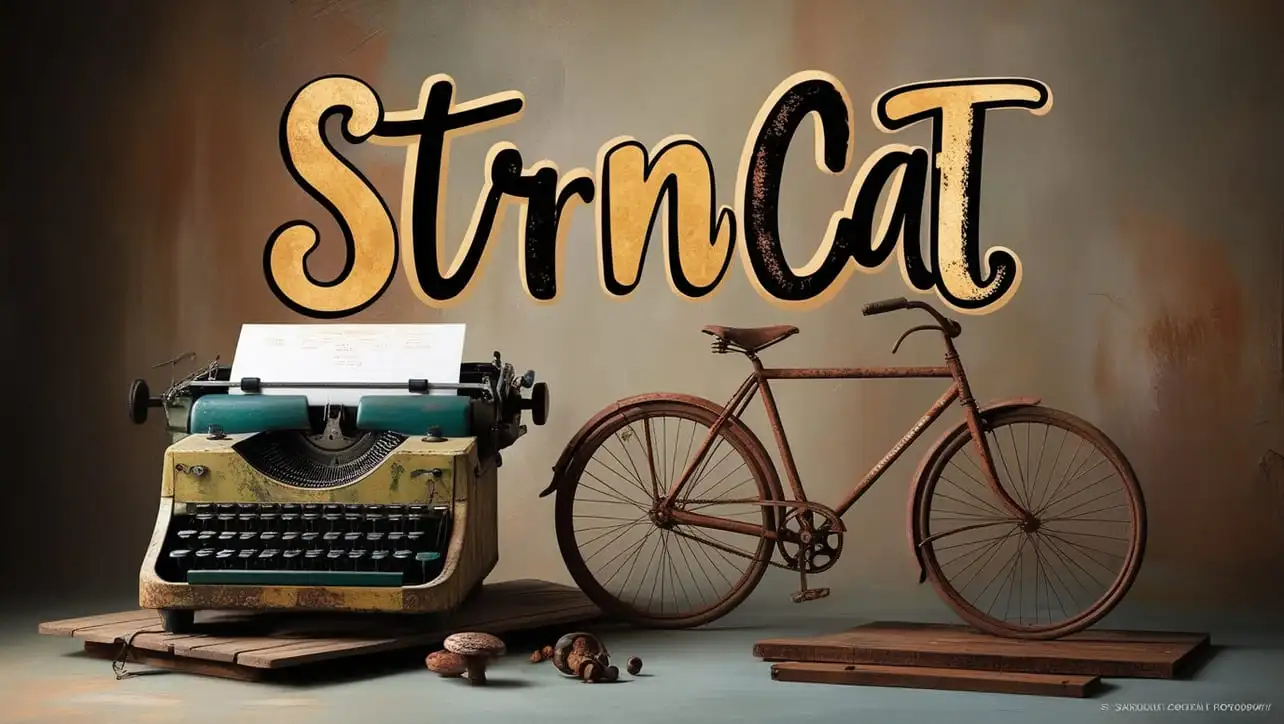
C++ String strcpy() Function
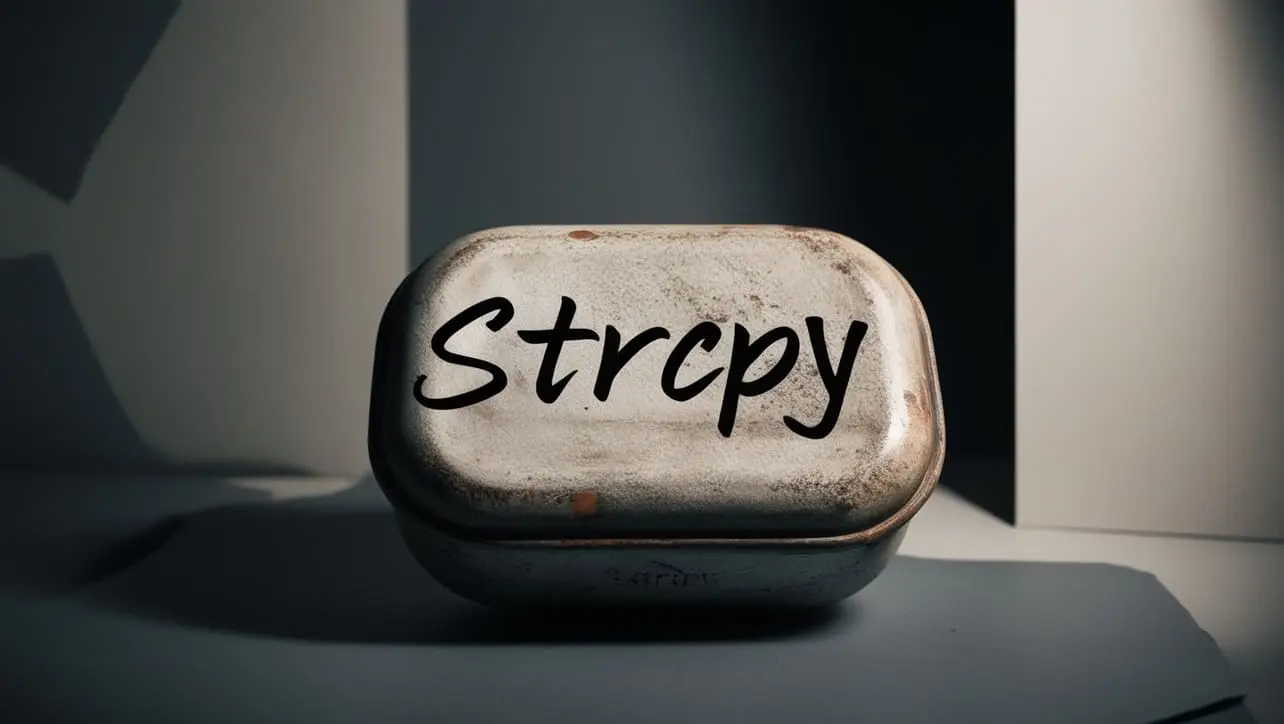
Photo Credit to CodeToFun
Introduction
In C++ programming, manipulating strings is a common task, and the strcpy()
function is a fundamental part of string handling.
The strcpy()
function is used to copy a C-style string (a sequence of characters ending with a null character) from one location to another.
In this tutorial, we'll explore the usage and functionality of the strcpy()
function in C++.
Syntax
The signature of the strcpy()
function is as follows:
char* strcpy(char* destination, const char* source);
This function takes two parameters - destination and source - and copies the string from source to destination.
Example
Let's delve into an example to illustrate how the strcpy()
function works.
#include <iostream>
#include <cstring>
int main() {
const char * source = "Hello, C++!";
char destination[20]; // Make sure it's large enough to hold the source string
// Copy the string
strcpy(destination, source);
// Output the result
std::cout << "Source: " << source << std::endl;
std::cout << "Destination: " << destination << std::endl;
return 0;
}
Output
Source: Hello, C++! Destination: Hello, C++!
How the Program Works
In this example, the strcpy()
function is used to copy the string "Hello, C++!" from source to destination.
Return Value
The strcpy()
function returns a pointer to the destination string. The destination string is modified to contain a copy of the source string.
Common Use Cases
The strcpy()
function is useful when you need to duplicate or create a new copy of a C-style string. It's commonly used when working with arrays of characters in C++.
Notes
- Ensure that the destination array has enough space to accommodate the source string, including the null character at the end.
- Be cautious to avoid buffer overflows by ensuring the destination array size is sufficient.
Optimization
The strcpy()
function is optimized for simplicity and straightforward string copying. If you need more advanced string manipulation or dynamic memory allocation, consider using C++ Standard Library classes like std::string.
Conclusion
The strcpy()
function in C++ is a basic yet essential tool for copying C-style strings. While it has its use cases, be mindful of potential pitfalls related to buffer sizes and consider alternatives like std::string for more robust string handling.
Feel free to experiment with different strings and explore the behavior of the strcpy()
function in various scenarios. Happy coding!
Join our Community:
Author
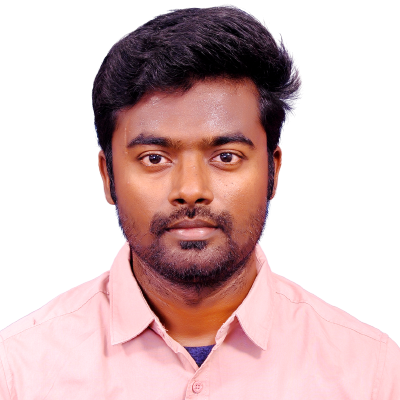
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strcpy() Function), please comment here. I will help you immediately.