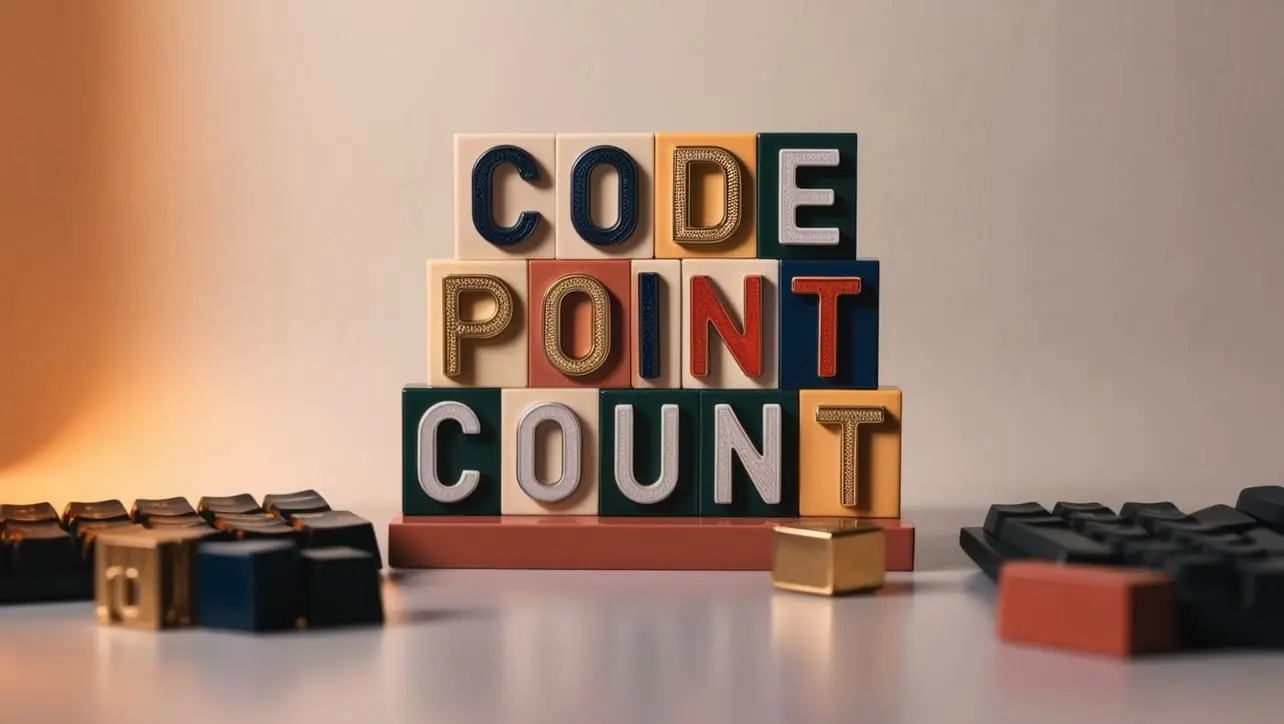
Java string equals() Method
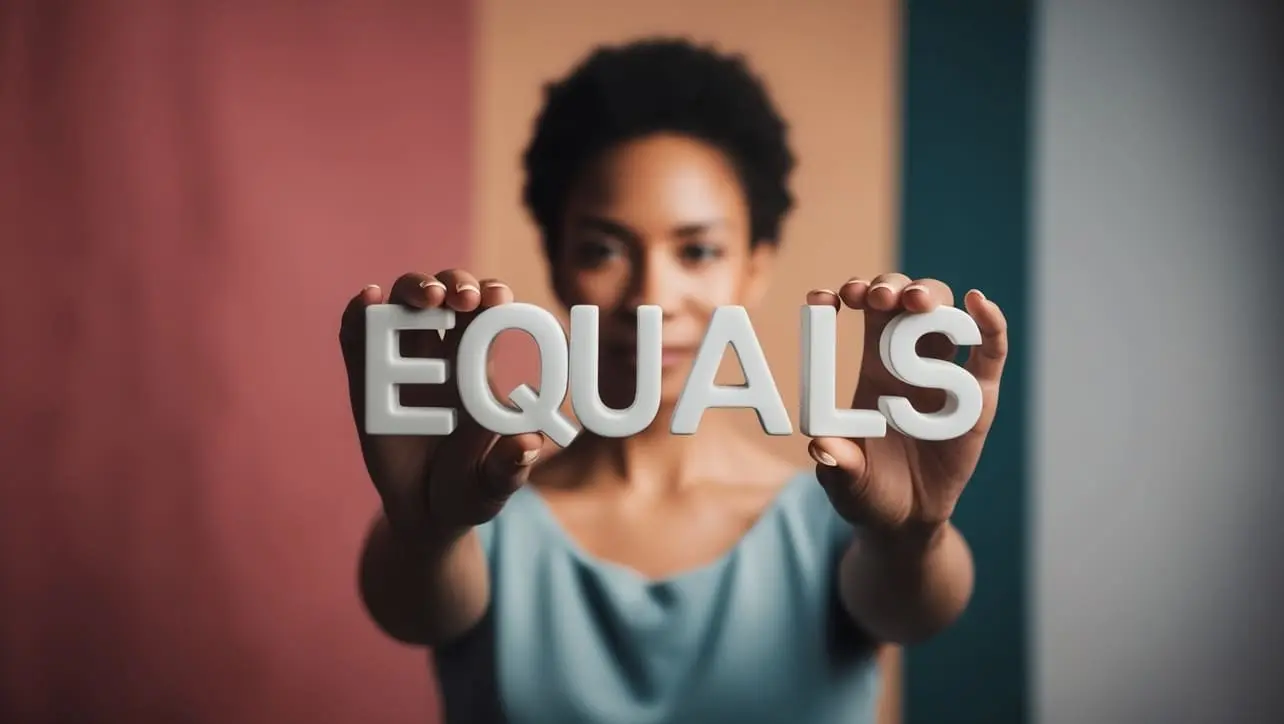
Photo Credit to CodeToFun
Introduction
In Java programming, strings are a fundamental data type, and comparing them is a common operation.
The equals()
method is a critical tool in Java for comparing the content of two strings.
In this tutorial, we'll explore the usage and functionality of the equals()
method in Java.
Syntax
The signature of the equals()
method is as follows:
public boolean equals(Object anotherObject);
This method compares the content of the current string with the content of the specified object.
Example
Let's delve into an example to illustrate how the equals()
method works.
public class StringEqualsExample {
public static void main(String[] args) {
String str1 = "Hello, World!";
String str2 = "Hello, World!";
String str3 = "Hello, Java!";
// Compare strings using equals()
boolean result1 = str1.equals(str2);
boolean result2 = str1.equals(str3);
// Output the results
System.out.println("Result 1: " + result1); // Output: true
System.out.println("Result 2: " + result2); // Output: false
}
}
Output
Result 1: true Result 2: false
How the Program Works
In this example, the equals()
method is used to compare two strings, "Hello, World!" and "Hello, Java!". The results are then printed.
Return Value
The equals()
method returns true if the content of the strings is the same and false otherwise.
Common Use Cases
The equals()
method is commonly used when you need to check whether two strings have the same content. It's crucial in scenarios such as input validation, user authentication, and when working with collections of strings.
Notes
- The
equals()
method is case-sensitive. If you need a case-insensitive comparison, you can use the equalsIgnoreCase() method. - When overriding the
equals()
method in your custom classes, ensure that you follow the contract defined in the Object class.
Optimization
The equals()
method is optimized for string comparison. However, for performance-critical applications or large-scale string processing, you may consider alternative approaches or explore specific optimizations based on your use case.
Conclusion
The equals()
method in Java is a fundamental tool for comparing the content of strings. It provides a standardized and efficient way to perform this task, contributing to the reliability and robustness of your Java applications.
Feel free to experiment with different strings and explore the behavior of the equals()
method in various scenarios. Happy coding!
Join our Community:
Author
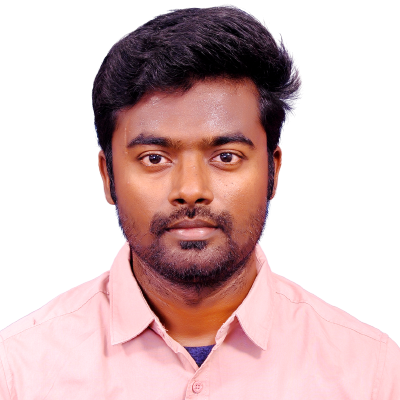
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
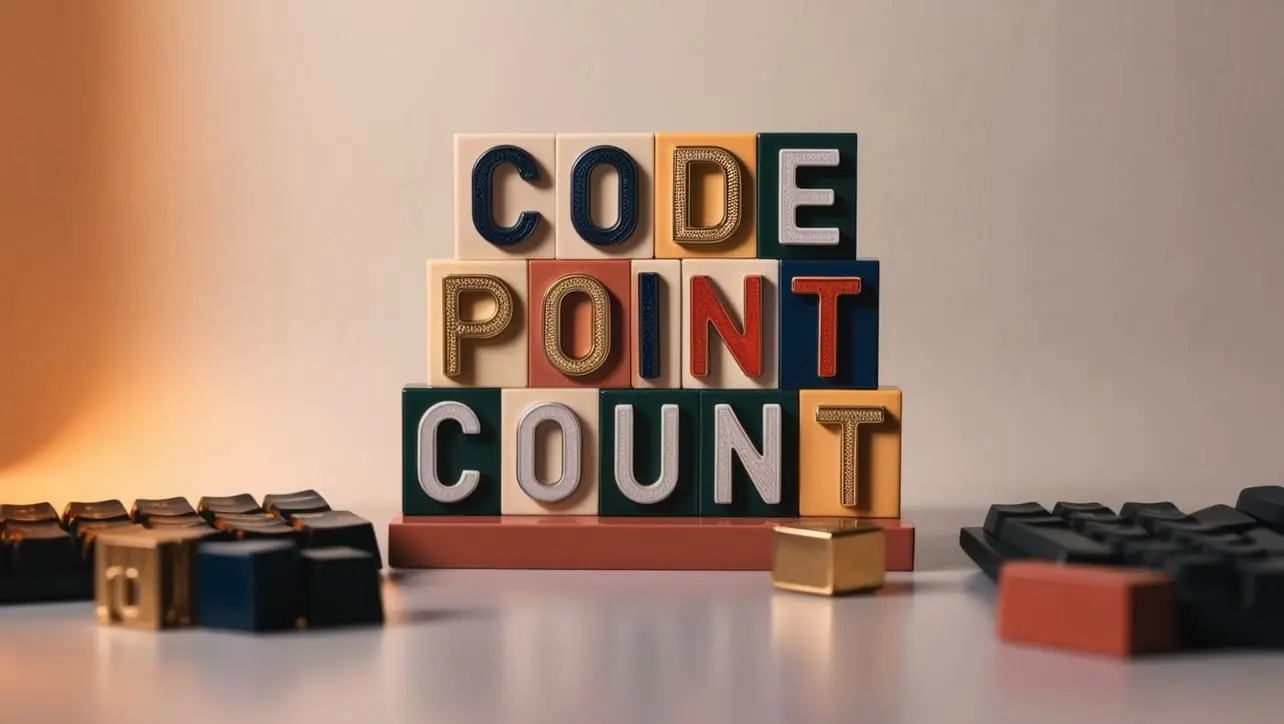
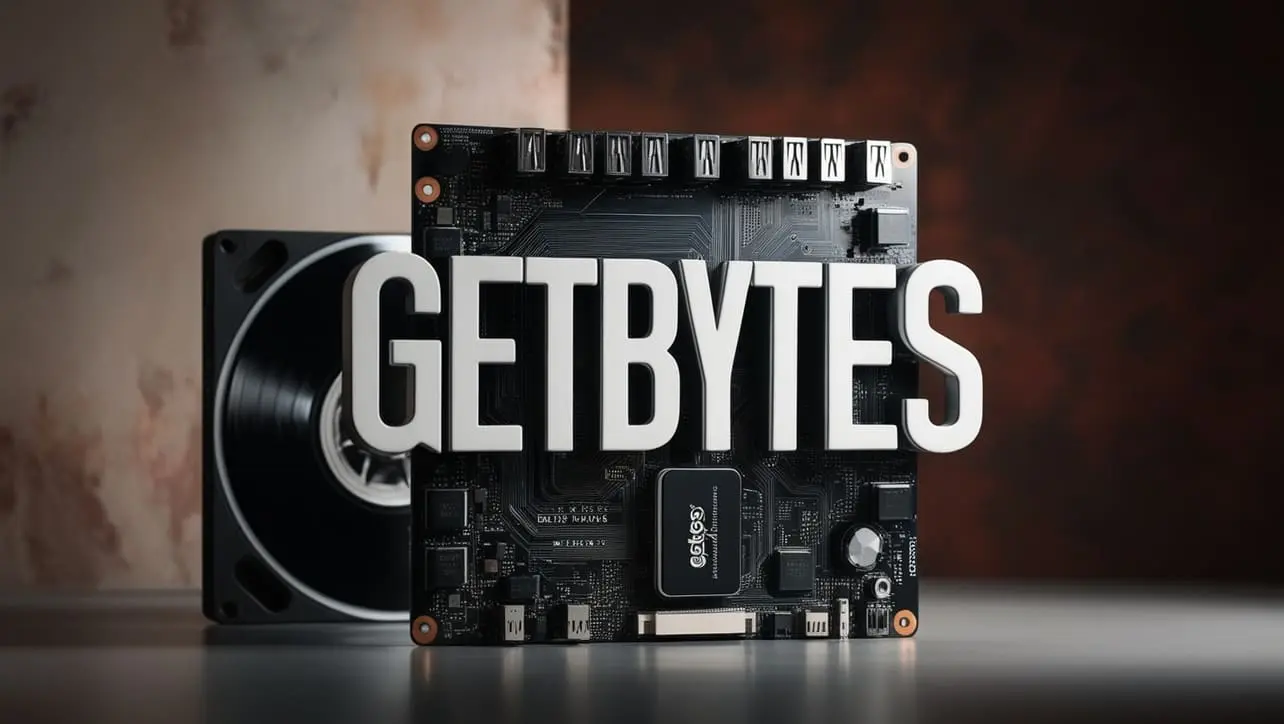
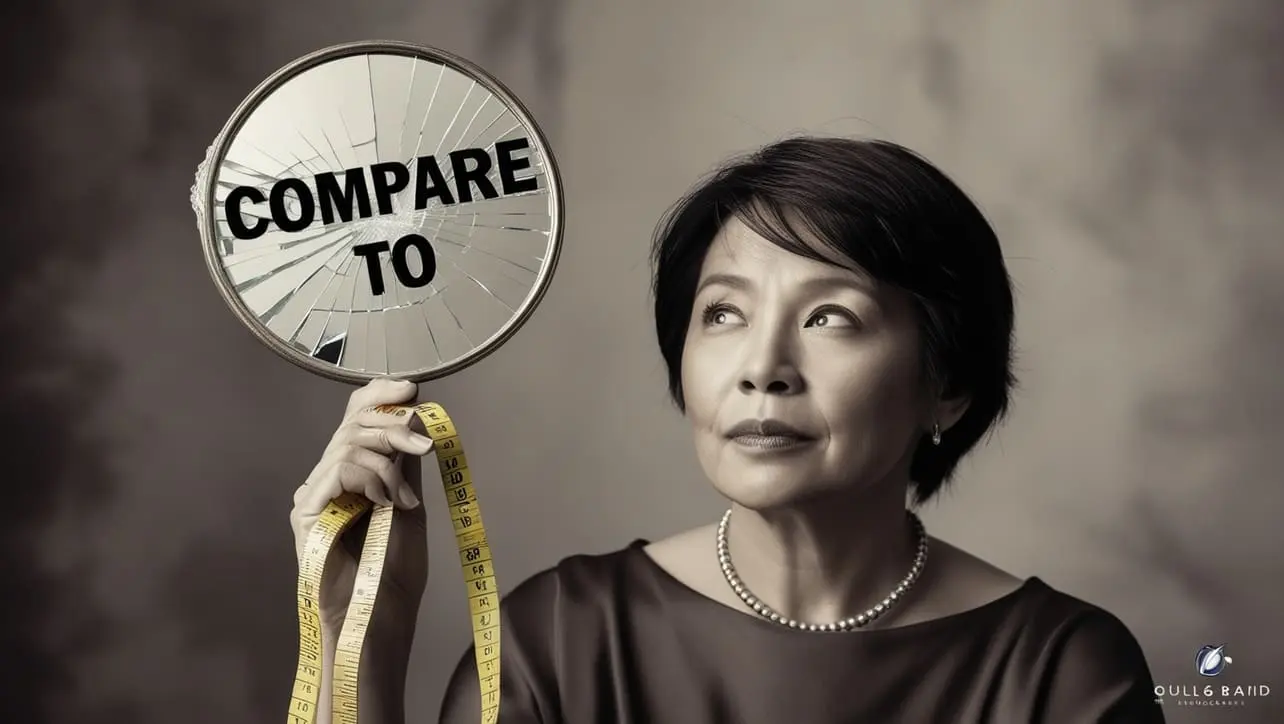
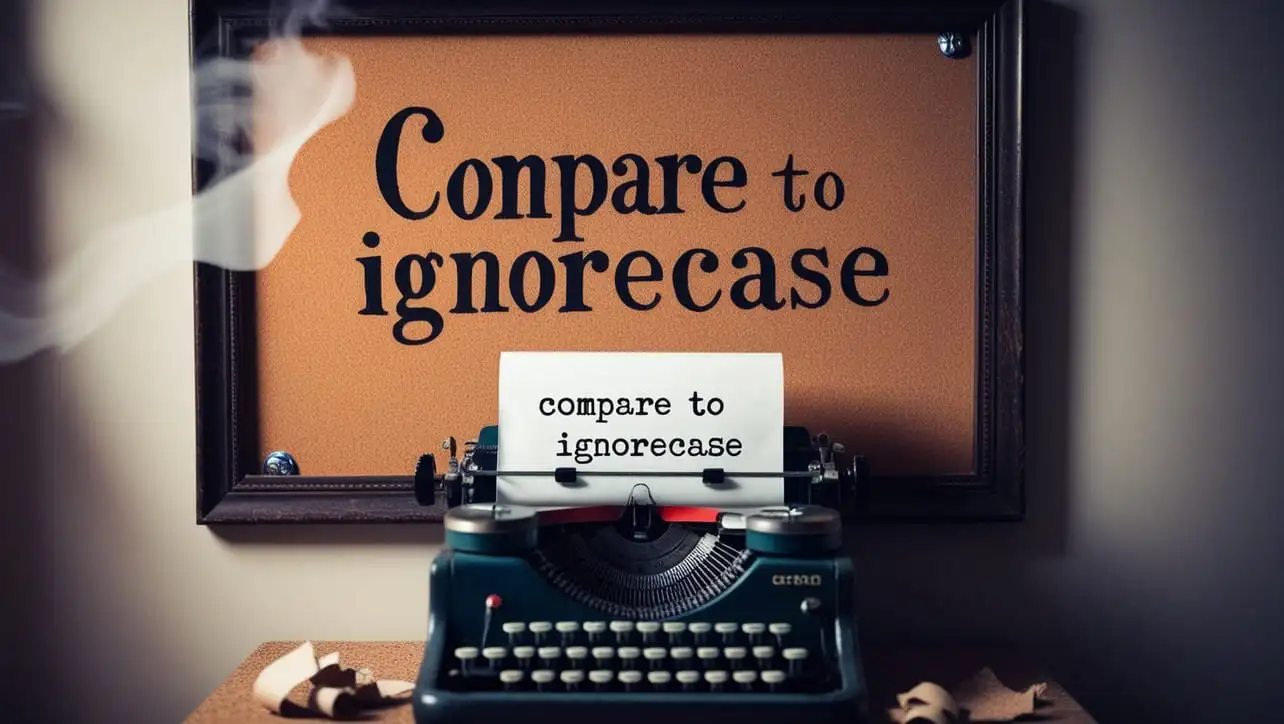
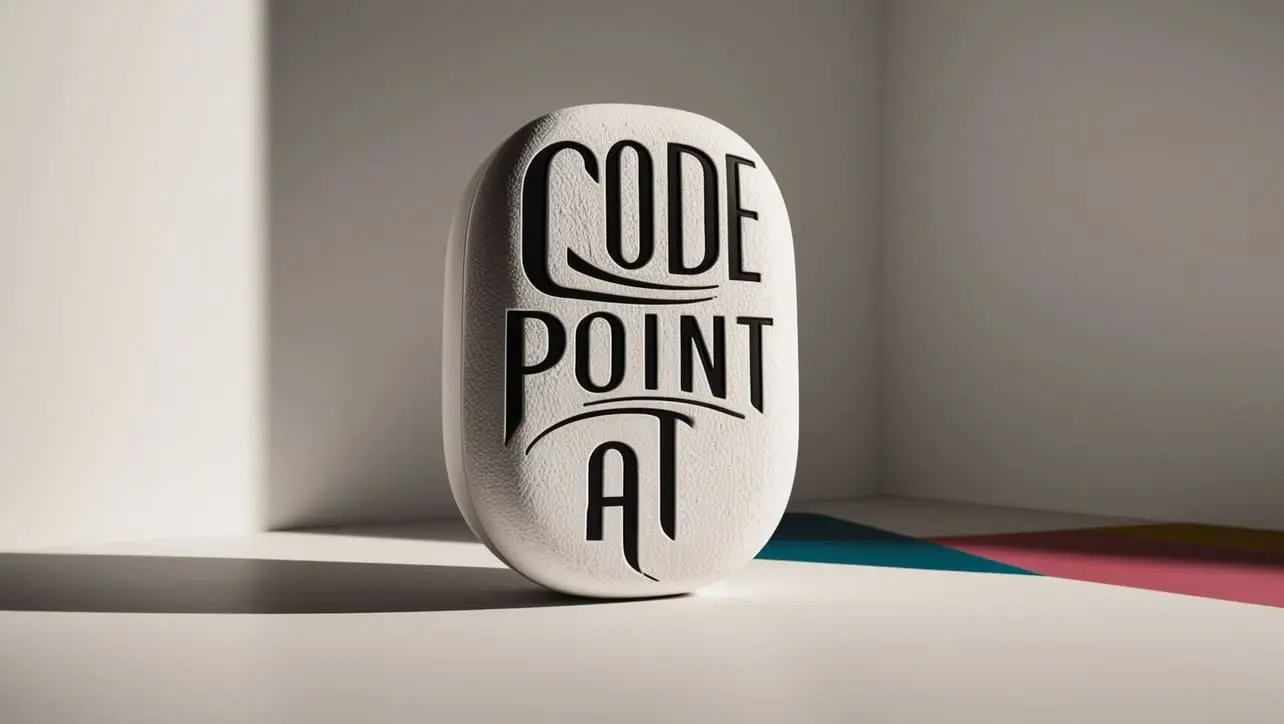
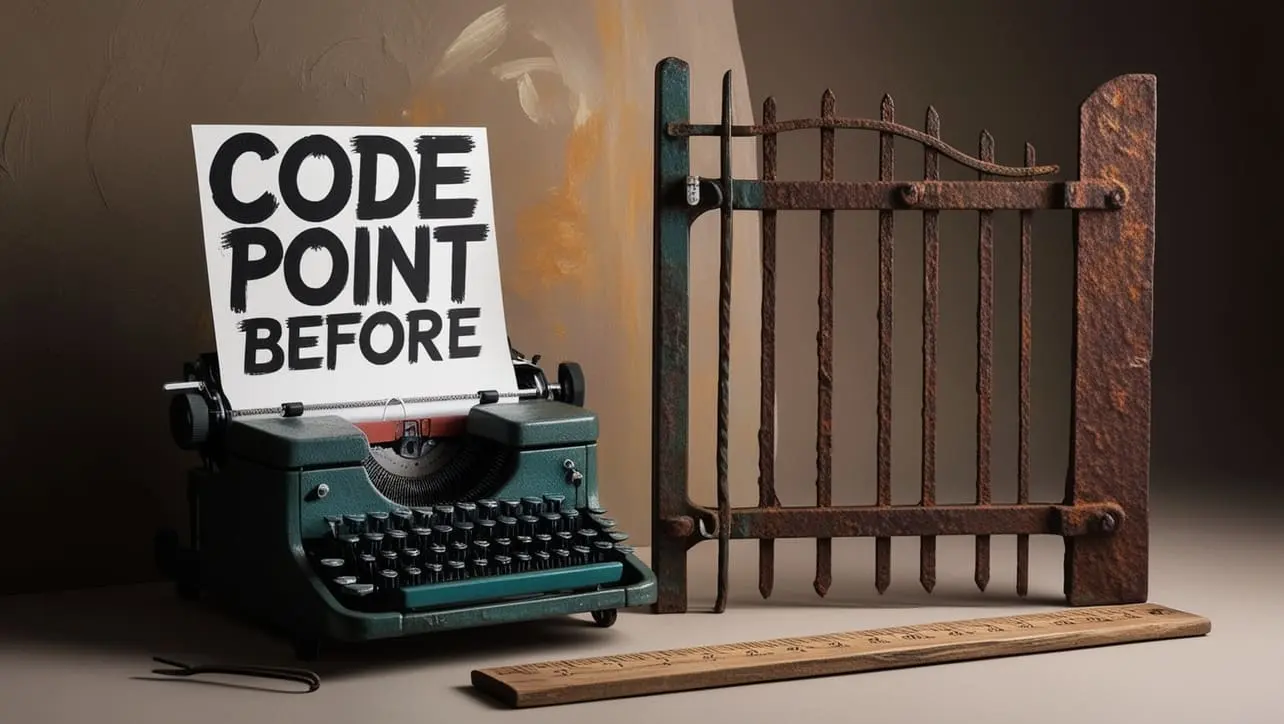
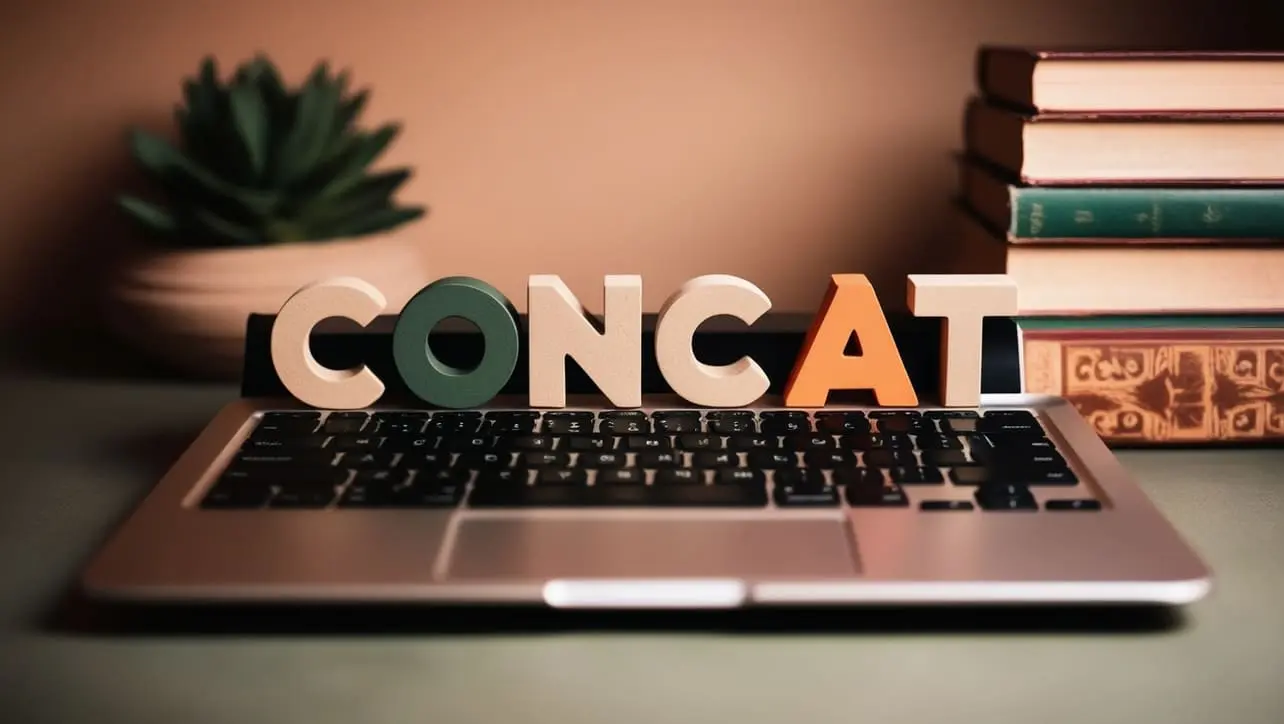
If you have any doubts regarding this article (Java string equals() Method), please comment here. I will help you immediately.