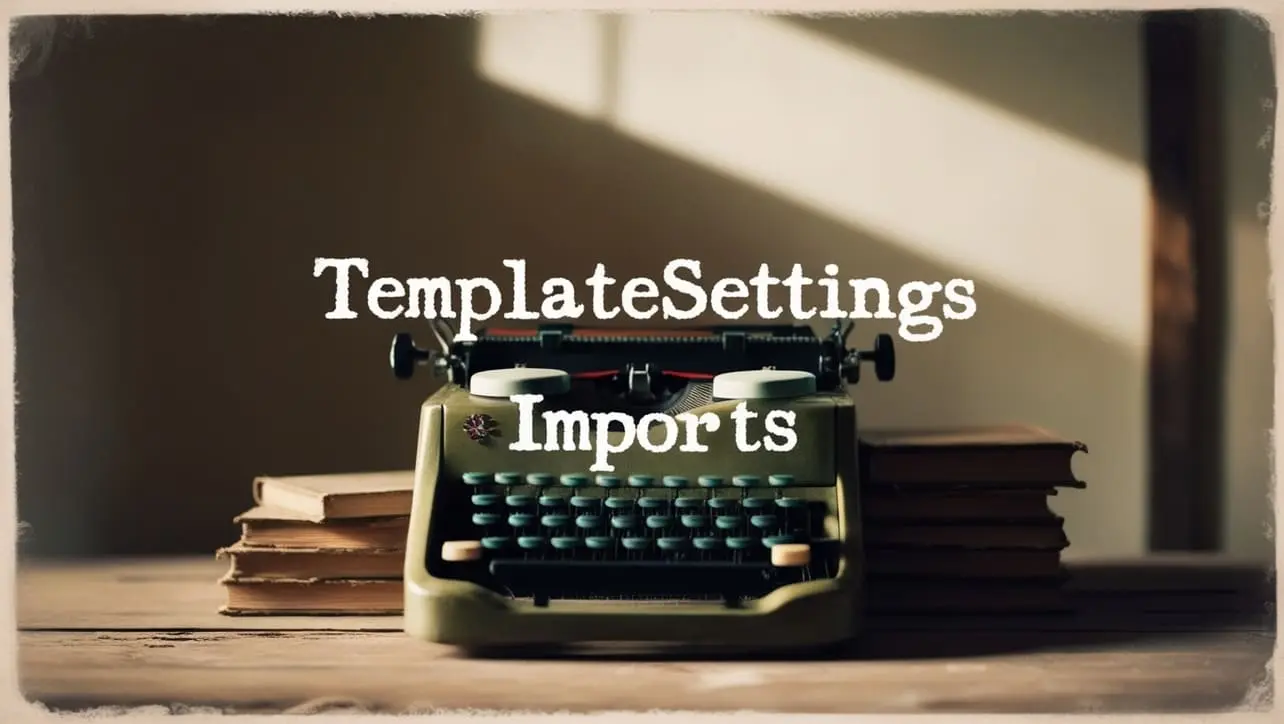
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.head() Array Method
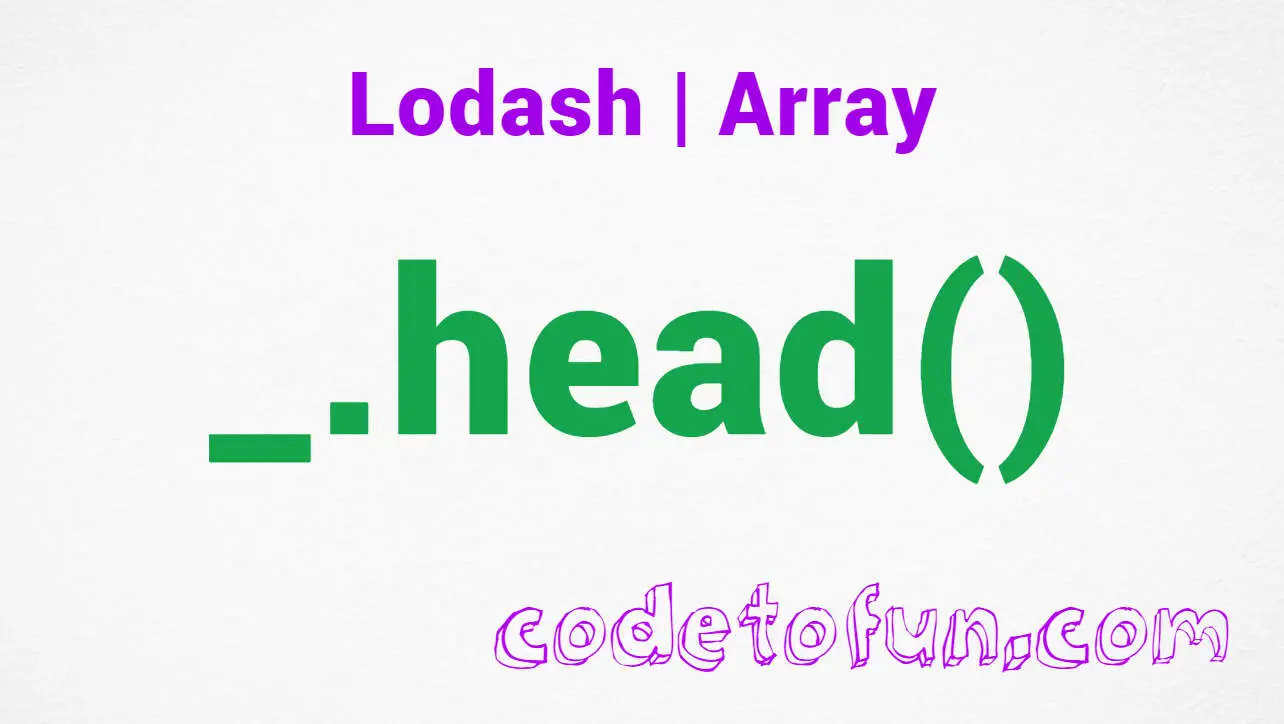
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, efficient array manipulation is essential. Lodash, a widely-used utility library, provides a range of functions to streamline these tasks. One such handy method is _.head()
, designed to retrieve the first element of an array.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of _.head()
.
🧠 Understanding _.head()
The _.head()
method in Lodash is a simple yet powerful function that extracts the first element from an array. Whether you're working with large datasets or need a quick way to access the initial element, _.head()
proves to be a valuable tool.
💡 Syntax
_.head(array)
- array: The array from which to retrieve the first element.
📝 Example
Let's illustrate the functionality of _.head()
with a practical example:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const myArray = [10, 20, 30, 40, 50];
const firstElement = _.head(myArray);
console.log(firstElement);
// Output: 10
In this example, _.head()
efficiently retrieves the first element of the myArray.
🏆 Best Practices
Check for Empty Arrays:
Before using
_.head()
, validate that the array is not empty to avoid unexpected results.check-for-empty-arrays.jsCopiedconst emptyArray = []; const firstElement = _.head(emptyArray); console.log(firstElement); // Output: undefined
Optimize for Readability:
When using
_.head()
to extract the first element, consider if it enhances or hinders code readability. In some cases, using array indexing might be more straightforward.optimize-for-readability.jsCopiedconst alternativeMethod = myArray[0]; console.log(alternativeMethod); // Output: 10
Contextual Use:
Evaluate whether using
_.head()
aligns with the overall context and purpose of your code. For simple cases, direct array access might be more appropriate.
📚 Use Cases
Quick Access to Initial Data:
When you need rapid access to the first element of an array,
_.head()
provides a concise and readable solution.access-initial-data.jsCopiedconst dataArray = /* ...fetch data from API or elsewhere... */; const firstDataItem = _.head(dataArray); console.log(firstDataItem);
Conditional Checks:
In scenarios where you need to perform actions based on the existence of the first element,
_.head()
simplifies the process.conditional-checks.jsCopiedconst checkFirstElement = (arr) => { if (_.head(arr) === 42) { console.log('The answer to everything!'); } else { console.log('Not quite there yet.'); } }; checkFirstElement([42, 1, 2]); // Output: The answer to everything!
🎉 Conclusion
The _.head()
method in Lodash is a concise and efficient way to retrieve the first element of an array. While its simplicity makes it appealing, consider the context and readability of your code when deciding to use _.head()
. Incorporate this method thoughtfully into your projects, leveraging its power for quick array access.
Explore the vast capabilities of Lodash, and make your array manipulations in JavaScript more streamlined with _.head()
!
👨💻 Join our Community:
Author
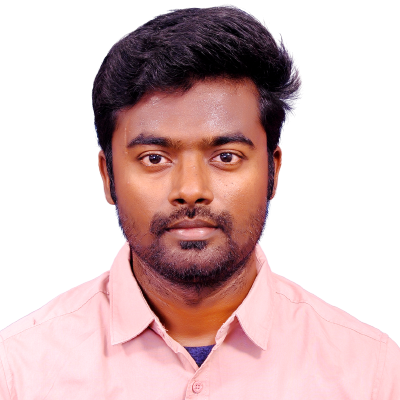
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
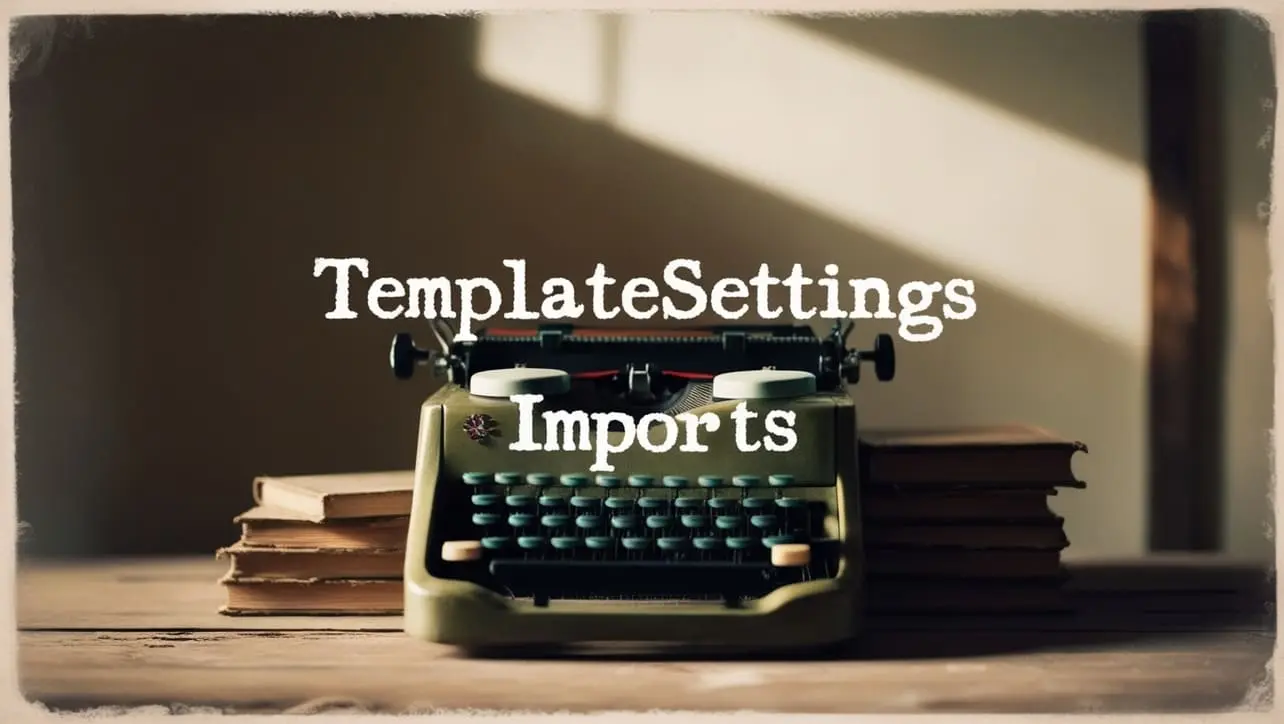
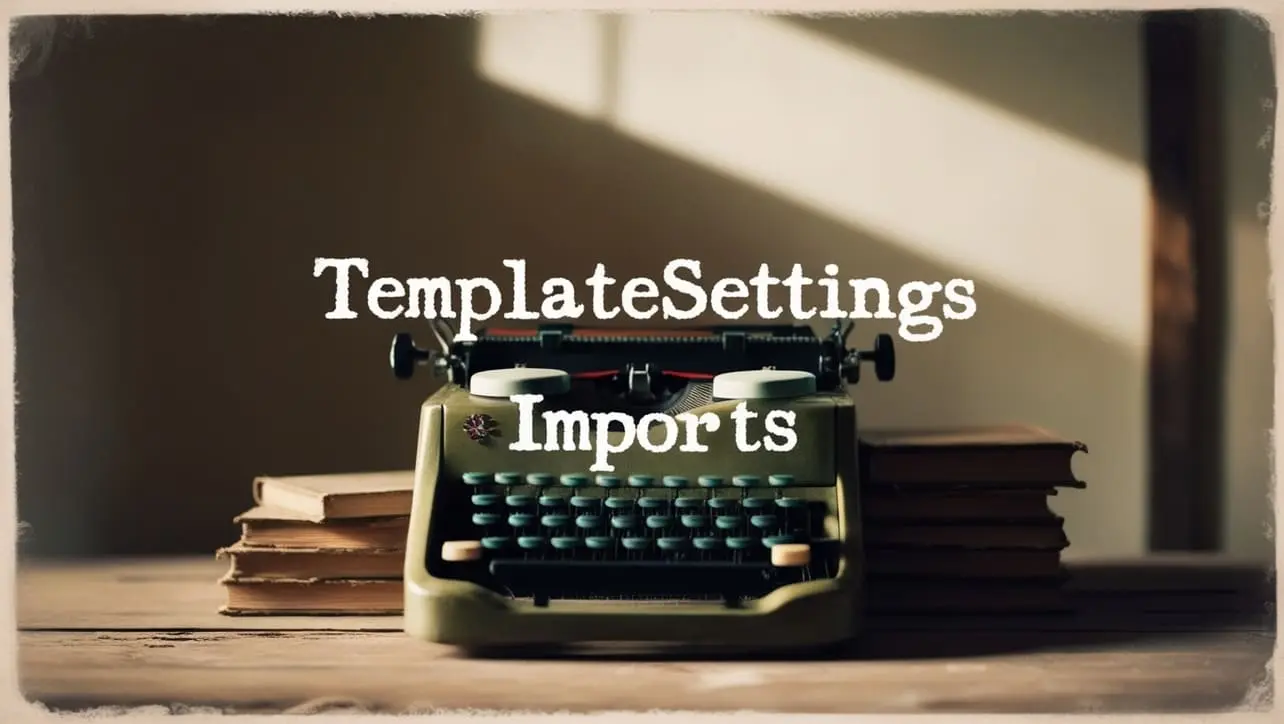
Lodash _.templateSettings.imports Property
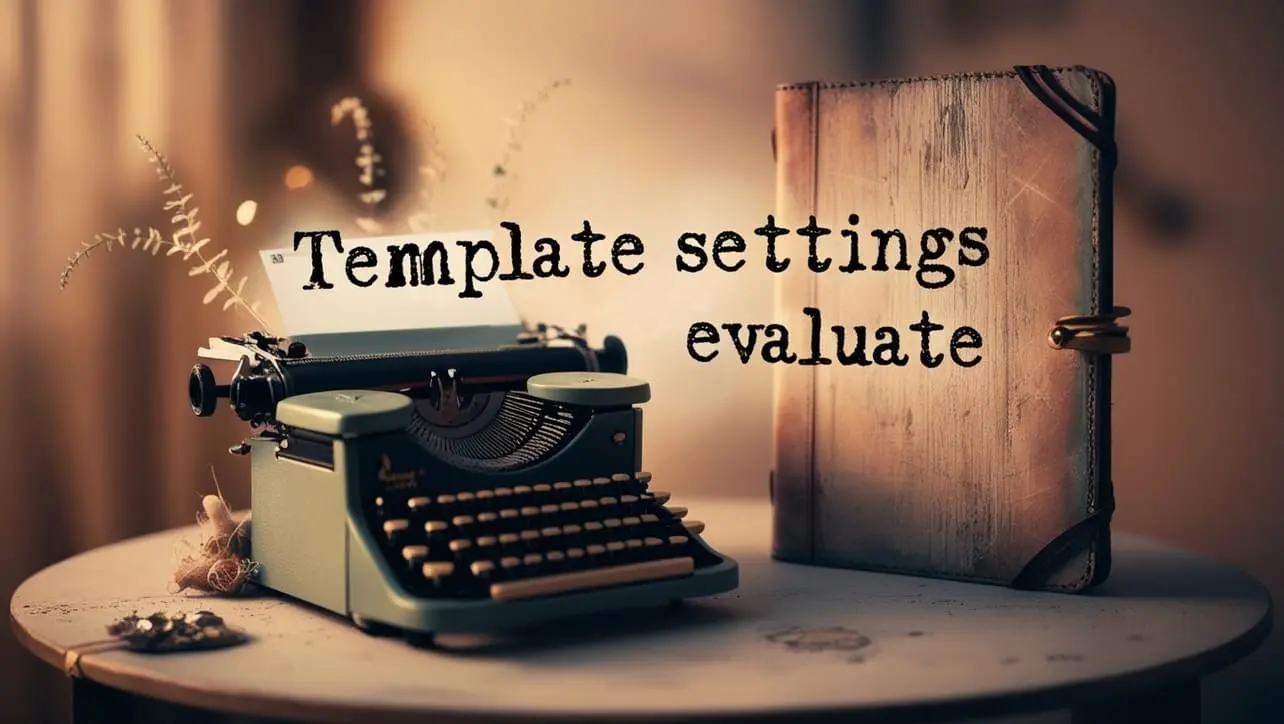
Lodash _.templateSettings.evaluate Property
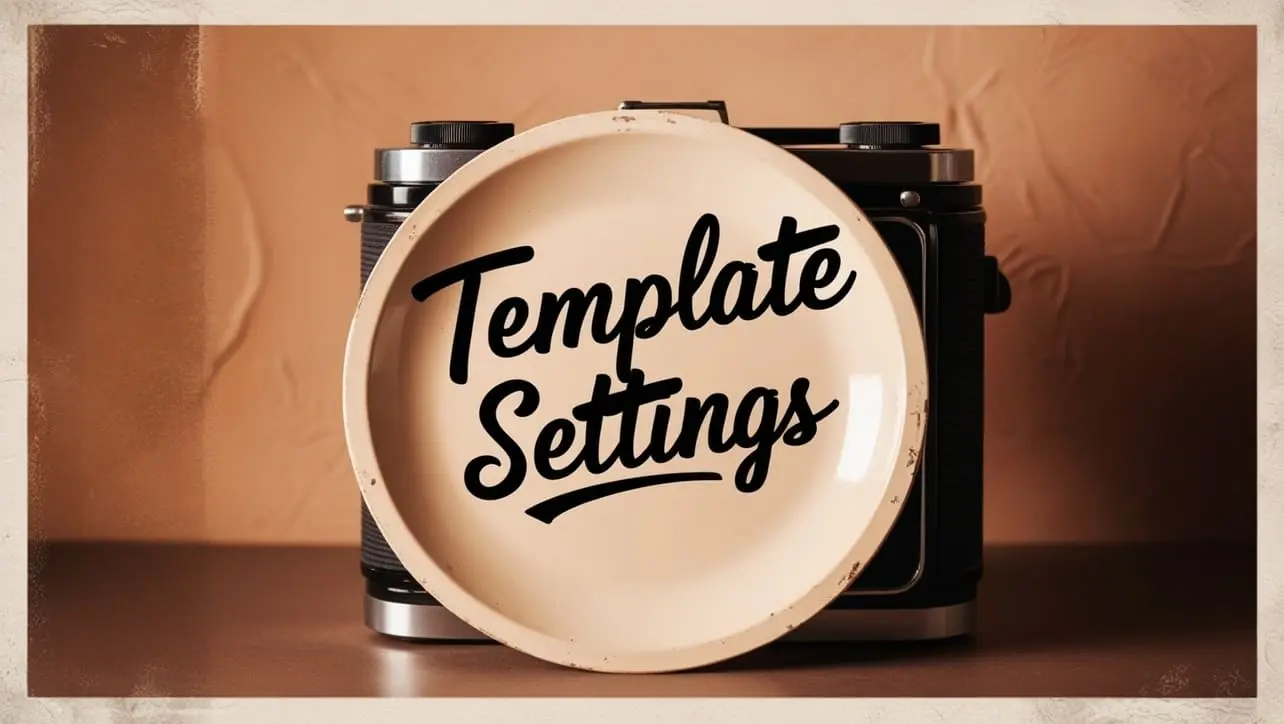
Lodash _.templateSettings Property
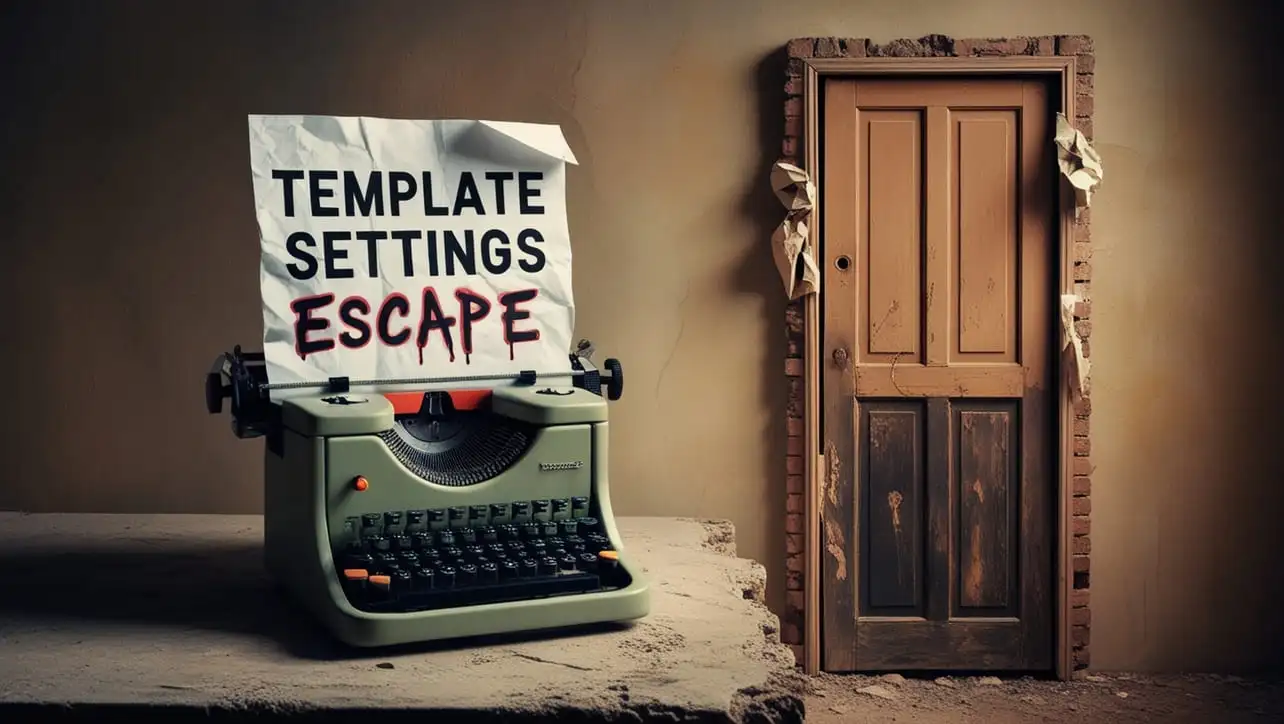
Lodash _.templateSettings.escape Property
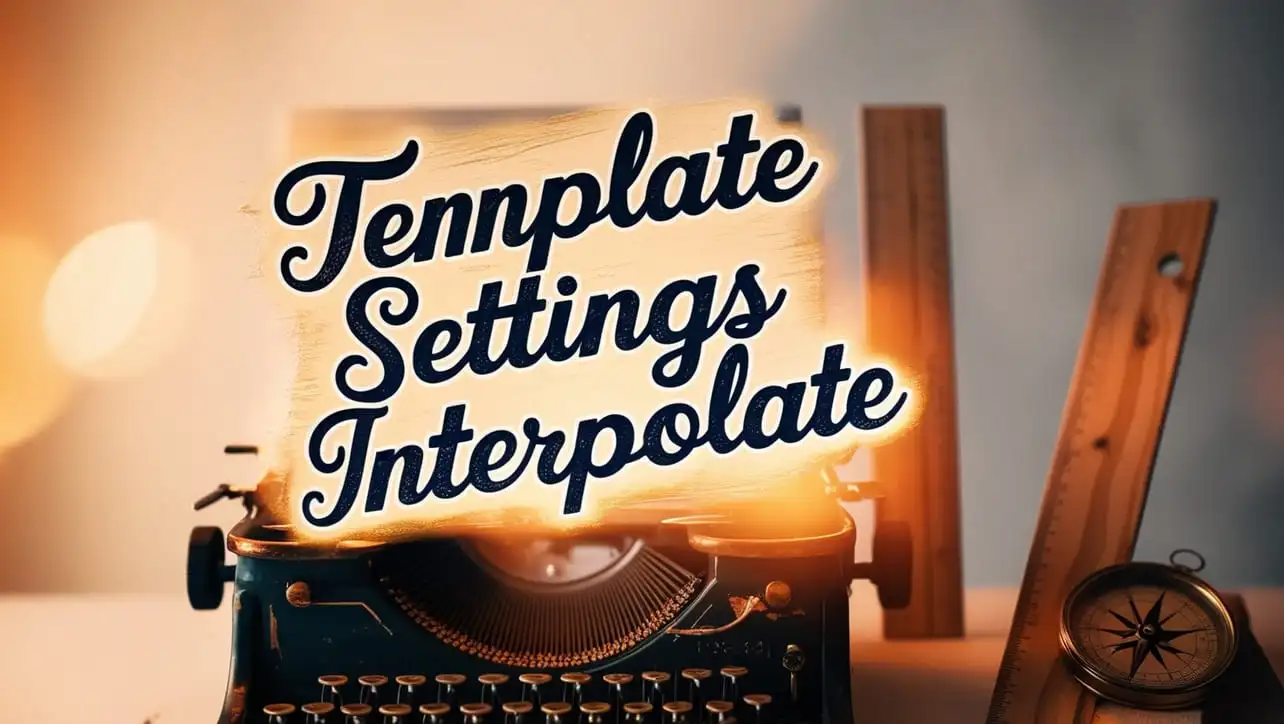
Lodash _.templateSettings.interpolate Property
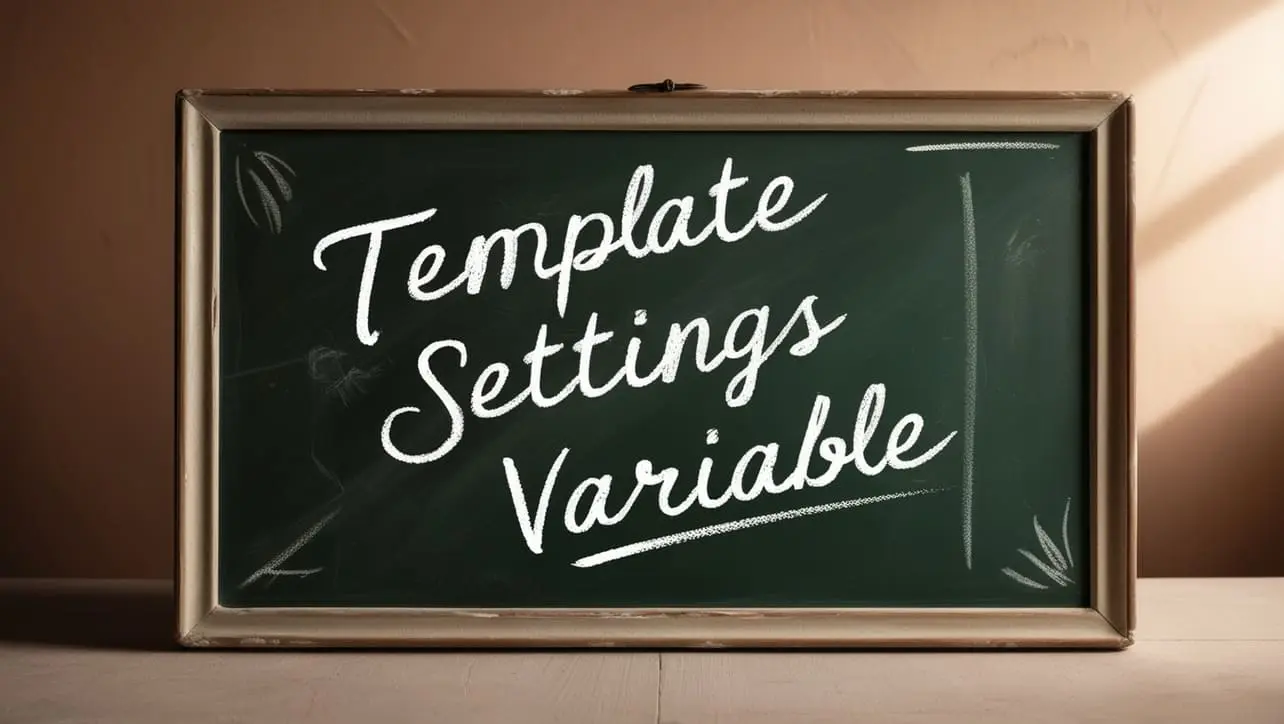
If you have any doubts regarding this article (Lodash _.head() Array Method), please comment here. I will help you immediately.