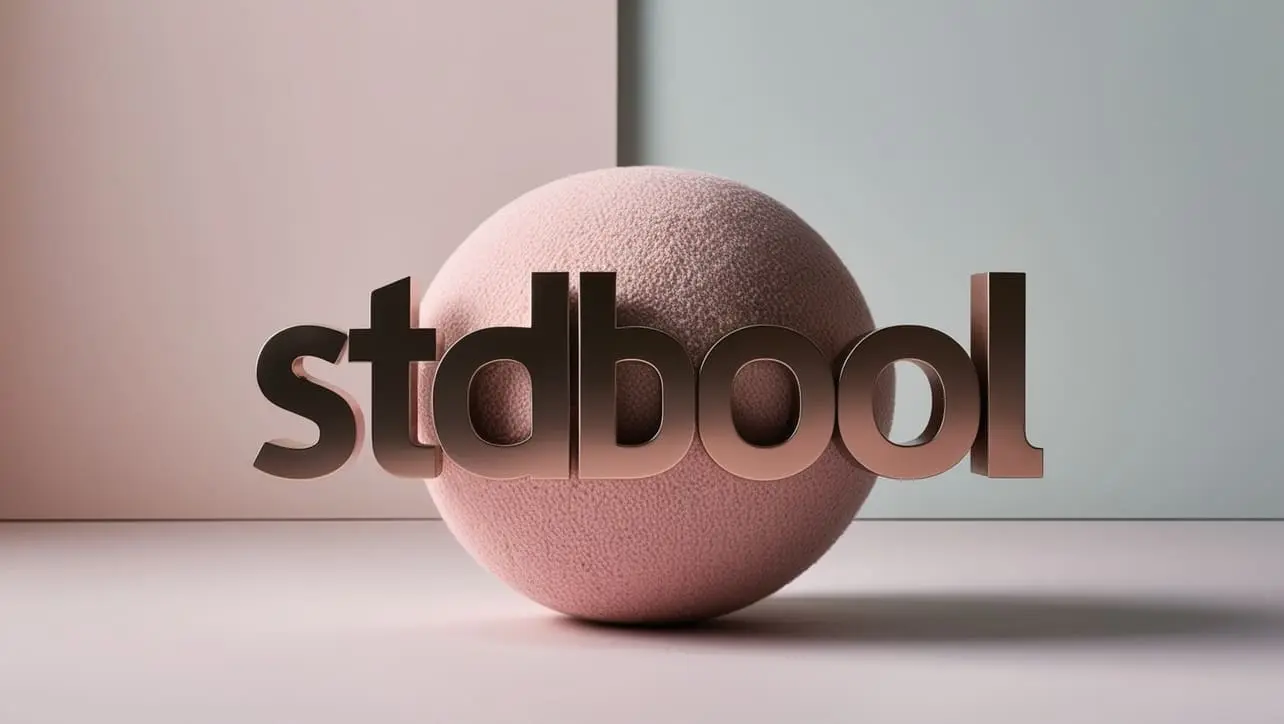
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to find Maximum Value of an Array
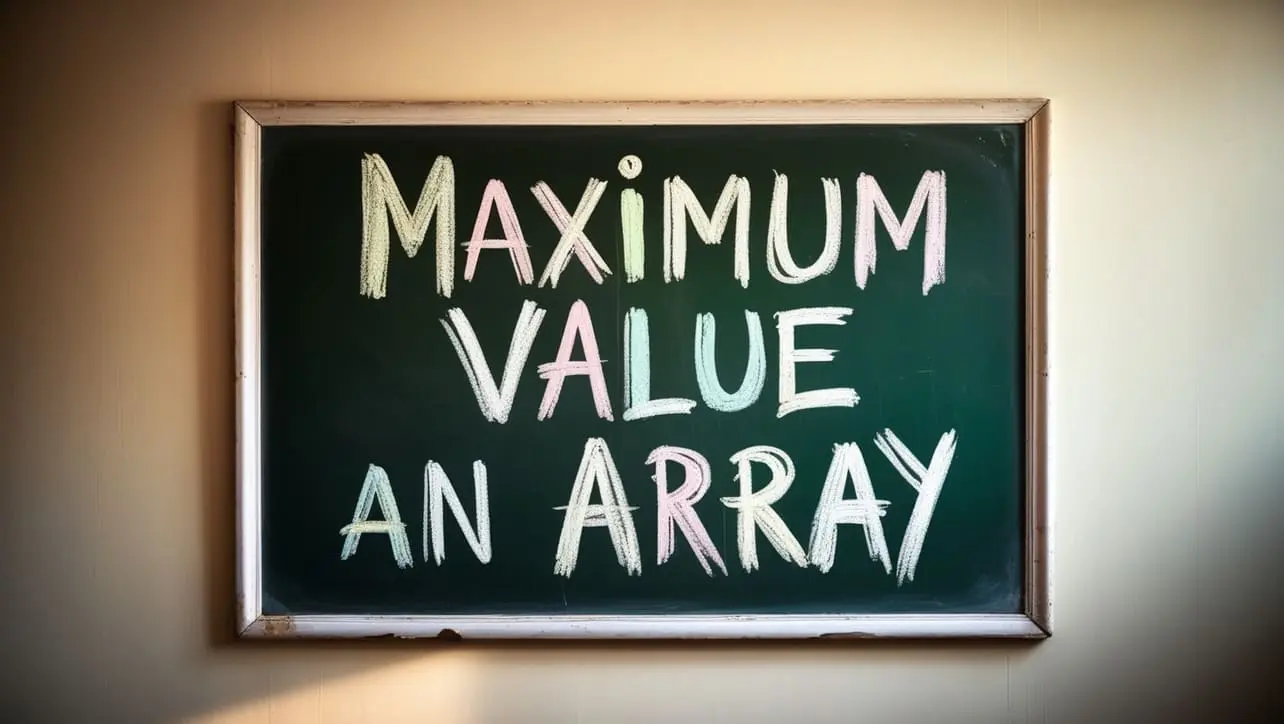
Photo Credit to CodeToFun
π Introduction
When working with arrays in C programming, it's common to perform operations like finding the maximum value stored in an array. This operation is fundamental in various applications, from data analysis to algorithmic problem-solving.
In this tutorial, we'll explore a simple yet effective C program to find the maximum value of an array.
π Example
Let's dive into the C code that achieves this functionality.
#include <stdio.h>
// Function to find the maximum value in an array
int findMax(int arr[], int size) {
// Assume the first element is the maximum
int max = arr[0];
// Iterate through the array to find the maximum
for (int i = 1; i < size; ++i) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Driver program
int main() {
// Replace these values with your array
int array[] = {14, 7, 25, 31, 10, 42};
int size = sizeof(array) / sizeof(array[0]);
// Call the function to find the maximum value
int maxValue = findMax(array, size);
// Print the result
printf("Maximum value in the array: %d\n", maxValue);
return 0;
}
π» Testing the Program
To test the program with a different array, replace the values of the array variable and adjust the size accordingly.
Maximum value in the array: 42
Compile and run the program to find the maximum value in the new array.
π§ How the Program Works
- The program defines a function findMax that takes an array and its size as input and returns the maximum value in the array.
- Inside the function, it assumes the first element of the array is the maximum.
- It then iterates through the array, comparing each element with the current maximum and updating it if a larger element is found.
- The main function initializes an array and its size, calls the findMax function, and prints the result.
π§ Understanding the Concept of Maximum Value of an Array
Understanding how to find the maximum value in an array is crucial in various programming scenarios.
The algorithm used in this program iterates through the array once, making it an efficient way to find the maximum value.
π’ Optimizing the Program
While the provided program is straightforward, there are alternative algorithms and optimizations that can be explored for finding the maximum value in an array. Consider researching more advanced techniques to enhance the efficiency of your code.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
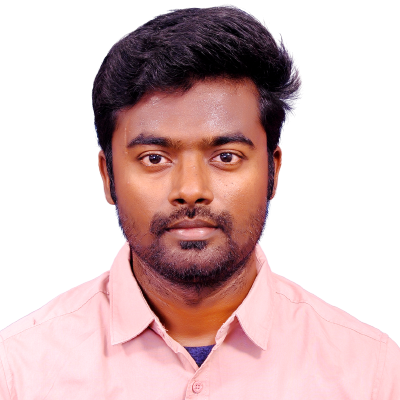
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to find Maximum Value of an Array), please comment here. I will help you immediately.