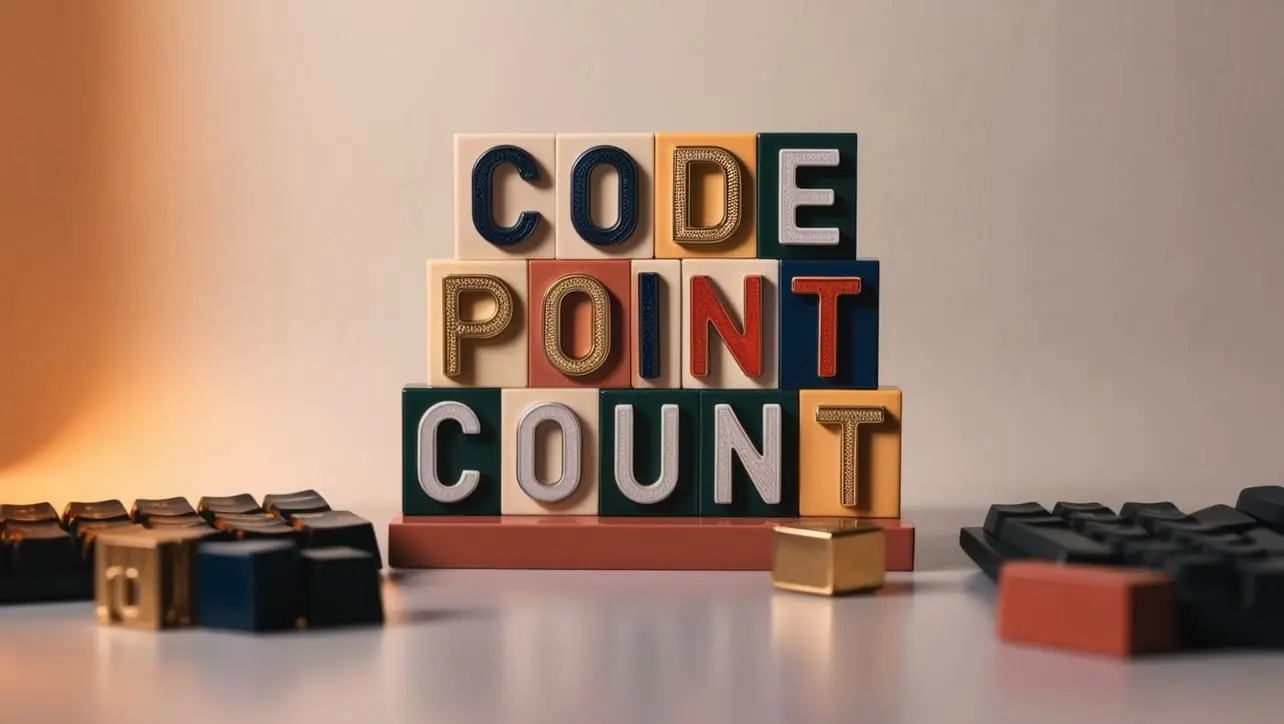
Java Topics
- Java Intro
- Java String Methods
- Java Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Java Star Pattern
- Java Number Pattern
- Java Alphabet Pattern
Java Program to find Minimum Value of an Array
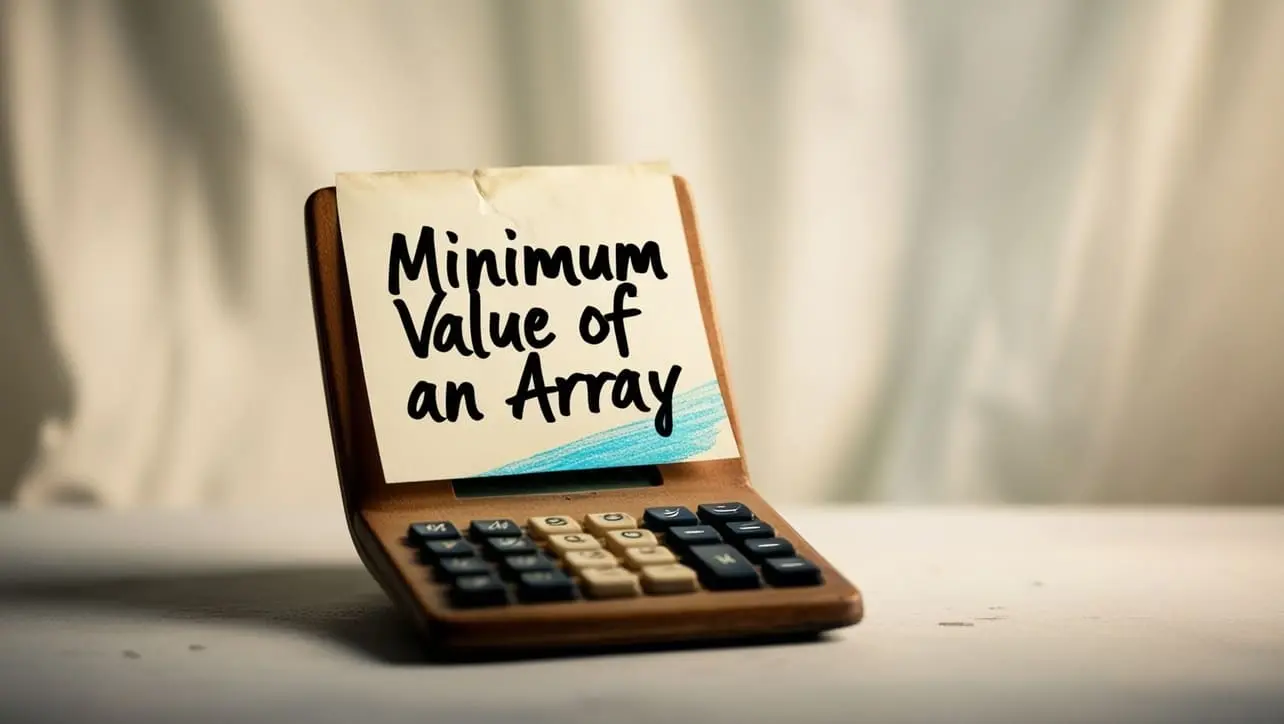
Photo Credit to CodeToFun
π Introduction
In the world of programming, manipulating arrays is a fundamental skill. One common task is finding the minimum value within an array.
This involves iterating through the array elements and identifying the smallest value present.
In this tutorial, we will walk through a java program that efficiently finds the minimum value of an array..
π Example
Let's dive into the java code to achieve this functionality.
public class MinValueOfArray {
// Function to find the minimum value in an array
static int findMinValue(int[] arr) {
// Assume the first element is the minimum
int minValue = arr[0];
// Iterate through the array to find the minimum value
for (int i = 1; i < arr.length; ++i) {
if (arr[i] < minValue) {
minValue = arr[i];
}
}
return minValue;
}
// Driver program
public static void main(String[] args) {
// Replace these values with your array
int[] array = {12, 5, 7, 3, 2, 8, 10};
// Call the function to find the minimum value
int minValue = findMinValue(array);
// Print the result
System.out.println("The minimum value in the array is: " + minValue);
}
}
π» Testing the Program
To test the program with a different array, simply replace the values in the array declaration in the main function.
The minimum value in the array is: 2
Compile and run the program to see the minimum value of the array.
π§ How the Program Works
- The program defines a class MinValueOfArray containing a static method findMinValue that takes an array as input and returns the minimum value.
- Inside the method, it assumes the first element of the array is the minimum value.
- It then iterates through the array, updating the minimum value whenever a smaller element is encountered.
- The driver program initializes an array before calling the function and printing the result.
π§ Understanding the Concept of Minimum Value of an Array
Before diving into the code, let's understand the concept of finding the minimum value in an array.
The minimum value is the smallest element present in the array. The algorithm iterates through the array elements, updating the minimum value whenever a smaller element is encountered.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations, such as using additional data structures or algorithms, depending on the specific requirements and constraints of your application.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
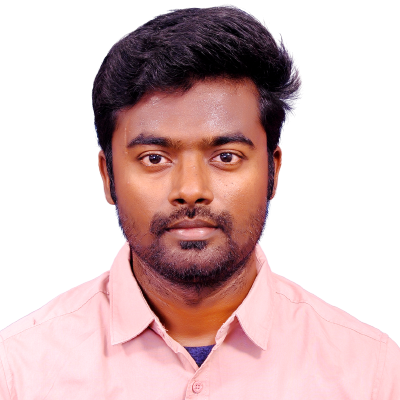
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
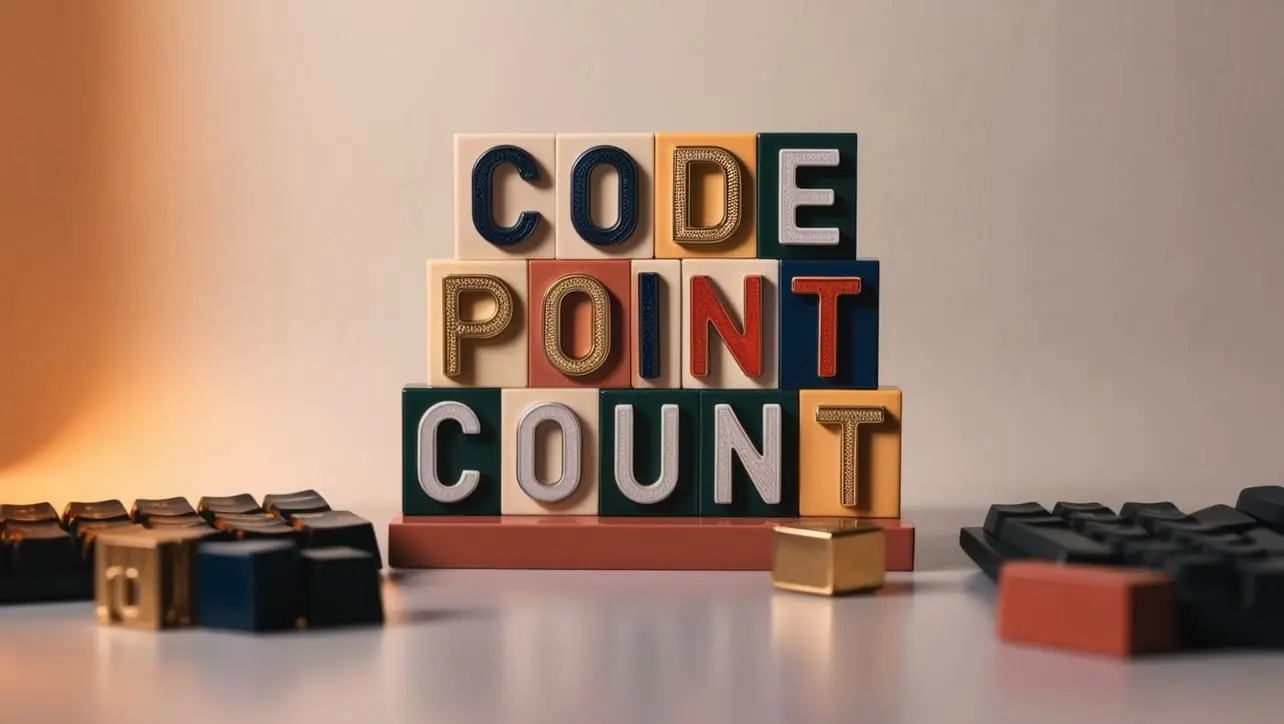
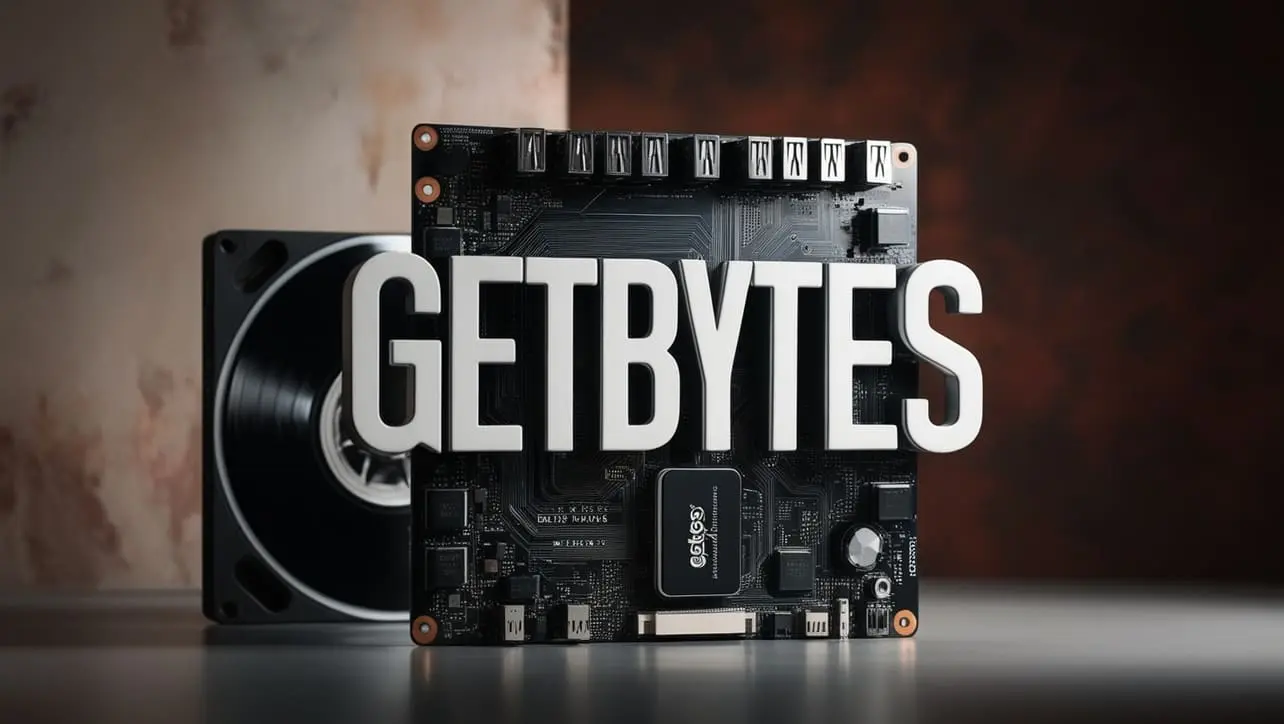
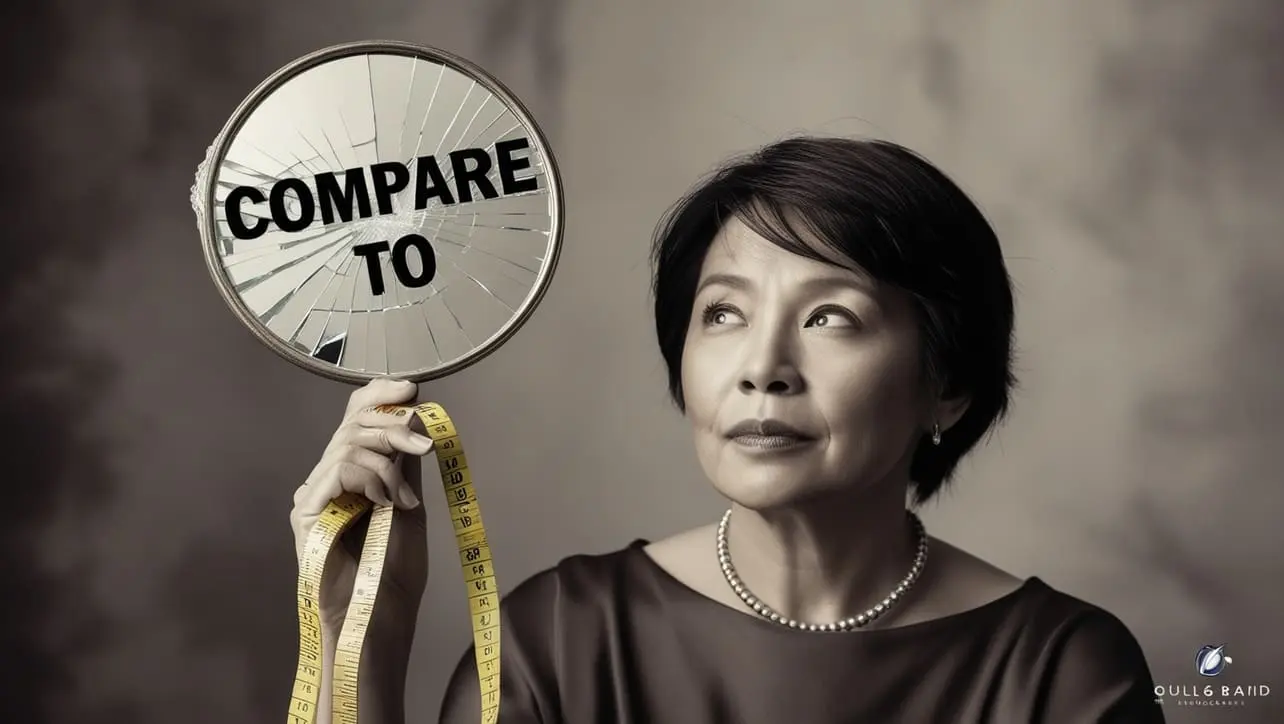
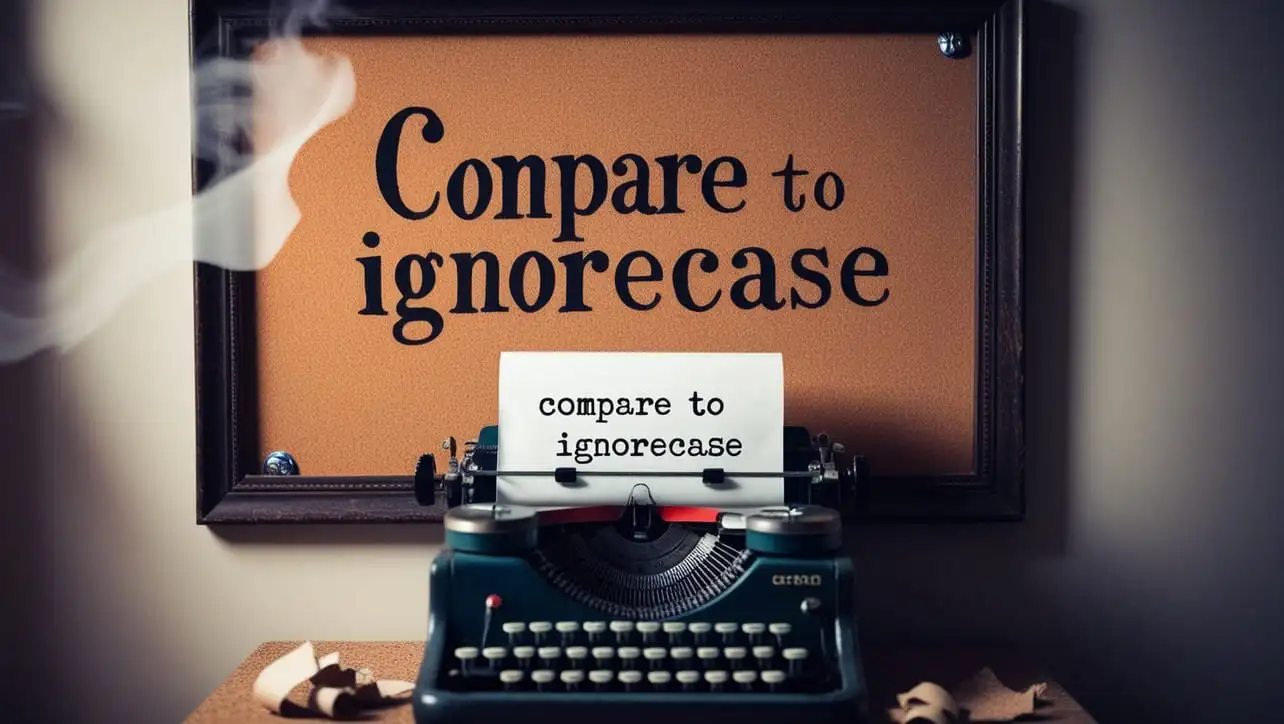
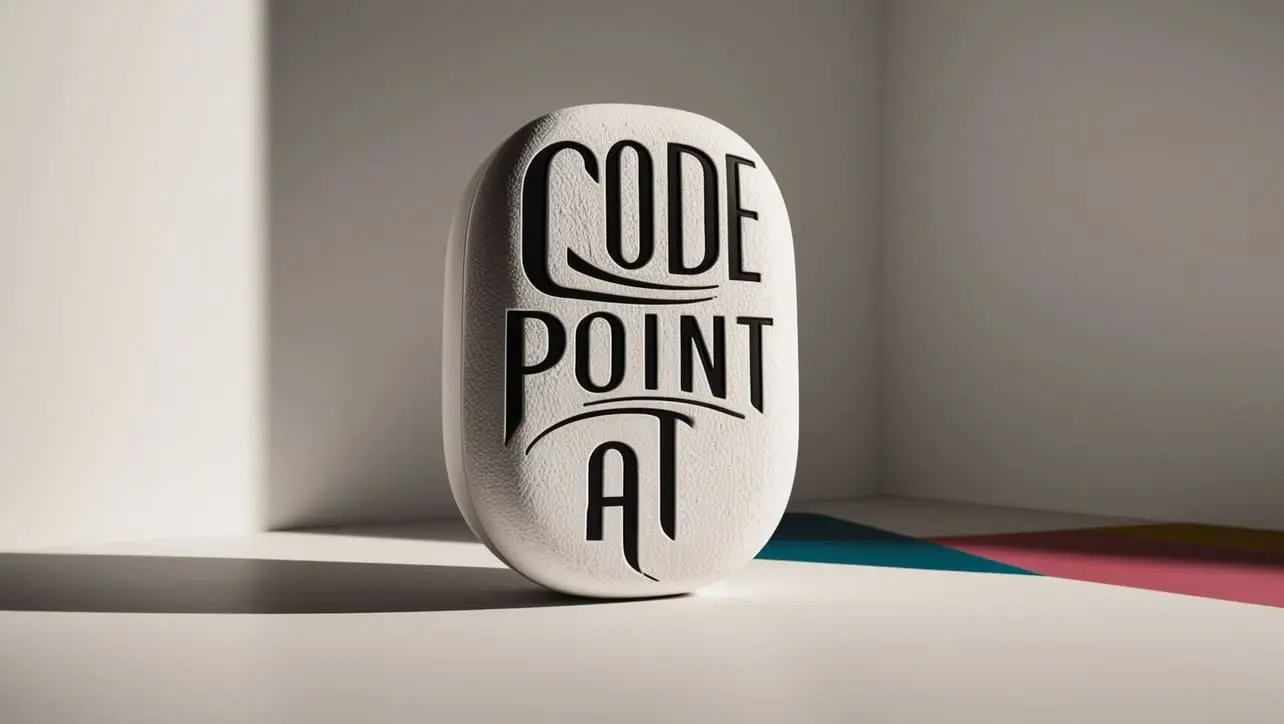
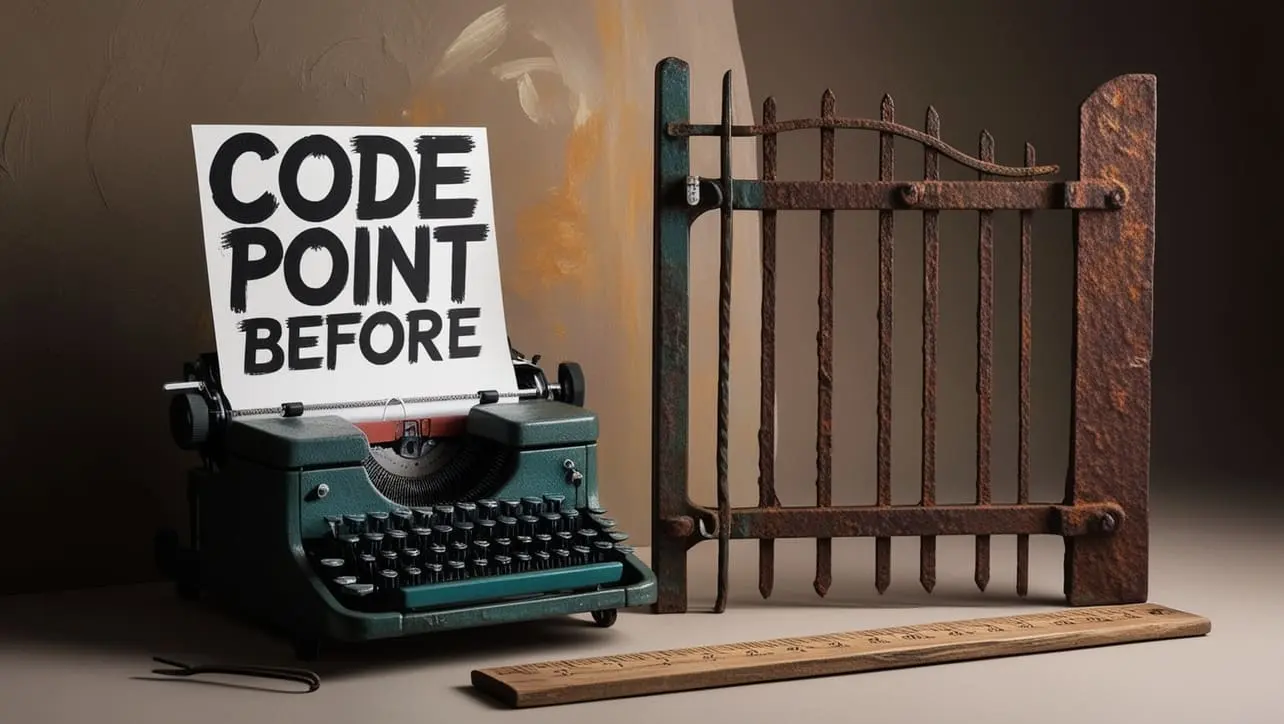
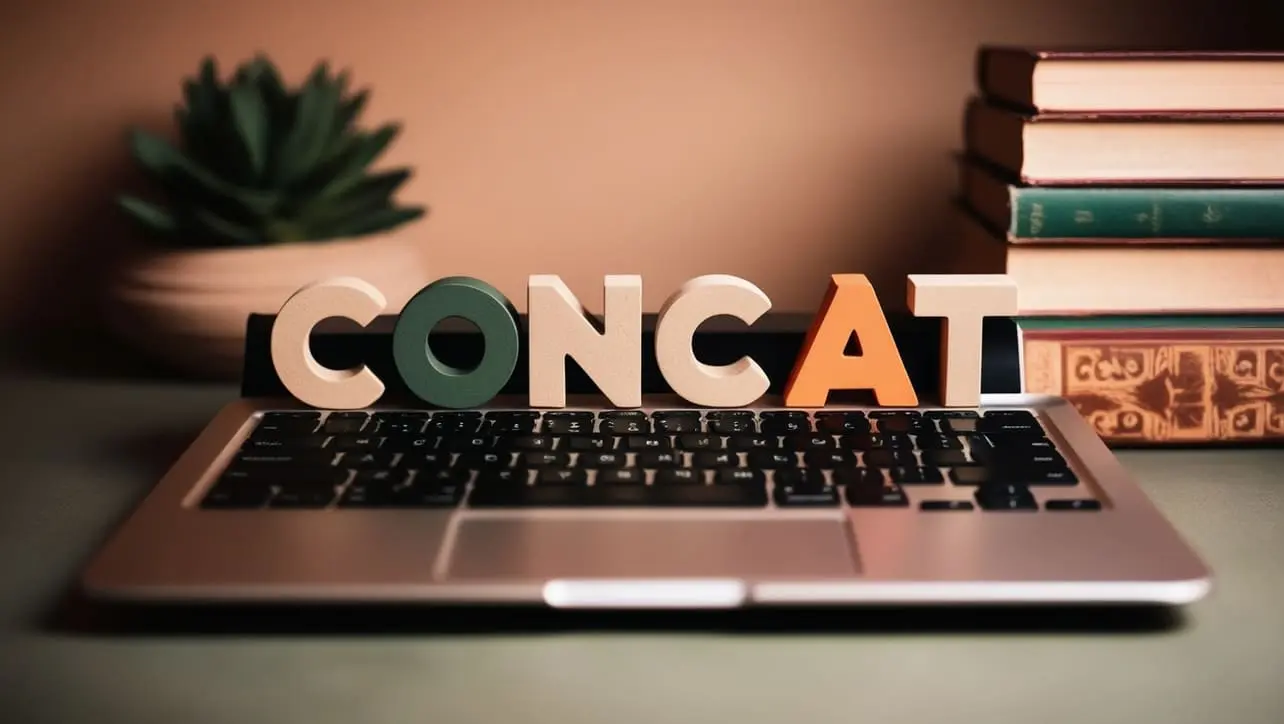
If you have any doubts regarding this article (Java Program to find Minimum Value of an Array), please comment here. I will help you immediately.