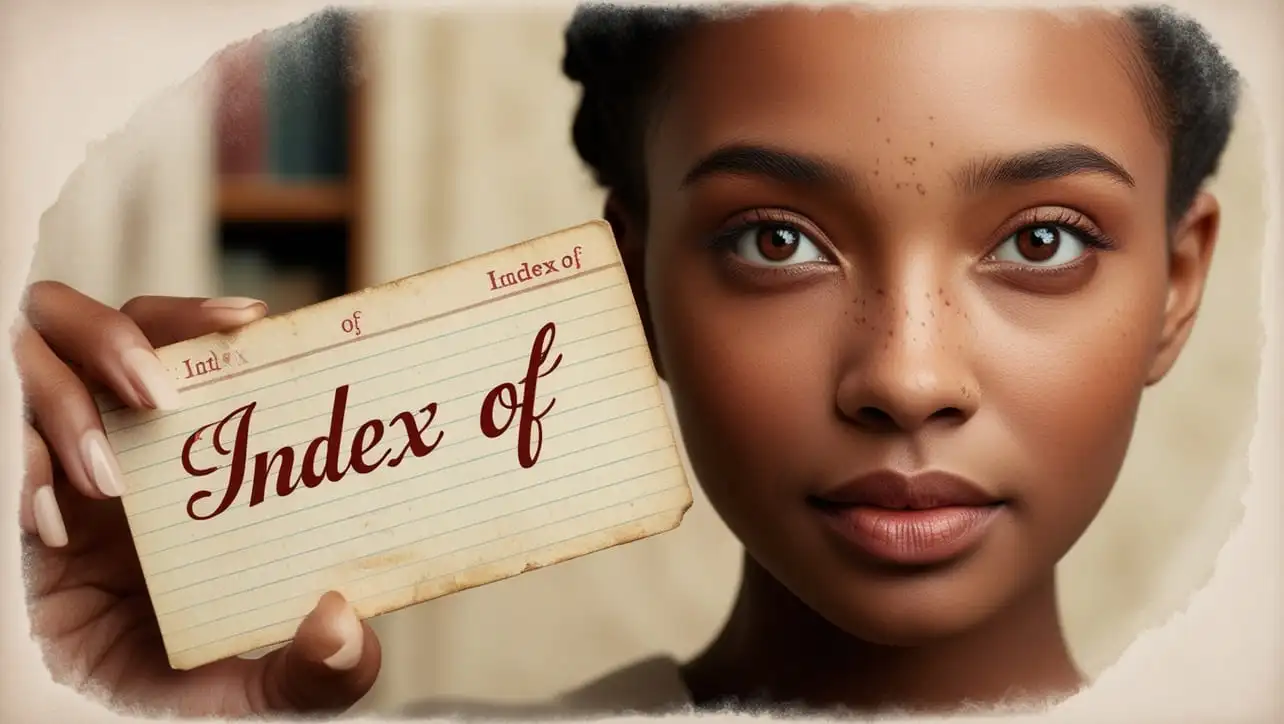
Photo Credit to CodeToFun
🙋 Introduction
Working with arrays in JavaScript often requires efficient ways to search for elements. The Lodash library offers a powerful utility method for this purpose: _.indexOf()
.
This method simplifies the process of finding the index of a specified element in an array, providing a robust solution for developers dealing with complex data structures or performance-critical scenarios.
🧠 Understanding _.indexOf()
The _.indexOf()
method in Lodash allows you to find the index of a given element in an array. This can be particularly useful when you need to determine the position of an item or check for its existence within the array.
💡 Syntax
_.indexOf(array, value, [fromIndex=0])
- array: The array to search.
- value: The value to search for.
- fromIndex: The index to start the search from (default is 0).
📝 Example
Let's delve into a practical example to illustrate the power of _.indexOf()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sampleArray = [10, 20, 30, 40, 50];
const indexOfValue = _.indexOf(sampleArray, 30);
console.log(indexOfValue);
// Output: 2
In this example, _.indexOf()
is used to find the index of the value 30 in the sampleArray, resulting in the output 2.
🏆 Best Practices
Handle Not Found Scenario:
When using
_.indexOf()
, be mindful that if the specified value is not present in the array, the method returns -1. Therefore, it's essential to handle scenarios where the element is not found.handle-not-found-scenario.jsCopiedconst valueToFind = 60; const indexOfValue = _.indexOf(sampleArray, valueToFind); if (indexOfValue !== -1) { console.log(`Index of ${valueToFind}: ${indexOfValue}`); } else { console.log(`${valueToFind} not found in the array.`); }
Specify Starting Index:
Utilize the fromIndex parameter to narrow down the search. This can be beneficial when searching for multiple occurrences of an element.
specify-starting-index.jsCopiedconst repeatedValue = 30; const firstOccurrenceIndex = _.indexOf(sampleArray, repeatedValue); const secondOccurrenceIndex = _.indexOf(sampleArray, repeatedValue, firstOccurrenceIndex + 1); console.log(`First occurrence index: ${firstOccurrenceIndex}`); console.log(`Second occurrence index: ${secondOccurrenceIndex}`);
Strict Equality Comparison:
Keep in mind that
_.indexOf()
uses strict equality (===) to compare values. Ensure that your comparison aligns with this behavior.strict-equality-comparison.jsCopiedconst stringValue = '30'; const indexOfStringValue = _.indexOf(sampleArray, stringValue); console.log(`Index of ${stringValue}: ${indexOfStringValue}`); // Output: Index of 30: -1 (strict equality not met)
📚 Use Cases
Element Existence Check:
Determine whether a specific element exists in an array using
_.indexOf()
.element-existence-check.jsCopiedconst elementToCheck = 40; const doesExist = _.indexOf(sampleArray, elementToCheck) !== -1; console.log(`Does ${elementToCheck} exist? ${doesExist}`);
Custom Search Functions:
Combine
_.indexOf()
with custom search logic to create specialized search functions tailored to your needs.custom-search-functions.jsCopiedconst customSearchFunction = (array, searchTerm) => { const index = _.indexOf(array, searchTerm); return index !== -1 ? `Found at index ${index}` : 'Not found'; }; console.log(customSearchFunction(sampleArray, 40));
🎉 Conclusion
The _.indexOf()
method in Lodash is a valuable tool for JavaScript developers seeking efficient ways to search for elements in arrays. Whether you're checking for the existence of an element, finding its position, or implementing custom search logic, _.indexOf()
provides a versatile solution.
Explore the capabilities of Lodash and streamline your array search operations with _.indexOf()
!
👨💻 Join our Community:
Author
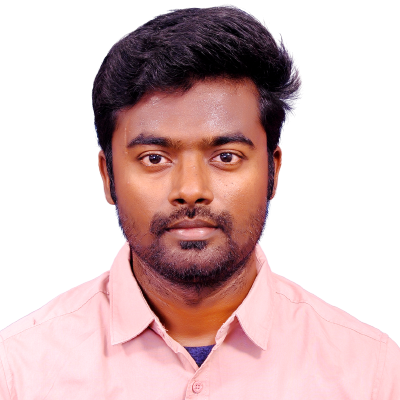
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
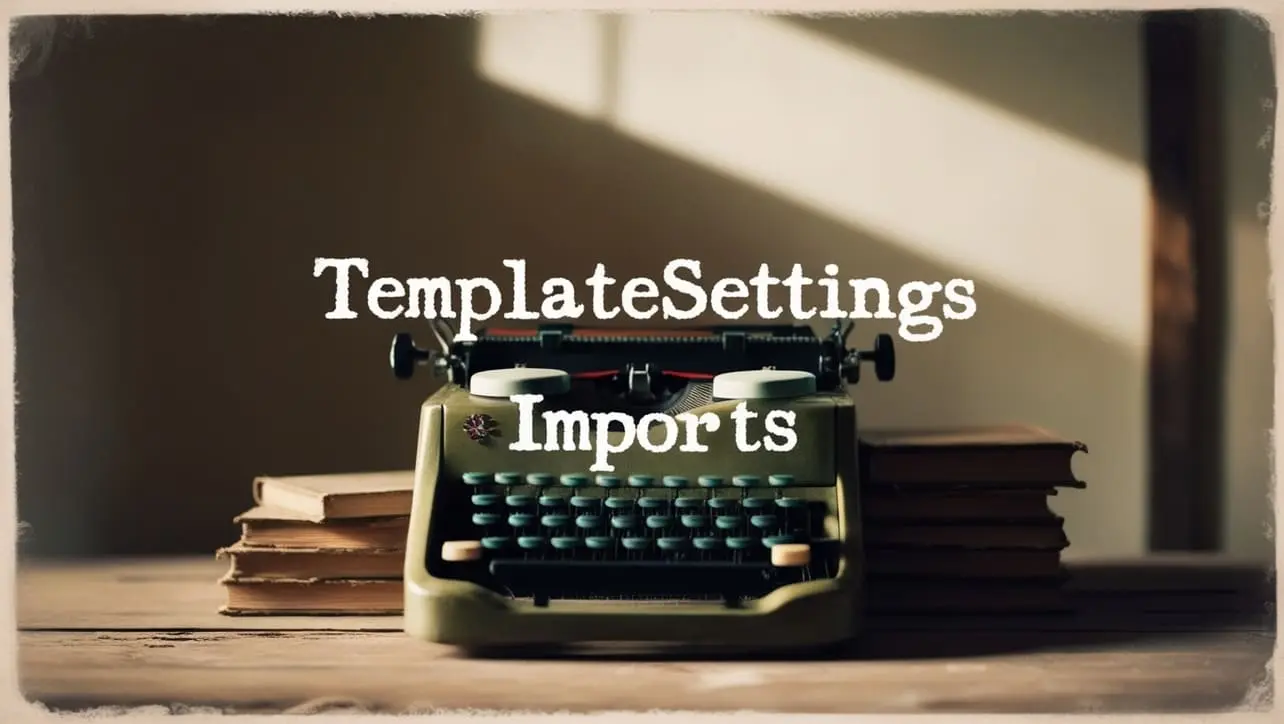
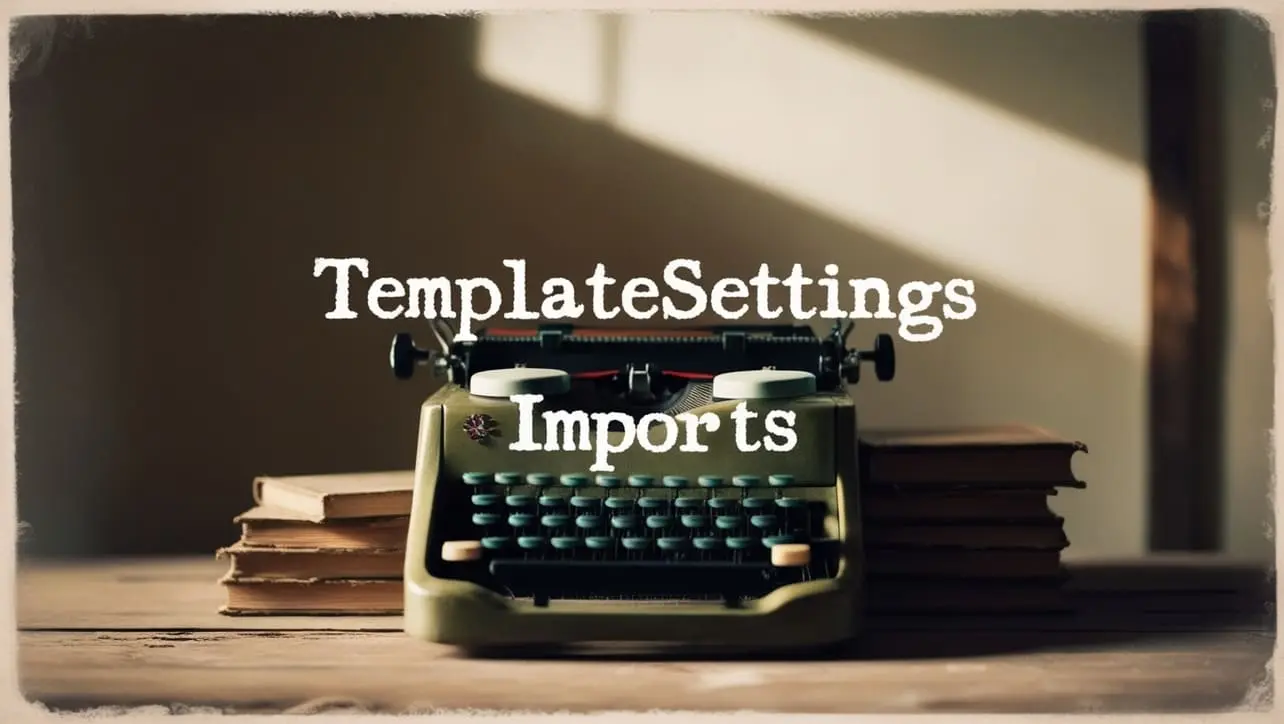
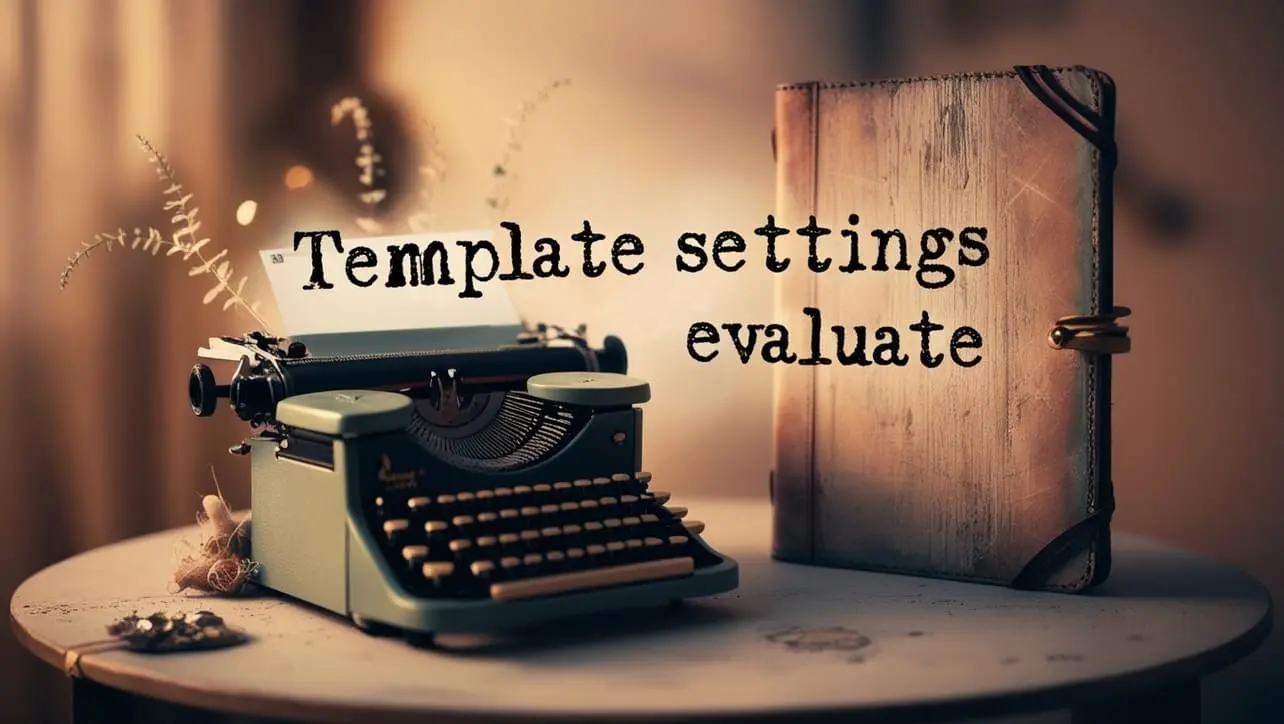
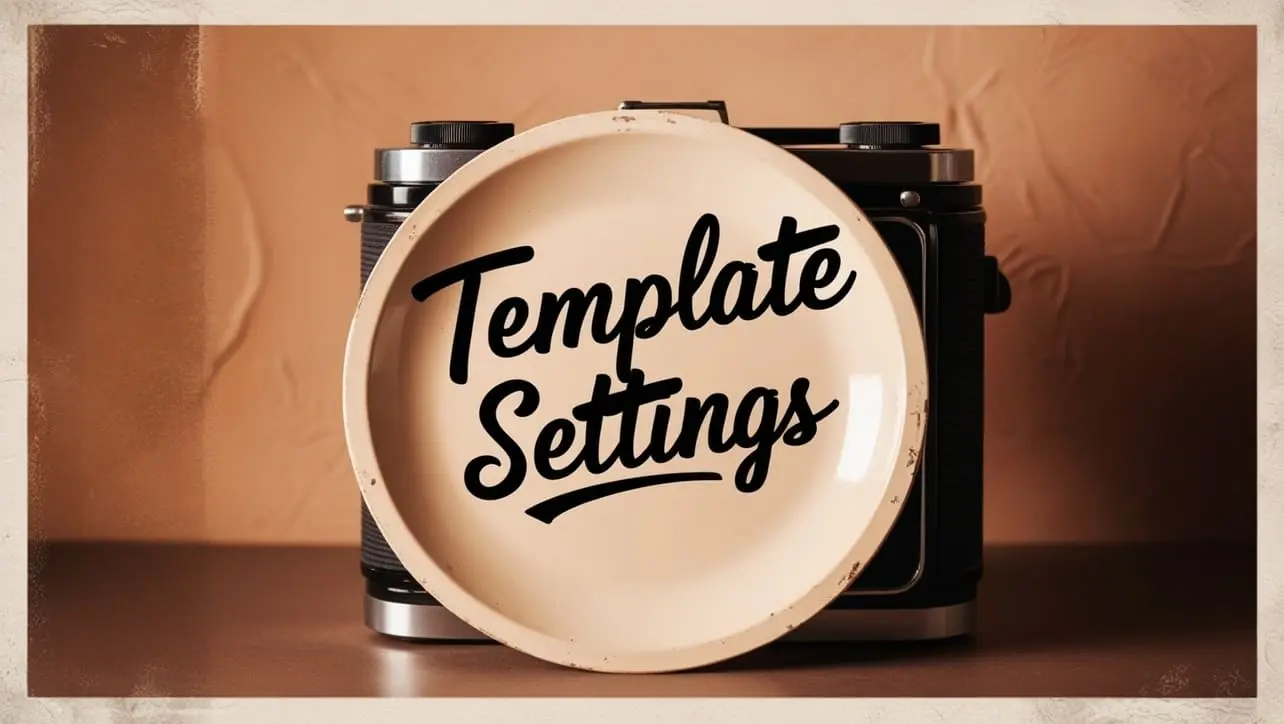
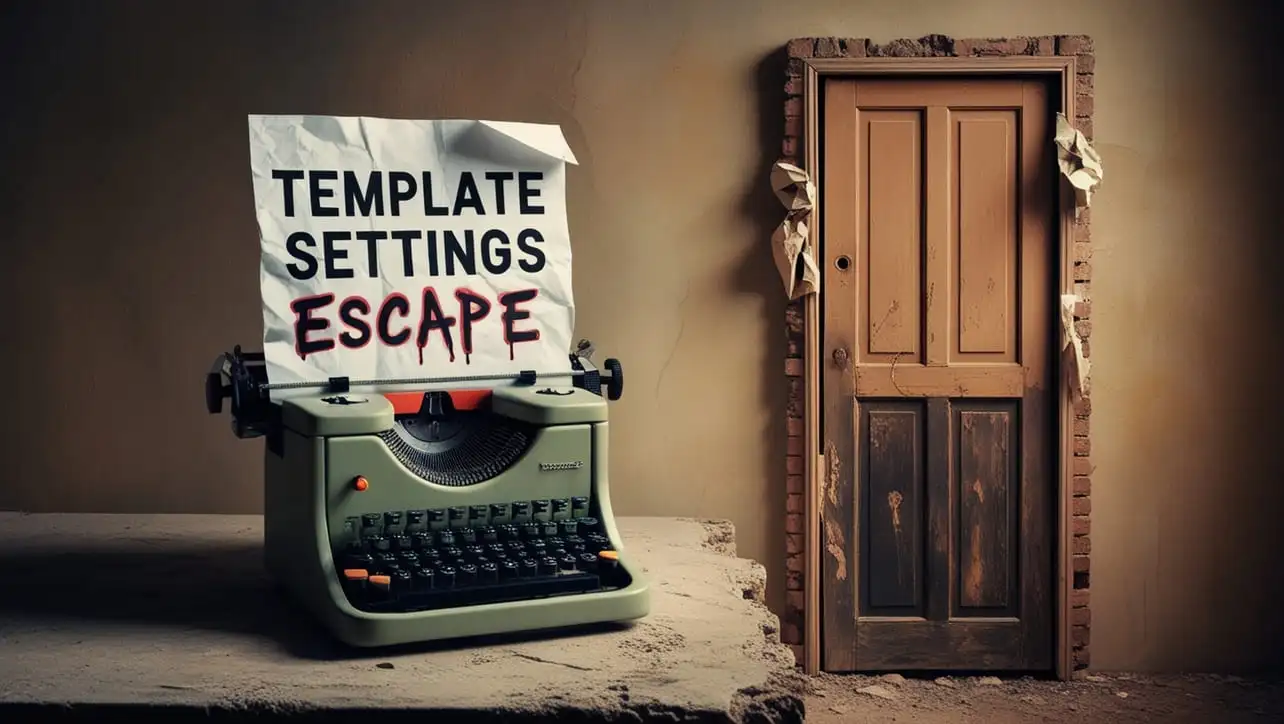
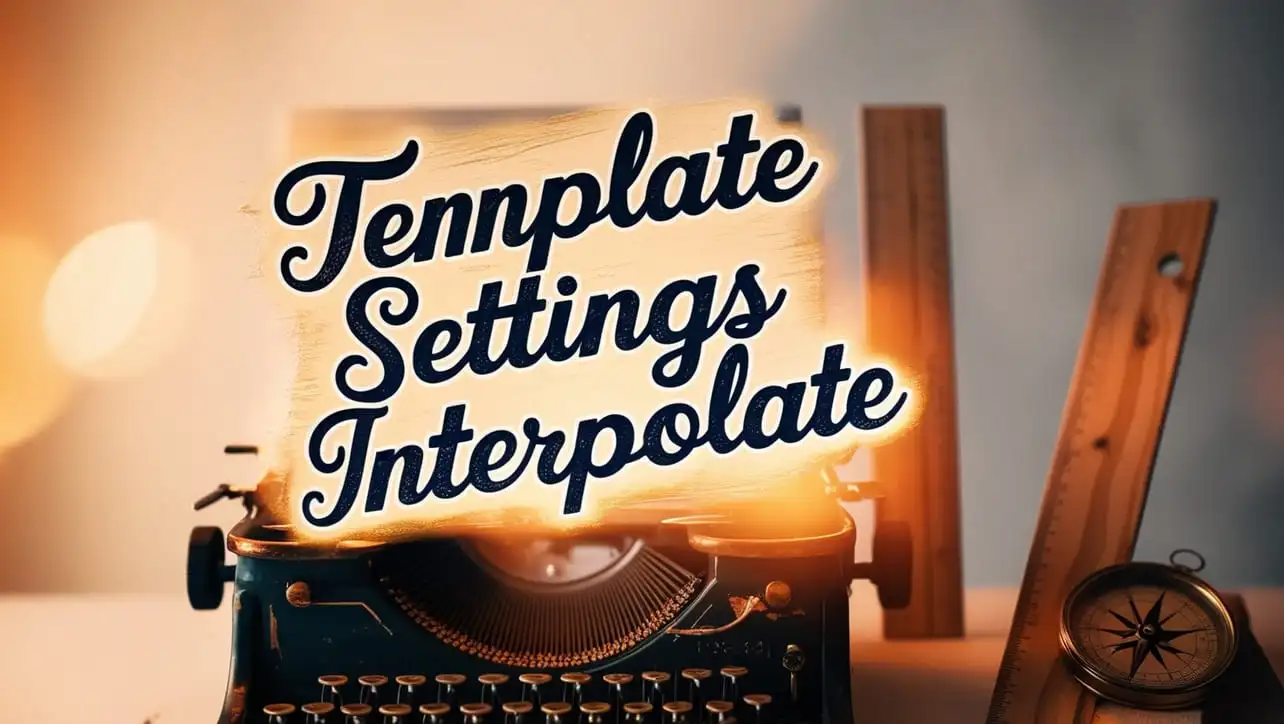
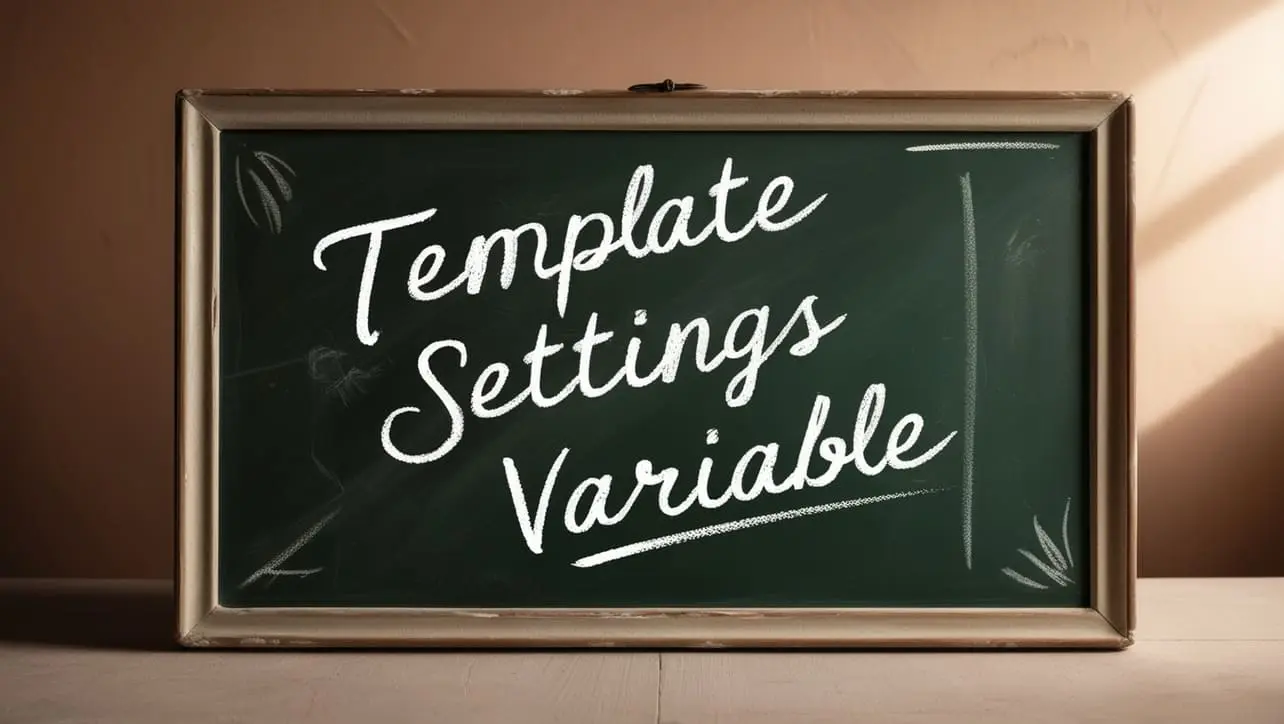
If you have any doubts regarding this article (Lodash _.indexOf() Array Method), please comment here. I will help you immediately.