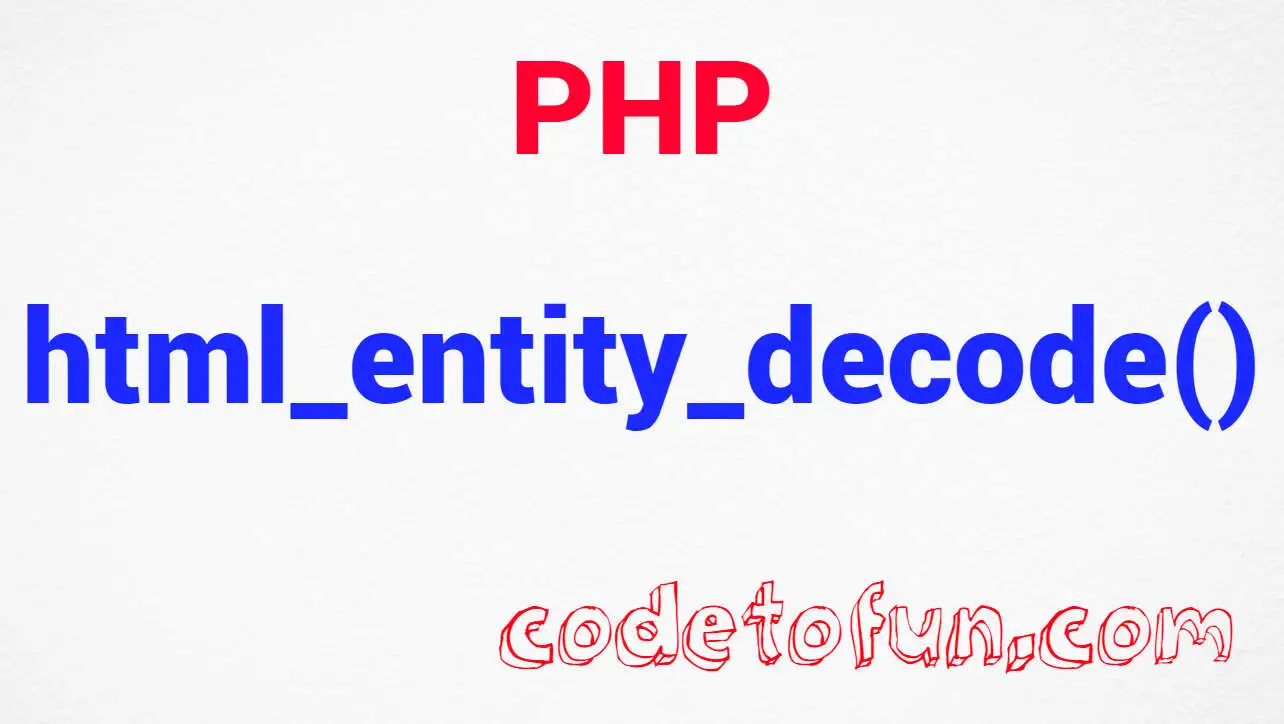
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to find Composite Number
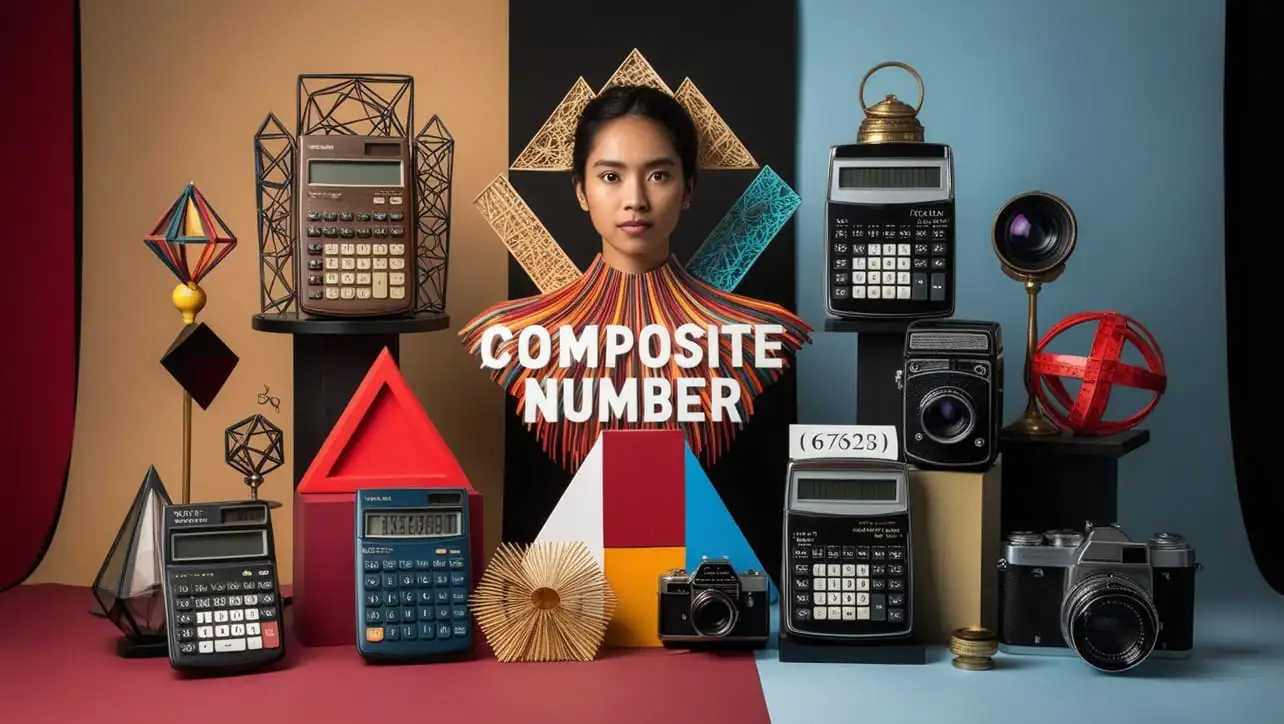
Photo Credit to CodeToFun
π Introduction
In the realm of programming, identifying and working with different types of numbers is a fundamental skill.
A composite number is one that has more than two positive divisors, excluding 1 and itself. In this tutorial, we'll explore a php program that efficiently determines whether a given number is composite or not.
π Example
Let's dive into the php code that checks whether a number is composite or not.
<?php
// Function to check if a number is composite
function isComposite($number)
{
if ($number <= 1)
{
return false;
}
// Check for factors other than 1 and the number itself
for ($i = 2;$i < $number;$i++)
{
if ($number % $i === 0)
{
return true;
}
}
return false;
}
// Default input number
$defaultNumber = 12;
// Call the function to check if the default number is composite
$result = isComposite($defaultNumber);
// Output the result
if ($result)
{
echo "$defaultNumber is a composite number.\n";
}
else
{
echo "$defaultNumber is not a composite number.\n";
}
?>
π» Testing the Program
To test the program with different numbers, simply replace the value of $defaultNumber with your desired input.
12 is a composite number.
Run the script to see whether the number is a composite number.
π§ How the Program Works
- The program defines a function isComposite that takes a number as input and returns true if it is a composite number and false otherwise.
- Inside the function, it checks for factors other than 1 and the number itself by iterating through numbers from 2 to the number-1.
- If any factor is found, the number is considered composite.
- The default input number is set to 12, and the function is called to check whether it is a composite number.
- The result is then echoed to the console.
π Between the Given Range
Let's delve into the PHP code that checks for composite numbers in the specified range.
<?php
// Function to check if a number is composite
function isComposite($number)
{
if ($number <= 1)
{
return false;
}
// Check for divisors other than 1 and the number itself
for ($i = 2;$i < $number;$i++)
{
if ($number % $i == 0)
{
return true;
}
}
return false;
}
// Driver program to check for composite numbers in the range 1 to 10
echo "Composite numbers in the range 1 to 10 are: \n";
for ($i = 1;$i <= 10;$i++)
{
if (isComposite($i))
{
echo "$i ";
}
}
?>
π» Testing the Program
Composite numbers in the range 1 to 10 are: 4 6 8 9 10
The provided program checks for composite numbers in the range 1 to 10. To test the program, simply run it.
π§ How the Program Works
- The program defines a function isComposite that checks whether a given number is composite.
- Inside the function, it first checks if the number is less than or equal to 1 (not composite).
- It then iterates through numbers from 2 to the number itself, checking for divisors other than 1 and the number itself.
- The main section of the program tests and prints composite numbers in the range 1 to 10.
π§ Understanding the Concept of Composite Numbers
Composite numbers are integers greater than 1 that have divisors other than 1 and themselves.
For example, the number 12 is composite because it can be formed by multiplying 2 and 6 or 3 and 4.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations for larger numbers, such as checking divisors up to the square root of the number for improved efficiency.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
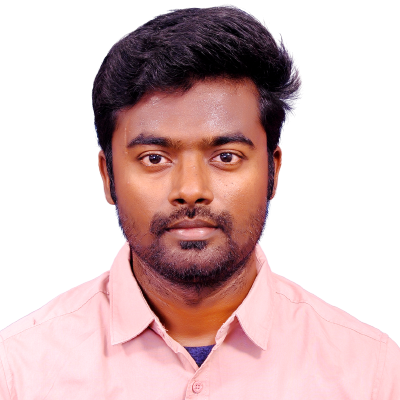
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to find Composite Number), please comment here. I will help you immediately.