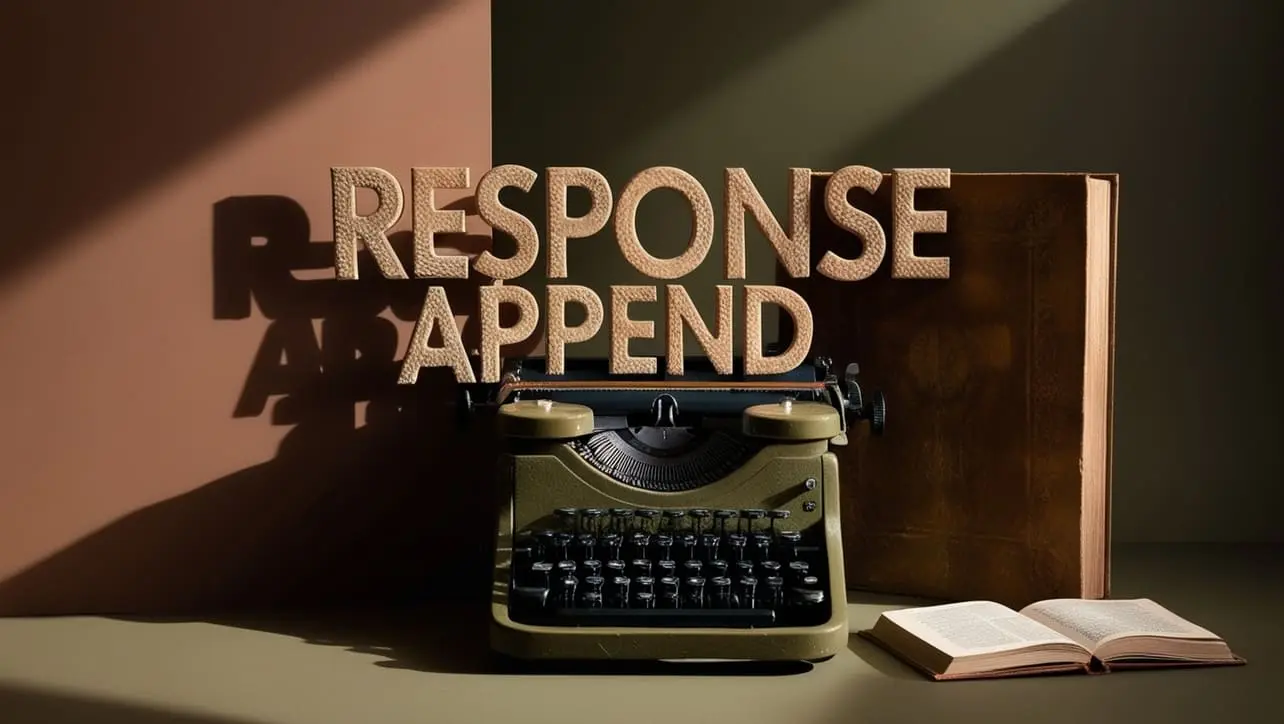
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.all() Method
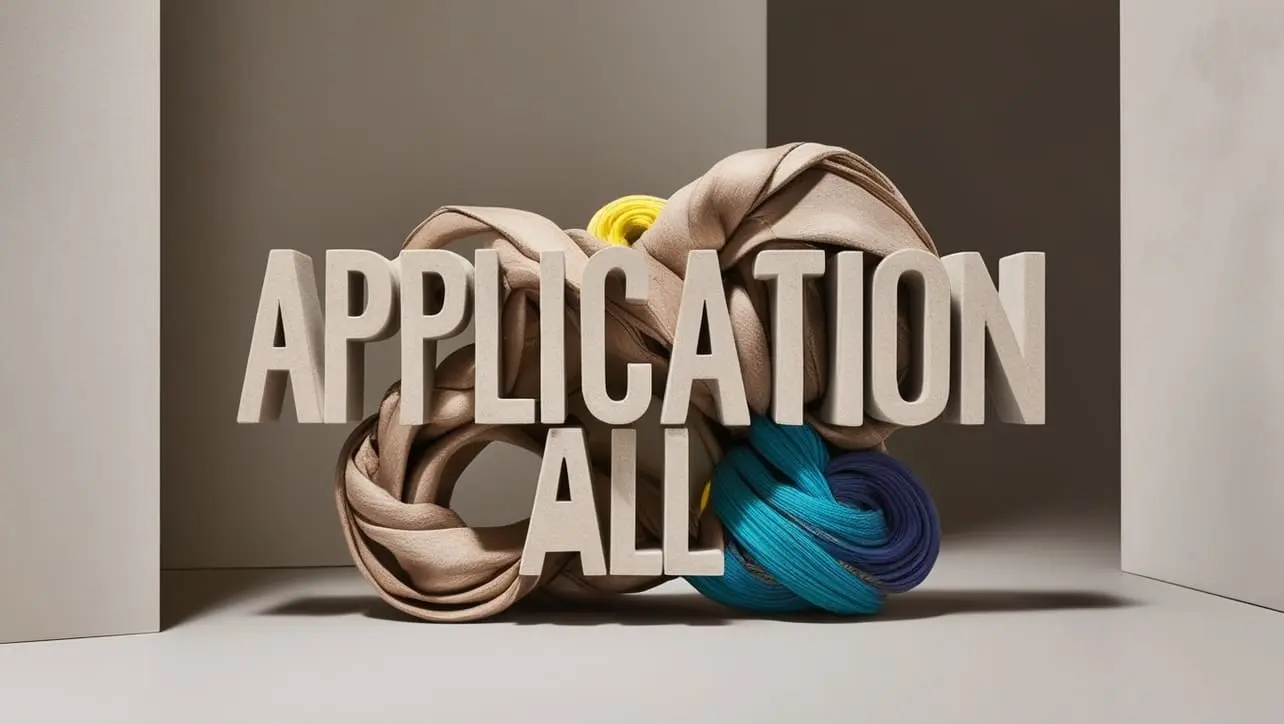
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a powerful web application framework for Node.js, provides developers with a wide range of tools to handle HTTP requests. One such versatile tool is the app.all()
method, designed to handle all HTTP methods for a specific route.
In this guide, we'll explore the syntax, usage, and practical examples of the app.all()
method to empower you in building flexible and efficient Express.js applications.
💡 Syntax
The syntax for the app.all()
method is simple:
app.all(path, callback)
- path: A string representing the route or path for which the middleware function is invoked.
- callback: The middleware function that handles requests for the specified route.
❓ How app.all() Works
Unlike specific HTTP method handlers like app.get() or app.post(), app.all()
is not restricted to a particular HTTP method. It matches all HTTP methods (GET, POST, PUT, DELETE, etc.) for the specified route. This makes it a convenient choice when you want a middleware function to be executed regardless of the HTTP method used.
app.all('/api/*', (req, res, next) => {
console.log(`Request received for path: ${req.path}`);
next();
});
In this example, the middleware logs information for all requests under the '/api/' route, regardless of the HTTP method used.
📚 Use Cases
Logging Middleware:
This middleware logs information for all requests under the /api/ route, regardless of the HTTP method used.
example.jsCopiedapp.all('/api/*', (req, res, next) => { console.log(`Request received for path: ${req.path}`); next(); });
Authentication:
Here, the middleware ensures that only authenticated users can access routes under /admin/.
example.jsCopiedapp.all('/admin/*', (req, res, next) => { // Check if the user is authenticated if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } });
🏆 Best Practices
Order Matters:
When using multiple
app.all()
handlers, the order in which they are defined matters. Express executes them in the order they are declared, so place more specific routes before generic ones.example.jsCopied// Specific route handler app.all('/api/users', (req, res, next) => { // Specific logic for /api/users next(); }); // Generic route handler app.all('/api/*', (req, res, next) => { // Generic logic for /api/* next(); });
Keep It DRY (Don't Repeat Yourself):
If you find yourself duplicating code across multiple routes, consider using a separate function and calling it from each route handler.
example.jsCopiedconst logRequest = (req, res, next) => { console.log(`Request received for path: ${req.path}`); next(); }; app.all('/api/*', logRequest); app.all('/admin/*', logRequest);
🎉 Conclusion
The app.all()
method in Express.js is a powerful tool for handling middleware functions across all HTTP methods for a specified route. Understanding its use cases and best practices will empower you to build robust and maintainable Express applications.
Now, go ahead and leverage the flexibility of app.all()
in your Express.js projects!
👨💻 Join our Community:
Author
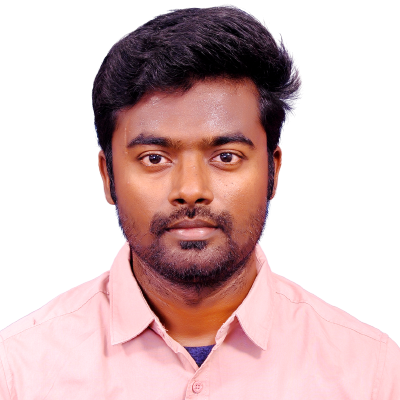
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.all() Method), please comment here. I will help you immediately.