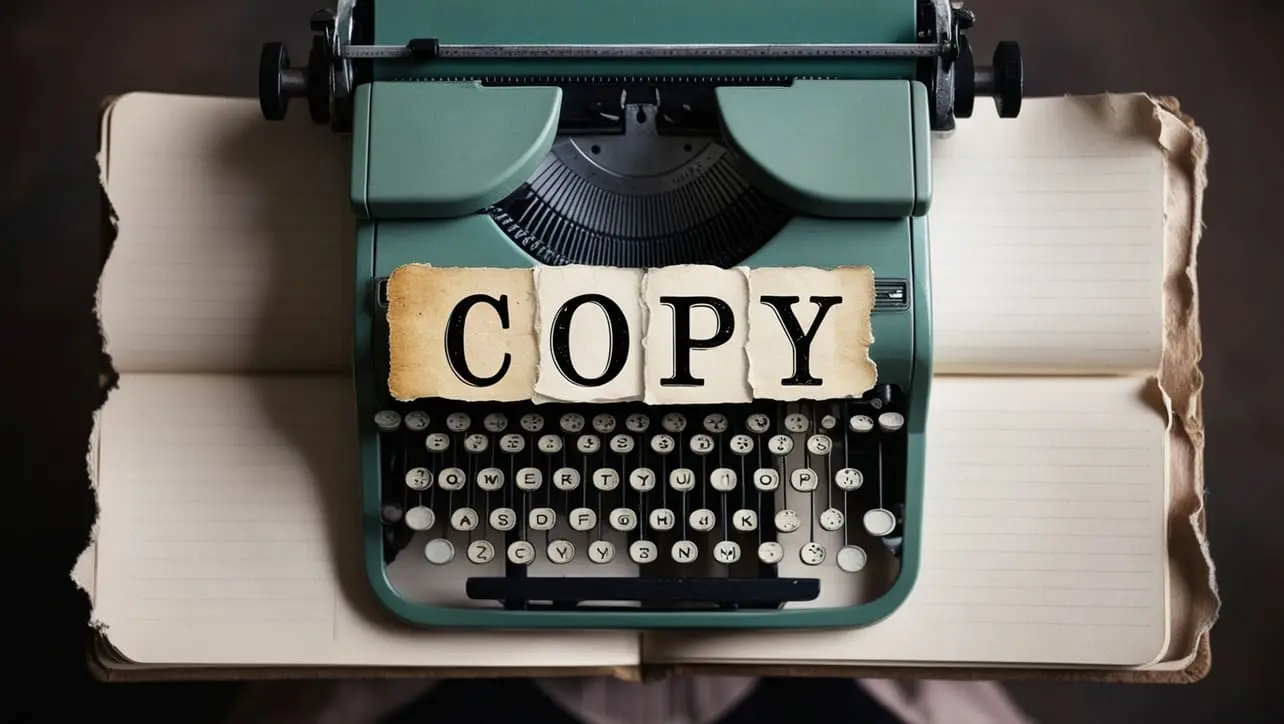
C# Basic
C# String Format() Method
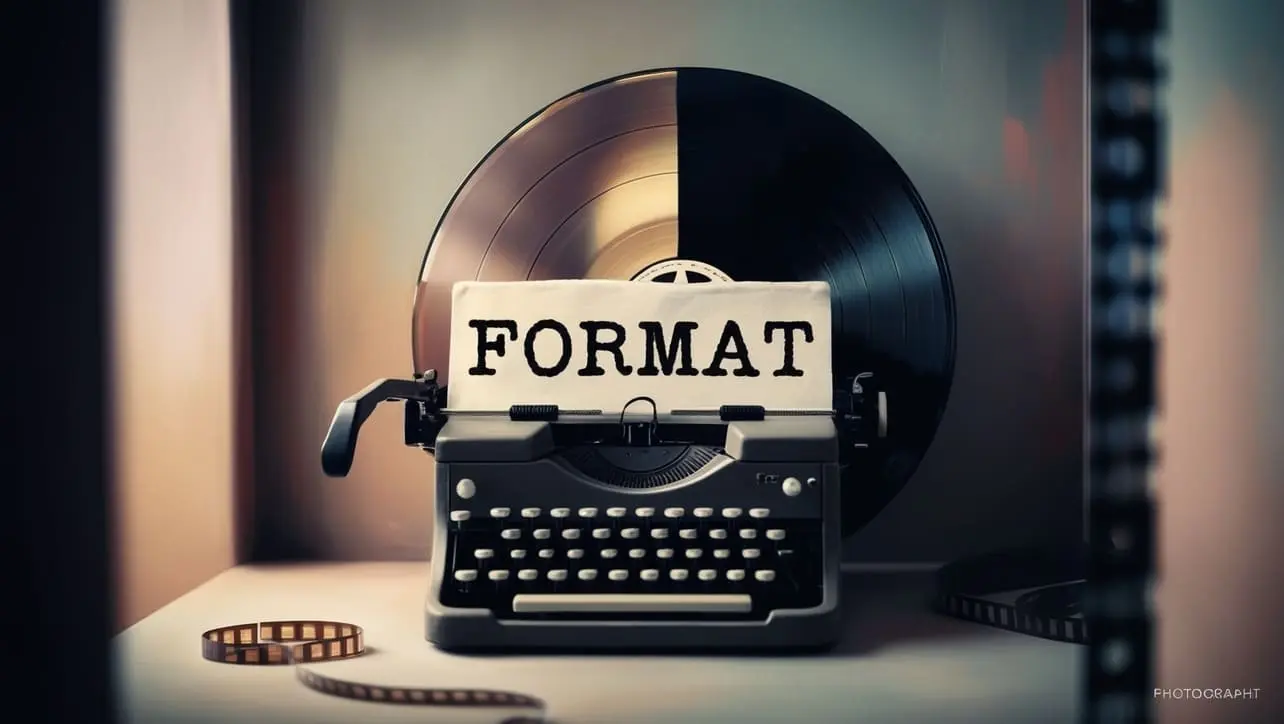
Photo Credit to CodeToFun
đ Introduction
In C# programming, the String.Format() method is a powerful tool for formatting strings by substituting placeholders with values. It belongs to the System.String class and provides a convenient way to create formatted strings.
In this tutorial, we'll explore the usage and functionality of the Format()
method in C#.
đĄ Syntax
The syntax for the Format()
method is as follows:
public static string Format(string format, params object[] args);
- format: A composite format string that contains placeholders.
- args: An array of objects to format and insert into the string.
đ Example
Let's dive into an example to illustrate how the Format()
method works.
using System;
class Program {
static void Main() {
string name = "John";
int age = 30;
// Format a string using placeholders
string formattedString = string.Format("Hello, my name is {0} and I'm {1} years old.", name, age);
// Output the formatted string
Console.WriteLine(formattedString);
}
}
đģ Testing the Program
The output of the above example will be:
Hello, my name is John and I'm 30 years old.
đ§ How the Program Works
In this example, the Format()
method is used to create a formatted string by replacing placeholders {0} and {1}> with the values of the variables name and age, respectively.
âŠī¸ Return Value
The Format()
method returns a new string that is the result of formatting the specified format string with the provided arguments.
đ Common Use Cases
The Format()
method is particularly useful when you need to create complex strings with dynamic content. It simplifies the process of concatenating values into a string, making the code more readable and maintainable.
đ Notes
- Placeholders in the format string are represented by curly braces {} with an index inside, such as {0}, {1}, etc.
- The order of the values in the args array determines their placement in the formatted string.
- The
Format()
method supports various formatting options, including alignment, precision, and format specifiers.
đĸ Optimization
The Format()
method is optimized for performance, and its usage is efficient. If you have a fixed number of placeholders, consider using string interpolation ($"") in C# for improved readability.
đ Conclusion
The Format()
method in C# is a versatile tool for creating formatted strings. It simplifies the process of string construction and enhances code readability. Understanding its usage is valuable in various scenarios, especially when dealing with dynamic content in strings.
Feel free to experiment with different format strings and explore the various formatting options provided by the Format()
method. Happy coding!
đ¨âđģ Join our Community:
Author
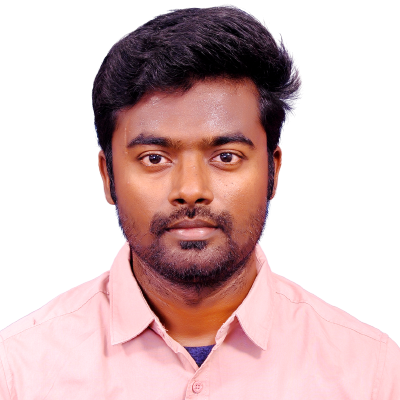
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String Format() Method), please comment here. I will help you immediately.