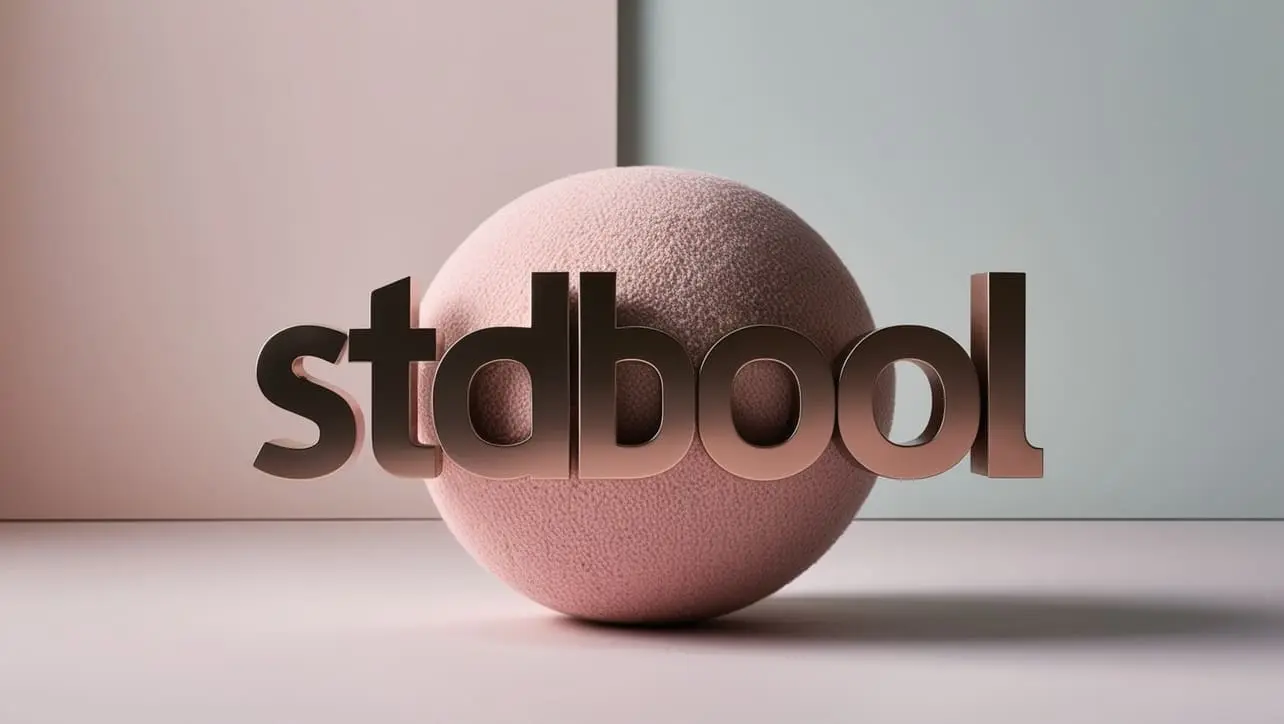
C strcoll() Function
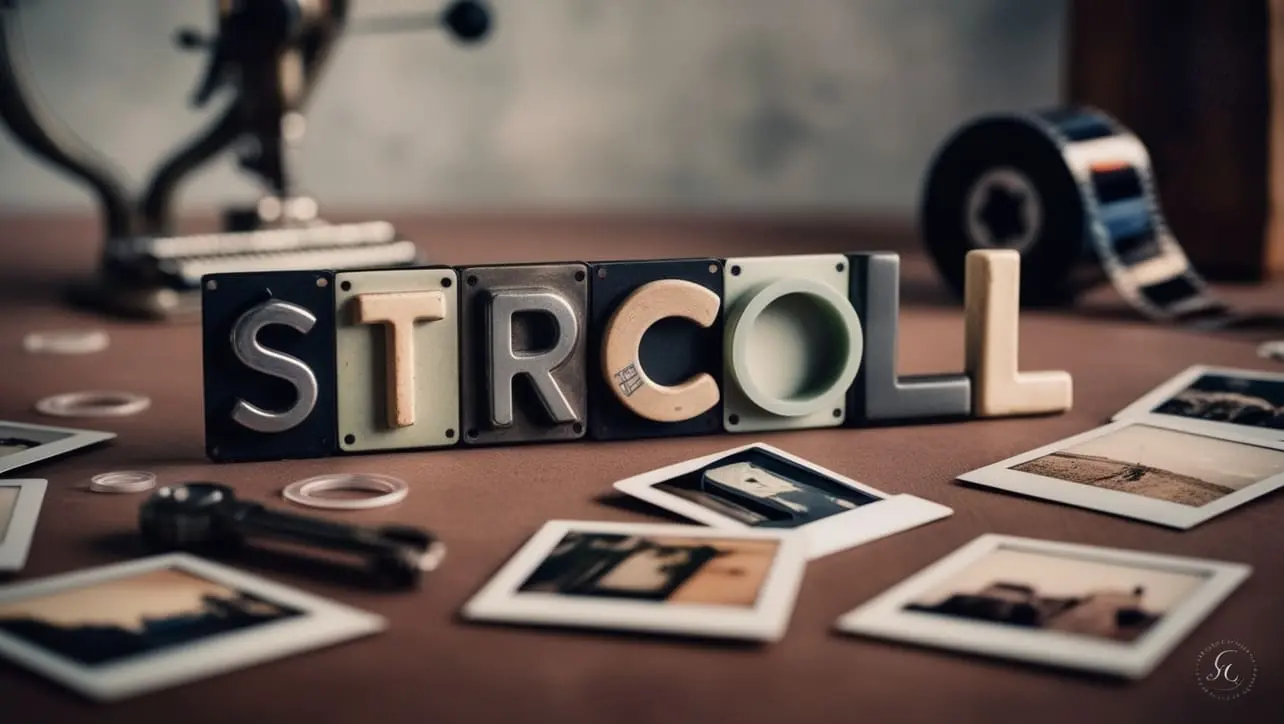
Photo Credit to CodeToFun
đ Introduction
In C programming, working with strings is a common and crucial task.
The strcoll()
function is a part of the C Standard Library, specifically in the <string.h> header.
It is used for comparing two strings based on the current locale.
In this tutorial, we'll explore the usage and functionality of the strcoll()
function in C.
đĄ Syntax
The syntax for the strcoll()
function is as follows:
int strcoll(const char *str1, const char *str2);
- str1: The first string to be compared.
- str2: The second string to be compared.
đ Example
Let's dive into an example to illustrate how the strcoll()
function works.
#include <stdio.h>
#include <string.h>
#include <locale.h>
int main() {
setlocale(LC_COLLATE, ""); // Set the locale based on the environment
const char * string1 = "apple";
const char * string2 = "Orange";
int result = strcoll(string1, string2);
if (result < 0) {
printf("%s comes before %s in the current locale.\n", string1, string2);
} else if (result > 0) {
printf("%s comes after %s in the current locale.\n", string1, string2);
} else {
printf("%s and %s are equivalent in the current locale.\n", string1, string2);
}
return 0;
}
đģ Output
apple comes after Orange in the current locale.
đ§ How the Program Works
In this example, the strcoll()
function is used to compare two strings, "apple" and "Orange," based on the current locale.
âŠī¸ Return Value
The strcoll()
function returns an integer value that indicates the relationship between the two strings:
- 0 if the strings are equal.
- Less than 0 if the first string is less than the second.
- Greater than 0 if the first string is greater than the second.
đ Common Use Cases
The strcoll()
function is particularly useful when sorting strings in a way that respects the rules of the current locale. It takes into account language-specific rules for character comparison.
đ Notes
- The setlocale() function is used to set the locale for the program. It influences the behavior of
strcoll()
. - For ASCII strings or when locale-specific comparison is not required, the strcmp() function can be used for a simpler comparison.
đĸ Optimization
The strcoll()
function is optimized for locale-specific string comparison. No additional optimization is typically needed.
đ Conclusion
The strcoll()
function in C is a valuable tool for comparing strings based on the rules of the current locale. It is essential for tasks where language-specific string comparison is required, such as sorting strings in alphabetical order.
Feel free to experiment with different strings and locales to observe how the strcoll()
function behaves in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
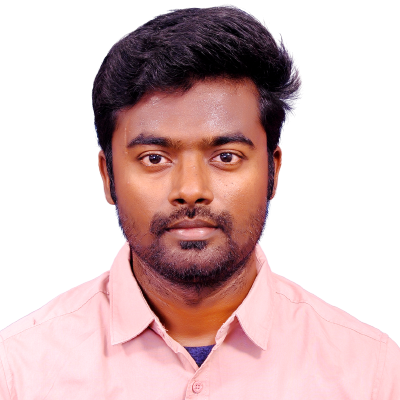
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strcoll() Function) please comment here. I will help you immediately.