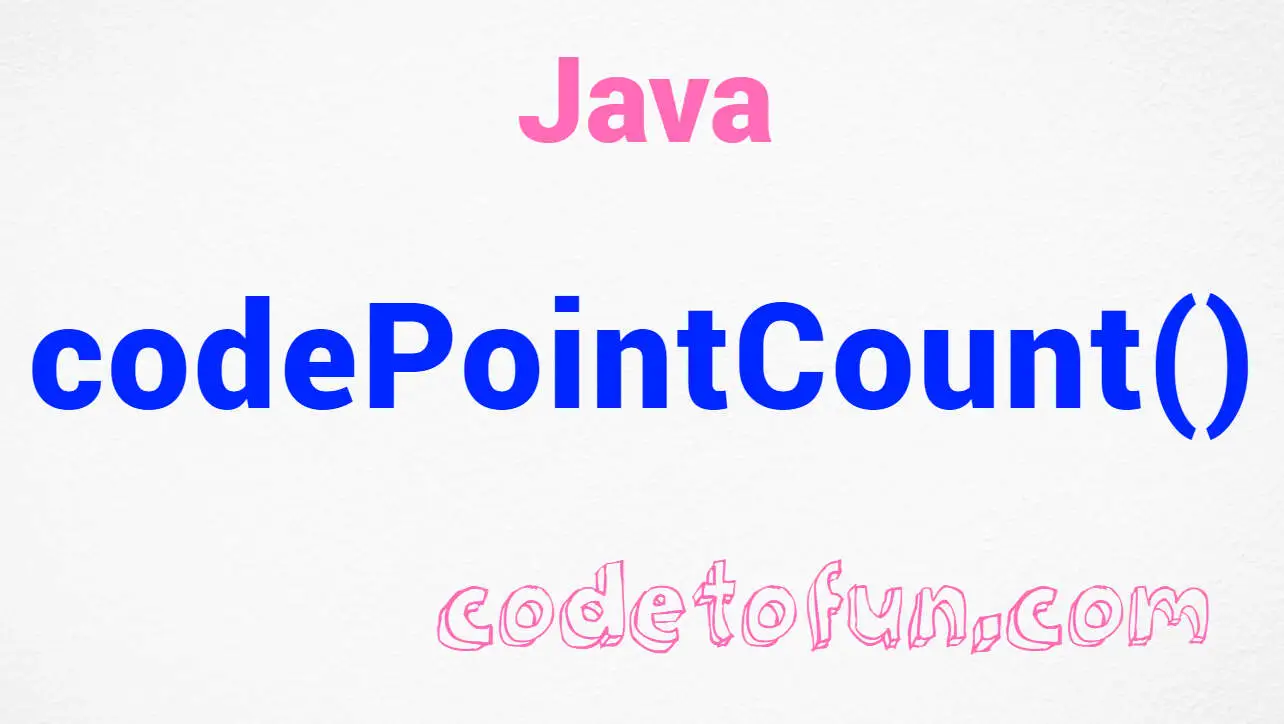
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Check Disarium Number
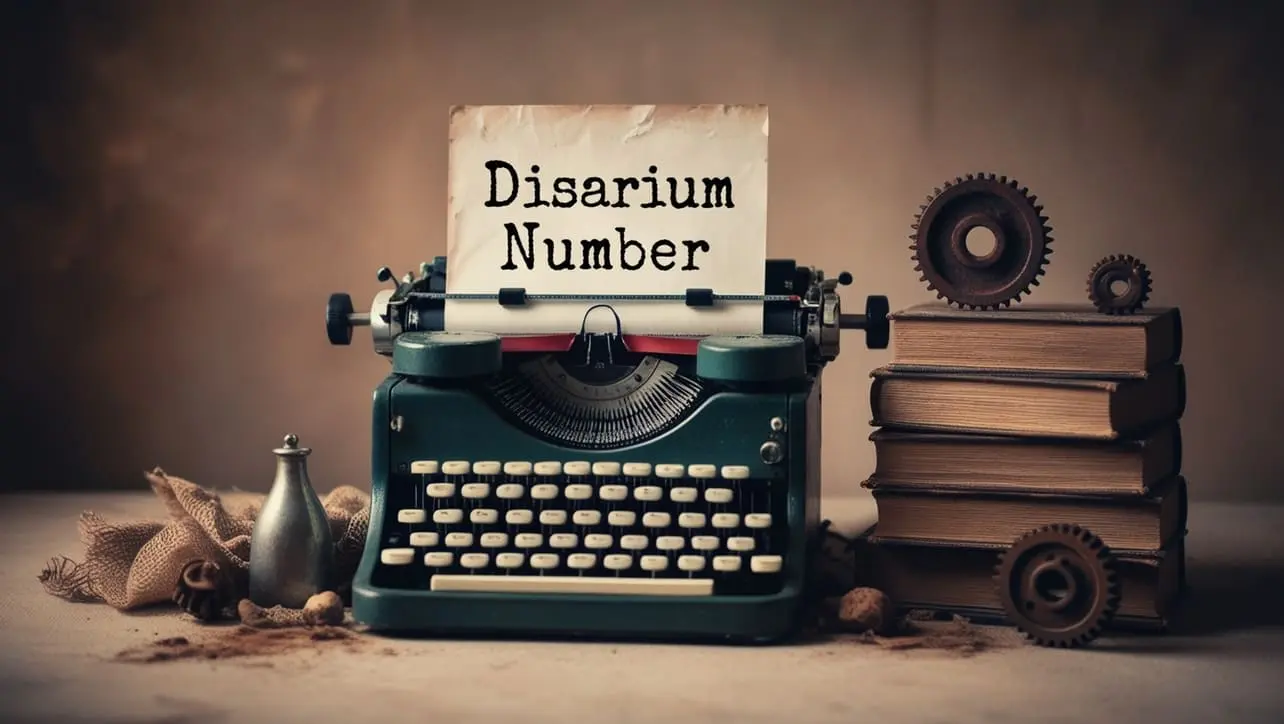
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring mathematical patterns is a fascinating endeavor. One such numeric pattern is the Disarium number.
A Disarium number is a number defined by the sum of its digits each raised to the power of its respective position.
In this tutorial, we'll delve into a Java program designed to check whether a given number is a Disarium number.
π Example
Let's explore the Java code that checks whether a given number is a Disarium number.
public class DisariumChecker {
// Function to count the number of digits in a given number
static int countDigits(int number) {
int count = 0;
while (number != 0) {
count++;
number /= 10;
}
return count;
}
// Function to check if a number is a Disarium number
static boolean isDisarium(int number) {
int originalNumber = number;
int digitCount = countDigits(number);
int sum = 0;
while (number != 0) {
int digit = number % 10;
sum += Math.pow(digit, digitCount);
digitCount--;
number /= 10;
}
return (sum == originalNumber);
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired number
int inputNumber = 89;
// Check if the number is Disarium
if (isDisarium(inputNumber)) {
System.out.println(inputNumber + " is a Disarium number.");
} else {
System.out.println(inputNumber + " is not a Disarium number.");
}
}
}
π» Testing the Program
To test the program with different numbers, replace the value of inputNumber in the main method.
89 is a Disarium number.
Compile and run the program to check if the number is a Disarium number.
π§ How the Program Works
- The program defines a class DisariumChecker containing static methods to count the number of digits and check if a number is a Disarium number.
- The isDisarium method checks if a number is a Disarium number by summing the digits each raised to the power of its position.
- The main method tests the isDisarium method with a sample number (replace it with your desired number).
π Between the Given Range
Let's dive into the Java code that checks for Disarium numbers in the specified range.
public class DisariumChecker {
// Function to check if a number is a Disarium number
static boolean isDisarium(int number) {
int originalNumber = number;
int sum = 0;
int position = 1;
while (number > 0) {
int digit = number % 10;
sum += Math.pow(digit, position);
number /= 10;
position++;
}
return sum == originalNumber;
}
// Driver program
public static void main(String[] args) {
System.out.print("Disarium numbers in the range 1 to 100: ");
for (int i = 1; i <= 100; i++) {
if (isDisarium(i)) {
System.out.print(i + " ");
}
}
}
}
π» Testing the Program
Disarium numbers in the range 1 to 100: 1 2 3 4 5 6 7 8 9 89
Simply compile and run the program to see the Disarium numbers in the specified range.
π§ How the Program Works
- The program defines a class DisariumChecker containing a static method isDisarium that checks if a number is a Disarium number.
- Inside the method, it iterates through each digit of the number, raising it to the power of its respective position and accumulating the sum.
- The main function tests the Disarium check for numbers in the range from 1 to 100 and prints the Disarium numbers.
π§ Understanding the Concept of Disarium Numbers
Before we dive into the code, let's grasp the concept of Disarium numbers.
A Disarium number is a number such that the sum of its digits, each raised to the power of its position, equals the number itself.
For example, the number 89 is a Disarium number because 8^1 + 9^2 equals 89.
π Conclusion
Understanding and implementing programs to identify numeric patterns, such as Disarium numbers, is a valuable skill in the world of programming.
The provided java program offers a practical example of checking whether a given number follows the Disarium pattern.
Feel free to use and modify this code for your specific use cases. Happy coding!
π¨βπ» Join our Community:
Author
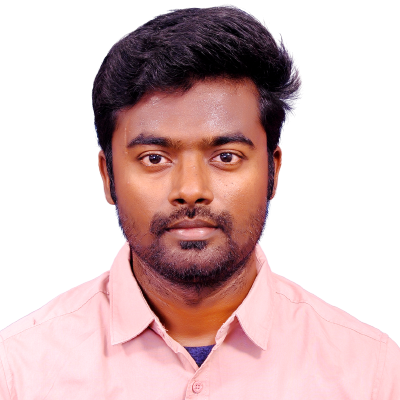
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Check Disarium Number), please comment here. I will help you immediately.