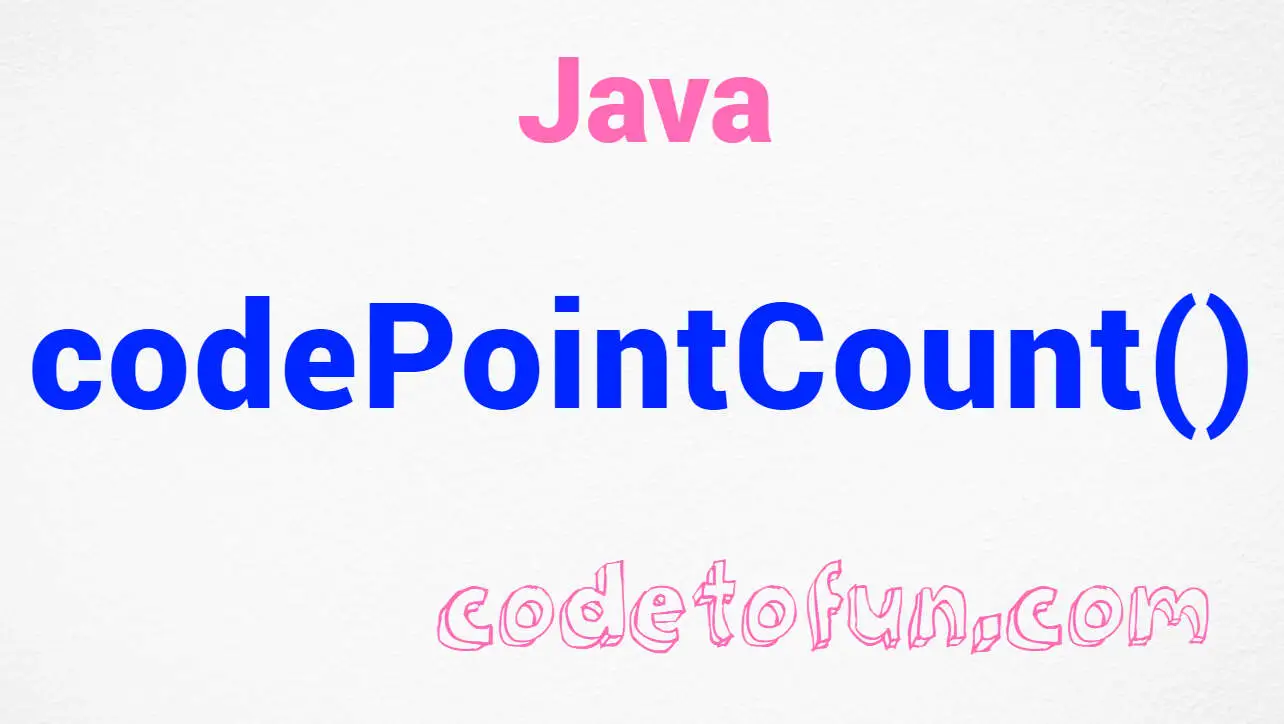
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Check Leap Year
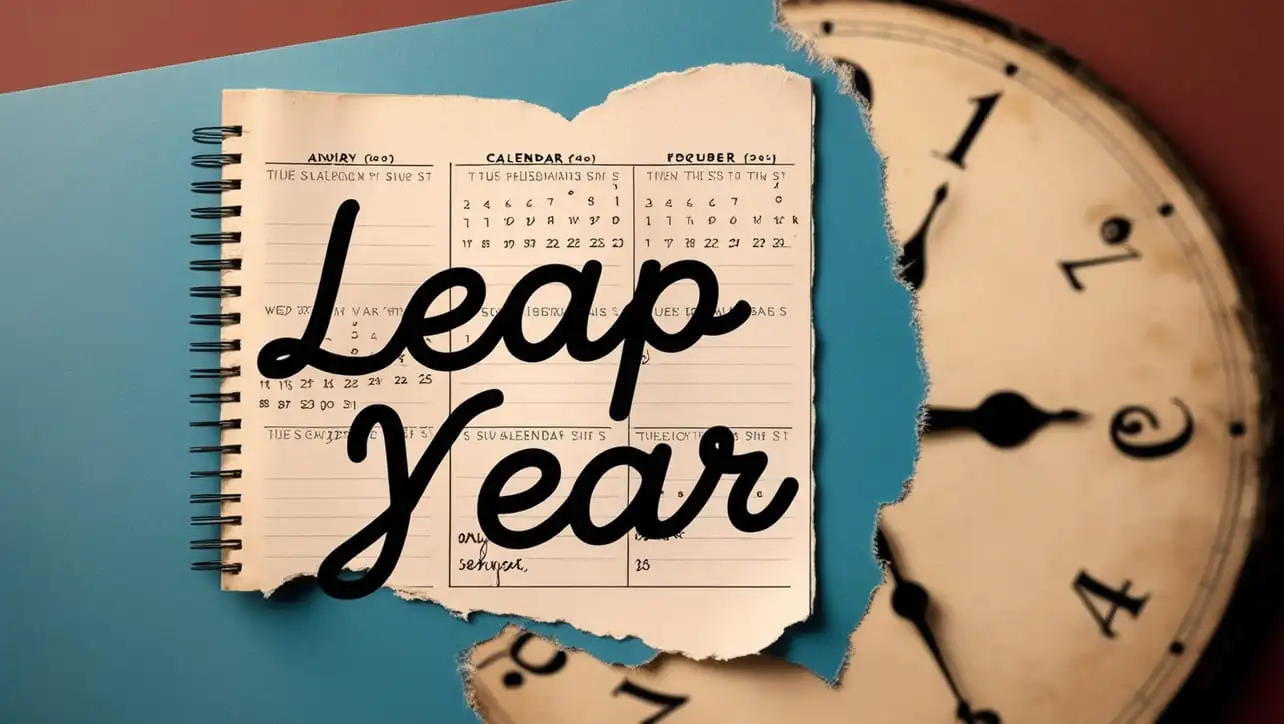
Photo Credit to CodeToFun
π Introduction
In the realm of programming, determining whether a given year is a leap year is a common requirement. A leap year is a year that is evenly divisible by 4, except for end-of-century years, which must be divisible by 400 to be a leap year. Checking for leap years involves implementing specific rules to validate the year.
In this tutorial, we will explore a Java program designed to check whether a given year is a leap year.
π Example
Let's delve into the Java code that accomplishes this task.
import java.util.Scanner;
public class LeapYearChecker {
// Function to check if a year is a leap year
static boolean isLeapYear(int year) {
// Leap year conditions
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// Driver program
public static void main(String[] args) {
// Replace this value with the year you want to check
int year = 2024;
// Call the function to check if the year is a leap year
if (isLeapYear(year)) {
System.out.println(year + " is a leap year.");
} else {
System.out.println(year + " is not a leap year.");
}
}
}
π» Testing the Program
To test the program with different years, modify the value of year in the main method.
2024 is a leap year.
Compile and run the program to check if the specified year is a leap year.
π§ How the Program Works
- The program defines a class LeapYearChecker containing a static method isLeapYear that takes an integer year as input and returns true if the year is a leap year, and false otherwise.
- The method checks leap year conditions: the year must be divisible by 4 and not divisible by 100, or it must be divisible by 400.
- Inside the main method, replace the value of year with the desired year you want to check.
- The program calls the isLeapYear method and prints the result.
π Between the Given Range
Let's dive into the java code that checks for leap years in the specified range.
public class LeapYearChecker {
// Function to check for leap years in the range
static void checkLeapYears(int startYear, int endYear) {
System.out.printf("Leap Years in the range %d to %d:\n", startYear, endYear);
for (int year = startYear; year <= endYear; year++) {
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
System.out.print(year + " ");
}
}
System.out.println();
}
// Driver program
public static void main(String[] args) {
// Define the range
int startYear = 2024;
int endYear = 2050;
// Call the function to check leap years
checkLeapYears(startYear, endYear);
}
}
π» Testing the Program
The provided code is already set to check for leap years in the range from 2024 to 2050.
Leap years in the range 2024 to 2050: 2024 2028 2032 2036 2040 2044 2048
Compile and run the program to see the leap years in the specified range.
π§ How the Program Works
- The program defines a class LeapYearChecker containing a static method checkLeapYears that takes the start and end years of the range as input and prints the leap years within that range.
- Inside the method, it iterates through each year in the range and checks if it satisfies the leap year conditions.
- If a year meets the conditions, it is printed as a leap year.
- The main method tests the program by providing the range from 2024 to 2050.
π§ Understanding the Concept of Leap Year
Before delving into the code, let's understand the concept of leap years. Leap years are years that are evenly divisible by 4, except for end-of-century years, which must be divisible by 400 to be a leap year.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing alternative approaches or optimizations for checking leap years.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
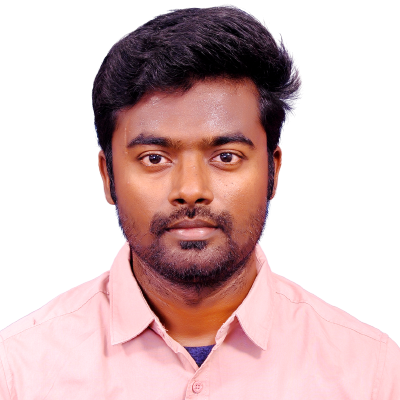
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Check Leap Year), please comment here. I will help you immediately.