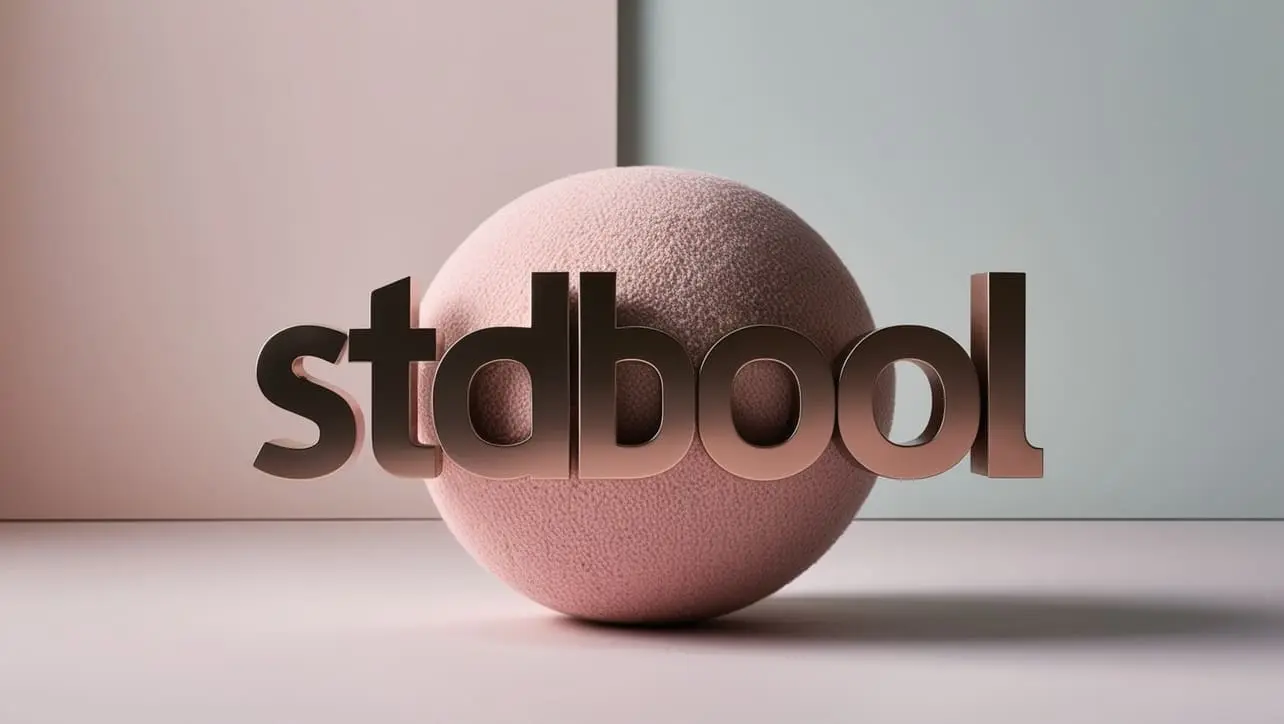
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strdup() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, working with strings is a common and essential task.
The strdup()
function is a useful standard library function that duplicates a given string.
It allocates memory for the new string and copies the content of the original string into the newly allocated memory.
In this tutorial, we'll explore the usage and functionality of the strdup()
function in C.
đĄ Syntax
The syntax for the strdup()
function is as follows:
char *strdup(const char *source);
- source: The string to be duplicated.
đ Example
Let's dive into an example to illustrate how the strdup()
function works.
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
const char * originalString = "Hello, C!";
// Duplicate the string
char * copiedString = strdup(originalString);
// Check if the duplication was successful
if (copiedString != NULL) {
printf("Original: %s\n", originalString);
printf("Copied: %s\n", copiedString);
// Free the memory allocated by strdup
free(copiedString);
} else {
fprintf(stderr, "Memory allocation failed.\n");
}
return 0;
}
đģ Output
Original: Hello, C! Copied: Hello, C!
đ§ How the Program Works
In this example, the strdup()
function is used to duplicate the string "Hello, C!" into a new dynamically allocated memory. The original and copied strings are then printed, and the dynamically allocated memory is freed using the free() function.
âŠī¸ Return Value
The strdup()
function returns a pointer to the duplicated string if the allocation is successful. If there is insufficient memory, it returns NULL.
đ Common Use Cases
The strdup()
function is particularly useful when you need to create a duplicate of a string and modify the duplicated string without affecting the original. It simplifies memory management by handling the allocation for you.
đ Notes
- Remember to free the memory allocated by
strdup()
using the free() function to prevent memory leaks. - If you're working in an environment where
strdup()
is not available, you can implement a similar function using malloc() and strcpy().
đĸ Optimization
The strdup()
function is optimized for convenience, but keep in mind that it involves dynamic memory allocation, which can lead to memory fragmentation if not managed properly. Be mindful of freeing the allocated memory when it is no longer needed.
đ Conclusion
The strdup()
function in C is a valuable tool for duplicating strings and simplifying memory management in string operations. It provides a convenient way to create independent copies of strings for manipulation.
Feel free to experiment with different strings and explore the behavior of the strdup()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
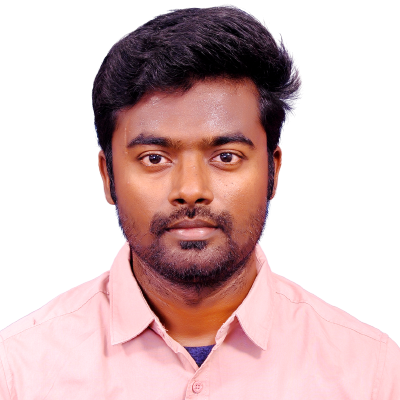
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strdup() Function) please comment here. I will help you immediately.