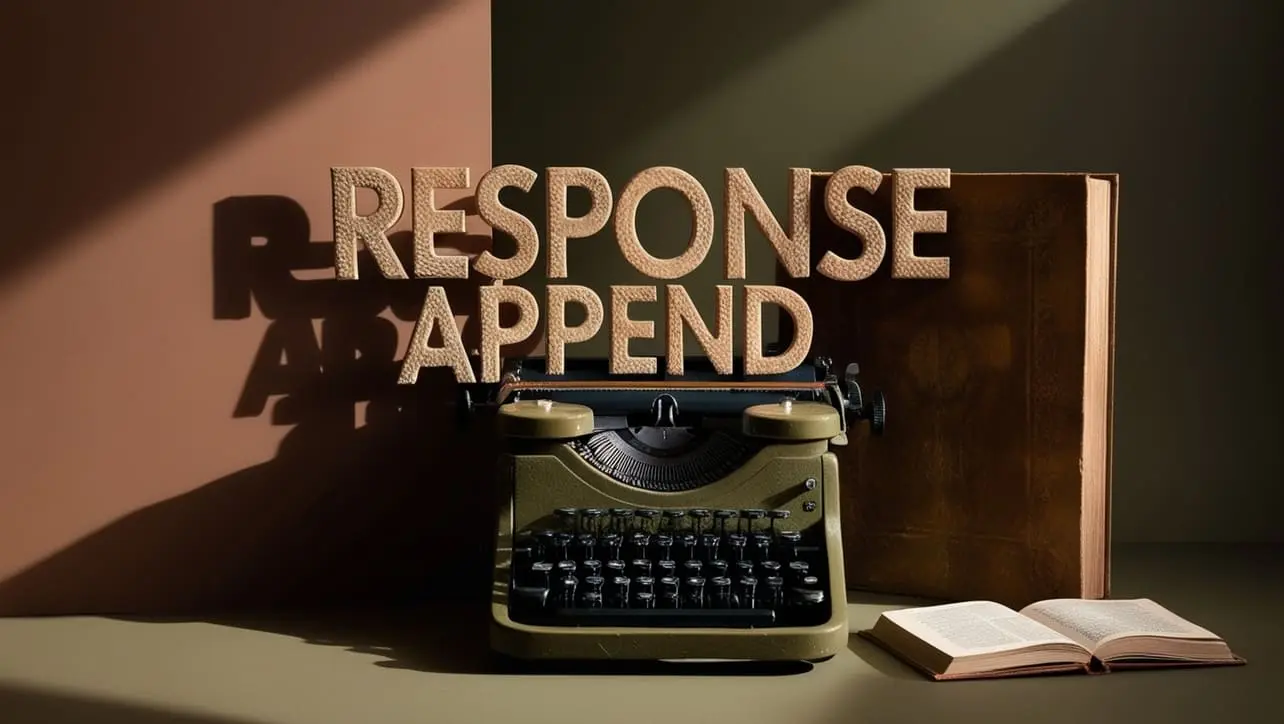
Express.js Router
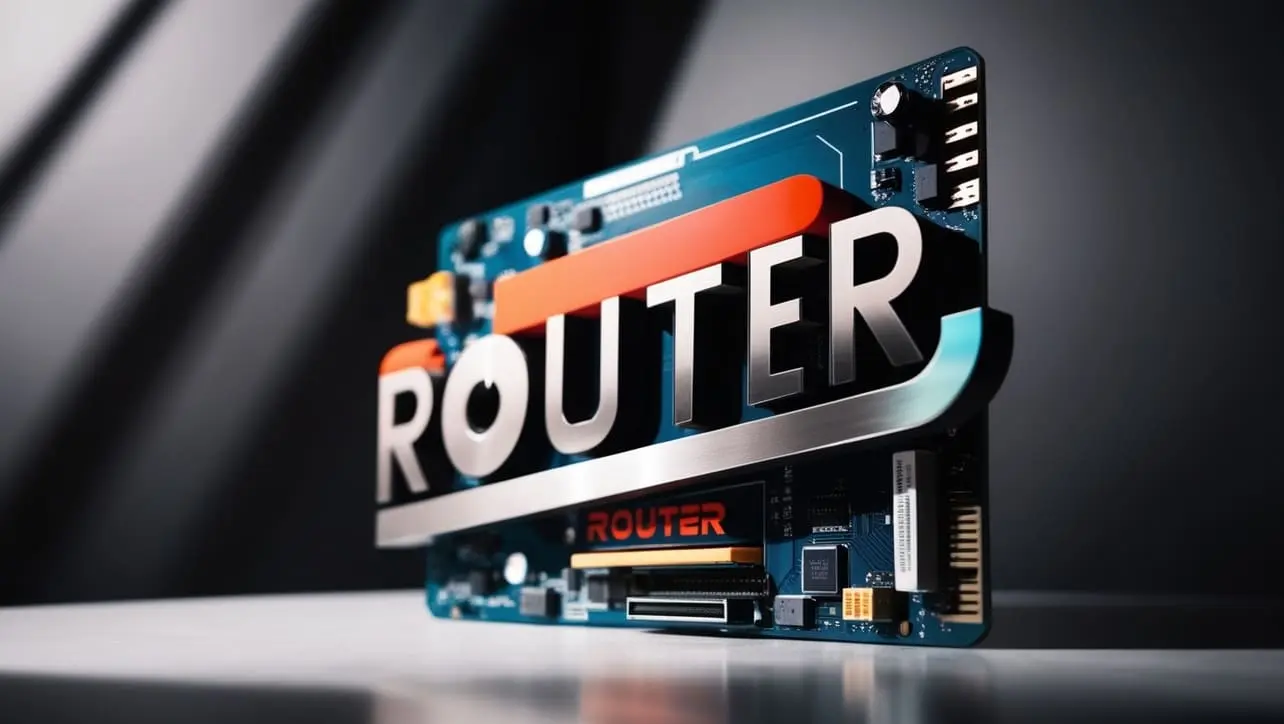
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of Express.js, the Router
is a powerful tool that aids in organizing and modularizing route handling. It allows developers to create modular sets of routes, enhancing code organization and maintainability.
This page serves as a comprehensive guide to the Router
in Express.js, providing insights into its structure, usage, and best practices.
🧐 What is the Express.js Router?
The Router
in Express.js is a middleware that helps in defining and organizing routes in a more modular and structured manner. It allows developers to group related routes together and provides a mechanism for creating a mini-application with its own set of routes and middleware.
The Router
plays a key role in breaking down the application into smaller, manageable components, improving code readability and maintainability.
🏁 Initializing the Express.js Router
To utilize the Router, it needs to be initialized using the express.Router() method. This method returns an instance of a router
that can be used to define routes and middleware specific to that router.
const express = require('express');
const router = express.Router();
This creates an instance of the Router, and you can now define routes and middleware for this specific router.
🧠 Anatomy of the Express.js Router
Basic Structure:
The
Router
object is similar to the main app object but provides a more focused scope for defining routes and middleware. Routes can be defined in the same way as in the main application, making it easy to group related functionality.
🔑 Key Features and Methods
router.get(), router.post(), etc.:
The
Router
object allows you to define routes using HTTP methods such as get, post, put, delete, etc. This is similar to how routes are defined in the main application using the app object.router.jsCopied// Define a route using the Router router.get('/users', (req, res) => { res.send('List of users'); });
Middleware with router.use():
Middleware functions can be applied to a specific
router
using the router.use() method. This allows you to add middleware that will only affect routes defined within that router.router-use.jsCopied// Middleware function for the router const routerMiddleware = (req, res, next) => { console.log('Middleware for the router'); next(); }; // Apply middleware to the router router.use(routerMiddleware);
Mounting the Router:
Once routes and middleware are defined within the Router, it needs to be mounted on the main Express application. This is done using the app.use() method.
mounting-router.jsCopied// Mount the router on the main app app.use('/api', router);
In this example, all routes defined in the
Router
will be prefixed with /api.
🚀 Advanced Features
Nested Routers:
Routers can be nested within each other, allowing for even more modularization. This is particularly useful for breaking down complex applications into smaller, manageable components.
nested-routers.jsCopiedconst nestedRouter = express.Router(); nestedRouter.get('/example', (req, res) => { res.send('Nested router example'); }); router.use('/nested', nestedRouter);
In this example, the nested
router
is mounted under the /nested path in the main router.
📝 Example
Let's illustrate the usage of the Router
with a comprehensive example:
const express = require('express');
const app = express();
const router = express.Router();
// Middleware function for the router
const routerMiddleware = (req, res, next) => {
console.log('Middleware for the router');
next();
};
// Apply middleware to the router
router.use(routerMiddleware);
// Define a route using the Router
router.get('/users', (req, res) => {
res.send('List of users');
});
// Mount the router on the main app
app.use('/api', router);
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, a simple Router
is defined with a middleware and a route. The router
is then mounted on the main application under the /api path.
🎉 Conclusion
In conclusion, the Router
in Express.js is a powerful tool for organizing and modularizing route handling in your applications. It provides a structured way to group related routes and middleware, enhancing code organization and maintainability.
This overview has covered the basic structure, key methods, and advanced features of the Router, empowering you to efficiently manage routes in your Express.js applications.
👨💻 Join our Community:
Author
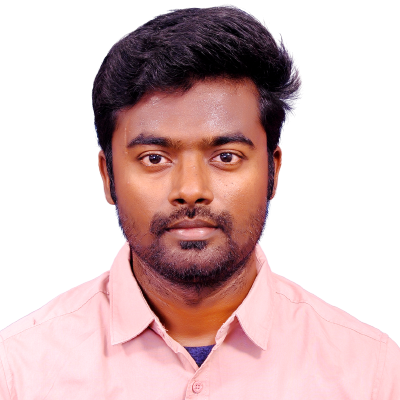
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js Router), please comment here. I will help you immediately.