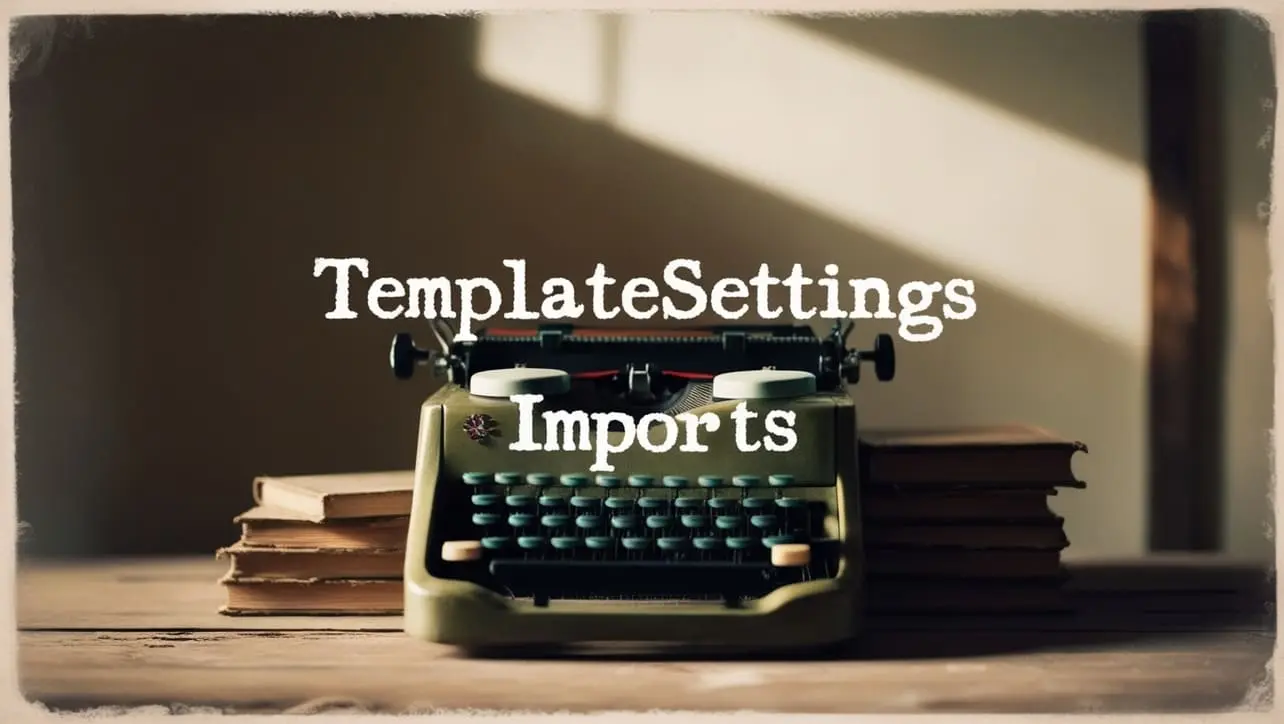
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.difference() Array Method
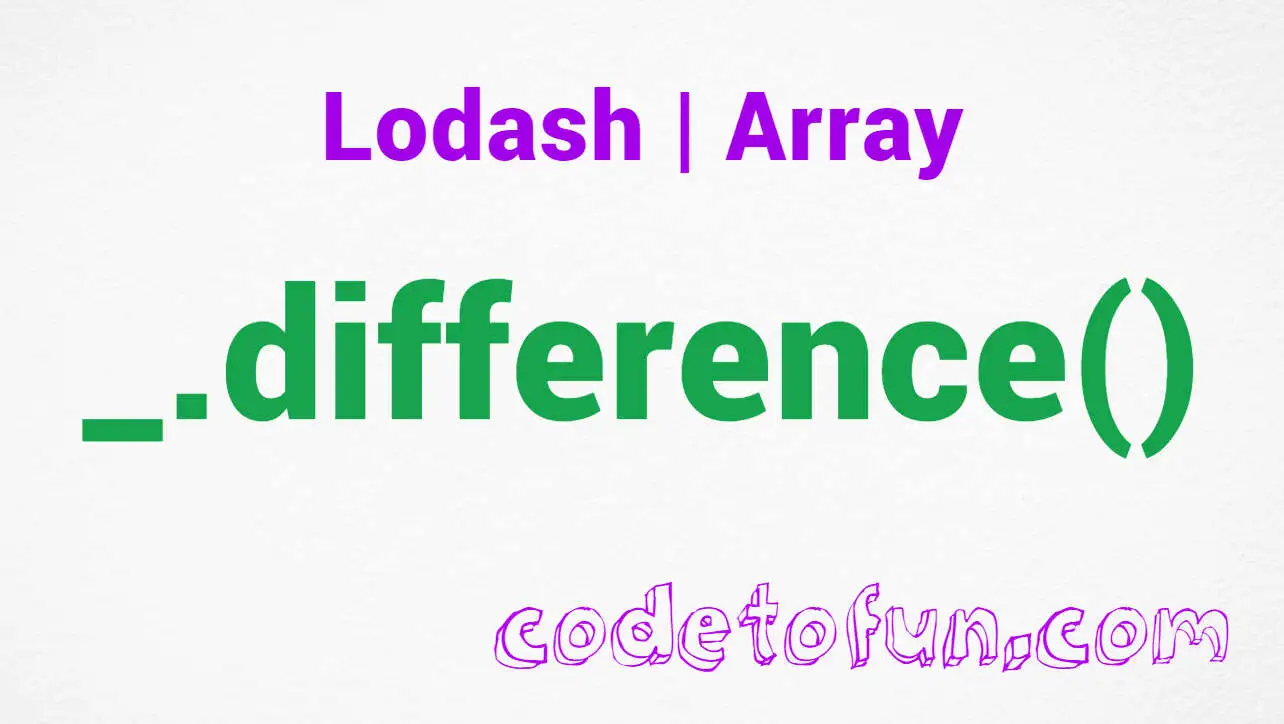
Photo Credit to CodeToFun
🙋 Introduction
When working with arrays in JavaScript, developers often encounter scenarios where they need to find the difference between two arrays. Lodash, a powerful utility library, provides the _.difference()
method to simplify this process.
This method efficiently computes the set difference between two arrays, making it a valuable tool for array manipulation.
🧠 Understanding _.difference()
The _.difference()
method in Lodash compares two arrays and returns a new array containing the elements that exist in the first array but not in the second. This can be particularly useful when you want to filter out common elements or identify unique values in an array.
💡 Syntax
_.difference(array, [values])
- array: The array to inspect.
- values: The values to exclude.
📝 Example
Let's delve into an example to illustrate the functionality of _.difference()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [1, 2, 3, 4, 5];
const array2 = [3, 4, 5, 6, 7];
const differenceArray = _.difference(array1, array2);
console.log(differenceArray);
// Output: [1, 2]
In this example, differenceArray contains the elements that are unique to array1 and not present in array2.
🏆 Best Practices
Validate Inputs:
Before using
_.difference()
, ensure that both input arrays are valid. Handle scenarios where either of the arrays is empty or not an array.validate-inputs.jsCopiedif (!Array.isArray(array1) || !Array.isArray(array2)) { console.error('Invalid input arrays'); return; } const validatedDifferenceArray = _.difference(array1, array2); console.log(validatedDifferenceArray);
Handle Edge Cases:
Consider edge cases, such as both arrays being empty or containing duplicate values. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const arrayWithDuplicates = [1, 2, 2, 3, 4, 4, 5]; const emptyArrayDifference = _.difference(emptyArray, array2); // Returns: [] const duplicateArrayDifference = _.difference(arrayWithDuplicates, array2); // Returns: [1, 2] console.log(emptyArrayDifference); console.log(duplicateArrayDifference);
Case-Sensitive Comparison:
By default,
_.difference()
uses strict equality (===) for comparison. If case-insensitive comparison is needed, consider converting the arrays to lowercase or uppercase before using the method.case-sensitive-comparison.jsCopiedconst caseInsensitiveArray1 = ['apple', 'Banana', 'Orange']; const caseInsensitiveArray2 = ['banana', 'orange']; const caseInsensitiveDifference = _.difference( caseInsensitiveArray1.map(item => item.toLowerCase()), caseInsensitiveArray2.map(item => item.toLowerCase()) ); console.log(caseInsensitiveDifference);
📚 Use Cases
Filtering Unique Values:
_.difference()
is ideal for scenarios where you want to filter out values that are common between two arrays, leaving only the unique elements.filtering-unique-values.jsCopiedconst uniqueValuesArray = _.difference([1, 2, 3, 4, 5], [3, 4, 5]); console.log(uniqueValuesArray); // Output: [1, 2]
Excluding Unwanted Elements:
In situations where you want to exclude specific elements from an array,
_.difference()
provides a concise solution.excluding-unwanted-elements.jsCopiedconst excludeElements = [2, 4, 6]; const filteredArray = _.difference([1, 2, 3, 4, 5, 6], excludeElements); console.log(filteredArray); // Output: [1, 3, 5]
🎉 Conclusion
The _.difference()
method in Lodash offers a streamlined way to find the set difference between two arrays. Whether you're filtering unique values or excluding unwanted elements, this method simplifies array manipulation tasks. By incorporating _.difference()
into your projects, you can achieve more efficient and readable code.
Explore the capabilities of Lodash and elevate your array manipulation with _.difference()
!
👨💻 Join our Community:
Author
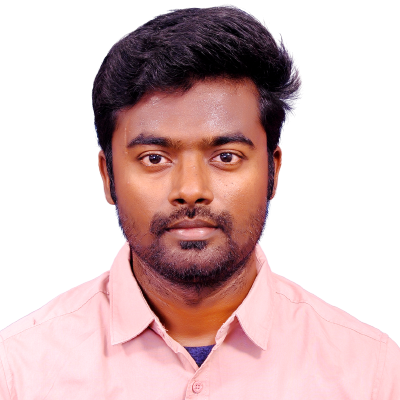
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
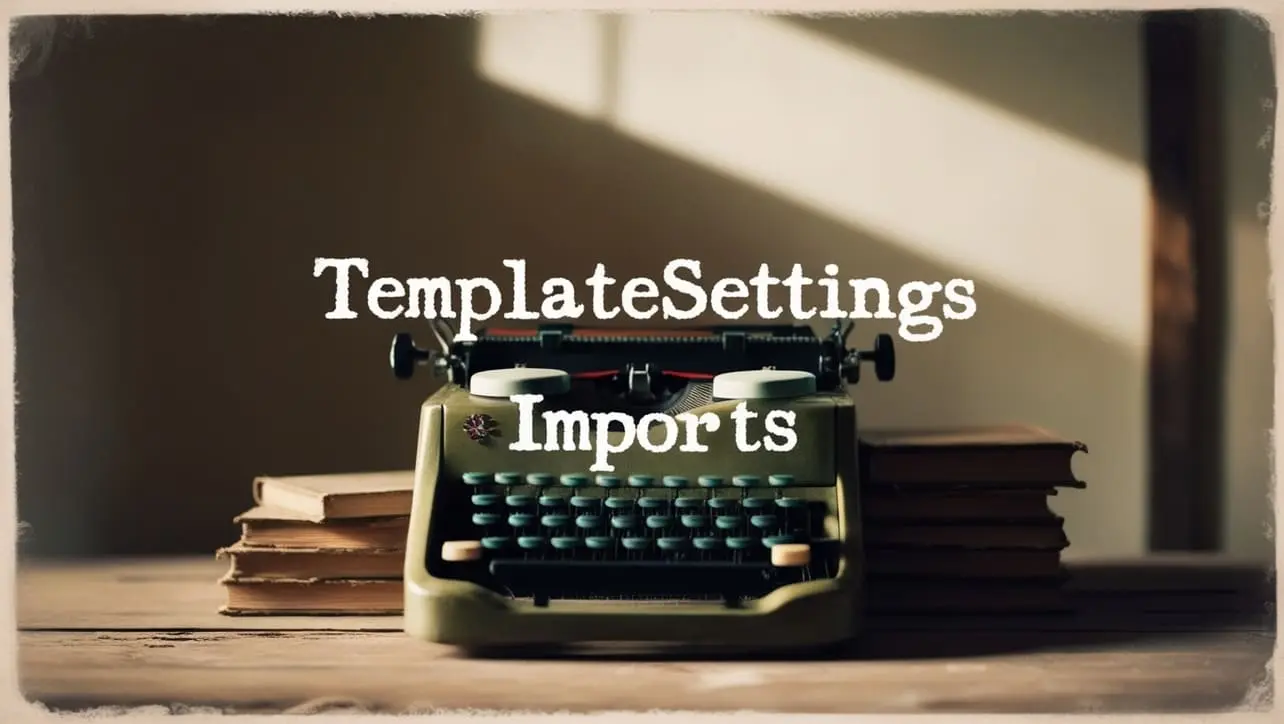
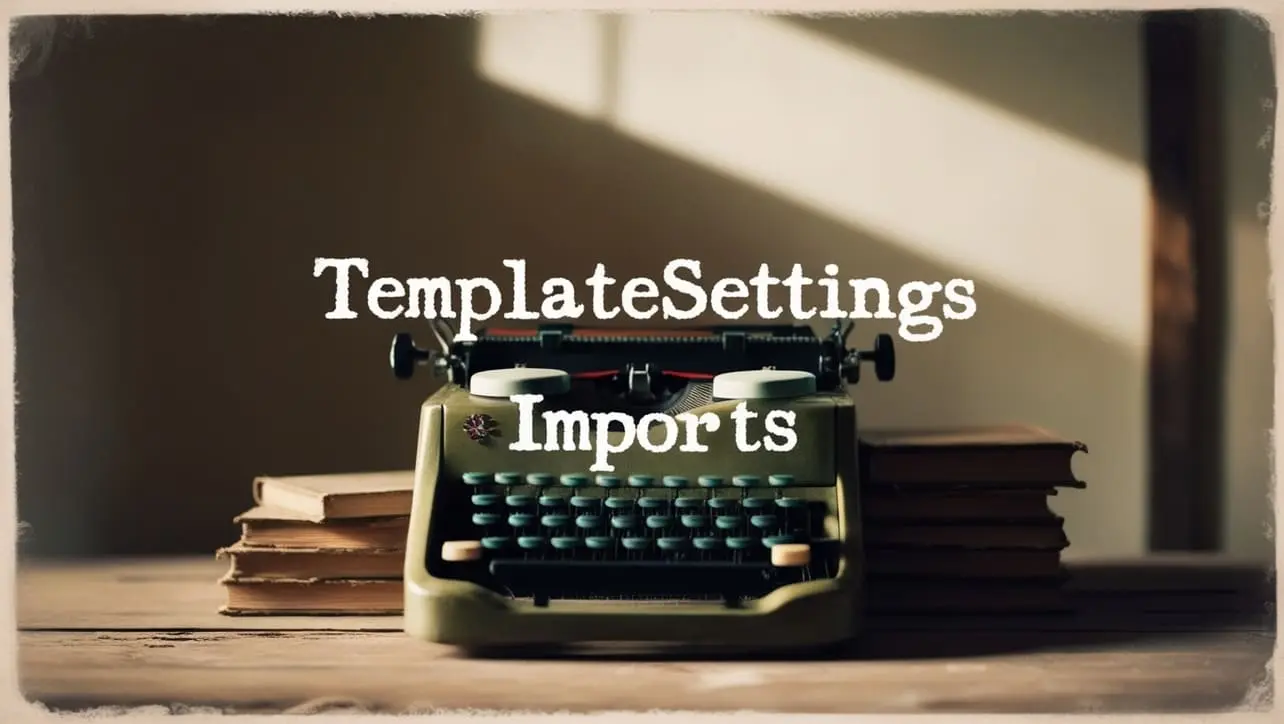
Lodash _.templateSettings.imports Property
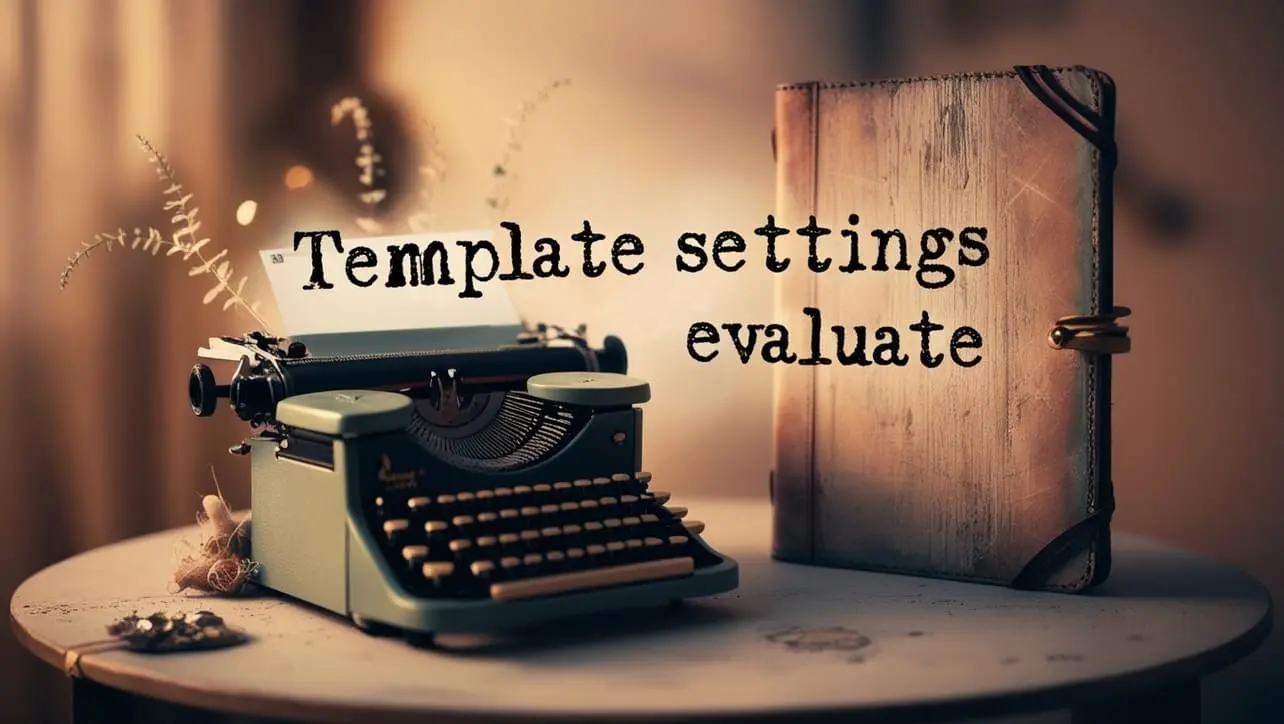
Lodash _.templateSettings.evaluate Property
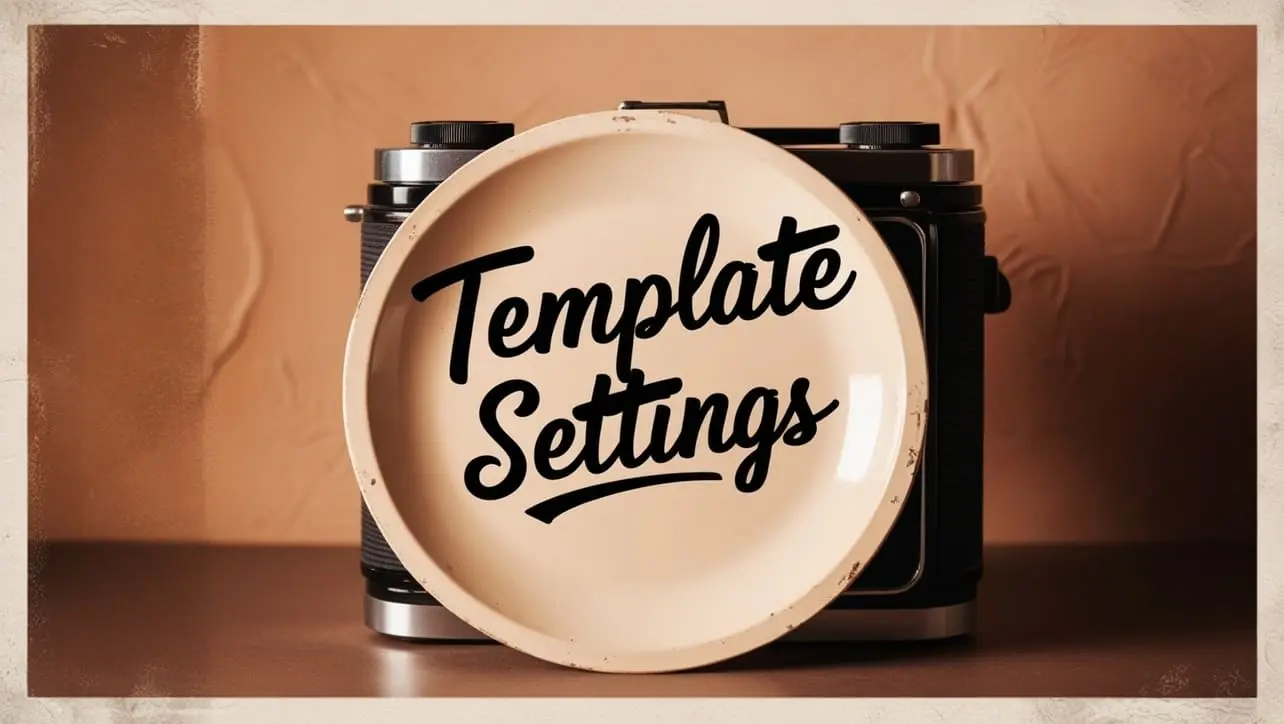
Lodash _.templateSettings Property
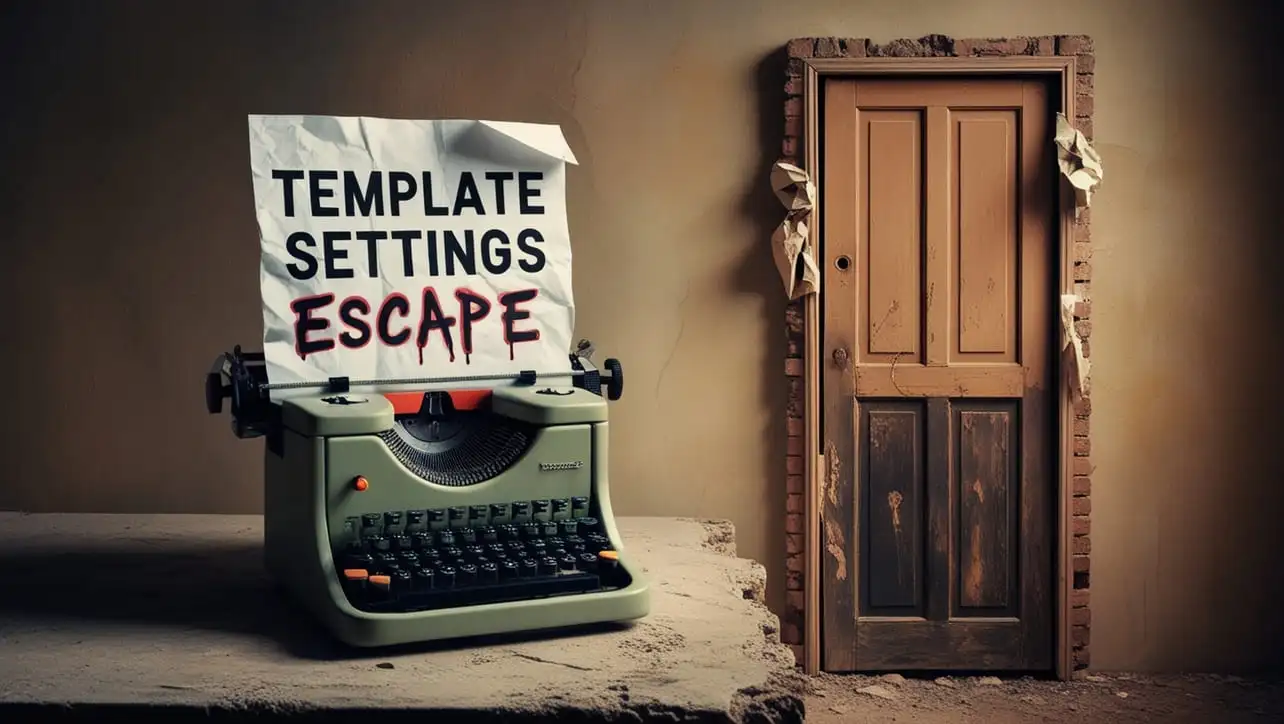
Lodash _.templateSettings.escape Property
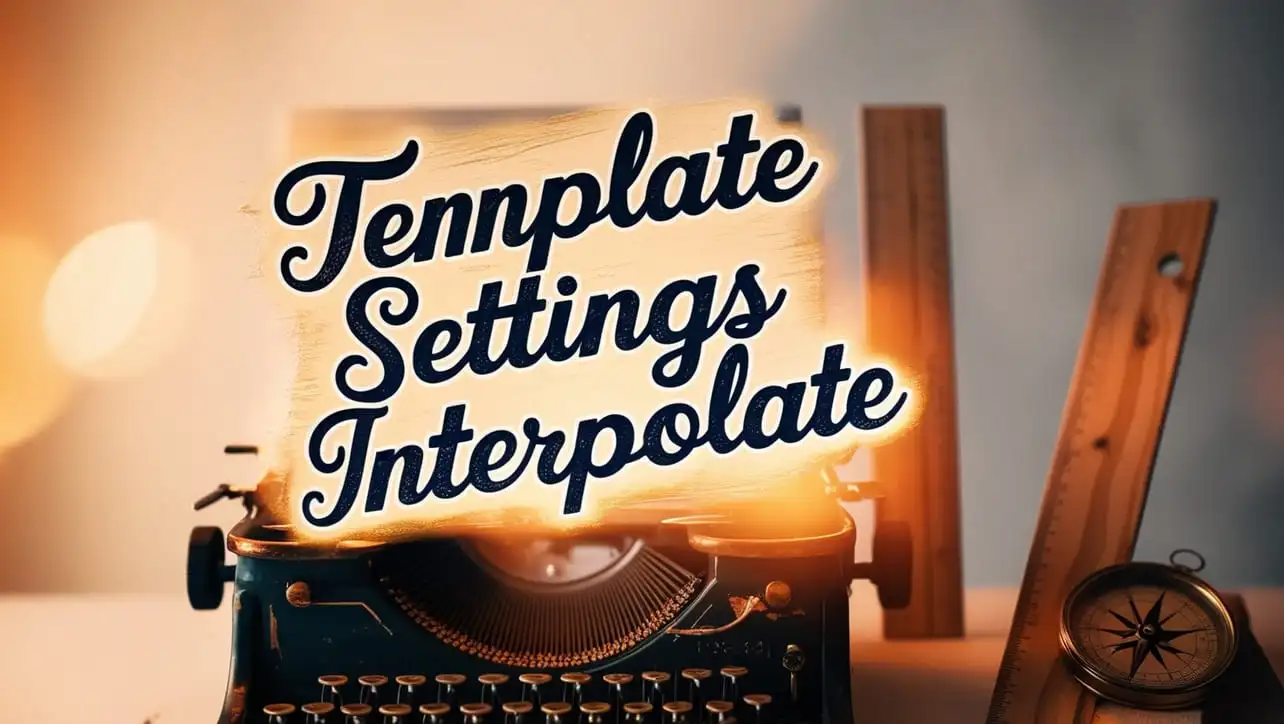
Lodash _.templateSettings.interpolate Property
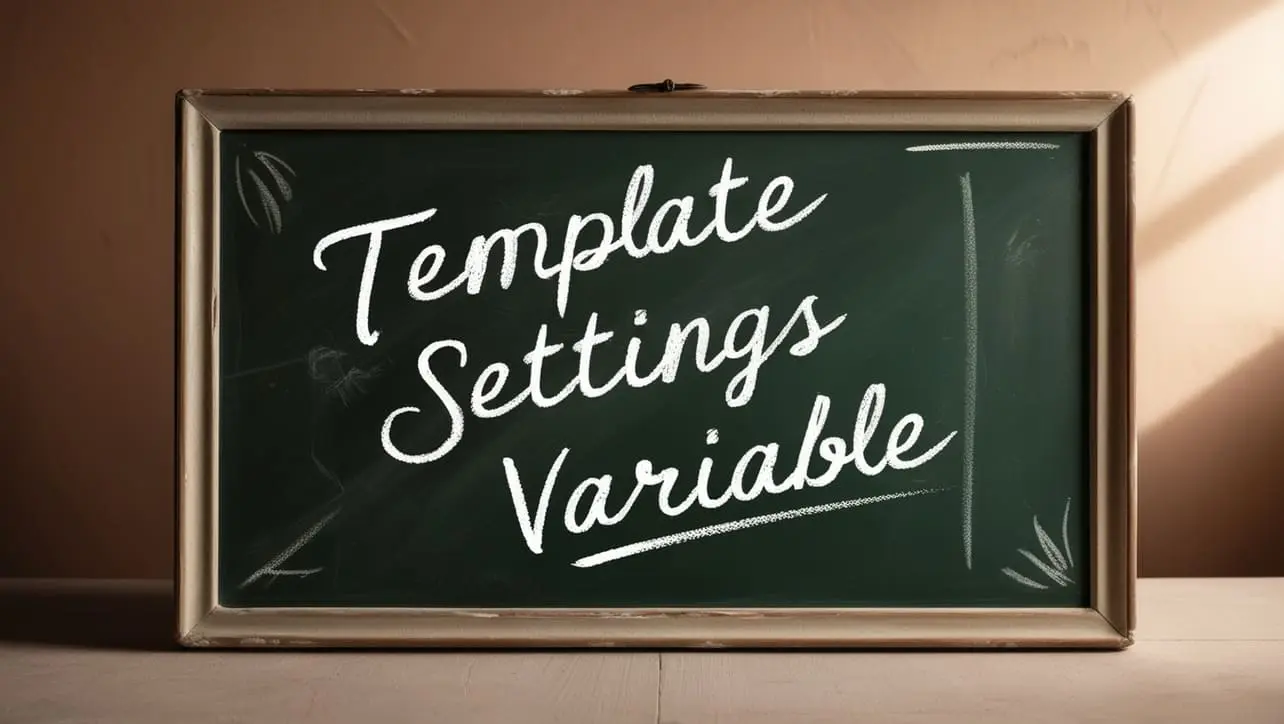
If you have any doubts regarding this article (Lodash _.difference() Array Method), please comment here. I will help you immediately.