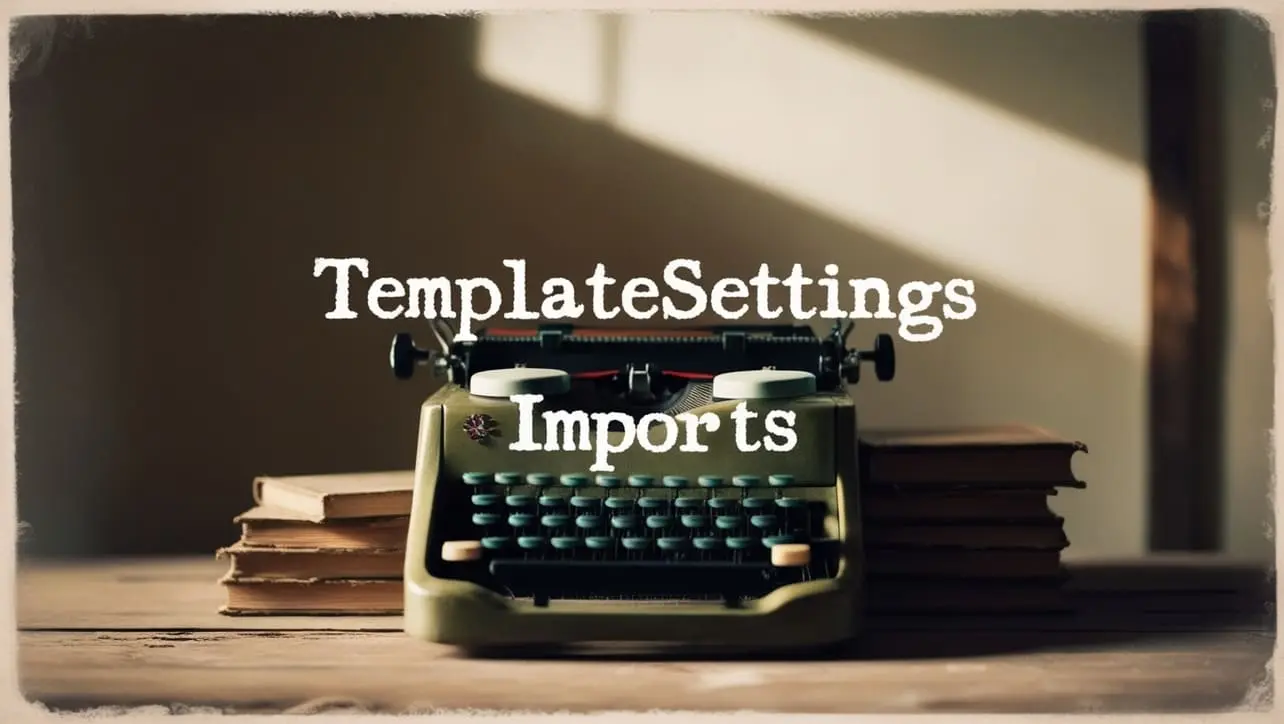
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.flattenDeep() Array Method
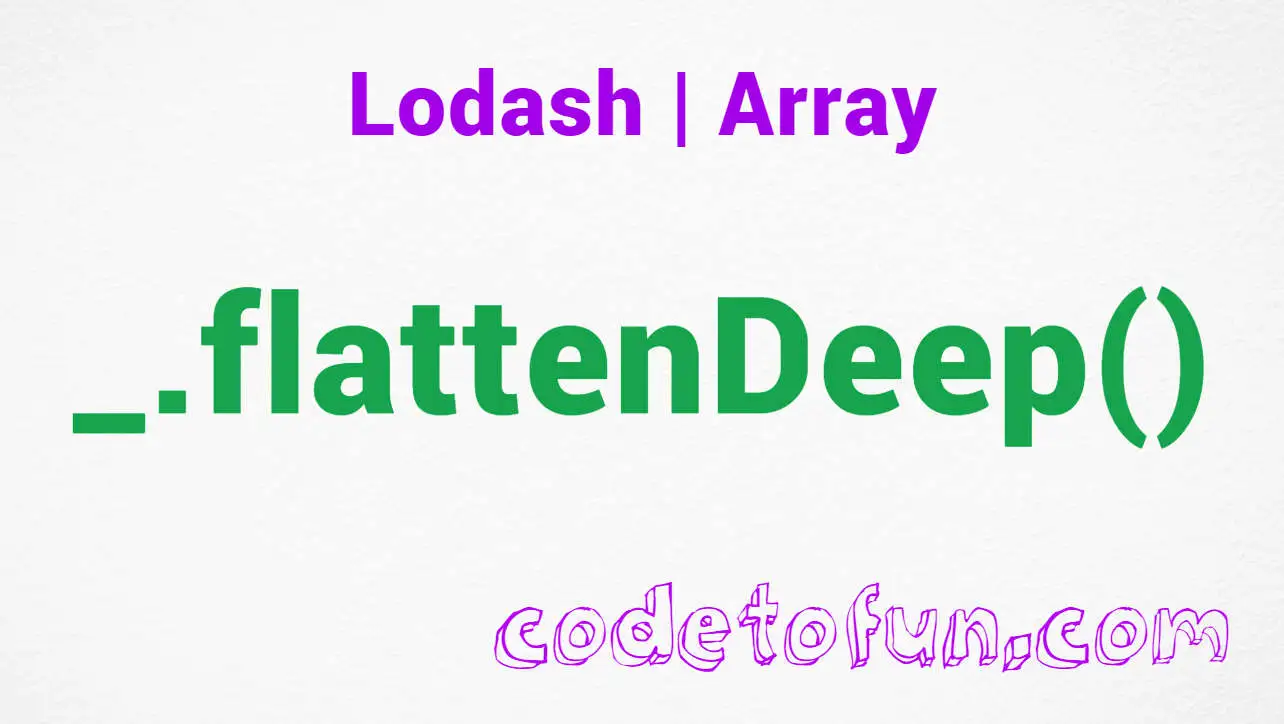
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, managing nested arrays can be challenging. The Lodash library provides a solution with the _.flattenDeep()
method.
This function simplifies the process of flattening deeply nested arrays, offering a clean and efficient way to handle complex data structures.
🧠 Understanding _.flattenDeep()
The _.flattenDeep()
method in Lodash is designed to recursively flatten arrays, no matter how deeply nested they are. This is particularly useful when dealing with data structures that involve multiple layers of arrays.
💡 Syntax
_.flattenDeep(array)
- array: The array to recursively flatten.
📝 Example
Let's delve into a practical example to illustrate the effectiveness of _.flattenDeep()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const nestedArray = [1, [2, [3, [4]], 5]];
const flattenedArray = _.flattenDeep(nestedArray);
console.log(flattenedArray);
// Output: [1, 2, 3, 4, 5]
In this example, the nestedArray contains multiple levels of nesting. The _.flattenDeep()
method is used to flatten it into a single-level array.
🏆 Best Practices
Verify Input:
Before utilizing
_.flattenDeep()
, ensure that the input is a valid array with nested structures. This helps prevent unexpected behavior and ensures the method works as intended.verify-input.jsCopiedif (!Array.isArray(nestedArray)) { console.error('Invalid input: not an array'); return; } const flattenedResult = _.flattenDeep(nestedArray); console.log(flattenedResult);
Recursive Flattening:
Understand that
_.flattenDeep()
operates recursively, flattening arrays at all levels. This behavior can be powerful when dealing with deeply nested data structures.recursive-flattening.jsCopiedconst deeplyNestedArray = [1, [2, [3, [4, [5]]]]]; const deeplyFlattenedArray = _.flattenDeep(deeplyNestedArray); console.log(deeplyFlattenedArray); // Output: [1, 2, 3, 4, 5]
Preserving Order:
_.flattenDeep()
maintains the order of elements in the flattened array. This can be crucial when working with data that relies on specific arrangements.preserving-order.jsCopiedconst complexNestedArray = [1, [2, [3, [4, [5]]]], [6, [7, [8]]]]; const orderedFlattenedArray = _.flattenDeep(complexNestedArray); console.log(orderedFlattenedArray); // Output: [1, 2, 3, 4, 5, 6, 7, 8]
📚 Use Cases
Simplifying Data Structures:
_.flattenDeep()
is particularly handy when dealing with complex data structures, simplifying arrays for easier processing and manipulation.simplifying-data-structures.jsCopiedconst complexData = /* ...fetch data from API or elsewhere... */; const flattenedData = _.flattenDeep(complexData); console.log(flattenedData);
Working with JSON:
When working with JSON data that includes deeply nested arrays,
_.flattenDeep()
can be a powerful ally.working-with-json.jsCopiedconst jsonData = /* ...fetch JSON data from API or elsewhere... */; const flattenedJson = _.flattenDeep(jsonData); console.log(flattenedJson);
Recursive Search:
If you need to perform a recursive search or analysis of nested arrays,
_.flattenDeep()
provides a convenient way to structure your data.recursive-search.jsCopiedconst nestedDataToSearch = /* ...some nested data... */; const flattenedForSearch = _.flattenDeep(nestedDataToSearch); // Perform search or analysis on the flattened array /* ... */
🎉 Conclusion
The _.flattenDeep()
method in Lodash is a valuable asset for JavaScript developers dealing with intricate data structures. Its ability to recursively flatten deeply nested arrays simplifies array manipulation and enhances code readability. By incorporating this method into your projects, you can streamline the handling of complex data and focus on the core logic of your applications.
Explore the capabilities of _.flattenDeep()
in Lodash and unlock new possibilities for managing nested arrays!
👨💻 Join our Community:
Author
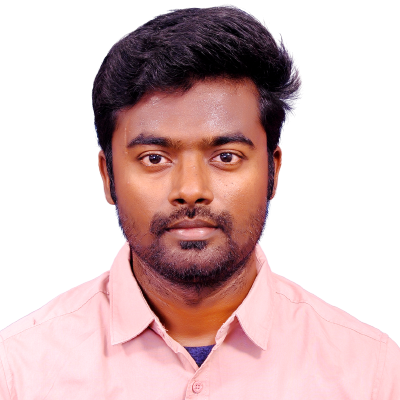
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
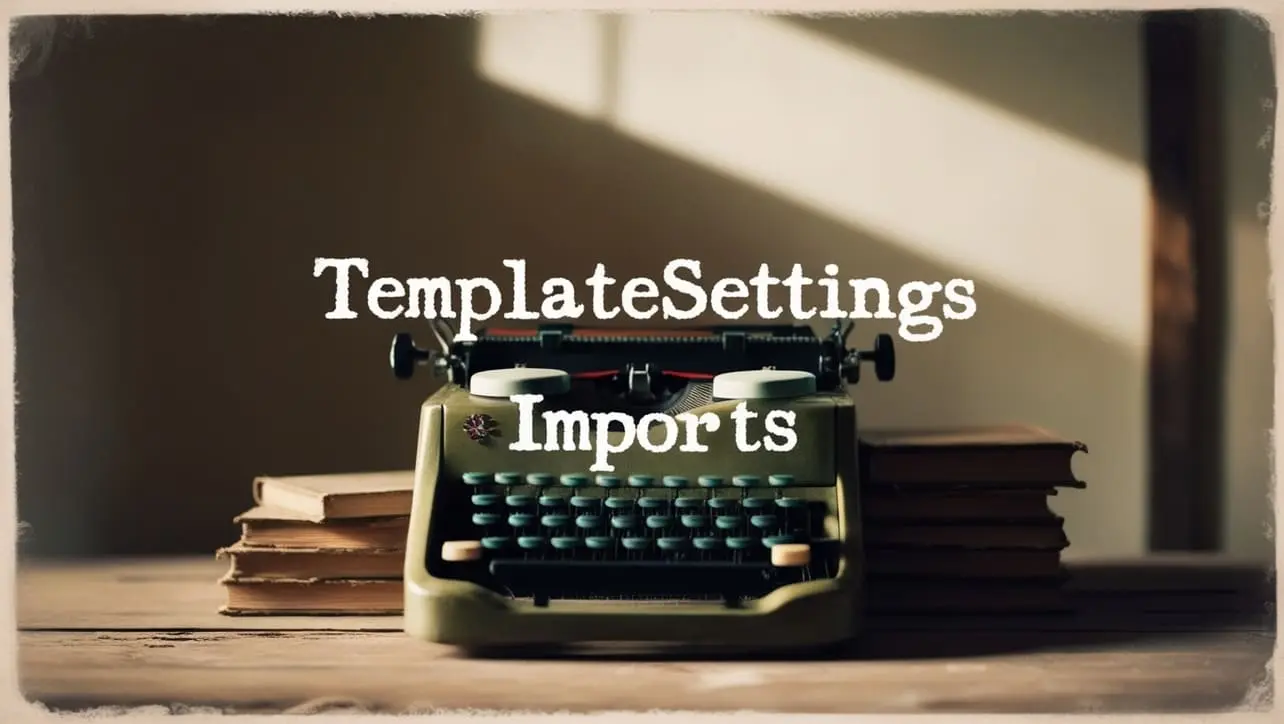
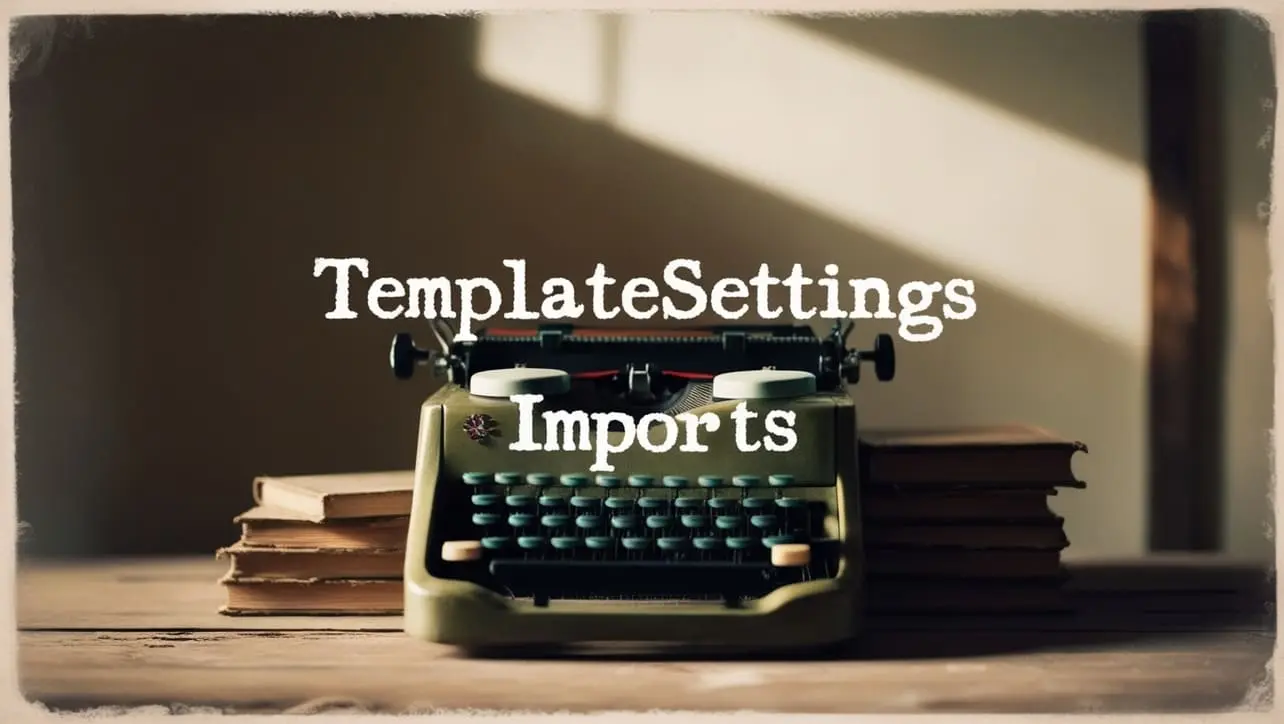
Lodash _.templateSettings.imports Property
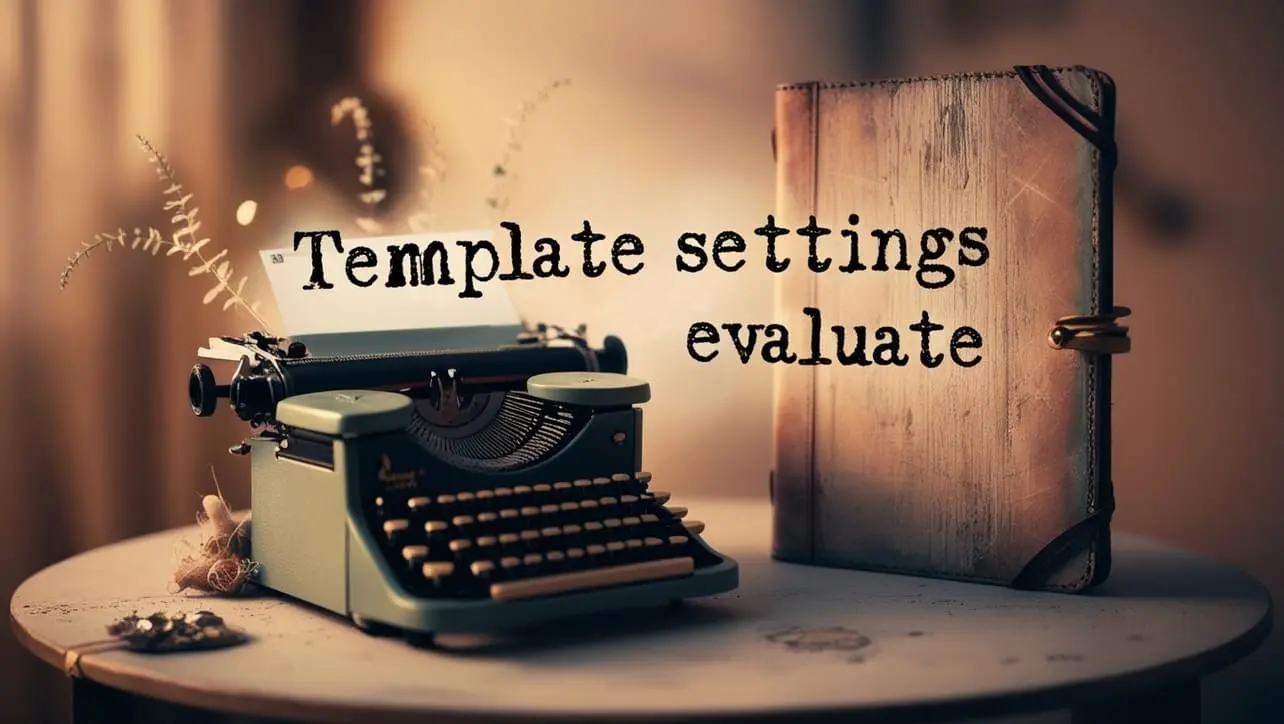
Lodash _.templateSettings.evaluate Property
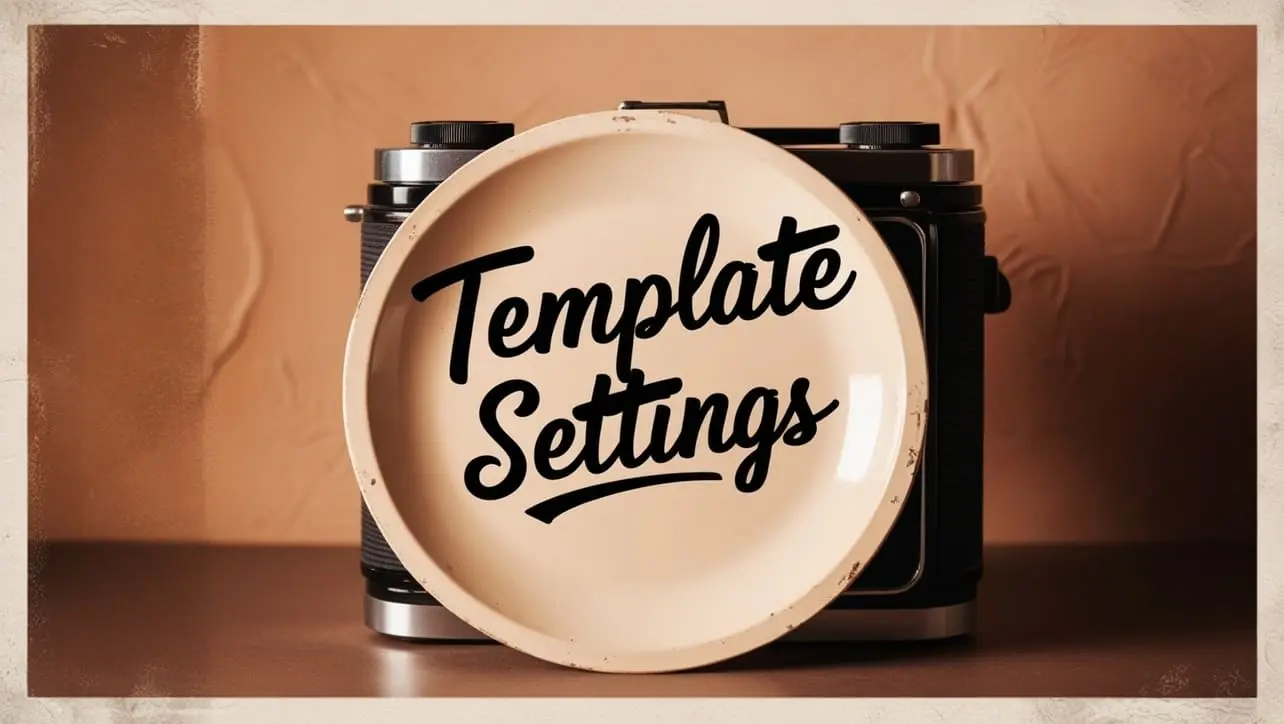
Lodash _.templateSettings Property
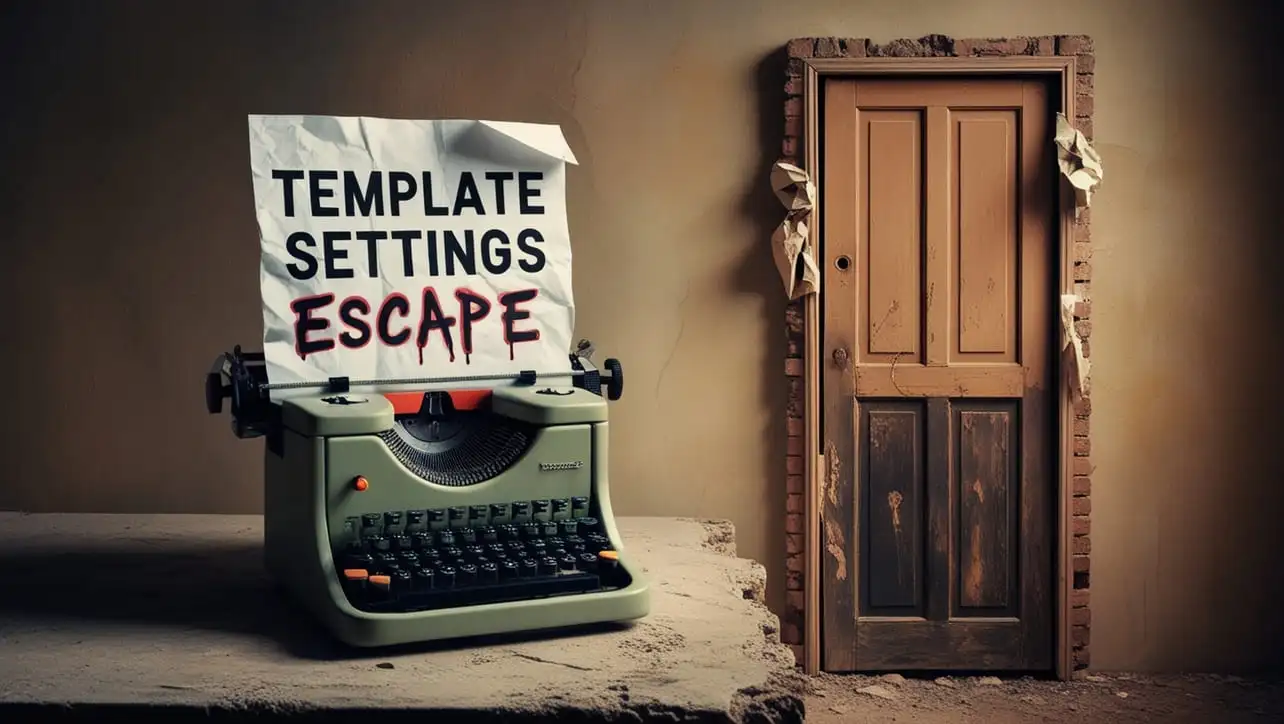
Lodash _.templateSettings.escape Property
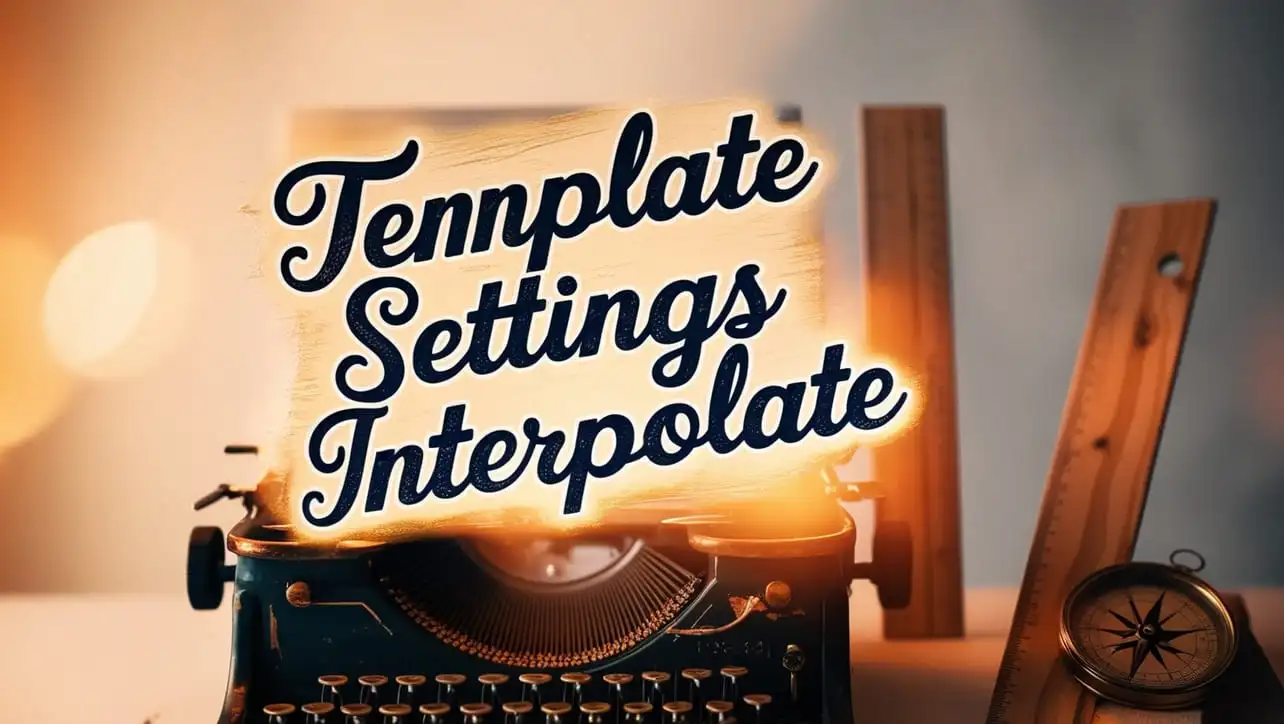
Lodash _.templateSettings.interpolate Property
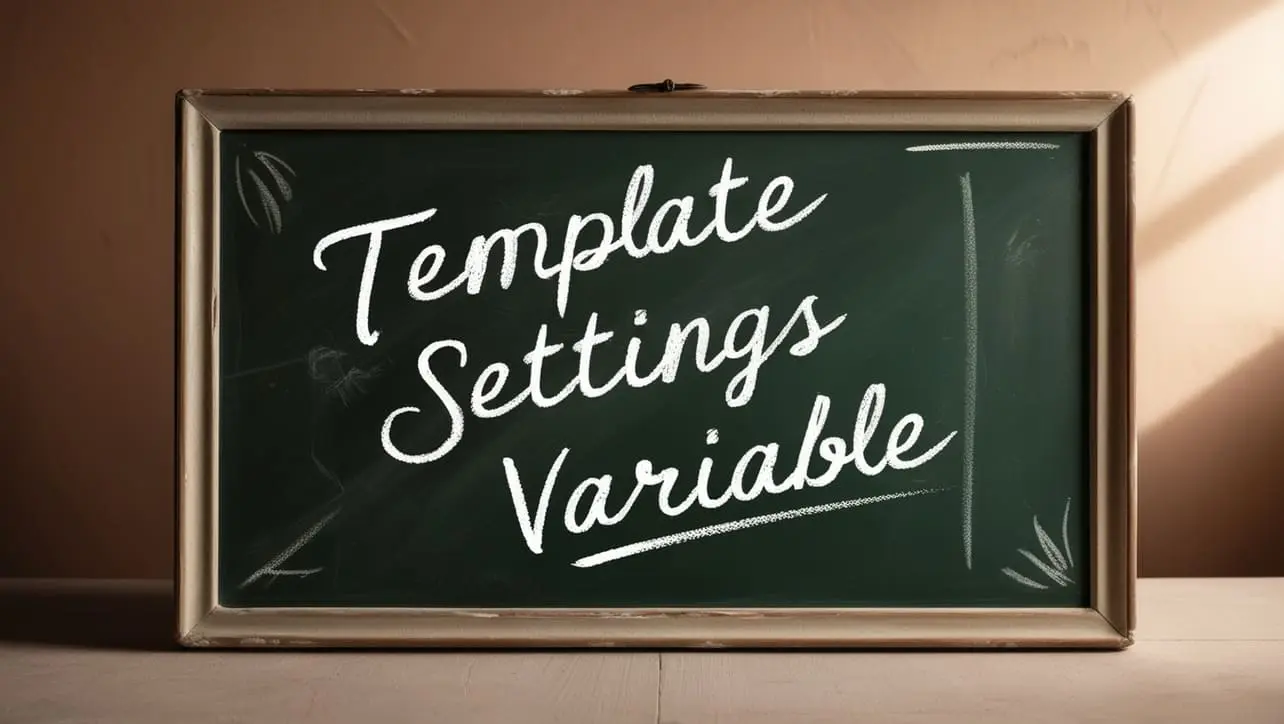
If you have any doubts regarding this article (Lodash _.flattenDeep() Array Method), please comment here. I will help you immediately.