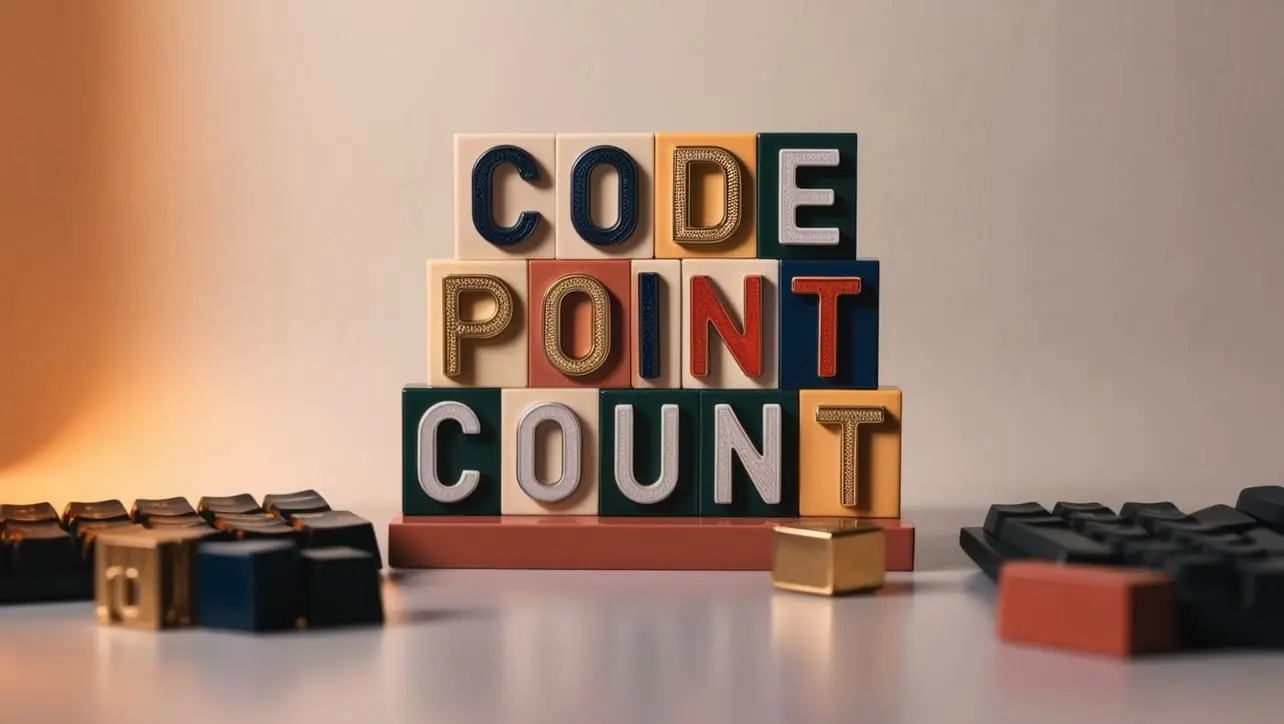
Java Topics
- Java Intro
- Java String Methods
- Java Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Java Star Pattern
- Java Number Pattern
- Java Alphabet Pattern
Java Program to Swap Two Numbers
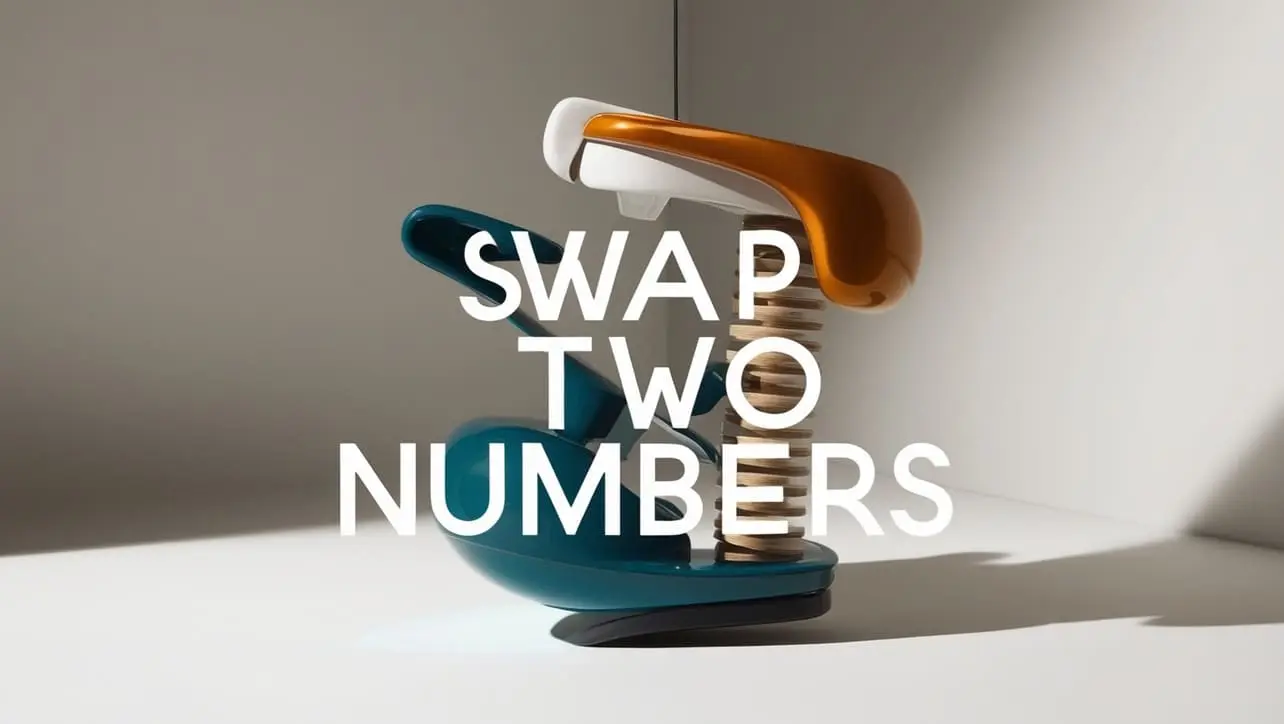
Photo Credit to CodeToFun
π Introduction
In the world of programming, performing basic operations on numbers is a fundamental task. Swapping two numbers is one such operation that involves exchanging the values of two variables. This operation is commonly used in various algorithms and programs.
In this tutorial, we will explore a simple Java program designed to swap the values of two numbers.
π Example
Let's delve into the Java code that accomplishes this task.
import java.util.Scanner;
public class SwapNumbers {
// Function to swap two numbers
static void swapNumbers(int[] arr) {
// Ensure that the array has exactly two elements
if (arr.length == 2) {
// Swap the elements using a temporary variable
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
} else {
System.out.println("Array must have exactly two elements.");
}
}
// Driver program
public static void main(String[] args) {
// Replace these values with the numbers you want to swap
int[] numbers = {
5,
10
};
System.out.printf("Before swapping: num1 = %d, num2 = %d%n", numbers[0], numbers[1]);
// Call the function to swap the numbers
swapNumbers(numbers);
System.out.printf("After swapping: num1 = %d, num2 = %d%n", numbers[0], numbers[1]);
}
}
π» Testing the Program
To test the program with different numbers, modify the values of the numbers array in the main method.
Before swapping: num1 = 5, num2 = 10 After swapping: num1 = 10, num2 = 5
Compile and run the program to see the result of swapping the two numbers.
π§ How the Program Works
- The program defines a class SwapNumbers containing a static method swapNumbers that takes an array of integers as a parameter and swaps the values of the two elements in the array.
- Inside the main method, replace the values of the numbers array with the numbers you want to swap.
- The program prints the values of the two numbers before and after swapping.
π§ Understanding the Concept of Swapping Two Numbers
Swapping two numbers involves exchanging their values. In the provided program, a temporary variable is used to hold the value of one variable while the values of the two variables are exchanged.
π’ Optimizing the Program
While the provided program is a straightforward way to swap two numbers, consider exploring and implementing alternative approaches or optimizations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!.
π¨βπ» Join our Community:
Author
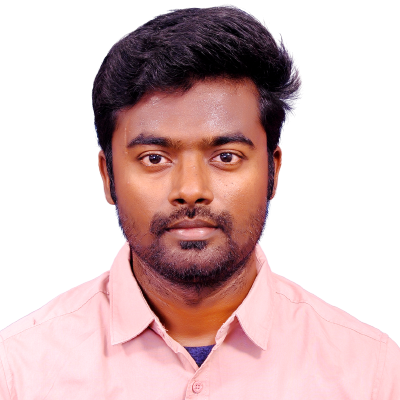
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
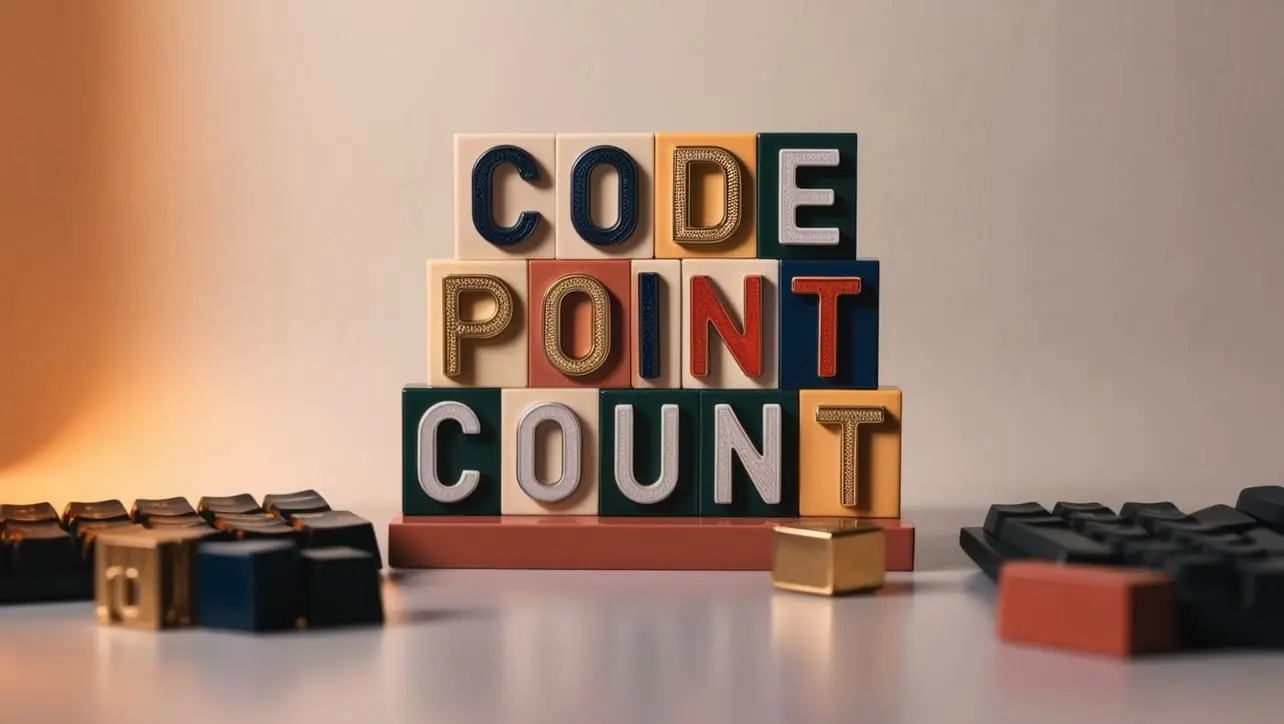
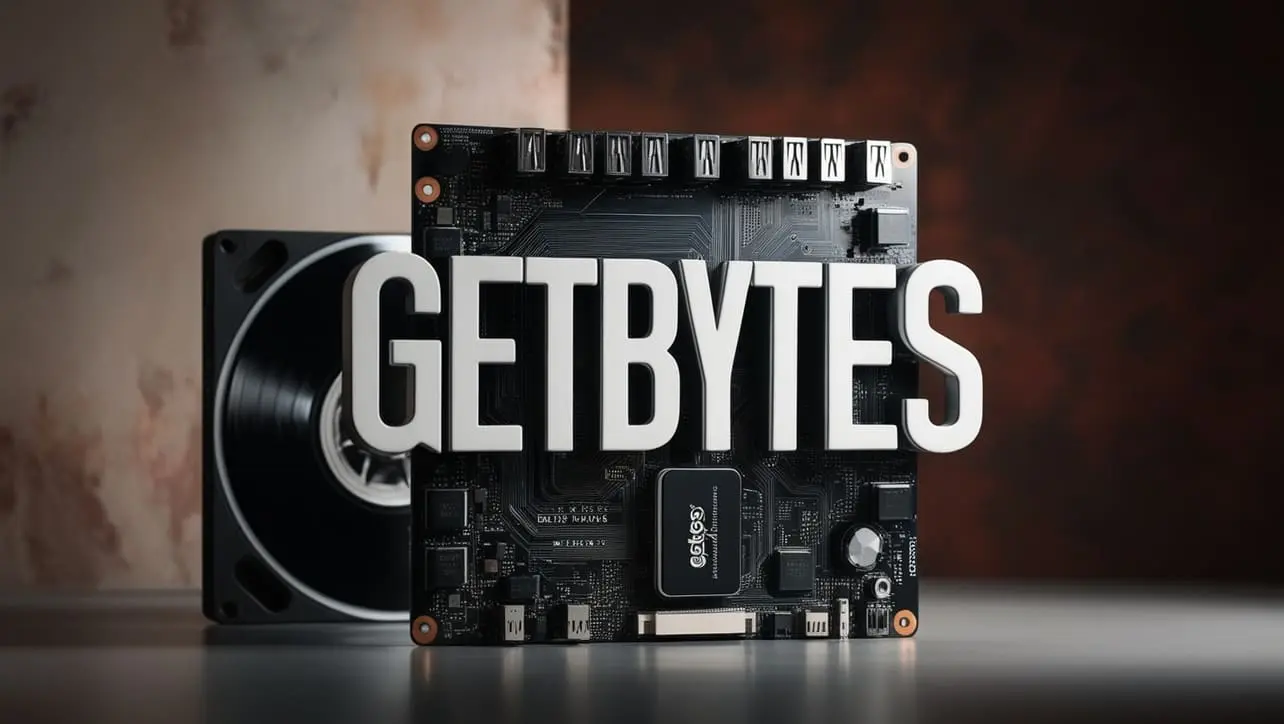
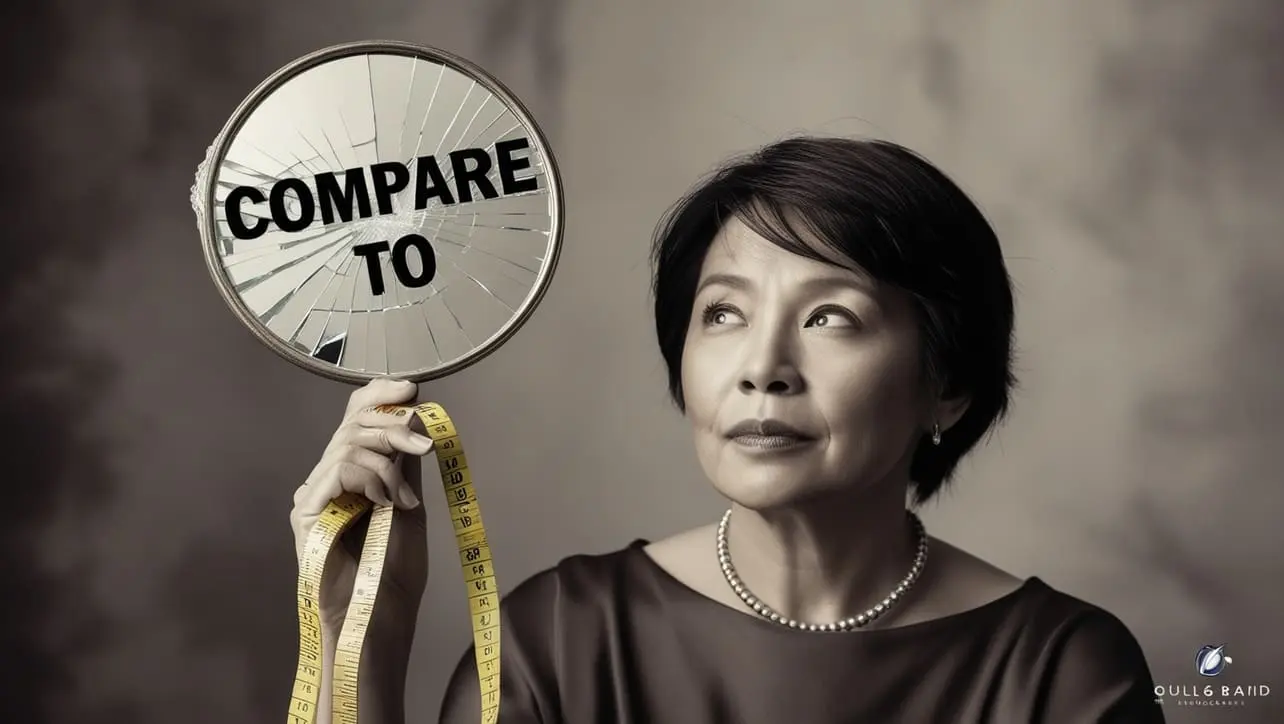
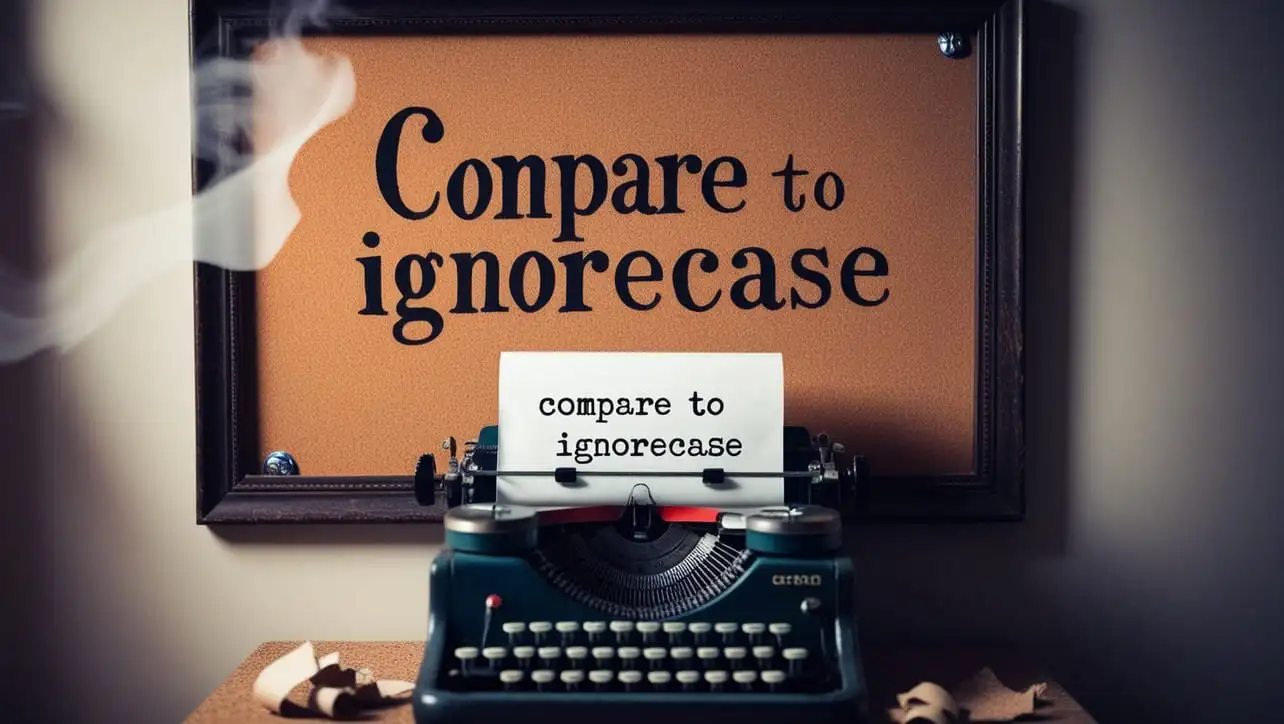
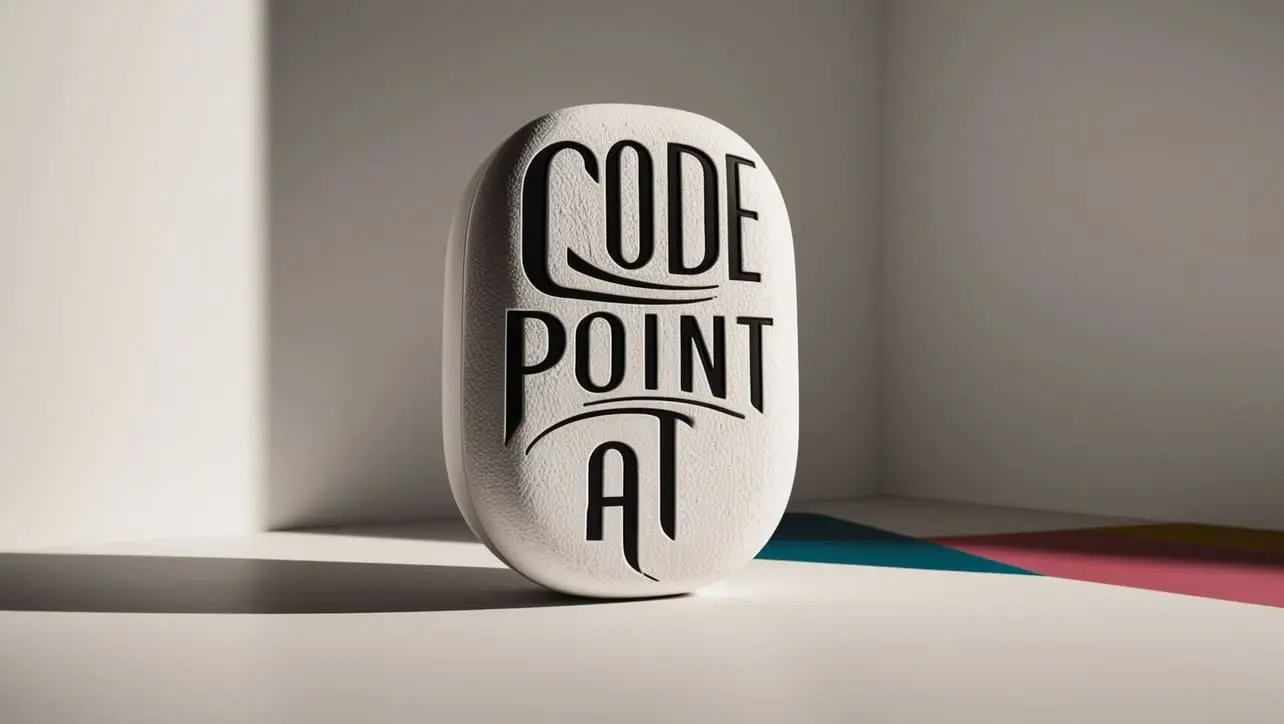
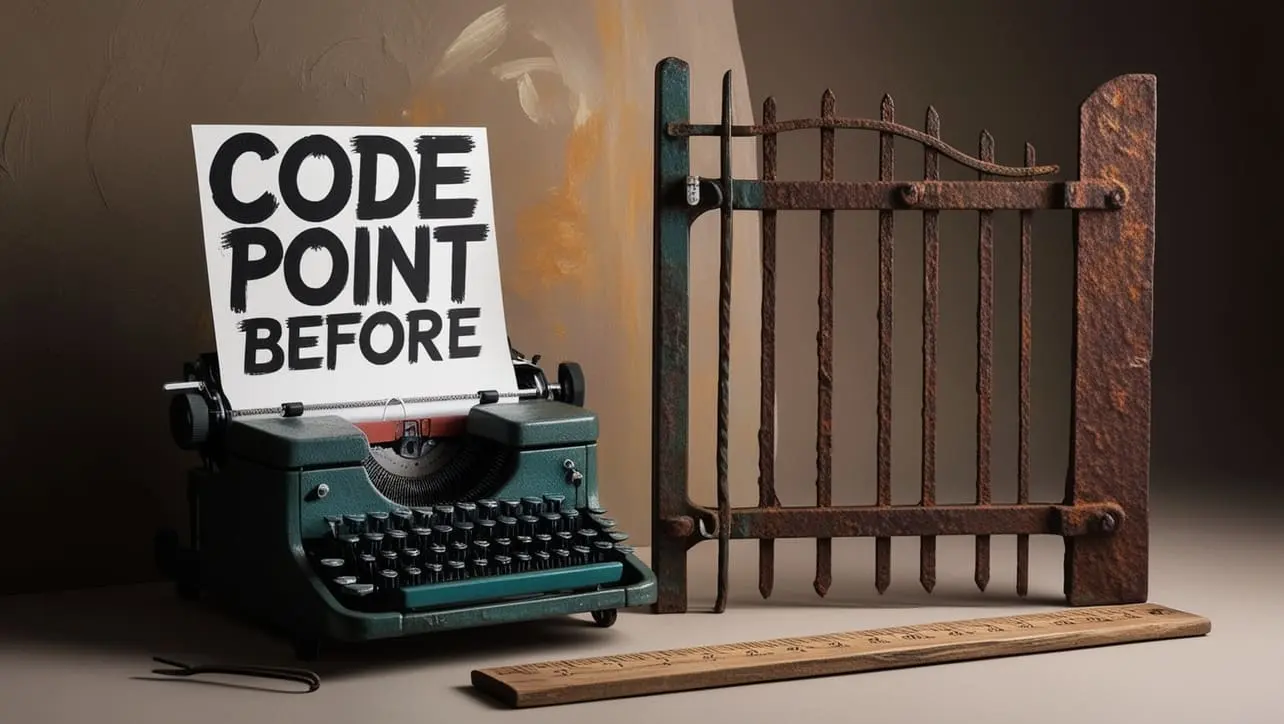
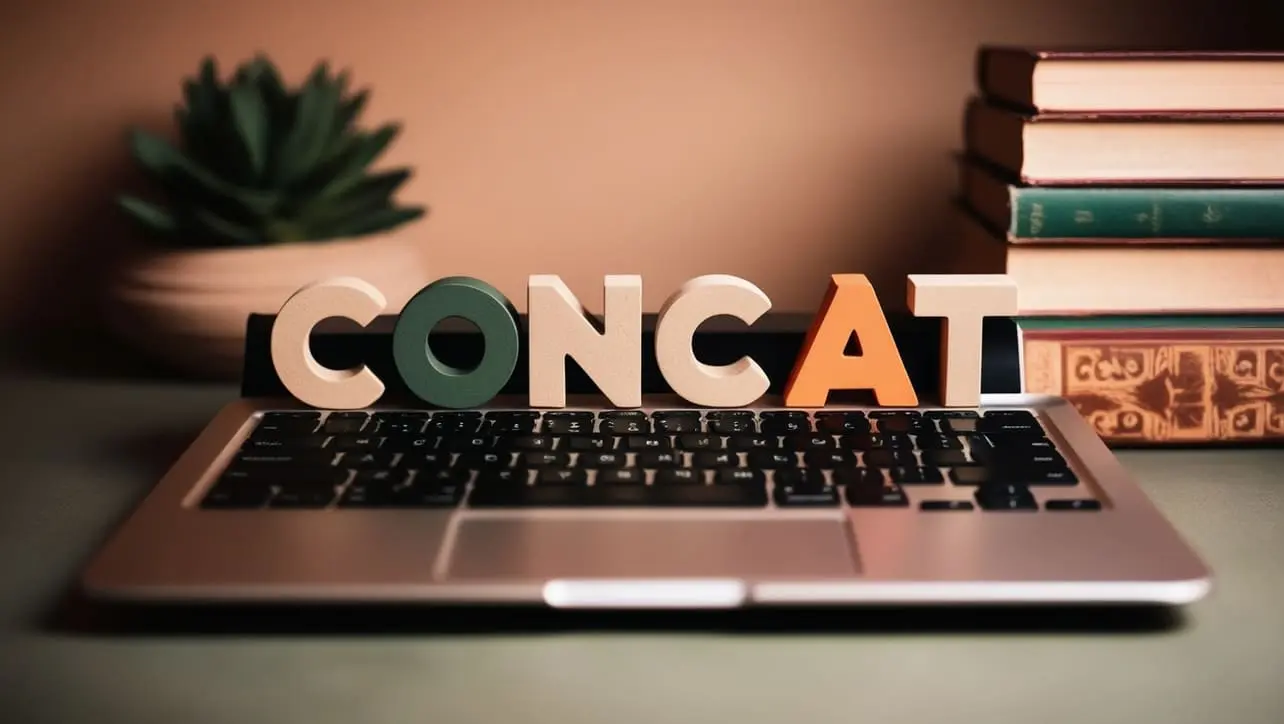
If you have any doubts regarding this article (Java Program to Swap Two Numbers), please comment here. I will help you immediately.